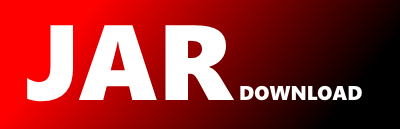
com.amazonaws.services.apprunner.AWSAppRunnerAsync Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.apprunner;
import javax.annotation.Generated;
import com.amazonaws.services.apprunner.model.*;
/**
* Interface for accessing AWS App Runner asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.apprunner.AbstractAWSAppRunnerAsync} instead.
*
*
* App Runner
*
* App Runner is an application service that provides a fast, simple, and cost-effective way to go directly from an
* existing container image or source code to a running service in the Amazon Web Services Cloud in seconds. You don't
* need to learn new technologies, decide which compute service to use, or understand how to provision and configure
* Amazon Web Services resources.
*
*
* App Runner connects directly to your container registry or source code repository. It provides an automatic delivery
* pipeline with fully managed operations, high performance, scalability, and security.
*
*
* For more information about App Runner, see the App Runner
* Developer Guide. For release information, see the App Runner Release Notes.
*
*
* To install the Software Development Kits (SDKs), Integrated Development Environment (IDE) Toolkits, and command line
* tools that you can use to access the API, see Tools for Amazon Web
* Services.
*
*
* Endpoints
*
*
* For a list of Region-specific endpoints that App Runner supports, see App Runner endpoints and quotas in the
* Amazon Web Services General Reference.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSAppRunnerAsync extends AWSAppRunner {
/**
*
* Associate your own domain name with the App Runner subdomain URL of your App Runner service.
*
*
* After you call AssociateCustomDomain
and receive a successful response, use the information in the
* CustomDomain record that's returned to add CNAME records to your Domain Name System (DNS). For each mapped
* domain name, add a mapping to the target App Runner subdomain and one or more certificate validation records. App
* Runner then performs DNS validation to verify that you own or control the domain name that you associated. App
* Runner tracks domain validity in a certificate stored in AWS Certificate Manager (ACM).
*
*
* @param associateCustomDomainRequest
* @return A Java Future containing the result of the AssociateCustomDomain operation returned by the service.
* @sample AWSAppRunnerAsync.AssociateCustomDomain
* @see AWS API Documentation
*/
java.util.concurrent.Future associateCustomDomainAsync(AssociateCustomDomainRequest associateCustomDomainRequest);
/**
*
* Associate your own domain name with the App Runner subdomain URL of your App Runner service.
*
*
* After you call AssociateCustomDomain
and receive a successful response, use the information in the
* CustomDomain record that's returned to add CNAME records to your Domain Name System (DNS). For each mapped
* domain name, add a mapping to the target App Runner subdomain and one or more certificate validation records. App
* Runner then performs DNS validation to verify that you own or control the domain name that you associated. App
* Runner tracks domain validity in a certificate stored in AWS Certificate Manager (ACM).
*
*
* @param associateCustomDomainRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateCustomDomain operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.AssociateCustomDomain
* @see AWS API Documentation
*/
java.util.concurrent.Future associateCustomDomainAsync(AssociateCustomDomainRequest associateCustomDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create an App Runner automatic scaling configuration resource. App Runner requires this resource when you create
* or update App Runner services and you require non-default auto scaling settings. You can share an auto scaling
* configuration across multiple services.
*
*
* Create multiple revisions of a configuration by calling this action multiple times using the same
* AutoScalingConfigurationName
. The call returns incremental
* AutoScalingConfigurationRevision
values. When you create a service and configure an auto scaling
* configuration resource, the service uses the latest active revision of the auto scaling configuration by default.
* You can optionally configure the service to use a specific revision.
*
*
* Configure a higher MinSize
to increase the spread of your App Runner service over more Availability
* Zones in the Amazon Web Services Region. The tradeoff is a higher minimal cost.
*
*
* Configure a lower MaxSize
to control your cost. The tradeoff is lower responsiveness during peak
* demand.
*
*
* @param createAutoScalingConfigurationRequest
* @return A Java Future containing the result of the CreateAutoScalingConfiguration operation returned by the
* service.
* @sample AWSAppRunnerAsync.CreateAutoScalingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createAutoScalingConfigurationAsync(
CreateAutoScalingConfigurationRequest createAutoScalingConfigurationRequest);
/**
*
* Create an App Runner automatic scaling configuration resource. App Runner requires this resource when you create
* or update App Runner services and you require non-default auto scaling settings. You can share an auto scaling
* configuration across multiple services.
*
*
* Create multiple revisions of a configuration by calling this action multiple times using the same
* AutoScalingConfigurationName
. The call returns incremental
* AutoScalingConfigurationRevision
values. When you create a service and configure an auto scaling
* configuration resource, the service uses the latest active revision of the auto scaling configuration by default.
* You can optionally configure the service to use a specific revision.
*
*
* Configure a higher MinSize
to increase the spread of your App Runner service over more Availability
* Zones in the Amazon Web Services Region. The tradeoff is a higher minimal cost.
*
*
* Configure a lower MaxSize
to control your cost. The tradeoff is lower responsiveness during peak
* demand.
*
*
* @param createAutoScalingConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAutoScalingConfiguration operation returned by the
* service.
* @sample AWSAppRunnerAsyncHandler.CreateAutoScalingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createAutoScalingConfigurationAsync(
CreateAutoScalingConfigurationRequest createAutoScalingConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create an App Runner connection resource. App Runner requires a connection resource when you create App Runner
* services that access private repositories from certain third-party providers. You can share a connection across
* multiple services.
*
*
* A connection resource is needed to access GitHub and Bitbucket repositories. Both require a user interface
* approval process through the App Runner console before you can use the connection.
*
*
* @param createConnectionRequest
* @return A Java Future containing the result of the CreateConnection operation returned by the service.
* @sample AWSAppRunnerAsync.CreateConnection
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createConnectionAsync(CreateConnectionRequest createConnectionRequest);
/**
*
* Create an App Runner connection resource. App Runner requires a connection resource when you create App Runner
* services that access private repositories from certain third-party providers. You can share a connection across
* multiple services.
*
*
* A connection resource is needed to access GitHub and Bitbucket repositories. Both require a user interface
* approval process through the App Runner console before you can use the connection.
*
*
* @param createConnectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateConnection operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.CreateConnection
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createConnectionAsync(CreateConnectionRequest createConnectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create an App Runner observability configuration resource. App Runner requires this resource when you create or
* update App Runner services and you want to enable non-default observability features. You can share an
* observability configuration across multiple services.
*
*
* Create multiple revisions of a configuration by calling this action multiple times using the same
* ObservabilityConfigurationName
. The call returns incremental
* ObservabilityConfigurationRevision
values. When you create a service and configure an observability
* configuration resource, the service uses the latest active revision of the observability configuration by
* default. You can optionally configure the service to use a specific revision.
*
*
* The observability configuration resource is designed to configure multiple features (currently one feature,
* tracing). This action takes optional parameters that describe the configuration of these features (currently one
* parameter, TraceConfiguration
). If you don't specify a feature parameter, App Runner doesn't enable
* the feature.
*
*
* @param createObservabilityConfigurationRequest
* @return A Java Future containing the result of the CreateObservabilityConfiguration operation returned by the
* service.
* @sample AWSAppRunnerAsync.CreateObservabilityConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createObservabilityConfigurationAsync(
CreateObservabilityConfigurationRequest createObservabilityConfigurationRequest);
/**
*
* Create an App Runner observability configuration resource. App Runner requires this resource when you create or
* update App Runner services and you want to enable non-default observability features. You can share an
* observability configuration across multiple services.
*
*
* Create multiple revisions of a configuration by calling this action multiple times using the same
* ObservabilityConfigurationName
. The call returns incremental
* ObservabilityConfigurationRevision
values. When you create a service and configure an observability
* configuration resource, the service uses the latest active revision of the observability configuration by
* default. You can optionally configure the service to use a specific revision.
*
*
* The observability configuration resource is designed to configure multiple features (currently one feature,
* tracing). This action takes optional parameters that describe the configuration of these features (currently one
* parameter, TraceConfiguration
). If you don't specify a feature parameter, App Runner doesn't enable
* the feature.
*
*
* @param createObservabilityConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateObservabilityConfiguration operation returned by the
* service.
* @sample AWSAppRunnerAsyncHandler.CreateObservabilityConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createObservabilityConfigurationAsync(
CreateObservabilityConfigurationRequest createObservabilityConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create an App Runner service. After the service is created, the action also automatically starts a deployment.
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to
* track the operation's progress.
*
*
* @param createServiceRequest
* @return A Java Future containing the result of the CreateService operation returned by the service.
* @sample AWSAppRunnerAsync.CreateService
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createServiceAsync(CreateServiceRequest createServiceRequest);
/**
*
* Create an App Runner service. After the service is created, the action also automatically starts a deployment.
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to
* track the operation's progress.
*
*
* @param createServiceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateService operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.CreateService
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createServiceAsync(CreateServiceRequest createServiceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create an App Runner VPC connector resource. App Runner requires this resource when you want to associate your
* App Runner service to a custom Amazon Virtual Private Cloud (Amazon VPC).
*
*
* @param createVpcConnectorRequest
* @return A Java Future containing the result of the CreateVpcConnector operation returned by the service.
* @sample AWSAppRunnerAsync.CreateVpcConnector
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createVpcConnectorAsync(CreateVpcConnectorRequest createVpcConnectorRequest);
/**
*
* Create an App Runner VPC connector resource. App Runner requires this resource when you want to associate your
* App Runner service to a custom Amazon Virtual Private Cloud (Amazon VPC).
*
*
* @param createVpcConnectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateVpcConnector operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.CreateVpcConnector
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createVpcConnectorAsync(CreateVpcConnectorRequest createVpcConnectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create an App Runner VPC Ingress Connection resource. App Runner requires this resource when you want to
* associate your App Runner service with an Amazon VPC endpoint.
*
*
* @param createVpcIngressConnectionRequest
* @return A Java Future containing the result of the CreateVpcIngressConnection operation returned by the service.
* @sample AWSAppRunnerAsync.CreateVpcIngressConnection
* @see AWS API Documentation
*/
java.util.concurrent.Future createVpcIngressConnectionAsync(
CreateVpcIngressConnectionRequest createVpcIngressConnectionRequest);
/**
*
* Create an App Runner VPC Ingress Connection resource. App Runner requires this resource when you want to
* associate your App Runner service with an Amazon VPC endpoint.
*
*
* @param createVpcIngressConnectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateVpcIngressConnection operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.CreateVpcIngressConnection
* @see AWS API Documentation
*/
java.util.concurrent.Future createVpcIngressConnectionAsync(
CreateVpcIngressConnectionRequest createVpcIngressConnectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete an App Runner automatic scaling configuration resource. You can delete a top level auto scaling
* configuration, a specific revision of one, or all revisions associated with the top level configuration. You
* can't delete the default auto scaling configuration or a configuration that's used by one or more App Runner
* services.
*
*
* @param deleteAutoScalingConfigurationRequest
* @return A Java Future containing the result of the DeleteAutoScalingConfiguration operation returned by the
* service.
* @sample AWSAppRunnerAsync.DeleteAutoScalingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAutoScalingConfigurationAsync(
DeleteAutoScalingConfigurationRequest deleteAutoScalingConfigurationRequest);
/**
*
* Delete an App Runner automatic scaling configuration resource. You can delete a top level auto scaling
* configuration, a specific revision of one, or all revisions associated with the top level configuration. You
* can't delete the default auto scaling configuration or a configuration that's used by one or more App Runner
* services.
*
*
* @param deleteAutoScalingConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAutoScalingConfiguration operation returned by the
* service.
* @sample AWSAppRunnerAsyncHandler.DeleteAutoScalingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAutoScalingConfigurationAsync(
DeleteAutoScalingConfigurationRequest deleteAutoScalingConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete an App Runner connection. You must first ensure that there are no running App Runner services that use
* this connection. If there are any, the DeleteConnection
action fails.
*
*
* @param deleteConnectionRequest
* @return A Java Future containing the result of the DeleteConnection operation returned by the service.
* @sample AWSAppRunnerAsync.DeleteConnection
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteConnectionAsync(DeleteConnectionRequest deleteConnectionRequest);
/**
*
* Delete an App Runner connection. You must first ensure that there are no running App Runner services that use
* this connection. If there are any, the DeleteConnection
action fails.
*
*
* @param deleteConnectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteConnection operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.DeleteConnection
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteConnectionAsync(DeleteConnectionRequest deleteConnectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete an App Runner observability configuration resource. You can delete a specific revision or the latest
* active revision. You can't delete a configuration that's used by one or more App Runner services.
*
*
* @param deleteObservabilityConfigurationRequest
* @return A Java Future containing the result of the DeleteObservabilityConfiguration operation returned by the
* service.
* @sample AWSAppRunnerAsync.DeleteObservabilityConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteObservabilityConfigurationAsync(
DeleteObservabilityConfigurationRequest deleteObservabilityConfigurationRequest);
/**
*
* Delete an App Runner observability configuration resource. You can delete a specific revision or the latest
* active revision. You can't delete a configuration that's used by one or more App Runner services.
*
*
* @param deleteObservabilityConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteObservabilityConfiguration operation returned by the
* service.
* @sample AWSAppRunnerAsyncHandler.DeleteObservabilityConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteObservabilityConfigurationAsync(
DeleteObservabilityConfigurationRequest deleteObservabilityConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete an App Runner service.
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to track the operation's progress.
*
*
*
* Make sure that you don't have any active VPCIngressConnections associated with the service you want to delete.
*
*
*
* @param deleteServiceRequest
* @return A Java Future containing the result of the DeleteService operation returned by the service.
* @sample AWSAppRunnerAsync.DeleteService
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteServiceAsync(DeleteServiceRequest deleteServiceRequest);
/**
*
* Delete an App Runner service.
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to track the operation's progress.
*
*
*
* Make sure that you don't have any active VPCIngressConnections associated with the service you want to delete.
*
*
*
* @param deleteServiceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteService operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.DeleteService
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteServiceAsync(DeleteServiceRequest deleteServiceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete an App Runner VPC connector resource. You can't delete a connector that's used by one or more App Runner
* services.
*
*
* @param deleteVpcConnectorRequest
* @return A Java Future containing the result of the DeleteVpcConnector operation returned by the service.
* @sample AWSAppRunnerAsync.DeleteVpcConnector
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteVpcConnectorAsync(DeleteVpcConnectorRequest deleteVpcConnectorRequest);
/**
*
* Delete an App Runner VPC connector resource. You can't delete a connector that's used by one or more App Runner
* services.
*
*
* @param deleteVpcConnectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVpcConnector operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.DeleteVpcConnector
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteVpcConnectorAsync(DeleteVpcConnectorRequest deleteVpcConnectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete an App Runner VPC Ingress Connection resource that's associated with an App Runner service. The VPC
* Ingress Connection must be in one of the following states to be deleted:
*
*
* -
*
* AVAILABLE
*
*
* -
*
* FAILED_CREATION
*
*
* -
*
* FAILED_UPDATE
*
*
* -
*
* FAILED_DELETION
*
*
*
*
* @param deleteVpcIngressConnectionRequest
* @return A Java Future containing the result of the DeleteVpcIngressConnection operation returned by the service.
* @sample AWSAppRunnerAsync.DeleteVpcIngressConnection
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteVpcIngressConnectionAsync(
DeleteVpcIngressConnectionRequest deleteVpcIngressConnectionRequest);
/**
*
* Delete an App Runner VPC Ingress Connection resource that's associated with an App Runner service. The VPC
* Ingress Connection must be in one of the following states to be deleted:
*
*
* -
*
* AVAILABLE
*
*
* -
*
* FAILED_CREATION
*
*
* -
*
* FAILED_UPDATE
*
*
* -
*
* FAILED_DELETION
*
*
*
*
* @param deleteVpcIngressConnectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVpcIngressConnection operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.DeleteVpcIngressConnection
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteVpcIngressConnectionAsync(
DeleteVpcIngressConnectionRequest deleteVpcIngressConnectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Return a full description of an App Runner automatic scaling configuration resource.
*
*
* @param describeAutoScalingConfigurationRequest
* @return A Java Future containing the result of the DescribeAutoScalingConfiguration operation returned by the
* service.
* @sample AWSAppRunnerAsync.DescribeAutoScalingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAutoScalingConfigurationAsync(
DescribeAutoScalingConfigurationRequest describeAutoScalingConfigurationRequest);
/**
*
* Return a full description of an App Runner automatic scaling configuration resource.
*
*
* @param describeAutoScalingConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAutoScalingConfiguration operation returned by the
* service.
* @sample AWSAppRunnerAsyncHandler.DescribeAutoScalingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAutoScalingConfigurationAsync(
DescribeAutoScalingConfigurationRequest describeAutoScalingConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Return a description of custom domain names that are associated with an App Runner service.
*
*
* @param describeCustomDomainsRequest
* @return A Java Future containing the result of the DescribeCustomDomains operation returned by the service.
* @sample AWSAppRunnerAsync.DescribeCustomDomains
* @see AWS API Documentation
*/
java.util.concurrent.Future describeCustomDomainsAsync(DescribeCustomDomainsRequest describeCustomDomainsRequest);
/**
*
* Return a description of custom domain names that are associated with an App Runner service.
*
*
* @param describeCustomDomainsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeCustomDomains operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.DescribeCustomDomains
* @see AWS API Documentation
*/
java.util.concurrent.Future describeCustomDomainsAsync(DescribeCustomDomainsRequest describeCustomDomainsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Return a full description of an App Runner observability configuration resource.
*
*
* @param describeObservabilityConfigurationRequest
* @return A Java Future containing the result of the DescribeObservabilityConfiguration operation returned by the
* service.
* @sample AWSAppRunnerAsync.DescribeObservabilityConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeObservabilityConfigurationAsync(
DescribeObservabilityConfigurationRequest describeObservabilityConfigurationRequest);
/**
*
* Return a full description of an App Runner observability configuration resource.
*
*
* @param describeObservabilityConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeObservabilityConfiguration operation returned by the
* service.
* @sample AWSAppRunnerAsyncHandler.DescribeObservabilityConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeObservabilityConfigurationAsync(
DescribeObservabilityConfigurationRequest describeObservabilityConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Return a full description of an App Runner service.
*
*
* @param describeServiceRequest
* @return A Java Future containing the result of the DescribeService operation returned by the service.
* @sample AWSAppRunnerAsync.DescribeService
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeServiceAsync(DescribeServiceRequest describeServiceRequest);
/**
*
* Return a full description of an App Runner service.
*
*
* @param describeServiceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeService operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.DescribeService
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeServiceAsync(DescribeServiceRequest describeServiceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Return a description of an App Runner VPC connector resource.
*
*
* @param describeVpcConnectorRequest
* @return A Java Future containing the result of the DescribeVpcConnector operation returned by the service.
* @sample AWSAppRunnerAsync.DescribeVpcConnector
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeVpcConnectorAsync(DescribeVpcConnectorRequest describeVpcConnectorRequest);
/**
*
* Return a description of an App Runner VPC connector resource.
*
*
* @param describeVpcConnectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeVpcConnector operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.DescribeVpcConnector
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeVpcConnectorAsync(DescribeVpcConnectorRequest describeVpcConnectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Return a full description of an App Runner VPC Ingress Connection resource.
*
*
* @param describeVpcIngressConnectionRequest
* @return A Java Future containing the result of the DescribeVpcIngressConnection operation returned by the
* service.
* @sample AWSAppRunnerAsync.DescribeVpcIngressConnection
* @see AWS API Documentation
*/
java.util.concurrent.Future describeVpcIngressConnectionAsync(
DescribeVpcIngressConnectionRequest describeVpcIngressConnectionRequest);
/**
*
* Return a full description of an App Runner VPC Ingress Connection resource.
*
*
* @param describeVpcIngressConnectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeVpcIngressConnection operation returned by the
* service.
* @sample AWSAppRunnerAsyncHandler.DescribeVpcIngressConnection
* @see AWS API Documentation
*/
java.util.concurrent.Future describeVpcIngressConnectionAsync(
DescribeVpcIngressConnectionRequest describeVpcIngressConnectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociate a custom domain name from an App Runner service.
*
*
* Certificates tracking domain validity are associated with a custom domain and are stored in AWS Certificate Manager (ACM). These certificates
* aren't deleted as part of this action. App Runner delays certificate deletion for 30 days after a domain is
* disassociated from your service.
*
*
* @param disassociateCustomDomainRequest
* @return A Java Future containing the result of the DisassociateCustomDomain operation returned by the service.
* @sample AWSAppRunnerAsync.DisassociateCustomDomain
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateCustomDomainAsync(DisassociateCustomDomainRequest disassociateCustomDomainRequest);
/**
*
* Disassociate a custom domain name from an App Runner service.
*
*
* Certificates tracking domain validity are associated with a custom domain and are stored in AWS Certificate Manager (ACM). These certificates
* aren't deleted as part of this action. App Runner delays certificate deletion for 30 days after a domain is
* disassociated from your service.
*
*
* @param disassociateCustomDomainRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateCustomDomain operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.DisassociateCustomDomain
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateCustomDomainAsync(DisassociateCustomDomainRequest disassociateCustomDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of active App Runner automatic scaling configurations in your Amazon Web Services account. You can
* query the revisions for a specific configuration name or the revisions for all active configurations in your
* account. You can optionally query only the latest revision of each requested name.
*
*
* To retrieve a full description of a particular configuration revision, call and provide one of the ARNs returned
* by ListAutoScalingConfigurations
.
*
*
* @param listAutoScalingConfigurationsRequest
* @return A Java Future containing the result of the ListAutoScalingConfigurations operation returned by the
* service.
* @sample AWSAppRunnerAsync.ListAutoScalingConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listAutoScalingConfigurationsAsync(
ListAutoScalingConfigurationsRequest listAutoScalingConfigurationsRequest);
/**
*
* Returns a list of active App Runner automatic scaling configurations in your Amazon Web Services account. You can
* query the revisions for a specific configuration name or the revisions for all active configurations in your
* account. You can optionally query only the latest revision of each requested name.
*
*
* To retrieve a full description of a particular configuration revision, call and provide one of the ARNs returned
* by ListAutoScalingConfigurations
.
*
*
* @param listAutoScalingConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAutoScalingConfigurations operation returned by the
* service.
* @sample AWSAppRunnerAsyncHandler.ListAutoScalingConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listAutoScalingConfigurationsAsync(
ListAutoScalingConfigurationsRequest listAutoScalingConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of App Runner connections that are associated with your Amazon Web Services account.
*
*
* @param listConnectionsRequest
* @return A Java Future containing the result of the ListConnections operation returned by the service.
* @sample AWSAppRunnerAsync.ListConnections
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listConnectionsAsync(ListConnectionsRequest listConnectionsRequest);
/**
*
* Returns a list of App Runner connections that are associated with your Amazon Web Services account.
*
*
* @param listConnectionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListConnections operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.ListConnections
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listConnectionsAsync(ListConnectionsRequest listConnectionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of active App Runner observability configurations in your Amazon Web Services account. You can
* query the revisions for a specific configuration name or the revisions for all active configurations in your
* account. You can optionally query only the latest revision of each requested name.
*
*
* To retrieve a full description of a particular configuration revision, call and provide one of the ARNs returned
* by ListObservabilityConfigurations
.
*
*
* @param listObservabilityConfigurationsRequest
* @return A Java Future containing the result of the ListObservabilityConfigurations operation returned by the
* service.
* @sample AWSAppRunnerAsync.ListObservabilityConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listObservabilityConfigurationsAsync(
ListObservabilityConfigurationsRequest listObservabilityConfigurationsRequest);
/**
*
* Returns a list of active App Runner observability configurations in your Amazon Web Services account. You can
* query the revisions for a specific configuration name or the revisions for all active configurations in your
* account. You can optionally query only the latest revision of each requested name.
*
*
* To retrieve a full description of a particular configuration revision, call and provide one of the ARNs returned
* by ListObservabilityConfigurations
.
*
*
* @param listObservabilityConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListObservabilityConfigurations operation returned by the
* service.
* @sample AWSAppRunnerAsyncHandler.ListObservabilityConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listObservabilityConfigurationsAsync(
ListObservabilityConfigurationsRequest listObservabilityConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Return a list of operations that occurred on an App Runner service.
*
*
* The resulting list of OperationSummary objects is sorted in reverse chronological order. The first object
* on the list represents the last started operation.
*
*
* @param listOperationsRequest
* @return A Java Future containing the result of the ListOperations operation returned by the service.
* @sample AWSAppRunnerAsync.ListOperations
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listOperationsAsync(ListOperationsRequest listOperationsRequest);
/**
*
* Return a list of operations that occurred on an App Runner service.
*
*
* The resulting list of OperationSummary objects is sorted in reverse chronological order. The first object
* on the list represents the last started operation.
*
*
* @param listOperationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListOperations operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.ListOperations
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listOperationsAsync(ListOperationsRequest listOperationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of running App Runner services in your Amazon Web Services account.
*
*
* @param listServicesRequest
* @return A Java Future containing the result of the ListServices operation returned by the service.
* @sample AWSAppRunnerAsync.ListServices
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listServicesAsync(ListServicesRequest listServicesRequest);
/**
*
* Returns a list of running App Runner services in your Amazon Web Services account.
*
*
* @param listServicesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListServices operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.ListServices
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listServicesAsync(ListServicesRequest listServicesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of the associated App Runner services using an auto scaling configuration.
*
*
* @param listServicesForAutoScalingConfigurationRequest
* @return A Java Future containing the result of the ListServicesForAutoScalingConfiguration operation returned by
* the service.
* @sample AWSAppRunnerAsync.ListServicesForAutoScalingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future listServicesForAutoScalingConfigurationAsync(
ListServicesForAutoScalingConfigurationRequest listServicesForAutoScalingConfigurationRequest);
/**
*
* Returns a list of the associated App Runner services using an auto scaling configuration.
*
*
* @param listServicesForAutoScalingConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListServicesForAutoScalingConfiguration operation returned by
* the service.
* @sample AWSAppRunnerAsyncHandler.ListServicesForAutoScalingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future listServicesForAutoScalingConfigurationAsync(
ListServicesForAutoScalingConfigurationRequest listServicesForAutoScalingConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List tags that are associated with for an App Runner resource. The response contains a list of tag key-value
* pairs.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSAppRunnerAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* List tags that are associated with for an App Runner resource. The response contains a list of tag key-value
* pairs.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of App Runner VPC connectors in your Amazon Web Services account.
*
*
* @param listVpcConnectorsRequest
* @return A Java Future containing the result of the ListVpcConnectors operation returned by the service.
* @sample AWSAppRunnerAsync.ListVpcConnectors
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listVpcConnectorsAsync(ListVpcConnectorsRequest listVpcConnectorsRequest);
/**
*
* Returns a list of App Runner VPC connectors in your Amazon Web Services account.
*
*
* @param listVpcConnectorsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListVpcConnectors operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.ListVpcConnectors
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listVpcConnectorsAsync(ListVpcConnectorsRequest listVpcConnectorsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Return a list of App Runner VPC Ingress Connections in your Amazon Web Services account.
*
*
* @param listVpcIngressConnectionsRequest
* @return A Java Future containing the result of the ListVpcIngressConnections operation returned by the service.
* @sample AWSAppRunnerAsync.ListVpcIngressConnections
* @see AWS API Documentation
*/
java.util.concurrent.Future listVpcIngressConnectionsAsync(
ListVpcIngressConnectionsRequest listVpcIngressConnectionsRequest);
/**
*
* Return a list of App Runner VPC Ingress Connections in your Amazon Web Services account.
*
*
* @param listVpcIngressConnectionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListVpcIngressConnections operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.ListVpcIngressConnections
* @see AWS API Documentation
*/
java.util.concurrent.Future listVpcIngressConnectionsAsync(
ListVpcIngressConnectionsRequest listVpcIngressConnectionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Pause an active App Runner service. App Runner reduces compute capacity for the service to zero and loses state
* (for example, ephemeral storage is removed).
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to track the operation's progress.
*
*
* @param pauseServiceRequest
* @return A Java Future containing the result of the PauseService operation returned by the service.
* @sample AWSAppRunnerAsync.PauseService
* @see AWS API
* Documentation
*/
java.util.concurrent.Future pauseServiceAsync(PauseServiceRequest pauseServiceRequest);
/**
*
* Pause an active App Runner service. App Runner reduces compute capacity for the service to zero and loses state
* (for example, ephemeral storage is removed).
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to track the operation's progress.
*
*
* @param pauseServiceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PauseService operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.PauseService
* @see AWS API
* Documentation
*/
java.util.concurrent.Future pauseServiceAsync(PauseServiceRequest pauseServiceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Resume an active App Runner service. App Runner provisions compute capacity for the service.
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to track the operation's progress.
*
*
* @param resumeServiceRequest
* @return A Java Future containing the result of the ResumeService operation returned by the service.
* @sample AWSAppRunnerAsync.ResumeService
* @see AWS API
* Documentation
*/
java.util.concurrent.Future resumeServiceAsync(ResumeServiceRequest resumeServiceRequest);
/**
*
* Resume an active App Runner service. App Runner provisions compute capacity for the service.
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to track the operation's progress.
*
*
* @param resumeServiceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ResumeService operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.ResumeService
* @see AWS API
* Documentation
*/
java.util.concurrent.Future resumeServiceAsync(ResumeServiceRequest resumeServiceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Initiate a manual deployment of the latest commit in a source code repository or the latest image in a source
* image repository to an App Runner service.
*
*
* For a source code repository, App Runner retrieves the commit and builds a Docker image. For a source image
* repository, App Runner retrieves the latest Docker image. In both cases, App Runner then deploys the new image to
* your service and starts a new container instance.
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to track the operation's progress.
*
*
* @param startDeploymentRequest
* @return A Java Future containing the result of the StartDeployment operation returned by the service.
* @sample AWSAppRunnerAsync.StartDeployment
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startDeploymentAsync(StartDeploymentRequest startDeploymentRequest);
/**
*
* Initiate a manual deployment of the latest commit in a source code repository or the latest image in a source
* image repository to an App Runner service.
*
*
* For a source code repository, App Runner retrieves the commit and builds a Docker image. For a source image
* repository, App Runner retrieves the latest Docker image. In both cases, App Runner then deploys the new image to
* your service and starts a new container instance.
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to track the operation's progress.
*
*
* @param startDeploymentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartDeployment operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.StartDeployment
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startDeploymentAsync(StartDeploymentRequest startDeploymentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Add tags to, or update the tag values of, an App Runner resource. A tag is a key-value pair.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSAppRunnerAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Add tags to, or update the tag values of, an App Runner resource. A tag is a key-value pair.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Remove tags from an App Runner resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSAppRunnerAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Remove tags from an App Runner resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update an auto scaling configuration to be the default. The existing default auto scaling configuration will be
* set to non-default automatically.
*
*
* @param updateDefaultAutoScalingConfigurationRequest
* @return A Java Future containing the result of the UpdateDefaultAutoScalingConfiguration operation returned by
* the service.
* @sample AWSAppRunnerAsync.UpdateDefaultAutoScalingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDefaultAutoScalingConfigurationAsync(
UpdateDefaultAutoScalingConfigurationRequest updateDefaultAutoScalingConfigurationRequest);
/**
*
* Update an auto scaling configuration to be the default. The existing default auto scaling configuration will be
* set to non-default automatically.
*
*
* @param updateDefaultAutoScalingConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDefaultAutoScalingConfiguration operation returned by
* the service.
* @sample AWSAppRunnerAsyncHandler.UpdateDefaultAutoScalingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDefaultAutoScalingConfigurationAsync(
UpdateDefaultAutoScalingConfigurationRequest updateDefaultAutoScalingConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update an App Runner service. You can update the source configuration and instance configuration of the service.
* You can also update the ARN of the auto scaling configuration resource that's associated with the service.
* However, you can't change the name or the encryption configuration of the service. These can be set only when you
* create the service.
*
*
* To update the tags applied to your service, use the separate actions TagResource and UntagResource.
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to track the operation's progress.
*
*
* @param updateServiceRequest
* @return A Java Future containing the result of the UpdateService operation returned by the service.
* @sample AWSAppRunnerAsync.UpdateService
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateServiceAsync(UpdateServiceRequest updateServiceRequest);
/**
*
* Update an App Runner service. You can update the source configuration and instance configuration of the service.
* You can also update the ARN of the auto scaling configuration resource that's associated with the service.
* However, you can't change the name or the encryption configuration of the service. These can be set only when you
* create the service.
*
*
* To update the tags applied to your service, use the separate actions TagResource and UntagResource.
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to track the operation's progress.
*
*
* @param updateServiceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateService operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.UpdateService
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateServiceAsync(UpdateServiceRequest updateServiceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update an existing App Runner VPC Ingress Connection resource. The VPC Ingress Connection must be in one of the
* following states to be updated:
*
*
* -
*
* AVAILABLE
*
*
* -
*
* FAILED_CREATION
*
*
* -
*
* FAILED_UPDATE
*
*
*
*
* @param updateVpcIngressConnectionRequest
* @return A Java Future containing the result of the UpdateVpcIngressConnection operation returned by the service.
* @sample AWSAppRunnerAsync.UpdateVpcIngressConnection
* @see AWS API Documentation
*/
java.util.concurrent.Future updateVpcIngressConnectionAsync(
UpdateVpcIngressConnectionRequest updateVpcIngressConnectionRequest);
/**
*
* Update an existing App Runner VPC Ingress Connection resource. The VPC Ingress Connection must be in one of the
* following states to be updated:
*
*
* -
*
* AVAILABLE
*
*
* -
*
* FAILED_CREATION
*
*
* -
*
* FAILED_UPDATE
*
*
*
*
* @param updateVpcIngressConnectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateVpcIngressConnection operation returned by the service.
* @sample AWSAppRunnerAsyncHandler.UpdateVpcIngressConnection
* @see AWS API Documentation
*/
java.util.concurrent.Future updateVpcIngressConnectionAsync(
UpdateVpcIngressConnectionRequest updateVpcIngressConnectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}