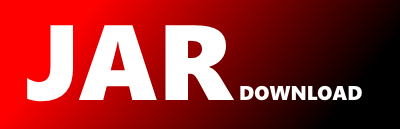
com.amazonaws.services.apprunner.AWSAppRunnerClient Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.apprunner;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.apprunner.AWSAppRunnerClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.apprunner.model.*;
import com.amazonaws.services.apprunner.model.transform.*;
/**
* Client for accessing AWS App Runner. All service calls made using this client are blocking, and will not return until
* the service call completes.
*
* App Runner
*
* App Runner is an application service that provides a fast, simple, and cost-effective way to go directly from an
* existing container image or source code to a running service in the Amazon Web Services Cloud in seconds. You don't
* need to learn new technologies, decide which compute service to use, or understand how to provision and configure
* Amazon Web Services resources.
*
*
* App Runner connects directly to your container registry or source code repository. It provides an automatic delivery
* pipeline with fully managed operations, high performance, scalability, and security.
*
*
* For more information about App Runner, see the App Runner
* Developer Guide. For release information, see the App Runner Release Notes.
*
*
* To install the Software Development Kits (SDKs), Integrated Development Environment (IDE) Toolkits, and command line
* tools that you can use to access the API, see Tools for Amazon Web
* Services.
*
*
* Endpoints
*
*
* For a list of Region-specific endpoints that App Runner supports, see App Runner endpoints and quotas in the
* Amazon Web Services General Reference.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSAppRunnerClient extends AmazonWebServiceClient implements AWSAppRunner {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSAppRunner.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "apprunner";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.0")
.withSupportsCbor(false)
.withSupportsIon(false)
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidRequestException").withExceptionUnmarshaller(
com.amazonaws.services.apprunner.model.transform.InvalidRequestExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceQuotaExceededException").withExceptionUnmarshaller(
com.amazonaws.services.apprunner.model.transform.ServiceQuotaExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidStateException").withExceptionUnmarshaller(
com.amazonaws.services.apprunner.model.transform.InvalidStateExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.apprunner.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServiceErrorException").withExceptionUnmarshaller(
com.amazonaws.services.apprunner.model.transform.InternalServiceErrorExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.apprunner.model.AWSAppRunnerException.class));
public static AWSAppRunnerClientBuilder builder() {
return AWSAppRunnerClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on AWS App Runner using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSAppRunnerClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on AWS App Runner using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSAppRunnerClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("apprunner.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/apprunner/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/apprunner/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Associate your own domain name with the App Runner subdomain URL of your App Runner service.
*
*
* After you call AssociateCustomDomain
and receive a successful response, use the information in the
* CustomDomain record that's returned to add CNAME records to your Domain Name System (DNS). For each mapped
* domain name, add a mapping to the target App Runner subdomain and one or more certificate validation records. App
* Runner then performs DNS validation to verify that you own or control the domain name that you associated. App
* Runner tracks domain validity in a certificate stored in AWS Certificate Manager (ACM).
*
*
* @param associateCustomDomainRequest
* @return Result of the AssociateCustomDomain operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @sample AWSAppRunner.AssociateCustomDomain
* @see AWS API Documentation
*/
@Override
public AssociateCustomDomainResult associateCustomDomain(AssociateCustomDomainRequest request) {
request = beforeClientExecution(request);
return executeAssociateCustomDomain(request);
}
@SdkInternalApi
final AssociateCustomDomainResult executeAssociateCustomDomain(AssociateCustomDomainRequest associateCustomDomainRequest) {
ExecutionContext executionContext = createExecutionContext(associateCustomDomainRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateCustomDomainRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(associateCustomDomainRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateCustomDomain");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateCustomDomainResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Create an App Runner automatic scaling configuration resource. App Runner requires this resource when you create
* or update App Runner services and you require non-default auto scaling settings. You can share an auto scaling
* configuration across multiple services.
*
*
* Create multiple revisions of a configuration by calling this action multiple times using the same
* AutoScalingConfigurationName
. The call returns incremental
* AutoScalingConfigurationRevision
values. When you create a service and configure an auto scaling
* configuration resource, the service uses the latest active revision of the auto scaling configuration by default.
* You can optionally configure the service to use a specific revision.
*
*
* Configure a higher MinSize
to increase the spread of your App Runner service over more Availability
* Zones in the Amazon Web Services Region. The tradeoff is a higher minimal cost.
*
*
* Configure a lower MaxSize
to control your cost. The tradeoff is lower responsiveness during peak
* demand.
*
*
* @param createAutoScalingConfigurationRequest
* @return Result of the CreateAutoScalingConfiguration operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ServiceQuotaExceededException
* App Runner can't create this resource. You've reached your account quota for this resource type.
*
* For App Runner per-resource quotas, see App Runner endpoints and quotas
* in the Amazon Web Services General Reference.
* @sample AWSAppRunner.CreateAutoScalingConfiguration
* @see AWS API Documentation
*/
@Override
public CreateAutoScalingConfigurationResult createAutoScalingConfiguration(CreateAutoScalingConfigurationRequest request) {
request = beforeClientExecution(request);
return executeCreateAutoScalingConfiguration(request);
}
@SdkInternalApi
final CreateAutoScalingConfigurationResult executeCreateAutoScalingConfiguration(CreateAutoScalingConfigurationRequest createAutoScalingConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(createAutoScalingConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAutoScalingConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createAutoScalingConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAutoScalingConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateAutoScalingConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Create an App Runner connection resource. App Runner requires a connection resource when you create App Runner
* services that access private repositories from certain third-party providers. You can share a connection across
* multiple services.
*
*
* A connection resource is needed to access GitHub and Bitbucket repositories. Both require a user interface
* approval process through the App Runner console before you can use the connection.
*
*
* @param createConnectionRequest
* @return Result of the CreateConnection operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ServiceQuotaExceededException
* App Runner can't create this resource. You've reached your account quota for this resource type.
*
* For App Runner per-resource quotas, see App Runner endpoints and quotas
* in the Amazon Web Services General Reference.
* @sample AWSAppRunner.CreateConnection
* @see AWS API
* Documentation
*/
@Override
public CreateConnectionResult createConnection(CreateConnectionRequest request) {
request = beforeClientExecution(request);
return executeCreateConnection(request);
}
@SdkInternalApi
final CreateConnectionResult executeCreateConnection(CreateConnectionRequest createConnectionRequest) {
ExecutionContext executionContext = createExecutionContext(createConnectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateConnectionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createConnectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateConnection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateConnectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Create an App Runner observability configuration resource. App Runner requires this resource when you create or
* update App Runner services and you want to enable non-default observability features. You can share an
* observability configuration across multiple services.
*
*
* Create multiple revisions of a configuration by calling this action multiple times using the same
* ObservabilityConfigurationName
. The call returns incremental
* ObservabilityConfigurationRevision
values. When you create a service and configure an observability
* configuration resource, the service uses the latest active revision of the observability configuration by
* default. You can optionally configure the service to use a specific revision.
*
*
* The observability configuration resource is designed to configure multiple features (currently one feature,
* tracing). This action takes optional parameters that describe the configuration of these features (currently one
* parameter, TraceConfiguration
). If you don't specify a feature parameter, App Runner doesn't enable
* the feature.
*
*
* @param createObservabilityConfigurationRequest
* @return Result of the CreateObservabilityConfiguration operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ServiceQuotaExceededException
* App Runner can't create this resource. You've reached your account quota for this resource type.
*
* For App Runner per-resource quotas, see App Runner endpoints and quotas
* in the Amazon Web Services General Reference.
* @sample AWSAppRunner.CreateObservabilityConfiguration
* @see AWS API Documentation
*/
@Override
public CreateObservabilityConfigurationResult createObservabilityConfiguration(CreateObservabilityConfigurationRequest request) {
request = beforeClientExecution(request);
return executeCreateObservabilityConfiguration(request);
}
@SdkInternalApi
final CreateObservabilityConfigurationResult executeCreateObservabilityConfiguration(
CreateObservabilityConfigurationRequest createObservabilityConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(createObservabilityConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateObservabilityConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createObservabilityConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateObservabilityConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateObservabilityConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Create an App Runner service. After the service is created, the action also automatically starts a deployment.
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to
* track the operation's progress.
*
*
* @param createServiceRequest
* @return Result of the CreateService operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ServiceQuotaExceededException
* App Runner can't create this resource. You've reached your account quota for this resource type.
*
* For App Runner per-resource quotas, see App Runner endpoints and quotas
* in the Amazon Web Services General Reference.
* @sample AWSAppRunner.CreateService
* @see AWS API
* Documentation
*/
@Override
public CreateServiceResult createService(CreateServiceRequest request) {
request = beforeClientExecution(request);
return executeCreateService(request);
}
@SdkInternalApi
final CreateServiceResult executeCreateService(CreateServiceRequest createServiceRequest) {
ExecutionContext executionContext = createExecutionContext(createServiceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateServiceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createServiceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateService");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateServiceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Create an App Runner VPC connector resource. App Runner requires this resource when you want to associate your
* App Runner service to a custom Amazon Virtual Private Cloud (Amazon VPC).
*
*
* @param createVpcConnectorRequest
* @return Result of the CreateVpcConnector operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ServiceQuotaExceededException
* App Runner can't create this resource. You've reached your account quota for this resource type.
*
* For App Runner per-resource quotas, see App Runner endpoints and quotas
* in the Amazon Web Services General Reference.
* @sample AWSAppRunner.CreateVpcConnector
* @see AWS
* API Documentation
*/
@Override
public CreateVpcConnectorResult createVpcConnector(CreateVpcConnectorRequest request) {
request = beforeClientExecution(request);
return executeCreateVpcConnector(request);
}
@SdkInternalApi
final CreateVpcConnectorResult executeCreateVpcConnector(CreateVpcConnectorRequest createVpcConnectorRequest) {
ExecutionContext executionContext = createExecutionContext(createVpcConnectorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateVpcConnectorRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createVpcConnectorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateVpcConnector");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateVpcConnectorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Create an App Runner VPC Ingress Connection resource. App Runner requires this resource when you want to
* associate your App Runner service with an Amazon VPC endpoint.
*
*
* @param createVpcIngressConnectionRequest
* @return Result of the CreateVpcIngressConnection operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ServiceQuotaExceededException
* App Runner can't create this resource. You've reached your account quota for this resource type.
*
* For App Runner per-resource quotas, see App Runner endpoints and quotas
* in the Amazon Web Services General Reference.
* @sample AWSAppRunner.CreateVpcIngressConnection
* @see AWS API Documentation
*/
@Override
public CreateVpcIngressConnectionResult createVpcIngressConnection(CreateVpcIngressConnectionRequest request) {
request = beforeClientExecution(request);
return executeCreateVpcIngressConnection(request);
}
@SdkInternalApi
final CreateVpcIngressConnectionResult executeCreateVpcIngressConnection(CreateVpcIngressConnectionRequest createVpcIngressConnectionRequest) {
ExecutionContext executionContext = createExecutionContext(createVpcIngressConnectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateVpcIngressConnectionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createVpcIngressConnectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateVpcIngressConnection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateVpcIngressConnectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Delete an App Runner automatic scaling configuration resource. You can delete a top level auto scaling
* configuration, a specific revision of one, or all revisions associated with the top level configuration. You
* can't delete the default auto scaling configuration or a configuration that's used by one or more App Runner
* services.
*
*
* @param deleteAutoScalingConfigurationRequest
* @return Result of the DeleteAutoScalingConfiguration operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @sample AWSAppRunner.DeleteAutoScalingConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteAutoScalingConfigurationResult deleteAutoScalingConfiguration(DeleteAutoScalingConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDeleteAutoScalingConfiguration(request);
}
@SdkInternalApi
final DeleteAutoScalingConfigurationResult executeDeleteAutoScalingConfiguration(DeleteAutoScalingConfigurationRequest deleteAutoScalingConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAutoScalingConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAutoScalingConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteAutoScalingConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAutoScalingConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteAutoScalingConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Delete an App Runner connection. You must first ensure that there are no running App Runner services that use
* this connection. If there are any, the DeleteConnection
action fails.
*
*
* @param deleteConnectionRequest
* @return Result of the DeleteConnection operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @sample AWSAppRunner.DeleteConnection
* @see AWS API
* Documentation
*/
@Override
public DeleteConnectionResult deleteConnection(DeleteConnectionRequest request) {
request = beforeClientExecution(request);
return executeDeleteConnection(request);
}
@SdkInternalApi
final DeleteConnectionResult executeDeleteConnection(DeleteConnectionRequest deleteConnectionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteConnectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteConnectionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteConnectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteConnection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteConnectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Delete an App Runner observability configuration resource. You can delete a specific revision or the latest
* active revision. You can't delete a configuration that's used by one or more App Runner services.
*
*
* @param deleteObservabilityConfigurationRequest
* @return Result of the DeleteObservabilityConfiguration operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @sample AWSAppRunner.DeleteObservabilityConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteObservabilityConfigurationResult deleteObservabilityConfiguration(DeleteObservabilityConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDeleteObservabilityConfiguration(request);
}
@SdkInternalApi
final DeleteObservabilityConfigurationResult executeDeleteObservabilityConfiguration(
DeleteObservabilityConfigurationRequest deleteObservabilityConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteObservabilityConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteObservabilityConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteObservabilityConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteObservabilityConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteObservabilityConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Delete an App Runner service.
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to track the operation's progress.
*
*
*
* Make sure that you don't have any active VPCIngressConnections associated with the service you want to delete.
*
*
*
* @param deleteServiceRequest
* @return Result of the DeleteService operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @sample AWSAppRunner.DeleteService
* @see AWS API
* Documentation
*/
@Override
public DeleteServiceResult deleteService(DeleteServiceRequest request) {
request = beforeClientExecution(request);
return executeDeleteService(request);
}
@SdkInternalApi
final DeleteServiceResult executeDeleteService(DeleteServiceRequest deleteServiceRequest) {
ExecutionContext executionContext = createExecutionContext(deleteServiceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteServiceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteServiceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteService");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteServiceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Delete an App Runner VPC connector resource. You can't delete a connector that's used by one or more App Runner
* services.
*
*
* @param deleteVpcConnectorRequest
* @return Result of the DeleteVpcConnector operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @sample AWSAppRunner.DeleteVpcConnector
* @see AWS
* API Documentation
*/
@Override
public DeleteVpcConnectorResult deleteVpcConnector(DeleteVpcConnectorRequest request) {
request = beforeClientExecution(request);
return executeDeleteVpcConnector(request);
}
@SdkInternalApi
final DeleteVpcConnectorResult executeDeleteVpcConnector(DeleteVpcConnectorRequest deleteVpcConnectorRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVpcConnectorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVpcConnectorRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteVpcConnectorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVpcConnector");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteVpcConnectorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Delete an App Runner VPC Ingress Connection resource that's associated with an App Runner service. The VPC
* Ingress Connection must be in one of the following states to be deleted:
*
*
* -
*
* AVAILABLE
*
*
* -
*
* FAILED_CREATION
*
*
* -
*
* FAILED_UPDATE
*
*
* -
*
* FAILED_DELETION
*
*
*
*
* @param deleteVpcIngressConnectionRequest
* @return Result of the DeleteVpcIngressConnection operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @sample AWSAppRunner.DeleteVpcIngressConnection
* @see AWS API Documentation
*/
@Override
public DeleteVpcIngressConnectionResult deleteVpcIngressConnection(DeleteVpcIngressConnectionRequest request) {
request = beforeClientExecution(request);
return executeDeleteVpcIngressConnection(request);
}
@SdkInternalApi
final DeleteVpcIngressConnectionResult executeDeleteVpcIngressConnection(DeleteVpcIngressConnectionRequest deleteVpcIngressConnectionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVpcIngressConnectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVpcIngressConnectionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteVpcIngressConnectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVpcIngressConnection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteVpcIngressConnectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Return a full description of an App Runner automatic scaling configuration resource.
*
*
* @param describeAutoScalingConfigurationRequest
* @return Result of the DescribeAutoScalingConfiguration operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @sample AWSAppRunner.DescribeAutoScalingConfiguration
* @see AWS API Documentation
*/
@Override
public DescribeAutoScalingConfigurationResult describeAutoScalingConfiguration(DescribeAutoScalingConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDescribeAutoScalingConfiguration(request);
}
@SdkInternalApi
final DescribeAutoScalingConfigurationResult executeDescribeAutoScalingConfiguration(
DescribeAutoScalingConfigurationRequest describeAutoScalingConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(describeAutoScalingConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAutoScalingConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeAutoScalingConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAutoScalingConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeAutoScalingConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Return a description of custom domain names that are associated with an App Runner service.
*
*
* @param describeCustomDomainsRequest
* @return Result of the DescribeCustomDomains operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @sample AWSAppRunner.DescribeCustomDomains
* @see AWS API Documentation
*/
@Override
public DescribeCustomDomainsResult describeCustomDomains(DescribeCustomDomainsRequest request) {
request = beforeClientExecution(request);
return executeDescribeCustomDomains(request);
}
@SdkInternalApi
final DescribeCustomDomainsResult executeDescribeCustomDomains(DescribeCustomDomainsRequest describeCustomDomainsRequest) {
ExecutionContext executionContext = createExecutionContext(describeCustomDomainsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeCustomDomainsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeCustomDomainsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeCustomDomains");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeCustomDomainsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Return a full description of an App Runner observability configuration resource.
*
*
* @param describeObservabilityConfigurationRequest
* @return Result of the DescribeObservabilityConfiguration operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @sample AWSAppRunner.DescribeObservabilityConfiguration
* @see AWS API Documentation
*/
@Override
public DescribeObservabilityConfigurationResult describeObservabilityConfiguration(DescribeObservabilityConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDescribeObservabilityConfiguration(request);
}
@SdkInternalApi
final DescribeObservabilityConfigurationResult executeDescribeObservabilityConfiguration(
DescribeObservabilityConfigurationRequest describeObservabilityConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(describeObservabilityConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeObservabilityConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeObservabilityConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeObservabilityConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeObservabilityConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Return a full description of an App Runner service.
*
*
* @param describeServiceRequest
* @return Result of the DescribeService operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @sample AWSAppRunner.DescribeService
* @see AWS API
* Documentation
*/
@Override
public DescribeServiceResult describeService(DescribeServiceRequest request) {
request = beforeClientExecution(request);
return executeDescribeService(request);
}
@SdkInternalApi
final DescribeServiceResult executeDescribeService(DescribeServiceRequest describeServiceRequest) {
ExecutionContext executionContext = createExecutionContext(describeServiceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeServiceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeServiceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeService");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeServiceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Return a description of an App Runner VPC connector resource.
*
*
* @param describeVpcConnectorRequest
* @return Result of the DescribeVpcConnector operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @sample AWSAppRunner.DescribeVpcConnector
* @see AWS
* API Documentation
*/
@Override
public DescribeVpcConnectorResult describeVpcConnector(DescribeVpcConnectorRequest request) {
request = beforeClientExecution(request);
return executeDescribeVpcConnector(request);
}
@SdkInternalApi
final DescribeVpcConnectorResult executeDescribeVpcConnector(DescribeVpcConnectorRequest describeVpcConnectorRequest) {
ExecutionContext executionContext = createExecutionContext(describeVpcConnectorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeVpcConnectorRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeVpcConnectorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeVpcConnector");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeVpcConnectorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Return a full description of an App Runner VPC Ingress Connection resource.
*
*
* @param describeVpcIngressConnectionRequest
* @return Result of the DescribeVpcIngressConnection operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @sample AWSAppRunner.DescribeVpcIngressConnection
* @see AWS API Documentation
*/
@Override
public DescribeVpcIngressConnectionResult describeVpcIngressConnection(DescribeVpcIngressConnectionRequest request) {
request = beforeClientExecution(request);
return executeDescribeVpcIngressConnection(request);
}
@SdkInternalApi
final DescribeVpcIngressConnectionResult executeDescribeVpcIngressConnection(DescribeVpcIngressConnectionRequest describeVpcIngressConnectionRequest) {
ExecutionContext executionContext = createExecutionContext(describeVpcIngressConnectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeVpcIngressConnectionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeVpcIngressConnectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeVpcIngressConnection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeVpcIngressConnectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociate a custom domain name from an App Runner service.
*
*
* Certificates tracking domain validity are associated with a custom domain and are stored in AWS Certificate Manager (ACM). These certificates
* aren't deleted as part of this action. App Runner delays certificate deletion for 30 days after a domain is
* disassociated from your service.
*
*
* @param disassociateCustomDomainRequest
* @return Result of the DisassociateCustomDomain operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @sample AWSAppRunner.DisassociateCustomDomain
* @see AWS API Documentation
*/
@Override
public DisassociateCustomDomainResult disassociateCustomDomain(DisassociateCustomDomainRequest request) {
request = beforeClientExecution(request);
return executeDisassociateCustomDomain(request);
}
@SdkInternalApi
final DisassociateCustomDomainResult executeDisassociateCustomDomain(DisassociateCustomDomainRequest disassociateCustomDomainRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateCustomDomainRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateCustomDomainRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateCustomDomainRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateCustomDomain");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateCustomDomainResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of active App Runner automatic scaling configurations in your Amazon Web Services account. You can
* query the revisions for a specific configuration name or the revisions for all active configurations in your
* account. You can optionally query only the latest revision of each requested name.
*
*
* To retrieve a full description of a particular configuration revision, call and provide one of the ARNs returned
* by ListAutoScalingConfigurations
.
*
*
* @param listAutoScalingConfigurationsRequest
* @return Result of the ListAutoScalingConfigurations operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @sample AWSAppRunner.ListAutoScalingConfigurations
* @see AWS API Documentation
*/
@Override
public ListAutoScalingConfigurationsResult listAutoScalingConfigurations(ListAutoScalingConfigurationsRequest request) {
request = beforeClientExecution(request);
return executeListAutoScalingConfigurations(request);
}
@SdkInternalApi
final ListAutoScalingConfigurationsResult executeListAutoScalingConfigurations(ListAutoScalingConfigurationsRequest listAutoScalingConfigurationsRequest) {
ExecutionContext executionContext = createExecutionContext(listAutoScalingConfigurationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAutoScalingConfigurationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listAutoScalingConfigurationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAutoScalingConfigurations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListAutoScalingConfigurationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of App Runner connections that are associated with your Amazon Web Services account.
*
*
* @param listConnectionsRequest
* @return Result of the ListConnections operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @sample AWSAppRunner.ListConnections
* @see AWS API
* Documentation
*/
@Override
public ListConnectionsResult listConnections(ListConnectionsRequest request) {
request = beforeClientExecution(request);
return executeListConnections(request);
}
@SdkInternalApi
final ListConnectionsResult executeListConnections(ListConnectionsRequest listConnectionsRequest) {
ExecutionContext executionContext = createExecutionContext(listConnectionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListConnectionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listConnectionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListConnections");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListConnectionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of active App Runner observability configurations in your Amazon Web Services account. You can
* query the revisions for a specific configuration name or the revisions for all active configurations in your
* account. You can optionally query only the latest revision of each requested name.
*
*
* To retrieve a full description of a particular configuration revision, call and provide one of the ARNs returned
* by ListObservabilityConfigurations
.
*
*
* @param listObservabilityConfigurationsRequest
* @return Result of the ListObservabilityConfigurations operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @sample AWSAppRunner.ListObservabilityConfigurations
* @see AWS API Documentation
*/
@Override
public ListObservabilityConfigurationsResult listObservabilityConfigurations(ListObservabilityConfigurationsRequest request) {
request = beforeClientExecution(request);
return executeListObservabilityConfigurations(request);
}
@SdkInternalApi
final ListObservabilityConfigurationsResult executeListObservabilityConfigurations(
ListObservabilityConfigurationsRequest listObservabilityConfigurationsRequest) {
ExecutionContext executionContext = createExecutionContext(listObservabilityConfigurationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListObservabilityConfigurationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listObservabilityConfigurationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListObservabilityConfigurations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListObservabilityConfigurationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Return a list of operations that occurred on an App Runner service.
*
*
* The resulting list of OperationSummary objects is sorted in reverse chronological order. The first object
* on the list represents the last started operation.
*
*
* @param listOperationsRequest
* @return Result of the ListOperations operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @sample AWSAppRunner.ListOperations
* @see AWS API
* Documentation
*/
@Override
public ListOperationsResult listOperations(ListOperationsRequest request) {
request = beforeClientExecution(request);
return executeListOperations(request);
}
@SdkInternalApi
final ListOperationsResult executeListOperations(ListOperationsRequest listOperationsRequest) {
ExecutionContext executionContext = createExecutionContext(listOperationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListOperationsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listOperationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListOperations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListOperationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of running App Runner services in your Amazon Web Services account.
*
*
* @param listServicesRequest
* @return Result of the ListServices operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @sample AWSAppRunner.ListServices
* @see AWS API
* Documentation
*/
@Override
public ListServicesResult listServices(ListServicesRequest request) {
request = beforeClientExecution(request);
return executeListServices(request);
}
@SdkInternalApi
final ListServicesResult executeListServices(ListServicesRequest listServicesRequest) {
ExecutionContext executionContext = createExecutionContext(listServicesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListServicesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listServicesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListServices");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListServicesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of the associated App Runner services using an auto scaling configuration.
*
*
* @param listServicesForAutoScalingConfigurationRequest
* @return Result of the ListServicesForAutoScalingConfiguration operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @sample AWSAppRunner.ListServicesForAutoScalingConfiguration
* @see AWS API Documentation
*/
@Override
public ListServicesForAutoScalingConfigurationResult listServicesForAutoScalingConfiguration(ListServicesForAutoScalingConfigurationRequest request) {
request = beforeClientExecution(request);
return executeListServicesForAutoScalingConfiguration(request);
}
@SdkInternalApi
final ListServicesForAutoScalingConfigurationResult executeListServicesForAutoScalingConfiguration(
ListServicesForAutoScalingConfigurationRequest listServicesForAutoScalingConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(listServicesForAutoScalingConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListServicesForAutoScalingConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listServicesForAutoScalingConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListServicesForAutoScalingConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListServicesForAutoScalingConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List tags that are associated with for an App Runner resource. The response contains a list of tag key-value
* pairs.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @sample AWSAppRunner.ListTagsForResource
* @see AWS
* API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of App Runner VPC connectors in your Amazon Web Services account.
*
*
* @param listVpcConnectorsRequest
* @return Result of the ListVpcConnectors operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @sample AWSAppRunner.ListVpcConnectors
* @see AWS
* API Documentation
*/
@Override
public ListVpcConnectorsResult listVpcConnectors(ListVpcConnectorsRequest request) {
request = beforeClientExecution(request);
return executeListVpcConnectors(request);
}
@SdkInternalApi
final ListVpcConnectorsResult executeListVpcConnectors(ListVpcConnectorsRequest listVpcConnectorsRequest) {
ExecutionContext executionContext = createExecutionContext(listVpcConnectorsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListVpcConnectorsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listVpcConnectorsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListVpcConnectors");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListVpcConnectorsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Return a list of App Runner VPC Ingress Connections in your Amazon Web Services account.
*
*
* @param listVpcIngressConnectionsRequest
* @return Result of the ListVpcIngressConnections operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @sample AWSAppRunner.ListVpcIngressConnections
* @see AWS API Documentation
*/
@Override
public ListVpcIngressConnectionsResult listVpcIngressConnections(ListVpcIngressConnectionsRequest request) {
request = beforeClientExecution(request);
return executeListVpcIngressConnections(request);
}
@SdkInternalApi
final ListVpcIngressConnectionsResult executeListVpcIngressConnections(ListVpcIngressConnectionsRequest listVpcIngressConnectionsRequest) {
ExecutionContext executionContext = createExecutionContext(listVpcIngressConnectionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListVpcIngressConnectionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listVpcIngressConnectionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListVpcIngressConnections");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListVpcIngressConnectionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Pause an active App Runner service. App Runner reduces compute capacity for the service to zero and loses state
* (for example, ephemeral storage is removed).
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to track the operation's progress.
*
*
* @param pauseServiceRequest
* @return Result of the PauseService operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @sample AWSAppRunner.PauseService
* @see AWS API
* Documentation
*/
@Override
public PauseServiceResult pauseService(PauseServiceRequest request) {
request = beforeClientExecution(request);
return executePauseService(request);
}
@SdkInternalApi
final PauseServiceResult executePauseService(PauseServiceRequest pauseServiceRequest) {
ExecutionContext executionContext = createExecutionContext(pauseServiceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PauseServiceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(pauseServiceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PauseService");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new PauseServiceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Resume an active App Runner service. App Runner provisions compute capacity for the service.
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to track the operation's progress.
*
*
* @param resumeServiceRequest
* @return Result of the ResumeService operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @sample AWSAppRunner.ResumeService
* @see AWS API
* Documentation
*/
@Override
public ResumeServiceResult resumeService(ResumeServiceRequest request) {
request = beforeClientExecution(request);
return executeResumeService(request);
}
@SdkInternalApi
final ResumeServiceResult executeResumeService(ResumeServiceRequest resumeServiceRequest) {
ExecutionContext executionContext = createExecutionContext(resumeServiceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ResumeServiceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(resumeServiceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ResumeService");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ResumeServiceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Initiate a manual deployment of the latest commit in a source code repository or the latest image in a source
* image repository to an App Runner service.
*
*
* For a source code repository, App Runner retrieves the commit and builds a Docker image. For a source image
* repository, App Runner retrieves the latest Docker image. In both cases, App Runner then deploys the new image to
* your service and starts a new container instance.
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to track the operation's progress.
*
*
* @param startDeploymentRequest
* @return Result of the StartDeployment operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @sample AWSAppRunner.StartDeployment
* @see AWS API
* Documentation
*/
@Override
public StartDeploymentResult startDeployment(StartDeploymentRequest request) {
request = beforeClientExecution(request);
return executeStartDeployment(request);
}
@SdkInternalApi
final StartDeploymentResult executeStartDeployment(StartDeploymentRequest startDeploymentRequest) {
ExecutionContext executionContext = createExecutionContext(startDeploymentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartDeploymentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startDeploymentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartDeployment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StartDeploymentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Add tags to, or update the tag values of, an App Runner resource. A tag is a key-value pair.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @sample AWSAppRunner.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Remove tags from an App Runner resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @sample AWSAppRunner.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Update an auto scaling configuration to be the default. The existing default auto scaling configuration will be
* set to non-default automatically.
*
*
* @param updateDefaultAutoScalingConfigurationRequest
* @return Result of the UpdateDefaultAutoScalingConfiguration operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @sample AWSAppRunner.UpdateDefaultAutoScalingConfiguration
* @see AWS API Documentation
*/
@Override
public UpdateDefaultAutoScalingConfigurationResult updateDefaultAutoScalingConfiguration(UpdateDefaultAutoScalingConfigurationRequest request) {
request = beforeClientExecution(request);
return executeUpdateDefaultAutoScalingConfiguration(request);
}
@SdkInternalApi
final UpdateDefaultAutoScalingConfigurationResult executeUpdateDefaultAutoScalingConfiguration(
UpdateDefaultAutoScalingConfigurationRequest updateDefaultAutoScalingConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(updateDefaultAutoScalingConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateDefaultAutoScalingConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateDefaultAutoScalingConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateDefaultAutoScalingConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateDefaultAutoScalingConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Update an App Runner service. You can update the source configuration and instance configuration of the service.
* You can also update the ARN of the auto scaling configuration resource that's associated with the service.
* However, you can't change the name or the encryption configuration of the service. These can be set only when you
* create the service.
*
*
* To update the tags applied to your service, use the separate actions TagResource and UntagResource.
*
*
* This is an asynchronous operation. On a successful call, you can use the returned OperationId
and
* the ListOperations call to track the operation's progress.
*
*
* @param updateServiceRequest
* @return Result of the UpdateService operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @sample AWSAppRunner.UpdateService
* @see AWS API
* Documentation
*/
@Override
public UpdateServiceResult updateService(UpdateServiceRequest request) {
request = beforeClientExecution(request);
return executeUpdateService(request);
}
@SdkInternalApi
final UpdateServiceResult executeUpdateService(UpdateServiceRequest updateServiceRequest) {
ExecutionContext executionContext = createExecutionContext(updateServiceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateServiceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateServiceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateService");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateServiceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Update an existing App Runner VPC Ingress Connection resource. The VPC Ingress Connection must be in one of the
* following states to be updated:
*
*
* -
*
* AVAILABLE
*
*
* -
*
* FAILED_CREATION
*
*
* -
*
* FAILED_UPDATE
*
*
*
*
* @param updateVpcIngressConnectionRequest
* @return Result of the UpdateVpcIngressConnection operation returned by the service.
* @throws InvalidRequestException
* One or more input parameters aren't valid. Refer to the API action's document page, correct the input
* parameters, and try the action again.
* @throws ResourceNotFoundException
* A resource doesn't exist for the specified Amazon Resource Name (ARN) in your Amazon Web Services
* account.
* @throws InvalidStateException
* You can't perform this action when the resource is in its current state.
* @throws InternalServiceErrorException
* An unexpected service exception occurred.
* @sample AWSAppRunner.UpdateVpcIngressConnection
* @see AWS API Documentation
*/
@Override
public UpdateVpcIngressConnectionResult updateVpcIngressConnection(UpdateVpcIngressConnectionRequest request) {
request = beforeClientExecution(request);
return executeUpdateVpcIngressConnection(request);
}
@SdkInternalApi
final UpdateVpcIngressConnectionResult executeUpdateVpcIngressConnection(UpdateVpcIngressConnectionRequest updateVpcIngressConnectionRequest) {
ExecutionContext executionContext = createExecutionContext(updateVpcIngressConnectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateVpcIngressConnectionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateVpcIngressConnectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppRunner");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateVpcIngressConnection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateVpcIngressConnectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}