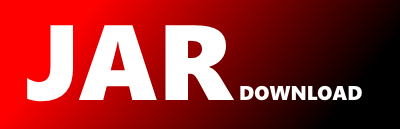
com.amazonaws.services.appstream.AmazonAppStreamClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appstream Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appstream;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.appstream.AmazonAppStreamClientBuilder;
import com.amazonaws.services.appstream.waiters.AmazonAppStreamWaiters;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.appstream.model.*;
import com.amazonaws.services.appstream.model.transform.*;
/**
* Client for accessing Amazon AppStream. All service calls made using this client are blocking, and will not return
* until the service call completes.
*
* Amazon AppStream 2.0
*
* This is the Amazon AppStream 2.0 API Reference. This documentation provides descriptions and syntax for each
* of the actions and data types in AppStream 2.0. AppStream 2.0 is a fully managed, secure application streaming
* service that lets you stream desktop applications to users without rewriting applications. AppStream 2.0 manages the
* AWS resources that are required to host and run your applications, scales automatically, and provides access to your
* users on demand.
*
*
*
* You can call the AppStream 2.0 API operations by using an interface VPC endpoint (interface endpoint). For more
* information, see Access AppStream 2.0 API Operations and CLI Commands Through an Interface VPC Endpoint in the Amazon
* AppStream 2.0 Administration Guide.
*
*
*
* To learn more about AppStream 2.0, see the following resources:
*
*
* -
*
*
* -
*
*
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonAppStreamClient extends AmazonWebServiceClient implements AmazonAppStream {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonAppStream.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "appstream";
private volatile AmazonAppStreamWaiters waiters;
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConcurrentModificationException").withExceptionUnmarshaller(
com.amazonaws.services.appstream.model.transform.ConcurrentModificationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("RequestLimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.appstream.model.transform.RequestLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceInUseException").withExceptionUnmarshaller(
com.amazonaws.services.appstream.model.transform.ResourceInUseExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("IncompatibleImageException").withExceptionUnmarshaller(
com.amazonaws.services.appstream.model.transform.IncompatibleImageExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotAvailableException").withExceptionUnmarshaller(
com.amazonaws.services.appstream.model.transform.ResourceNotAvailableExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidAccountStatusException").withExceptionUnmarshaller(
com.amazonaws.services.appstream.model.transform.InvalidAccountStatusExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("OperationNotPermittedException").withExceptionUnmarshaller(
com.amazonaws.services.appstream.model.transform.OperationNotPermittedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("LimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.appstream.model.transform.LimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.appstream.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidRoleException").withExceptionUnmarshaller(
com.amazonaws.services.appstream.model.transform.InvalidRoleExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidParameterCombinationException").withExceptionUnmarshaller(
com.amazonaws.services.appstream.model.transform.InvalidParameterCombinationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceAlreadyExistsException").withExceptionUnmarshaller(
com.amazonaws.services.appstream.model.transform.ResourceAlreadyExistsExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.appstream.model.AmazonAppStreamException.class));
/**
* Constructs a new client to invoke service methods on Amazon AppStream. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AmazonAppStreamClientBuilder#defaultClient()}
*/
@Deprecated
public AmazonAppStreamClient() {
this(DefaultAWSCredentialsProviderChain.getInstance(), configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Amazon AppStream. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client connects to Amazon AppStream (ex: proxy
* settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AmazonAppStreamClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonAppStreamClient(ClientConfiguration clientConfiguration) {
this(DefaultAWSCredentialsProviderChain.getInstance(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on Amazon AppStream using the specified AWS account
* credentials.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @deprecated use {@link AmazonAppStreamClientBuilder#withCredentials(AWSCredentialsProvider)} for example:
* {@code AmazonAppStreamClientBuilder.standard().withCredentials(new AWSStaticCredentialsProvider(awsCredentials)).build();}
*/
@Deprecated
public AmazonAppStreamClient(AWSCredentials awsCredentials) {
this(awsCredentials, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Amazon AppStream using the specified AWS account credentials
* and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to Amazon AppStream (ex: proxy
* settings, retry counts, etc.).
* @deprecated use {@link AmazonAppStreamClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonAppStreamClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonAppStreamClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
/**
* Constructs a new client to invoke service methods on Amazon AppStream using the specified AWS account credentials
* provider.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @deprecated use {@link AmazonAppStreamClientBuilder#withCredentials(AWSCredentialsProvider)}
*/
@Deprecated
public AmazonAppStreamClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Amazon AppStream using the specified AWS account credentials
* provider and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to Amazon AppStream (ex: proxy
* settings, retry counts, etc.).
* @deprecated use {@link AmazonAppStreamClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonAppStreamClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonAppStreamClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on Amazon AppStream using the specified AWS account credentials
* provider, client configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to Amazon AppStream (ex: proxy
* settings, retry counts, etc.).
* @param requestMetricCollector
* optional request metric collector
* @deprecated use {@link AmazonAppStreamClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonAppStreamClientBuilder#withClientConfiguration(ClientConfiguration)} and
* {@link AmazonAppStreamClientBuilder#withMetricsCollector(RequestMetricCollector)}
*/
@Deprecated
public AmazonAppStreamClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
public static AmazonAppStreamClientBuilder builder() {
return AmazonAppStreamClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Amazon AppStream using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonAppStreamClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on Amazon AppStream using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonAppStreamClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("appstream2.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/appstream/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/appstream/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Associates the specified fleet with the specified stack.
*
*
* @param associateFleetRequest
* @return Result of the AssociateFleet operation returned by the service.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws IncompatibleImageException
* The image does not support storage connectors.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @sample AmazonAppStream.AssociateFleet
* @see AWS API
* Documentation
*/
@Override
public AssociateFleetResult associateFleet(AssociateFleetRequest request) {
request = beforeClientExecution(request);
return executeAssociateFleet(request);
}
@SdkInternalApi
final AssociateFleetResult executeAssociateFleet(AssociateFleetRequest associateFleetRequest) {
ExecutionContext executionContext = createExecutionContext(associateFleetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateFleetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(associateFleetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateFleet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new AssociateFleetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates the specified users with the specified stacks. Users in a user pool cannot be assigned to stacks with
* fleets that are joined to an Active Directory domain.
*
*
* @param batchAssociateUserStackRequest
* @return Result of the BatchAssociateUserStack operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @sample AmazonAppStream.BatchAssociateUserStack
* @see AWS API Documentation
*/
@Override
public BatchAssociateUserStackResult batchAssociateUserStack(BatchAssociateUserStackRequest request) {
request = beforeClientExecution(request);
return executeBatchAssociateUserStack(request);
}
@SdkInternalApi
final BatchAssociateUserStackResult executeBatchAssociateUserStack(BatchAssociateUserStackRequest batchAssociateUserStackRequest) {
ExecutionContext executionContext = createExecutionContext(batchAssociateUserStackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchAssociateUserStackRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(batchAssociateUserStackRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchAssociateUserStack");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new BatchAssociateUserStackResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates the specified users from the specified stacks.
*
*
* @param batchDisassociateUserStackRequest
* @return Result of the BatchDisassociateUserStack operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @sample AmazonAppStream.BatchDisassociateUserStack
* @see AWS API Documentation
*/
@Override
public BatchDisassociateUserStackResult batchDisassociateUserStack(BatchDisassociateUserStackRequest request) {
request = beforeClientExecution(request);
return executeBatchDisassociateUserStack(request);
}
@SdkInternalApi
final BatchDisassociateUserStackResult executeBatchDisassociateUserStack(BatchDisassociateUserStackRequest batchDisassociateUserStackRequest) {
ExecutionContext executionContext = createExecutionContext(batchDisassociateUserStackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchDisassociateUserStackRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(batchDisassociateUserStackRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchDisassociateUserStack");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new BatchDisassociateUserStackResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Copies the image within the same region or to a new region within the same AWS account. Note that any tags you
* added to the image will not be copied.
*
*
* @param copyImageRequest
* @return Result of the CopyImage operation returned by the service.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws IncompatibleImageException
* The image does not support storage connectors.
* @sample AmazonAppStream.CopyImage
* @see AWS API
* Documentation
*/
@Override
public CopyImageResult copyImage(CopyImageRequest request) {
request = beforeClientExecution(request);
return executeCopyImage(request);
}
@SdkInternalApi
final CopyImageResult executeCopyImage(CopyImageRequest copyImageRequest) {
ExecutionContext executionContext = createExecutionContext(copyImageRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CopyImageRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(copyImageRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CopyImage");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new CopyImageResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a Directory Config object in AppStream 2.0. This object includes the configuration information required
* to join fleets and image builders to Microsoft Active Directory domains.
*
*
* @param createDirectoryConfigRequest
* @return Result of the CreateDirectoryConfig operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws InvalidRoleException
* The specified role is invalid.
* @sample AmazonAppStream.CreateDirectoryConfig
* @see AWS API Documentation
*/
@Override
public CreateDirectoryConfigResult createDirectoryConfig(CreateDirectoryConfigRequest request) {
request = beforeClientExecution(request);
return executeCreateDirectoryConfig(request);
}
@SdkInternalApi
final CreateDirectoryConfigResult executeCreateDirectoryConfig(CreateDirectoryConfigRequest createDirectoryConfigRequest) {
ExecutionContext executionContext = createExecutionContext(createDirectoryConfigRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDirectoryConfigRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createDirectoryConfigRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDirectoryConfig");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateDirectoryConfigResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a fleet. A fleet consists of streaming instances that run a specified image.
*
*
* @param createFleetRequest
* @return Result of the CreateFleet operation returned by the service.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws RequestLimitExceededException
* AppStream 2.0 can’t process the request right now because the Describe calls from your AWS account are
* being throttled by Amazon EC2. Try again later.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws InvalidRoleException
* The specified role is invalid.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws IncompatibleImageException
* The image does not support storage connectors.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @sample AmazonAppStream.CreateFleet
* @see AWS API
* Documentation
*/
@Override
public CreateFleetResult createFleet(CreateFleetRequest request) {
request = beforeClientExecution(request);
return executeCreateFleet(request);
}
@SdkInternalApi
final CreateFleetResult executeCreateFleet(CreateFleetRequest createFleetRequest) {
ExecutionContext executionContext = createExecutionContext(createFleetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateFleetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createFleetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateFleet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateFleetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an image builder. An image builder is a virtual machine that is used to create an image.
*
*
* The initial state of the builder is PENDING
. When it is ready, the state is RUNNING
.
*
*
* @param createImageBuilderRequest
* @return Result of the CreateImageBuilder operation returned by the service.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws RequestLimitExceededException
* AppStream 2.0 can’t process the request right now because the Describe calls from your AWS account are
* being throttled by Amazon EC2. Try again later.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidRoleException
* The specified role is invalid.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws IncompatibleImageException
* The image does not support storage connectors.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @sample AmazonAppStream.CreateImageBuilder
* @see AWS
* API Documentation
*/
@Override
public CreateImageBuilderResult createImageBuilder(CreateImageBuilderRequest request) {
request = beforeClientExecution(request);
return executeCreateImageBuilder(request);
}
@SdkInternalApi
final CreateImageBuilderResult executeCreateImageBuilder(CreateImageBuilderRequest createImageBuilderRequest) {
ExecutionContext executionContext = createExecutionContext(createImageBuilderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateImageBuilderRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createImageBuilderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateImageBuilder");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateImageBuilderResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a URL to start an image builder streaming session.
*
*
* @param createImageBuilderStreamingURLRequest
* @return Result of the CreateImageBuilderStreamingURL operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.CreateImageBuilderStreamingURL
* @see AWS API Documentation
*/
@Override
public CreateImageBuilderStreamingURLResult createImageBuilderStreamingURL(CreateImageBuilderStreamingURLRequest request) {
request = beforeClientExecution(request);
return executeCreateImageBuilderStreamingURL(request);
}
@SdkInternalApi
final CreateImageBuilderStreamingURLResult executeCreateImageBuilderStreamingURL(CreateImageBuilderStreamingURLRequest createImageBuilderStreamingURLRequest) {
ExecutionContext executionContext = createExecutionContext(createImageBuilderStreamingURLRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateImageBuilderStreamingURLRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createImageBuilderStreamingURLRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateImageBuilderStreamingURL");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateImageBuilderStreamingURLResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a stack to start streaming applications to users. A stack consists of an associated fleet, user access
* policies, and storage configurations.
*
*
* @param createStackRequest
* @return Result of the CreateStack operation returned by the service.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws InvalidRoleException
* The specified role is invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @sample AmazonAppStream.CreateStack
* @see AWS API
* Documentation
*/
@Override
public CreateStackResult createStack(CreateStackRequest request) {
request = beforeClientExecution(request);
return executeCreateStack(request);
}
@SdkInternalApi
final CreateStackResult executeCreateStack(CreateStackRequest createStackRequest) {
ExecutionContext executionContext = createExecutionContext(createStackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateStackRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createStackRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateStack");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateStackResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a temporary URL to start an AppStream 2.0 streaming session for the specified user. A streaming URL
* enables application streaming to be tested without user setup.
*
*
* @param createStreamingURLRequest
* @return Result of the CreateStreamingURL operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @sample AmazonAppStream.CreateStreamingURL
* @see AWS
* API Documentation
*/
@Override
public CreateStreamingURLResult createStreamingURL(CreateStreamingURLRequest request) {
request = beforeClientExecution(request);
return executeCreateStreamingURL(request);
}
@SdkInternalApi
final CreateStreamingURLResult executeCreateStreamingURL(CreateStreamingURLRequest createStreamingURLRequest) {
ExecutionContext executionContext = createExecutionContext(createStreamingURLRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateStreamingURLRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createStreamingURLRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateStreamingURL");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateStreamingURLResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a usage report subscription. Usage reports are generated daily.
*
*
* @param createUsageReportSubscriptionRequest
* @return Result of the CreateUsageReportSubscription operation returned by the service.
* @throws InvalidRoleException
* The specified role is invalid.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @sample AmazonAppStream.CreateUsageReportSubscription
* @see AWS API Documentation
*/
@Override
public CreateUsageReportSubscriptionResult createUsageReportSubscription(CreateUsageReportSubscriptionRequest request) {
request = beforeClientExecution(request);
return executeCreateUsageReportSubscription(request);
}
@SdkInternalApi
final CreateUsageReportSubscriptionResult executeCreateUsageReportSubscription(CreateUsageReportSubscriptionRequest createUsageReportSubscriptionRequest) {
ExecutionContext executionContext = createExecutionContext(createUsageReportSubscriptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateUsageReportSubscriptionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createUsageReportSubscriptionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateUsageReportSubscription");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateUsageReportSubscriptionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new user in the user pool.
*
*
* @param createUserRequest
* @return Result of the CreateUser operation returned by the service.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @sample AmazonAppStream.CreateUser
* @see AWS API
* Documentation
*/
@Override
public CreateUserResult createUser(CreateUserRequest request) {
request = beforeClientExecution(request);
return executeCreateUser(request);
}
@SdkInternalApi
final CreateUserResult executeCreateUser(CreateUserRequest createUserRequest) {
ExecutionContext executionContext = createExecutionContext(createUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateUserRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified Directory Config object from AppStream 2.0. This object includes the information required
* to join streaming instances to an Active Directory domain.
*
*
* @param deleteDirectoryConfigRequest
* @return Result of the DeleteDirectoryConfig operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DeleteDirectoryConfig
* @see AWS API Documentation
*/
@Override
public DeleteDirectoryConfigResult deleteDirectoryConfig(DeleteDirectoryConfigRequest request) {
request = beforeClientExecution(request);
return executeDeleteDirectoryConfig(request);
}
@SdkInternalApi
final DeleteDirectoryConfigResult executeDeleteDirectoryConfig(DeleteDirectoryConfigRequest deleteDirectoryConfigRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDirectoryConfigRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDirectoryConfigRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteDirectoryConfigRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDirectoryConfig");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteDirectoryConfigResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified fleet.
*
*
* @param deleteFleetRequest
* @return Result of the DeleteFleet operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @sample AmazonAppStream.DeleteFleet
* @see AWS API
* Documentation
*/
@Override
public DeleteFleetResult deleteFleet(DeleteFleetRequest request) {
request = beforeClientExecution(request);
return executeDeleteFleet(request);
}
@SdkInternalApi
final DeleteFleetResult executeDeleteFleet(DeleteFleetRequest deleteFleetRequest) {
ExecutionContext executionContext = createExecutionContext(deleteFleetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteFleetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteFleetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteFleet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteFleetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified image. You cannot delete an image when it is in use. After you delete an image, you cannot
* provision new capacity using the image.
*
*
* @param deleteImageRequest
* @return Result of the DeleteImage operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @sample AmazonAppStream.DeleteImage
* @see AWS API
* Documentation
*/
@Override
public DeleteImageResult deleteImage(DeleteImageRequest request) {
request = beforeClientExecution(request);
return executeDeleteImage(request);
}
@SdkInternalApi
final DeleteImageResult executeDeleteImage(DeleteImageRequest deleteImageRequest) {
ExecutionContext executionContext = createExecutionContext(deleteImageRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteImageRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteImageRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteImage");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteImageResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified image builder and releases the capacity.
*
*
* @param deleteImageBuilderRequest
* @return Result of the DeleteImageBuilder operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @sample AmazonAppStream.DeleteImageBuilder
* @see AWS
* API Documentation
*/
@Override
public DeleteImageBuilderResult deleteImageBuilder(DeleteImageBuilderRequest request) {
request = beforeClientExecution(request);
return executeDeleteImageBuilder(request);
}
@SdkInternalApi
final DeleteImageBuilderResult executeDeleteImageBuilder(DeleteImageBuilderRequest deleteImageBuilderRequest) {
ExecutionContext executionContext = createExecutionContext(deleteImageBuilderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteImageBuilderRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteImageBuilderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteImageBuilder");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteImageBuilderResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes permissions for the specified private image. After you delete permissions for an image, AWS accounts to
* which you previously granted these permissions can no longer use the image.
*
*
* @param deleteImagePermissionsRequest
* @return Result of the DeleteImagePermissions operation returned by the service.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DeleteImagePermissions
* @see AWS API Documentation
*/
@Override
public DeleteImagePermissionsResult deleteImagePermissions(DeleteImagePermissionsRequest request) {
request = beforeClientExecution(request);
return executeDeleteImagePermissions(request);
}
@SdkInternalApi
final DeleteImagePermissionsResult executeDeleteImagePermissions(DeleteImagePermissionsRequest deleteImagePermissionsRequest) {
ExecutionContext executionContext = createExecutionContext(deleteImagePermissionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteImagePermissionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteImagePermissionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteImagePermissions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteImagePermissionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified stack. After the stack is deleted, the application streaming environment provided by the
* stack is no longer available to users. Also, any reservations made for application streaming sessions for the
* stack are released.
*
*
* @param deleteStackRequest
* @return Result of the DeleteStack operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @sample AmazonAppStream.DeleteStack
* @see AWS API
* Documentation
*/
@Override
public DeleteStackResult deleteStack(DeleteStackRequest request) {
request = beforeClientExecution(request);
return executeDeleteStack(request);
}
@SdkInternalApi
final DeleteStackResult executeDeleteStack(DeleteStackRequest deleteStackRequest) {
ExecutionContext executionContext = createExecutionContext(deleteStackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteStackRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteStackRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteStack");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteStackResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disables usage report generation.
*
*
* @param deleteUsageReportSubscriptionRequest
* @return Result of the DeleteUsageReportSubscription operation returned by the service.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DeleteUsageReportSubscription
* @see AWS API Documentation
*/
@Override
public DeleteUsageReportSubscriptionResult deleteUsageReportSubscription(DeleteUsageReportSubscriptionRequest request) {
request = beforeClientExecution(request);
return executeDeleteUsageReportSubscription(request);
}
@SdkInternalApi
final DeleteUsageReportSubscriptionResult executeDeleteUsageReportSubscription(DeleteUsageReportSubscriptionRequest deleteUsageReportSubscriptionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteUsageReportSubscriptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteUsageReportSubscriptionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteUsageReportSubscriptionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteUsageReportSubscription");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteUsageReportSubscriptionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a user from the user pool.
*
*
* @param deleteUserRequest
* @return Result of the DeleteUser operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DeleteUser
* @see AWS API
* Documentation
*/
@Override
public DeleteUserResult deleteUser(DeleteUserRequest request) {
request = beforeClientExecution(request);
return executeDeleteUser(request);
}
@SdkInternalApi
final DeleteUserResult executeDeleteUser(DeleteUserRequest deleteUserRequest) {
ExecutionContext executionContext = createExecutionContext(deleteUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteUserRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a list that describes one or more specified Directory Config objects for AppStream 2.0, if the names
* for these objects are provided. Otherwise, all Directory Config objects in the account are described. These
* objects include the configuration information required to join fleets and image builders to Microsoft Active
* Directory domains.
*
*
* Although the response syntax in this topic includes the account password, this password is not returned in the
* actual response.
*
*
* @param describeDirectoryConfigsRequest
* @return Result of the DescribeDirectoryConfigs operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DescribeDirectoryConfigs
* @see AWS API Documentation
*/
@Override
public DescribeDirectoryConfigsResult describeDirectoryConfigs(DescribeDirectoryConfigsRequest request) {
request = beforeClientExecution(request);
return executeDescribeDirectoryConfigs(request);
}
@SdkInternalApi
final DescribeDirectoryConfigsResult executeDescribeDirectoryConfigs(DescribeDirectoryConfigsRequest describeDirectoryConfigsRequest) {
ExecutionContext executionContext = createExecutionContext(describeDirectoryConfigsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDirectoryConfigsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeDirectoryConfigsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDirectoryConfigs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeDirectoryConfigsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a list that describes one or more specified fleets, if the fleet names are provided. Otherwise, all
* fleets in the account are described.
*
*
* @param describeFleetsRequest
* @return Result of the DescribeFleets operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DescribeFleets
* @see AWS API
* Documentation
*/
@Override
public DescribeFleetsResult describeFleets(DescribeFleetsRequest request) {
request = beforeClientExecution(request);
return executeDescribeFleets(request);
}
@SdkInternalApi
final DescribeFleetsResult executeDescribeFleets(DescribeFleetsRequest describeFleetsRequest) {
ExecutionContext executionContext = createExecutionContext(describeFleetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeFleetsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeFleetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeFleets");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeFleetsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a list that describes one or more specified image builders, if the image builder names are provided.
* Otherwise, all image builders in the account are described.
*
*
* @param describeImageBuildersRequest
* @return Result of the DescribeImageBuilders operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DescribeImageBuilders
* @see AWS API Documentation
*/
@Override
public DescribeImageBuildersResult describeImageBuilders(DescribeImageBuildersRequest request) {
request = beforeClientExecution(request);
return executeDescribeImageBuilders(request);
}
@SdkInternalApi
final DescribeImageBuildersResult executeDescribeImageBuilders(DescribeImageBuildersRequest describeImageBuildersRequest) {
ExecutionContext executionContext = createExecutionContext(describeImageBuildersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeImageBuildersRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeImageBuildersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeImageBuilders");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeImageBuildersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a list that describes the permissions for shared AWS account IDs on a private image that you own.
*
*
* @param describeImagePermissionsRequest
* @return Result of the DescribeImagePermissions operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DescribeImagePermissions
* @see AWS API Documentation
*/
@Override
public DescribeImagePermissionsResult describeImagePermissions(DescribeImagePermissionsRequest request) {
request = beforeClientExecution(request);
return executeDescribeImagePermissions(request);
}
@SdkInternalApi
final DescribeImagePermissionsResult executeDescribeImagePermissions(DescribeImagePermissionsRequest describeImagePermissionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeImagePermissionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeImagePermissionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeImagePermissionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeImagePermissions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeImagePermissionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a list that describes one or more specified images, if the image names or image ARNs are provided.
* Otherwise, all images in the account are described.
*
*
* @param describeImagesRequest
* @return Result of the DescribeImages operation returned by the service.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DescribeImages
* @see AWS API
* Documentation
*/
@Override
public DescribeImagesResult describeImages(DescribeImagesRequest request) {
request = beforeClientExecution(request);
return executeDescribeImages(request);
}
@SdkInternalApi
final DescribeImagesResult executeDescribeImages(DescribeImagesRequest describeImagesRequest) {
ExecutionContext executionContext = createExecutionContext(describeImagesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeImagesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeImagesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeImages");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeImagesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a list that describes the streaming sessions for a specified stack and fleet. If a UserId is provided
* for the stack and fleet, only streaming sessions for that user are described. If an authentication type is not
* provided, the default is to authenticate users using a streaming URL.
*
*
* @param describeSessionsRequest
* @return Result of the DescribeSessions operation returned by the service.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @sample AmazonAppStream.DescribeSessions
* @see AWS API
* Documentation
*/
@Override
public DescribeSessionsResult describeSessions(DescribeSessionsRequest request) {
request = beforeClientExecution(request);
return executeDescribeSessions(request);
}
@SdkInternalApi
final DescribeSessionsResult executeDescribeSessions(DescribeSessionsRequest describeSessionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeSessionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeSessionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeSessionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeSessions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeSessionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a list that describes one or more specified stacks, if the stack names are provided. Otherwise, all
* stacks in the account are described.
*
*
* @param describeStacksRequest
* @return Result of the DescribeStacks operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DescribeStacks
* @see AWS API
* Documentation
*/
@Override
public DescribeStacksResult describeStacks(DescribeStacksRequest request) {
request = beforeClientExecution(request);
return executeDescribeStacks(request);
}
@SdkInternalApi
final DescribeStacksResult executeDescribeStacks(DescribeStacksRequest describeStacksRequest) {
ExecutionContext executionContext = createExecutionContext(describeStacksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeStacksRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeStacksRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeStacks");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeStacksResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a list that describes one or more usage report subscriptions.
*
*
* @param describeUsageReportSubscriptionsRequest
* @return Result of the DescribeUsageReportSubscriptions operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @sample AmazonAppStream.DescribeUsageReportSubscriptions
* @see AWS API Documentation
*/
@Override
public DescribeUsageReportSubscriptionsResult describeUsageReportSubscriptions(DescribeUsageReportSubscriptionsRequest request) {
request = beforeClientExecution(request);
return executeDescribeUsageReportSubscriptions(request);
}
@SdkInternalApi
final DescribeUsageReportSubscriptionsResult executeDescribeUsageReportSubscriptions(
DescribeUsageReportSubscriptionsRequest describeUsageReportSubscriptionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeUsageReportSubscriptionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeUsageReportSubscriptionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeUsageReportSubscriptionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeUsageReportSubscriptions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeUsageReportSubscriptionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a list that describes the UserStackAssociation objects. You must specify either or both of the
* following:
*
*
* -
*
* The stack name
*
*
* -
*
* The user name (email address of the user associated with the stack) and the authentication type for the user
*
*
*
*
* @param describeUserStackAssociationsRequest
* @return Result of the DescribeUserStackAssociations operation returned by the service.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @sample AmazonAppStream.DescribeUserStackAssociations
* @see AWS API Documentation
*/
@Override
public DescribeUserStackAssociationsResult describeUserStackAssociations(DescribeUserStackAssociationsRequest request) {
request = beforeClientExecution(request);
return executeDescribeUserStackAssociations(request);
}
@SdkInternalApi
final DescribeUserStackAssociationsResult executeDescribeUserStackAssociations(DescribeUserStackAssociationsRequest describeUserStackAssociationsRequest) {
ExecutionContext executionContext = createExecutionContext(describeUserStackAssociationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeUserStackAssociationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeUserStackAssociationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeUserStackAssociations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeUserStackAssociationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a list that describes one or more specified users in the user pool.
*
*
* @param describeUsersRequest
* @return Result of the DescribeUsers operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @sample AmazonAppStream.DescribeUsers
* @see AWS API
* Documentation
*/
@Override
public DescribeUsersResult describeUsers(DescribeUsersRequest request) {
request = beforeClientExecution(request);
return executeDescribeUsers(request);
}
@SdkInternalApi
final DescribeUsersResult executeDescribeUsers(DescribeUsersRequest describeUsersRequest) {
ExecutionContext executionContext = createExecutionContext(describeUsersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeUsersRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeUsersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeUsers");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeUsersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disables the specified user in the user pool. Users can't sign in to AppStream 2.0 until they are re-enabled.
* This action does not delete the user.
*
*
* @param disableUserRequest
* @return Result of the DisableUser operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DisableUser
* @see AWS API
* Documentation
*/
@Override
public DisableUserResult disableUser(DisableUserRequest request) {
request = beforeClientExecution(request);
return executeDisableUser(request);
}
@SdkInternalApi
final DisableUserResult executeDisableUser(DisableUserRequest disableUserRequest) {
ExecutionContext executionContext = createExecutionContext(disableUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisableUserRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(disableUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisableUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DisableUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates the specified fleet from the specified stack.
*
*
* @param disassociateFleetRequest
* @return Result of the DisassociateFleet operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @sample AmazonAppStream.DisassociateFleet
* @see AWS
* API Documentation
*/
@Override
public DisassociateFleetResult disassociateFleet(DisassociateFleetRequest request) {
request = beforeClientExecution(request);
return executeDisassociateFleet(request);
}
@SdkInternalApi
final DisassociateFleetResult executeDisassociateFleet(DisassociateFleetRequest disassociateFleetRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateFleetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateFleetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(disassociateFleetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateFleet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DisassociateFleetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables a user in the user pool. After being enabled, users can sign in to AppStream 2.0 and open applications
* from the stacks to which they are assigned.
*
*
* @param enableUserRequest
* @return Result of the EnableUser operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @sample AmazonAppStream.EnableUser
* @see AWS API
* Documentation
*/
@Override
public EnableUserResult enableUser(EnableUserRequest request) {
request = beforeClientExecution(request);
return executeEnableUser(request);
}
@SdkInternalApi
final EnableUserResult executeEnableUser(EnableUserRequest enableUserRequest) {
ExecutionContext executionContext = createExecutionContext(enableUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new EnableUserRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(enableUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "EnableUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new EnableUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Immediately stops the specified streaming session.
*
*
* @param expireSessionRequest
* @return Result of the ExpireSession operation returned by the service.
* @sample AmazonAppStream.ExpireSession
* @see AWS API
* Documentation
*/
@Override
public ExpireSessionResult expireSession(ExpireSessionRequest request) {
request = beforeClientExecution(request);
return executeExpireSession(request);
}
@SdkInternalApi
final ExpireSessionResult executeExpireSession(ExpireSessionRequest expireSessionRequest) {
ExecutionContext executionContext = createExecutionContext(expireSessionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ExpireSessionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(expireSessionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ExpireSession");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ExpireSessionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the name of the fleet that is associated with the specified stack.
*
*
* @param listAssociatedFleetsRequest
* @return Result of the ListAssociatedFleets operation returned by the service.
* @sample AmazonAppStream.ListAssociatedFleets
* @see AWS
* API Documentation
*/
@Override
public ListAssociatedFleetsResult listAssociatedFleets(ListAssociatedFleetsRequest request) {
request = beforeClientExecution(request);
return executeListAssociatedFleets(request);
}
@SdkInternalApi
final ListAssociatedFleetsResult executeListAssociatedFleets(ListAssociatedFleetsRequest listAssociatedFleetsRequest) {
ExecutionContext executionContext = createExecutionContext(listAssociatedFleetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAssociatedFleetsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listAssociatedFleetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAssociatedFleets");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListAssociatedFleetsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the name of the stack with which the specified fleet is associated.
*
*
* @param listAssociatedStacksRequest
* @return Result of the ListAssociatedStacks operation returned by the service.
* @sample AmazonAppStream.ListAssociatedStacks
* @see AWS
* API Documentation
*/
@Override
public ListAssociatedStacksResult listAssociatedStacks(ListAssociatedStacksRequest request) {
request = beforeClientExecution(request);
return executeListAssociatedStacks(request);
}
@SdkInternalApi
final ListAssociatedStacksResult executeListAssociatedStacks(ListAssociatedStacksRequest listAssociatedStacksRequest) {
ExecutionContext executionContext = createExecutionContext(listAssociatedStacksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAssociatedStacksRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listAssociatedStacksRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAssociatedStacks");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListAssociatedStacksResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a list of all tags for the specified AppStream 2.0 resource. You can tag AppStream 2.0 image builders,
* images, fleets, and stacks.
*
*
* For more information about tags, see Tagging Your Resources
* in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.ListTagsForResource
* @see AWS
* API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts the specified fleet.
*
*
* @param startFleetRequest
* @return Result of the StartFleet operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws RequestLimitExceededException
* AppStream 2.0 can’t process the request right now because the Describe calls from your AWS account are
* being throttled by Amazon EC2. Try again later.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws InvalidRoleException
* The specified role is invalid.
* @sample AmazonAppStream.StartFleet
* @see AWS API
* Documentation
*/
@Override
public StartFleetResult startFleet(StartFleetRequest request) {
request = beforeClientExecution(request);
return executeStartFleet(request);
}
@SdkInternalApi
final StartFleetResult executeStartFleet(StartFleetRequest startFleetRequest) {
ExecutionContext executionContext = createExecutionContext(startFleetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartFleetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startFleetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartFleet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new StartFleetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts the specified image builder.
*
*
* @param startImageBuilderRequest
* @return Result of the StartImageBuilder operation returned by the service.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws IncompatibleImageException
* The image does not support storage connectors.
* @sample AmazonAppStream.StartImageBuilder
* @see AWS
* API Documentation
*/
@Override
public StartImageBuilderResult startImageBuilder(StartImageBuilderRequest request) {
request = beforeClientExecution(request);
return executeStartImageBuilder(request);
}
@SdkInternalApi
final StartImageBuilderResult executeStartImageBuilder(StartImageBuilderRequest startImageBuilderRequest) {
ExecutionContext executionContext = createExecutionContext(startImageBuilderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartImageBuilderRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startImageBuilderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartImageBuilder");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StartImageBuilderResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Stops the specified fleet.
*
*
* @param stopFleetRequest
* @return Result of the StopFleet operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @sample AmazonAppStream.StopFleet
* @see AWS API
* Documentation
*/
@Override
public StopFleetResult stopFleet(StopFleetRequest request) {
request = beforeClientExecution(request);
return executeStopFleet(request);
}
@SdkInternalApi
final StopFleetResult executeStopFleet(StopFleetRequest stopFleetRequest) {
ExecutionContext executionContext = createExecutionContext(stopFleetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StopFleetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(stopFleetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StopFleet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new StopFleetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Stops the specified image builder.
*
*
* @param stopImageBuilderRequest
* @return Result of the StopImageBuilder operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @sample AmazonAppStream.StopImageBuilder
* @see AWS API
* Documentation
*/
@Override
public StopImageBuilderResult stopImageBuilder(StopImageBuilderRequest request) {
request = beforeClientExecution(request);
return executeStopImageBuilder(request);
}
@SdkInternalApi
final StopImageBuilderResult executeStopImageBuilder(StopImageBuilderRequest stopImageBuilderRequest) {
ExecutionContext executionContext = createExecutionContext(stopImageBuilderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StopImageBuilderRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(stopImageBuilderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StopImageBuilder");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StopImageBuilderResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds or overwrites one or more tags for the specified AppStream 2.0 resource. You can tag AppStream 2.0 image
* builders, images, fleets, and stacks.
*
*
* Each tag consists of a key and an optional value. If a resource already has a tag with the same key, this
* operation updates its value.
*
*
* To list the current tags for your resources, use ListTagsForResource. To disassociate tags from your
* resources, use UntagResource.
*
*
* For more information about tags, see Tagging Your Resources
* in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates one or more specified tags from the specified AppStream 2.0 resource.
*
*
* To list the current tags for your resources, use ListTagsForResource.
*
*
* For more information about tags, see Tagging Your Resources
* in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the specified Directory Config object in AppStream 2.0. This object includes the configuration
* information required to join fleets and image builders to Microsoft Active Directory domains.
*
*
* @param updateDirectoryConfigRequest
* @return Result of the UpdateDirectoryConfig operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws InvalidRoleException
* The specified role is invalid.
* @sample AmazonAppStream.UpdateDirectoryConfig
* @see AWS API Documentation
*/
@Override
public UpdateDirectoryConfigResult updateDirectoryConfig(UpdateDirectoryConfigRequest request) {
request = beforeClientExecution(request);
return executeUpdateDirectoryConfig(request);
}
@SdkInternalApi
final UpdateDirectoryConfigResult executeUpdateDirectoryConfig(UpdateDirectoryConfigRequest updateDirectoryConfigRequest) {
ExecutionContext executionContext = createExecutionContext(updateDirectoryConfigRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateDirectoryConfigRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateDirectoryConfigRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateDirectoryConfig");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateDirectoryConfigResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the specified fleet.
*
*
* If the fleet is in the STOPPED
state, you can update any attribute except the fleet name. If the
* fleet is in the RUNNING
state, you can update the DisplayName
,
* ComputeCapacity
, ImageARN
, ImageName
,
* IdleDisconnectTimeoutInSeconds
, and DisconnectTimeoutInSeconds
attributes. If the fleet
* is in the STARTING
or STOPPING
state, you can't update it.
*
*
* @param updateFleetRequest
* @return Result of the UpdateFleet operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws RequestLimitExceededException
* AppStream 2.0 can’t process the request right now because the Describe calls from your AWS account are
* being throttled by Amazon EC2. Try again later.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws InvalidRoleException
* The specified role is invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws IncompatibleImageException
* The image does not support storage connectors.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @sample AmazonAppStream.UpdateFleet
* @see AWS API
* Documentation
*/
@Override
public UpdateFleetResult updateFleet(UpdateFleetRequest request) {
request = beforeClientExecution(request);
return executeUpdateFleet(request);
}
@SdkInternalApi
final UpdateFleetResult executeUpdateFleet(UpdateFleetRequest updateFleetRequest) {
ExecutionContext executionContext = createExecutionContext(updateFleetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateFleetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateFleetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateFleet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateFleetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds or updates permissions for the specified private image.
*
*
* @param updateImagePermissionsRequest
* @return Result of the UpdateImagePermissions operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @sample AmazonAppStream.UpdateImagePermissions
* @see AWS API Documentation
*/
@Override
public UpdateImagePermissionsResult updateImagePermissions(UpdateImagePermissionsRequest request) {
request = beforeClientExecution(request);
return executeUpdateImagePermissions(request);
}
@SdkInternalApi
final UpdateImagePermissionsResult executeUpdateImagePermissions(UpdateImagePermissionsRequest updateImagePermissionsRequest) {
ExecutionContext executionContext = createExecutionContext(updateImagePermissionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateImagePermissionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateImagePermissionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateImagePermissions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateImagePermissionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the specified fields for the specified stack.
*
*
* @param updateStackRequest
* @return Result of the UpdateStack operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws InvalidRoleException
* The specified role is invalid.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws IncompatibleImageException
* The image does not support storage connectors.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @sample AmazonAppStream.UpdateStack
* @see AWS API
* Documentation
*/
@Override
public UpdateStackResult updateStack(UpdateStackRequest request) {
request = beforeClientExecution(request);
return executeUpdateStack(request);
}
@SdkInternalApi
final UpdateStackResult executeUpdateStack(UpdateStackRequest updateStackRequest) {
ExecutionContext executionContext = createExecutionContext(updateStackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateStackRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateStackRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppStream");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateStack");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateStackResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public AmazonAppStreamWaiters waiters() {
if (waiters == null) {
synchronized (this) {
if (waiters == null) {
waiters = new AmazonAppStreamWaiters(this);
}
}
}
return waiters;
}
@Override
public void shutdown() {
super.shutdown();
if (waiters != null) {
waiters.shutdown();
}
}
}