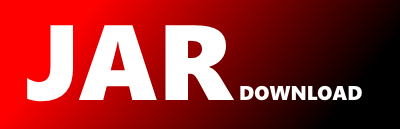
com.amazonaws.services.appstream.AmazonAppStreamAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appstream Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appstream;
import javax.annotation.Generated;
import com.amazonaws.services.appstream.model.*;
/**
* Interface for accessing Amazon AppStream asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.appstream.AbstractAmazonAppStreamAsync} instead.
*
*
* Amazon AppStream 2.0
*
* This is the Amazon AppStream 2.0 API Reference. This documentation provides descriptions and syntax for each
* of the actions and data types in AppStream 2.0. AppStream 2.0 is a fully managed, secure application streaming
* service that lets you stream desktop applications to users without rewriting applications. AppStream 2.0 manages the
* AWS resources that are required to host and run your applications, scales automatically, and provides access to your
* users on demand.
*
*
*
* You can call the AppStream 2.0 API operations by using an interface VPC endpoint (interface endpoint). For more
* information, see Access AppStream 2.0 API Operations and CLI Commands Through an Interface VPC Endpoint in the Amazon
* AppStream 2.0 Administration Guide.
*
*
*
* To learn more about AppStream 2.0, see the following resources:
*
*
* -
*
*
* -
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonAppStreamAsync extends AmazonAppStream {
/**
*
* Associates the specified application with the specified fleet. This is only supported for Elastic fleets.
*
*
* @param associateApplicationFleetRequest
* @return A Java Future containing the result of the AssociateApplicationFleet operation returned by the service.
* @sample AmazonAppStreamAsync.AssociateApplicationFleet
* @see AWS API Documentation
*/
java.util.concurrent.Future associateApplicationFleetAsync(
AssociateApplicationFleetRequest associateApplicationFleetRequest);
/**
*
* Associates the specified application with the specified fleet. This is only supported for Elastic fleets.
*
*
* @param associateApplicationFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateApplicationFleet operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.AssociateApplicationFleet
* @see AWS API Documentation
*/
java.util.concurrent.Future associateApplicationFleetAsync(
AssociateApplicationFleetRequest associateApplicationFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates an application to entitle.
*
*
* @param associateApplicationToEntitlementRequest
* @return A Java Future containing the result of the AssociateApplicationToEntitlement operation returned by the
* service.
* @sample AmazonAppStreamAsync.AssociateApplicationToEntitlement
* @see AWS API Documentation
*/
java.util.concurrent.Future associateApplicationToEntitlementAsync(
AssociateApplicationToEntitlementRequest associateApplicationToEntitlementRequest);
/**
*
* Associates an application to entitle.
*
*
* @param associateApplicationToEntitlementRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateApplicationToEntitlement operation returned by the
* service.
* @sample AmazonAppStreamAsyncHandler.AssociateApplicationToEntitlement
* @see AWS API Documentation
*/
java.util.concurrent.Future associateApplicationToEntitlementAsync(
AssociateApplicationToEntitlementRequest associateApplicationToEntitlementRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates the specified fleet with the specified stack.
*
*
* @param associateFleetRequest
* @return A Java Future containing the result of the AssociateFleet operation returned by the service.
* @sample AmazonAppStreamAsync.AssociateFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateFleetAsync(AssociateFleetRequest associateFleetRequest);
/**
*
* Associates the specified fleet with the specified stack.
*
*
* @param associateFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateFleet operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.AssociateFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateFleetAsync(AssociateFleetRequest associateFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates the specified users with the specified stacks. Users in a user pool cannot be assigned to stacks with
* fleets that are joined to an Active Directory domain.
*
*
* @param batchAssociateUserStackRequest
* @return A Java Future containing the result of the BatchAssociateUserStack operation returned by the service.
* @sample AmazonAppStreamAsync.BatchAssociateUserStack
* @see AWS API Documentation
*/
java.util.concurrent.Future batchAssociateUserStackAsync(BatchAssociateUserStackRequest batchAssociateUserStackRequest);
/**
*
* Associates the specified users with the specified stacks. Users in a user pool cannot be assigned to stacks with
* fleets that are joined to an Active Directory domain.
*
*
* @param batchAssociateUserStackRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchAssociateUserStack operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.BatchAssociateUserStack
* @see AWS API Documentation
*/
java.util.concurrent.Future batchAssociateUserStackAsync(BatchAssociateUserStackRequest batchAssociateUserStackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates the specified users from the specified stacks.
*
*
* @param batchDisassociateUserStackRequest
* @return A Java Future containing the result of the BatchDisassociateUserStack operation returned by the service.
* @sample AmazonAppStreamAsync.BatchDisassociateUserStack
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDisassociateUserStackAsync(
BatchDisassociateUserStackRequest batchDisassociateUserStackRequest);
/**
*
* Disassociates the specified users from the specified stacks.
*
*
* @param batchDisassociateUserStackRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchDisassociateUserStack operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.BatchDisassociateUserStack
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDisassociateUserStackAsync(
BatchDisassociateUserStackRequest batchDisassociateUserStackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Copies the image within the same region or to a new region within the same AWS account. Note that any tags you
* added to the image will not be copied.
*
*
* @param copyImageRequest
* @return A Java Future containing the result of the CopyImage operation returned by the service.
* @sample AmazonAppStreamAsync.CopyImage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyImageAsync(CopyImageRequest copyImageRequest);
/**
*
* Copies the image within the same region or to a new region within the same AWS account. Note that any tags you
* added to the image will not be copied.
*
*
* @param copyImageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopyImage operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.CopyImage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyImageAsync(CopyImageRequest copyImageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an app block.
*
*
* App blocks are an Amazon AppStream 2.0 resource that stores the details about the virtual hard disk in an S3
* bucket. It also stores the setup script with details about how to mount the virtual hard disk. The virtual hard
* disk includes the application binaries and other files necessary to launch your applications. Multiple
* applications can be assigned to a single app block.
*
*
* This is only supported for Elastic fleets.
*
*
* @param createAppBlockRequest
* @return A Java Future containing the result of the CreateAppBlock operation returned by the service.
* @sample AmazonAppStreamAsync.CreateAppBlock
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAppBlockAsync(CreateAppBlockRequest createAppBlockRequest);
/**
*
* Creates an app block.
*
*
* App blocks are an Amazon AppStream 2.0 resource that stores the details about the virtual hard disk in an S3
* bucket. It also stores the setup script with details about how to mount the virtual hard disk. The virtual hard
* disk includes the application binaries and other files necessary to launch your applications. Multiple
* applications can be assigned to a single app block.
*
*
* This is only supported for Elastic fleets.
*
*
* @param createAppBlockRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAppBlock operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.CreateAppBlock
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAppBlockAsync(CreateAppBlockRequest createAppBlockRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an application.
*
*
* Applications are an Amazon AppStream 2.0 resource that stores the details about how to launch applications on
* Elastic fleet streaming instances. An application consists of the launch details, icon, and display name.
* Applications are associated with an app block that contains the application binaries and other files. The
* applications assigned to an Elastic fleet are the applications users can launch.
*
*
* This is only supported for Elastic fleets.
*
*
* @param createApplicationRequest
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* @sample AmazonAppStreamAsync.CreateApplication
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createApplicationAsync(CreateApplicationRequest createApplicationRequest);
/**
*
* Creates an application.
*
*
* Applications are an Amazon AppStream 2.0 resource that stores the details about how to launch applications on
* Elastic fleet streaming instances. An application consists of the launch details, icon, and display name.
* Applications are associated with an app block that contains the application binaries and other files. The
* applications assigned to an Elastic fleet are the applications users can launch.
*
*
* This is only supported for Elastic fleets.
*
*
* @param createApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.CreateApplication
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createApplicationAsync(CreateApplicationRequest createApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Directory Config object in AppStream 2.0. This object includes the configuration information required
* to join fleets and image builders to Microsoft Active Directory domains.
*
*
* @param createDirectoryConfigRequest
* @return A Java Future containing the result of the CreateDirectoryConfig operation returned by the service.
* @sample AmazonAppStreamAsync.CreateDirectoryConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future createDirectoryConfigAsync(CreateDirectoryConfigRequest createDirectoryConfigRequest);
/**
*
* Creates a Directory Config object in AppStream 2.0. This object includes the configuration information required
* to join fleets and image builders to Microsoft Active Directory domains.
*
*
* @param createDirectoryConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDirectoryConfig operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.CreateDirectoryConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future createDirectoryConfigAsync(CreateDirectoryConfigRequest createDirectoryConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new entitlement. Entitlements control access to specific applications within a stack, based on user
* attributes. Entitlements apply to SAML 2.0 federated user identities. Amazon AppStream 2.0 user pool and
* streaming URL users are entitled to all applications in a stack. Entitlements don't apply to the desktop stream
* view application, or to applications managed by a dynamic app provider using the Dynamic Application Framework.
*
*
* @param createEntitlementRequest
* @return A Java Future containing the result of the CreateEntitlement operation returned by the service.
* @sample AmazonAppStreamAsync.CreateEntitlement
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createEntitlementAsync(CreateEntitlementRequest createEntitlementRequest);
/**
*
* Creates a new entitlement. Entitlements control access to specific applications within a stack, based on user
* attributes. Entitlements apply to SAML 2.0 federated user identities. Amazon AppStream 2.0 user pool and
* streaming URL users are entitled to all applications in a stack. Entitlements don't apply to the desktop stream
* view application, or to applications managed by a dynamic app provider using the Dynamic Application Framework.
*
*
* @param createEntitlementRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEntitlement operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.CreateEntitlement
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createEntitlementAsync(CreateEntitlementRequest createEntitlementRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a fleet. A fleet consists of streaming instances that your users access for their applications and
* desktops.
*
*
* @param createFleetRequest
* @return A Java Future containing the result of the CreateFleet operation returned by the service.
* @sample AmazonAppStreamAsync.CreateFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFleetAsync(CreateFleetRequest createFleetRequest);
/**
*
* Creates a fleet. A fleet consists of streaming instances that your users access for their applications and
* desktops.
*
*
* @param createFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFleet operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.CreateFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFleetAsync(CreateFleetRequest createFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an image builder. An image builder is a virtual machine that is used to create an image.
*
*
* The initial state of the builder is PENDING
. When it is ready, the state is RUNNING
.
*
*
* @param createImageBuilderRequest
* @return A Java Future containing the result of the CreateImageBuilder operation returned by the service.
* @sample AmazonAppStreamAsync.CreateImageBuilder
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createImageBuilderAsync(CreateImageBuilderRequest createImageBuilderRequest);
/**
*
* Creates an image builder. An image builder is a virtual machine that is used to create an image.
*
*
* The initial state of the builder is PENDING
. When it is ready, the state is RUNNING
.
*
*
* @param createImageBuilderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateImageBuilder operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.CreateImageBuilder
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createImageBuilderAsync(CreateImageBuilderRequest createImageBuilderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a URL to start an image builder streaming session.
*
*
* @param createImageBuilderStreamingURLRequest
* @return A Java Future containing the result of the CreateImageBuilderStreamingURL operation returned by the
* service.
* @sample AmazonAppStreamAsync.CreateImageBuilderStreamingURL
* @see AWS API Documentation
*/
java.util.concurrent.Future createImageBuilderStreamingURLAsync(
CreateImageBuilderStreamingURLRequest createImageBuilderStreamingURLRequest);
/**
*
* Creates a URL to start an image builder streaming session.
*
*
* @param createImageBuilderStreamingURLRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateImageBuilderStreamingURL operation returned by the
* service.
* @sample AmazonAppStreamAsyncHandler.CreateImageBuilderStreamingURL
* @see AWS API Documentation
*/
java.util.concurrent.Future createImageBuilderStreamingURLAsync(
CreateImageBuilderStreamingURLRequest createImageBuilderStreamingURLRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a stack to start streaming applications to users. A stack consists of an associated fleet, user access
* policies, and storage configurations.
*
*
* @param createStackRequest
* @return A Java Future containing the result of the CreateStack operation returned by the service.
* @sample AmazonAppStreamAsync.CreateStack
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createStackAsync(CreateStackRequest createStackRequest);
/**
*
* Creates a stack to start streaming applications to users. A stack consists of an associated fleet, user access
* policies, and storage configurations.
*
*
* @param createStackRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateStack operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.CreateStack
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createStackAsync(CreateStackRequest createStackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a temporary URL to start an AppStream 2.0 streaming session for the specified user. A streaming URL
* enables application streaming to be tested without user setup.
*
*
* @param createStreamingURLRequest
* @return A Java Future containing the result of the CreateStreamingURL operation returned by the service.
* @sample AmazonAppStreamAsync.CreateStreamingURL
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createStreamingURLAsync(CreateStreamingURLRequest createStreamingURLRequest);
/**
*
* Creates a temporary URL to start an AppStream 2.0 streaming session for the specified user. A streaming URL
* enables application streaming to be tested without user setup.
*
*
* @param createStreamingURLRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateStreamingURL operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.CreateStreamingURL
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createStreamingURLAsync(CreateStreamingURLRequest createStreamingURLRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new image with the latest Windows operating system updates, driver updates, and AppStream 2.0 agent
* software.
*
*
* For more information, see the "Update an Image by Using Managed AppStream 2.0 Image Updates" section in Administer Your
* AppStream 2.0 Images, in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param createUpdatedImageRequest
* @return A Java Future containing the result of the CreateUpdatedImage operation returned by the service.
* @sample AmazonAppStreamAsync.CreateUpdatedImage
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createUpdatedImageAsync(CreateUpdatedImageRequest createUpdatedImageRequest);
/**
*
* Creates a new image with the latest Windows operating system updates, driver updates, and AppStream 2.0 agent
* software.
*
*
* For more information, see the "Update an Image by Using Managed AppStream 2.0 Image Updates" section in Administer Your
* AppStream 2.0 Images, in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param createUpdatedImageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateUpdatedImage operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.CreateUpdatedImage
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createUpdatedImageAsync(CreateUpdatedImageRequest createUpdatedImageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a usage report subscription. Usage reports are generated daily.
*
*
* @param createUsageReportSubscriptionRequest
* @return A Java Future containing the result of the CreateUsageReportSubscription operation returned by the
* service.
* @sample AmazonAppStreamAsync.CreateUsageReportSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future createUsageReportSubscriptionAsync(
CreateUsageReportSubscriptionRequest createUsageReportSubscriptionRequest);
/**
*
* Creates a usage report subscription. Usage reports are generated daily.
*
*
* @param createUsageReportSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateUsageReportSubscription operation returned by the
* service.
* @sample AmazonAppStreamAsyncHandler.CreateUsageReportSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future createUsageReportSubscriptionAsync(
CreateUsageReportSubscriptionRequest createUsageReportSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new user in the user pool.
*
*
* @param createUserRequest
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* @sample AmazonAppStreamAsync.CreateUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createUserAsync(CreateUserRequest createUserRequest);
/**
*
* Creates a new user in the user pool.
*
*
* @param createUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.CreateUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createUserAsync(CreateUserRequest createUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an app block.
*
*
* @param deleteAppBlockRequest
* @return A Java Future containing the result of the DeleteAppBlock operation returned by the service.
* @sample AmazonAppStreamAsync.DeleteAppBlock
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAppBlockAsync(DeleteAppBlockRequest deleteAppBlockRequest);
/**
*
* Deletes an app block.
*
*
* @param deleteAppBlockRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAppBlock operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DeleteAppBlock
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAppBlockAsync(DeleteAppBlockRequest deleteAppBlockRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an application.
*
*
* @param deleteApplicationRequest
* @return A Java Future containing the result of the DeleteApplication operation returned by the service.
* @sample AmazonAppStreamAsync.DeleteApplication
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteApplicationAsync(DeleteApplicationRequest deleteApplicationRequest);
/**
*
* Deletes an application.
*
*
* @param deleteApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteApplication operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DeleteApplication
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteApplicationAsync(DeleteApplicationRequest deleteApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified Directory Config object from AppStream 2.0. This object includes the information required
* to join streaming instances to an Active Directory domain.
*
*
* @param deleteDirectoryConfigRequest
* @return A Java Future containing the result of the DeleteDirectoryConfig operation returned by the service.
* @sample AmazonAppStreamAsync.DeleteDirectoryConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDirectoryConfigAsync(DeleteDirectoryConfigRequest deleteDirectoryConfigRequest);
/**
*
* Deletes the specified Directory Config object from AppStream 2.0. This object includes the information required
* to join streaming instances to an Active Directory domain.
*
*
* @param deleteDirectoryConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDirectoryConfig operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DeleteDirectoryConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDirectoryConfigAsync(DeleteDirectoryConfigRequest deleteDirectoryConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified entitlement.
*
*
* @param deleteEntitlementRequest
* @return A Java Future containing the result of the DeleteEntitlement operation returned by the service.
* @sample AmazonAppStreamAsync.DeleteEntitlement
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteEntitlementAsync(DeleteEntitlementRequest deleteEntitlementRequest);
/**
*
* Deletes the specified entitlement.
*
*
* @param deleteEntitlementRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEntitlement operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DeleteEntitlement
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteEntitlementAsync(DeleteEntitlementRequest deleteEntitlementRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified fleet.
*
*
* @param deleteFleetRequest
* @return A Java Future containing the result of the DeleteFleet operation returned by the service.
* @sample AmazonAppStreamAsync.DeleteFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFleetAsync(DeleteFleetRequest deleteFleetRequest);
/**
*
* Deletes the specified fleet.
*
*
* @param deleteFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFleet operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DeleteFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFleetAsync(DeleteFleetRequest deleteFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified image. You cannot delete an image when it is in use. After you delete an image, you cannot
* provision new capacity using the image.
*
*
* @param deleteImageRequest
* @return A Java Future containing the result of the DeleteImage operation returned by the service.
* @sample AmazonAppStreamAsync.DeleteImage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteImageAsync(DeleteImageRequest deleteImageRequest);
/**
*
* Deletes the specified image. You cannot delete an image when it is in use. After you delete an image, you cannot
* provision new capacity using the image.
*
*
* @param deleteImageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteImage operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DeleteImage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteImageAsync(DeleteImageRequest deleteImageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified image builder and releases the capacity.
*
*
* @param deleteImageBuilderRequest
* @return A Java Future containing the result of the DeleteImageBuilder operation returned by the service.
* @sample AmazonAppStreamAsync.DeleteImageBuilder
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteImageBuilderAsync(DeleteImageBuilderRequest deleteImageBuilderRequest);
/**
*
* Deletes the specified image builder and releases the capacity.
*
*
* @param deleteImageBuilderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteImageBuilder operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DeleteImageBuilder
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteImageBuilderAsync(DeleteImageBuilderRequest deleteImageBuilderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes permissions for the specified private image. After you delete permissions for an image, AWS accounts to
* which you previously granted these permissions can no longer use the image.
*
*
* @param deleteImagePermissionsRequest
* @return A Java Future containing the result of the DeleteImagePermissions operation returned by the service.
* @sample AmazonAppStreamAsync.DeleteImagePermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteImagePermissionsAsync(DeleteImagePermissionsRequest deleteImagePermissionsRequest);
/**
*
* Deletes permissions for the specified private image. After you delete permissions for an image, AWS accounts to
* which you previously granted these permissions can no longer use the image.
*
*
* @param deleteImagePermissionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteImagePermissions operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DeleteImagePermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteImagePermissionsAsync(DeleteImagePermissionsRequest deleteImagePermissionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified stack. After the stack is deleted, the application streaming environment provided by the
* stack is no longer available to users. Also, any reservations made for application streaming sessions for the
* stack are released.
*
*
* @param deleteStackRequest
* @return A Java Future containing the result of the DeleteStack operation returned by the service.
* @sample AmazonAppStreamAsync.DeleteStack
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteStackAsync(DeleteStackRequest deleteStackRequest);
/**
*
* Deletes the specified stack. After the stack is deleted, the application streaming environment provided by the
* stack is no longer available to users. Also, any reservations made for application streaming sessions for the
* stack are released.
*
*
* @param deleteStackRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteStack operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DeleteStack
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteStackAsync(DeleteStackRequest deleteStackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disables usage report generation.
*
*
* @param deleteUsageReportSubscriptionRequest
* @return A Java Future containing the result of the DeleteUsageReportSubscription operation returned by the
* service.
* @sample AmazonAppStreamAsync.DeleteUsageReportSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteUsageReportSubscriptionAsync(
DeleteUsageReportSubscriptionRequest deleteUsageReportSubscriptionRequest);
/**
*
* Disables usage report generation.
*
*
* @param deleteUsageReportSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteUsageReportSubscription operation returned by the
* service.
* @sample AmazonAppStreamAsyncHandler.DeleteUsageReportSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteUsageReportSubscriptionAsync(
DeleteUsageReportSubscriptionRequest deleteUsageReportSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a user from the user pool.
*
*
* @param deleteUserRequest
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* @sample AmazonAppStreamAsync.DeleteUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteUserAsync(DeleteUserRequest deleteUserRequest);
/**
*
* Deletes a user from the user pool.
*
*
* @param deleteUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DeleteUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteUserAsync(DeleteUserRequest deleteUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list that describes one or more app blocks.
*
*
* @param describeAppBlocksRequest
* @return A Java Future containing the result of the DescribeAppBlocks operation returned by the service.
* @sample AmazonAppStreamAsync.DescribeAppBlocks
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeAppBlocksAsync(DescribeAppBlocksRequest describeAppBlocksRequest);
/**
*
* Retrieves a list that describes one or more app blocks.
*
*
* @param describeAppBlocksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAppBlocks operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DescribeAppBlocks
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeAppBlocksAsync(DescribeAppBlocksRequest describeAppBlocksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list that describes one or more application fleet associations. Either ApplicationArn or FleetName
* must be specified.
*
*
* @param describeApplicationFleetAssociationsRequest
* @return A Java Future containing the result of the DescribeApplicationFleetAssociations operation returned by the
* service.
* @sample AmazonAppStreamAsync.DescribeApplicationFleetAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeApplicationFleetAssociationsAsync(
DescribeApplicationFleetAssociationsRequest describeApplicationFleetAssociationsRequest);
/**
*
* Retrieves a list that describes one or more application fleet associations. Either ApplicationArn or FleetName
* must be specified.
*
*
* @param describeApplicationFleetAssociationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeApplicationFleetAssociations operation returned by the
* service.
* @sample AmazonAppStreamAsyncHandler.DescribeApplicationFleetAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeApplicationFleetAssociationsAsync(
DescribeApplicationFleetAssociationsRequest describeApplicationFleetAssociationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list that describes one or more applications.
*
*
* @param describeApplicationsRequest
* @return A Java Future containing the result of the DescribeApplications operation returned by the service.
* @sample AmazonAppStreamAsync.DescribeApplications
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeApplicationsAsync(DescribeApplicationsRequest describeApplicationsRequest);
/**
*
* Retrieves a list that describes one or more applications.
*
*
* @param describeApplicationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeApplications operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DescribeApplications
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeApplicationsAsync(DescribeApplicationsRequest describeApplicationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list that describes one or more specified Directory Config objects for AppStream 2.0, if the names
* for these objects are provided. Otherwise, all Directory Config objects in the account are described. These
* objects include the configuration information required to join fleets and image builders to Microsoft Active
* Directory domains.
*
*
* Although the response syntax in this topic includes the account password, this password is not returned in the
* actual response.
*
*
* @param describeDirectoryConfigsRequest
* @return A Java Future containing the result of the DescribeDirectoryConfigs operation returned by the service.
* @sample AmazonAppStreamAsync.DescribeDirectoryConfigs
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDirectoryConfigsAsync(DescribeDirectoryConfigsRequest describeDirectoryConfigsRequest);
/**
*
* Retrieves a list that describes one or more specified Directory Config objects for AppStream 2.0, if the names
* for these objects are provided. Otherwise, all Directory Config objects in the account are described. These
* objects include the configuration information required to join fleets and image builders to Microsoft Active
* Directory domains.
*
*
* Although the response syntax in this topic includes the account password, this password is not returned in the
* actual response.
*
*
* @param describeDirectoryConfigsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDirectoryConfigs operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DescribeDirectoryConfigs
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDirectoryConfigsAsync(DescribeDirectoryConfigsRequest describeDirectoryConfigsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list that describes one of more entitlements.
*
*
* @param describeEntitlementsRequest
* @return A Java Future containing the result of the DescribeEntitlements operation returned by the service.
* @sample AmazonAppStreamAsync.DescribeEntitlements
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeEntitlementsAsync(DescribeEntitlementsRequest describeEntitlementsRequest);
/**
*
* Retrieves a list that describes one of more entitlements.
*
*
* @param describeEntitlementsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEntitlements operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DescribeEntitlements
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeEntitlementsAsync(DescribeEntitlementsRequest describeEntitlementsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list that describes one or more specified fleets, if the fleet names are provided. Otherwise, all
* fleets in the account are described.
*
*
* @param describeFleetsRequest
* @return A Java Future containing the result of the DescribeFleets operation returned by the service.
* @sample AmazonAppStreamAsync.DescribeFleets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeFleetsAsync(DescribeFleetsRequest describeFleetsRequest);
/**
*
* Retrieves a list that describes one or more specified fleets, if the fleet names are provided. Otherwise, all
* fleets in the account are described.
*
*
* @param describeFleetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFleets operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DescribeFleets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeFleetsAsync(DescribeFleetsRequest describeFleetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list that describes one or more specified image builders, if the image builder names are provided.
* Otherwise, all image builders in the account are described.
*
*
* @param describeImageBuildersRequest
* @return A Java Future containing the result of the DescribeImageBuilders operation returned by the service.
* @sample AmazonAppStreamAsync.DescribeImageBuilders
* @see AWS API Documentation
*/
java.util.concurrent.Future describeImageBuildersAsync(DescribeImageBuildersRequest describeImageBuildersRequest);
/**
*
* Retrieves a list that describes one or more specified image builders, if the image builder names are provided.
* Otherwise, all image builders in the account are described.
*
*
* @param describeImageBuildersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeImageBuilders operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DescribeImageBuilders
* @see AWS API Documentation
*/
java.util.concurrent.Future describeImageBuildersAsync(DescribeImageBuildersRequest describeImageBuildersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list that describes the permissions for shared AWS account IDs on a private image that you own.
*
*
* @param describeImagePermissionsRequest
* @return A Java Future containing the result of the DescribeImagePermissions operation returned by the service.
* @sample AmazonAppStreamAsync.DescribeImagePermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeImagePermissionsAsync(DescribeImagePermissionsRequest describeImagePermissionsRequest);
/**
*
* Retrieves a list that describes the permissions for shared AWS account IDs on a private image that you own.
*
*
* @param describeImagePermissionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeImagePermissions operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DescribeImagePermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeImagePermissionsAsync(DescribeImagePermissionsRequest describeImagePermissionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list that describes one or more specified images, if the image names or image ARNs are provided.
* Otherwise, all images in the account are described.
*
*
* @param describeImagesRequest
* @return A Java Future containing the result of the DescribeImages operation returned by the service.
* @sample AmazonAppStreamAsync.DescribeImages
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeImagesAsync(DescribeImagesRequest describeImagesRequest);
/**
*
* Retrieves a list that describes one or more specified images, if the image names or image ARNs are provided.
* Otherwise, all images in the account are described.
*
*
* @param describeImagesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeImages operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DescribeImages
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeImagesAsync(DescribeImagesRequest describeImagesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list that describes the streaming sessions for a specified stack and fleet. If a UserId is provided
* for the stack and fleet, only streaming sessions for that user are described. If an authentication type is not
* provided, the default is to authenticate users using a streaming URL.
*
*
* @param describeSessionsRequest
* @return A Java Future containing the result of the DescribeSessions operation returned by the service.
* @sample AmazonAppStreamAsync.DescribeSessions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSessionsAsync(DescribeSessionsRequest describeSessionsRequest);
/**
*
* Retrieves a list that describes the streaming sessions for a specified stack and fleet. If a UserId is provided
* for the stack and fleet, only streaming sessions for that user are described. If an authentication type is not
* provided, the default is to authenticate users using a streaming URL.
*
*
* @param describeSessionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSessions operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DescribeSessions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSessionsAsync(DescribeSessionsRequest describeSessionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list that describes one or more specified stacks, if the stack names are provided. Otherwise, all
* stacks in the account are described.
*
*
* @param describeStacksRequest
* @return A Java Future containing the result of the DescribeStacks operation returned by the service.
* @sample AmazonAppStreamAsync.DescribeStacks
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeStacksAsync(DescribeStacksRequest describeStacksRequest);
/**
*
* Retrieves a list that describes one or more specified stacks, if the stack names are provided. Otherwise, all
* stacks in the account are described.
*
*
* @param describeStacksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeStacks operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DescribeStacks
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeStacksAsync(DescribeStacksRequest describeStacksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list that describes one or more usage report subscriptions.
*
*
* @param describeUsageReportSubscriptionsRequest
* @return A Java Future containing the result of the DescribeUsageReportSubscriptions operation returned by the
* service.
* @sample AmazonAppStreamAsync.DescribeUsageReportSubscriptions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeUsageReportSubscriptionsAsync(
DescribeUsageReportSubscriptionsRequest describeUsageReportSubscriptionsRequest);
/**
*
* Retrieves a list that describes one or more usage report subscriptions.
*
*
* @param describeUsageReportSubscriptionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeUsageReportSubscriptions operation returned by the
* service.
* @sample AmazonAppStreamAsyncHandler.DescribeUsageReportSubscriptions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeUsageReportSubscriptionsAsync(
DescribeUsageReportSubscriptionsRequest describeUsageReportSubscriptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list that describes the UserStackAssociation objects. You must specify either or both of the
* following:
*
*
* -
*
* The stack name
*
*
* -
*
* The user name (email address of the user associated with the stack) and the authentication type for the user
*
*
*
*
* @param describeUserStackAssociationsRequest
* @return A Java Future containing the result of the DescribeUserStackAssociations operation returned by the
* service.
* @sample AmazonAppStreamAsync.DescribeUserStackAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeUserStackAssociationsAsync(
DescribeUserStackAssociationsRequest describeUserStackAssociationsRequest);
/**
*
* Retrieves a list that describes the UserStackAssociation objects. You must specify either or both of the
* following:
*
*
* -
*
* The stack name
*
*
* -
*
* The user name (email address of the user associated with the stack) and the authentication type for the user
*
*
*
*
* @param describeUserStackAssociationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeUserStackAssociations operation returned by the
* service.
* @sample AmazonAppStreamAsyncHandler.DescribeUserStackAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeUserStackAssociationsAsync(
DescribeUserStackAssociationsRequest describeUserStackAssociationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list that describes one or more specified users in the user pool.
*
*
* @param describeUsersRequest
* @return A Java Future containing the result of the DescribeUsers operation returned by the service.
* @sample AmazonAppStreamAsync.DescribeUsers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeUsersAsync(DescribeUsersRequest describeUsersRequest);
/**
*
* Retrieves a list that describes one or more specified users in the user pool.
*
*
* @param describeUsersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeUsers operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DescribeUsers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeUsersAsync(DescribeUsersRequest describeUsersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disables the specified user in the user pool. Users can't sign in to AppStream 2.0 until they are re-enabled.
* This action does not delete the user.
*
*
* @param disableUserRequest
* @return A Java Future containing the result of the DisableUser operation returned by the service.
* @sample AmazonAppStreamAsync.DisableUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future disableUserAsync(DisableUserRequest disableUserRequest);
/**
*
* Disables the specified user in the user pool. Users can't sign in to AppStream 2.0 until they are re-enabled.
* This action does not delete the user.
*
*
* @param disableUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisableUser operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DisableUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future disableUserAsync(DisableUserRequest disableUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates the specified application from the fleet.
*
*
* @param disassociateApplicationFleetRequest
* @return A Java Future containing the result of the DisassociateApplicationFleet operation returned by the
* service.
* @sample AmazonAppStreamAsync.DisassociateApplicationFleet
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateApplicationFleetAsync(
DisassociateApplicationFleetRequest disassociateApplicationFleetRequest);
/**
*
* Disassociates the specified application from the fleet.
*
*
* @param disassociateApplicationFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateApplicationFleet operation returned by the
* service.
* @sample AmazonAppStreamAsyncHandler.DisassociateApplicationFleet
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateApplicationFleetAsync(
DisassociateApplicationFleetRequest disassociateApplicationFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified application from the specified entitlement.
*
*
* @param disassociateApplicationFromEntitlementRequest
* @return A Java Future containing the result of the DisassociateApplicationFromEntitlement operation returned by
* the service.
* @sample AmazonAppStreamAsync.DisassociateApplicationFromEntitlement
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateApplicationFromEntitlementAsync(
DisassociateApplicationFromEntitlementRequest disassociateApplicationFromEntitlementRequest);
/**
*
* Deletes the specified application from the specified entitlement.
*
*
* @param disassociateApplicationFromEntitlementRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateApplicationFromEntitlement operation returned by
* the service.
* @sample AmazonAppStreamAsyncHandler.DisassociateApplicationFromEntitlement
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateApplicationFromEntitlementAsync(
DisassociateApplicationFromEntitlementRequest disassociateApplicationFromEntitlementRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates the specified fleet from the specified stack.
*
*
* @param disassociateFleetRequest
* @return A Java Future containing the result of the DisassociateFleet operation returned by the service.
* @sample AmazonAppStreamAsync.DisassociateFleet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future disassociateFleetAsync(DisassociateFleetRequest disassociateFleetRequest);
/**
*
* Disassociates the specified fleet from the specified stack.
*
*
* @param disassociateFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateFleet operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.DisassociateFleet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future disassociateFleetAsync(DisassociateFleetRequest disassociateFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables a user in the user pool. After being enabled, users can sign in to AppStream 2.0 and open applications
* from the stacks to which they are assigned.
*
*
* @param enableUserRequest
* @return A Java Future containing the result of the EnableUser operation returned by the service.
* @sample AmazonAppStreamAsync.EnableUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future enableUserAsync(EnableUserRequest enableUserRequest);
/**
*
* Enables a user in the user pool. After being enabled, users can sign in to AppStream 2.0 and open applications
* from the stacks to which they are assigned.
*
*
* @param enableUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the EnableUser operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.EnableUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future enableUserAsync(EnableUserRequest enableUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Immediately stops the specified streaming session.
*
*
* @param expireSessionRequest
* @return A Java Future containing the result of the ExpireSession operation returned by the service.
* @sample AmazonAppStreamAsync.ExpireSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future expireSessionAsync(ExpireSessionRequest expireSessionRequest);
/**
*
* Immediately stops the specified streaming session.
*
*
* @param expireSessionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ExpireSession operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.ExpireSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future expireSessionAsync(ExpireSessionRequest expireSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the name of the fleet that is associated with the specified stack.
*
*
* @param listAssociatedFleetsRequest
* @return A Java Future containing the result of the ListAssociatedFleets operation returned by the service.
* @sample AmazonAppStreamAsync.ListAssociatedFleets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAssociatedFleetsAsync(ListAssociatedFleetsRequest listAssociatedFleetsRequest);
/**
*
* Retrieves the name of the fleet that is associated with the specified stack.
*
*
* @param listAssociatedFleetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAssociatedFleets operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.ListAssociatedFleets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAssociatedFleetsAsync(ListAssociatedFleetsRequest listAssociatedFleetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the name of the stack with which the specified fleet is associated.
*
*
* @param listAssociatedStacksRequest
* @return A Java Future containing the result of the ListAssociatedStacks operation returned by the service.
* @sample AmazonAppStreamAsync.ListAssociatedStacks
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAssociatedStacksAsync(ListAssociatedStacksRequest listAssociatedStacksRequest);
/**
*
* Retrieves the name of the stack with which the specified fleet is associated.
*
*
* @param listAssociatedStacksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAssociatedStacks operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.ListAssociatedStacks
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAssociatedStacksAsync(ListAssociatedStacksRequest listAssociatedStacksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of entitled applications.
*
*
* @param listEntitledApplicationsRequest
* @return A Java Future containing the result of the ListEntitledApplications operation returned by the service.
* @sample AmazonAppStreamAsync.ListEntitledApplications
* @see AWS API Documentation
*/
java.util.concurrent.Future listEntitledApplicationsAsync(ListEntitledApplicationsRequest listEntitledApplicationsRequest);
/**
*
* Retrieves a list of entitled applications.
*
*
* @param listEntitledApplicationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListEntitledApplications operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.ListEntitledApplications
* @see AWS API Documentation
*/
java.util.concurrent.Future listEntitledApplicationsAsync(ListEntitledApplicationsRequest listEntitledApplicationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of all tags for the specified AppStream 2.0 resource. You can tag AppStream 2.0 image builders,
* images, fleets, and stacks.
*
*
* For more information about tags, see Tagging Your Resources
* in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonAppStreamAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Retrieves a list of all tags for the specified AppStream 2.0 resource. You can tag AppStream 2.0 image builders,
* images, fleets, and stacks.
*
*
* For more information about tags, see Tagging Your Resources
* in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts the specified fleet.
*
*
* @param startFleetRequest
* @return A Java Future containing the result of the StartFleet operation returned by the service.
* @sample AmazonAppStreamAsync.StartFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startFleetAsync(StartFleetRequest startFleetRequest);
/**
*
* Starts the specified fleet.
*
*
* @param startFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartFleet operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.StartFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startFleetAsync(StartFleetRequest startFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts the specified image builder.
*
*
* @param startImageBuilderRequest
* @return A Java Future containing the result of the StartImageBuilder operation returned by the service.
* @sample AmazonAppStreamAsync.StartImageBuilder
* @see AWS
* API Documentation
*/
java.util.concurrent.Future startImageBuilderAsync(StartImageBuilderRequest startImageBuilderRequest);
/**
*
* Starts the specified image builder.
*
*
* @param startImageBuilderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartImageBuilder operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.StartImageBuilder
* @see AWS
* API Documentation
*/
java.util.concurrent.Future startImageBuilderAsync(StartImageBuilderRequest startImageBuilderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops the specified fleet.
*
*
* @param stopFleetRequest
* @return A Java Future containing the result of the StopFleet operation returned by the service.
* @sample AmazonAppStreamAsync.StopFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopFleetAsync(StopFleetRequest stopFleetRequest);
/**
*
* Stops the specified fleet.
*
*
* @param stopFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopFleet operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.StopFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopFleetAsync(StopFleetRequest stopFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops the specified image builder.
*
*
* @param stopImageBuilderRequest
* @return A Java Future containing the result of the StopImageBuilder operation returned by the service.
* @sample AmazonAppStreamAsync.StopImageBuilder
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopImageBuilderAsync(StopImageBuilderRequest stopImageBuilderRequest);
/**
*
* Stops the specified image builder.
*
*
* @param stopImageBuilderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopImageBuilder operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.StopImageBuilder
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopImageBuilderAsync(StopImageBuilderRequest stopImageBuilderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds or overwrites one or more tags for the specified AppStream 2.0 resource. You can tag AppStream 2.0 image
* builders, images, fleets, and stacks.
*
*
* Each tag consists of a key and an optional value. If a resource already has a tag with the same key, this
* operation updates its value.
*
*
* To list the current tags for your resources, use ListTagsForResource. To disassociate tags from your
* resources, use UntagResource.
*
*
* For more information about tags, see Tagging Your Resources
* in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonAppStreamAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds or overwrites one or more tags for the specified AppStream 2.0 resource. You can tag AppStream 2.0 image
* builders, images, fleets, and stacks.
*
*
* Each tag consists of a key and an optional value. If a resource already has a tag with the same key, this
* operation updates its value.
*
*
* To list the current tags for your resources, use ListTagsForResource. To disassociate tags from your
* resources, use UntagResource.
*
*
* For more information about tags, see Tagging Your Resources
* in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates one or more specified tags from the specified AppStream 2.0 resource.
*
*
* To list the current tags for your resources, use ListTagsForResource.
*
*
* For more information about tags, see Tagging Your Resources
* in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonAppStreamAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Disassociates one or more specified tags from the specified AppStream 2.0 resource.
*
*
* To list the current tags for your resources, use ListTagsForResource.
*
*
* For more information about tags, see Tagging Your Resources
* in the Amazon AppStream 2.0 Administration Guide.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified application.
*
*
* @param updateApplicationRequest
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* @sample AmazonAppStreamAsync.UpdateApplication
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateApplicationAsync(UpdateApplicationRequest updateApplicationRequest);
/**
*
* Updates the specified application.
*
*
* @param updateApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.UpdateApplication
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateApplicationAsync(UpdateApplicationRequest updateApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified Directory Config object in AppStream 2.0. This object includes the configuration
* information required to join fleets and image builders to Microsoft Active Directory domains.
*
*
* @param updateDirectoryConfigRequest
* @return A Java Future containing the result of the UpdateDirectoryConfig operation returned by the service.
* @sample AmazonAppStreamAsync.UpdateDirectoryConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDirectoryConfigAsync(UpdateDirectoryConfigRequest updateDirectoryConfigRequest);
/**
*
* Updates the specified Directory Config object in AppStream 2.0. This object includes the configuration
* information required to join fleets and image builders to Microsoft Active Directory domains.
*
*
* @param updateDirectoryConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDirectoryConfig operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.UpdateDirectoryConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDirectoryConfigAsync(UpdateDirectoryConfigRequest updateDirectoryConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified entitlement.
*
*
* @param updateEntitlementRequest
* @return A Java Future containing the result of the UpdateEntitlement operation returned by the service.
* @sample AmazonAppStreamAsync.UpdateEntitlement
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateEntitlementAsync(UpdateEntitlementRequest updateEntitlementRequest);
/**
*
* Updates the specified entitlement.
*
*
* @param updateEntitlementRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateEntitlement operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.UpdateEntitlement
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateEntitlementAsync(UpdateEntitlementRequest updateEntitlementRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified fleet.
*
*
* If the fleet is in the STOPPED
state, you can update any attribute except the fleet name.
*
*
* If the fleet is in the RUNNING
state, you can update the following based on the fleet type:
*
*
* -
*
* Always-On and On-Demand fleet types
*
*
* You can update the DisplayName
, ComputeCapacity
, ImageARN
,
* ImageName
, IdleDisconnectTimeoutInSeconds
, and DisconnectTimeoutInSeconds
* attributes.
*
*
* -
*
* Elastic fleet type
*
*
* You can update the DisplayName
, IdleDisconnectTimeoutInSeconds
,
* DisconnectTimeoutInSeconds
, MaxConcurrentSessions
, SessionScriptS3Location
* and UsbDeviceFilterStrings
attributes.
*
*
*
*
* If the fleet is in the STARTING
or STOPPED
state, you can't update it.
*
*
* @param updateFleetRequest
* @return A Java Future containing the result of the UpdateFleet operation returned by the service.
* @sample AmazonAppStreamAsync.UpdateFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateFleetAsync(UpdateFleetRequest updateFleetRequest);
/**
*
* Updates the specified fleet.
*
*
* If the fleet is in the STOPPED
state, you can update any attribute except the fleet name.
*
*
* If the fleet is in the RUNNING
state, you can update the following based on the fleet type:
*
*
* -
*
* Always-On and On-Demand fleet types
*
*
* You can update the DisplayName
, ComputeCapacity
, ImageARN
,
* ImageName
, IdleDisconnectTimeoutInSeconds
, and DisconnectTimeoutInSeconds
* attributes.
*
*
* -
*
* Elastic fleet type
*
*
* You can update the DisplayName
, IdleDisconnectTimeoutInSeconds
,
* DisconnectTimeoutInSeconds
, MaxConcurrentSessions
, SessionScriptS3Location
* and UsbDeviceFilterStrings
attributes.
*
*
*
*
* If the fleet is in the STARTING
or STOPPED
state, you can't update it.
*
*
* @param updateFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateFleet operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.UpdateFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateFleetAsync(UpdateFleetRequest updateFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds or updates permissions for the specified private image.
*
*
* @param updateImagePermissionsRequest
* @return A Java Future containing the result of the UpdateImagePermissions operation returned by the service.
* @sample AmazonAppStreamAsync.UpdateImagePermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future updateImagePermissionsAsync(UpdateImagePermissionsRequest updateImagePermissionsRequest);
/**
*
* Adds or updates permissions for the specified private image.
*
*
* @param updateImagePermissionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateImagePermissions operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.UpdateImagePermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future updateImagePermissionsAsync(UpdateImagePermissionsRequest updateImagePermissionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified fields for the specified stack.
*
*
* @param updateStackRequest
* @return A Java Future containing the result of the UpdateStack operation returned by the service.
* @sample AmazonAppStreamAsync.UpdateStack
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateStackAsync(UpdateStackRequest updateStackRequest);
/**
*
* Updates the specified fields for the specified stack.
*
*
* @param updateStackRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateStack operation returned by the service.
* @sample AmazonAppStreamAsyncHandler.UpdateStack
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateStackAsync(UpdateStackRequest updateStackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}