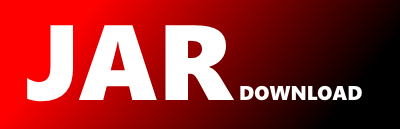
com.amazonaws.services.applicationsignals.AmazonApplicationSignalsAsync Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.applicationsignals;
import javax.annotation.Generated;
import com.amazonaws.services.applicationsignals.model.*;
/**
* Interface for accessing Amazon CloudWatch Application Signals asynchronously. Each asynchronous method will return a
* Java Future object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be
* used to receive notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.applicationsignals.AbstractAmazonApplicationSignalsAsync} instead.
*
*
*
* Use CloudWatch Application Signals for comprehensive observability of your cloud-based applications. It enables
* real-time service health dashboards and helps you track long-term performance trends against your business goals. The
* application-centric view provides you with unified visibility across your applications, services, and dependencies,
* so you can proactively monitor and efficiently triage any issues that may arise, ensuring optimal customer
* experience.
*
*
* Application Signals provides the following benefits:
*
*
* -
*
* Automatically collect metrics and traces from your applications, and display key metrics such as call volume,
* availability, latency, faults, and errors.
*
*
* -
*
* Create and monitor service level objectives (SLOs).
*
*
* -
*
* See a map of your application topology that Application Signals automatically discovers, that gives you a visual
* representation of your applications, dependencies, and their connectivity.
*
*
*
*
* Application Signals works with CloudWatch RUM, CloudWatch Synthetics canaries, and Amazon Web Services Service
* Catalog AppRegistry, to display your client pages, Synthetics canaries, and application names within dashboards and
* maps.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonApplicationSignalsAsync extends AmazonApplicationSignals {
/**
*
* Use this operation to retrieve one or more service level objective (SLO) budget reports.
*
*
* An error budget is the amount of time in unhealthy periods that your service can accumulate during an
* interval before your overall SLO budget health is breached and the SLO is considered to be unmet. For example, an
* SLO with a threshold of 99.95% and a monthly interval translates to an error budget of 21.9 minutes of downtime
* in a 30-day month.
*
*
* Budget reports include a health indicator, the attainment value, and remaining budget.
*
*
* For more information about SLO error budgets, see SLO concepts.
*
*
* @param batchGetServiceLevelObjectiveBudgetReportRequest
* @return A Java Future containing the result of the BatchGetServiceLevelObjectiveBudgetReport operation returned
* by the service.
* @sample AmazonApplicationSignalsAsync.BatchGetServiceLevelObjectiveBudgetReport
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetServiceLevelObjectiveBudgetReportAsync(
BatchGetServiceLevelObjectiveBudgetReportRequest batchGetServiceLevelObjectiveBudgetReportRequest);
/**
*
* Use this operation to retrieve one or more service level objective (SLO) budget reports.
*
*
* An error budget is the amount of time in unhealthy periods that your service can accumulate during an
* interval before your overall SLO budget health is breached and the SLO is considered to be unmet. For example, an
* SLO with a threshold of 99.95% and a monthly interval translates to an error budget of 21.9 minutes of downtime
* in a 30-day month.
*
*
* Budget reports include a health indicator, the attainment value, and remaining budget.
*
*
* For more information about SLO error budgets, see SLO concepts.
*
*
* @param batchGetServiceLevelObjectiveBudgetReportRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchGetServiceLevelObjectiveBudgetReport operation returned
* by the service.
* @sample AmazonApplicationSignalsAsyncHandler.BatchGetServiceLevelObjectiveBudgetReport
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetServiceLevelObjectiveBudgetReportAsync(
BatchGetServiceLevelObjectiveBudgetReportRequest batchGetServiceLevelObjectiveBudgetReportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a service level objective (SLO), which can help you ensure that your critical business operations are
* meeting customer expectations. Use SLOs to set and track specific target levels for the reliability and
* availability of your applications and services. SLOs use service level indicators (SLIs) to calculate whether the
* application is performing at the level that you want.
*
*
* Create an SLO to set a target for a service or operation’s availability or latency. CloudWatch measures this
* target frequently you can find whether it has been breached.
*
*
* When you create an SLO, you set an attainment goal for it. An attainment goal is the ratio of good
* periods that meet the threshold requirements to the total periods within the interval. For example, an attainment
* goal of 99.9% means that within your interval, you are targeting 99.9% of the periods to be in healthy state.
*
*
* After you have created an SLO, you can retrieve error budget reports for it. An error budget is the number
* of periods or amount of time that your service can accumulate during an interval before your overall SLO budget
* health is breached and the SLO is considered to be unmet. for example, an SLO with a threshold that 99.95% of
* requests must be completed under 2000ms every month translates to an error budget of 21.9 minutes of downtime per
* month.
*
*
* When you call this operation, Application Signals creates the
* AWSServiceRoleForCloudWatchApplicationSignals service-linked role, if it doesn't already exist in your
* account. This service- linked role has the following permissions:
*
*
* -
*
* xray:GetServiceGraph
*
*
* -
*
* logs:StartQuery
*
*
* -
*
* logs:GetQueryResults
*
*
* -
*
* cloudwatch:GetMetricData
*
*
* -
*
* cloudwatch:ListMetrics
*
*
* -
*
* tag:GetResources
*
*
* -
*
* autoscaling:DescribeAutoScalingGroups
*
*
*
*
* You can easily set SLO targets for your applications that are discovered by Application Signals, using critical
* metrics such as latency and availability. You can also set SLOs against any CloudWatch metric or math expression
* that produces a time series.
*
*
* For more information about SLOs, see
* Service level objectives (SLOs).
*
*
* @param createServiceLevelObjectiveRequest
* @return A Java Future containing the result of the CreateServiceLevelObjective operation returned by the service.
* @sample AmazonApplicationSignalsAsync.CreateServiceLevelObjective
* @see AWS API Documentation
*/
java.util.concurrent.Future createServiceLevelObjectiveAsync(
CreateServiceLevelObjectiveRequest createServiceLevelObjectiveRequest);
/**
*
* Creates a service level objective (SLO), which can help you ensure that your critical business operations are
* meeting customer expectations. Use SLOs to set and track specific target levels for the reliability and
* availability of your applications and services. SLOs use service level indicators (SLIs) to calculate whether the
* application is performing at the level that you want.
*
*
* Create an SLO to set a target for a service or operation’s availability or latency. CloudWatch measures this
* target frequently you can find whether it has been breached.
*
*
* When you create an SLO, you set an attainment goal for it. An attainment goal is the ratio of good
* periods that meet the threshold requirements to the total periods within the interval. For example, an attainment
* goal of 99.9% means that within your interval, you are targeting 99.9% of the periods to be in healthy state.
*
*
* After you have created an SLO, you can retrieve error budget reports for it. An error budget is the number
* of periods or amount of time that your service can accumulate during an interval before your overall SLO budget
* health is breached and the SLO is considered to be unmet. for example, an SLO with a threshold that 99.95% of
* requests must be completed under 2000ms every month translates to an error budget of 21.9 minutes of downtime per
* month.
*
*
* When you call this operation, Application Signals creates the
* AWSServiceRoleForCloudWatchApplicationSignals service-linked role, if it doesn't already exist in your
* account. This service- linked role has the following permissions:
*
*
* -
*
* xray:GetServiceGraph
*
*
* -
*
* logs:StartQuery
*
*
* -
*
* logs:GetQueryResults
*
*
* -
*
* cloudwatch:GetMetricData
*
*
* -
*
* cloudwatch:ListMetrics
*
*
* -
*
* tag:GetResources
*
*
* -
*
* autoscaling:DescribeAutoScalingGroups
*
*
*
*
* You can easily set SLO targets for your applications that are discovered by Application Signals, using critical
* metrics such as latency and availability. You can also set SLOs against any CloudWatch metric or math expression
* that produces a time series.
*
*
* For more information about SLOs, see
* Service level objectives (SLOs).
*
*
* @param createServiceLevelObjectiveRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateServiceLevelObjective operation returned by the service.
* @sample AmazonApplicationSignalsAsyncHandler.CreateServiceLevelObjective
* @see AWS API Documentation
*/
java.util.concurrent.Future createServiceLevelObjectiveAsync(
CreateServiceLevelObjectiveRequest createServiceLevelObjectiveRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified service level objective.
*
*
* @param deleteServiceLevelObjectiveRequest
* @return A Java Future containing the result of the DeleteServiceLevelObjective operation returned by the service.
* @sample AmazonApplicationSignalsAsync.DeleteServiceLevelObjective
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteServiceLevelObjectiveAsync(
DeleteServiceLevelObjectiveRequest deleteServiceLevelObjectiveRequest);
/**
*
* Deletes the specified service level objective.
*
*
* @param deleteServiceLevelObjectiveRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteServiceLevelObjective operation returned by the service.
* @sample AmazonApplicationSignalsAsyncHandler.DeleteServiceLevelObjective
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteServiceLevelObjectiveAsync(
DeleteServiceLevelObjectiveRequest deleteServiceLevelObjectiveRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a service discovered by Application Signals.
*
*
* @param getServiceRequest
* @return A Java Future containing the result of the GetService operation returned by the service.
* @sample AmazonApplicationSignalsAsync.GetService
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getServiceAsync(GetServiceRequest getServiceRequest);
/**
*
* Returns information about a service discovered by Application Signals.
*
*
* @param getServiceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetService operation returned by the service.
* @sample AmazonApplicationSignalsAsyncHandler.GetService
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getServiceAsync(GetServiceRequest getServiceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about one SLO created in the account.
*
*
* @param getServiceLevelObjectiveRequest
* @return A Java Future containing the result of the GetServiceLevelObjective operation returned by the service.
* @sample AmazonApplicationSignalsAsync.GetServiceLevelObjective
* @see AWS API Documentation
*/
java.util.concurrent.Future getServiceLevelObjectiveAsync(GetServiceLevelObjectiveRequest getServiceLevelObjectiveRequest);
/**
*
* Returns information about one SLO created in the account.
*
*
* @param getServiceLevelObjectiveRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetServiceLevelObjective operation returned by the service.
* @sample AmazonApplicationSignalsAsyncHandler.GetServiceLevelObjective
* @see AWS API Documentation
*/
java.util.concurrent.Future getServiceLevelObjectiveAsync(GetServiceLevelObjectiveRequest getServiceLevelObjectiveRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of service dependencies of the service that you specify. A dependency is an infrastructure
* component that an operation of this service connects with. Dependencies can include Amazon Web Services services,
* Amazon Web Services resources, and third-party services.
*
*
* @param listServiceDependenciesRequest
* @return A Java Future containing the result of the ListServiceDependencies operation returned by the service.
* @sample AmazonApplicationSignalsAsync.ListServiceDependencies
* @see AWS API Documentation
*/
java.util.concurrent.Future listServiceDependenciesAsync(ListServiceDependenciesRequest listServiceDependenciesRequest);
/**
*
* Returns a list of service dependencies of the service that you specify. A dependency is an infrastructure
* component that an operation of this service connects with. Dependencies can include Amazon Web Services services,
* Amazon Web Services resources, and third-party services.
*
*
* @param listServiceDependenciesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListServiceDependencies operation returned by the service.
* @sample AmazonApplicationSignalsAsyncHandler.ListServiceDependencies
* @see AWS API Documentation
*/
java.util.concurrent.Future listServiceDependenciesAsync(ListServiceDependenciesRequest listServiceDependenciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the list of dependents that invoked the specified service during the provided time range. Dependents
* include other services, CloudWatch Synthetics canaries, and clients that are instrumented with CloudWatch RUM app
* monitors.
*
*
* @param listServiceDependentsRequest
* @return A Java Future containing the result of the ListServiceDependents operation returned by the service.
* @sample AmazonApplicationSignalsAsync.ListServiceDependents
* @see AWS API Documentation
*/
java.util.concurrent.Future listServiceDependentsAsync(ListServiceDependentsRequest listServiceDependentsRequest);
/**
*
* Returns the list of dependents that invoked the specified service during the provided time range. Dependents
* include other services, CloudWatch Synthetics canaries, and clients that are instrumented with CloudWatch RUM app
* monitors.
*
*
* @param listServiceDependentsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListServiceDependents operation returned by the service.
* @sample AmazonApplicationSignalsAsyncHandler.ListServiceDependents
* @see AWS API Documentation
*/
java.util.concurrent.Future listServiceDependentsAsync(ListServiceDependentsRequest listServiceDependentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of SLOs created in this account.
*
*
* @param listServiceLevelObjectivesRequest
* @return A Java Future containing the result of the ListServiceLevelObjectives operation returned by the service.
* @sample AmazonApplicationSignalsAsync.ListServiceLevelObjectives
* @see AWS API Documentation
*/
java.util.concurrent.Future listServiceLevelObjectivesAsync(
ListServiceLevelObjectivesRequest listServiceLevelObjectivesRequest);
/**
*
* Returns a list of SLOs created in this account.
*
*
* @param listServiceLevelObjectivesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListServiceLevelObjectives operation returned by the service.
* @sample AmazonApplicationSignalsAsyncHandler.ListServiceLevelObjectives
* @see AWS API Documentation
*/
java.util.concurrent.Future listServiceLevelObjectivesAsync(
ListServiceLevelObjectivesRequest listServiceLevelObjectivesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of the operations of this service that have been discovered by Application Signals. Only
* the operations that were invoked during the specified time range are returned.
*
*
* @param listServiceOperationsRequest
* @return A Java Future containing the result of the ListServiceOperations operation returned by the service.
* @sample AmazonApplicationSignalsAsync.ListServiceOperations
* @see AWS API Documentation
*/
java.util.concurrent.Future listServiceOperationsAsync(ListServiceOperationsRequest listServiceOperationsRequest);
/**
*
* Returns a list of the operations of this service that have been discovered by Application Signals. Only
* the operations that were invoked during the specified time range are returned.
*
*
* @param listServiceOperationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListServiceOperations operation returned by the service.
* @sample AmazonApplicationSignalsAsyncHandler.ListServiceOperations
* @see AWS API Documentation
*/
java.util.concurrent.Future listServiceOperationsAsync(ListServiceOperationsRequest listServiceOperationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of services that have been discovered by Application Signals. A service represents a minimum
* logical and transactional unit that completes a business function. Services are discovered through Application
* Signals instrumentation.
*
*
* @param listServicesRequest
* @return A Java Future containing the result of the ListServices operation returned by the service.
* @sample AmazonApplicationSignalsAsync.ListServices
* @see AWS API Documentation
*/
java.util.concurrent.Future listServicesAsync(ListServicesRequest listServicesRequest);
/**
*
* Returns a list of services that have been discovered by Application Signals. A service represents a minimum
* logical and transactional unit that completes a business function. Services are discovered through Application
* Signals instrumentation.
*
*
* @param listServicesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListServices operation returned by the service.
* @sample AmazonApplicationSignalsAsyncHandler.ListServices
* @see AWS API Documentation
*/
java.util.concurrent.Future listServicesAsync(ListServicesRequest listServicesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Displays the tags associated with a CloudWatch resource. Tags can be assigned to service level objectives.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonApplicationSignalsAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Displays the tags associated with a CloudWatch resource. Tags can be assigned to service level objectives.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonApplicationSignalsAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables this Amazon Web Services account to be able to use CloudWatch Application Signals by creating the
* AWSServiceRoleForCloudWatchApplicationSignals service-linked role. This service- linked role has the
* following permissions:
*
*
* -
*
* xray:GetServiceGraph
*
*
* -
*
* logs:StartQuery
*
*
* -
*
* logs:GetQueryResults
*
*
* -
*
* cloudwatch:GetMetricData
*
*
* -
*
* cloudwatch:ListMetrics
*
*
* -
*
* tag:GetResources
*
*
* -
*
* autoscaling:DescribeAutoScalingGroups
*
*
*
*
* After completing this step, you still need to instrument your Java and Python applications to send data to
* Application Signals. For more information, see
* Enabling Application Signals.
*
*
* @param startDiscoveryRequest
* @return A Java Future containing the result of the StartDiscovery operation returned by the service.
* @sample AmazonApplicationSignalsAsync.StartDiscovery
* @see AWS API Documentation
*/
java.util.concurrent.Future startDiscoveryAsync(StartDiscoveryRequest startDiscoveryRequest);
/**
*
* Enables this Amazon Web Services account to be able to use CloudWatch Application Signals by creating the
* AWSServiceRoleForCloudWatchApplicationSignals service-linked role. This service- linked role has the
* following permissions:
*
*
* -
*
* xray:GetServiceGraph
*
*
* -
*
* logs:StartQuery
*
*
* -
*
* logs:GetQueryResults
*
*
* -
*
* cloudwatch:GetMetricData
*
*
* -
*
* cloudwatch:ListMetrics
*
*
* -
*
* tag:GetResources
*
*
* -
*
* autoscaling:DescribeAutoScalingGroups
*
*
*
*
* After completing this step, you still need to instrument your Java and Python applications to send data to
* Application Signals. For more information, see
* Enabling Application Signals.
*
*
* @param startDiscoveryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartDiscovery operation returned by the service.
* @sample AmazonApplicationSignalsAsyncHandler.StartDiscovery
* @see AWS API Documentation
*/
java.util.concurrent.Future startDiscoveryAsync(StartDiscoveryRequest startDiscoveryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns one or more tags (key-value pairs) to the specified CloudWatch resource, such as a service level
* objective.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by
* granting a user permission to access or change only resources with certain tag values.
*
*
* Tags don't have any semantic meaning to Amazon Web Services and are interpreted strictly as strings of
* characters.
*
*
* You can use the TagResource
action with an alarm that already has tags. If you specify a new tag key
* for the alarm, this tag is appended to the list of tags associated with the alarm. If you specify a tag key that
* is already associated with the alarm, the new tag value that you specify replaces the previous value for that
* tag.
*
*
* You can associate as many as 50 tags with a CloudWatch resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonApplicationSignalsAsync.TagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Assigns one or more tags (key-value pairs) to the specified CloudWatch resource, such as a service level
* objective.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by
* granting a user permission to access or change only resources with certain tag values.
*
*
* Tags don't have any semantic meaning to Amazon Web Services and are interpreted strictly as strings of
* characters.
*
*
* You can use the TagResource
action with an alarm that already has tags. If you specify a new tag key
* for the alarm, this tag is appended to the list of tags associated with the alarm. If you specify a tag key that
* is already associated with the alarm, the new tag value that you specify replaces the previous value for that
* tag.
*
*
* You can associate as many as 50 tags with a CloudWatch resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonApplicationSignalsAsyncHandler.TagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes one or more tags from the specified resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonApplicationSignalsAsync.UntagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes one or more tags from the specified resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonApplicationSignalsAsyncHandler.UntagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing service level objective (SLO). If you omit parameters, the previous values of those
* parameters are retained.
*
*
* @param updateServiceLevelObjectiveRequest
* @return A Java Future containing the result of the UpdateServiceLevelObjective operation returned by the service.
* @sample AmazonApplicationSignalsAsync.UpdateServiceLevelObjective
* @see AWS API Documentation
*/
java.util.concurrent.Future updateServiceLevelObjectiveAsync(
UpdateServiceLevelObjectiveRequest updateServiceLevelObjectiveRequest);
/**
*
* Updates an existing service level objective (SLO). If you omit parameters, the previous values of those
* parameters are retained.
*
*
* @param updateServiceLevelObjectiveRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateServiceLevelObjective operation returned by the service.
* @sample AmazonApplicationSignalsAsyncHandler.UpdateServiceLevelObjective
* @see AWS API Documentation
*/
java.util.concurrent.Future updateServiceLevelObjectiveAsync(
UpdateServiceLevelObjectiveRequest updateServiceLevelObjectiveRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}