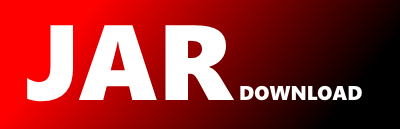
com.amazonaws.services.appstream.AmazonAppStreamAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appstream Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appstream;
import javax.annotation.Generated;
import com.amazonaws.services.appstream.model.*;
/**
* Interface for accessing Amazon AppStream asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.appstream.AbstractAmazonAppStreamAsync} instead.
*
*
* Amazon AppStream 2.0
*
* API documentation for Amazon AppStream 2.0.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonAppStreamAsync extends AmazonAppStream {
/**
*
* Associate a fleet to a stack.
*
*
* @param associateFleetRequest
* @return A Java Future containing the result of the AssociateFleet operation returned by the service.
* @sample AmazonAppStreamAsync.AssociateFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateFleetAsync(AssociateFleetRequest associateFleetRequest);
/**
*
* Associate a fleet to a stack.
*
*
* @param associateFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateFleet operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.AssociateFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateFleetAsync(AssociateFleetRequest associateFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new fleet.
*
*
* @param createFleetRequest
* Contains the parameters for the new fleet to create.
* @return A Java Future containing the result of the CreateFleet operation returned by the service.
* @sample AmazonAppStreamAsync.CreateFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFleetAsync(CreateFleetRequest createFleetRequest);
/**
*
* Creates a new fleet.
*
*
* @param createFleetRequest
* Contains the parameters for the new fleet to create.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFleet operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.CreateFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFleetAsync(CreateFleetRequest createFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create a new stack.
*
*
* @param createStackRequest
* @return A Java Future containing the result of the CreateStack operation returned by the service.
* @sample AmazonAppStreamAsync.CreateStack
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createStackAsync(CreateStackRequest createStackRequest);
/**
*
* Create a new stack.
*
*
* @param createStackRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateStack operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.CreateStack
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createStackAsync(CreateStackRequest createStackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a URL to start an AppStream 2.0 streaming session for a user. By default, the URL is valid only for 1
* minute from the time that it is generated.
*
*
* @param createStreamingURLRequest
* @return A Java Future containing the result of the CreateStreamingURL operation returned by the service.
* @sample AmazonAppStreamAsync.CreateStreamingURL
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createStreamingURLAsync(CreateStreamingURLRequest createStreamingURLRequest);
/**
*
* Creates a URL to start an AppStream 2.0 streaming session for a user. By default, the URL is valid only for 1
* minute from the time that it is generated.
*
*
* @param createStreamingURLRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateStreamingURL operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.CreateStreamingURL
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createStreamingURLAsync(CreateStreamingURLRequest createStreamingURLRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a fleet.
*
*
* @param deleteFleetRequest
* @return A Java Future containing the result of the DeleteFleet operation returned by the service.
* @sample AmazonAppStreamAsync.DeleteFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFleetAsync(DeleteFleetRequest deleteFleetRequest);
/**
*
* Deletes a fleet.
*
*
* @param deleteFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFleet operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DeleteFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFleetAsync(DeleteFleetRequest deleteFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the stack. After this operation completes, the environment can no longer be activated, and any
* reservations made for the stack are released.
*
*
* @param deleteStackRequest
* @return A Java Future containing the result of the DeleteStack operation returned by the service.
* @sample AmazonAppStreamAsync.DeleteStack
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteStackAsync(DeleteStackRequest deleteStackRequest);
/**
*
* Deletes the stack. After this operation completes, the environment can no longer be activated, and any
* reservations made for the stack are released.
*
*
* @param deleteStackRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteStack operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DeleteStack
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteStackAsync(DeleteStackRequest deleteStackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* If fleet names are provided, this operation describes the specified fleets; otherwise, all the fleets in the
* account are described.
*
*
* @param describeFleetsRequest
* @return A Java Future containing the result of the DescribeFleets operation returned by the service.
* @sample AmazonAppStreamAsync.DescribeFleets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeFleetsAsync(DescribeFleetsRequest describeFleetsRequest);
/**
*
* If fleet names are provided, this operation describes the specified fleets; otherwise, all the fleets in the
* account are described.
*
*
* @param describeFleetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFleets operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DescribeFleets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeFleetsAsync(DescribeFleetsRequest describeFleetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the images. If a list of names is not provided, all images in your account are returned. This operation
* does not return a paginated result.
*
*
* @param describeImagesRequest
* @return A Java Future containing the result of the DescribeImages operation returned by the service.
* @sample AmazonAppStreamAsync.DescribeImages
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeImagesAsync(DescribeImagesRequest describeImagesRequest);
/**
*
* Describes the images. If a list of names is not provided, all images in your account are returned. This operation
* does not return a paginated result.
*
*
* @param describeImagesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeImages operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DescribeImages
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeImagesAsync(DescribeImagesRequest describeImagesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the streaming sessions for a stack and a fleet. If a user ID is provided, this operation returns
* streaming sessions for only that user. Pass this value for the nextToken
parameter in a subsequent
* call to this operation to retrieve the next set of items. If an authentication type is not provided, the
* operation defaults to users authenticated using a streaming URL.
*
*
* @param describeSessionsRequest
* @return A Java Future containing the result of the DescribeSessions operation returned by the service.
* @sample AmazonAppStreamAsync.DescribeSessions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSessionsAsync(DescribeSessionsRequest describeSessionsRequest);
/**
*
* Describes the streaming sessions for a stack and a fleet. If a user ID is provided, this operation returns
* streaming sessions for only that user. Pass this value for the nextToken
parameter in a subsequent
* call to this operation to retrieve the next set of items. If an authentication type is not provided, the
* operation defaults to users authenticated using a streaming URL.
*
*
* @param describeSessionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSessions operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DescribeSessions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSessionsAsync(DescribeSessionsRequest describeSessionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* If stack names are not provided, this operation describes the specified stacks; otherwise, all stacks in the
* account are described. Pass the nextToken
value in a subsequent call to this operation to retrieve
* the next set of items.
*
*
* @param describeStacksRequest
* @return A Java Future containing the result of the DescribeStacks operation returned by the service.
* @sample AmazonAppStreamAsync.DescribeStacks
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeStacksAsync(DescribeStacksRequest describeStacksRequest);
/**
*
* If stack names are not provided, this operation describes the specified stacks; otherwise, all stacks in the
* account are described. Pass the nextToken
value in a subsequent call to this operation to retrieve
* the next set of items.
*
*
* @param describeStacksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeStacks operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DescribeStacks
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeStacksAsync(DescribeStacksRequest describeStacksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates a fleet from a stack.
*
*
* @param disassociateFleetRequest
* @return A Java Future containing the result of the DisassociateFleet operation returned by the service.
* @sample AmazonAppStreamAsync.DisassociateFleet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future disassociateFleetAsync(DisassociateFleetRequest disassociateFleetRequest);
/**
*
* Disassociates a fleet from a stack.
*
*
* @param disassociateFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateFleet operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DisassociateFleet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future disassociateFleetAsync(DisassociateFleetRequest disassociateFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation immediately stops a streaming session.
*
*
* @param expireSessionRequest
* @return A Java Future containing the result of the ExpireSession operation returned by the service.
* @sample AmazonAppStreamAsync.ExpireSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future expireSessionAsync(ExpireSessionRequest expireSessionRequest);
/**
*
* This operation immediately stops a streaming session.
*
*
* @param expireSessionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ExpireSession operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.ExpireSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future expireSessionAsync(ExpireSessionRequest expireSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all fleets associated with the stack.
*
*
* @param listAssociatedFleetsRequest
* @return A Java Future containing the result of the ListAssociatedFleets operation returned by the service.
* @sample AmazonAppStreamAsync.ListAssociatedFleets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAssociatedFleetsAsync(ListAssociatedFleetsRequest listAssociatedFleetsRequest);
/**
*
* Lists all fleets associated with the stack.
*
*
* @param listAssociatedFleetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAssociatedFleets operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.ListAssociatedFleets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAssociatedFleetsAsync(ListAssociatedFleetsRequest listAssociatedFleetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all stacks to which the specified fleet is associated.
*
*
* @param listAssociatedStacksRequest
* @return A Java Future containing the result of the ListAssociatedStacks operation returned by the service.
* @sample AmazonAppStreamAsync.ListAssociatedStacks
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAssociatedStacksAsync(ListAssociatedStacksRequest listAssociatedStacksRequest);
/**
*
* Lists all stacks to which the specified fleet is associated.
*
*
* @param listAssociatedStacksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAssociatedStacks operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.ListAssociatedStacks
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAssociatedStacksAsync(ListAssociatedStacksRequest listAssociatedStacksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts a fleet.
*
*
* @param startFleetRequest
* @return A Java Future containing the result of the StartFleet operation returned by the service.
* @sample AmazonAppStreamAsync.StartFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startFleetAsync(StartFleetRequest startFleetRequest);
/**
*
* Starts a fleet.
*
*
* @param startFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartFleet operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.StartFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startFleetAsync(StartFleetRequest startFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops a fleet.
*
*
* @param stopFleetRequest
* @return A Java Future containing the result of the StopFleet operation returned by the service.
* @sample AmazonAppStreamAsync.StopFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopFleetAsync(StopFleetRequest stopFleetRequest);
/**
*
* Stops a fleet.
*
*
* @param stopFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopFleet operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.StopFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopFleetAsync(StopFleetRequest stopFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing fleet. All the attributes except the fleet name can be updated in the STOPPED state.
* When a fleet is in the RUNNING state, only DisplayName
and ComputeCapacity
can
* be updated. A fleet cannot be updated in a status of STARTING or STOPPING.
*
*
* @param updateFleetRequest
* @return A Java Future containing the result of the UpdateFleet operation returned by the service.
* @sample AmazonAppStreamAsync.UpdateFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateFleetAsync(UpdateFleetRequest updateFleetRequest);
/**
*
* Updates an existing fleet. All the attributes except the fleet name can be updated in the STOPPED state.
* When a fleet is in the RUNNING state, only DisplayName
and ComputeCapacity
can
* be updated. A fleet cannot be updated in a status of STARTING or STOPPING.
*
*
* @param updateFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateFleet operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.UpdateFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateFleetAsync(UpdateFleetRequest updateFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified fields in the stack with the specified name.
*
*
* @param updateStackRequest
* @return A Java Future containing the result of the UpdateStack operation returned by the service.
* @sample AmazonAppStreamAsync.UpdateStack
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateStackAsync(UpdateStackRequest updateStackRequest);
/**
*
* Updates the specified fields in the stack with the specified name.
*
*
* @param updateStackRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateStack operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.UpdateStack
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateStackAsync(UpdateStackRequest updateStackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}