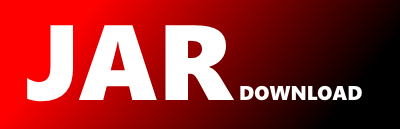
com.amazonaws.services.apprunner.model.InstanceConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-apprunner Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.apprunner.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes the runtime configuration of an App Runner service instance (scaling unit).
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class InstanceConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* The number of CPU units reserved for each instance of your App Runner service.
*
*
* Default: 1 vCPU
*
*/
private String cpu;
/**
*
* The amount of memory, in MB or GB, reserved for each instance of your App Runner service.
*
*
* Default: 2 GB
*
*/
private String memory;
/**
*
* The Amazon Resource Name (ARN) of an IAM role that provides permissions to your App Runner service. These are
* permissions that your code needs when it calls any Amazon Web Services APIs.
*
*/
private String instanceRoleArn;
/**
*
* The number of CPU units reserved for each instance of your App Runner service.
*
*
* Default: 1 vCPU
*
*
* @param cpu
* The number of CPU units reserved for each instance of your App Runner service.
*
* Default: 1 vCPU
*/
public void setCpu(String cpu) {
this.cpu = cpu;
}
/**
*
* The number of CPU units reserved for each instance of your App Runner service.
*
*
* Default: 1 vCPU
*
*
* @return The number of CPU units reserved for each instance of your App Runner service.
*
* Default: 1 vCPU
*/
public String getCpu() {
return this.cpu;
}
/**
*
* The number of CPU units reserved for each instance of your App Runner service.
*
*
* Default: 1 vCPU
*
*
* @param cpu
* The number of CPU units reserved for each instance of your App Runner service.
*
* Default: 1 vCPU
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InstanceConfiguration withCpu(String cpu) {
setCpu(cpu);
return this;
}
/**
*
* The amount of memory, in MB or GB, reserved for each instance of your App Runner service.
*
*
* Default: 2 GB
*
*
* @param memory
* The amount of memory, in MB or GB, reserved for each instance of your App Runner service.
*
* Default: 2 GB
*/
public void setMemory(String memory) {
this.memory = memory;
}
/**
*
* The amount of memory, in MB or GB, reserved for each instance of your App Runner service.
*
*
* Default: 2 GB
*
*
* @return The amount of memory, in MB or GB, reserved for each instance of your App Runner service.
*
* Default: 2 GB
*/
public String getMemory() {
return this.memory;
}
/**
*
* The amount of memory, in MB or GB, reserved for each instance of your App Runner service.
*
*
* Default: 2 GB
*
*
* @param memory
* The amount of memory, in MB or GB, reserved for each instance of your App Runner service.
*
* Default: 2 GB
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InstanceConfiguration withMemory(String memory) {
setMemory(memory);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that provides permissions to your App Runner service. These are
* permissions that your code needs when it calls any Amazon Web Services APIs.
*
*
* @param instanceRoleArn
* The Amazon Resource Name (ARN) of an IAM role that provides permissions to your App Runner service. These
* are permissions that your code needs when it calls any Amazon Web Services APIs.
*/
public void setInstanceRoleArn(String instanceRoleArn) {
this.instanceRoleArn = instanceRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that provides permissions to your App Runner service. These are
* permissions that your code needs when it calls any Amazon Web Services APIs.
*
*
* @return The Amazon Resource Name (ARN) of an IAM role that provides permissions to your App Runner service. These
* are permissions that your code needs when it calls any Amazon Web Services APIs.
*/
public String getInstanceRoleArn() {
return this.instanceRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that provides permissions to your App Runner service. These are
* permissions that your code needs when it calls any Amazon Web Services APIs.
*
*
* @param instanceRoleArn
* The Amazon Resource Name (ARN) of an IAM role that provides permissions to your App Runner service. These
* are permissions that your code needs when it calls any Amazon Web Services APIs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InstanceConfiguration withInstanceRoleArn(String instanceRoleArn) {
setInstanceRoleArn(instanceRoleArn);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCpu() != null)
sb.append("Cpu: ").append(getCpu()).append(",");
if (getMemory() != null)
sb.append("Memory: ").append(getMemory()).append(",");
if (getInstanceRoleArn() != null)
sb.append("InstanceRoleArn: ").append(getInstanceRoleArn());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof InstanceConfiguration == false)
return false;
InstanceConfiguration other = (InstanceConfiguration) obj;
if (other.getCpu() == null ^ this.getCpu() == null)
return false;
if (other.getCpu() != null && other.getCpu().equals(this.getCpu()) == false)
return false;
if (other.getMemory() == null ^ this.getMemory() == null)
return false;
if (other.getMemory() != null && other.getMemory().equals(this.getMemory()) == false)
return false;
if (other.getInstanceRoleArn() == null ^ this.getInstanceRoleArn() == null)
return false;
if (other.getInstanceRoleArn() != null && other.getInstanceRoleArn().equals(this.getInstanceRoleArn()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCpu() == null) ? 0 : getCpu().hashCode());
hashCode = prime * hashCode + ((getMemory() == null) ? 0 : getMemory().hashCode());
hashCode = prime * hashCode + ((getInstanceRoleArn() == null) ? 0 : getInstanceRoleArn().hashCode());
return hashCode;
}
@Override
public InstanceConfiguration clone() {
try {
return (InstanceConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.apprunner.model.transform.InstanceConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}