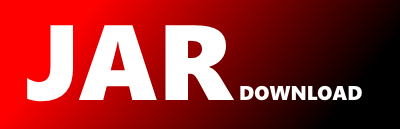
com.amazonaws.services.appstream.model.CreateUserRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appstream Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appstream.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateUserRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The email address of the user.
*
*
*
* Users' email addresses are case-sensitive. During login, if they specify an email address that doesn't use the
* same capitalization as the email address specified when their user pool account was created, a
* "user does not exist" error message displays.
*
*
*/
private String userName;
/**
*
* The action to take for the welcome email that is sent to a user after the user is created in the user pool. If
* you specify SUPPRESS, no email is sent. If you specify RESEND, do not specify the first name or last name of the
* user. If the value is null, the email is sent.
*
*
*
* The temporary password in the welcome email is valid for only 7 days. If users don’t set their passwords within 7
* days, you must send them a new welcome email.
*
*
*/
private String messageAction;
/**
*
* The first name, or given name, of the user.
*
*/
private String firstName;
/**
*
* The last name, or surname, of the user.
*
*/
private String lastName;
/**
*
* The authentication type for the user. You must specify USERPOOL.
*
*/
private String authenticationType;
/**
*
* The email address of the user.
*
*
*
* Users' email addresses are case-sensitive. During login, if they specify an email address that doesn't use the
* same capitalization as the email address specified when their user pool account was created, a
* "user does not exist" error message displays.
*
*
*
* @param userName
* The email address of the user.
*
* Users' email addresses are case-sensitive. During login, if they specify an email address that doesn't use
* the same capitalization as the email address specified when their user pool account was created, a
* "user does not exist" error message displays.
*
*/
public void setUserName(String userName) {
this.userName = userName;
}
/**
*
* The email address of the user.
*
*
*
* Users' email addresses are case-sensitive. During login, if they specify an email address that doesn't use the
* same capitalization as the email address specified when their user pool account was created, a
* "user does not exist" error message displays.
*
*
*
* @return The email address of the user.
*
* Users' email addresses are case-sensitive. During login, if they specify an email address that doesn't
* use the same capitalization as the email address specified when their user pool account was created, a
* "user does not exist" error message displays.
*
*/
public String getUserName() {
return this.userName;
}
/**
*
* The email address of the user.
*
*
*
* Users' email addresses are case-sensitive. During login, if they specify an email address that doesn't use the
* same capitalization as the email address specified when their user pool account was created, a
* "user does not exist" error message displays.
*
*
*
* @param userName
* The email address of the user.
*
* Users' email addresses are case-sensitive. During login, if they specify an email address that doesn't use
* the same capitalization as the email address specified when their user pool account was created, a
* "user does not exist" error message displays.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateUserRequest withUserName(String userName) {
setUserName(userName);
return this;
}
/**
*
* The action to take for the welcome email that is sent to a user after the user is created in the user pool. If
* you specify SUPPRESS, no email is sent. If you specify RESEND, do not specify the first name or last name of the
* user. If the value is null, the email is sent.
*
*
*
* The temporary password in the welcome email is valid for only 7 days. If users don’t set their passwords within 7
* days, you must send them a new welcome email.
*
*
*
* @param messageAction
* The action to take for the welcome email that is sent to a user after the user is created in the user
* pool. If you specify SUPPRESS, no email is sent. If you specify RESEND, do not specify the first name or
* last name of the user. If the value is null, the email is sent.
*
* The temporary password in the welcome email is valid for only 7 days. If users don’t set their passwords
* within 7 days, you must send them a new welcome email.
*
* @see MessageAction
*/
public void setMessageAction(String messageAction) {
this.messageAction = messageAction;
}
/**
*
* The action to take for the welcome email that is sent to a user after the user is created in the user pool. If
* you specify SUPPRESS, no email is sent. If you specify RESEND, do not specify the first name or last name of the
* user. If the value is null, the email is sent.
*
*
*
* The temporary password in the welcome email is valid for only 7 days. If users don’t set their passwords within 7
* days, you must send them a new welcome email.
*
*
*
* @return The action to take for the welcome email that is sent to a user after the user is created in the user
* pool. If you specify SUPPRESS, no email is sent. If you specify RESEND, do not specify the first name or
* last name of the user. If the value is null, the email is sent.
*
* The temporary password in the welcome email is valid for only 7 days. If users don’t set their passwords
* within 7 days, you must send them a new welcome email.
*
* @see MessageAction
*/
public String getMessageAction() {
return this.messageAction;
}
/**
*
* The action to take for the welcome email that is sent to a user after the user is created in the user pool. If
* you specify SUPPRESS, no email is sent. If you specify RESEND, do not specify the first name or last name of the
* user. If the value is null, the email is sent.
*
*
*
* The temporary password in the welcome email is valid for only 7 days. If users don’t set their passwords within 7
* days, you must send them a new welcome email.
*
*
*
* @param messageAction
* The action to take for the welcome email that is sent to a user after the user is created in the user
* pool. If you specify SUPPRESS, no email is sent. If you specify RESEND, do not specify the first name or
* last name of the user. If the value is null, the email is sent.
*
* The temporary password in the welcome email is valid for only 7 days. If users don’t set their passwords
* within 7 days, you must send them a new welcome email.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see MessageAction
*/
public CreateUserRequest withMessageAction(String messageAction) {
setMessageAction(messageAction);
return this;
}
/**
*
* The action to take for the welcome email that is sent to a user after the user is created in the user pool. If
* you specify SUPPRESS, no email is sent. If you specify RESEND, do not specify the first name or last name of the
* user. If the value is null, the email is sent.
*
*
*
* The temporary password in the welcome email is valid for only 7 days. If users don’t set their passwords within 7
* days, you must send them a new welcome email.
*
*
*
* @param messageAction
* The action to take for the welcome email that is sent to a user after the user is created in the user
* pool. If you specify SUPPRESS, no email is sent. If you specify RESEND, do not specify the first name or
* last name of the user. If the value is null, the email is sent.
*
* The temporary password in the welcome email is valid for only 7 days. If users don’t set their passwords
* within 7 days, you must send them a new welcome email.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see MessageAction
*/
public CreateUserRequest withMessageAction(MessageAction messageAction) {
this.messageAction = messageAction.toString();
return this;
}
/**
*
* The first name, or given name, of the user.
*
*
* @param firstName
* The first name, or given name, of the user.
*/
public void setFirstName(String firstName) {
this.firstName = firstName;
}
/**
*
* The first name, or given name, of the user.
*
*
* @return The first name, or given name, of the user.
*/
public String getFirstName() {
return this.firstName;
}
/**
*
* The first name, or given name, of the user.
*
*
* @param firstName
* The first name, or given name, of the user.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateUserRequest withFirstName(String firstName) {
setFirstName(firstName);
return this;
}
/**
*
* The last name, or surname, of the user.
*
*
* @param lastName
* The last name, or surname, of the user.
*/
public void setLastName(String lastName) {
this.lastName = lastName;
}
/**
*
* The last name, or surname, of the user.
*
*
* @return The last name, or surname, of the user.
*/
public String getLastName() {
return this.lastName;
}
/**
*
* The last name, or surname, of the user.
*
*
* @param lastName
* The last name, or surname, of the user.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateUserRequest withLastName(String lastName) {
setLastName(lastName);
return this;
}
/**
*
* The authentication type for the user. You must specify USERPOOL.
*
*
* @param authenticationType
* The authentication type for the user. You must specify USERPOOL.
* @see AuthenticationType
*/
public void setAuthenticationType(String authenticationType) {
this.authenticationType = authenticationType;
}
/**
*
* The authentication type for the user. You must specify USERPOOL.
*
*
* @return The authentication type for the user. You must specify USERPOOL.
* @see AuthenticationType
*/
public String getAuthenticationType() {
return this.authenticationType;
}
/**
*
* The authentication type for the user. You must specify USERPOOL.
*
*
* @param authenticationType
* The authentication type for the user. You must specify USERPOOL.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthenticationType
*/
public CreateUserRequest withAuthenticationType(String authenticationType) {
setAuthenticationType(authenticationType);
return this;
}
/**
*
* The authentication type for the user. You must specify USERPOOL.
*
*
* @param authenticationType
* The authentication type for the user. You must specify USERPOOL.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthenticationType
*/
public CreateUserRequest withAuthenticationType(AuthenticationType authenticationType) {
this.authenticationType = authenticationType.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getUserName() != null)
sb.append("UserName: ").append("***Sensitive Data Redacted***").append(",");
if (getMessageAction() != null)
sb.append("MessageAction: ").append(getMessageAction()).append(",");
if (getFirstName() != null)
sb.append("FirstName: ").append("***Sensitive Data Redacted***").append(",");
if (getLastName() != null)
sb.append("LastName: ").append("***Sensitive Data Redacted***").append(",");
if (getAuthenticationType() != null)
sb.append("AuthenticationType: ").append(getAuthenticationType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateUserRequest == false)
return false;
CreateUserRequest other = (CreateUserRequest) obj;
if (other.getUserName() == null ^ this.getUserName() == null)
return false;
if (other.getUserName() != null && other.getUserName().equals(this.getUserName()) == false)
return false;
if (other.getMessageAction() == null ^ this.getMessageAction() == null)
return false;
if (other.getMessageAction() != null && other.getMessageAction().equals(this.getMessageAction()) == false)
return false;
if (other.getFirstName() == null ^ this.getFirstName() == null)
return false;
if (other.getFirstName() != null && other.getFirstName().equals(this.getFirstName()) == false)
return false;
if (other.getLastName() == null ^ this.getLastName() == null)
return false;
if (other.getLastName() != null && other.getLastName().equals(this.getLastName()) == false)
return false;
if (other.getAuthenticationType() == null ^ this.getAuthenticationType() == null)
return false;
if (other.getAuthenticationType() != null && other.getAuthenticationType().equals(this.getAuthenticationType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getUserName() == null) ? 0 : getUserName().hashCode());
hashCode = prime * hashCode + ((getMessageAction() == null) ? 0 : getMessageAction().hashCode());
hashCode = prime * hashCode + ((getFirstName() == null) ? 0 : getFirstName().hashCode());
hashCode = prime * hashCode + ((getLastName() == null) ? 0 : getLastName().hashCode());
hashCode = prime * hashCode + ((getAuthenticationType() == null) ? 0 : getAuthenticationType().hashCode());
return hashCode;
}
@Override
public CreateUserRequest clone() {
return (CreateUserRequest) super.clone();
}
}