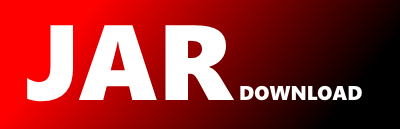
com.amazonaws.services.apprunner.model.AutoScalingConfiguration Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.apprunner.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes an App Runner automatic scaling configuration resource.
*
*
* A higher MinSize
increases the spread of your App Runner service over more Availability Zones in the
* Amazon Web Services Region. The tradeoff is a higher minimal cost.
*
*
* A lower MaxSize
controls your cost. The tradeoff is lower responsiveness during peak demand.
*
*
* Multiple revisions of a configuration might have the same AutoScalingConfigurationName
and different
* AutoScalingConfigurationRevision
values.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AutoScalingConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource Name (ARN) of this auto scaling configuration.
*
*/
private String autoScalingConfigurationArn;
/**
*
* The customer-provided auto scaling configuration name. It can be used in multiple revisions of a configuration.
*
*/
private String autoScalingConfigurationName;
/**
*
* The revision of this auto scaling configuration. It's unique among all the active configurations (
* "Status": "ACTIVE"
) that share the same AutoScalingConfigurationName
.
*
*/
private Integer autoScalingConfigurationRevision;
/**
*
* It's set to true
for the configuration with the highest Revision
among all
* configurations that share the same AutoScalingConfigurationName
. It's set to false
* otherwise.
*
*/
private Boolean latest;
/**
*
* The current state of the auto scaling configuration. If the status of a configuration revision is
* INACTIVE
, it was deleted and can't be used. Inactive configuration revisions are permanently removed
* some time after they are deleted.
*
*/
private String status;
/**
*
* The maximum number of concurrent requests that an instance processes. If the number of concurrent requests
* exceeds this limit, App Runner scales the service up.
*
*/
private Integer maxConcurrency;
/**
*
* The minimum number of instances that App Runner provisions for a service. The service always has at least
* MinSize
provisioned instances. Some of them actively serve traffic. The rest of them (provisioned
* and inactive instances) are a cost-effective compute capacity reserve and are ready to be quickly activated. You
* pay for memory usage of all the provisioned instances. You pay for CPU usage of only the active subset.
*
*
* App Runner temporarily doubles the number of provisioned instances during deployments, to maintain the same
* capacity for both old and new code.
*
*/
private Integer minSize;
/**
*
* The maximum number of instances that a service scales up to. At most MaxSize
instances actively
* serve traffic for your service.
*
*/
private Integer maxSize;
/**
*
* The time when the auto scaling configuration was created. It's in Unix time stamp format.
*
*/
private java.util.Date createdAt;
/**
*
* The time when the auto scaling configuration was deleted. It's in Unix time stamp format.
*
*/
private java.util.Date deletedAt;
/**
*
* Indicates if this auto scaling configuration has an App Runner service associated with it. A value of
* true
indicates one or more services are associated. A value of false
indicates no
* services are associated.
*
*/
private Boolean hasAssociatedService;
/**
*
* Indicates if this auto scaling configuration should be used as the default for a new App Runner service that does
* not have an auto scaling configuration ARN specified during creation. Each account can have only one default
* AutoScalingConfiguration
per region. The default AutoScalingConfiguration
can be any
* revision under the same AutoScalingConfigurationName
.
*
*/
private Boolean isDefault;
/**
*
* The Amazon Resource Name (ARN) of this auto scaling configuration.
*
*
* @param autoScalingConfigurationArn
* The Amazon Resource Name (ARN) of this auto scaling configuration.
*/
public void setAutoScalingConfigurationArn(String autoScalingConfigurationArn) {
this.autoScalingConfigurationArn = autoScalingConfigurationArn;
}
/**
*
* The Amazon Resource Name (ARN) of this auto scaling configuration.
*
*
* @return The Amazon Resource Name (ARN) of this auto scaling configuration.
*/
public String getAutoScalingConfigurationArn() {
return this.autoScalingConfigurationArn;
}
/**
*
* The Amazon Resource Name (ARN) of this auto scaling configuration.
*
*
* @param autoScalingConfigurationArn
* The Amazon Resource Name (ARN) of this auto scaling configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingConfiguration withAutoScalingConfigurationArn(String autoScalingConfigurationArn) {
setAutoScalingConfigurationArn(autoScalingConfigurationArn);
return this;
}
/**
*
* The customer-provided auto scaling configuration name. It can be used in multiple revisions of a configuration.
*
*
* @param autoScalingConfigurationName
* The customer-provided auto scaling configuration name. It can be used in multiple revisions of a
* configuration.
*/
public void setAutoScalingConfigurationName(String autoScalingConfigurationName) {
this.autoScalingConfigurationName = autoScalingConfigurationName;
}
/**
*
* The customer-provided auto scaling configuration name. It can be used in multiple revisions of a configuration.
*
*
* @return The customer-provided auto scaling configuration name. It can be used in multiple revisions of a
* configuration.
*/
public String getAutoScalingConfigurationName() {
return this.autoScalingConfigurationName;
}
/**
*
* The customer-provided auto scaling configuration name. It can be used in multiple revisions of a configuration.
*
*
* @param autoScalingConfigurationName
* The customer-provided auto scaling configuration name. It can be used in multiple revisions of a
* configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingConfiguration withAutoScalingConfigurationName(String autoScalingConfigurationName) {
setAutoScalingConfigurationName(autoScalingConfigurationName);
return this;
}
/**
*
* The revision of this auto scaling configuration. It's unique among all the active configurations (
* "Status": "ACTIVE"
) that share the same AutoScalingConfigurationName
.
*
*
* @param autoScalingConfigurationRevision
* The revision of this auto scaling configuration. It's unique among all the active configurations (
* "Status": "ACTIVE"
) that share the same AutoScalingConfigurationName
.
*/
public void setAutoScalingConfigurationRevision(Integer autoScalingConfigurationRevision) {
this.autoScalingConfigurationRevision = autoScalingConfigurationRevision;
}
/**
*
* The revision of this auto scaling configuration. It's unique among all the active configurations (
* "Status": "ACTIVE"
) that share the same AutoScalingConfigurationName
.
*
*
* @return The revision of this auto scaling configuration. It's unique among all the active configurations (
* "Status": "ACTIVE"
) that share the same AutoScalingConfigurationName
.
*/
public Integer getAutoScalingConfigurationRevision() {
return this.autoScalingConfigurationRevision;
}
/**
*
* The revision of this auto scaling configuration. It's unique among all the active configurations (
* "Status": "ACTIVE"
) that share the same AutoScalingConfigurationName
.
*
*
* @param autoScalingConfigurationRevision
* The revision of this auto scaling configuration. It's unique among all the active configurations (
* "Status": "ACTIVE"
) that share the same AutoScalingConfigurationName
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingConfiguration withAutoScalingConfigurationRevision(Integer autoScalingConfigurationRevision) {
setAutoScalingConfigurationRevision(autoScalingConfigurationRevision);
return this;
}
/**
*
* It's set to true
for the configuration with the highest Revision
among all
* configurations that share the same AutoScalingConfigurationName
. It's set to false
* otherwise.
*
*
* @param latest
* It's set to true
for the configuration with the highest Revision
among all
* configurations that share the same AutoScalingConfigurationName
. It's set to
* false
otherwise.
*/
public void setLatest(Boolean latest) {
this.latest = latest;
}
/**
*
* It's set to true
for the configuration with the highest Revision
among all
* configurations that share the same AutoScalingConfigurationName
. It's set to false
* otherwise.
*
*
* @return It's set to true
for the configuration with the highest Revision
among all
* configurations that share the same AutoScalingConfigurationName
. It's set to
* false
otherwise.
*/
public Boolean getLatest() {
return this.latest;
}
/**
*
* It's set to true
for the configuration with the highest Revision
among all
* configurations that share the same AutoScalingConfigurationName
. It's set to false
* otherwise.
*
*
* @param latest
* It's set to true
for the configuration with the highest Revision
among all
* configurations that share the same AutoScalingConfigurationName
. It's set to
* false
otherwise.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingConfiguration withLatest(Boolean latest) {
setLatest(latest);
return this;
}
/**
*
* It's set to true
for the configuration with the highest Revision
among all
* configurations that share the same AutoScalingConfigurationName
. It's set to false
* otherwise.
*
*
* @return It's set to true
for the configuration with the highest Revision
among all
* configurations that share the same AutoScalingConfigurationName
. It's set to
* false
otherwise.
*/
public Boolean isLatest() {
return this.latest;
}
/**
*
* The current state of the auto scaling configuration. If the status of a configuration revision is
* INACTIVE
, it was deleted and can't be used. Inactive configuration revisions are permanently removed
* some time after they are deleted.
*
*
* @param status
* The current state of the auto scaling configuration. If the status of a configuration revision is
* INACTIVE
, it was deleted and can't be used. Inactive configuration revisions are permanently
* removed some time after they are deleted.
* @see AutoScalingConfigurationStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The current state of the auto scaling configuration. If the status of a configuration revision is
* INACTIVE
, it was deleted and can't be used. Inactive configuration revisions are permanently removed
* some time after they are deleted.
*
*
* @return The current state of the auto scaling configuration. If the status of a configuration revision is
* INACTIVE
, it was deleted and can't be used. Inactive configuration revisions are permanently
* removed some time after they are deleted.
* @see AutoScalingConfigurationStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The current state of the auto scaling configuration. If the status of a configuration revision is
* INACTIVE
, it was deleted and can't be used. Inactive configuration revisions are permanently removed
* some time after they are deleted.
*
*
* @param status
* The current state of the auto scaling configuration. If the status of a configuration revision is
* INACTIVE
, it was deleted and can't be used. Inactive configuration revisions are permanently
* removed some time after they are deleted.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AutoScalingConfigurationStatus
*/
public AutoScalingConfiguration withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The current state of the auto scaling configuration. If the status of a configuration revision is
* INACTIVE
, it was deleted and can't be used. Inactive configuration revisions are permanently removed
* some time after they are deleted.
*
*
* @param status
* The current state of the auto scaling configuration. If the status of a configuration revision is
* INACTIVE
, it was deleted and can't be used. Inactive configuration revisions are permanently
* removed some time after they are deleted.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AutoScalingConfigurationStatus
*/
public AutoScalingConfiguration withStatus(AutoScalingConfigurationStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The maximum number of concurrent requests that an instance processes. If the number of concurrent requests
* exceeds this limit, App Runner scales the service up.
*
*
* @param maxConcurrency
* The maximum number of concurrent requests that an instance processes. If the number of concurrent requests
* exceeds this limit, App Runner scales the service up.
*/
public void setMaxConcurrency(Integer maxConcurrency) {
this.maxConcurrency = maxConcurrency;
}
/**
*
* The maximum number of concurrent requests that an instance processes. If the number of concurrent requests
* exceeds this limit, App Runner scales the service up.
*
*
* @return The maximum number of concurrent requests that an instance processes. If the number of concurrent
* requests exceeds this limit, App Runner scales the service up.
*/
public Integer getMaxConcurrency() {
return this.maxConcurrency;
}
/**
*
* The maximum number of concurrent requests that an instance processes. If the number of concurrent requests
* exceeds this limit, App Runner scales the service up.
*
*
* @param maxConcurrency
* The maximum number of concurrent requests that an instance processes. If the number of concurrent requests
* exceeds this limit, App Runner scales the service up.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingConfiguration withMaxConcurrency(Integer maxConcurrency) {
setMaxConcurrency(maxConcurrency);
return this;
}
/**
*
* The minimum number of instances that App Runner provisions for a service. The service always has at least
* MinSize
provisioned instances. Some of them actively serve traffic. The rest of them (provisioned
* and inactive instances) are a cost-effective compute capacity reserve and are ready to be quickly activated. You
* pay for memory usage of all the provisioned instances. You pay for CPU usage of only the active subset.
*
*
* App Runner temporarily doubles the number of provisioned instances during deployments, to maintain the same
* capacity for both old and new code.
*
*
* @param minSize
* The minimum number of instances that App Runner provisions for a service. The service always has at least
* MinSize
provisioned instances. Some of them actively serve traffic. The rest of them
* (provisioned and inactive instances) are a cost-effective compute capacity reserve and are ready to be
* quickly activated. You pay for memory usage of all the provisioned instances. You pay for CPU usage of
* only the active subset.
*
* App Runner temporarily doubles the number of provisioned instances during deployments, to maintain the
* same capacity for both old and new code.
*/
public void setMinSize(Integer minSize) {
this.minSize = minSize;
}
/**
*
* The minimum number of instances that App Runner provisions for a service. The service always has at least
* MinSize
provisioned instances. Some of them actively serve traffic. The rest of them (provisioned
* and inactive instances) are a cost-effective compute capacity reserve and are ready to be quickly activated. You
* pay for memory usage of all the provisioned instances. You pay for CPU usage of only the active subset.
*
*
* App Runner temporarily doubles the number of provisioned instances during deployments, to maintain the same
* capacity for both old and new code.
*
*
* @return The minimum number of instances that App Runner provisions for a service. The service always has at least
* MinSize
provisioned instances. Some of them actively serve traffic. The rest of them
* (provisioned and inactive instances) are a cost-effective compute capacity reserve and are ready to be
* quickly activated. You pay for memory usage of all the provisioned instances. You pay for CPU usage of
* only the active subset.
*
* App Runner temporarily doubles the number of provisioned instances during deployments, to maintain the
* same capacity for both old and new code.
*/
public Integer getMinSize() {
return this.minSize;
}
/**
*
* The minimum number of instances that App Runner provisions for a service. The service always has at least
* MinSize
provisioned instances. Some of them actively serve traffic. The rest of them (provisioned
* and inactive instances) are a cost-effective compute capacity reserve and are ready to be quickly activated. You
* pay for memory usage of all the provisioned instances. You pay for CPU usage of only the active subset.
*
*
* App Runner temporarily doubles the number of provisioned instances during deployments, to maintain the same
* capacity for both old and new code.
*
*
* @param minSize
* The minimum number of instances that App Runner provisions for a service. The service always has at least
* MinSize
provisioned instances. Some of them actively serve traffic. The rest of them
* (provisioned and inactive instances) are a cost-effective compute capacity reserve and are ready to be
* quickly activated. You pay for memory usage of all the provisioned instances. You pay for CPU usage of
* only the active subset.
*
* App Runner temporarily doubles the number of provisioned instances during deployments, to maintain the
* same capacity for both old and new code.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingConfiguration withMinSize(Integer minSize) {
setMinSize(minSize);
return this;
}
/**
*
* The maximum number of instances that a service scales up to. At most MaxSize
instances actively
* serve traffic for your service.
*
*
* @param maxSize
* The maximum number of instances that a service scales up to. At most MaxSize
instances
* actively serve traffic for your service.
*/
public void setMaxSize(Integer maxSize) {
this.maxSize = maxSize;
}
/**
*
* The maximum number of instances that a service scales up to. At most MaxSize
instances actively
* serve traffic for your service.
*
*
* @return The maximum number of instances that a service scales up to. At most MaxSize
instances
* actively serve traffic for your service.
*/
public Integer getMaxSize() {
return this.maxSize;
}
/**
*
* The maximum number of instances that a service scales up to. At most MaxSize
instances actively
* serve traffic for your service.
*
*
* @param maxSize
* The maximum number of instances that a service scales up to. At most MaxSize
instances
* actively serve traffic for your service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingConfiguration withMaxSize(Integer maxSize) {
setMaxSize(maxSize);
return this;
}
/**
*
* The time when the auto scaling configuration was created. It's in Unix time stamp format.
*
*
* @param createdAt
* The time when the auto scaling configuration was created. It's in Unix time stamp format.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The time when the auto scaling configuration was created. It's in Unix time stamp format.
*
*
* @return The time when the auto scaling configuration was created. It's in Unix time stamp format.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The time when the auto scaling configuration was created. It's in Unix time stamp format.
*
*
* @param createdAt
* The time when the auto scaling configuration was created. It's in Unix time stamp format.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingConfiguration withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The time when the auto scaling configuration was deleted. It's in Unix time stamp format.
*
*
* @param deletedAt
* The time when the auto scaling configuration was deleted. It's in Unix time stamp format.
*/
public void setDeletedAt(java.util.Date deletedAt) {
this.deletedAt = deletedAt;
}
/**
*
* The time when the auto scaling configuration was deleted. It's in Unix time stamp format.
*
*
* @return The time when the auto scaling configuration was deleted. It's in Unix time stamp format.
*/
public java.util.Date getDeletedAt() {
return this.deletedAt;
}
/**
*
* The time when the auto scaling configuration was deleted. It's in Unix time stamp format.
*
*
* @param deletedAt
* The time when the auto scaling configuration was deleted. It's in Unix time stamp format.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingConfiguration withDeletedAt(java.util.Date deletedAt) {
setDeletedAt(deletedAt);
return this;
}
/**
*
* Indicates if this auto scaling configuration has an App Runner service associated with it. A value of
* true
indicates one or more services are associated. A value of false
indicates no
* services are associated.
*
*
* @param hasAssociatedService
* Indicates if this auto scaling configuration has an App Runner service associated with it. A value of
* true
indicates one or more services are associated. A value of false
indicates
* no services are associated.
*/
public void setHasAssociatedService(Boolean hasAssociatedService) {
this.hasAssociatedService = hasAssociatedService;
}
/**
*
* Indicates if this auto scaling configuration has an App Runner service associated with it. A value of
* true
indicates one or more services are associated. A value of false
indicates no
* services are associated.
*
*
* @return Indicates if this auto scaling configuration has an App Runner service associated with it. A value of
* true
indicates one or more services are associated. A value of false
indicates
* no services are associated.
*/
public Boolean getHasAssociatedService() {
return this.hasAssociatedService;
}
/**
*
* Indicates if this auto scaling configuration has an App Runner service associated with it. A value of
* true
indicates one or more services are associated. A value of false
indicates no
* services are associated.
*
*
* @param hasAssociatedService
* Indicates if this auto scaling configuration has an App Runner service associated with it. A value of
* true
indicates one or more services are associated. A value of false
indicates
* no services are associated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingConfiguration withHasAssociatedService(Boolean hasAssociatedService) {
setHasAssociatedService(hasAssociatedService);
return this;
}
/**
*
* Indicates if this auto scaling configuration has an App Runner service associated with it. A value of
* true
indicates one or more services are associated. A value of false
indicates no
* services are associated.
*
*
* @return Indicates if this auto scaling configuration has an App Runner service associated with it. A value of
* true
indicates one or more services are associated. A value of false
indicates
* no services are associated.
*/
public Boolean isHasAssociatedService() {
return this.hasAssociatedService;
}
/**
*
* Indicates if this auto scaling configuration should be used as the default for a new App Runner service that does
* not have an auto scaling configuration ARN specified during creation. Each account can have only one default
* AutoScalingConfiguration
per region. The default AutoScalingConfiguration
can be any
* revision under the same AutoScalingConfigurationName
.
*
*
* @param isDefault
* Indicates if this auto scaling configuration should be used as the default for a new App Runner service
* that does not have an auto scaling configuration ARN specified during creation. Each account can have only
* one default AutoScalingConfiguration
per region. The default
* AutoScalingConfiguration
can be any revision under the same
* AutoScalingConfigurationName
.
*/
public void setIsDefault(Boolean isDefault) {
this.isDefault = isDefault;
}
/**
*
* Indicates if this auto scaling configuration should be used as the default for a new App Runner service that does
* not have an auto scaling configuration ARN specified during creation. Each account can have only one default
* AutoScalingConfiguration
per region. The default AutoScalingConfiguration
can be any
* revision under the same AutoScalingConfigurationName
.
*
*
* @return Indicates if this auto scaling configuration should be used as the default for a new App Runner service
* that does not have an auto scaling configuration ARN specified during creation. Each account can have
* only one default AutoScalingConfiguration
per region. The default
* AutoScalingConfiguration
can be any revision under the same
* AutoScalingConfigurationName
.
*/
public Boolean getIsDefault() {
return this.isDefault;
}
/**
*
* Indicates if this auto scaling configuration should be used as the default for a new App Runner service that does
* not have an auto scaling configuration ARN specified during creation. Each account can have only one default
* AutoScalingConfiguration
per region. The default AutoScalingConfiguration
can be any
* revision under the same AutoScalingConfigurationName
.
*
*
* @param isDefault
* Indicates if this auto scaling configuration should be used as the default for a new App Runner service
* that does not have an auto scaling configuration ARN specified during creation. Each account can have only
* one default AutoScalingConfiguration
per region. The default
* AutoScalingConfiguration
can be any revision under the same
* AutoScalingConfigurationName
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AutoScalingConfiguration withIsDefault(Boolean isDefault) {
setIsDefault(isDefault);
return this;
}
/**
*
* Indicates if this auto scaling configuration should be used as the default for a new App Runner service that does
* not have an auto scaling configuration ARN specified during creation. Each account can have only one default
* AutoScalingConfiguration
per region. The default AutoScalingConfiguration
can be any
* revision under the same AutoScalingConfigurationName
.
*
*
* @return Indicates if this auto scaling configuration should be used as the default for a new App Runner service
* that does not have an auto scaling configuration ARN specified during creation. Each account can have
* only one default AutoScalingConfiguration
per region. The default
* AutoScalingConfiguration
can be any revision under the same
* AutoScalingConfigurationName
.
*/
public Boolean isDefault() {
return this.isDefault;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAutoScalingConfigurationArn() != null)
sb.append("AutoScalingConfigurationArn: ").append(getAutoScalingConfigurationArn()).append(",");
if (getAutoScalingConfigurationName() != null)
sb.append("AutoScalingConfigurationName: ").append(getAutoScalingConfigurationName()).append(",");
if (getAutoScalingConfigurationRevision() != null)
sb.append("AutoScalingConfigurationRevision: ").append(getAutoScalingConfigurationRevision()).append(",");
if (getLatest() != null)
sb.append("Latest: ").append(getLatest()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getMaxConcurrency() != null)
sb.append("MaxConcurrency: ").append(getMaxConcurrency()).append(",");
if (getMinSize() != null)
sb.append("MinSize: ").append(getMinSize()).append(",");
if (getMaxSize() != null)
sb.append("MaxSize: ").append(getMaxSize()).append(",");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getDeletedAt() != null)
sb.append("DeletedAt: ").append(getDeletedAt()).append(",");
if (getHasAssociatedService() != null)
sb.append("HasAssociatedService: ").append(getHasAssociatedService()).append(",");
if (getIsDefault() != null)
sb.append("IsDefault: ").append(getIsDefault());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AutoScalingConfiguration == false)
return false;
AutoScalingConfiguration other = (AutoScalingConfiguration) obj;
if (other.getAutoScalingConfigurationArn() == null ^ this.getAutoScalingConfigurationArn() == null)
return false;
if (other.getAutoScalingConfigurationArn() != null && other.getAutoScalingConfigurationArn().equals(this.getAutoScalingConfigurationArn()) == false)
return false;
if (other.getAutoScalingConfigurationName() == null ^ this.getAutoScalingConfigurationName() == null)
return false;
if (other.getAutoScalingConfigurationName() != null && other.getAutoScalingConfigurationName().equals(this.getAutoScalingConfigurationName()) == false)
return false;
if (other.getAutoScalingConfigurationRevision() == null ^ this.getAutoScalingConfigurationRevision() == null)
return false;
if (other.getAutoScalingConfigurationRevision() != null
&& other.getAutoScalingConfigurationRevision().equals(this.getAutoScalingConfigurationRevision()) == false)
return false;
if (other.getLatest() == null ^ this.getLatest() == null)
return false;
if (other.getLatest() != null && other.getLatest().equals(this.getLatest()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getMaxConcurrency() == null ^ this.getMaxConcurrency() == null)
return false;
if (other.getMaxConcurrency() != null && other.getMaxConcurrency().equals(this.getMaxConcurrency()) == false)
return false;
if (other.getMinSize() == null ^ this.getMinSize() == null)
return false;
if (other.getMinSize() != null && other.getMinSize().equals(this.getMinSize()) == false)
return false;
if (other.getMaxSize() == null ^ this.getMaxSize() == null)
return false;
if (other.getMaxSize() != null && other.getMaxSize().equals(this.getMaxSize()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getDeletedAt() == null ^ this.getDeletedAt() == null)
return false;
if (other.getDeletedAt() != null && other.getDeletedAt().equals(this.getDeletedAt()) == false)
return false;
if (other.getHasAssociatedService() == null ^ this.getHasAssociatedService() == null)
return false;
if (other.getHasAssociatedService() != null && other.getHasAssociatedService().equals(this.getHasAssociatedService()) == false)
return false;
if (other.getIsDefault() == null ^ this.getIsDefault() == null)
return false;
if (other.getIsDefault() != null && other.getIsDefault().equals(this.getIsDefault()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAutoScalingConfigurationArn() == null) ? 0 : getAutoScalingConfigurationArn().hashCode());
hashCode = prime * hashCode + ((getAutoScalingConfigurationName() == null) ? 0 : getAutoScalingConfigurationName().hashCode());
hashCode = prime * hashCode + ((getAutoScalingConfigurationRevision() == null) ? 0 : getAutoScalingConfigurationRevision().hashCode());
hashCode = prime * hashCode + ((getLatest() == null) ? 0 : getLatest().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getMaxConcurrency() == null) ? 0 : getMaxConcurrency().hashCode());
hashCode = prime * hashCode + ((getMinSize() == null) ? 0 : getMinSize().hashCode());
hashCode = prime * hashCode + ((getMaxSize() == null) ? 0 : getMaxSize().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getDeletedAt() == null) ? 0 : getDeletedAt().hashCode());
hashCode = prime * hashCode + ((getHasAssociatedService() == null) ? 0 : getHasAssociatedService().hashCode());
hashCode = prime * hashCode + ((getIsDefault() == null) ? 0 : getIsDefault().hashCode());
return hashCode;
}
@Override
public AutoScalingConfiguration clone() {
try {
return (AutoScalingConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.apprunner.model.transform.AutoScalingConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}