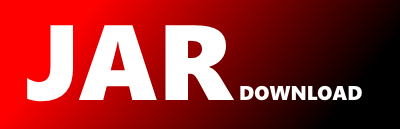
com.amazonaws.services.appmesh.AWSAppMesh Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appmesh Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appmesh;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.appmesh.model.*;
/**
* Interface for accessing AWS App Mesh.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.appmesh.AbstractAWSAppMesh} instead.
*
*
*
* AWS App Mesh is a service mesh based on the Envoy proxy that makes it easy to monitor and control microservices. App
* Mesh standardizes how your microservices communicate, giving you end-to-end visibility and helping to ensure high
* availability for your applications.
*
*
* App Mesh gives you consistent visibility and network traffic controls for every microservice in an application. You
* can use App Mesh with AWS Fargate, Amazon ECS, Amazon EKS, Kubernetes on AWS, and Amazon EC2.
*
*
*
* App Mesh supports microservice applications that use service discovery naming for their components. For more
* information about service discovery on Amazon ECS, see Service Discovery in the
* Amazon Elastic Container Service Developer Guide. Kubernetes kube-dns
and coredns
* are supported. For more information, see DNS for Services and Pods in the
* Kubernetes documentation.
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSAppMesh {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "appmesh";
/**
*
* Creates a service mesh. A service mesh is a logical boundary for network traffic between the services that reside
* within it.
*
*
* After you create your service mesh, you can create virtual services, virtual nodes, virtual routers, and routes
* to distribute traffic between the applications in your mesh.
*
*
* @param createMeshRequest
* @return Result of the CreateMesh operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the AWS App Mesh User Guide.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.CreateMesh
* @see AWS API
* Documentation
*/
CreateMeshResult createMesh(CreateMeshRequest createMeshRequest);
/**
*
* Creates a route that is associated with a virtual router.
*
*
* You can use the prefix
parameter in your route specification for path-based routing of requests. For
* example, if your virtual service name is my-service.local
and you want the route to match requests
* to my-service.local/metrics
, your prefix should be /metrics
.
*
*
* If your route matches a request, you can distribute traffic to one or more target virtual nodes with relative
* weighting.
*
*
* @param createRouteRequest
* @return Result of the CreateRoute operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the AWS App Mesh User Guide.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.CreateRoute
* @see AWS API
* Documentation
*/
CreateRouteResult createRoute(CreateRouteRequest createRouteRequest);
/**
*
* Creates a virtual node within a service mesh.
*
*
* A virtual node acts as a logical pointer to a particular task group, such as an Amazon ECS service or a
* Kubernetes deployment. When you create a virtual node, you can specify the service discovery information for your
* task group.
*
*
* Any inbound traffic that your virtual node expects should be specified as a listener
. Any outbound
* traffic that your virtual node expects to reach should be specified as a backend
.
*
*
* The response metadata for your new virtual node contains the arn
that is associated with the virtual
* node. Set this value (either the full ARN or the truncated resource name: for example,
* mesh/default/virtualNode/simpleapp
) as the APPMESH_VIRTUAL_NODE_NAME
environment
* variable for your task group's Envoy proxy container in your task definition or pod spec. This is then mapped to
* the node.id
and node.cluster
Envoy parameters.
*
*
*
* If you require your Envoy stats or tracing to use a different name, you can override the
* node.cluster
value that is set by APPMESH_VIRTUAL_NODE_NAME
with the
* APPMESH_VIRTUAL_NODE_CLUSTER
environment variable.
*
*
*
* @param createVirtualNodeRequest
* @return Result of the CreateVirtualNode operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the AWS App Mesh User Guide.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.CreateVirtualNode
* @see AWS API
* Documentation
*/
CreateVirtualNodeResult createVirtualNode(CreateVirtualNodeRequest createVirtualNodeRequest);
/**
*
* Creates a virtual router within a service mesh.
*
*
* Any inbound traffic that your virtual router expects should be specified as a listener
.
*
*
* Virtual routers handle traffic for one or more virtual services within your mesh. After you create your virtual
* router, create and associate routes for your virtual router that direct incoming requests to different virtual
* nodes.
*
*
* @param createVirtualRouterRequest
* @return Result of the CreateVirtualRouter operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the AWS App Mesh User Guide.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.CreateVirtualRouter
* @see AWS
* API Documentation
*/
CreateVirtualRouterResult createVirtualRouter(CreateVirtualRouterRequest createVirtualRouterRequest);
/**
*
* Creates a virtual service within a service mesh.
*
*
* A virtual service is an abstraction of a real service that is provided by a virtual node directly or indirectly
* by means of a virtual router. Dependent services call your virtual service by its virtualServiceName
* , and those requests are routed to the virtual node or virtual router that is specified as the provider for the
* virtual service.
*
*
* @param createVirtualServiceRequest
* @return Result of the CreateVirtualService operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the AWS App Mesh User Guide.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.CreateVirtualService
* @see AWS
* API Documentation
*/
CreateVirtualServiceResult createVirtualService(CreateVirtualServiceRequest createVirtualServiceRequest);
/**
*
* Deletes an existing service mesh.
*
*
* You must delete all resources (virtual services, routes, virtual routers, and virtual nodes) in the service mesh
* before you can delete the mesh itself.
*
*
* @param deleteMeshRequest
* @return Result of the DeleteMesh operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ResourceInUseException
* You can't delete the specified resource because it's in use or required by another resource.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.DeleteMesh
* @see AWS API
* Documentation
*/
DeleteMeshResult deleteMesh(DeleteMeshRequest deleteMeshRequest);
/**
*
* Deletes an existing route.
*
*
* @param deleteRouteRequest
* @return Result of the DeleteRoute operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ResourceInUseException
* You can't delete the specified resource because it's in use or required by another resource.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.DeleteRoute
* @see AWS API
* Documentation
*/
DeleteRouteResult deleteRoute(DeleteRouteRequest deleteRouteRequest);
/**
*
* Deletes an existing virtual node.
*
*
* You must delete any virtual services that list a virtual node as a service provider before you can delete the
* virtual node itself.
*
*
* @param deleteVirtualNodeRequest
* @return Result of the DeleteVirtualNode operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ResourceInUseException
* You can't delete the specified resource because it's in use or required by another resource.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.DeleteVirtualNode
* @see AWS API
* Documentation
*/
DeleteVirtualNodeResult deleteVirtualNode(DeleteVirtualNodeRequest deleteVirtualNodeRequest);
/**
*
* Deletes an existing virtual router.
*
*
* You must delete any routes associated with the virtual router before you can delete the router itself.
*
*
* @param deleteVirtualRouterRequest
* @return Result of the DeleteVirtualRouter operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ResourceInUseException
* You can't delete the specified resource because it's in use or required by another resource.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.DeleteVirtualRouter
* @see AWS
* API Documentation
*/
DeleteVirtualRouterResult deleteVirtualRouter(DeleteVirtualRouterRequest deleteVirtualRouterRequest);
/**
*
* Deletes an existing virtual service.
*
*
* @param deleteVirtualServiceRequest
* @return Result of the DeleteVirtualService operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.DeleteVirtualService
* @see AWS
* API Documentation
*/
DeleteVirtualServiceResult deleteVirtualService(DeleteVirtualServiceRequest deleteVirtualServiceRequest);
/**
*
* Describes an existing service mesh.
*
*
* @param describeMeshRequest
* @return Result of the DescribeMesh operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.DescribeMesh
* @see AWS API
* Documentation
*/
DescribeMeshResult describeMesh(DescribeMeshRequest describeMeshRequest);
/**
*
* Describes an existing route.
*
*
* @param describeRouteRequest
* @return Result of the DescribeRoute operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.DescribeRoute
* @see AWS API
* Documentation
*/
DescribeRouteResult describeRoute(DescribeRouteRequest describeRouteRequest);
/**
*
* Describes an existing virtual node.
*
*
* @param describeVirtualNodeRequest
* @return Result of the DescribeVirtualNode operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.DescribeVirtualNode
* @see AWS
* API Documentation
*/
DescribeVirtualNodeResult describeVirtualNode(DescribeVirtualNodeRequest describeVirtualNodeRequest);
/**
*
* Describes an existing virtual router.
*
*
* @param describeVirtualRouterRequest
* @return Result of the DescribeVirtualRouter operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.DescribeVirtualRouter
* @see AWS
* API Documentation
*/
DescribeVirtualRouterResult describeVirtualRouter(DescribeVirtualRouterRequest describeVirtualRouterRequest);
/**
*
* Describes an existing virtual service.
*
*
* @param describeVirtualServiceRequest
* @return Result of the DescribeVirtualService operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.DescribeVirtualService
* @see AWS
* API Documentation
*/
DescribeVirtualServiceResult describeVirtualService(DescribeVirtualServiceRequest describeVirtualServiceRequest);
/**
*
* Returns a list of existing service meshes.
*
*
* @param listMeshesRequest
* @return Result of the ListMeshes operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.ListMeshes
* @see AWS API
* Documentation
*/
ListMeshesResult listMeshes(ListMeshesRequest listMeshesRequest);
/**
*
* Returns a list of existing routes in a service mesh.
*
*
* @param listRoutesRequest
* @return Result of the ListRoutes operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.ListRoutes
* @see AWS API
* Documentation
*/
ListRoutesResult listRoutes(ListRoutesRequest listRoutesRequest);
/**
*
* List the tags for an App Mesh resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.ListTagsForResource
* @see AWS
* API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Returns a list of existing virtual nodes.
*
*
* @param listVirtualNodesRequest
* @return Result of the ListVirtualNodes operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.ListVirtualNodes
* @see AWS API
* Documentation
*/
ListVirtualNodesResult listVirtualNodes(ListVirtualNodesRequest listVirtualNodesRequest);
/**
*
* Returns a list of existing virtual routers in a service mesh.
*
*
* @param listVirtualRoutersRequest
* @return Result of the ListVirtualRouters operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.ListVirtualRouters
* @see AWS API
* Documentation
*/
ListVirtualRoutersResult listVirtualRouters(ListVirtualRoutersRequest listVirtualRoutersRequest);
/**
*
* Returns a list of existing virtual services in a service mesh.
*
*
* @param listVirtualServicesRequest
* @return Result of the ListVirtualServices operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.ListVirtualServices
* @see AWS
* API Documentation
*/
ListVirtualServicesResult listVirtualServices(ListVirtualServicesRequest listVirtualServicesRequest);
/**
*
* Associates the specified tags to a resource with the specified resourceArn
. If existing tags on a
* resource aren't specified in the request parameters, they aren't changed. When a resource is deleted, the tags
* associated with that resource are also deleted.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws TooManyTagsException
* The request exceeds the maximum allowed number of tags allowed per resource. The current limit is 50 user
* tags per resource. You must reduce the number of tags in the request. None of the tags in this request
* were applied.
* @sample AWSAppMesh.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Deletes specified tags from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates an existing service mesh.
*
*
* @param updateMeshRequest
* @return Result of the UpdateMesh operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.UpdateMesh
* @see AWS API
* Documentation
*/
UpdateMeshResult updateMesh(UpdateMeshRequest updateMeshRequest);
/**
*
* Updates an existing route for a specified service mesh and virtual router.
*
*
* @param updateRouteRequest
* @return Result of the UpdateRoute operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the AWS App Mesh User Guide.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.UpdateRoute
* @see AWS API
* Documentation
*/
UpdateRouteResult updateRoute(UpdateRouteRequest updateRouteRequest);
/**
*
* Updates an existing virtual node in a specified service mesh.
*
*
* @param updateVirtualNodeRequest
* @return Result of the UpdateVirtualNode operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the AWS App Mesh User Guide.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.UpdateVirtualNode
* @see AWS API
* Documentation
*/
UpdateVirtualNodeResult updateVirtualNode(UpdateVirtualNodeRequest updateVirtualNodeRequest);
/**
*
* Updates an existing virtual router in a specified service mesh.
*
*
* @param updateVirtualRouterRequest
* @return Result of the UpdateVirtualRouter operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the AWS App Mesh User Guide.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.UpdateVirtualRouter
* @see AWS
* API Documentation
*/
UpdateVirtualRouterResult updateVirtualRouter(UpdateVirtualRouterRequest updateVirtualRouterRequest);
/**
*
* Updates an existing virtual service in a specified service mesh.
*
*
* @param updateVirtualServiceRequest
* @return Result of the UpdateVirtualService operation returned by the service.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the AWS App Mesh User Guide.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @sample AWSAppMesh.UpdateVirtualService
* @see AWS
* API Documentation
*/
UpdateVirtualServiceResult updateVirtualService(UpdateVirtualServiceRequest updateVirtualServiceRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}