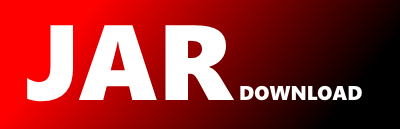
com.amazonaws.services.apprunner.model.ImageConfiguration Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.apprunner.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes the configuration that App Runner uses to run an App Runner service using an image pulled from a source
* image repository.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ImageConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* Environment variables that are available to your running App Runner service. An array of key-value pairs.
*
*/
private java.util.Map runtimeEnvironmentVariables;
/**
*
* An optional command that App Runner runs to start the application in the source image. If specified, this command
* overrides the Docker image’s default start command.
*
*/
private String startCommand;
/**
*
* The port that your application listens to in the container.
*
*
* Default: 8080
*
*/
private String port;
/**
*
* An array of key-value pairs representing the secrets and parameters that get referenced to your service as an
* environment variable. The supported values are either the full Amazon Resource Name (ARN) of the Secrets Manager
* secret or the full ARN of the parameter in the Amazon Web Services Systems Manager Parameter Store.
*
*
*
* -
*
* If the Amazon Web Services Systems Manager Parameter Store parameter exists in the same Amazon Web Services
* Region as the service that you're launching, you can use either the full ARN or name of the secret. If the
* parameter exists in a different Region, then the full ARN must be specified.
*
*
* -
*
* Currently, cross account referencing of Amazon Web Services Systems Manager Parameter Store parameter is not
* supported.
*
*
*
*
*/
private java.util.Map runtimeEnvironmentSecrets;
/**
*
* Environment variables that are available to your running App Runner service. An array of key-value pairs.
*
*
* @return Environment variables that are available to your running App Runner service. An array of key-value pairs.
*/
public java.util.Map getRuntimeEnvironmentVariables() {
return runtimeEnvironmentVariables;
}
/**
*
* Environment variables that are available to your running App Runner service. An array of key-value pairs.
*
*
* @param runtimeEnvironmentVariables
* Environment variables that are available to your running App Runner service. An array of key-value pairs.
*/
public void setRuntimeEnvironmentVariables(java.util.Map runtimeEnvironmentVariables) {
this.runtimeEnvironmentVariables = runtimeEnvironmentVariables;
}
/**
*
* Environment variables that are available to your running App Runner service. An array of key-value pairs.
*
*
* @param runtimeEnvironmentVariables
* Environment variables that are available to your running App Runner service. An array of key-value pairs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageConfiguration withRuntimeEnvironmentVariables(java.util.Map runtimeEnvironmentVariables) {
setRuntimeEnvironmentVariables(runtimeEnvironmentVariables);
return this;
}
/**
* Add a single RuntimeEnvironmentVariables entry
*
* @see ImageConfiguration#withRuntimeEnvironmentVariables
* @returns a reference to this object so that method calls can be chained together.
*/
public ImageConfiguration addRuntimeEnvironmentVariablesEntry(String key, String value) {
if (null == this.runtimeEnvironmentVariables) {
this.runtimeEnvironmentVariables = new java.util.HashMap();
}
if (this.runtimeEnvironmentVariables.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.runtimeEnvironmentVariables.put(key, value);
return this;
}
/**
* Removes all the entries added into RuntimeEnvironmentVariables.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageConfiguration clearRuntimeEnvironmentVariablesEntries() {
this.runtimeEnvironmentVariables = null;
return this;
}
/**
*
* An optional command that App Runner runs to start the application in the source image. If specified, this command
* overrides the Docker image’s default start command.
*
*
* @param startCommand
* An optional command that App Runner runs to start the application in the source image. If specified, this
* command overrides the Docker image’s default start command.
*/
public void setStartCommand(String startCommand) {
this.startCommand = startCommand;
}
/**
*
* An optional command that App Runner runs to start the application in the source image. If specified, this command
* overrides the Docker image’s default start command.
*
*
* @return An optional command that App Runner runs to start the application in the source image. If specified, this
* command overrides the Docker image’s default start command.
*/
public String getStartCommand() {
return this.startCommand;
}
/**
*
* An optional command that App Runner runs to start the application in the source image. If specified, this command
* overrides the Docker image’s default start command.
*
*
* @param startCommand
* An optional command that App Runner runs to start the application in the source image. If specified, this
* command overrides the Docker image’s default start command.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageConfiguration withStartCommand(String startCommand) {
setStartCommand(startCommand);
return this;
}
/**
*
* The port that your application listens to in the container.
*
*
* Default: 8080
*
*
* @param port
* The port that your application listens to in the container.
*
* Default: 8080
*/
public void setPort(String port) {
this.port = port;
}
/**
*
* The port that your application listens to in the container.
*
*
* Default: 8080
*
*
* @return The port that your application listens to in the container.
*
* Default: 8080
*/
public String getPort() {
return this.port;
}
/**
*
* The port that your application listens to in the container.
*
*
* Default: 8080
*
*
* @param port
* The port that your application listens to in the container.
*
* Default: 8080
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageConfiguration withPort(String port) {
setPort(port);
return this;
}
/**
*
* An array of key-value pairs representing the secrets and parameters that get referenced to your service as an
* environment variable. The supported values are either the full Amazon Resource Name (ARN) of the Secrets Manager
* secret or the full ARN of the parameter in the Amazon Web Services Systems Manager Parameter Store.
*
*
*
* -
*
* If the Amazon Web Services Systems Manager Parameter Store parameter exists in the same Amazon Web Services
* Region as the service that you're launching, you can use either the full ARN or name of the secret. If the
* parameter exists in a different Region, then the full ARN must be specified.
*
*
* -
*
* Currently, cross account referencing of Amazon Web Services Systems Manager Parameter Store parameter is not
* supported.
*
*
*
*
*
* @return An array of key-value pairs representing the secrets and parameters that get referenced to your service
* as an environment variable. The supported values are either the full Amazon Resource Name (ARN) of the
* Secrets Manager secret or the full ARN of the parameter in the Amazon Web Services Systems Manager
* Parameter Store.
*
* -
*
* If the Amazon Web Services Systems Manager Parameter Store parameter exists in the same Amazon Web
* Services Region as the service that you're launching, you can use either the full ARN or name of the
* secret. If the parameter exists in a different Region, then the full ARN must be specified.
*
*
* -
*
* Currently, cross account referencing of Amazon Web Services Systems Manager Parameter Store parameter is
* not supported.
*
*
*
*/
public java.util.Map getRuntimeEnvironmentSecrets() {
return runtimeEnvironmentSecrets;
}
/**
*
* An array of key-value pairs representing the secrets and parameters that get referenced to your service as an
* environment variable. The supported values are either the full Amazon Resource Name (ARN) of the Secrets Manager
* secret or the full ARN of the parameter in the Amazon Web Services Systems Manager Parameter Store.
*
*
*
* -
*
* If the Amazon Web Services Systems Manager Parameter Store parameter exists in the same Amazon Web Services
* Region as the service that you're launching, you can use either the full ARN or name of the secret. If the
* parameter exists in a different Region, then the full ARN must be specified.
*
*
* -
*
* Currently, cross account referencing of Amazon Web Services Systems Manager Parameter Store parameter is not
* supported.
*
*
*
*
*
* @param runtimeEnvironmentSecrets
* An array of key-value pairs representing the secrets and parameters that get referenced to your service as
* an environment variable. The supported values are either the full Amazon Resource Name (ARN) of the
* Secrets Manager secret or the full ARN of the parameter in the Amazon Web Services Systems Manager
* Parameter Store.
*
* -
*
* If the Amazon Web Services Systems Manager Parameter Store parameter exists in the same Amazon Web
* Services Region as the service that you're launching, you can use either the full ARN or name of the
* secret. If the parameter exists in a different Region, then the full ARN must be specified.
*
*
* -
*
* Currently, cross account referencing of Amazon Web Services Systems Manager Parameter Store parameter is
* not supported.
*
*
*
*/
public void setRuntimeEnvironmentSecrets(java.util.Map runtimeEnvironmentSecrets) {
this.runtimeEnvironmentSecrets = runtimeEnvironmentSecrets;
}
/**
*
* An array of key-value pairs representing the secrets and parameters that get referenced to your service as an
* environment variable. The supported values are either the full Amazon Resource Name (ARN) of the Secrets Manager
* secret or the full ARN of the parameter in the Amazon Web Services Systems Manager Parameter Store.
*
*
*
* -
*
* If the Amazon Web Services Systems Manager Parameter Store parameter exists in the same Amazon Web Services
* Region as the service that you're launching, you can use either the full ARN or name of the secret. If the
* parameter exists in a different Region, then the full ARN must be specified.
*
*
* -
*
* Currently, cross account referencing of Amazon Web Services Systems Manager Parameter Store parameter is not
* supported.
*
*
*
*
*
* @param runtimeEnvironmentSecrets
* An array of key-value pairs representing the secrets and parameters that get referenced to your service as
* an environment variable. The supported values are either the full Amazon Resource Name (ARN) of the
* Secrets Manager secret or the full ARN of the parameter in the Amazon Web Services Systems Manager
* Parameter Store.
*
* -
*
* If the Amazon Web Services Systems Manager Parameter Store parameter exists in the same Amazon Web
* Services Region as the service that you're launching, you can use either the full ARN or name of the
* secret. If the parameter exists in a different Region, then the full ARN must be specified.
*
*
* -
*
* Currently, cross account referencing of Amazon Web Services Systems Manager Parameter Store parameter is
* not supported.
*
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageConfiguration withRuntimeEnvironmentSecrets(java.util.Map runtimeEnvironmentSecrets) {
setRuntimeEnvironmentSecrets(runtimeEnvironmentSecrets);
return this;
}
/**
* Add a single RuntimeEnvironmentSecrets entry
*
* @see ImageConfiguration#withRuntimeEnvironmentSecrets
* @returns a reference to this object so that method calls can be chained together.
*/
public ImageConfiguration addRuntimeEnvironmentSecretsEntry(String key, String value) {
if (null == this.runtimeEnvironmentSecrets) {
this.runtimeEnvironmentSecrets = new java.util.HashMap();
}
if (this.runtimeEnvironmentSecrets.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.runtimeEnvironmentSecrets.put(key, value);
return this;
}
/**
* Removes all the entries added into RuntimeEnvironmentSecrets.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageConfiguration clearRuntimeEnvironmentSecretsEntries() {
this.runtimeEnvironmentSecrets = null;
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getRuntimeEnvironmentVariables() != null)
sb.append("RuntimeEnvironmentVariables: ").append("***Sensitive Data Redacted***").append(",");
if (getStartCommand() != null)
sb.append("StartCommand: ").append("***Sensitive Data Redacted***").append(",");
if (getPort() != null)
sb.append("Port: ").append(getPort()).append(",");
if (getRuntimeEnvironmentSecrets() != null)
sb.append("RuntimeEnvironmentSecrets: ").append("***Sensitive Data Redacted***");
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ImageConfiguration == false)
return false;
ImageConfiguration other = (ImageConfiguration) obj;
if (other.getRuntimeEnvironmentVariables() == null ^ this.getRuntimeEnvironmentVariables() == null)
return false;
if (other.getRuntimeEnvironmentVariables() != null && other.getRuntimeEnvironmentVariables().equals(this.getRuntimeEnvironmentVariables()) == false)
return false;
if (other.getStartCommand() == null ^ this.getStartCommand() == null)
return false;
if (other.getStartCommand() != null && other.getStartCommand().equals(this.getStartCommand()) == false)
return false;
if (other.getPort() == null ^ this.getPort() == null)
return false;
if (other.getPort() != null && other.getPort().equals(this.getPort()) == false)
return false;
if (other.getRuntimeEnvironmentSecrets() == null ^ this.getRuntimeEnvironmentSecrets() == null)
return false;
if (other.getRuntimeEnvironmentSecrets() != null && other.getRuntimeEnvironmentSecrets().equals(this.getRuntimeEnvironmentSecrets()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getRuntimeEnvironmentVariables() == null) ? 0 : getRuntimeEnvironmentVariables().hashCode());
hashCode = prime * hashCode + ((getStartCommand() == null) ? 0 : getStartCommand().hashCode());
hashCode = prime * hashCode + ((getPort() == null) ? 0 : getPort().hashCode());
hashCode = prime * hashCode + ((getRuntimeEnvironmentSecrets() == null) ? 0 : getRuntimeEnvironmentSecrets().hashCode());
return hashCode;
}
@Override
public ImageConfiguration clone() {
try {
return (ImageConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.apprunner.model.transform.ImageConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}