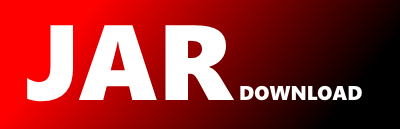
com.amazonaws.services.appmesh.AWSAppMeshAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appmesh Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appmesh;
import javax.annotation.Generated;
import com.amazonaws.services.appmesh.model.*;
/**
* Interface for accessing AWS App Mesh asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.appmesh.AbstractAWSAppMeshAsync} instead.
*
*
*
* App Mesh is a service mesh based on the Envoy proxy that makes it easy to monitor and control microservices. App Mesh
* standardizes how your microservices communicate, giving you end-to-end visibility and helping to ensure high
* availability for your applications.
*
*
* App Mesh gives you consistent visibility and network traffic controls for every microservice in an application. You
* can use App Mesh with Amazon Web Services Fargate, Amazon ECS, Amazon EKS, Kubernetes on Amazon Web Services, and
* Amazon EC2.
*
*
*
* App Mesh supports microservice applications that use service discovery naming for their components. For more
* information about service discovery on Amazon ECS, see Service Discovery in
* the Amazon Elastic Container Service Developer Guide. Kubernetes kube-dns
and
* coredns
are supported. For more information, see DNS for Services and Pods in the
* Kubernetes documentation.
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSAppMeshAsync extends AWSAppMesh {
/**
*
* Creates a gateway route.
*
*
* A gateway route is attached to a virtual gateway and routes traffic to an existing virtual service. If a route
* matches a request, it can distribute traffic to a target virtual service.
*
*
* For more information about gateway routes, see Gateway routes.
*
*
* @param createGatewayRouteRequest
* @return A Java Future containing the result of the CreateGatewayRoute operation returned by the service.
* @sample AWSAppMeshAsync.CreateGatewayRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createGatewayRouteAsync(CreateGatewayRouteRequest createGatewayRouteRequest);
/**
*
* Creates a gateway route.
*
*
* A gateway route is attached to a virtual gateway and routes traffic to an existing virtual service. If a route
* matches a request, it can distribute traffic to a target virtual service.
*
*
* For more information about gateway routes, see Gateway routes.
*
*
* @param createGatewayRouteRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateGatewayRoute operation returned by the service.
* @sample AWSAppMeshAsyncHandler.CreateGatewayRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createGatewayRouteAsync(CreateGatewayRouteRequest createGatewayRouteRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a service mesh.
*
*
* A service mesh is a logical boundary for network traffic between services that are represented by resources
* within the mesh. After you create your service mesh, you can create virtual services, virtual nodes, virtual
* routers, and routes to distribute traffic between the applications in your mesh.
*
*
* For more information about service meshes, see Service meshes.
*
*
* @param createMeshRequest
* @return A Java Future containing the result of the CreateMesh operation returned by the service.
* @sample AWSAppMeshAsync.CreateMesh
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createMeshAsync(CreateMeshRequest createMeshRequest);
/**
*
* Creates a service mesh.
*
*
* A service mesh is a logical boundary for network traffic between services that are represented by resources
* within the mesh. After you create your service mesh, you can create virtual services, virtual nodes, virtual
* routers, and routes to distribute traffic between the applications in your mesh.
*
*
* For more information about service meshes, see Service meshes.
*
*
* @param createMeshRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMesh operation returned by the service.
* @sample AWSAppMeshAsyncHandler.CreateMesh
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createMeshAsync(CreateMeshRequest createMeshRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a route that is associated with a virtual router.
*
*
* You can route several different protocols and define a retry policy for a route. Traffic can be routed to one or
* more virtual nodes.
*
*
* For more information about routes, see Routes.
*
*
* @param createRouteRequest
* @return A Java Future containing the result of the CreateRoute operation returned by the service.
* @sample AWSAppMeshAsync.CreateRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createRouteAsync(CreateRouteRequest createRouteRequest);
/**
*
* Creates a route that is associated with a virtual router.
*
*
* You can route several different protocols and define a retry policy for a route. Traffic can be routed to one or
* more virtual nodes.
*
*
* For more information about routes, see Routes.
*
*
* @param createRouteRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRoute operation returned by the service.
* @sample AWSAppMeshAsyncHandler.CreateRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createRouteAsync(CreateRouteRequest createRouteRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a virtual gateway.
*
*
* A virtual gateway allows resources outside your mesh to communicate to resources that are inside your mesh. The
* virtual gateway represents an Envoy proxy running in an Amazon ECS task, in a Kubernetes service, or on an Amazon
* EC2 instance. Unlike a virtual node, which represents an Envoy running with an application, a virtual gateway
* represents Envoy deployed by itself.
*
*
* For more information about virtual gateways, see Virtual gateways.
*
*
* @param createVirtualGatewayRequest
* @return A Java Future containing the result of the CreateVirtualGateway operation returned by the service.
* @sample AWSAppMeshAsync.CreateVirtualGateway
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createVirtualGatewayAsync(CreateVirtualGatewayRequest createVirtualGatewayRequest);
/**
*
* Creates a virtual gateway.
*
*
* A virtual gateway allows resources outside your mesh to communicate to resources that are inside your mesh. The
* virtual gateway represents an Envoy proxy running in an Amazon ECS task, in a Kubernetes service, or on an Amazon
* EC2 instance. Unlike a virtual node, which represents an Envoy running with an application, a virtual gateway
* represents Envoy deployed by itself.
*
*
* For more information about virtual gateways, see Virtual gateways.
*
*
* @param createVirtualGatewayRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateVirtualGateway operation returned by the service.
* @sample AWSAppMeshAsyncHandler.CreateVirtualGateway
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createVirtualGatewayAsync(CreateVirtualGatewayRequest createVirtualGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a virtual node within a service mesh.
*
*
* A virtual node acts as a logical pointer to a particular task group, such as an Amazon ECS service or a
* Kubernetes deployment. When you create a virtual node, you can specify the service discovery information for your
* task group, and whether the proxy running in a task group will communicate with other proxies using Transport
* Layer Security (TLS).
*
*
* You define a listener
for any inbound traffic that your virtual node expects. Any virtual service
* that your virtual node expects to communicate to is specified as a backend
.
*
*
* The response metadata for your new virtual node contains the arn
that is associated with the virtual
* node. Set this value to the full ARN; for example,
* arn:aws:appmesh:us-west-2:123456789012:myMesh/default/virtualNode/myApp
) as the
* APPMESH_RESOURCE_ARN
environment variable for your task group's Envoy proxy container in your task
* definition or pod spec. This is then mapped to the node.id
and node.cluster
Envoy
* parameters.
*
*
*
* By default, App Mesh uses the name of the resource you specified in APPMESH_RESOURCE_ARN
when Envoy
* is referring to itself in metrics and traces. You can override this behavior by setting the
* APPMESH_RESOURCE_CLUSTER
environment variable with your own name.
*
*
*
* For more information about virtual nodes, see Virtual nodes. You must be
* using 1.15.0
or later of the Envoy image when setting these variables. For more information aboutApp
* Mesh Envoy variables, see Envoy
* image in the App Mesh User Guide.
*
*
* @param createVirtualNodeRequest
* @return A Java Future containing the result of the CreateVirtualNode operation returned by the service.
* @sample AWSAppMeshAsync.CreateVirtualNode
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createVirtualNodeAsync(CreateVirtualNodeRequest createVirtualNodeRequest);
/**
*
* Creates a virtual node within a service mesh.
*
*
* A virtual node acts as a logical pointer to a particular task group, such as an Amazon ECS service or a
* Kubernetes deployment. When you create a virtual node, you can specify the service discovery information for your
* task group, and whether the proxy running in a task group will communicate with other proxies using Transport
* Layer Security (TLS).
*
*
* You define a listener
for any inbound traffic that your virtual node expects. Any virtual service
* that your virtual node expects to communicate to is specified as a backend
.
*
*
* The response metadata for your new virtual node contains the arn
that is associated with the virtual
* node. Set this value to the full ARN; for example,
* arn:aws:appmesh:us-west-2:123456789012:myMesh/default/virtualNode/myApp
) as the
* APPMESH_RESOURCE_ARN
environment variable for your task group's Envoy proxy container in your task
* definition or pod spec. This is then mapped to the node.id
and node.cluster
Envoy
* parameters.
*
*
*
* By default, App Mesh uses the name of the resource you specified in APPMESH_RESOURCE_ARN
when Envoy
* is referring to itself in metrics and traces. You can override this behavior by setting the
* APPMESH_RESOURCE_CLUSTER
environment variable with your own name.
*
*
*
* For more information about virtual nodes, see Virtual nodes. You must be
* using 1.15.0
or later of the Envoy image when setting these variables. For more information aboutApp
* Mesh Envoy variables, see Envoy
* image in the App Mesh User Guide.
*
*
* @param createVirtualNodeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateVirtualNode operation returned by the service.
* @sample AWSAppMeshAsyncHandler.CreateVirtualNode
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createVirtualNodeAsync(CreateVirtualNodeRequest createVirtualNodeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a virtual router within a service mesh.
*
*
* Specify a listener
for any inbound traffic that your virtual router receives. Create a virtual
* router for each protocol and port that you need to route. Virtual routers handle traffic for one or more virtual
* services within your mesh. After you create your virtual router, create and associate routes for your virtual
* router that direct incoming requests to different virtual nodes.
*
*
* For more information about virtual routers, see Virtual routers.
*
*
* @param createVirtualRouterRequest
* @return A Java Future containing the result of the CreateVirtualRouter operation returned by the service.
* @sample AWSAppMeshAsync.CreateVirtualRouter
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createVirtualRouterAsync(CreateVirtualRouterRequest createVirtualRouterRequest);
/**
*
* Creates a virtual router within a service mesh.
*
*
* Specify a listener
for any inbound traffic that your virtual router receives. Create a virtual
* router for each protocol and port that you need to route. Virtual routers handle traffic for one or more virtual
* services within your mesh. After you create your virtual router, create and associate routes for your virtual
* router that direct incoming requests to different virtual nodes.
*
*
* For more information about virtual routers, see Virtual routers.
*
*
* @param createVirtualRouterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateVirtualRouter operation returned by the service.
* @sample AWSAppMeshAsyncHandler.CreateVirtualRouter
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createVirtualRouterAsync(CreateVirtualRouterRequest createVirtualRouterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a virtual service within a service mesh.
*
*
* A virtual service is an abstraction of a real service that is provided by a virtual node directly or indirectly
* by means of a virtual router. Dependent services call your virtual service by its virtualServiceName
* , and those requests are routed to the virtual node or virtual router that is specified as the provider for the
* virtual service.
*
*
* For more information about virtual services, see Virtual services.
*
*
* @param createVirtualServiceRequest
* @return A Java Future containing the result of the CreateVirtualService operation returned by the service.
* @sample AWSAppMeshAsync.CreateVirtualService
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createVirtualServiceAsync(CreateVirtualServiceRequest createVirtualServiceRequest);
/**
*
* Creates a virtual service within a service mesh.
*
*
* A virtual service is an abstraction of a real service that is provided by a virtual node directly or indirectly
* by means of a virtual router. Dependent services call your virtual service by its virtualServiceName
* , and those requests are routed to the virtual node or virtual router that is specified as the provider for the
* virtual service.
*
*
* For more information about virtual services, see Virtual services.
*
*
* @param createVirtualServiceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateVirtualService operation returned by the service.
* @sample AWSAppMeshAsyncHandler.CreateVirtualService
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createVirtualServiceAsync(CreateVirtualServiceRequest createVirtualServiceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing gateway route.
*
*
* @param deleteGatewayRouteRequest
* @return A Java Future containing the result of the DeleteGatewayRoute operation returned by the service.
* @sample AWSAppMeshAsync.DeleteGatewayRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteGatewayRouteAsync(DeleteGatewayRouteRequest deleteGatewayRouteRequest);
/**
*
* Deletes an existing gateway route.
*
*
* @param deleteGatewayRouteRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteGatewayRoute operation returned by the service.
* @sample AWSAppMeshAsyncHandler.DeleteGatewayRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteGatewayRouteAsync(DeleteGatewayRouteRequest deleteGatewayRouteRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing service mesh.
*
*
* You must delete all resources (virtual services, routes, virtual routers, and virtual nodes) in the service mesh
* before you can delete the mesh itself.
*
*
* @param deleteMeshRequest
* @return A Java Future containing the result of the DeleteMesh operation returned by the service.
* @sample AWSAppMeshAsync.DeleteMesh
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteMeshAsync(DeleteMeshRequest deleteMeshRequest);
/**
*
* Deletes an existing service mesh.
*
*
* You must delete all resources (virtual services, routes, virtual routers, and virtual nodes) in the service mesh
* before you can delete the mesh itself.
*
*
* @param deleteMeshRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMesh operation returned by the service.
* @sample AWSAppMeshAsyncHandler.DeleteMesh
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteMeshAsync(DeleteMeshRequest deleteMeshRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing route.
*
*
* @param deleteRouteRequest
* @return A Java Future containing the result of the DeleteRoute operation returned by the service.
* @sample AWSAppMeshAsync.DeleteRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteRouteAsync(DeleteRouteRequest deleteRouteRequest);
/**
*
* Deletes an existing route.
*
*
* @param deleteRouteRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRoute operation returned by the service.
* @sample AWSAppMeshAsyncHandler.DeleteRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteRouteAsync(DeleteRouteRequest deleteRouteRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing virtual gateway. You cannot delete a virtual gateway if any gateway routes are associated to
* it.
*
*
* @param deleteVirtualGatewayRequest
* @return A Java Future containing the result of the DeleteVirtualGateway operation returned by the service.
* @sample AWSAppMeshAsync.DeleteVirtualGateway
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteVirtualGatewayAsync(DeleteVirtualGatewayRequest deleteVirtualGatewayRequest);
/**
*
* Deletes an existing virtual gateway. You cannot delete a virtual gateway if any gateway routes are associated to
* it.
*
*
* @param deleteVirtualGatewayRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVirtualGateway operation returned by the service.
* @sample AWSAppMeshAsyncHandler.DeleteVirtualGateway
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteVirtualGatewayAsync(DeleteVirtualGatewayRequest deleteVirtualGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing virtual node.
*
*
* You must delete any virtual services that list a virtual node as a service provider before you can delete the
* virtual node itself.
*
*
* @param deleteVirtualNodeRequest
* Deletes a virtual node input.
* @return A Java Future containing the result of the DeleteVirtualNode operation returned by the service.
* @sample AWSAppMeshAsync.DeleteVirtualNode
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteVirtualNodeAsync(DeleteVirtualNodeRequest deleteVirtualNodeRequest);
/**
*
* Deletes an existing virtual node.
*
*
* You must delete any virtual services that list a virtual node as a service provider before you can delete the
* virtual node itself.
*
*
* @param deleteVirtualNodeRequest
* Deletes a virtual node input.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVirtualNode operation returned by the service.
* @sample AWSAppMeshAsyncHandler.DeleteVirtualNode
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteVirtualNodeAsync(DeleteVirtualNodeRequest deleteVirtualNodeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing virtual router.
*
*
* You must delete any routes associated with the virtual router before you can delete the router itself.
*
*
* @param deleteVirtualRouterRequest
* @return A Java Future containing the result of the DeleteVirtualRouter operation returned by the service.
* @sample AWSAppMeshAsync.DeleteVirtualRouter
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteVirtualRouterAsync(DeleteVirtualRouterRequest deleteVirtualRouterRequest);
/**
*
* Deletes an existing virtual router.
*
*
* You must delete any routes associated with the virtual router before you can delete the router itself.
*
*
* @param deleteVirtualRouterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVirtualRouter operation returned by the service.
* @sample AWSAppMeshAsyncHandler.DeleteVirtualRouter
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteVirtualRouterAsync(DeleteVirtualRouterRequest deleteVirtualRouterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing virtual service.
*
*
* @param deleteVirtualServiceRequest
* @return A Java Future containing the result of the DeleteVirtualService operation returned by the service.
* @sample AWSAppMeshAsync.DeleteVirtualService
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteVirtualServiceAsync(DeleteVirtualServiceRequest deleteVirtualServiceRequest);
/**
*
* Deletes an existing virtual service.
*
*
* @param deleteVirtualServiceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVirtualService operation returned by the service.
* @sample AWSAppMeshAsyncHandler.DeleteVirtualService
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteVirtualServiceAsync(DeleteVirtualServiceRequest deleteVirtualServiceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an existing gateway route.
*
*
* @param describeGatewayRouteRequest
* @return A Java Future containing the result of the DescribeGatewayRoute operation returned by the service.
* @sample AWSAppMeshAsync.DescribeGatewayRoute
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeGatewayRouteAsync(DescribeGatewayRouteRequest describeGatewayRouteRequest);
/**
*
* Describes an existing gateway route.
*
*
* @param describeGatewayRouteRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeGatewayRoute operation returned by the service.
* @sample AWSAppMeshAsyncHandler.DescribeGatewayRoute
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeGatewayRouteAsync(DescribeGatewayRouteRequest describeGatewayRouteRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an existing service mesh.
*
*
* @param describeMeshRequest
* @return A Java Future containing the result of the DescribeMesh operation returned by the service.
* @sample AWSAppMeshAsync.DescribeMesh
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeMeshAsync(DescribeMeshRequest describeMeshRequest);
/**
*
* Describes an existing service mesh.
*
*
* @param describeMeshRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeMesh operation returned by the service.
* @sample AWSAppMeshAsyncHandler.DescribeMesh
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeMeshAsync(DescribeMeshRequest describeMeshRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an existing route.
*
*
* @param describeRouteRequest
* @return A Java Future containing the result of the DescribeRoute operation returned by the service.
* @sample AWSAppMeshAsync.DescribeRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeRouteAsync(DescribeRouteRequest describeRouteRequest);
/**
*
* Describes an existing route.
*
*
* @param describeRouteRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRoute operation returned by the service.
* @sample AWSAppMeshAsyncHandler.DescribeRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeRouteAsync(DescribeRouteRequest describeRouteRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an existing virtual gateway.
*
*
* @param describeVirtualGatewayRequest
* @return A Java Future containing the result of the DescribeVirtualGateway operation returned by the service.
* @sample AWSAppMeshAsync.DescribeVirtualGateway
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeVirtualGatewayAsync(DescribeVirtualGatewayRequest describeVirtualGatewayRequest);
/**
*
* Describes an existing virtual gateway.
*
*
* @param describeVirtualGatewayRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeVirtualGateway operation returned by the service.
* @sample AWSAppMeshAsyncHandler.DescribeVirtualGateway
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeVirtualGatewayAsync(DescribeVirtualGatewayRequest describeVirtualGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an existing virtual node.
*
*
* @param describeVirtualNodeRequest
* @return A Java Future containing the result of the DescribeVirtualNode operation returned by the service.
* @sample AWSAppMeshAsync.DescribeVirtualNode
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeVirtualNodeAsync(DescribeVirtualNodeRequest describeVirtualNodeRequest);
/**
*
* Describes an existing virtual node.
*
*
* @param describeVirtualNodeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeVirtualNode operation returned by the service.
* @sample AWSAppMeshAsyncHandler.DescribeVirtualNode
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeVirtualNodeAsync(DescribeVirtualNodeRequest describeVirtualNodeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an existing virtual router.
*
*
* @param describeVirtualRouterRequest
* @return A Java Future containing the result of the DescribeVirtualRouter operation returned by the service.
* @sample AWSAppMeshAsync.DescribeVirtualRouter
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeVirtualRouterAsync(DescribeVirtualRouterRequest describeVirtualRouterRequest);
/**
*
* Describes an existing virtual router.
*
*
* @param describeVirtualRouterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeVirtualRouter operation returned by the service.
* @sample AWSAppMeshAsyncHandler.DescribeVirtualRouter
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeVirtualRouterAsync(DescribeVirtualRouterRequest describeVirtualRouterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an existing virtual service.
*
*
* @param describeVirtualServiceRequest
* @return A Java Future containing the result of the DescribeVirtualService operation returned by the service.
* @sample AWSAppMeshAsync.DescribeVirtualService
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeVirtualServiceAsync(DescribeVirtualServiceRequest describeVirtualServiceRequest);
/**
*
* Describes an existing virtual service.
*
*
* @param describeVirtualServiceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeVirtualService operation returned by the service.
* @sample AWSAppMeshAsyncHandler.DescribeVirtualService
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeVirtualServiceAsync(DescribeVirtualServiceRequest describeVirtualServiceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of existing gateway routes that are associated to a virtual gateway.
*
*
* @param listGatewayRoutesRequest
* @return A Java Future containing the result of the ListGatewayRoutes operation returned by the service.
* @sample AWSAppMeshAsync.ListGatewayRoutes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listGatewayRoutesAsync(ListGatewayRoutesRequest listGatewayRoutesRequest);
/**
*
* Returns a list of existing gateway routes that are associated to a virtual gateway.
*
*
* @param listGatewayRoutesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListGatewayRoutes operation returned by the service.
* @sample AWSAppMeshAsyncHandler.ListGatewayRoutes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listGatewayRoutesAsync(ListGatewayRoutesRequest listGatewayRoutesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of existing service meshes.
*
*
* @param listMeshesRequest
* @return A Java Future containing the result of the ListMeshes operation returned by the service.
* @sample AWSAppMeshAsync.ListMeshes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listMeshesAsync(ListMeshesRequest listMeshesRequest);
/**
*
* Returns a list of existing service meshes.
*
*
* @param listMeshesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMeshes operation returned by the service.
* @sample AWSAppMeshAsyncHandler.ListMeshes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listMeshesAsync(ListMeshesRequest listMeshesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of existing routes in a service mesh.
*
*
* @param listRoutesRequest
* @return A Java Future containing the result of the ListRoutes operation returned by the service.
* @sample AWSAppMeshAsync.ListRoutes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listRoutesAsync(ListRoutesRequest listRoutesRequest);
/**
*
* Returns a list of existing routes in a service mesh.
*
*
* @param listRoutesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRoutes operation returned by the service.
* @sample AWSAppMeshAsyncHandler.ListRoutes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listRoutesAsync(ListRoutesRequest listRoutesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List the tags for an App Mesh resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSAppMeshAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* List the tags for an App Mesh resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSAppMeshAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of existing virtual gateways in a service mesh.
*
*
* @param listVirtualGatewaysRequest
* @return A Java Future containing the result of the ListVirtualGateways operation returned by the service.
* @sample AWSAppMeshAsync.ListVirtualGateways
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listVirtualGatewaysAsync(ListVirtualGatewaysRequest listVirtualGatewaysRequest);
/**
*
* Returns a list of existing virtual gateways in a service mesh.
*
*
* @param listVirtualGatewaysRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListVirtualGateways operation returned by the service.
* @sample AWSAppMeshAsyncHandler.ListVirtualGateways
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listVirtualGatewaysAsync(ListVirtualGatewaysRequest listVirtualGatewaysRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of existing virtual nodes.
*
*
* @param listVirtualNodesRequest
* @return A Java Future containing the result of the ListVirtualNodes operation returned by the service.
* @sample AWSAppMeshAsync.ListVirtualNodes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listVirtualNodesAsync(ListVirtualNodesRequest listVirtualNodesRequest);
/**
*
* Returns a list of existing virtual nodes.
*
*
* @param listVirtualNodesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListVirtualNodes operation returned by the service.
* @sample AWSAppMeshAsyncHandler.ListVirtualNodes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listVirtualNodesAsync(ListVirtualNodesRequest listVirtualNodesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of existing virtual routers in a service mesh.
*
*
* @param listVirtualRoutersRequest
* @return A Java Future containing the result of the ListVirtualRouters operation returned by the service.
* @sample AWSAppMeshAsync.ListVirtualRouters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listVirtualRoutersAsync(ListVirtualRoutersRequest listVirtualRoutersRequest);
/**
*
* Returns a list of existing virtual routers in a service mesh.
*
*
* @param listVirtualRoutersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListVirtualRouters operation returned by the service.
* @sample AWSAppMeshAsyncHandler.ListVirtualRouters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listVirtualRoutersAsync(ListVirtualRoutersRequest listVirtualRoutersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of existing virtual services in a service mesh.
*
*
* @param listVirtualServicesRequest
* @return A Java Future containing the result of the ListVirtualServices operation returned by the service.
* @sample AWSAppMeshAsync.ListVirtualServices
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listVirtualServicesAsync(ListVirtualServicesRequest listVirtualServicesRequest);
/**
*
* Returns a list of existing virtual services in a service mesh.
*
*
* @param listVirtualServicesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListVirtualServices operation returned by the service.
* @sample AWSAppMeshAsyncHandler.ListVirtualServices
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listVirtualServicesAsync(ListVirtualServicesRequest listVirtualServicesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates the specified tags to a resource with the specified resourceArn
. If existing tags on a
* resource aren't specified in the request parameters, they aren't changed. When a resource is deleted, the tags
* associated with that resource are also deleted.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSAppMeshAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Associates the specified tags to a resource with the specified resourceArn
. If existing tags on a
* resource aren't specified in the request parameters, they aren't changed. When a resource is deleted, the tags
* associated with that resource are also deleted.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSAppMeshAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes specified tags from a resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSAppMeshAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Deletes specified tags from a resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSAppMeshAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing gateway route that is associated to a specified virtual gateway in a service mesh.
*
*
* @param updateGatewayRouteRequest
* @return A Java Future containing the result of the UpdateGatewayRoute operation returned by the service.
* @sample AWSAppMeshAsync.UpdateGatewayRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateGatewayRouteAsync(UpdateGatewayRouteRequest updateGatewayRouteRequest);
/**
*
* Updates an existing gateway route that is associated to a specified virtual gateway in a service mesh.
*
*
* @param updateGatewayRouteRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateGatewayRoute operation returned by the service.
* @sample AWSAppMeshAsyncHandler.UpdateGatewayRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateGatewayRouteAsync(UpdateGatewayRouteRequest updateGatewayRouteRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing service mesh.
*
*
* @param updateMeshRequest
* @return A Java Future containing the result of the UpdateMesh operation returned by the service.
* @sample AWSAppMeshAsync.UpdateMesh
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateMeshAsync(UpdateMeshRequest updateMeshRequest);
/**
*
* Updates an existing service mesh.
*
*
* @param updateMeshRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateMesh operation returned by the service.
* @sample AWSAppMeshAsyncHandler.UpdateMesh
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateMeshAsync(UpdateMeshRequest updateMeshRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing route for a specified service mesh and virtual router.
*
*
* @param updateRouteRequest
* @return A Java Future containing the result of the UpdateRoute operation returned by the service.
* @sample AWSAppMeshAsync.UpdateRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateRouteAsync(UpdateRouteRequest updateRouteRequest);
/**
*
* Updates an existing route for a specified service mesh and virtual router.
*
*
* @param updateRouteRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateRoute operation returned by the service.
* @sample AWSAppMeshAsyncHandler.UpdateRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateRouteAsync(UpdateRouteRequest updateRouteRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing virtual gateway in a specified service mesh.
*
*
* @param updateVirtualGatewayRequest
* @return A Java Future containing the result of the UpdateVirtualGateway operation returned by the service.
* @sample AWSAppMeshAsync.UpdateVirtualGateway
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateVirtualGatewayAsync(UpdateVirtualGatewayRequest updateVirtualGatewayRequest);
/**
*
* Updates an existing virtual gateway in a specified service mesh.
*
*
* @param updateVirtualGatewayRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateVirtualGateway operation returned by the service.
* @sample AWSAppMeshAsyncHandler.UpdateVirtualGateway
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateVirtualGatewayAsync(UpdateVirtualGatewayRequest updateVirtualGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing virtual node in a specified service mesh.
*
*
* @param updateVirtualNodeRequest
* @return A Java Future containing the result of the UpdateVirtualNode operation returned by the service.
* @sample AWSAppMeshAsync.UpdateVirtualNode
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateVirtualNodeAsync(UpdateVirtualNodeRequest updateVirtualNodeRequest);
/**
*
* Updates an existing virtual node in a specified service mesh.
*
*
* @param updateVirtualNodeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateVirtualNode operation returned by the service.
* @sample AWSAppMeshAsyncHandler.UpdateVirtualNode
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateVirtualNodeAsync(UpdateVirtualNodeRequest updateVirtualNodeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing virtual router in a specified service mesh.
*
*
* @param updateVirtualRouterRequest
* @return A Java Future containing the result of the UpdateVirtualRouter operation returned by the service.
* @sample AWSAppMeshAsync.UpdateVirtualRouter
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateVirtualRouterAsync(UpdateVirtualRouterRequest updateVirtualRouterRequest);
/**
*
* Updates an existing virtual router in a specified service mesh.
*
*
* @param updateVirtualRouterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateVirtualRouter operation returned by the service.
* @sample AWSAppMeshAsyncHandler.UpdateVirtualRouter
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateVirtualRouterAsync(UpdateVirtualRouterRequest updateVirtualRouterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing virtual service in a specified service mesh.
*
*
* @param updateVirtualServiceRequest
* @return A Java Future containing the result of the UpdateVirtualService operation returned by the service.
* @sample AWSAppMeshAsync.UpdateVirtualService
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateVirtualServiceAsync(UpdateVirtualServiceRequest updateVirtualServiceRequest);
/**
*
* Updates an existing virtual service in a specified service mesh.
*
*
* @param updateVirtualServiceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateVirtualService operation returned by the service.
* @sample AWSAppMeshAsyncHandler.UpdateVirtualService
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateVirtualServiceAsync(UpdateVirtualServiceRequest updateVirtualServiceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}