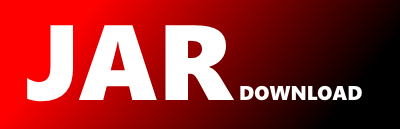
com.amazonaws.services.appregistry.AWSAppRegistryAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appregistry Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appregistry;
import javax.annotation.Generated;
import com.amazonaws.services.appregistry.model.*;
/**
* Interface for accessing AppRegistry asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.appregistry.AbstractAWSAppRegistryAsync} instead.
*
*
*
* Amazon Web Services Service Catalog AppRegistry enables organizations to understand the application context of their
* Amazon Web Services resources. AppRegistry provides a repository of your applications, their resources, and the
* application metadata that you use within your enterprise.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSAppRegistryAsync extends AWSAppRegistry {
/**
*
* Associates an attribute group with an application to augment the application's metadata with the group's
* attributes. This feature enables applications to be described with user-defined details that are
* machine-readable, such as third-party integrations.
*
*
* @param associateAttributeGroupRequest
* @return A Java Future containing the result of the AssociateAttributeGroup operation returned by the service.
* @sample AWSAppRegistryAsync.AssociateAttributeGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future associateAttributeGroupAsync(AssociateAttributeGroupRequest associateAttributeGroupRequest);
/**
*
* Associates an attribute group with an application to augment the application's metadata with the group's
* attributes. This feature enables applications to be described with user-defined details that are
* machine-readable, such as third-party integrations.
*
*
* @param associateAttributeGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateAttributeGroup operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.AssociateAttributeGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future associateAttributeGroupAsync(AssociateAttributeGroupRequest associateAttributeGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a resource with an application. The resource can be specified by its ARN or name. The application can
* be specified by ARN, ID, or name.
*
*
* Minimum permissions
*
*
* You must have the following permissions to associate a resource using the OPTIONS
parameter set to
* APPLY_APPLICATION_TAG
.
*
*
* -
*
* tag:GetResources
*
*
* -
*
* tag:TagResources
*
*
*
*
* You must also have these additional permissions if you don't use the
* AWSServiceCatalogAppRegistryFullAccess
policy. For more information, see AWSServiceCatalogAppRegistryFullAccess in the AppRegistry Administrator Guide.
*
*
* -
*
* resource-groups:AssociateResource
*
*
* -
*
* cloudformation:UpdateStack
*
*
* -
*
* cloudformation:DescribeStacks
*
*
*
*
*
* In addition, you must have the tagging permission defined by the Amazon Web Services service that creates the
* resource. For more information, see TagResources in the Resource Groups Tagging API Reference.
*
*
*
* @param associateResourceRequest
* @return A Java Future containing the result of the AssociateResource operation returned by the service.
* @sample AWSAppRegistryAsync.AssociateResource
* @see AWS API Documentation
*/
java.util.concurrent.Future associateResourceAsync(AssociateResourceRequest associateResourceRequest);
/**
*
* Associates a resource with an application. The resource can be specified by its ARN or name. The application can
* be specified by ARN, ID, or name.
*
*
* Minimum permissions
*
*
* You must have the following permissions to associate a resource using the OPTIONS
parameter set to
* APPLY_APPLICATION_TAG
.
*
*
* -
*
* tag:GetResources
*
*
* -
*
* tag:TagResources
*
*
*
*
* You must also have these additional permissions if you don't use the
* AWSServiceCatalogAppRegistryFullAccess
policy. For more information, see AWSServiceCatalogAppRegistryFullAccess in the AppRegistry Administrator Guide.
*
*
* -
*
* resource-groups:AssociateResource
*
*
* -
*
* cloudformation:UpdateStack
*
*
* -
*
* cloudformation:DescribeStacks
*
*
*
*
*
* In addition, you must have the tagging permission defined by the Amazon Web Services service that creates the
* resource. For more information, see TagResources in the Resource Groups Tagging API Reference.
*
*
*
* @param associateResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateResource operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.AssociateResource
* @see AWS API Documentation
*/
java.util.concurrent.Future associateResourceAsync(AssociateResourceRequest associateResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new application that is the top-level node in a hierarchy of related cloud resource abstractions.
*
*
* @param createApplicationRequest
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* @sample AWSAppRegistryAsync.CreateApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future createApplicationAsync(CreateApplicationRequest createApplicationRequest);
/**
*
* Creates a new application that is the top-level node in a hierarchy of related cloud resource abstractions.
*
*
* @param createApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.CreateApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future createApplicationAsync(CreateApplicationRequest createApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new attribute group as a container for user-defined attributes. This feature enables users to have full
* control over their cloud application's metadata in a rich machine-readable format to facilitate integration with
* automated workflows and third-party tools.
*
*
* @param createAttributeGroupRequest
* @return A Java Future containing the result of the CreateAttributeGroup operation returned by the service.
* @sample AWSAppRegistryAsync.CreateAttributeGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createAttributeGroupAsync(CreateAttributeGroupRequest createAttributeGroupRequest);
/**
*
* Creates a new attribute group as a container for user-defined attributes. This feature enables users to have full
* control over their cloud application's metadata in a rich machine-readable format to facilitate integration with
* automated workflows and third-party tools.
*
*
* @param createAttributeGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAttributeGroup operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.CreateAttributeGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createAttributeGroupAsync(CreateAttributeGroupRequest createAttributeGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an application that is specified either by its application ID, name, or ARN. All associated attribute
* groups and resources must be disassociated from it before deleting an application.
*
*
* @param deleteApplicationRequest
* @return A Java Future containing the result of the DeleteApplication operation returned by the service.
* @sample AWSAppRegistryAsync.DeleteApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteApplicationAsync(DeleteApplicationRequest deleteApplicationRequest);
/**
*
* Deletes an application that is specified either by its application ID, name, or ARN. All associated attribute
* groups and resources must be disassociated from it before deleting an application.
*
*
* @param deleteApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteApplication operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.DeleteApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteApplicationAsync(DeleteApplicationRequest deleteApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an attribute group, specified either by its attribute group ID, name, or ARN.
*
*
* @param deleteAttributeGroupRequest
* @return A Java Future containing the result of the DeleteAttributeGroup operation returned by the service.
* @sample AWSAppRegistryAsync.DeleteAttributeGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAttributeGroupAsync(DeleteAttributeGroupRequest deleteAttributeGroupRequest);
/**
*
* Deletes an attribute group, specified either by its attribute group ID, name, or ARN.
*
*
* @param deleteAttributeGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAttributeGroup operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.DeleteAttributeGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAttributeGroupAsync(DeleteAttributeGroupRequest deleteAttributeGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates an attribute group from an application to remove the extra attributes contained in the attribute
* group from the application's metadata. This operation reverts AssociateAttributeGroup
.
*
*
* @param disassociateAttributeGroupRequest
* @return A Java Future containing the result of the DisassociateAttributeGroup operation returned by the service.
* @sample AWSAppRegistryAsync.DisassociateAttributeGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateAttributeGroupAsync(
DisassociateAttributeGroupRequest disassociateAttributeGroupRequest);
/**
*
* Disassociates an attribute group from an application to remove the extra attributes contained in the attribute
* group from the application's metadata. This operation reverts AssociateAttributeGroup
.
*
*
* @param disassociateAttributeGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateAttributeGroup operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.DisassociateAttributeGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateAttributeGroupAsync(
DisassociateAttributeGroupRequest disassociateAttributeGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates a resource from application. Both the resource and the application can be specified either by ID or
* name.
*
*
* Minimum permissions
*
*
* You must have the following permissions to remove a resource that's been associated with an application using the
* APPLY_APPLICATION_TAG
option for AssociateResource.
*
*
* -
*
* tag:GetResources
*
*
* -
*
* tag:UntagResources
*
*
*
*
* You must also have the following permissions if you don't use the
* AWSServiceCatalogAppRegistryFullAccess
policy. For more information, see AWSServiceCatalogAppRegistryFullAccess in the AppRegistry Administrator Guide.
*
*
* -
*
* resource-groups:DisassociateResource
*
*
* -
*
* cloudformation:UpdateStack
*
*
* -
*
* cloudformation:DescribeStacks
*
*
*
*
*
* In addition, you must have the tagging permission defined by the Amazon Web Services service that creates the
* resource. For more information, see UntagResources in the Resource Groups Tagging API Reference.
*
*
*
* @param disassociateResourceRequest
* @return A Java Future containing the result of the DisassociateResource operation returned by the service.
* @sample AWSAppRegistryAsync.DisassociateResource
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateResourceAsync(DisassociateResourceRequest disassociateResourceRequest);
/**
*
* Disassociates a resource from application. Both the resource and the application can be specified either by ID or
* name.
*
*
* Minimum permissions
*
*
* You must have the following permissions to remove a resource that's been associated with an application using the
* APPLY_APPLICATION_TAG
option for AssociateResource.
*
*
* -
*
* tag:GetResources
*
*
* -
*
* tag:UntagResources
*
*
*
*
* You must also have the following permissions if you don't use the
* AWSServiceCatalogAppRegistryFullAccess
policy. For more information, see AWSServiceCatalogAppRegistryFullAccess in the AppRegistry Administrator Guide.
*
*
* -
*
* resource-groups:DisassociateResource
*
*
* -
*
* cloudformation:UpdateStack
*
*
* -
*
* cloudformation:DescribeStacks
*
*
*
*
*
* In addition, you must have the tagging permission defined by the Amazon Web Services service that creates the
* resource. For more information, see UntagResources in the Resource Groups Tagging API Reference.
*
*
*
* @param disassociateResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateResource operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.DisassociateResource
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateResourceAsync(DisassociateResourceRequest disassociateResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves metadata information about one of your applications. The application can be specified by its ARN, ID,
* or name (which is unique within one account in one region at a given point in time). Specify by ARN or ID in
* automated workflows if you want to make sure that the exact same application is returned or a
* ResourceNotFoundException
is thrown, avoiding the ABA addressing problem.
*
*
* @param getApplicationRequest
* @return A Java Future containing the result of the GetApplication operation returned by the service.
* @sample AWSAppRegistryAsync.GetApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future getApplicationAsync(GetApplicationRequest getApplicationRequest);
/**
*
* Retrieves metadata information about one of your applications. The application can be specified by its ARN, ID,
* or name (which is unique within one account in one region at a given point in time). Specify by ARN or ID in
* automated workflows if you want to make sure that the exact same application is returned or a
* ResourceNotFoundException
is thrown, avoiding the ABA addressing problem.
*
*
* @param getApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetApplication operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.GetApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future getApplicationAsync(GetApplicationRequest getApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the resource associated with the application.
*
*
* @param getAssociatedResourceRequest
* @return A Java Future containing the result of the GetAssociatedResource operation returned by the service.
* @sample AWSAppRegistryAsync.GetAssociatedResource
* @see AWS API Documentation
*/
java.util.concurrent.Future getAssociatedResourceAsync(GetAssociatedResourceRequest getAssociatedResourceRequest);
/**
*
* Gets the resource associated with the application.
*
*
* @param getAssociatedResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAssociatedResource operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.GetAssociatedResource
* @see AWS API Documentation
*/
java.util.concurrent.Future getAssociatedResourceAsync(GetAssociatedResourceRequest getAssociatedResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves an attribute group by its ARN, ID, or name. The attribute group can be specified by its ARN, ID, or
* name.
*
*
* @param getAttributeGroupRequest
* @return A Java Future containing the result of the GetAttributeGroup operation returned by the service.
* @sample AWSAppRegistryAsync.GetAttributeGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future getAttributeGroupAsync(GetAttributeGroupRequest getAttributeGroupRequest);
/**
*
* Retrieves an attribute group by its ARN, ID, or name. The attribute group can be specified by its ARN, ID, or
* name.
*
*
* @param getAttributeGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAttributeGroup operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.GetAttributeGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future getAttributeGroupAsync(GetAttributeGroupRequest getAttributeGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a TagKey
configuration from an account.
*
*
* @param getConfigurationRequest
* @return A Java Future containing the result of the GetConfiguration operation returned by the service.
* @sample AWSAppRegistryAsync.GetConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getConfigurationAsync(GetConfigurationRequest getConfigurationRequest);
/**
*
* Retrieves a TagKey
configuration from an account.
*
*
* @param getConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetConfiguration operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.GetConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getConfigurationAsync(GetConfigurationRequest getConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of all of your applications. Results are paginated.
*
*
* @param listApplicationsRequest
* @return A Java Future containing the result of the ListApplications operation returned by the service.
* @sample AWSAppRegistryAsync.ListApplications
* @see AWS API Documentation
*/
java.util.concurrent.Future listApplicationsAsync(ListApplicationsRequest listApplicationsRequest);
/**
*
* Retrieves a list of all of your applications. Results are paginated.
*
*
* @param listApplicationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListApplications operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.ListApplications
* @see AWS API Documentation
*/
java.util.concurrent.Future listApplicationsAsync(ListApplicationsRequest listApplicationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all attribute groups that are associated with specified application. Results are paginated.
*
*
* @param listAssociatedAttributeGroupsRequest
* @return A Java Future containing the result of the ListAssociatedAttributeGroups operation returned by the
* service.
* @sample AWSAppRegistryAsync.ListAssociatedAttributeGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future listAssociatedAttributeGroupsAsync(
ListAssociatedAttributeGroupsRequest listAssociatedAttributeGroupsRequest);
/**
*
* Lists all attribute groups that are associated with specified application. Results are paginated.
*
*
* @param listAssociatedAttributeGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAssociatedAttributeGroups operation returned by the
* service.
* @sample AWSAppRegistryAsyncHandler.ListAssociatedAttributeGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future listAssociatedAttributeGroupsAsync(
ListAssociatedAttributeGroupsRequest listAssociatedAttributeGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all of the resources that are associated with the specified application. Results are paginated.
*
*
*
* If you share an application, and a consumer account associates a tag query to the application, all of the users
* who can access the application can also view the tag values in all accounts that are associated with it using
* this API.
*
*
*
* @param listAssociatedResourcesRequest
* @return A Java Future containing the result of the ListAssociatedResources operation returned by the service.
* @sample AWSAppRegistryAsync.ListAssociatedResources
* @see AWS API Documentation
*/
java.util.concurrent.Future listAssociatedResourcesAsync(ListAssociatedResourcesRequest listAssociatedResourcesRequest);
/**
*
* Lists all of the resources that are associated with the specified application. Results are paginated.
*
*
*
* If you share an application, and a consumer account associates a tag query to the application, all of the users
* who can access the application can also view the tag values in all accounts that are associated with it using
* this API.
*
*
*
* @param listAssociatedResourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAssociatedResources operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.ListAssociatedResources
* @see AWS API Documentation
*/
java.util.concurrent.Future listAssociatedResourcesAsync(ListAssociatedResourcesRequest listAssociatedResourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all attribute groups which you have access to. Results are paginated.
*
*
* @param listAttributeGroupsRequest
* @return A Java Future containing the result of the ListAttributeGroups operation returned by the service.
* @sample AWSAppRegistryAsync.ListAttributeGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future listAttributeGroupsAsync(ListAttributeGroupsRequest listAttributeGroupsRequest);
/**
*
* Lists all attribute groups which you have access to. Results are paginated.
*
*
* @param listAttributeGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAttributeGroups operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.ListAttributeGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future listAttributeGroupsAsync(ListAttributeGroupsRequest listAttributeGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the details of all attribute groups associated with a specific application. The results display in pages.
*
*
* @param listAttributeGroupsForApplicationRequest
* @return A Java Future containing the result of the ListAttributeGroupsForApplication operation returned by the
* service.
* @sample AWSAppRegistryAsync.ListAttributeGroupsForApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future listAttributeGroupsForApplicationAsync(
ListAttributeGroupsForApplicationRequest listAttributeGroupsForApplicationRequest);
/**
*
* Lists the details of all attribute groups associated with a specific application. The results display in pages.
*
*
* @param listAttributeGroupsForApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAttributeGroupsForApplication operation returned by the
* service.
* @sample AWSAppRegistryAsyncHandler.ListAttributeGroupsForApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future listAttributeGroupsForApplicationAsync(
ListAttributeGroupsForApplicationRequest listAttributeGroupsForApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all of the tags on the resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSAppRegistryAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists all of the tags on the resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a TagKey
configuration to an account.
*
*
* @param putConfigurationRequest
* @return A Java Future containing the result of the PutConfiguration operation returned by the service.
* @sample AWSAppRegistryAsync.PutConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future putConfigurationAsync(PutConfigurationRequest putConfigurationRequest);
/**
*
* Associates a TagKey
configuration to an account.
*
*
* @param putConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutConfiguration operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.PutConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future putConfigurationAsync(PutConfigurationRequest putConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Syncs the resource with current AppRegistry records.
*
*
* Specifically, the resource’s AppRegistry system tags sync with its associated application. We remove the
* resource's AppRegistry system tags if it does not associate with the application. The caller must have
* permissions to read and update the resource.
*
*
* @param syncResourceRequest
* @return A Java Future containing the result of the SyncResource operation returned by the service.
* @sample AWSAppRegistryAsync.SyncResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future syncResourceAsync(SyncResourceRequest syncResourceRequest);
/**
*
* Syncs the resource with current AppRegistry records.
*
*
* Specifically, the resource’s AppRegistry system tags sync with its associated application. We remove the
* resource's AppRegistry system tags if it does not associate with the application. The caller must have
* permissions to read and update the resource.
*
*
* @param syncResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SyncResource operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.SyncResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future syncResourceAsync(SyncResourceRequest syncResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns one or more tags (key-value pairs) to the specified resource.
*
*
* Each tag consists of a key and an optional value. If a tag with the same key is already associated with the
* resource, this action updates its value.
*
*
* This operation returns an empty response if the call was successful.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSAppRegistryAsync.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Assigns one or more tags (key-value pairs) to the specified resource.
*
*
* Each tag consists of a key and an optional value. If a tag with the same key is already associated with the
* resource, this action updates its value.
*
*
* This operation returns an empty response if the call was successful.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes tags from a resource.
*
*
* This operation returns an empty response if the call was successful.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSAppRegistryAsync.UntagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes tags from a resource.
*
*
* This operation returns an empty response if the call was successful.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.UntagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing application with new attributes.
*
*
* @param updateApplicationRequest
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* @sample AWSAppRegistryAsync.UpdateApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future updateApplicationAsync(UpdateApplicationRequest updateApplicationRequest);
/**
*
* Updates an existing application with new attributes.
*
*
* @param updateApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.UpdateApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future updateApplicationAsync(UpdateApplicationRequest updateApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing attribute group with new details.
*
*
* @param updateAttributeGroupRequest
* @return A Java Future containing the result of the UpdateAttributeGroup operation returned by the service.
* @sample AWSAppRegistryAsync.UpdateAttributeGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAttributeGroupAsync(UpdateAttributeGroupRequest updateAttributeGroupRequest);
/**
*
* Updates an existing attribute group with new details.
*
*
* @param updateAttributeGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAttributeGroup operation returned by the service.
* @sample AWSAppRegistryAsyncHandler.UpdateAttributeGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAttributeGroupAsync(UpdateAttributeGroupRequest updateAttributeGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}