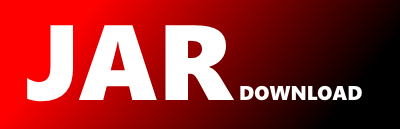
com.amazonaws.services.applicationsignals.model.MetricReference Maven / Gradle / Ivy
Show all versions of aws-java-sdk-applicationsignals Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.applicationsignals.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* This structure contains information about one CloudWatch metric associated with this entity discovered by Application
* Signals.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class MetricReference implements Serializable, Cloneable, StructuredPojo {
/**
*
* The namespace of the metric. For more information, see CloudWatchNamespaces.
*
*/
private String namespace;
/**
*
* Used to display the appropriate statistics in the CloudWatch console.
*
*/
private String metricType;
/**
*
* An array of one or more dimensions that further define the metric. For more information, see CloudWatchDimensions.
*
*/
private java.util.List dimensions;
/**
*
* The name of the metric.
*
*/
private String metricName;
/**
*
* The namespace of the metric. For more information, see CloudWatchNamespaces.
*
*
* @param namespace
* The namespace of the metric. For more information, see CloudWatchNamespaces.
*/
public void setNamespace(String namespace) {
this.namespace = namespace;
}
/**
*
* The namespace of the metric. For more information, see CloudWatchNamespaces.
*
*
* @return The namespace of the metric. For more information, see CloudWatchNamespaces.
*/
public String getNamespace() {
return this.namespace;
}
/**
*
* The namespace of the metric. For more information, see CloudWatchNamespaces.
*
*
* @param namespace
* The namespace of the metric. For more information, see CloudWatchNamespaces.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MetricReference withNamespace(String namespace) {
setNamespace(namespace);
return this;
}
/**
*
* Used to display the appropriate statistics in the CloudWatch console.
*
*
* @param metricType
* Used to display the appropriate statistics in the CloudWatch console.
*/
public void setMetricType(String metricType) {
this.metricType = metricType;
}
/**
*
* Used to display the appropriate statistics in the CloudWatch console.
*
*
* @return Used to display the appropriate statistics in the CloudWatch console.
*/
public String getMetricType() {
return this.metricType;
}
/**
*
* Used to display the appropriate statistics in the CloudWatch console.
*
*
* @param metricType
* Used to display the appropriate statistics in the CloudWatch console.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MetricReference withMetricType(String metricType) {
setMetricType(metricType);
return this;
}
/**
*
* An array of one or more dimensions that further define the metric. For more information, see CloudWatchDimensions.
*
*
* @return An array of one or more dimensions that further define the metric. For more information, see CloudWatchDimensions.
*/
public java.util.List getDimensions() {
return dimensions;
}
/**
*
* An array of one or more dimensions that further define the metric. For more information, see CloudWatchDimensions.
*
*
* @param dimensions
* An array of one or more dimensions that further define the metric. For more information, see CloudWatchDimensions.
*/
public void setDimensions(java.util.Collection dimensions) {
if (dimensions == null) {
this.dimensions = null;
return;
}
this.dimensions = new java.util.ArrayList(dimensions);
}
/**
*
* An array of one or more dimensions that further define the metric. For more information, see CloudWatchDimensions.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDimensions(java.util.Collection)} or {@link #withDimensions(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param dimensions
* An array of one or more dimensions that further define the metric. For more information, see CloudWatchDimensions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MetricReference withDimensions(Dimension... dimensions) {
if (this.dimensions == null) {
setDimensions(new java.util.ArrayList(dimensions.length));
}
for (Dimension ele : dimensions) {
this.dimensions.add(ele);
}
return this;
}
/**
*
* An array of one or more dimensions that further define the metric. For more information, see CloudWatchDimensions.
*
*
* @param dimensions
* An array of one or more dimensions that further define the metric. For more information, see CloudWatchDimensions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MetricReference withDimensions(java.util.Collection dimensions) {
setDimensions(dimensions);
return this;
}
/**
*
* The name of the metric.
*
*
* @param metricName
* The name of the metric.
*/
public void setMetricName(String metricName) {
this.metricName = metricName;
}
/**
*
* The name of the metric.
*
*
* @return The name of the metric.
*/
public String getMetricName() {
return this.metricName;
}
/**
*
* The name of the metric.
*
*
* @param metricName
* The name of the metric.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MetricReference withMetricName(String metricName) {
setMetricName(metricName);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getNamespace() != null)
sb.append("Namespace: ").append(getNamespace()).append(",");
if (getMetricType() != null)
sb.append("MetricType: ").append(getMetricType()).append(",");
if (getDimensions() != null)
sb.append("Dimensions: ").append(getDimensions()).append(",");
if (getMetricName() != null)
sb.append("MetricName: ").append(getMetricName());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof MetricReference == false)
return false;
MetricReference other = (MetricReference) obj;
if (other.getNamespace() == null ^ this.getNamespace() == null)
return false;
if (other.getNamespace() != null && other.getNamespace().equals(this.getNamespace()) == false)
return false;
if (other.getMetricType() == null ^ this.getMetricType() == null)
return false;
if (other.getMetricType() != null && other.getMetricType().equals(this.getMetricType()) == false)
return false;
if (other.getDimensions() == null ^ this.getDimensions() == null)
return false;
if (other.getDimensions() != null && other.getDimensions().equals(this.getDimensions()) == false)
return false;
if (other.getMetricName() == null ^ this.getMetricName() == null)
return false;
if (other.getMetricName() != null && other.getMetricName().equals(this.getMetricName()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getNamespace() == null) ? 0 : getNamespace().hashCode());
hashCode = prime * hashCode + ((getMetricType() == null) ? 0 : getMetricType().hashCode());
hashCode = prime * hashCode + ((getDimensions() == null) ? 0 : getDimensions().hashCode());
hashCode = prime * hashCode + ((getMetricName() == null) ? 0 : getMetricName().hashCode());
return hashCode;
}
@Override
public MetricReference clone() {
try {
return (MetricReference) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.applicationsignals.model.transform.MetricReferenceMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}