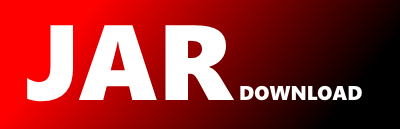
com.amazonaws.services.appregistry.model.GetAttributeGroupResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appregistry Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appregistry.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetAttributeGroupResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The identifier of the attribute group.
*
*/
private String id;
/**
*
* The Amazon resource name (ARN) that specifies the attribute group across services.
*
*/
private String arn;
/**
*
* The name of the attribute group.
*
*/
private String name;
/**
*
* The description of the attribute group that the user provides.
*
*/
private String description;
/**
*
* A JSON string in the form of nested key-value pairs that represent the attributes in the group and describes an
* application and its components.
*
*/
private String attributes;
/**
*
* The ISO-8601 formatted timestamp of the moment the attribute group was created.
*
*/
private java.util.Date creationTime;
/**
*
* The ISO-8601 formatted timestamp of the moment the attribute group was last updated. This time is the same as the
* creationTime for a newly created attribute group.
*
*/
private java.util.Date lastUpdateTime;
/**
*
* Key-value pairs associated with the attribute group.
*
*/
private java.util.Map tags;
/**
*
* The service principal that created the attribute group.
*
*/
private String createdBy;
/**
*
* The identifier of the attribute group.
*
*
* @param id
* The identifier of the attribute group.
*/
public void setId(String id) {
this.id = id;
}
/**
*
* The identifier of the attribute group.
*
*
* @return The identifier of the attribute group.
*/
public String getId() {
return this.id;
}
/**
*
* The identifier of the attribute group.
*
*
* @param id
* The identifier of the attribute group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetAttributeGroupResult withId(String id) {
setId(id);
return this;
}
/**
*
* The Amazon resource name (ARN) that specifies the attribute group across services.
*
*
* @param arn
* The Amazon resource name (ARN) that specifies the attribute group across services.
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
*
* The Amazon resource name (ARN) that specifies the attribute group across services.
*
*
* @return The Amazon resource name (ARN) that specifies the attribute group across services.
*/
public String getArn() {
return this.arn;
}
/**
*
* The Amazon resource name (ARN) that specifies the attribute group across services.
*
*
* @param arn
* The Amazon resource name (ARN) that specifies the attribute group across services.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetAttributeGroupResult withArn(String arn) {
setArn(arn);
return this;
}
/**
*
* The name of the attribute group.
*
*
* @param name
* The name of the attribute group.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the attribute group.
*
*
* @return The name of the attribute group.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the attribute group.
*
*
* @param name
* The name of the attribute group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetAttributeGroupResult withName(String name) {
setName(name);
return this;
}
/**
*
* The description of the attribute group that the user provides.
*
*
* @param description
* The description of the attribute group that the user provides.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The description of the attribute group that the user provides.
*
*
* @return The description of the attribute group that the user provides.
*/
public String getDescription() {
return this.description;
}
/**
*
* The description of the attribute group that the user provides.
*
*
* @param description
* The description of the attribute group that the user provides.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetAttributeGroupResult withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* A JSON string in the form of nested key-value pairs that represent the attributes in the group and describes an
* application and its components.
*
*
* @param attributes
* A JSON string in the form of nested key-value pairs that represent the attributes in the group and
* describes an application and its components.
*/
public void setAttributes(String attributes) {
this.attributes = attributes;
}
/**
*
* A JSON string in the form of nested key-value pairs that represent the attributes in the group and describes an
* application and its components.
*
*
* @return A JSON string in the form of nested key-value pairs that represent the attributes in the group and
* describes an application and its components.
*/
public String getAttributes() {
return this.attributes;
}
/**
*
* A JSON string in the form of nested key-value pairs that represent the attributes in the group and describes an
* application and its components.
*
*
* @param attributes
* A JSON string in the form of nested key-value pairs that represent the attributes in the group and
* describes an application and its components.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetAttributeGroupResult withAttributes(String attributes) {
setAttributes(attributes);
return this;
}
/**
*
* The ISO-8601 formatted timestamp of the moment the attribute group was created.
*
*
* @param creationTime
* The ISO-8601 formatted timestamp of the moment the attribute group was created.
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* The ISO-8601 formatted timestamp of the moment the attribute group was created.
*
*
* @return The ISO-8601 formatted timestamp of the moment the attribute group was created.
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* The ISO-8601 formatted timestamp of the moment the attribute group was created.
*
*
* @param creationTime
* The ISO-8601 formatted timestamp of the moment the attribute group was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetAttributeGroupResult withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* The ISO-8601 formatted timestamp of the moment the attribute group was last updated. This time is the same as the
* creationTime for a newly created attribute group.
*
*
* @param lastUpdateTime
* The ISO-8601 formatted timestamp of the moment the attribute group was last updated. This time is the same
* as the creationTime for a newly created attribute group.
*/
public void setLastUpdateTime(java.util.Date lastUpdateTime) {
this.lastUpdateTime = lastUpdateTime;
}
/**
*
* The ISO-8601 formatted timestamp of the moment the attribute group was last updated. This time is the same as the
* creationTime for a newly created attribute group.
*
*
* @return The ISO-8601 formatted timestamp of the moment the attribute group was last updated. This time is the
* same as the creationTime for a newly created attribute group.
*/
public java.util.Date getLastUpdateTime() {
return this.lastUpdateTime;
}
/**
*
* The ISO-8601 formatted timestamp of the moment the attribute group was last updated. This time is the same as the
* creationTime for a newly created attribute group.
*
*
* @param lastUpdateTime
* The ISO-8601 formatted timestamp of the moment the attribute group was last updated. This time is the same
* as the creationTime for a newly created attribute group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetAttributeGroupResult withLastUpdateTime(java.util.Date lastUpdateTime) {
setLastUpdateTime(lastUpdateTime);
return this;
}
/**
*
* Key-value pairs associated with the attribute group.
*
*
* @return Key-value pairs associated with the attribute group.
*/
public java.util.Map getTags() {
return tags;
}
/**
*
* Key-value pairs associated with the attribute group.
*
*
* @param tags
* Key-value pairs associated with the attribute group.
*/
public void setTags(java.util.Map tags) {
this.tags = tags;
}
/**
*
* Key-value pairs associated with the attribute group.
*
*
* @param tags
* Key-value pairs associated with the attribute group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetAttributeGroupResult withTags(java.util.Map tags) {
setTags(tags);
return this;
}
/**
* Add a single Tags entry
*
* @see GetAttributeGroupResult#withTags
* @returns a reference to this object so that method calls can be chained together.
*/
public GetAttributeGroupResult addTagsEntry(String key, String value) {
if (null == this.tags) {
this.tags = new java.util.HashMap();
}
if (this.tags.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.tags.put(key, value);
return this;
}
/**
* Removes all the entries added into Tags.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetAttributeGroupResult clearTagsEntries() {
this.tags = null;
return this;
}
/**
*
* The service principal that created the attribute group.
*
*
* @param createdBy
* The service principal that created the attribute group.
*/
public void setCreatedBy(String createdBy) {
this.createdBy = createdBy;
}
/**
*
* The service principal that created the attribute group.
*
*
* @return The service principal that created the attribute group.
*/
public String getCreatedBy() {
return this.createdBy;
}
/**
*
* The service principal that created the attribute group.
*
*
* @param createdBy
* The service principal that created the attribute group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetAttributeGroupResult withCreatedBy(String createdBy) {
setCreatedBy(createdBy);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getId() != null)
sb.append("Id: ").append(getId()).append(",");
if (getArn() != null)
sb.append("Arn: ").append(getArn()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getAttributes() != null)
sb.append("Attributes: ").append(getAttributes()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getLastUpdateTime() != null)
sb.append("LastUpdateTime: ").append(getLastUpdateTime()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getCreatedBy() != null)
sb.append("CreatedBy: ").append(getCreatedBy());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetAttributeGroupResult == false)
return false;
GetAttributeGroupResult other = (GetAttributeGroupResult) obj;
if (other.getId() == null ^ this.getId() == null)
return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false)
return false;
if (other.getArn() == null ^ this.getArn() == null)
return false;
if (other.getArn() != null && other.getArn().equals(this.getArn()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getAttributes() == null ^ this.getAttributes() == null)
return false;
if (other.getAttributes() != null && other.getAttributes().equals(this.getAttributes()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getLastUpdateTime() == null ^ this.getLastUpdateTime() == null)
return false;
if (other.getLastUpdateTime() != null && other.getLastUpdateTime().equals(this.getLastUpdateTime()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getCreatedBy() == null ^ this.getCreatedBy() == null)
return false;
if (other.getCreatedBy() != null && other.getCreatedBy().equals(this.getCreatedBy()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getArn() == null) ? 0 : getArn().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getAttributes() == null) ? 0 : getAttributes().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getLastUpdateTime() == null) ? 0 : getLastUpdateTime().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getCreatedBy() == null) ? 0 : getCreatedBy().hashCode());
return hashCode;
}
@Override
public GetAttributeGroupResult clone() {
try {
return (GetAttributeGroupResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}