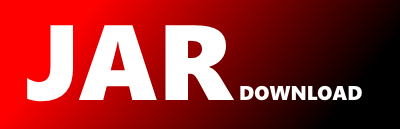
com.amazonaws.services.applicationsignals.model.GetServiceRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-applicationsignals Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.applicationsignals.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetServiceRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The start of the time period to retrieve information about. When used in a raw HTTP Query API, it is formatted as
* be epoch time in seconds. For example: 1698778057
*
*
* Your requested start time will be rounded to the nearest hour.
*
*/
private java.util.Date startTime;
/**
*
* The end of the time period to retrieve information about. When used in a raw HTTP Query API, it is formatted as
* be epoch time in seconds. For example: 1698778057
*
*
* Your requested start time will be rounded to the nearest hour.
*
*/
private java.util.Date endTime;
/**
*
* Use this field to specify which service you want to retrieve information for. You must specify at least the
* Type
, Name
, and Environment
attributes.
*
*
* This is a string-to-string map. It can include the following fields.
*
*
* -
*
* Type
designates the type of object this is.
*
*
* -
*
* ResourceType
specifies the type of the resource. This field is used only when the value of the
* Type
field is Resource
or AWS::Resource
.
*
*
* -
*
* Name
specifies the name of the object. This is used only if the value of the Type
field
* is Service
, RemoteService
, or AWS::Service
.
*
*
* -
*
* Identifier
identifies the resource objects of this resource. This is used only if the value of the
* Type
field is Resource
or AWS::Resource
.
*
*
* -
*
* Environment
specifies the location where this object is hosted, or what it belongs to.
*
*
*
*/
private java.util.Map keyAttributes;
/**
*
* The start of the time period to retrieve information about. When used in a raw HTTP Query API, it is formatted as
* be epoch time in seconds. For example: 1698778057
*
*
* Your requested start time will be rounded to the nearest hour.
*
*
* @param startTime
* The start of the time period to retrieve information about. When used in a raw HTTP Query API, it is
* formatted as be epoch time in seconds. For example: 1698778057
*
* Your requested start time will be rounded to the nearest hour.
*/
public void setStartTime(java.util.Date startTime) {
this.startTime = startTime;
}
/**
*
* The start of the time period to retrieve information about. When used in a raw HTTP Query API, it is formatted as
* be epoch time in seconds. For example: 1698778057
*
*
* Your requested start time will be rounded to the nearest hour.
*
*
* @return The start of the time period to retrieve information about. When used in a raw HTTP Query API, it is
* formatted as be epoch time in seconds. For example: 1698778057
*
* Your requested start time will be rounded to the nearest hour.
*/
public java.util.Date getStartTime() {
return this.startTime;
}
/**
*
* The start of the time period to retrieve information about. When used in a raw HTTP Query API, it is formatted as
* be epoch time in seconds. For example: 1698778057
*
*
* Your requested start time will be rounded to the nearest hour.
*
*
* @param startTime
* The start of the time period to retrieve information about. When used in a raw HTTP Query API, it is
* formatted as be epoch time in seconds. For example: 1698778057
*
* Your requested start time will be rounded to the nearest hour.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetServiceRequest withStartTime(java.util.Date startTime) {
setStartTime(startTime);
return this;
}
/**
*
* The end of the time period to retrieve information about. When used in a raw HTTP Query API, it is formatted as
* be epoch time in seconds. For example: 1698778057
*
*
* Your requested start time will be rounded to the nearest hour.
*
*
* @param endTime
* The end of the time period to retrieve information about. When used in a raw HTTP Query API, it is
* formatted as be epoch time in seconds. For example: 1698778057
*
* Your requested start time will be rounded to the nearest hour.
*/
public void setEndTime(java.util.Date endTime) {
this.endTime = endTime;
}
/**
*
* The end of the time period to retrieve information about. When used in a raw HTTP Query API, it is formatted as
* be epoch time in seconds. For example: 1698778057
*
*
* Your requested start time will be rounded to the nearest hour.
*
*
* @return The end of the time period to retrieve information about. When used in a raw HTTP Query API, it is
* formatted as be epoch time in seconds. For example: 1698778057
*
* Your requested start time will be rounded to the nearest hour.
*/
public java.util.Date getEndTime() {
return this.endTime;
}
/**
*
* The end of the time period to retrieve information about. When used in a raw HTTP Query API, it is formatted as
* be epoch time in seconds. For example: 1698778057
*
*
* Your requested start time will be rounded to the nearest hour.
*
*
* @param endTime
* The end of the time period to retrieve information about. When used in a raw HTTP Query API, it is
* formatted as be epoch time in seconds. For example: 1698778057
*
* Your requested start time will be rounded to the nearest hour.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetServiceRequest withEndTime(java.util.Date endTime) {
setEndTime(endTime);
return this;
}
/**
*
* Use this field to specify which service you want to retrieve information for. You must specify at least the
* Type
, Name
, and Environment
attributes.
*
*
* This is a string-to-string map. It can include the following fields.
*
*
* -
*
* Type
designates the type of object this is.
*
*
* -
*
* ResourceType
specifies the type of the resource. This field is used only when the value of the
* Type
field is Resource
or AWS::Resource
.
*
*
* -
*
* Name
specifies the name of the object. This is used only if the value of the Type
field
* is Service
, RemoteService
, or AWS::Service
.
*
*
* -
*
* Identifier
identifies the resource objects of this resource. This is used only if the value of the
* Type
field is Resource
or AWS::Resource
.
*
*
* -
*
* Environment
specifies the location where this object is hosted, or what it belongs to.
*
*
*
*
* @return Use this field to specify which service you want to retrieve information for. You must specify at least
* the Type
, Name
, and Environment
attributes.
*
* This is a string-to-string map. It can include the following fields.
*
*
* -
*
* Type
designates the type of object this is.
*
*
* -
*
* ResourceType
specifies the type of the resource. This field is used only when the value of
* the Type
field is Resource
or AWS::Resource
.
*
*
* -
*
* Name
specifies the name of the object. This is used only if the value of the
* Type
field is Service
, RemoteService
, or AWS::Service
* .
*
*
* -
*
* Identifier
identifies the resource objects of this resource. This is used only if the value
* of the Type
field is Resource
or AWS::Resource
.
*
*
* -
*
* Environment
specifies the location where this object is hosted, or what it belongs to.
*
*
*/
public java.util.Map getKeyAttributes() {
return keyAttributes;
}
/**
*
* Use this field to specify which service you want to retrieve information for. You must specify at least the
* Type
, Name
, and Environment
attributes.
*
*
* This is a string-to-string map. It can include the following fields.
*
*
* -
*
* Type
designates the type of object this is.
*
*
* -
*
* ResourceType
specifies the type of the resource. This field is used only when the value of the
* Type
field is Resource
or AWS::Resource
.
*
*
* -
*
* Name
specifies the name of the object. This is used only if the value of the Type
field
* is Service
, RemoteService
, or AWS::Service
.
*
*
* -
*
* Identifier
identifies the resource objects of this resource. This is used only if the value of the
* Type
field is Resource
or AWS::Resource
.
*
*
* -
*
* Environment
specifies the location where this object is hosted, or what it belongs to.
*
*
*
*
* @param keyAttributes
* Use this field to specify which service you want to retrieve information for. You must specify at least
* the Type
, Name
, and Environment
attributes.
*
* This is a string-to-string map. It can include the following fields.
*
*
* -
*
* Type
designates the type of object this is.
*
*
* -
*
* ResourceType
specifies the type of the resource. This field is used only when the value of
* the Type
field is Resource
or AWS::Resource
.
*
*
* -
*
* Name
specifies the name of the object. This is used only if the value of the
* Type
field is Service
, RemoteService
, or AWS::Service
.
*
*
* -
*
* Identifier
identifies the resource objects of this resource. This is used only if the value
* of the Type
field is Resource
or AWS::Resource
.
*
*
* -
*
* Environment
specifies the location where this object is hosted, or what it belongs to.
*
*
*/
public void setKeyAttributes(java.util.Map keyAttributes) {
this.keyAttributes = keyAttributes;
}
/**
*
* Use this field to specify which service you want to retrieve information for. You must specify at least the
* Type
, Name
, and Environment
attributes.
*
*
* This is a string-to-string map. It can include the following fields.
*
*
* -
*
* Type
designates the type of object this is.
*
*
* -
*
* ResourceType
specifies the type of the resource. This field is used only when the value of the
* Type
field is Resource
or AWS::Resource
.
*
*
* -
*
* Name
specifies the name of the object. This is used only if the value of the Type
field
* is Service
, RemoteService
, or AWS::Service
.
*
*
* -
*
* Identifier
identifies the resource objects of this resource. This is used only if the value of the
* Type
field is Resource
or AWS::Resource
.
*
*
* -
*
* Environment
specifies the location where this object is hosted, or what it belongs to.
*
*
*
*
* @param keyAttributes
* Use this field to specify which service you want to retrieve information for. You must specify at least
* the Type
, Name
, and Environment
attributes.
*
* This is a string-to-string map. It can include the following fields.
*
*
* -
*
* Type
designates the type of object this is.
*
*
* -
*
* ResourceType
specifies the type of the resource. This field is used only when the value of
* the Type
field is Resource
or AWS::Resource
.
*
*
* -
*
* Name
specifies the name of the object. This is used only if the value of the
* Type
field is Service
, RemoteService
, or AWS::Service
.
*
*
* -
*
* Identifier
identifies the resource objects of this resource. This is used only if the value
* of the Type
field is Resource
or AWS::Resource
.
*
*
* -
*
* Environment
specifies the location where this object is hosted, or what it belongs to.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetServiceRequest withKeyAttributes(java.util.Map keyAttributes) {
setKeyAttributes(keyAttributes);
return this;
}
/**
* Add a single KeyAttributes entry
*
* @see GetServiceRequest#withKeyAttributes
* @returns a reference to this object so that method calls can be chained together.
*/
public GetServiceRequest addKeyAttributesEntry(String key, String value) {
if (null == this.keyAttributes) {
this.keyAttributes = new java.util.HashMap();
}
if (this.keyAttributes.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.keyAttributes.put(key, value);
return this;
}
/**
* Removes all the entries added into KeyAttributes.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetServiceRequest clearKeyAttributesEntries() {
this.keyAttributes = null;
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getStartTime() != null)
sb.append("StartTime: ").append(getStartTime()).append(",");
if (getEndTime() != null)
sb.append("EndTime: ").append(getEndTime()).append(",");
if (getKeyAttributes() != null)
sb.append("KeyAttributes: ").append(getKeyAttributes());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetServiceRequest == false)
return false;
GetServiceRequest other = (GetServiceRequest) obj;
if (other.getStartTime() == null ^ this.getStartTime() == null)
return false;
if (other.getStartTime() != null && other.getStartTime().equals(this.getStartTime()) == false)
return false;
if (other.getEndTime() == null ^ this.getEndTime() == null)
return false;
if (other.getEndTime() != null && other.getEndTime().equals(this.getEndTime()) == false)
return false;
if (other.getKeyAttributes() == null ^ this.getKeyAttributes() == null)
return false;
if (other.getKeyAttributes() != null && other.getKeyAttributes().equals(this.getKeyAttributes()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getStartTime() == null) ? 0 : getStartTime().hashCode());
hashCode = prime * hashCode + ((getEndTime() == null) ? 0 : getEndTime().hashCode());
hashCode = prime * hashCode + ((getKeyAttributes() == null) ? 0 : getKeyAttributes().hashCode());
return hashCode;
}
@Override
public GetServiceRequest clone() {
return (GetServiceRequest) super.clone();
}
}