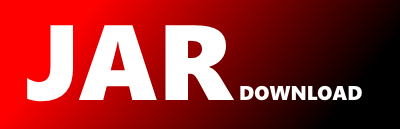
com.amazonaws.services.appsync.AWSAppSync Maven / Gradle / Ivy
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appsync;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.appsync.model.*;
/**
* Interface for accessing AWSAppSync.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.appsync.AbstractAWSAppSync} instead.
*
*
*
* AWS AppSync provides API actions for creating and interacting with data sources using GraphQL from your application.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSAppSync {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "appsync";
/**
*
* Creates a unique key that you can distribute to clients who are executing your API.
*
*
* @param createApiKeyRequest
* @return Result of the CreateApiKey operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @throws ApiKeyLimitExceededException
* The API key exceeded a limit. Try your request again.
* @sample AWSAppSync.CreateApiKey
* @see AWS API
* Documentation
*/
CreateApiKeyResult createApiKey(CreateApiKeyRequest createApiKeyRequest);
/**
*
* Creates a DataSource
object.
*
*
* @param createDataSourceRequest
* @return Result of the CreateDataSource operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws ConcurrentModificationException
* Another modification is being made. That modification must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.CreateDataSource
* @see AWS API
* Documentation
*/
CreateDataSourceResult createDataSource(CreateDataSourceRequest createDataSourceRequest);
/**
*
* Creates a GraphqlApi
object.
*
*
* @param createGraphqlApiRequest
* @return Result of the CreateGraphqlApi operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws ConcurrentModificationException
* Another modification is being made. That modification must complete before you can make your change.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws ApiLimitExceededException
* The GraphQL API exceeded a limit. Try your request again.
* @sample AWSAppSync.CreateGraphqlApi
* @see AWS API
* Documentation
*/
CreateGraphqlApiResult createGraphqlApi(CreateGraphqlApiRequest createGraphqlApiRequest);
/**
*
* Creates a Resolver
object.
*
*
* A resolver converts incoming requests into a format that a data source can understand and converts the data
* source's responses into GraphQL.
*
*
* @param createResolverRequest
* @return Result of the CreateResolver operation returned by the service.
* @throws ConcurrentModificationException
* Another modification is being made. That modification must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.CreateResolver
* @see AWS API
* Documentation
*/
CreateResolverResult createResolver(CreateResolverRequest createResolverRequest);
/**
*
* Creates a Type
object.
*
*
* @param createTypeRequest
* @return Result of the CreateType operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws ConcurrentModificationException
* Another modification is being made. That modification must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.CreateType
* @see AWS API
* Documentation
*/
CreateTypeResult createType(CreateTypeRequest createTypeRequest);
/**
*
* Deletes an API key.
*
*
* @param deleteApiKeyRequest
* @return Result of the DeleteApiKey operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.DeleteApiKey
* @see AWS API
* Documentation
*/
DeleteApiKeyResult deleteApiKey(DeleteApiKeyRequest deleteApiKeyRequest);
/**
*
* Deletes a DataSource
object.
*
*
* @param deleteDataSourceRequest
* @return Result of the DeleteDataSource operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws ConcurrentModificationException
* Another modification is being made. That modification must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.DeleteDataSource
* @see AWS API
* Documentation
*/
DeleteDataSourceResult deleteDataSource(DeleteDataSourceRequest deleteDataSourceRequest);
/**
*
* Deletes a GraphqlApi
object.
*
*
* @param deleteGraphqlApiRequest
* @return Result of the DeleteGraphqlApi operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws ConcurrentModificationException
* Another modification is being made. That modification must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.DeleteGraphqlApi
* @see AWS API
* Documentation
*/
DeleteGraphqlApiResult deleteGraphqlApi(DeleteGraphqlApiRequest deleteGraphqlApiRequest);
/**
*
* Deletes a Resolver
object.
*
*
* @param deleteResolverRequest
* @return Result of the DeleteResolver operation returned by the service.
* @throws ConcurrentModificationException
* Another modification is being made. That modification must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.DeleteResolver
* @see AWS API
* Documentation
*/
DeleteResolverResult deleteResolver(DeleteResolverRequest deleteResolverRequest);
/**
*
* Deletes a Type
object.
*
*
* @param deleteTypeRequest
* @return Result of the DeleteType operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws ConcurrentModificationException
* Another modification is being made. That modification must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.DeleteType
* @see AWS API
* Documentation
*/
DeleteTypeResult deleteType(DeleteTypeRequest deleteTypeRequest);
/**
*
* Retrieves a DataSource
object.
*
*
* @param getDataSourceRequest
* @return Result of the GetDataSource operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws ConcurrentModificationException
* Another modification is being made. That modification must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.GetDataSource
* @see AWS API
* Documentation
*/
GetDataSourceResult getDataSource(GetDataSourceRequest getDataSourceRequest);
/**
*
* Retrieves a GraphqlApi
object.
*
*
* @param getGraphqlApiRequest
* @return Result of the GetGraphqlApi operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.GetGraphqlApi
* @see AWS API
* Documentation
*/
GetGraphqlApiResult getGraphqlApi(GetGraphqlApiRequest getGraphqlApiRequest);
/**
*
* Retrieves the introspection schema for a GraphQL API.
*
*
* @param getIntrospectionSchemaRequest
* @return Result of the GetIntrospectionSchema operation returned by the service.
* @throws GraphQLSchemaException
* The GraphQL schema is not valid.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.GetIntrospectionSchema
* @see AWS
* API Documentation
*/
GetIntrospectionSchemaResult getIntrospectionSchema(GetIntrospectionSchemaRequest getIntrospectionSchemaRequest);
/**
*
* Retrieves a Resolver
object.
*
*
* @param getResolverRequest
* @return Result of the GetResolver operation returned by the service.
* @throws ConcurrentModificationException
* Another modification is being made. That modification must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @sample AWSAppSync.GetResolver
* @see AWS API
* Documentation
*/
GetResolverResult getResolver(GetResolverRequest getResolverRequest);
/**
*
* Retrieves the current status of a schema creation operation.
*
*
* @param getSchemaCreationStatusRequest
* @return Result of the GetSchemaCreationStatus operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.GetSchemaCreationStatus
* @see AWS API Documentation
*/
GetSchemaCreationStatusResult getSchemaCreationStatus(GetSchemaCreationStatusRequest getSchemaCreationStatusRequest);
/**
*
* Retrieves a Type
object.
*
*
* @param getTypeRequest
* @return Result of the GetType operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws ConcurrentModificationException
* Another modification is being made. That modification must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.GetType
* @see AWS API
* Documentation
*/
GetTypeResult getType(GetTypeRequest getTypeRequest);
/**
*
* Lists the API keys for a given API.
*
*
* @param listApiKeysRequest
* @return Result of the ListApiKeys operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListApiKeys
* @see AWS API
* Documentation
*/
ListApiKeysResult listApiKeys(ListApiKeysRequest listApiKeysRequest);
/**
*
* Lists the data sources for a given API.
*
*
* @param listDataSourcesRequest
* @return Result of the ListDataSources operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListDataSources
* @see AWS API
* Documentation
*/
ListDataSourcesResult listDataSources(ListDataSourcesRequest listDataSourcesRequest);
/**
*
* Lists your GraphQL APIs.
*
*
* @param listGraphqlApisRequest
* @return Result of the ListGraphqlApis operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListGraphqlApis
* @see AWS API
* Documentation
*/
ListGraphqlApisResult listGraphqlApis(ListGraphqlApisRequest listGraphqlApisRequest);
/**
*
* Lists the resolvers for a given API and type.
*
*
* @param listResolversRequest
* @return Result of the ListResolvers operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListResolvers
* @see AWS API
* Documentation
*/
ListResolversResult listResolvers(ListResolversRequest listResolversRequest);
/**
*
* Lists the types for a given API.
*
*
* @param listTypesRequest
* @return Result of the ListTypes operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws ConcurrentModificationException
* Another modification is being made. That modification must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListTypes
* @see AWS API
* Documentation
*/
ListTypesResult listTypes(ListTypesRequest listTypesRequest);
/**
*
* Adds a new schema to your GraphQL API.
*
*
* This operation is asynchronous. Use to determine when it has completed.
*
*
* @param startSchemaCreationRequest
* @return Result of the StartSchemaCreation operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws ConcurrentModificationException
* Another modification is being made. That modification must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.StartSchemaCreation
* @see AWS
* API Documentation
*/
StartSchemaCreationResult startSchemaCreation(StartSchemaCreationRequest startSchemaCreationRequest);
/**
*
* Updates a DataSource
object.
*
*
* @param updateDataSourceRequest
* @return Result of the UpdateDataSource operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws ConcurrentModificationException
* Another modification is being made. That modification must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.UpdateDataSource
* @see AWS API
* Documentation
*/
UpdateDataSourceResult updateDataSource(UpdateDataSourceRequest updateDataSourceRequest);
/**
*
* Updates a GraphqlApi
object.
*
*
* @param updateGraphqlApiRequest
* @return Result of the UpdateGraphqlApi operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws ConcurrentModificationException
* Another modification is being made. That modification must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.UpdateGraphqlApi
* @see AWS API
* Documentation
*/
UpdateGraphqlApiResult updateGraphqlApi(UpdateGraphqlApiRequest updateGraphqlApiRequest);
/**
*
* Updates a Resolver
object.
*
*
* @param updateResolverRequest
* @return Result of the UpdateResolver operation returned by the service.
* @throws ConcurrentModificationException
* Another modification is being made. That modification must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.UpdateResolver
* @see AWS API
* Documentation
*/
UpdateResolverResult updateResolver(UpdateResolverRequest updateResolverRequest);
/**
*
* Updates a Type
object.
*
*
* @param updateTypeRequest
* @return Result of the UpdateType operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws ConcurrentModificationException
* Another modification is being made. That modification must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws UnauthorizedException
* You are not authorized to perform this operation.
* @throws InternalFailureException
* An internal AWS AppSync error occurred. Try your request again.
* @sample AWSAppSync.UpdateType
* @see AWS API
* Documentation
*/
UpdateTypeResult updateType(UpdateTypeRequest updateTypeRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}