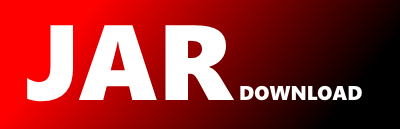
com.amazonaws.services.appmesh.AWSAppMeshClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appmesh Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appmesh;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.appmesh.AWSAppMeshClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.appmesh.model.*;
import com.amazonaws.services.appmesh.model.transform.*;
/**
* Client for accessing AWS App Mesh. All service calls made using this client are blocking, and will not return until
* the service call completes.
*
*
* App Mesh is a service mesh based on the Envoy proxy that makes it easy to monitor and control microservices. App Mesh
* standardizes how your microservices communicate, giving you end-to-end visibility and helping to ensure high
* availability for your applications.
*
*
* App Mesh gives you consistent visibility and network traffic controls for every microservice in an application. You
* can use App Mesh with Amazon Web Services Fargate, Amazon ECS, Amazon EKS, Kubernetes on Amazon Web Services, and
* Amazon EC2.
*
*
*
* App Mesh supports microservice applications that use service discovery naming for their components. For more
* information about service discovery on Amazon ECS, see Service Discovery in
* the Amazon Elastic Container Service Developer Guide. Kubernetes kube-dns
and
* coredns
are supported. For more information, see DNS for Services and Pods in the
* Kubernetes documentation.
*
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSAppMeshClient extends AmazonWebServiceClient implements AWSAppMesh {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSAppMesh.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "appmesh";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("LimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.appmesh.model.transform.LimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ForbiddenException").withExceptionUnmarshaller(
com.amazonaws.services.appmesh.model.transform.ForbiddenExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceInUseException").withExceptionUnmarshaller(
com.amazonaws.services.appmesh.model.transform.ResourceInUseExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("NotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.appmesh.model.transform.NotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.appmesh.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TooManyTagsException").withExceptionUnmarshaller(
com.amazonaws.services.appmesh.model.transform.TooManyTagsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceUnavailableException").withExceptionUnmarshaller(
com.amazonaws.services.appmesh.model.transform.ServiceUnavailableExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TooManyRequestsException").withExceptionUnmarshaller(
com.amazonaws.services.appmesh.model.transform.TooManyRequestsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("BadRequestException").withExceptionUnmarshaller(
com.amazonaws.services.appmesh.model.transform.BadRequestExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerErrorException").withExceptionUnmarshaller(
com.amazonaws.services.appmesh.model.transform.InternalServerErrorExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.appmesh.model.AWSAppMeshException.class));
public static AWSAppMeshClientBuilder builder() {
return AWSAppMeshClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on AWS App Mesh using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSAppMeshClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on AWS App Mesh using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSAppMeshClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("appmesh.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/appmesh/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/appmesh/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Creates a gateway route.
*
*
* A gateway route is attached to a virtual gateway and routes traffic to an existing virtual service. If a route
* matches a request, it can distribute traffic to a target virtual service.
*
*
* For more information about gateway routes, see Gateway routes.
*
*
* @param createGatewayRouteRequest
* @return Result of the CreateGatewayRoute operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the App Mesh User Guide.
* @sample AWSAppMesh.CreateGatewayRoute
* @see AWS API
* Documentation
*/
@Override
public CreateGatewayRouteResult createGatewayRoute(CreateGatewayRouteRequest request) {
request = beforeClientExecution(request);
return executeCreateGatewayRoute(request);
}
@SdkInternalApi
final CreateGatewayRouteResult executeCreateGatewayRoute(CreateGatewayRouteRequest createGatewayRouteRequest) {
ExecutionContext executionContext = createExecutionContext(createGatewayRouteRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateGatewayRouteRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createGatewayRouteRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateGatewayRoute");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateGatewayRouteResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a service mesh.
*
*
* A service mesh is a logical boundary for network traffic between services that are represented by resources
* within the mesh. After you create your service mesh, you can create virtual services, virtual nodes, virtual
* routers, and routes to distribute traffic between the applications in your mesh.
*
*
* For more information about service meshes, see Service meshes.
*
*
* @param createMeshRequest
* @return Result of the CreateMesh operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the App Mesh User Guide.
* @sample AWSAppMesh.CreateMesh
* @see AWS API
* Documentation
*/
@Override
public CreateMeshResult createMesh(CreateMeshRequest request) {
request = beforeClientExecution(request);
return executeCreateMesh(request);
}
@SdkInternalApi
final CreateMeshResult executeCreateMesh(CreateMeshRequest createMeshRequest) {
ExecutionContext executionContext = createExecutionContext(createMeshRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMeshRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createMeshRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMesh");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateMeshResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a route that is associated with a virtual router.
*
*
* You can route several different protocols and define a retry policy for a route. Traffic can be routed to one or
* more virtual nodes.
*
*
* For more information about routes, see Routes.
*
*
* @param createRouteRequest
* @return Result of the CreateRoute operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the App Mesh User Guide.
* @sample AWSAppMesh.CreateRoute
* @see AWS API
* Documentation
*/
@Override
public CreateRouteResult createRoute(CreateRouteRequest request) {
request = beforeClientExecution(request);
return executeCreateRoute(request);
}
@SdkInternalApi
final CreateRouteResult executeCreateRoute(CreateRouteRequest createRouteRequest) {
ExecutionContext executionContext = createExecutionContext(createRouteRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateRouteRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createRouteRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateRoute");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateRouteResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a virtual gateway.
*
*
* A virtual gateway allows resources outside your mesh to communicate to resources that are inside your mesh. The
* virtual gateway represents an Envoy proxy running in an Amazon ECS task, in a Kubernetes service, or on an Amazon
* EC2 instance. Unlike a virtual node, which represents an Envoy running with an application, a virtual gateway
* represents Envoy deployed by itself.
*
*
* For more information about virtual gateways, see Virtual gateways.
*
*
* @param createVirtualGatewayRequest
* @return Result of the CreateVirtualGateway operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the App Mesh User Guide.
* @sample AWSAppMesh.CreateVirtualGateway
* @see AWS
* API Documentation
*/
@Override
public CreateVirtualGatewayResult createVirtualGateway(CreateVirtualGatewayRequest request) {
request = beforeClientExecution(request);
return executeCreateVirtualGateway(request);
}
@SdkInternalApi
final CreateVirtualGatewayResult executeCreateVirtualGateway(CreateVirtualGatewayRequest createVirtualGatewayRequest) {
ExecutionContext executionContext = createExecutionContext(createVirtualGatewayRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateVirtualGatewayRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createVirtualGatewayRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateVirtualGateway");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateVirtualGatewayResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a virtual node within a service mesh.
*
*
* A virtual node acts as a logical pointer to a particular task group, such as an Amazon ECS service or a
* Kubernetes deployment. When you create a virtual node, you can specify the service discovery information for your
* task group, and whether the proxy running in a task group will communicate with other proxies using Transport
* Layer Security (TLS).
*
*
* You define a listener
for any inbound traffic that your virtual node expects. Any virtual service
* that your virtual node expects to communicate to is specified as a backend
.
*
*
* The response metadata for your new virtual node contains the arn
that is associated with the virtual
* node. Set this value to the full ARN; for example,
* arn:aws:appmesh:us-west-2:123456789012:myMesh/default/virtualNode/myApp
) as the
* APPMESH_RESOURCE_ARN
environment variable for your task group's Envoy proxy container in your task
* definition or pod spec. This is then mapped to the node.id
and node.cluster
Envoy
* parameters.
*
*
*
* By default, App Mesh uses the name of the resource you specified in APPMESH_RESOURCE_ARN
when Envoy
* is referring to itself in metrics and traces. You can override this behavior by setting the
* APPMESH_RESOURCE_CLUSTER
environment variable with your own name.
*
*
*
* For more information about virtual nodes, see Virtual nodes. You must be
* using 1.15.0
or later of the Envoy image when setting these variables. For more information aboutApp
* Mesh Envoy variables, see Envoy
* image in the App Mesh User Guide.
*
*
* @param createVirtualNodeRequest
* @return Result of the CreateVirtualNode operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the App Mesh User Guide.
* @sample AWSAppMesh.CreateVirtualNode
* @see AWS API
* Documentation
*/
@Override
public CreateVirtualNodeResult createVirtualNode(CreateVirtualNodeRequest request) {
request = beforeClientExecution(request);
return executeCreateVirtualNode(request);
}
@SdkInternalApi
final CreateVirtualNodeResult executeCreateVirtualNode(CreateVirtualNodeRequest createVirtualNodeRequest) {
ExecutionContext executionContext = createExecutionContext(createVirtualNodeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateVirtualNodeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createVirtualNodeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateVirtualNode");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateVirtualNodeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a virtual router within a service mesh.
*
*
* Specify a listener
for any inbound traffic that your virtual router receives. Create a virtual
* router for each protocol and port that you need to route. Virtual routers handle traffic for one or more virtual
* services within your mesh. After you create your virtual router, create and associate routes for your virtual
* router that direct incoming requests to different virtual nodes.
*
*
* For more information about virtual routers, see Virtual routers.
*
*
* @param createVirtualRouterRequest
* @return Result of the CreateVirtualRouter operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the App Mesh User Guide.
* @sample AWSAppMesh.CreateVirtualRouter
* @see AWS
* API Documentation
*/
@Override
public CreateVirtualRouterResult createVirtualRouter(CreateVirtualRouterRequest request) {
request = beforeClientExecution(request);
return executeCreateVirtualRouter(request);
}
@SdkInternalApi
final CreateVirtualRouterResult executeCreateVirtualRouter(CreateVirtualRouterRequest createVirtualRouterRequest) {
ExecutionContext executionContext = createExecutionContext(createVirtualRouterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateVirtualRouterRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createVirtualRouterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateVirtualRouter");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateVirtualRouterResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a virtual service within a service mesh.
*
*
* A virtual service is an abstraction of a real service that is provided by a virtual node directly or indirectly
* by means of a virtual router. Dependent services call your virtual service by its virtualServiceName
* , and those requests are routed to the virtual node or virtual router that is specified as the provider for the
* virtual service.
*
*
* For more information about virtual services, see Virtual services.
*
*
* @param createVirtualServiceRequest
* @return Result of the CreateVirtualService operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the App Mesh User Guide.
* @sample AWSAppMesh.CreateVirtualService
* @see AWS
* API Documentation
*/
@Override
public CreateVirtualServiceResult createVirtualService(CreateVirtualServiceRequest request) {
request = beforeClientExecution(request);
return executeCreateVirtualService(request);
}
@SdkInternalApi
final CreateVirtualServiceResult executeCreateVirtualService(CreateVirtualServiceRequest createVirtualServiceRequest) {
ExecutionContext executionContext = createExecutionContext(createVirtualServiceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateVirtualServiceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createVirtualServiceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateVirtualService");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateVirtualServiceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an existing gateway route.
*
*
* @param deleteGatewayRouteRequest
* @return Result of the DeleteGatewayRoute operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ResourceInUseException
* You can't delete the specified resource because it's in use or required by another resource.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.DeleteGatewayRoute
* @see AWS API
* Documentation
*/
@Override
public DeleteGatewayRouteResult deleteGatewayRoute(DeleteGatewayRouteRequest request) {
request = beforeClientExecution(request);
return executeDeleteGatewayRoute(request);
}
@SdkInternalApi
final DeleteGatewayRouteResult executeDeleteGatewayRoute(DeleteGatewayRouteRequest deleteGatewayRouteRequest) {
ExecutionContext executionContext = createExecutionContext(deleteGatewayRouteRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteGatewayRouteRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteGatewayRouteRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteGatewayRoute");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteGatewayRouteResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an existing service mesh.
*
*
* You must delete all resources (virtual services, routes, virtual routers, and virtual nodes) in the service mesh
* before you can delete the mesh itself.
*
*
* @param deleteMeshRequest
* @return Result of the DeleteMesh operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ResourceInUseException
* You can't delete the specified resource because it's in use or required by another resource.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.DeleteMesh
* @see AWS API
* Documentation
*/
@Override
public DeleteMeshResult deleteMesh(DeleteMeshRequest request) {
request = beforeClientExecution(request);
return executeDeleteMesh(request);
}
@SdkInternalApi
final DeleteMeshResult executeDeleteMesh(DeleteMeshRequest deleteMeshRequest) {
ExecutionContext executionContext = createExecutionContext(deleteMeshRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteMeshRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteMeshRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteMesh");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteMeshResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an existing route.
*
*
* @param deleteRouteRequest
* @return Result of the DeleteRoute operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ResourceInUseException
* You can't delete the specified resource because it's in use or required by another resource.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.DeleteRoute
* @see AWS API
* Documentation
*/
@Override
public DeleteRouteResult deleteRoute(DeleteRouteRequest request) {
request = beforeClientExecution(request);
return executeDeleteRoute(request);
}
@SdkInternalApi
final DeleteRouteResult executeDeleteRoute(DeleteRouteRequest deleteRouteRequest) {
ExecutionContext executionContext = createExecutionContext(deleteRouteRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteRouteRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteRouteRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteRoute");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteRouteResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an existing virtual gateway. You cannot delete a virtual gateway if any gateway routes are associated to
* it.
*
*
* @param deleteVirtualGatewayRequest
* @return Result of the DeleteVirtualGateway operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ResourceInUseException
* You can't delete the specified resource because it's in use or required by another resource.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.DeleteVirtualGateway
* @see AWS
* API Documentation
*/
@Override
public DeleteVirtualGatewayResult deleteVirtualGateway(DeleteVirtualGatewayRequest request) {
request = beforeClientExecution(request);
return executeDeleteVirtualGateway(request);
}
@SdkInternalApi
final DeleteVirtualGatewayResult executeDeleteVirtualGateway(DeleteVirtualGatewayRequest deleteVirtualGatewayRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVirtualGatewayRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVirtualGatewayRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteVirtualGatewayRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVirtualGateway");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteVirtualGatewayResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an existing virtual node.
*
*
* You must delete any virtual services that list a virtual node as a service provider before you can delete the
* virtual node itself.
*
*
* @param deleteVirtualNodeRequest
* Deletes a virtual node input.
* @return Result of the DeleteVirtualNode operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ResourceInUseException
* You can't delete the specified resource because it's in use or required by another resource.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.DeleteVirtualNode
* @see AWS API
* Documentation
*/
@Override
public DeleteVirtualNodeResult deleteVirtualNode(DeleteVirtualNodeRequest request) {
request = beforeClientExecution(request);
return executeDeleteVirtualNode(request);
}
@SdkInternalApi
final DeleteVirtualNodeResult executeDeleteVirtualNode(DeleteVirtualNodeRequest deleteVirtualNodeRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVirtualNodeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVirtualNodeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteVirtualNodeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVirtualNode");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteVirtualNodeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an existing virtual router.
*
*
* You must delete any routes associated with the virtual router before you can delete the router itself.
*
*
* @param deleteVirtualRouterRequest
* @return Result of the DeleteVirtualRouter operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ResourceInUseException
* You can't delete the specified resource because it's in use or required by another resource.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.DeleteVirtualRouter
* @see AWS
* API Documentation
*/
@Override
public DeleteVirtualRouterResult deleteVirtualRouter(DeleteVirtualRouterRequest request) {
request = beforeClientExecution(request);
return executeDeleteVirtualRouter(request);
}
@SdkInternalApi
final DeleteVirtualRouterResult executeDeleteVirtualRouter(DeleteVirtualRouterRequest deleteVirtualRouterRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVirtualRouterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVirtualRouterRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteVirtualRouterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVirtualRouter");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteVirtualRouterResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an existing virtual service.
*
*
* @param deleteVirtualServiceRequest
* @return Result of the DeleteVirtualService operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ResourceInUseException
* You can't delete the specified resource because it's in use or required by another resource.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.DeleteVirtualService
* @see AWS
* API Documentation
*/
@Override
public DeleteVirtualServiceResult deleteVirtualService(DeleteVirtualServiceRequest request) {
request = beforeClientExecution(request);
return executeDeleteVirtualService(request);
}
@SdkInternalApi
final DeleteVirtualServiceResult executeDeleteVirtualService(DeleteVirtualServiceRequest deleteVirtualServiceRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVirtualServiceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVirtualServiceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteVirtualServiceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVirtualService");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteVirtualServiceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes an existing gateway route.
*
*
* @param describeGatewayRouteRequest
* @return Result of the DescribeGatewayRoute operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.DescribeGatewayRoute
* @see AWS
* API Documentation
*/
@Override
public DescribeGatewayRouteResult describeGatewayRoute(DescribeGatewayRouteRequest request) {
request = beforeClientExecution(request);
return executeDescribeGatewayRoute(request);
}
@SdkInternalApi
final DescribeGatewayRouteResult executeDescribeGatewayRoute(DescribeGatewayRouteRequest describeGatewayRouteRequest) {
ExecutionContext executionContext = createExecutionContext(describeGatewayRouteRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeGatewayRouteRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeGatewayRouteRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeGatewayRoute");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeGatewayRouteResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes an existing service mesh.
*
*
* @param describeMeshRequest
* @return Result of the DescribeMesh operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.DescribeMesh
* @see AWS API
* Documentation
*/
@Override
public DescribeMeshResult describeMesh(DescribeMeshRequest request) {
request = beforeClientExecution(request);
return executeDescribeMesh(request);
}
@SdkInternalApi
final DescribeMeshResult executeDescribeMesh(DescribeMeshRequest describeMeshRequest) {
ExecutionContext executionContext = createExecutionContext(describeMeshRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeMeshRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeMeshRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeMesh");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeMeshResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes an existing route.
*
*
* @param describeRouteRequest
* @return Result of the DescribeRoute operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.DescribeRoute
* @see AWS API
* Documentation
*/
@Override
public DescribeRouteResult describeRoute(DescribeRouteRequest request) {
request = beforeClientExecution(request);
return executeDescribeRoute(request);
}
@SdkInternalApi
final DescribeRouteResult executeDescribeRoute(DescribeRouteRequest describeRouteRequest) {
ExecutionContext executionContext = createExecutionContext(describeRouteRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeRouteRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeRouteRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeRoute");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeRouteResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes an existing virtual gateway.
*
*
* @param describeVirtualGatewayRequest
* @return Result of the DescribeVirtualGateway operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.DescribeVirtualGateway
* @see AWS
* API Documentation
*/
@Override
public DescribeVirtualGatewayResult describeVirtualGateway(DescribeVirtualGatewayRequest request) {
request = beforeClientExecution(request);
return executeDescribeVirtualGateway(request);
}
@SdkInternalApi
final DescribeVirtualGatewayResult executeDescribeVirtualGateway(DescribeVirtualGatewayRequest describeVirtualGatewayRequest) {
ExecutionContext executionContext = createExecutionContext(describeVirtualGatewayRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeVirtualGatewayRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeVirtualGatewayRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeVirtualGateway");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeVirtualGatewayResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes an existing virtual node.
*
*
* @param describeVirtualNodeRequest
* @return Result of the DescribeVirtualNode operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.DescribeVirtualNode
* @see AWS
* API Documentation
*/
@Override
public DescribeVirtualNodeResult describeVirtualNode(DescribeVirtualNodeRequest request) {
request = beforeClientExecution(request);
return executeDescribeVirtualNode(request);
}
@SdkInternalApi
final DescribeVirtualNodeResult executeDescribeVirtualNode(DescribeVirtualNodeRequest describeVirtualNodeRequest) {
ExecutionContext executionContext = createExecutionContext(describeVirtualNodeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeVirtualNodeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeVirtualNodeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeVirtualNode");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeVirtualNodeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes an existing virtual router.
*
*
* @param describeVirtualRouterRequest
* @return Result of the DescribeVirtualRouter operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.DescribeVirtualRouter
* @see AWS
* API Documentation
*/
@Override
public DescribeVirtualRouterResult describeVirtualRouter(DescribeVirtualRouterRequest request) {
request = beforeClientExecution(request);
return executeDescribeVirtualRouter(request);
}
@SdkInternalApi
final DescribeVirtualRouterResult executeDescribeVirtualRouter(DescribeVirtualRouterRequest describeVirtualRouterRequest) {
ExecutionContext executionContext = createExecutionContext(describeVirtualRouterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeVirtualRouterRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeVirtualRouterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeVirtualRouter");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeVirtualRouterResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes an existing virtual service.
*
*
* @param describeVirtualServiceRequest
* @return Result of the DescribeVirtualService operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.DescribeVirtualService
* @see AWS
* API Documentation
*/
@Override
public DescribeVirtualServiceResult describeVirtualService(DescribeVirtualServiceRequest request) {
request = beforeClientExecution(request);
return executeDescribeVirtualService(request);
}
@SdkInternalApi
final DescribeVirtualServiceResult executeDescribeVirtualService(DescribeVirtualServiceRequest describeVirtualServiceRequest) {
ExecutionContext executionContext = createExecutionContext(describeVirtualServiceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeVirtualServiceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeVirtualServiceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeVirtualService");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeVirtualServiceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of existing gateway routes that are associated to a virtual gateway.
*
*
* @param listGatewayRoutesRequest
* @return Result of the ListGatewayRoutes operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.ListGatewayRoutes
* @see AWS API
* Documentation
*/
@Override
public ListGatewayRoutesResult listGatewayRoutes(ListGatewayRoutesRequest request) {
request = beforeClientExecution(request);
return executeListGatewayRoutes(request);
}
@SdkInternalApi
final ListGatewayRoutesResult executeListGatewayRoutes(ListGatewayRoutesRequest listGatewayRoutesRequest) {
ExecutionContext executionContext = createExecutionContext(listGatewayRoutesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListGatewayRoutesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listGatewayRoutesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListGatewayRoutes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListGatewayRoutesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of existing service meshes.
*
*
* @param listMeshesRequest
* @return Result of the ListMeshes operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.ListMeshes
* @see AWS API
* Documentation
*/
@Override
public ListMeshesResult listMeshes(ListMeshesRequest request) {
request = beforeClientExecution(request);
return executeListMeshes(request);
}
@SdkInternalApi
final ListMeshesResult executeListMeshes(ListMeshesRequest listMeshesRequest) {
ExecutionContext executionContext = createExecutionContext(listMeshesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListMeshesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listMeshesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListMeshes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListMeshesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of existing routes in a service mesh.
*
*
* @param listRoutesRequest
* @return Result of the ListRoutes operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.ListRoutes
* @see AWS API
* Documentation
*/
@Override
public ListRoutesResult listRoutes(ListRoutesRequest request) {
request = beforeClientExecution(request);
return executeListRoutes(request);
}
@SdkInternalApi
final ListRoutesResult executeListRoutes(ListRoutesRequest listRoutesRequest) {
ExecutionContext executionContext = createExecutionContext(listRoutesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListRoutesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listRoutesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListRoutes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListRoutesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List the tags for an App Mesh resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.ListTagsForResource
* @see AWS
* API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of existing virtual gateways in a service mesh.
*
*
* @param listVirtualGatewaysRequest
* @return Result of the ListVirtualGateways operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.ListVirtualGateways
* @see AWS
* API Documentation
*/
@Override
public ListVirtualGatewaysResult listVirtualGateways(ListVirtualGatewaysRequest request) {
request = beforeClientExecution(request);
return executeListVirtualGateways(request);
}
@SdkInternalApi
final ListVirtualGatewaysResult executeListVirtualGateways(ListVirtualGatewaysRequest listVirtualGatewaysRequest) {
ExecutionContext executionContext = createExecutionContext(listVirtualGatewaysRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListVirtualGatewaysRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listVirtualGatewaysRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListVirtualGateways");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListVirtualGatewaysResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of existing virtual nodes.
*
*
* @param listVirtualNodesRequest
* @return Result of the ListVirtualNodes operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.ListVirtualNodes
* @see AWS API
* Documentation
*/
@Override
public ListVirtualNodesResult listVirtualNodes(ListVirtualNodesRequest request) {
request = beforeClientExecution(request);
return executeListVirtualNodes(request);
}
@SdkInternalApi
final ListVirtualNodesResult executeListVirtualNodes(ListVirtualNodesRequest listVirtualNodesRequest) {
ExecutionContext executionContext = createExecutionContext(listVirtualNodesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListVirtualNodesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listVirtualNodesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListVirtualNodes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListVirtualNodesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of existing virtual routers in a service mesh.
*
*
* @param listVirtualRoutersRequest
* @return Result of the ListVirtualRouters operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.ListVirtualRouters
* @see AWS API
* Documentation
*/
@Override
public ListVirtualRoutersResult listVirtualRouters(ListVirtualRoutersRequest request) {
request = beforeClientExecution(request);
return executeListVirtualRouters(request);
}
@SdkInternalApi
final ListVirtualRoutersResult executeListVirtualRouters(ListVirtualRoutersRequest listVirtualRoutersRequest) {
ExecutionContext executionContext = createExecutionContext(listVirtualRoutersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListVirtualRoutersRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listVirtualRoutersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListVirtualRouters");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListVirtualRoutersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of existing virtual services in a service mesh.
*
*
* @param listVirtualServicesRequest
* @return Result of the ListVirtualServices operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.ListVirtualServices
* @see AWS
* API Documentation
*/
@Override
public ListVirtualServicesResult listVirtualServices(ListVirtualServicesRequest request) {
request = beforeClientExecution(request);
return executeListVirtualServices(request);
}
@SdkInternalApi
final ListVirtualServicesResult executeListVirtualServices(ListVirtualServicesRequest listVirtualServicesRequest) {
ExecutionContext executionContext = createExecutionContext(listVirtualServicesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListVirtualServicesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listVirtualServicesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListVirtualServices");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListVirtualServicesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates the specified tags to a resource with the specified resourceArn
. If existing tags on a
* resource aren't specified in the request parameters, they aren't changed. When a resource is deleted, the tags
* associated with that resource are also deleted.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyTagsException
* The request exceeds the maximum allowed number of tags allowed per resource. The current limit is 50 user
* tags per resource. You must reduce the number of tags in the request. None of the tags in this request
* were applied.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes specified tags from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an existing gateway route that is associated to a specified virtual gateway in a service mesh.
*
*
* @param updateGatewayRouteRequest
* @return Result of the UpdateGatewayRoute operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the App Mesh User Guide.
* @sample AWSAppMesh.UpdateGatewayRoute
* @see AWS API
* Documentation
*/
@Override
public UpdateGatewayRouteResult updateGatewayRoute(UpdateGatewayRouteRequest request) {
request = beforeClientExecution(request);
return executeUpdateGatewayRoute(request);
}
@SdkInternalApi
final UpdateGatewayRouteResult executeUpdateGatewayRoute(UpdateGatewayRouteRequest updateGatewayRouteRequest) {
ExecutionContext executionContext = createExecutionContext(updateGatewayRouteRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateGatewayRouteRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateGatewayRouteRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateGatewayRoute");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateGatewayRouteResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an existing service mesh.
*
*
* @param updateMeshRequest
* @return Result of the UpdateMesh operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AWSAppMesh.UpdateMesh
* @see AWS API
* Documentation
*/
@Override
public UpdateMeshResult updateMesh(UpdateMeshRequest request) {
request = beforeClientExecution(request);
return executeUpdateMesh(request);
}
@SdkInternalApi
final UpdateMeshResult executeUpdateMesh(UpdateMeshRequest updateMeshRequest) {
ExecutionContext executionContext = createExecutionContext(updateMeshRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateMeshRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateMeshRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateMesh");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateMeshResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an existing route for a specified service mesh and virtual router.
*
*
* @param updateRouteRequest
* @return Result of the UpdateRoute operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the App Mesh User Guide.
* @sample AWSAppMesh.UpdateRoute
* @see AWS API
* Documentation
*/
@Override
public UpdateRouteResult updateRoute(UpdateRouteRequest request) {
request = beforeClientExecution(request);
return executeUpdateRoute(request);
}
@SdkInternalApi
final UpdateRouteResult executeUpdateRoute(UpdateRouteRequest updateRouteRequest) {
ExecutionContext executionContext = createExecutionContext(updateRouteRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateRouteRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateRouteRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateRoute");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateRouteResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an existing virtual gateway in a specified service mesh.
*
*
* @param updateVirtualGatewayRequest
* @return Result of the UpdateVirtualGateway operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the App Mesh User Guide.
* @sample AWSAppMesh.UpdateVirtualGateway
* @see AWS
* API Documentation
*/
@Override
public UpdateVirtualGatewayResult updateVirtualGateway(UpdateVirtualGatewayRequest request) {
request = beforeClientExecution(request);
return executeUpdateVirtualGateway(request);
}
@SdkInternalApi
final UpdateVirtualGatewayResult executeUpdateVirtualGateway(UpdateVirtualGatewayRequest updateVirtualGatewayRequest) {
ExecutionContext executionContext = createExecutionContext(updateVirtualGatewayRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateVirtualGatewayRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateVirtualGatewayRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateVirtualGateway");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateVirtualGatewayResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an existing virtual node in a specified service mesh.
*
*
* @param updateVirtualNodeRequest
* @return Result of the UpdateVirtualNode operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the App Mesh User Guide.
* @sample AWSAppMesh.UpdateVirtualNode
* @see AWS API
* Documentation
*/
@Override
public UpdateVirtualNodeResult updateVirtualNode(UpdateVirtualNodeRequest request) {
request = beforeClientExecution(request);
return executeUpdateVirtualNode(request);
}
@SdkInternalApi
final UpdateVirtualNodeResult executeUpdateVirtualNode(UpdateVirtualNodeRequest updateVirtualNodeRequest) {
ExecutionContext executionContext = createExecutionContext(updateVirtualNodeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateVirtualNodeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateVirtualNodeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateVirtualNode");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateVirtualNodeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an existing virtual router in a specified service mesh.
*
*
* @param updateVirtualRouterRequest
* @return Result of the UpdateVirtualRouter operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the App Mesh User Guide.
* @sample AWSAppMesh.UpdateVirtualRouter
* @see AWS
* API Documentation
*/
@Override
public UpdateVirtualRouterResult updateVirtualRouter(UpdateVirtualRouterRequest request) {
request = beforeClientExecution(request);
return executeUpdateVirtualRouter(request);
}
@SdkInternalApi
final UpdateVirtualRouterResult executeUpdateVirtualRouter(UpdateVirtualRouterRequest updateVirtualRouterRequest) {
ExecutionContext executionContext = createExecutionContext(updateVirtualRouterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateVirtualRouterRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateVirtualRouterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateVirtualRouter");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateVirtualRouterResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an existing virtual service in a specified service mesh.
*
*
* @param updateVirtualServiceRequest
* @return Result of the UpdateVirtualService operation returned by the service.
* @throws NotFoundException
* The specified resource doesn't exist. Check your request syntax and try again.
* @throws BadRequestException
* The request syntax was malformed. Check your request syntax and try again.
* @throws ConflictException
* The request contains a client token that was used for a previous update resource call with different
* specifications. Try the request again with a new client token.
* @throws TooManyRequestsException
* The maximum request rate permitted by the App Mesh APIs has been exceeded for your account. For best
* results, use an increasing or variable sleep interval between requests.
* @throws ForbiddenException
* You don't have permissions to perform this action.
* @throws ServiceUnavailableException
* The request has failed due to a temporary failure of the service.
* @throws InternalServerErrorException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws LimitExceededException
* You have exceeded a service limit for your account. For more information, see Service Limits in
* the App Mesh User Guide.
* @sample AWSAppMesh.UpdateVirtualService
* @see AWS
* API Documentation
*/
@Override
public UpdateVirtualServiceResult updateVirtualService(UpdateVirtualServiceRequest request) {
request = beforeClientExecution(request);
return executeUpdateVirtualService(request);
}
@SdkInternalApi
final UpdateVirtualServiceResult executeUpdateVirtualService(UpdateVirtualServiceRequest updateVirtualServiceRequest) {
ExecutionContext executionContext = createExecutionContext(updateVirtualServiceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateVirtualServiceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateVirtualServiceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "App Mesh");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateVirtualService");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateVirtualServiceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}