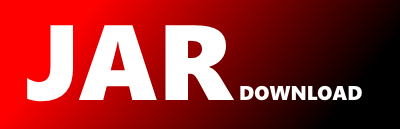
com.amazonaws.services.appstream.model.ImageBuilder Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appstream Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appstream.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes a virtual machine that is used to create an image.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ImageBuilder implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of the image builder.
*
*/
private String name;
/**
*
* The ARN for the image builder.
*
*/
private String arn;
/**
*
* The ARN of the image from which this builder was created.
*
*/
private String imageArn;
/**
*
* The description to display.
*
*/
private String description;
/**
*
* The image builder name to display.
*
*/
private String displayName;
/**
*
* The VPC configuration of the image builder.
*
*/
private VpcConfig vpcConfig;
/**
*
* The instance type for the image builder. The following instance types are available:
*
*
* -
*
* stream.standard.medium
*
*
* -
*
* stream.standard.large
*
*
* -
*
* stream.compute.large
*
*
* -
*
* stream.compute.xlarge
*
*
* -
*
* stream.compute.2xlarge
*
*
* -
*
* stream.compute.4xlarge
*
*
* -
*
* stream.compute.8xlarge
*
*
* -
*
* stream.memory.large
*
*
* -
*
* stream.memory.xlarge
*
*
* -
*
* stream.memory.2xlarge
*
*
* -
*
* stream.memory.4xlarge
*
*
* -
*
* stream.memory.8xlarge
*
*
* -
*
* stream.memory.z1d.large
*
*
* -
*
* stream.memory.z1d.xlarge
*
*
* -
*
* stream.memory.z1d.2xlarge
*
*
* -
*
* stream.memory.z1d.3xlarge
*
*
* -
*
* stream.memory.z1d.6xlarge
*
*
* -
*
* stream.memory.z1d.12xlarge
*
*
* -
*
* stream.graphics-design.large
*
*
* -
*
* stream.graphics-design.xlarge
*
*
* -
*
* stream.graphics-design.2xlarge
*
*
* -
*
* stream.graphics-design.4xlarge
*
*
* -
*
* stream.graphics-desktop.2xlarge
*
*
* -
*
* stream.graphics.g4dn.xlarge
*
*
* -
*
* stream.graphics.g4dn.2xlarge
*
*
* -
*
* stream.graphics.g4dn.4xlarge
*
*
* -
*
* stream.graphics.g4dn.8xlarge
*
*
* -
*
* stream.graphics.g4dn.12xlarge
*
*
* -
*
* stream.graphics.g4dn.16xlarge
*
*
* -
*
* stream.graphics-pro.4xlarge
*
*
* -
*
* stream.graphics-pro.8xlarge
*
*
* -
*
* stream.graphics-pro.16xlarge
*
*
*
*/
private String instanceType;
/**
*
* The operating system platform of the image builder.
*
*/
private String platform;
/**
*
* The ARN of the IAM role that is applied to the image builder. To assume a role, the image builder calls the AWS
* Security Token Service (STS) AssumeRole
API operation and passes the ARN of the role to use. The
* operation creates a new session with temporary credentials. AppStream 2.0 retrieves the temporary credentials and
* creates the appstream_machine_role credential profile on the instance.
*
*
* For more information, see Using an IAM Role to Grant Permissions to Applications and Scripts Running on AppStream 2.0 Streaming
* Instances in the Amazon AppStream 2.0 Administration Guide.
*
*/
private String iamRoleArn;
/**
*
* The state of the image builder.
*
*/
private String state;
/**
*
* The reason why the last state change occurred.
*
*/
private ImageBuilderStateChangeReason stateChangeReason;
/**
*
* The time stamp when the image builder was created.
*
*/
private java.util.Date createdTime;
/**
*
* Enables or disables default internet access for the image builder.
*
*/
private Boolean enableDefaultInternetAccess;
/**
*
* The name of the directory and organizational unit (OU) to use to join the image builder to a Microsoft Active
* Directory domain.
*
*/
private DomainJoinInfo domainJoinInfo;
private NetworkAccessConfiguration networkAccessConfiguration;
/**
*
* The image builder errors.
*
*/
private java.util.List imageBuilderErrors;
/**
*
* The version of the AppStream 2.0 agent that is currently being used by the image builder.
*
*/
private String appstreamAgentVersion;
/**
*
* The list of virtual private cloud (VPC) interface endpoint objects. Administrators can connect to the image
* builder only through the specified endpoints.
*
*/
private java.util.List accessEndpoints;
/**
*
* The name of the image builder.
*
*
* @param name
* The name of the image builder.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the image builder.
*
*
* @return The name of the image builder.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the image builder.
*
*
* @param name
* The name of the image builder.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageBuilder withName(String name) {
setName(name);
return this;
}
/**
*
* The ARN for the image builder.
*
*
* @param arn
* The ARN for the image builder.
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
*
* The ARN for the image builder.
*
*
* @return The ARN for the image builder.
*/
public String getArn() {
return this.arn;
}
/**
*
* The ARN for the image builder.
*
*
* @param arn
* The ARN for the image builder.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageBuilder withArn(String arn) {
setArn(arn);
return this;
}
/**
*
* The ARN of the image from which this builder was created.
*
*
* @param imageArn
* The ARN of the image from which this builder was created.
*/
public void setImageArn(String imageArn) {
this.imageArn = imageArn;
}
/**
*
* The ARN of the image from which this builder was created.
*
*
* @return The ARN of the image from which this builder was created.
*/
public String getImageArn() {
return this.imageArn;
}
/**
*
* The ARN of the image from which this builder was created.
*
*
* @param imageArn
* The ARN of the image from which this builder was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageBuilder withImageArn(String imageArn) {
setImageArn(imageArn);
return this;
}
/**
*
* The description to display.
*
*
* @param description
* The description to display.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The description to display.
*
*
* @return The description to display.
*/
public String getDescription() {
return this.description;
}
/**
*
* The description to display.
*
*
* @param description
* The description to display.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageBuilder withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The image builder name to display.
*
*
* @param displayName
* The image builder name to display.
*/
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
/**
*
* The image builder name to display.
*
*
* @return The image builder name to display.
*/
public String getDisplayName() {
return this.displayName;
}
/**
*
* The image builder name to display.
*
*
* @param displayName
* The image builder name to display.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageBuilder withDisplayName(String displayName) {
setDisplayName(displayName);
return this;
}
/**
*
* The VPC configuration of the image builder.
*
*
* @param vpcConfig
* The VPC configuration of the image builder.
*/
public void setVpcConfig(VpcConfig vpcConfig) {
this.vpcConfig = vpcConfig;
}
/**
*
* The VPC configuration of the image builder.
*
*
* @return The VPC configuration of the image builder.
*/
public VpcConfig getVpcConfig() {
return this.vpcConfig;
}
/**
*
* The VPC configuration of the image builder.
*
*
* @param vpcConfig
* The VPC configuration of the image builder.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageBuilder withVpcConfig(VpcConfig vpcConfig) {
setVpcConfig(vpcConfig);
return this;
}
/**
*
* The instance type for the image builder. The following instance types are available:
*
*
* -
*
* stream.standard.medium
*
*
* -
*
* stream.standard.large
*
*
* -
*
* stream.compute.large
*
*
* -
*
* stream.compute.xlarge
*
*
* -
*
* stream.compute.2xlarge
*
*
* -
*
* stream.compute.4xlarge
*
*
* -
*
* stream.compute.8xlarge
*
*
* -
*
* stream.memory.large
*
*
* -
*
* stream.memory.xlarge
*
*
* -
*
* stream.memory.2xlarge
*
*
* -
*
* stream.memory.4xlarge
*
*
* -
*
* stream.memory.8xlarge
*
*
* -
*
* stream.memory.z1d.large
*
*
* -
*
* stream.memory.z1d.xlarge
*
*
* -
*
* stream.memory.z1d.2xlarge
*
*
* -
*
* stream.memory.z1d.3xlarge
*
*
* -
*
* stream.memory.z1d.6xlarge
*
*
* -
*
* stream.memory.z1d.12xlarge
*
*
* -
*
* stream.graphics-design.large
*
*
* -
*
* stream.graphics-design.xlarge
*
*
* -
*
* stream.graphics-design.2xlarge
*
*
* -
*
* stream.graphics-design.4xlarge
*
*
* -
*
* stream.graphics-desktop.2xlarge
*
*
* -
*
* stream.graphics.g4dn.xlarge
*
*
* -
*
* stream.graphics.g4dn.2xlarge
*
*
* -
*
* stream.graphics.g4dn.4xlarge
*
*
* -
*
* stream.graphics.g4dn.8xlarge
*
*
* -
*
* stream.graphics.g4dn.12xlarge
*
*
* -
*
* stream.graphics.g4dn.16xlarge
*
*
* -
*
* stream.graphics-pro.4xlarge
*
*
* -
*
* stream.graphics-pro.8xlarge
*
*
* -
*
* stream.graphics-pro.16xlarge
*
*
*
*
* @param instanceType
* The instance type for the image builder. The following instance types are available:
*
* -
*
* stream.standard.medium
*
*
* -
*
* stream.standard.large
*
*
* -
*
* stream.compute.large
*
*
* -
*
* stream.compute.xlarge
*
*
* -
*
* stream.compute.2xlarge
*
*
* -
*
* stream.compute.4xlarge
*
*
* -
*
* stream.compute.8xlarge
*
*
* -
*
* stream.memory.large
*
*
* -
*
* stream.memory.xlarge
*
*
* -
*
* stream.memory.2xlarge
*
*
* -
*
* stream.memory.4xlarge
*
*
* -
*
* stream.memory.8xlarge
*
*
* -
*
* stream.memory.z1d.large
*
*
* -
*
* stream.memory.z1d.xlarge
*
*
* -
*
* stream.memory.z1d.2xlarge
*
*
* -
*
* stream.memory.z1d.3xlarge
*
*
* -
*
* stream.memory.z1d.6xlarge
*
*
* -
*
* stream.memory.z1d.12xlarge
*
*
* -
*
* stream.graphics-design.large
*
*
* -
*
* stream.graphics-design.xlarge
*
*
* -
*
* stream.graphics-design.2xlarge
*
*
* -
*
* stream.graphics-design.4xlarge
*
*
* -
*
* stream.graphics-desktop.2xlarge
*
*
* -
*
* stream.graphics.g4dn.xlarge
*
*
* -
*
* stream.graphics.g4dn.2xlarge
*
*
* -
*
* stream.graphics.g4dn.4xlarge
*
*
* -
*
* stream.graphics.g4dn.8xlarge
*
*
* -
*
* stream.graphics.g4dn.12xlarge
*
*
* -
*
* stream.graphics.g4dn.16xlarge
*
*
* -
*
* stream.graphics-pro.4xlarge
*
*
* -
*
* stream.graphics-pro.8xlarge
*
*
* -
*
* stream.graphics-pro.16xlarge
*
*
*/
public void setInstanceType(String instanceType) {
this.instanceType = instanceType;
}
/**
*
* The instance type for the image builder. The following instance types are available:
*
*
* -
*
* stream.standard.medium
*
*
* -
*
* stream.standard.large
*
*
* -
*
* stream.compute.large
*
*
* -
*
* stream.compute.xlarge
*
*
* -
*
* stream.compute.2xlarge
*
*
* -
*
* stream.compute.4xlarge
*
*
* -
*
* stream.compute.8xlarge
*
*
* -
*
* stream.memory.large
*
*
* -
*
* stream.memory.xlarge
*
*
* -
*
* stream.memory.2xlarge
*
*
* -
*
* stream.memory.4xlarge
*
*
* -
*
* stream.memory.8xlarge
*
*
* -
*
* stream.memory.z1d.large
*
*
* -
*
* stream.memory.z1d.xlarge
*
*
* -
*
* stream.memory.z1d.2xlarge
*
*
* -
*
* stream.memory.z1d.3xlarge
*
*
* -
*
* stream.memory.z1d.6xlarge
*
*
* -
*
* stream.memory.z1d.12xlarge
*
*
* -
*
* stream.graphics-design.large
*
*
* -
*
* stream.graphics-design.xlarge
*
*
* -
*
* stream.graphics-design.2xlarge
*
*
* -
*
* stream.graphics-design.4xlarge
*
*
* -
*
* stream.graphics-desktop.2xlarge
*
*
* -
*
* stream.graphics.g4dn.xlarge
*
*
* -
*
* stream.graphics.g4dn.2xlarge
*
*
* -
*
* stream.graphics.g4dn.4xlarge
*
*
* -
*
* stream.graphics.g4dn.8xlarge
*
*
* -
*
* stream.graphics.g4dn.12xlarge
*
*
* -
*
* stream.graphics.g4dn.16xlarge
*
*
* -
*
* stream.graphics-pro.4xlarge
*
*
* -
*
* stream.graphics-pro.8xlarge
*
*
* -
*
* stream.graphics-pro.16xlarge
*
*
*
*
* @return The instance type for the image builder. The following instance types are available:
*
* -
*
* stream.standard.medium
*
*
* -
*
* stream.standard.large
*
*
* -
*
* stream.compute.large
*
*
* -
*
* stream.compute.xlarge
*
*
* -
*
* stream.compute.2xlarge
*
*
* -
*
* stream.compute.4xlarge
*
*
* -
*
* stream.compute.8xlarge
*
*
* -
*
* stream.memory.large
*
*
* -
*
* stream.memory.xlarge
*
*
* -
*
* stream.memory.2xlarge
*
*
* -
*
* stream.memory.4xlarge
*
*
* -
*
* stream.memory.8xlarge
*
*
* -
*
* stream.memory.z1d.large
*
*
* -
*
* stream.memory.z1d.xlarge
*
*
* -
*
* stream.memory.z1d.2xlarge
*
*
* -
*
* stream.memory.z1d.3xlarge
*
*
* -
*
* stream.memory.z1d.6xlarge
*
*
* -
*
* stream.memory.z1d.12xlarge
*
*
* -
*
* stream.graphics-design.large
*
*
* -
*
* stream.graphics-design.xlarge
*
*
* -
*
* stream.graphics-design.2xlarge
*
*
* -
*
* stream.graphics-design.4xlarge
*
*
* -
*
* stream.graphics-desktop.2xlarge
*
*
* -
*
* stream.graphics.g4dn.xlarge
*
*
* -
*
* stream.graphics.g4dn.2xlarge
*
*
* -
*
* stream.graphics.g4dn.4xlarge
*
*
* -
*
* stream.graphics.g4dn.8xlarge
*
*
* -
*
* stream.graphics.g4dn.12xlarge
*
*
* -
*
* stream.graphics.g4dn.16xlarge
*
*
* -
*
* stream.graphics-pro.4xlarge
*
*
* -
*
* stream.graphics-pro.8xlarge
*
*
* -
*
* stream.graphics-pro.16xlarge
*
*
*/
public String getInstanceType() {
return this.instanceType;
}
/**
*
* The instance type for the image builder. The following instance types are available:
*
*
* -
*
* stream.standard.medium
*
*
* -
*
* stream.standard.large
*
*
* -
*
* stream.compute.large
*
*
* -
*
* stream.compute.xlarge
*
*
* -
*
* stream.compute.2xlarge
*
*
* -
*
* stream.compute.4xlarge
*
*
* -
*
* stream.compute.8xlarge
*
*
* -
*
* stream.memory.large
*
*
* -
*
* stream.memory.xlarge
*
*
* -
*
* stream.memory.2xlarge
*
*
* -
*
* stream.memory.4xlarge
*
*
* -
*
* stream.memory.8xlarge
*
*
* -
*
* stream.memory.z1d.large
*
*
* -
*
* stream.memory.z1d.xlarge
*
*
* -
*
* stream.memory.z1d.2xlarge
*
*
* -
*
* stream.memory.z1d.3xlarge
*
*
* -
*
* stream.memory.z1d.6xlarge
*
*
* -
*
* stream.memory.z1d.12xlarge
*
*
* -
*
* stream.graphics-design.large
*
*
* -
*
* stream.graphics-design.xlarge
*
*
* -
*
* stream.graphics-design.2xlarge
*
*
* -
*
* stream.graphics-design.4xlarge
*
*
* -
*
* stream.graphics-desktop.2xlarge
*
*
* -
*
* stream.graphics.g4dn.xlarge
*
*
* -
*
* stream.graphics.g4dn.2xlarge
*
*
* -
*
* stream.graphics.g4dn.4xlarge
*
*
* -
*
* stream.graphics.g4dn.8xlarge
*
*
* -
*
* stream.graphics.g4dn.12xlarge
*
*
* -
*
* stream.graphics.g4dn.16xlarge
*
*
* -
*
* stream.graphics-pro.4xlarge
*
*
* -
*
* stream.graphics-pro.8xlarge
*
*
* -
*
* stream.graphics-pro.16xlarge
*
*
*
*
* @param instanceType
* The instance type for the image builder. The following instance types are available:
*
* -
*
* stream.standard.medium
*
*
* -
*
* stream.standard.large
*
*
* -
*
* stream.compute.large
*
*
* -
*
* stream.compute.xlarge
*
*
* -
*
* stream.compute.2xlarge
*
*
* -
*
* stream.compute.4xlarge
*
*
* -
*
* stream.compute.8xlarge
*
*
* -
*
* stream.memory.large
*
*
* -
*
* stream.memory.xlarge
*
*
* -
*
* stream.memory.2xlarge
*
*
* -
*
* stream.memory.4xlarge
*
*
* -
*
* stream.memory.8xlarge
*
*
* -
*
* stream.memory.z1d.large
*
*
* -
*
* stream.memory.z1d.xlarge
*
*
* -
*
* stream.memory.z1d.2xlarge
*
*
* -
*
* stream.memory.z1d.3xlarge
*
*
* -
*
* stream.memory.z1d.6xlarge
*
*
* -
*
* stream.memory.z1d.12xlarge
*
*
* -
*
* stream.graphics-design.large
*
*
* -
*
* stream.graphics-design.xlarge
*
*
* -
*
* stream.graphics-design.2xlarge
*
*
* -
*
* stream.graphics-design.4xlarge
*
*
* -
*
* stream.graphics-desktop.2xlarge
*
*
* -
*
* stream.graphics.g4dn.xlarge
*
*
* -
*
* stream.graphics.g4dn.2xlarge
*
*
* -
*
* stream.graphics.g4dn.4xlarge
*
*
* -
*
* stream.graphics.g4dn.8xlarge
*
*
* -
*
* stream.graphics.g4dn.12xlarge
*
*
* -
*
* stream.graphics.g4dn.16xlarge
*
*
* -
*
* stream.graphics-pro.4xlarge
*
*
* -
*
* stream.graphics-pro.8xlarge
*
*
* -
*
* stream.graphics-pro.16xlarge
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageBuilder withInstanceType(String instanceType) {
setInstanceType(instanceType);
return this;
}
/**
*
* The operating system platform of the image builder.
*
*
* @param platform
* The operating system platform of the image builder.
* @see PlatformType
*/
public void setPlatform(String platform) {
this.platform = platform;
}
/**
*
* The operating system platform of the image builder.
*
*
* @return The operating system platform of the image builder.
* @see PlatformType
*/
public String getPlatform() {
return this.platform;
}
/**
*
* The operating system platform of the image builder.
*
*
* @param platform
* The operating system platform of the image builder.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PlatformType
*/
public ImageBuilder withPlatform(String platform) {
setPlatform(platform);
return this;
}
/**
*
* The operating system platform of the image builder.
*
*
* @param platform
* The operating system platform of the image builder.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PlatformType
*/
public ImageBuilder withPlatform(PlatformType platform) {
this.platform = platform.toString();
return this;
}
/**
*
* The ARN of the IAM role that is applied to the image builder. To assume a role, the image builder calls the AWS
* Security Token Service (STS) AssumeRole
API operation and passes the ARN of the role to use. The
* operation creates a new session with temporary credentials. AppStream 2.0 retrieves the temporary credentials and
* creates the appstream_machine_role credential profile on the instance.
*
*
* For more information, see Using an IAM Role to Grant Permissions to Applications and Scripts Running on AppStream 2.0 Streaming
* Instances in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param iamRoleArn
* The ARN of the IAM role that is applied to the image builder. To assume a role, the image builder calls
* the AWS Security Token Service (STS) AssumeRole
API operation and passes the ARN of the role
* to use. The operation creates a new session with temporary credentials. AppStream 2.0 retrieves the
* temporary credentials and creates the appstream_machine_role credential profile on the
* instance.
*
* For more information, see Using an IAM Role to Grant Permissions to Applications and Scripts Running on AppStream 2.0 Streaming
* Instances in the Amazon AppStream 2.0 Administration Guide.
*/
public void setIamRoleArn(String iamRoleArn) {
this.iamRoleArn = iamRoleArn;
}
/**
*
* The ARN of the IAM role that is applied to the image builder. To assume a role, the image builder calls the AWS
* Security Token Service (STS) AssumeRole
API operation and passes the ARN of the role to use. The
* operation creates a new session with temporary credentials. AppStream 2.0 retrieves the temporary credentials and
* creates the appstream_machine_role credential profile on the instance.
*
*
* For more information, see Using an IAM Role to Grant Permissions to Applications and Scripts Running on AppStream 2.0 Streaming
* Instances in the Amazon AppStream 2.0 Administration Guide.
*
*
* @return The ARN of the IAM role that is applied to the image builder. To assume a role, the image builder calls
* the AWS Security Token Service (STS) AssumeRole
API operation and passes the ARN of the role
* to use. The operation creates a new session with temporary credentials. AppStream 2.0 retrieves the
* temporary credentials and creates the appstream_machine_role credential profile on the
* instance.
*
* For more information, see Using an IAM Role to Grant Permissions to Applications and Scripts Running on AppStream 2.0 Streaming
* Instances in the Amazon AppStream 2.0 Administration Guide.
*/
public String getIamRoleArn() {
return this.iamRoleArn;
}
/**
*
* The ARN of the IAM role that is applied to the image builder. To assume a role, the image builder calls the AWS
* Security Token Service (STS) AssumeRole
API operation and passes the ARN of the role to use. The
* operation creates a new session with temporary credentials. AppStream 2.0 retrieves the temporary credentials and
* creates the appstream_machine_role credential profile on the instance.
*
*
* For more information, see Using an IAM Role to Grant Permissions to Applications and Scripts Running on AppStream 2.0 Streaming
* Instances in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param iamRoleArn
* The ARN of the IAM role that is applied to the image builder. To assume a role, the image builder calls
* the AWS Security Token Service (STS) AssumeRole
API operation and passes the ARN of the role
* to use. The operation creates a new session with temporary credentials. AppStream 2.0 retrieves the
* temporary credentials and creates the appstream_machine_role credential profile on the
* instance.
*
* For more information, see Using an IAM Role to Grant Permissions to Applications and Scripts Running on AppStream 2.0 Streaming
* Instances in the Amazon AppStream 2.0 Administration Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageBuilder withIamRoleArn(String iamRoleArn) {
setIamRoleArn(iamRoleArn);
return this;
}
/**
*
* The state of the image builder.
*
*
* @param state
* The state of the image builder.
* @see ImageBuilderState
*/
public void setState(String state) {
this.state = state;
}
/**
*
* The state of the image builder.
*
*
* @return The state of the image builder.
* @see ImageBuilderState
*/
public String getState() {
return this.state;
}
/**
*
* The state of the image builder.
*
*
* @param state
* The state of the image builder.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ImageBuilderState
*/
public ImageBuilder withState(String state) {
setState(state);
return this;
}
/**
*
* The state of the image builder.
*
*
* @param state
* The state of the image builder.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ImageBuilderState
*/
public ImageBuilder withState(ImageBuilderState state) {
this.state = state.toString();
return this;
}
/**
*
* The reason why the last state change occurred.
*
*
* @param stateChangeReason
* The reason why the last state change occurred.
*/
public void setStateChangeReason(ImageBuilderStateChangeReason stateChangeReason) {
this.stateChangeReason = stateChangeReason;
}
/**
*
* The reason why the last state change occurred.
*
*
* @return The reason why the last state change occurred.
*/
public ImageBuilderStateChangeReason getStateChangeReason() {
return this.stateChangeReason;
}
/**
*
* The reason why the last state change occurred.
*
*
* @param stateChangeReason
* The reason why the last state change occurred.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageBuilder withStateChangeReason(ImageBuilderStateChangeReason stateChangeReason) {
setStateChangeReason(stateChangeReason);
return this;
}
/**
*
* The time stamp when the image builder was created.
*
*
* @param createdTime
* The time stamp when the image builder was created.
*/
public void setCreatedTime(java.util.Date createdTime) {
this.createdTime = createdTime;
}
/**
*
* The time stamp when the image builder was created.
*
*
* @return The time stamp when the image builder was created.
*/
public java.util.Date getCreatedTime() {
return this.createdTime;
}
/**
*
* The time stamp when the image builder was created.
*
*
* @param createdTime
* The time stamp when the image builder was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageBuilder withCreatedTime(java.util.Date createdTime) {
setCreatedTime(createdTime);
return this;
}
/**
*
* Enables or disables default internet access for the image builder.
*
*
* @param enableDefaultInternetAccess
* Enables or disables default internet access for the image builder.
*/
public void setEnableDefaultInternetAccess(Boolean enableDefaultInternetAccess) {
this.enableDefaultInternetAccess = enableDefaultInternetAccess;
}
/**
*
* Enables or disables default internet access for the image builder.
*
*
* @return Enables or disables default internet access for the image builder.
*/
public Boolean getEnableDefaultInternetAccess() {
return this.enableDefaultInternetAccess;
}
/**
*
* Enables or disables default internet access for the image builder.
*
*
* @param enableDefaultInternetAccess
* Enables or disables default internet access for the image builder.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageBuilder withEnableDefaultInternetAccess(Boolean enableDefaultInternetAccess) {
setEnableDefaultInternetAccess(enableDefaultInternetAccess);
return this;
}
/**
*
* Enables or disables default internet access for the image builder.
*
*
* @return Enables or disables default internet access for the image builder.
*/
public Boolean isEnableDefaultInternetAccess() {
return this.enableDefaultInternetAccess;
}
/**
*
* The name of the directory and organizational unit (OU) to use to join the image builder to a Microsoft Active
* Directory domain.
*
*
* @param domainJoinInfo
* The name of the directory and organizational unit (OU) to use to join the image builder to a Microsoft
* Active Directory domain.
*/
public void setDomainJoinInfo(DomainJoinInfo domainJoinInfo) {
this.domainJoinInfo = domainJoinInfo;
}
/**
*
* The name of the directory and organizational unit (OU) to use to join the image builder to a Microsoft Active
* Directory domain.
*
*
* @return The name of the directory and organizational unit (OU) to use to join the image builder to a Microsoft
* Active Directory domain.
*/
public DomainJoinInfo getDomainJoinInfo() {
return this.domainJoinInfo;
}
/**
*
* The name of the directory and organizational unit (OU) to use to join the image builder to a Microsoft Active
* Directory domain.
*
*
* @param domainJoinInfo
* The name of the directory and organizational unit (OU) to use to join the image builder to a Microsoft
* Active Directory domain.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageBuilder withDomainJoinInfo(DomainJoinInfo domainJoinInfo) {
setDomainJoinInfo(domainJoinInfo);
return this;
}
/**
* @param networkAccessConfiguration
*/
public void setNetworkAccessConfiguration(NetworkAccessConfiguration networkAccessConfiguration) {
this.networkAccessConfiguration = networkAccessConfiguration;
}
/**
* @return
*/
public NetworkAccessConfiguration getNetworkAccessConfiguration() {
return this.networkAccessConfiguration;
}
/**
* @param networkAccessConfiguration
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageBuilder withNetworkAccessConfiguration(NetworkAccessConfiguration networkAccessConfiguration) {
setNetworkAccessConfiguration(networkAccessConfiguration);
return this;
}
/**
*
* The image builder errors.
*
*
* @return The image builder errors.
*/
public java.util.List getImageBuilderErrors() {
return imageBuilderErrors;
}
/**
*
* The image builder errors.
*
*
* @param imageBuilderErrors
* The image builder errors.
*/
public void setImageBuilderErrors(java.util.Collection imageBuilderErrors) {
if (imageBuilderErrors == null) {
this.imageBuilderErrors = null;
return;
}
this.imageBuilderErrors = new java.util.ArrayList(imageBuilderErrors);
}
/**
*
* The image builder errors.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setImageBuilderErrors(java.util.Collection)} or {@link #withImageBuilderErrors(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param imageBuilderErrors
* The image builder errors.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageBuilder withImageBuilderErrors(ResourceError... imageBuilderErrors) {
if (this.imageBuilderErrors == null) {
setImageBuilderErrors(new java.util.ArrayList(imageBuilderErrors.length));
}
for (ResourceError ele : imageBuilderErrors) {
this.imageBuilderErrors.add(ele);
}
return this;
}
/**
*
* The image builder errors.
*
*
* @param imageBuilderErrors
* The image builder errors.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageBuilder withImageBuilderErrors(java.util.Collection imageBuilderErrors) {
setImageBuilderErrors(imageBuilderErrors);
return this;
}
/**
*
* The version of the AppStream 2.0 agent that is currently being used by the image builder.
*
*
* @param appstreamAgentVersion
* The version of the AppStream 2.0 agent that is currently being used by the image builder.
*/
public void setAppstreamAgentVersion(String appstreamAgentVersion) {
this.appstreamAgentVersion = appstreamAgentVersion;
}
/**
*
* The version of the AppStream 2.0 agent that is currently being used by the image builder.
*
*
* @return The version of the AppStream 2.0 agent that is currently being used by the image builder.
*/
public String getAppstreamAgentVersion() {
return this.appstreamAgentVersion;
}
/**
*
* The version of the AppStream 2.0 agent that is currently being used by the image builder.
*
*
* @param appstreamAgentVersion
* The version of the AppStream 2.0 agent that is currently being used by the image builder.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageBuilder withAppstreamAgentVersion(String appstreamAgentVersion) {
setAppstreamAgentVersion(appstreamAgentVersion);
return this;
}
/**
*
* The list of virtual private cloud (VPC) interface endpoint objects. Administrators can connect to the image
* builder only through the specified endpoints.
*
*
* @return The list of virtual private cloud (VPC) interface endpoint objects. Administrators can connect to the
* image builder only through the specified endpoints.
*/
public java.util.List getAccessEndpoints() {
return accessEndpoints;
}
/**
*
* The list of virtual private cloud (VPC) interface endpoint objects. Administrators can connect to the image
* builder only through the specified endpoints.
*
*
* @param accessEndpoints
* The list of virtual private cloud (VPC) interface endpoint objects. Administrators can connect to the
* image builder only through the specified endpoints.
*/
public void setAccessEndpoints(java.util.Collection accessEndpoints) {
if (accessEndpoints == null) {
this.accessEndpoints = null;
return;
}
this.accessEndpoints = new java.util.ArrayList(accessEndpoints);
}
/**
*
* The list of virtual private cloud (VPC) interface endpoint objects. Administrators can connect to the image
* builder only through the specified endpoints.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAccessEndpoints(java.util.Collection)} or {@link #withAccessEndpoints(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param accessEndpoints
* The list of virtual private cloud (VPC) interface endpoint objects. Administrators can connect to the
* image builder only through the specified endpoints.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageBuilder withAccessEndpoints(AccessEndpoint... accessEndpoints) {
if (this.accessEndpoints == null) {
setAccessEndpoints(new java.util.ArrayList(accessEndpoints.length));
}
for (AccessEndpoint ele : accessEndpoints) {
this.accessEndpoints.add(ele);
}
return this;
}
/**
*
* The list of virtual private cloud (VPC) interface endpoint objects. Administrators can connect to the image
* builder only through the specified endpoints.
*
*
* @param accessEndpoints
* The list of virtual private cloud (VPC) interface endpoint objects. Administrators can connect to the
* image builder only through the specified endpoints.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ImageBuilder withAccessEndpoints(java.util.Collection accessEndpoints) {
setAccessEndpoints(accessEndpoints);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getArn() != null)
sb.append("Arn: ").append(getArn()).append(",");
if (getImageArn() != null)
sb.append("ImageArn: ").append(getImageArn()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getDisplayName() != null)
sb.append("DisplayName: ").append(getDisplayName()).append(",");
if (getVpcConfig() != null)
sb.append("VpcConfig: ").append(getVpcConfig()).append(",");
if (getInstanceType() != null)
sb.append("InstanceType: ").append(getInstanceType()).append(",");
if (getPlatform() != null)
sb.append("Platform: ").append(getPlatform()).append(",");
if (getIamRoleArn() != null)
sb.append("IamRoleArn: ").append(getIamRoleArn()).append(",");
if (getState() != null)
sb.append("State: ").append(getState()).append(",");
if (getStateChangeReason() != null)
sb.append("StateChangeReason: ").append(getStateChangeReason()).append(",");
if (getCreatedTime() != null)
sb.append("CreatedTime: ").append(getCreatedTime()).append(",");
if (getEnableDefaultInternetAccess() != null)
sb.append("EnableDefaultInternetAccess: ").append(getEnableDefaultInternetAccess()).append(",");
if (getDomainJoinInfo() != null)
sb.append("DomainJoinInfo: ").append(getDomainJoinInfo()).append(",");
if (getNetworkAccessConfiguration() != null)
sb.append("NetworkAccessConfiguration: ").append(getNetworkAccessConfiguration()).append(",");
if (getImageBuilderErrors() != null)
sb.append("ImageBuilderErrors: ").append(getImageBuilderErrors()).append(",");
if (getAppstreamAgentVersion() != null)
sb.append("AppstreamAgentVersion: ").append(getAppstreamAgentVersion()).append(",");
if (getAccessEndpoints() != null)
sb.append("AccessEndpoints: ").append(getAccessEndpoints());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ImageBuilder == false)
return false;
ImageBuilder other = (ImageBuilder) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getArn() == null ^ this.getArn() == null)
return false;
if (other.getArn() != null && other.getArn().equals(this.getArn()) == false)
return false;
if (other.getImageArn() == null ^ this.getImageArn() == null)
return false;
if (other.getImageArn() != null && other.getImageArn().equals(this.getImageArn()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getDisplayName() == null ^ this.getDisplayName() == null)
return false;
if (other.getDisplayName() != null && other.getDisplayName().equals(this.getDisplayName()) == false)
return false;
if (other.getVpcConfig() == null ^ this.getVpcConfig() == null)
return false;
if (other.getVpcConfig() != null && other.getVpcConfig().equals(this.getVpcConfig()) == false)
return false;
if (other.getInstanceType() == null ^ this.getInstanceType() == null)
return false;
if (other.getInstanceType() != null && other.getInstanceType().equals(this.getInstanceType()) == false)
return false;
if (other.getPlatform() == null ^ this.getPlatform() == null)
return false;
if (other.getPlatform() != null && other.getPlatform().equals(this.getPlatform()) == false)
return false;
if (other.getIamRoleArn() == null ^ this.getIamRoleArn() == null)
return false;
if (other.getIamRoleArn() != null && other.getIamRoleArn().equals(this.getIamRoleArn()) == false)
return false;
if (other.getState() == null ^ this.getState() == null)
return false;
if (other.getState() != null && other.getState().equals(this.getState()) == false)
return false;
if (other.getStateChangeReason() == null ^ this.getStateChangeReason() == null)
return false;
if (other.getStateChangeReason() != null && other.getStateChangeReason().equals(this.getStateChangeReason()) == false)
return false;
if (other.getCreatedTime() == null ^ this.getCreatedTime() == null)
return false;
if (other.getCreatedTime() != null && other.getCreatedTime().equals(this.getCreatedTime()) == false)
return false;
if (other.getEnableDefaultInternetAccess() == null ^ this.getEnableDefaultInternetAccess() == null)
return false;
if (other.getEnableDefaultInternetAccess() != null && other.getEnableDefaultInternetAccess().equals(this.getEnableDefaultInternetAccess()) == false)
return false;
if (other.getDomainJoinInfo() == null ^ this.getDomainJoinInfo() == null)
return false;
if (other.getDomainJoinInfo() != null && other.getDomainJoinInfo().equals(this.getDomainJoinInfo()) == false)
return false;
if (other.getNetworkAccessConfiguration() == null ^ this.getNetworkAccessConfiguration() == null)
return false;
if (other.getNetworkAccessConfiguration() != null && other.getNetworkAccessConfiguration().equals(this.getNetworkAccessConfiguration()) == false)
return false;
if (other.getImageBuilderErrors() == null ^ this.getImageBuilderErrors() == null)
return false;
if (other.getImageBuilderErrors() != null && other.getImageBuilderErrors().equals(this.getImageBuilderErrors()) == false)
return false;
if (other.getAppstreamAgentVersion() == null ^ this.getAppstreamAgentVersion() == null)
return false;
if (other.getAppstreamAgentVersion() != null && other.getAppstreamAgentVersion().equals(this.getAppstreamAgentVersion()) == false)
return false;
if (other.getAccessEndpoints() == null ^ this.getAccessEndpoints() == null)
return false;
if (other.getAccessEndpoints() != null && other.getAccessEndpoints().equals(this.getAccessEndpoints()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getArn() == null) ? 0 : getArn().hashCode());
hashCode = prime * hashCode + ((getImageArn() == null) ? 0 : getImageArn().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getDisplayName() == null) ? 0 : getDisplayName().hashCode());
hashCode = prime * hashCode + ((getVpcConfig() == null) ? 0 : getVpcConfig().hashCode());
hashCode = prime * hashCode + ((getInstanceType() == null) ? 0 : getInstanceType().hashCode());
hashCode = prime * hashCode + ((getPlatform() == null) ? 0 : getPlatform().hashCode());
hashCode = prime * hashCode + ((getIamRoleArn() == null) ? 0 : getIamRoleArn().hashCode());
hashCode = prime * hashCode + ((getState() == null) ? 0 : getState().hashCode());
hashCode = prime * hashCode + ((getStateChangeReason() == null) ? 0 : getStateChangeReason().hashCode());
hashCode = prime * hashCode + ((getCreatedTime() == null) ? 0 : getCreatedTime().hashCode());
hashCode = prime * hashCode + ((getEnableDefaultInternetAccess() == null) ? 0 : getEnableDefaultInternetAccess().hashCode());
hashCode = prime * hashCode + ((getDomainJoinInfo() == null) ? 0 : getDomainJoinInfo().hashCode());
hashCode = prime * hashCode + ((getNetworkAccessConfiguration() == null) ? 0 : getNetworkAccessConfiguration().hashCode());
hashCode = prime * hashCode + ((getImageBuilderErrors() == null) ? 0 : getImageBuilderErrors().hashCode());
hashCode = prime * hashCode + ((getAppstreamAgentVersion() == null) ? 0 : getAppstreamAgentVersion().hashCode());
hashCode = prime * hashCode + ((getAccessEndpoints() == null) ? 0 : getAccessEndpoints().hashCode());
return hashCode;
}
@Override
public ImageBuilder clone() {
try {
return (ImageBuilder) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.appstream.model.transform.ImageBuilderMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}