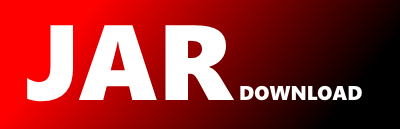
com.amazonaws.services.applicationsignals.model.ServiceLevelObjectiveBudgetReport Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.applicationsignals.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A structure containing an SLO budget report that you have requested.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ServiceLevelObjectiveBudgetReport implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ARN of the SLO that this report is for.
*
*/
private String arn;
/**
*
* The name of the SLO that this report is for.
*
*/
private String name;
/**
*
* The status of this SLO, as it relates to the error budget for the entire time interval.
*
*
* -
*
* OK
means that the SLO had remaining budget above the warning threshold, as of the time that you
* specified in TimeStamp
.
*
*
* -
*
* WARNING
means that the SLO's remaining budget was below the warning threshold, as of the time that
* you specified in TimeStamp
.
*
*
* -
*
* BREACHED
means that the SLO's budget was exhausted, as of the time that you specified in
* TimeStamp
.
*
*
* -
*
* INSUFFICIENT_DATA
means that the specifed start and end times were before the SLO was created, or
* that attainment data is missing.
*
*
*
*/
private String budgetStatus;
/**
*
* A number between 0 and 100 that represents the percentage of time periods that the service has attained the SLO's
* attainment goal, as of the time of the request.
*
*/
private Double attainment;
/**
*
* The total number of seconds in the error budget for the interval.
*
*/
private Integer totalBudgetSeconds;
/**
*
* The budget amount remaining before the SLO status becomes BREACHING
, at the time specified in the
* Timestemp
parameter of the request. If this value is negative, then the SLO is already in
* BREACHING
status.
*
*/
private Integer budgetSecondsRemaining;
/**
*
* A structure that contains information about the performance metric that this SLO monitors.
*
*/
private ServiceLevelIndicator sli;
private Goal goal;
/**
*
* The ARN of the SLO that this report is for.
*
*
* @param arn
* The ARN of the SLO that this report is for.
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
*
* The ARN of the SLO that this report is for.
*
*
* @return The ARN of the SLO that this report is for.
*/
public String getArn() {
return this.arn;
}
/**
*
* The ARN of the SLO that this report is for.
*
*
* @param arn
* The ARN of the SLO that this report is for.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceLevelObjectiveBudgetReport withArn(String arn) {
setArn(arn);
return this;
}
/**
*
* The name of the SLO that this report is for.
*
*
* @param name
* The name of the SLO that this report is for.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the SLO that this report is for.
*
*
* @return The name of the SLO that this report is for.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the SLO that this report is for.
*
*
* @param name
* The name of the SLO that this report is for.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceLevelObjectiveBudgetReport withName(String name) {
setName(name);
return this;
}
/**
*
* The status of this SLO, as it relates to the error budget for the entire time interval.
*
*
* -
*
* OK
means that the SLO had remaining budget above the warning threshold, as of the time that you
* specified in TimeStamp
.
*
*
* -
*
* WARNING
means that the SLO's remaining budget was below the warning threshold, as of the time that
* you specified in TimeStamp
.
*
*
* -
*
* BREACHED
means that the SLO's budget was exhausted, as of the time that you specified in
* TimeStamp
.
*
*
* -
*
* INSUFFICIENT_DATA
means that the specifed start and end times were before the SLO was created, or
* that attainment data is missing.
*
*
*
*
* @param budgetStatus
* The status of this SLO, as it relates to the error budget for the entire time interval.
*
* -
*
* OK
means that the SLO had remaining budget above the warning threshold, as of the time that
* you specified in TimeStamp
.
*
*
* -
*
* WARNING
means that the SLO's remaining budget was below the warning threshold, as of the time
* that you specified in TimeStamp
.
*
*
* -
*
* BREACHED
means that the SLO's budget was exhausted, as of the time that you specified in
* TimeStamp
.
*
*
* -
*
* INSUFFICIENT_DATA
means that the specifed start and end times were before the SLO was
* created, or that attainment data is missing.
*
*
* @see ServiceLevelObjectiveBudgetStatus
*/
public void setBudgetStatus(String budgetStatus) {
this.budgetStatus = budgetStatus;
}
/**
*
* The status of this SLO, as it relates to the error budget for the entire time interval.
*
*
* -
*
* OK
means that the SLO had remaining budget above the warning threshold, as of the time that you
* specified in TimeStamp
.
*
*
* -
*
* WARNING
means that the SLO's remaining budget was below the warning threshold, as of the time that
* you specified in TimeStamp
.
*
*
* -
*
* BREACHED
means that the SLO's budget was exhausted, as of the time that you specified in
* TimeStamp
.
*
*
* -
*
* INSUFFICIENT_DATA
means that the specifed start and end times were before the SLO was created, or
* that attainment data is missing.
*
*
*
*
* @return The status of this SLO, as it relates to the error budget for the entire time interval.
*
* -
*
* OK
means that the SLO had remaining budget above the warning threshold, as of the time that
* you specified in TimeStamp
.
*
*
* -
*
* WARNING
means that the SLO's remaining budget was below the warning threshold, as of the
* time that you specified in TimeStamp
.
*
*
* -
*
* BREACHED
means that the SLO's budget was exhausted, as of the time that you specified in
* TimeStamp
.
*
*
* -
*
* INSUFFICIENT_DATA
means that the specifed start and end times were before the SLO was
* created, or that attainment data is missing.
*
*
* @see ServiceLevelObjectiveBudgetStatus
*/
public String getBudgetStatus() {
return this.budgetStatus;
}
/**
*
* The status of this SLO, as it relates to the error budget for the entire time interval.
*
*
* -
*
* OK
means that the SLO had remaining budget above the warning threshold, as of the time that you
* specified in TimeStamp
.
*
*
* -
*
* WARNING
means that the SLO's remaining budget was below the warning threshold, as of the time that
* you specified in TimeStamp
.
*
*
* -
*
* BREACHED
means that the SLO's budget was exhausted, as of the time that you specified in
* TimeStamp
.
*
*
* -
*
* INSUFFICIENT_DATA
means that the specifed start and end times were before the SLO was created, or
* that attainment data is missing.
*
*
*
*
* @param budgetStatus
* The status of this SLO, as it relates to the error budget for the entire time interval.
*
* -
*
* OK
means that the SLO had remaining budget above the warning threshold, as of the time that
* you specified in TimeStamp
.
*
*
* -
*
* WARNING
means that the SLO's remaining budget was below the warning threshold, as of the time
* that you specified in TimeStamp
.
*
*
* -
*
* BREACHED
means that the SLO's budget was exhausted, as of the time that you specified in
* TimeStamp
.
*
*
* -
*
* INSUFFICIENT_DATA
means that the specifed start and end times were before the SLO was
* created, or that attainment data is missing.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServiceLevelObjectiveBudgetStatus
*/
public ServiceLevelObjectiveBudgetReport withBudgetStatus(String budgetStatus) {
setBudgetStatus(budgetStatus);
return this;
}
/**
*
* The status of this SLO, as it relates to the error budget for the entire time interval.
*
*
* -
*
* OK
means that the SLO had remaining budget above the warning threshold, as of the time that you
* specified in TimeStamp
.
*
*
* -
*
* WARNING
means that the SLO's remaining budget was below the warning threshold, as of the time that
* you specified in TimeStamp
.
*
*
* -
*
* BREACHED
means that the SLO's budget was exhausted, as of the time that you specified in
* TimeStamp
.
*
*
* -
*
* INSUFFICIENT_DATA
means that the specifed start and end times were before the SLO was created, or
* that attainment data is missing.
*
*
*
*
* @param budgetStatus
* The status of this SLO, as it relates to the error budget for the entire time interval.
*
* -
*
* OK
means that the SLO had remaining budget above the warning threshold, as of the time that
* you specified in TimeStamp
.
*
*
* -
*
* WARNING
means that the SLO's remaining budget was below the warning threshold, as of the time
* that you specified in TimeStamp
.
*
*
* -
*
* BREACHED
means that the SLO's budget was exhausted, as of the time that you specified in
* TimeStamp
.
*
*
* -
*
* INSUFFICIENT_DATA
means that the specifed start and end times were before the SLO was
* created, or that attainment data is missing.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServiceLevelObjectiveBudgetStatus
*/
public ServiceLevelObjectiveBudgetReport withBudgetStatus(ServiceLevelObjectiveBudgetStatus budgetStatus) {
this.budgetStatus = budgetStatus.toString();
return this;
}
/**
*
* A number between 0 and 100 that represents the percentage of time periods that the service has attained the SLO's
* attainment goal, as of the time of the request.
*
*
* @param attainment
* A number between 0 and 100 that represents the percentage of time periods that the service has attained
* the SLO's attainment goal, as of the time of the request.
*/
public void setAttainment(Double attainment) {
this.attainment = attainment;
}
/**
*
* A number between 0 and 100 that represents the percentage of time periods that the service has attained the SLO's
* attainment goal, as of the time of the request.
*
*
* @return A number between 0 and 100 that represents the percentage of time periods that the service has attained
* the SLO's attainment goal, as of the time of the request.
*/
public Double getAttainment() {
return this.attainment;
}
/**
*
* A number between 0 and 100 that represents the percentage of time periods that the service has attained the SLO's
* attainment goal, as of the time of the request.
*
*
* @param attainment
* A number between 0 and 100 that represents the percentage of time periods that the service has attained
* the SLO's attainment goal, as of the time of the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceLevelObjectiveBudgetReport withAttainment(Double attainment) {
setAttainment(attainment);
return this;
}
/**
*
* The total number of seconds in the error budget for the interval.
*
*
* @param totalBudgetSeconds
* The total number of seconds in the error budget for the interval.
*/
public void setTotalBudgetSeconds(Integer totalBudgetSeconds) {
this.totalBudgetSeconds = totalBudgetSeconds;
}
/**
*
* The total number of seconds in the error budget for the interval.
*
*
* @return The total number of seconds in the error budget for the interval.
*/
public Integer getTotalBudgetSeconds() {
return this.totalBudgetSeconds;
}
/**
*
* The total number of seconds in the error budget for the interval.
*
*
* @param totalBudgetSeconds
* The total number of seconds in the error budget for the interval.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceLevelObjectiveBudgetReport withTotalBudgetSeconds(Integer totalBudgetSeconds) {
setTotalBudgetSeconds(totalBudgetSeconds);
return this;
}
/**
*
* The budget amount remaining before the SLO status becomes BREACHING
, at the time specified in the
* Timestemp
parameter of the request. If this value is negative, then the SLO is already in
* BREACHING
status.
*
*
* @param budgetSecondsRemaining
* The budget amount remaining before the SLO status becomes BREACHING
, at the time specified in
* the Timestemp
parameter of the request. If this value is negative, then the SLO is already in
* BREACHING
status.
*/
public void setBudgetSecondsRemaining(Integer budgetSecondsRemaining) {
this.budgetSecondsRemaining = budgetSecondsRemaining;
}
/**
*
* The budget amount remaining before the SLO status becomes BREACHING
, at the time specified in the
* Timestemp
parameter of the request. If this value is negative, then the SLO is already in
* BREACHING
status.
*
*
* @return The budget amount remaining before the SLO status becomes BREACHING
, at the time specified
* in the Timestemp
parameter of the request. If this value is negative, then the SLO is
* already in BREACHING
status.
*/
public Integer getBudgetSecondsRemaining() {
return this.budgetSecondsRemaining;
}
/**
*
* The budget amount remaining before the SLO status becomes BREACHING
, at the time specified in the
* Timestemp
parameter of the request. If this value is negative, then the SLO is already in
* BREACHING
status.
*
*
* @param budgetSecondsRemaining
* The budget amount remaining before the SLO status becomes BREACHING
, at the time specified in
* the Timestemp
parameter of the request. If this value is negative, then the SLO is already in
* BREACHING
status.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceLevelObjectiveBudgetReport withBudgetSecondsRemaining(Integer budgetSecondsRemaining) {
setBudgetSecondsRemaining(budgetSecondsRemaining);
return this;
}
/**
*
* A structure that contains information about the performance metric that this SLO monitors.
*
*
* @param sli
* A structure that contains information about the performance metric that this SLO monitors.
*/
public void setSli(ServiceLevelIndicator sli) {
this.sli = sli;
}
/**
*
* A structure that contains information about the performance metric that this SLO monitors.
*
*
* @return A structure that contains information about the performance metric that this SLO monitors.
*/
public ServiceLevelIndicator getSli() {
return this.sli;
}
/**
*
* A structure that contains information about the performance metric that this SLO monitors.
*
*
* @param sli
* A structure that contains information about the performance metric that this SLO monitors.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceLevelObjectiveBudgetReport withSli(ServiceLevelIndicator sli) {
setSli(sli);
return this;
}
/**
* @param goal
*/
public void setGoal(Goal goal) {
this.goal = goal;
}
/**
* @return
*/
public Goal getGoal() {
return this.goal;
}
/**
* @param goal
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceLevelObjectiveBudgetReport withGoal(Goal goal) {
setGoal(goal);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getArn() != null)
sb.append("Arn: ").append(getArn()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getBudgetStatus() != null)
sb.append("BudgetStatus: ").append(getBudgetStatus()).append(",");
if (getAttainment() != null)
sb.append("Attainment: ").append(getAttainment()).append(",");
if (getTotalBudgetSeconds() != null)
sb.append("TotalBudgetSeconds: ").append(getTotalBudgetSeconds()).append(",");
if (getBudgetSecondsRemaining() != null)
sb.append("BudgetSecondsRemaining: ").append(getBudgetSecondsRemaining()).append(",");
if (getSli() != null)
sb.append("Sli: ").append(getSli()).append(",");
if (getGoal() != null)
sb.append("Goal: ").append(getGoal());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ServiceLevelObjectiveBudgetReport == false)
return false;
ServiceLevelObjectiveBudgetReport other = (ServiceLevelObjectiveBudgetReport) obj;
if (other.getArn() == null ^ this.getArn() == null)
return false;
if (other.getArn() != null && other.getArn().equals(this.getArn()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getBudgetStatus() == null ^ this.getBudgetStatus() == null)
return false;
if (other.getBudgetStatus() != null && other.getBudgetStatus().equals(this.getBudgetStatus()) == false)
return false;
if (other.getAttainment() == null ^ this.getAttainment() == null)
return false;
if (other.getAttainment() != null && other.getAttainment().equals(this.getAttainment()) == false)
return false;
if (other.getTotalBudgetSeconds() == null ^ this.getTotalBudgetSeconds() == null)
return false;
if (other.getTotalBudgetSeconds() != null && other.getTotalBudgetSeconds().equals(this.getTotalBudgetSeconds()) == false)
return false;
if (other.getBudgetSecondsRemaining() == null ^ this.getBudgetSecondsRemaining() == null)
return false;
if (other.getBudgetSecondsRemaining() != null && other.getBudgetSecondsRemaining().equals(this.getBudgetSecondsRemaining()) == false)
return false;
if (other.getSli() == null ^ this.getSli() == null)
return false;
if (other.getSli() != null && other.getSli().equals(this.getSli()) == false)
return false;
if (other.getGoal() == null ^ this.getGoal() == null)
return false;
if (other.getGoal() != null && other.getGoal().equals(this.getGoal()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getArn() == null) ? 0 : getArn().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getBudgetStatus() == null) ? 0 : getBudgetStatus().hashCode());
hashCode = prime * hashCode + ((getAttainment() == null) ? 0 : getAttainment().hashCode());
hashCode = prime * hashCode + ((getTotalBudgetSeconds() == null) ? 0 : getTotalBudgetSeconds().hashCode());
hashCode = prime * hashCode + ((getBudgetSecondsRemaining() == null) ? 0 : getBudgetSecondsRemaining().hashCode());
hashCode = prime * hashCode + ((getSli() == null) ? 0 : getSli().hashCode());
hashCode = prime * hashCode + ((getGoal() == null) ? 0 : getGoal().hashCode());
return hashCode;
}
@Override
public ServiceLevelObjectiveBudgetReport clone() {
try {
return (ServiceLevelObjectiveBudgetReport) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.applicationsignals.model.transform.ServiceLevelObjectiveBudgetReportMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}