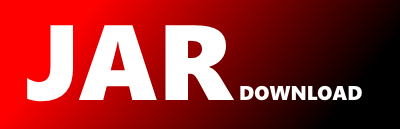
com.amazonaws.services.appregistry.AWSAppRegistry Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appregistry Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appregistry;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.appregistry.model.*;
/**
* Interface for accessing AppRegistry.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.appregistry.AbstractAWSAppRegistry} instead.
*
*
*
* Amazon Web Services Service Catalog AppRegistry enables organizations to understand the application context of their
* Amazon Web Services resources. AppRegistry provides a repository of your applications, their resources, and the
* application metadata that you use within your enterprise.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSAppRegistry {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "servicecatalog-appregistry";
/**
*
* Associates an attribute group with an application to augment the application's metadata with the group's
* attributes. This feature enables applications to be described with user-defined details that are
* machine-readable, such as third-party integrations.
*
*
* @param associateAttributeGroupRequest
* @return Result of the AssociateAttributeGroup operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws InternalServerException
* The service is experiencing internal problems.
* @throws ServiceQuotaExceededException
* The maximum number of resources per account has been reached.
* @throws ConflictException
* There was a conflict when processing the request (for example, a resource with the given name already
* exists within the account).
* @sample AWSAppRegistry.AssociateAttributeGroup
* @see AWS API Documentation
*/
AssociateAttributeGroupResult associateAttributeGroup(AssociateAttributeGroupRequest associateAttributeGroupRequest);
/**
*
* Associates a resource with an application. The resource can be specified by its ARN or name. The application can
* be specified by ARN, ID, or name.
*
*
* Minimum permissions
*
*
* You must have the following permissions to associate a resource using the OPTIONS
parameter set to
* APPLY_APPLICATION_TAG
.
*
*
* -
*
* tag:GetResources
*
*
* -
*
* tag:TagResources
*
*
*
*
* You must also have these additional permissions if you don't use the
* AWSServiceCatalogAppRegistryFullAccess
policy. For more information, see AWSServiceCatalogAppRegistryFullAccess in the AppRegistry Administrator Guide.
*
*
* -
*
* resource-groups:AssociateResource
*
*
* -
*
* cloudformation:UpdateStack
*
*
* -
*
* cloudformation:DescribeStacks
*
*
*
*
*
* In addition, you must have the tagging permission defined by the Amazon Web Services service that creates the
* resource. For more information, see TagResources in the Resource Groups Tagging API Reference.
*
*
*
* @param associateResourceRequest
* @return Result of the AssociateResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws InternalServerException
* The service is experiencing internal problems.
* @throws ServiceQuotaExceededException
* The maximum number of resources per account has been reached.
* @throws ConflictException
* There was a conflict when processing the request (for example, a resource with the given name already
* exists within the account).
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ThrottlingException
* The maximum number of API requests has been exceeded.
* @sample AWSAppRegistry.AssociateResource
* @see AWS API Documentation
*/
AssociateResourceResult associateResource(AssociateResourceRequest associateResourceRequest);
/**
*
* Creates a new application that is the top-level node in a hierarchy of related cloud resource abstractions.
*
*
* @param createApplicationRequest
* @return Result of the CreateApplication operation returned by the service.
* @throws ServiceQuotaExceededException
* The maximum number of resources per account has been reached.
* @throws ConflictException
* There was a conflict when processing the request (for example, a resource with the given name already
* exists within the account).
* @throws InternalServerException
* The service is experiencing internal problems.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ThrottlingException
* The maximum number of API requests has been exceeded.
* @sample AWSAppRegistry.CreateApplication
* @see AWS API Documentation
*/
CreateApplicationResult createApplication(CreateApplicationRequest createApplicationRequest);
/**
*
* Creates a new attribute group as a container for user-defined attributes. This feature enables users to have full
* control over their cloud application's metadata in a rich machine-readable format to facilitate integration with
* automated workflows and third-party tools.
*
*
* @param createAttributeGroupRequest
* @return Result of the CreateAttributeGroup operation returned by the service.
* @throws ServiceQuotaExceededException
* The maximum number of resources per account has been reached.
* @throws ConflictException
* There was a conflict when processing the request (for example, a resource with the given name already
* exists within the account).
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws InternalServerException
* The service is experiencing internal problems.
* @sample AWSAppRegistry.CreateAttributeGroup
* @see AWS API Documentation
*/
CreateAttributeGroupResult createAttributeGroup(CreateAttributeGroupRequest createAttributeGroupRequest);
/**
*
* Deletes an application that is specified either by its application ID, name, or ARN. All associated attribute
* groups and resources must be disassociated from it before deleting an application.
*
*
* @param deleteApplicationRequest
* @return Result of the DeleteApplication operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws InternalServerException
* The service is experiencing internal problems.
* @sample AWSAppRegistry.DeleteApplication
* @see AWS API Documentation
*/
DeleteApplicationResult deleteApplication(DeleteApplicationRequest deleteApplicationRequest);
/**
*
* Deletes an attribute group, specified either by its attribute group ID, name, or ARN.
*
*
* @param deleteAttributeGroupRequest
* @return Result of the DeleteAttributeGroup operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws InternalServerException
* The service is experiencing internal problems.
* @sample AWSAppRegistry.DeleteAttributeGroup
* @see AWS API Documentation
*/
DeleteAttributeGroupResult deleteAttributeGroup(DeleteAttributeGroupRequest deleteAttributeGroupRequest);
/**
*
* Disassociates an attribute group from an application to remove the extra attributes contained in the attribute
* group from the application's metadata. This operation reverts AssociateAttributeGroup
.
*
*
* @param disassociateAttributeGroupRequest
* @return Result of the DisassociateAttributeGroup operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws InternalServerException
* The service is experiencing internal problems.
* @sample AWSAppRegistry.DisassociateAttributeGroup
* @see AWS API Documentation
*/
DisassociateAttributeGroupResult disassociateAttributeGroup(DisassociateAttributeGroupRequest disassociateAttributeGroupRequest);
/**
*
* Disassociates a resource from application. Both the resource and the application can be specified either by ID or
* name.
*
*
* Minimum permissions
*
*
* You must have the following permissions to remove a resource that's been associated with an application using the
* APPLY_APPLICATION_TAG
option for AssociateResource.
*
*
* -
*
* tag:GetResources
*
*
* -
*
* tag:UntagResources
*
*
*
*
* You must also have the following permissions if you don't use the
* AWSServiceCatalogAppRegistryFullAccess
policy. For more information, see AWSServiceCatalogAppRegistryFullAccess in the AppRegistry Administrator Guide.
*
*
* -
*
* resource-groups:DisassociateResource
*
*
* -
*
* cloudformation:UpdateStack
*
*
* -
*
* cloudformation:DescribeStacks
*
*
*
*
*
* In addition, you must have the tagging permission defined by the Amazon Web Services service that creates the
* resource. For more information, see UntagResources in the Resource Groups Tagging API Reference.
*
*
*
* @param disassociateResourceRequest
* @return Result of the DisassociateResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws InternalServerException
* The service is experiencing internal problems.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ThrottlingException
* The maximum number of API requests has been exceeded.
* @sample AWSAppRegistry.DisassociateResource
* @see AWS API Documentation
*/
DisassociateResourceResult disassociateResource(DisassociateResourceRequest disassociateResourceRequest);
/**
*
* Retrieves metadata information about one of your applications. The application can be specified by its ARN, ID,
* or name (which is unique within one account in one region at a given point in time). Specify by ARN or ID in
* automated workflows if you want to make sure that the exact same application is returned or a
* ResourceNotFoundException
is thrown, avoiding the ABA addressing problem.
*
*
* @param getApplicationRequest
* @return Result of the GetApplication operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws InternalServerException
* The service is experiencing internal problems.
* @throws ConflictException
* There was a conflict when processing the request (for example, a resource with the given name already
* exists within the account).
* @sample AWSAppRegistry.GetApplication
* @see AWS API Documentation
*/
GetApplicationResult getApplication(GetApplicationRequest getApplicationRequest);
/**
*
* Gets the resource associated with the application.
*
*
* @param getAssociatedResourceRequest
* @return Result of the GetAssociatedResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws InternalServerException
* The service is experiencing internal problems.
* @sample AWSAppRegistry.GetAssociatedResource
* @see AWS API Documentation
*/
GetAssociatedResourceResult getAssociatedResource(GetAssociatedResourceRequest getAssociatedResourceRequest);
/**
*
* Retrieves an attribute group by its ARN, ID, or name. The attribute group can be specified by its ARN, ID, or
* name.
*
*
* @param getAttributeGroupRequest
* @return Result of the GetAttributeGroup operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws InternalServerException
* The service is experiencing internal problems.
* @throws ConflictException
* There was a conflict when processing the request (for example, a resource with the given name already
* exists within the account).
* @sample AWSAppRegistry.GetAttributeGroup
* @see AWS API Documentation
*/
GetAttributeGroupResult getAttributeGroup(GetAttributeGroupRequest getAttributeGroupRequest);
/**
*
* Retrieves a TagKey
configuration from an account.
*
*
* @param getConfigurationRequest
* @return Result of the GetConfiguration operation returned by the service.
* @throws InternalServerException
* The service is experiencing internal problems.
* @sample AWSAppRegistry.GetConfiguration
* @see AWS API Documentation
*/
GetConfigurationResult getConfiguration(GetConfigurationRequest getConfigurationRequest);
/**
*
* Retrieves a list of all of your applications. Results are paginated.
*
*
* @param listApplicationsRequest
* @return Result of the ListApplications operation returned by the service.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws InternalServerException
* The service is experiencing internal problems.
* @sample AWSAppRegistry.ListApplications
* @see AWS API Documentation
*/
ListApplicationsResult listApplications(ListApplicationsRequest listApplicationsRequest);
/**
*
* Lists all attribute groups that are associated with specified application. Results are paginated.
*
*
* @param listAssociatedAttributeGroupsRequest
* @return Result of the ListAssociatedAttributeGroups operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws InternalServerException
* The service is experiencing internal problems.
* @sample AWSAppRegistry.ListAssociatedAttributeGroups
* @see AWS API Documentation
*/
ListAssociatedAttributeGroupsResult listAssociatedAttributeGroups(ListAssociatedAttributeGroupsRequest listAssociatedAttributeGroupsRequest);
/**
*
* Lists all of the resources that are associated with the specified application. Results are paginated.
*
*
*
* If you share an application, and a consumer account associates a tag query to the application, all of the users
* who can access the application can also view the tag values in all accounts that are associated with it using
* this API.
*
*
*
* @param listAssociatedResourcesRequest
* @return Result of the ListAssociatedResources operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws InternalServerException
* The service is experiencing internal problems.
* @sample AWSAppRegistry.ListAssociatedResources
* @see AWS API Documentation
*/
ListAssociatedResourcesResult listAssociatedResources(ListAssociatedResourcesRequest listAssociatedResourcesRequest);
/**
*
* Lists all attribute groups which you have access to. Results are paginated.
*
*
* @param listAttributeGroupsRequest
* @return Result of the ListAttributeGroups operation returned by the service.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws InternalServerException
* The service is experiencing internal problems.
* @sample AWSAppRegistry.ListAttributeGroups
* @see AWS API Documentation
*/
ListAttributeGroupsResult listAttributeGroups(ListAttributeGroupsRequest listAttributeGroupsRequest);
/**
*
* Lists the details of all attribute groups associated with a specific application. The results display in pages.
*
*
* @param listAttributeGroupsForApplicationRequest
* @return Result of the ListAttributeGroupsForApplication operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws InternalServerException
* The service is experiencing internal problems.
* @sample AWSAppRegistry.ListAttributeGroupsForApplication
* @see AWS API Documentation
*/
ListAttributeGroupsForApplicationResult listAttributeGroupsForApplication(ListAttributeGroupsForApplicationRequest listAttributeGroupsForApplicationRequest);
/**
*
* Lists all of the tags on the resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws InternalServerException
* The service is experiencing internal problems.
* @sample AWSAppRegistry.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Associates a TagKey
configuration to an account.
*
*
* @param putConfigurationRequest
* @return Result of the PutConfiguration operation returned by the service.
* @throws ConflictException
* There was a conflict when processing the request (for example, a resource with the given name already
* exists within the account).
* @throws InternalServerException
* The service is experiencing internal problems.
* @throws ValidationException
* The request has invalid or missing parameters.
* @sample AWSAppRegistry.PutConfiguration
* @see AWS API Documentation
*/
PutConfigurationResult putConfiguration(PutConfigurationRequest putConfigurationRequest);
/**
*
* Syncs the resource with current AppRegistry records.
*
*
* Specifically, the resource’s AppRegistry system tags sync with its associated application. We remove the
* resource's AppRegistry system tags if it does not associate with the application. The caller must have
* permissions to read and update the resource.
*
*
* @param syncResourceRequest
* @return Result of the SyncResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws InternalServerException
* The service is experiencing internal problems.
* @throws ConflictException
* There was a conflict when processing the request (for example, a resource with the given name already
* exists within the account).
* @throws ThrottlingException
* The maximum number of API requests has been exceeded.
* @throws ValidationException
* The request has invalid or missing parameters.
* @sample AWSAppRegistry.SyncResource
* @see AWS
* API Documentation
*/
SyncResourceResult syncResource(SyncResourceRequest syncResourceRequest);
/**
*
* Assigns one or more tags (key-value pairs) to the specified resource.
*
*
* Each tag consists of a key and an optional value. If a tag with the same key is already associated with the
* resource, this action updates its value.
*
*
* This operation returns an empty response if the call was successful.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws InternalServerException
* The service is experiencing internal problems.
* @sample AWSAppRegistry.TagResource
* @see AWS
* API Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes tags from a resource.
*
*
* This operation returns an empty response if the call was successful.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws InternalServerException
* The service is experiencing internal problems.
* @sample AWSAppRegistry.UntagResource
* @see AWS API Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates an existing application with new attributes.
*
*
* @param updateApplicationRequest
* @return Result of the UpdateApplication operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws ConflictException
* There was a conflict when processing the request (for example, a resource with the given name already
* exists within the account).
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws InternalServerException
* The service is experiencing internal problems.
* @throws ThrottlingException
* The maximum number of API requests has been exceeded.
* @sample AWSAppRegistry.UpdateApplication
* @see AWS API Documentation
*/
UpdateApplicationResult updateApplication(UpdateApplicationRequest updateApplicationRequest);
/**
*
* Updates an existing attribute group with new details.
*
*
* @param updateAttributeGroupRequest
* @return Result of the UpdateAttributeGroup operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ConflictException
* There was a conflict when processing the request (for example, a resource with the given name already
* exists within the account).
* @throws InternalServerException
* The service is experiencing internal problems.
* @sample AWSAppRegistry.UpdateAttributeGroup
* @see AWS API Documentation
*/
UpdateAttributeGroupResult updateAttributeGroup(UpdateAttributeGroupRequest updateAttributeGroupRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}