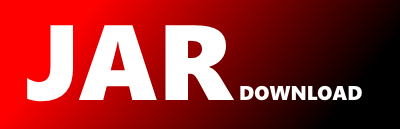
com.amazonaws.services.applicationsignals.model.CreateServiceLevelObjectiveRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-applicationsignals Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.applicationsignals.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateServiceLevelObjectiveRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* A name for this SLO.
*
*/
private String name;
/**
*
* An optional description for this SLO.
*
*/
private String description;
/**
*
* A structure that contains information about what service and what performance metric that this SLO will monitor.
*
*/
private ServiceLevelIndicatorConfig sliConfig;
/**
*
* A structure that contains the attributes that determine the goal of the SLO. This includes the time period for
* evaluation and the attainment threshold.
*
*/
private Goal goal;
/**
*
* A list of key-value pairs to associate with the SLO. You can associate as many as 50 tags with an SLO. To be able
* to associate tags with the SLO when you create the SLO, you must have the cloudwatch:TagResource
* permission.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by
* granting a user permission to access or change only resources with certain tag values.
*
*/
private java.util.List tags;
/**
*
* A name for this SLO.
*
*
* @param name
* A name for this SLO.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* A name for this SLO.
*
*
* @return A name for this SLO.
*/
public String getName() {
return this.name;
}
/**
*
* A name for this SLO.
*
*
* @param name
* A name for this SLO.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServiceLevelObjectiveRequest withName(String name) {
setName(name);
return this;
}
/**
*
* An optional description for this SLO.
*
*
* @param description
* An optional description for this SLO.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* An optional description for this SLO.
*
*
* @return An optional description for this SLO.
*/
public String getDescription() {
return this.description;
}
/**
*
* An optional description for this SLO.
*
*
* @param description
* An optional description for this SLO.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServiceLevelObjectiveRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* A structure that contains information about what service and what performance metric that this SLO will monitor.
*
*
* @param sliConfig
* A structure that contains information about what service and what performance metric that this SLO will
* monitor.
*/
public void setSliConfig(ServiceLevelIndicatorConfig sliConfig) {
this.sliConfig = sliConfig;
}
/**
*
* A structure that contains information about what service and what performance metric that this SLO will monitor.
*
*
* @return A structure that contains information about what service and what performance metric that this SLO will
* monitor.
*/
public ServiceLevelIndicatorConfig getSliConfig() {
return this.sliConfig;
}
/**
*
* A structure that contains information about what service and what performance metric that this SLO will monitor.
*
*
* @param sliConfig
* A structure that contains information about what service and what performance metric that this SLO will
* monitor.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServiceLevelObjectiveRequest withSliConfig(ServiceLevelIndicatorConfig sliConfig) {
setSliConfig(sliConfig);
return this;
}
/**
*
* A structure that contains the attributes that determine the goal of the SLO. This includes the time period for
* evaluation and the attainment threshold.
*
*
* @param goal
* A structure that contains the attributes that determine the goal of the SLO. This includes the time period
* for evaluation and the attainment threshold.
*/
public void setGoal(Goal goal) {
this.goal = goal;
}
/**
*
* A structure that contains the attributes that determine the goal of the SLO. This includes the time period for
* evaluation and the attainment threshold.
*
*
* @return A structure that contains the attributes that determine the goal of the SLO. This includes the time
* period for evaluation and the attainment threshold.
*/
public Goal getGoal() {
return this.goal;
}
/**
*
* A structure that contains the attributes that determine the goal of the SLO. This includes the time period for
* evaluation and the attainment threshold.
*
*
* @param goal
* A structure that contains the attributes that determine the goal of the SLO. This includes the time period
* for evaluation and the attainment threshold.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServiceLevelObjectiveRequest withGoal(Goal goal) {
setGoal(goal);
return this;
}
/**
*
* A list of key-value pairs to associate with the SLO. You can associate as many as 50 tags with an SLO. To be able
* to associate tags with the SLO when you create the SLO, you must have the cloudwatch:TagResource
* permission.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by
* granting a user permission to access or change only resources with certain tag values.
*
*
* @return A list of key-value pairs to associate with the SLO. You can associate as many as 50 tags with an SLO. To
* be able to associate tags with the SLO when you create the SLO, you must have the
* cloudwatch:TagResource
permission.
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions
* by granting a user permission to access or change only resources with certain tag values.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* A list of key-value pairs to associate with the SLO. You can associate as many as 50 tags with an SLO. To be able
* to associate tags with the SLO when you create the SLO, you must have the cloudwatch:TagResource
* permission.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by
* granting a user permission to access or change only resources with certain tag values.
*
*
* @param tags
* A list of key-value pairs to associate with the SLO. You can associate as many as 50 tags with an SLO. To
* be able to associate tags with the SLO when you create the SLO, you must have the
* cloudwatch:TagResource
permission.
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions
* by granting a user permission to access or change only resources with certain tag values.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* A list of key-value pairs to associate with the SLO. You can associate as many as 50 tags with an SLO. To be able
* to associate tags with the SLO when you create the SLO, you must have the cloudwatch:TagResource
* permission.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by
* granting a user permission to access or change only resources with certain tag values.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* A list of key-value pairs to associate with the SLO. You can associate as many as 50 tags with an SLO. To
* be able to associate tags with the SLO when you create the SLO, you must have the
* cloudwatch:TagResource
permission.
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions
* by granting a user permission to access or change only resources with certain tag values.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServiceLevelObjectiveRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* A list of key-value pairs to associate with the SLO. You can associate as many as 50 tags with an SLO. To be able
* to associate tags with the SLO when you create the SLO, you must have the cloudwatch:TagResource
* permission.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions by
* granting a user permission to access or change only resources with certain tag values.
*
*
* @param tags
* A list of key-value pairs to associate with the SLO. You can associate as many as 50 tags with an SLO. To
* be able to associate tags with the SLO when you create the SLO, you must have the
* cloudwatch:TagResource
permission.
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions
* by granting a user permission to access or change only resources with certain tag values.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateServiceLevelObjectiveRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getSliConfig() != null)
sb.append("SliConfig: ").append(getSliConfig()).append(",");
if (getGoal() != null)
sb.append("Goal: ").append(getGoal()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateServiceLevelObjectiveRequest == false)
return false;
CreateServiceLevelObjectiveRequest other = (CreateServiceLevelObjectiveRequest) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getSliConfig() == null ^ this.getSliConfig() == null)
return false;
if (other.getSliConfig() != null && other.getSliConfig().equals(this.getSliConfig()) == false)
return false;
if (other.getGoal() == null ^ this.getGoal() == null)
return false;
if (other.getGoal() != null && other.getGoal().equals(this.getGoal()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getSliConfig() == null) ? 0 : getSliConfig().hashCode());
hashCode = prime * hashCode + ((getGoal() == null) ? 0 : getGoal().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CreateServiceLevelObjectiveRequest clone() {
return (CreateServiceLevelObjectiveRequest) super.clone();
}
}