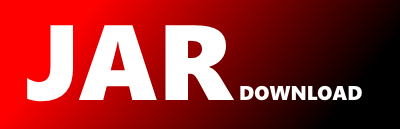
com.amazonaws.services.appstream.AmazonAppStream Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appstream Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appstream;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.appstream.model.*;
import com.amazonaws.services.appstream.waiters.AmazonAppStreamWaiters;
/**
* Interface for accessing Amazon AppStream.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.appstream.AbstractAmazonAppStream} instead.
*
*
* Amazon AppStream 2.0
*
* This is the Amazon AppStream 2.0 API Reference. This documentation provides descriptions and syntax for each
* of the actions and data types in AppStream 2.0. AppStream 2.0 is a fully managed, secure application streaming
* service that lets you stream desktop applications to users without rewriting applications. AppStream 2.0 manages the
* AWS resources that are required to host and run your applications, scales automatically, and provides access to your
* users on demand.
*
*
*
* You can call the AppStream 2.0 API operations by using an interface VPC endpoint (interface endpoint). For more
* information, see Access AppStream 2.0 API Operations and CLI Commands Through an Interface VPC Endpoint in the Amazon
* AppStream 2.0 Administration Guide.
*
*
*
* To learn more about AppStream 2.0, see the following resources:
*
*
* -
*
*
* -
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonAppStream {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "appstream2";
/**
* Overrides the default endpoint for this client ("appstream2.us-east-1.amazonaws.com"). Callers can use this
* method to control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "appstream2.us-east-1.amazonaws.com") or a full URL, including the
* protocol (ex: "appstream2.us-east-1.amazonaws.com"). If the protocol is not specified here, the default protocol
* from this client's {@link ClientConfiguration} will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and a complete list of all available
* endpoints for all AWS services, see: https://docs.aws.amazon.com/sdk-for-java/v1/developer-guide/java-dg-region-selection.html#region-selection-
* choose-endpoint
*
* This method is not threadsafe. An endpoint should be configured when the client is created and before any
* service requests are made. Changing it afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param endpoint
* The endpoint (ex: "appstream2.us-east-1.amazonaws.com") or a full URL, including the protocol (ex:
* "appstream2.us-east-1.amazonaws.com") of the region specific AWS endpoint this client will communicate
* with.
* @deprecated use {@link AwsClientBuilder#setEndpointConfiguration(AwsClientBuilder.EndpointConfiguration)} for
* example:
* {@code builder.setEndpointConfiguration(new EndpointConfiguration(endpoint, signingRegion));}
*/
@Deprecated
void setEndpoint(String endpoint);
/**
* An alternative to {@link AmazonAppStream#setEndpoint(String)}, sets the regional endpoint for this client's
* service calls. Callers can use this method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol. To use http instead, specify it in the
* {@link ClientConfiguration} supplied at construction.
*
* This method is not threadsafe. A region should be configured when the client is created and before any service
* requests are made. Changing it afterwards creates inevitable race conditions for any service requests in transit
* or retrying.
*
* @param region
* The region this client will communicate with. See {@link Region#getRegion(com.amazonaws.regions.Regions)}
* for accessing a given region. Must not be null and must be a region where the service is available.
*
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class, com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
* @see Region#isServiceSupported(String)
* @deprecated use {@link AwsClientBuilder#setRegion(String)}
*/
@Deprecated
void setRegion(Region region);
/**
*
* Associates the specified application with the specified fleet. This is only supported for Elastic fleets.
*
*
* @param associateApplicationFleetRequest
* @return Result of the AssociateApplicationFleet operation returned by the service.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @sample AmazonAppStream.AssociateApplicationFleet
* @see AWS API Documentation
*/
AssociateApplicationFleetResult associateApplicationFleet(AssociateApplicationFleetRequest associateApplicationFleetRequest);
/**
*
* Associates an application to entitle.
*
*
* @param associateApplicationToEntitlementRequest
* @return Result of the AssociateApplicationToEntitlement operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws EntitlementNotFoundException
* The entitlement can't be found.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @sample AmazonAppStream.AssociateApplicationToEntitlement
* @see AWS API Documentation
*/
AssociateApplicationToEntitlementResult associateApplicationToEntitlement(AssociateApplicationToEntitlementRequest associateApplicationToEntitlementRequest);
/**
*
* Associates the specified fleet with the specified stack.
*
*
* @param associateFleetRequest
* @return Result of the AssociateFleet operation returned by the service.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws IncompatibleImageException
* The image can't be updated because it's not compatible for updates.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @sample AmazonAppStream.AssociateFleet
* @see AWS API
* Documentation
*/
AssociateFleetResult associateFleet(AssociateFleetRequest associateFleetRequest);
/**
*
* Associates the specified users with the specified stacks. Users in a user pool cannot be assigned to stacks with
* fleets that are joined to an Active Directory domain.
*
*
* @param batchAssociateUserStackRequest
* @return Result of the BatchAssociateUserStack operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @sample AmazonAppStream.BatchAssociateUserStack
* @see AWS API Documentation
*/
BatchAssociateUserStackResult batchAssociateUserStack(BatchAssociateUserStackRequest batchAssociateUserStackRequest);
/**
*
* Disassociates the specified users from the specified stacks.
*
*
* @param batchDisassociateUserStackRequest
* @return Result of the BatchDisassociateUserStack operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @sample AmazonAppStream.BatchDisassociateUserStack
* @see AWS API Documentation
*/
BatchDisassociateUserStackResult batchDisassociateUserStack(BatchDisassociateUserStackRequest batchDisassociateUserStackRequest);
/**
*
* Copies the image within the same region or to a new region within the same AWS account. Note that any tags you
* added to the image will not be copied.
*
*
* @param copyImageRequest
* @return Result of the CopyImage operation returned by the service.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws IncompatibleImageException
* The image can't be updated because it's not compatible for updates.
* @sample AmazonAppStream.CopyImage
* @see AWS API
* Documentation
*/
CopyImageResult copyImage(CopyImageRequest copyImageRequest);
/**
*
* Creates an app block.
*
*
* App blocks are an Amazon AppStream 2.0 resource that stores the details about the virtual hard disk in an S3
* bucket. It also stores the setup script with details about how to mount the virtual hard disk. The virtual hard
* disk includes the application binaries and other files necessary to launch your applications. Multiple
* applications can be assigned to a single app block.
*
*
* This is only supported for Elastic fleets.
*
*
* @param createAppBlockRequest
* @return Result of the CreateAppBlock operation returned by the service.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @sample AmazonAppStream.CreateAppBlock
* @see AWS API
* Documentation
*/
CreateAppBlockResult createAppBlock(CreateAppBlockRequest createAppBlockRequest);
/**
*
* Creates an application.
*
*
* Applications are an Amazon AppStream 2.0 resource that stores the details about how to launch applications on
* Elastic fleet streaming instances. An application consists of the launch details, icon, and display name.
* Applications are associated with an app block that contains the application binaries and other files. The
* applications assigned to an Elastic fleet are the applications users can launch.
*
*
* This is only supported for Elastic fleets.
*
*
* @param createApplicationRequest
* @return Result of the CreateApplication operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.CreateApplication
* @see AWS
* API Documentation
*/
CreateApplicationResult createApplication(CreateApplicationRequest createApplicationRequest);
/**
*
* Creates a Directory Config object in AppStream 2.0. This object includes the configuration information required
* to join fleets and image builders to Microsoft Active Directory domains.
*
*
* @param createDirectoryConfigRequest
* @return Result of the CreateDirectoryConfig operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws InvalidRoleException
* The specified role is invalid.
* @sample AmazonAppStream.CreateDirectoryConfig
* @see AWS API Documentation
*/
CreateDirectoryConfigResult createDirectoryConfig(CreateDirectoryConfigRequest createDirectoryConfigRequest);
/**
*
* Creates a new entitlement. Entitlements control access to specific applications within a stack, based on user
* attributes. Entitlements apply to SAML 2.0 federated user identities. Amazon AppStream 2.0 user pool and
* streaming URL users are entitled to all applications in a stack. Entitlements don't apply to the desktop stream
* view application, or to applications managed by a dynamic app provider using the Dynamic Application Framework.
*
*
* @param createEntitlementRequest
* @return Result of the CreateEntitlement operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws EntitlementAlreadyExistsException
* The entitlement already exists.
* @sample AmazonAppStream.CreateEntitlement
* @see AWS
* API Documentation
*/
CreateEntitlementResult createEntitlement(CreateEntitlementRequest createEntitlementRequest);
/**
*
* Creates a fleet. A fleet consists of streaming instances that your users access for their applications and
* desktops.
*
*
* @param createFleetRequest
* @return Result of the CreateFleet operation returned by the service.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws RequestLimitExceededException
* AppStream 2.0 can’t process the request right now because the Describe calls from your AWS account are
* being throttled by Amazon EC2. Try again later.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws InvalidRoleException
* The specified role is invalid.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws IncompatibleImageException
* The image can't be updated because it's not compatible for updates.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @sample AmazonAppStream.CreateFleet
* @see AWS API
* Documentation
*/
CreateFleetResult createFleet(CreateFleetRequest createFleetRequest);
/**
*
* Creates an image builder. An image builder is a virtual machine that is used to create an image.
*
*
* The initial state of the builder is PENDING
. When it is ready, the state is RUNNING
.
*
*
* @param createImageBuilderRequest
* @return Result of the CreateImageBuilder operation returned by the service.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws RequestLimitExceededException
* AppStream 2.0 can’t process the request right now because the Describe calls from your AWS account are
* being throttled by Amazon EC2. Try again later.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidRoleException
* The specified role is invalid.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws IncompatibleImageException
* The image can't be updated because it's not compatible for updates.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @sample AmazonAppStream.CreateImageBuilder
* @see AWS
* API Documentation
*/
CreateImageBuilderResult createImageBuilder(CreateImageBuilderRequest createImageBuilderRequest);
/**
*
* Creates a URL to start an image builder streaming session.
*
*
* @param createImageBuilderStreamingURLRequest
* @return Result of the CreateImageBuilderStreamingURL operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.CreateImageBuilderStreamingURL
* @see AWS API Documentation
*/
CreateImageBuilderStreamingURLResult createImageBuilderStreamingURL(CreateImageBuilderStreamingURLRequest createImageBuilderStreamingURLRequest);
/**
*
* Creates a stack to start streaming applications to users. A stack consists of an associated fleet, user access
* policies, and storage configurations.
*
*
* @param createStackRequest
* @return Result of the CreateStack operation returned by the service.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws InvalidRoleException
* The specified role is invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @sample AmazonAppStream.CreateStack
* @see AWS API
* Documentation
*/
CreateStackResult createStack(CreateStackRequest createStackRequest);
/**
*
* Creates a temporary URL to start an AppStream 2.0 streaming session for the specified user. A streaming URL
* enables application streaming to be tested without user setup.
*
*
* @param createStreamingURLRequest
* @return Result of the CreateStreamingURL operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @sample AmazonAppStream.CreateStreamingURL
* @see AWS
* API Documentation
*/
CreateStreamingURLResult createStreamingURL(CreateStreamingURLRequest createStreamingURLRequest);
/**
*
* Creates a new image with the latest Windows operating system updates, driver updates, and AppStream 2.0 agent
* software.
*
*
* For more information, see the "Update an Image by Using Managed AppStream 2.0 Image Updates" section in Administer Your
* AppStream 2.0 Images, in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param createUpdatedImageRequest
* @return Result of the CreateUpdatedImage operation returned by the service.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws IncompatibleImageException
* The image can't be updated because it's not compatible for updates.
* @sample AmazonAppStream.CreateUpdatedImage
* @see AWS
* API Documentation
*/
CreateUpdatedImageResult createUpdatedImage(CreateUpdatedImageRequest createUpdatedImageRequest);
/**
*
* Creates a usage report subscription. Usage reports are generated daily.
*
*
* @param createUsageReportSubscriptionRequest
* @return Result of the CreateUsageReportSubscription operation returned by the service.
* @throws InvalidRoleException
* The specified role is invalid.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @sample AmazonAppStream.CreateUsageReportSubscription
* @see AWS API Documentation
*/
CreateUsageReportSubscriptionResult createUsageReportSubscription(CreateUsageReportSubscriptionRequest createUsageReportSubscriptionRequest);
/**
*
* Creates a new user in the user pool.
*
*
* @param createUserRequest
* @return Result of the CreateUser operation returned by the service.
* @throws ResourceAlreadyExistsException
* The specified resource already exists.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @sample AmazonAppStream.CreateUser
* @see AWS API
* Documentation
*/
CreateUserResult createUser(CreateUserRequest createUserRequest);
/**
*
* Deletes an app block.
*
*
* @param deleteAppBlockRequest
* @return Result of the DeleteAppBlock operation returned by the service.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DeleteAppBlock
* @see AWS API
* Documentation
*/
DeleteAppBlockResult deleteAppBlock(DeleteAppBlockRequest deleteAppBlockRequest);
/**
*
* Deletes an application.
*
*
* @param deleteApplicationRequest
* @return Result of the DeleteApplication operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @sample AmazonAppStream.DeleteApplication
* @see AWS
* API Documentation
*/
DeleteApplicationResult deleteApplication(DeleteApplicationRequest deleteApplicationRequest);
/**
*
* Deletes the specified Directory Config object from AppStream 2.0. This object includes the information required
* to join streaming instances to an Active Directory domain.
*
*
* @param deleteDirectoryConfigRequest
* @return Result of the DeleteDirectoryConfig operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DeleteDirectoryConfig
* @see AWS API Documentation
*/
DeleteDirectoryConfigResult deleteDirectoryConfig(DeleteDirectoryConfigRequest deleteDirectoryConfigRequest);
/**
*
* Deletes the specified entitlement.
*
*
* @param deleteEntitlementRequest
* @return Result of the DeleteEntitlement operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws EntitlementNotFoundException
* The entitlement can't be found.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @sample AmazonAppStream.DeleteEntitlement
* @see AWS
* API Documentation
*/
DeleteEntitlementResult deleteEntitlement(DeleteEntitlementRequest deleteEntitlementRequest);
/**
*
* Deletes the specified fleet.
*
*
* @param deleteFleetRequest
* @return Result of the DeleteFleet operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @sample AmazonAppStream.DeleteFleet
* @see AWS API
* Documentation
*/
DeleteFleetResult deleteFleet(DeleteFleetRequest deleteFleetRequest);
/**
*
* Deletes the specified image. You cannot delete an image when it is in use. After you delete an image, you cannot
* provision new capacity using the image.
*
*
* @param deleteImageRequest
* @return Result of the DeleteImage operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @sample AmazonAppStream.DeleteImage
* @see AWS API
* Documentation
*/
DeleteImageResult deleteImage(DeleteImageRequest deleteImageRequest);
/**
*
* Deletes the specified image builder and releases the capacity.
*
*
* @param deleteImageBuilderRequest
* @return Result of the DeleteImageBuilder operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @sample AmazonAppStream.DeleteImageBuilder
* @see AWS
* API Documentation
*/
DeleteImageBuilderResult deleteImageBuilder(DeleteImageBuilderRequest deleteImageBuilderRequest);
/**
*
* Deletes permissions for the specified private image. After you delete permissions for an image, AWS accounts to
* which you previously granted these permissions can no longer use the image.
*
*
* @param deleteImagePermissionsRequest
* @return Result of the DeleteImagePermissions operation returned by the service.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DeleteImagePermissions
* @see AWS API Documentation
*/
DeleteImagePermissionsResult deleteImagePermissions(DeleteImagePermissionsRequest deleteImagePermissionsRequest);
/**
*
* Deletes the specified stack. After the stack is deleted, the application streaming environment provided by the
* stack is no longer available to users. Also, any reservations made for application streaming sessions for the
* stack are released.
*
*
* @param deleteStackRequest
* @return Result of the DeleteStack operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @sample AmazonAppStream.DeleteStack
* @see AWS API
* Documentation
*/
DeleteStackResult deleteStack(DeleteStackRequest deleteStackRequest);
/**
*
* Disables usage report generation.
*
*
* @param deleteUsageReportSubscriptionRequest
* @return Result of the DeleteUsageReportSubscription operation returned by the service.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DeleteUsageReportSubscription
* @see AWS API Documentation
*/
DeleteUsageReportSubscriptionResult deleteUsageReportSubscription(DeleteUsageReportSubscriptionRequest deleteUsageReportSubscriptionRequest);
/**
*
* Deletes a user from the user pool.
*
*
* @param deleteUserRequest
* @return Result of the DeleteUser operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DeleteUser
* @see AWS API
* Documentation
*/
DeleteUserResult deleteUser(DeleteUserRequest deleteUserRequest);
/**
*
* Retrieves a list that describes one or more app blocks.
*
*
* @param describeAppBlocksRequest
* @return Result of the DescribeAppBlocks operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DescribeAppBlocks
* @see AWS
* API Documentation
*/
DescribeAppBlocksResult describeAppBlocks(DescribeAppBlocksRequest describeAppBlocksRequest);
/**
*
* Retrieves a list that describes one or more application fleet associations. Either ApplicationArn or FleetName
* must be specified.
*
*
* @param describeApplicationFleetAssociationsRequest
* @return Result of the DescribeApplicationFleetAssociations operation returned by the service.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @sample AmazonAppStream.DescribeApplicationFleetAssociations
* @see AWS API Documentation
*/
DescribeApplicationFleetAssociationsResult describeApplicationFleetAssociations(
DescribeApplicationFleetAssociationsRequest describeApplicationFleetAssociationsRequest);
/**
*
* Retrieves a list that describes one or more applications.
*
*
* @param describeApplicationsRequest
* @return Result of the DescribeApplications operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DescribeApplications
* @see AWS
* API Documentation
*/
DescribeApplicationsResult describeApplications(DescribeApplicationsRequest describeApplicationsRequest);
/**
*
* Retrieves a list that describes one or more specified Directory Config objects for AppStream 2.0, if the names
* for these objects are provided. Otherwise, all Directory Config objects in the account are described. These
* objects include the configuration information required to join fleets and image builders to Microsoft Active
* Directory domains.
*
*
* Although the response syntax in this topic includes the account password, this password is not returned in the
* actual response.
*
*
* @param describeDirectoryConfigsRequest
* @return Result of the DescribeDirectoryConfigs operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DescribeDirectoryConfigs
* @see AWS API Documentation
*/
DescribeDirectoryConfigsResult describeDirectoryConfigs(DescribeDirectoryConfigsRequest describeDirectoryConfigsRequest);
/**
*
* Retrieves a list that describes one of more entitlements.
*
*
* @param describeEntitlementsRequest
* @return Result of the DescribeEntitlements operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws EntitlementNotFoundException
* The entitlement can't be found.
* @sample AmazonAppStream.DescribeEntitlements
* @see AWS
* API Documentation
*/
DescribeEntitlementsResult describeEntitlements(DescribeEntitlementsRequest describeEntitlementsRequest);
/**
*
* Retrieves a list that describes one or more specified fleets, if the fleet names are provided. Otherwise, all
* fleets in the account are described.
*
*
* @param describeFleetsRequest
* @return Result of the DescribeFleets operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DescribeFleets
* @see AWS API
* Documentation
*/
DescribeFleetsResult describeFleets(DescribeFleetsRequest describeFleetsRequest);
/**
*
* Retrieves a list that describes one or more specified image builders, if the image builder names are provided.
* Otherwise, all image builders in the account are described.
*
*
* @param describeImageBuildersRequest
* @return Result of the DescribeImageBuilders operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DescribeImageBuilders
* @see AWS API Documentation
*/
DescribeImageBuildersResult describeImageBuilders(DescribeImageBuildersRequest describeImageBuildersRequest);
/**
*
* Retrieves a list that describes the permissions for shared AWS account IDs on a private image that you own.
*
*
* @param describeImagePermissionsRequest
* @return Result of the DescribeImagePermissions operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DescribeImagePermissions
* @see AWS API Documentation
*/
DescribeImagePermissionsResult describeImagePermissions(DescribeImagePermissionsRequest describeImagePermissionsRequest);
/**
*
* Retrieves a list that describes one or more specified images, if the image names or image ARNs are provided.
* Otherwise, all images in the account are described.
*
*
* @param describeImagesRequest
* @return Result of the DescribeImages operation returned by the service.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DescribeImages
* @see AWS API
* Documentation
*/
DescribeImagesResult describeImages(DescribeImagesRequest describeImagesRequest);
/**
*
* Retrieves a list that describes the streaming sessions for a specified stack and fleet. If a UserId is provided
* for the stack and fleet, only streaming sessions for that user are described. If an authentication type is not
* provided, the default is to authenticate users using a streaming URL.
*
*
* @param describeSessionsRequest
* @return Result of the DescribeSessions operation returned by the service.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @sample AmazonAppStream.DescribeSessions
* @see AWS API
* Documentation
*/
DescribeSessionsResult describeSessions(DescribeSessionsRequest describeSessionsRequest);
/**
*
* Retrieves a list that describes one or more specified stacks, if the stack names are provided. Otherwise, all
* stacks in the account are described.
*
*
* @param describeStacksRequest
* @return Result of the DescribeStacks operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DescribeStacks
* @see AWS API
* Documentation
*/
DescribeStacksResult describeStacks(DescribeStacksRequest describeStacksRequest);
/**
*
* Retrieves a list that describes one or more usage report subscriptions.
*
*
* @param describeUsageReportSubscriptionsRequest
* @return Result of the DescribeUsageReportSubscriptions operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @sample AmazonAppStream.DescribeUsageReportSubscriptions
* @see AWS API Documentation
*/
DescribeUsageReportSubscriptionsResult describeUsageReportSubscriptions(DescribeUsageReportSubscriptionsRequest describeUsageReportSubscriptionsRequest);
/**
*
* Retrieves a list that describes the UserStackAssociation objects. You must specify either or both of the
* following:
*
*
* -
*
* The stack name
*
*
* -
*
* The user name (email address of the user associated with the stack) and the authentication type for the user
*
*
*
*
* @param describeUserStackAssociationsRequest
* @return Result of the DescribeUserStackAssociations operation returned by the service.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @sample AmazonAppStream.DescribeUserStackAssociations
* @see AWS API Documentation
*/
DescribeUserStackAssociationsResult describeUserStackAssociations(DescribeUserStackAssociationsRequest describeUserStackAssociationsRequest);
/**
*
* Retrieves a list that describes one or more specified users in the user pool.
*
*
* @param describeUsersRequest
* @return Result of the DescribeUsers operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @sample AmazonAppStream.DescribeUsers
* @see AWS API
* Documentation
*/
DescribeUsersResult describeUsers(DescribeUsersRequest describeUsersRequest);
/**
*
* Disables the specified user in the user pool. Users can't sign in to AppStream 2.0 until they are re-enabled.
* This action does not delete the user.
*
*
* @param disableUserRequest
* @return Result of the DisableUser operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.DisableUser
* @see AWS API
* Documentation
*/
DisableUserResult disableUser(DisableUserRequest disableUserRequest);
/**
*
* Disassociates the specified application from the fleet.
*
*
* @param disassociateApplicationFleetRequest
* @return Result of the DisassociateApplicationFleet operation returned by the service.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @sample AmazonAppStream.DisassociateApplicationFleet
* @see AWS API Documentation
*/
DisassociateApplicationFleetResult disassociateApplicationFleet(DisassociateApplicationFleetRequest disassociateApplicationFleetRequest);
/**
*
* Deletes the specified application from the specified entitlement.
*
*
* @param disassociateApplicationFromEntitlementRequest
* @return Result of the DisassociateApplicationFromEntitlement operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws EntitlementNotFoundException
* The entitlement can't be found.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @sample AmazonAppStream.DisassociateApplicationFromEntitlement
* @see AWS API Documentation
*/
DisassociateApplicationFromEntitlementResult disassociateApplicationFromEntitlement(
DisassociateApplicationFromEntitlementRequest disassociateApplicationFromEntitlementRequest);
/**
*
* Disassociates the specified fleet from the specified stack.
*
*
* @param disassociateFleetRequest
* @return Result of the DisassociateFleet operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @sample AmazonAppStream.DisassociateFleet
* @see AWS
* API Documentation
*/
DisassociateFleetResult disassociateFleet(DisassociateFleetRequest disassociateFleetRequest);
/**
*
* Enables a user in the user pool. After being enabled, users can sign in to AppStream 2.0 and open applications
* from the stacks to which they are assigned.
*
*
* @param enableUserRequest
* @return Result of the EnableUser operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @sample AmazonAppStream.EnableUser
* @see AWS API
* Documentation
*/
EnableUserResult enableUser(EnableUserRequest enableUserRequest);
/**
*
* Immediately stops the specified streaming session.
*
*
* @param expireSessionRequest
* @return Result of the ExpireSession operation returned by the service.
* @sample AmazonAppStream.ExpireSession
* @see AWS API
* Documentation
*/
ExpireSessionResult expireSession(ExpireSessionRequest expireSessionRequest);
/**
*
* Retrieves the name of the fleet that is associated with the specified stack.
*
*
* @param listAssociatedFleetsRequest
* @return Result of the ListAssociatedFleets operation returned by the service.
* @sample AmazonAppStream.ListAssociatedFleets
* @see AWS
* API Documentation
*/
ListAssociatedFleetsResult listAssociatedFleets(ListAssociatedFleetsRequest listAssociatedFleetsRequest);
/**
*
* Retrieves the name of the stack with which the specified fleet is associated.
*
*
* @param listAssociatedStacksRequest
* @return Result of the ListAssociatedStacks operation returned by the service.
* @sample AmazonAppStream.ListAssociatedStacks
* @see AWS
* API Documentation
*/
ListAssociatedStacksResult listAssociatedStacks(ListAssociatedStacksRequest listAssociatedStacksRequest);
/**
*
* Retrieves a list of entitled applications.
*
*
* @param listEntitledApplicationsRequest
* @return Result of the ListEntitledApplications operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws EntitlementNotFoundException
* The entitlement can't be found.
* @sample AmazonAppStream.ListEntitledApplications
* @see AWS API Documentation
*/
ListEntitledApplicationsResult listEntitledApplications(ListEntitledApplicationsRequest listEntitledApplicationsRequest);
/**
*
* Retrieves a list of all tags for the specified AppStream 2.0 resource. You can tag AppStream 2.0 image builders,
* images, fleets, and stacks.
*
*
* For more information about tags, see Tagging Your Resources
* in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.ListTagsForResource
* @see AWS
* API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Starts the specified fleet.
*
*
* @param startFleetRequest
* @return Result of the StartFleet operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws RequestLimitExceededException
* AppStream 2.0 can’t process the request right now because the Describe calls from your AWS account are
* being throttled by Amazon EC2. Try again later.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws InvalidRoleException
* The specified role is invalid.
* @sample AmazonAppStream.StartFleet
* @see AWS API
* Documentation
*/
StartFleetResult startFleet(StartFleetRequest startFleetRequest);
/**
*
* Starts the specified image builder.
*
*
* @param startImageBuilderRequest
* @return Result of the StartImageBuilder operation returned by the service.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws IncompatibleImageException
* The image can't be updated because it's not compatible for updates.
* @sample AmazonAppStream.StartImageBuilder
* @see AWS
* API Documentation
*/
StartImageBuilderResult startImageBuilder(StartImageBuilderRequest startImageBuilderRequest);
/**
*
* Stops the specified fleet.
*
*
* @param stopFleetRequest
* @return Result of the StopFleet operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @sample AmazonAppStream.StopFleet
* @see AWS API
* Documentation
*/
StopFleetResult stopFleet(StopFleetRequest stopFleetRequest);
/**
*
* Stops the specified image builder.
*
*
* @param stopImageBuilderRequest
* @return Result of the StopImageBuilder operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @sample AmazonAppStream.StopImageBuilder
* @see AWS API
* Documentation
*/
StopImageBuilderResult stopImageBuilder(StopImageBuilderRequest stopImageBuilderRequest);
/**
*
* Adds or overwrites one or more tags for the specified AppStream 2.0 resource. You can tag AppStream 2.0 image
* builders, images, fleets, and stacks.
*
*
* Each tag consists of a key and an optional value. If a resource already has a tag with the same key, this
* operation updates its value.
*
*
* To list the current tags for your resources, use ListTagsForResource. To disassociate tags from your
* resources, use UntagResource.
*
*
* For more information about tags, see Tagging Your Resources
* in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Disassociates one or more specified tags from the specified AppStream 2.0 resource.
*
*
* To list the current tags for your resources, use ListTagsForResource.
*
*
* For more information about tags, see Tagging Your Resources
* in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates the specified application.
*
*
* @param updateApplicationRequest
* @return Result of the UpdateApplication operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @sample AmazonAppStream.UpdateApplication
* @see AWS
* API Documentation
*/
UpdateApplicationResult updateApplication(UpdateApplicationRequest updateApplicationRequest);
/**
*
* Updates the specified Directory Config object in AppStream 2.0. This object includes the configuration
* information required to join fleets and image builders to Microsoft Active Directory domains.
*
*
* @param updateDirectoryConfigRequest
* @return Result of the UpdateDirectoryConfig operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws InvalidRoleException
* The specified role is invalid.
* @sample AmazonAppStream.UpdateDirectoryConfig
* @see AWS API Documentation
*/
UpdateDirectoryConfigResult updateDirectoryConfig(UpdateDirectoryConfigRequest updateDirectoryConfigRequest);
/**
*
* Updates the specified entitlement.
*
*
* @param updateEntitlementRequest
* @return Result of the UpdateEntitlement operation returned by the service.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws EntitlementNotFoundException
* The entitlement can't be found.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @sample AmazonAppStream.UpdateEntitlement
* @see AWS
* API Documentation
*/
UpdateEntitlementResult updateEntitlement(UpdateEntitlementRequest updateEntitlementRequest);
/**
*
* Updates the specified fleet.
*
*
* If the fleet is in the STOPPED
state, you can update any attribute except the fleet name.
*
*
* If the fleet is in the RUNNING
state, you can update the following based on the fleet type:
*
*
* -
*
* Always-On and On-Demand fleet types
*
*
* You can update the DisplayName
, ComputeCapacity
, ImageARN
,
* ImageName
, IdleDisconnectTimeoutInSeconds
, and DisconnectTimeoutInSeconds
* attributes.
*
*
* -
*
* Elastic fleet type
*
*
* You can update the DisplayName
, IdleDisconnectTimeoutInSeconds
,
* DisconnectTimeoutInSeconds
, MaxConcurrentSessions
, SessionScriptS3Location
* and UsbDeviceFilterStrings
attributes.
*
*
*
*
* If the fleet is in the STARTING
or STOPPED
state, you can't update it.
*
*
* @param updateFleetRequest
* @return Result of the UpdateFleet operation returned by the service.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws RequestLimitExceededException
* AppStream 2.0 can’t process the request right now because the Describe calls from your AWS account are
* being throttled by Amazon EC2. Try again later.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws InvalidRoleException
* The specified role is invalid.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @throws IncompatibleImageException
* The image can't be updated because it's not compatible for updates.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @sample AmazonAppStream.UpdateFleet
* @see AWS API
* Documentation
*/
UpdateFleetResult updateFleet(UpdateFleetRequest updateFleetRequest);
/**
*
* Adds or updates permissions for the specified private image.
*
*
* @param updateImagePermissionsRequest
* @return Result of the UpdateImagePermissions operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceNotAvailableException
* The specified resource exists and is not in use, but isn't available.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @sample AmazonAppStream.UpdateImagePermissions
* @see AWS API Documentation
*/
UpdateImagePermissionsResult updateImagePermissions(UpdateImagePermissionsRequest updateImagePermissionsRequest);
/**
*
* Updates the specified fields for the specified stack.
*
*
* @param updateStackRequest
* @return Result of the UpdateStack operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource was not found.
* @throws ResourceInUseException
* The specified resource is in use.
* @throws InvalidRoleException
* The specified role is invalid.
* @throws InvalidParameterCombinationException
* Indicates an incorrect combination of parameters, or a missing parameter.
* @throws LimitExceededException
* The requested limit exceeds the permitted limit for an account.
* @throws InvalidAccountStatusException
* The resource cannot be created because your AWS account is suspended. For assistance, contact AWS
* Support.
* @throws IncompatibleImageException
* The image can't be updated because it's not compatible for updates.
* @throws OperationNotPermittedException
* The attempted operation is not permitted.
* @throws ConcurrentModificationException
* An API error occurred. Wait a few minutes and try again.
* @sample AmazonAppStream.UpdateStack
* @see AWS API
* Documentation
*/
UpdateStackResult updateStack(UpdateStackRequest updateStackRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
AmazonAppStreamWaiters waiters();
}