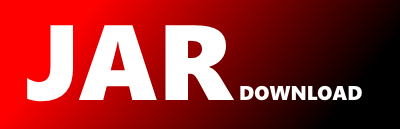
com.amazonaws.services.appsync.model.UserPoolConfig Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appsync Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appsync.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes an Amazon Cognito User Pool configuration.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UserPoolConfig implements Serializable, Cloneable, StructuredPojo {
/**
*
* The user pool ID.
*
*/
private String userPoolId;
/**
*
* The AWS region in which the user pool was created.
*
*/
private String awsRegion;
/**
*
* The action that you want your GraphQL API to take when a request that uses Amazon Cognito User Pool
* authentication doesn't match the Amazon Cognito User Pool configuration.
*
*/
private String defaultAction;
/**
*
* A regular expression for validating the incoming Amazon Cognito User Pool app client ID.
*
*/
private String appIdClientRegex;
/**
*
* The user pool ID.
*
*
* @param userPoolId
* The user pool ID.
*/
public void setUserPoolId(String userPoolId) {
this.userPoolId = userPoolId;
}
/**
*
* The user pool ID.
*
*
* @return The user pool ID.
*/
public String getUserPoolId() {
return this.userPoolId;
}
/**
*
* The user pool ID.
*
*
* @param userPoolId
* The user pool ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserPoolConfig withUserPoolId(String userPoolId) {
setUserPoolId(userPoolId);
return this;
}
/**
*
* The AWS region in which the user pool was created.
*
*
* @param awsRegion
* The AWS region in which the user pool was created.
*/
public void setAwsRegion(String awsRegion) {
this.awsRegion = awsRegion;
}
/**
*
* The AWS region in which the user pool was created.
*
*
* @return The AWS region in which the user pool was created.
*/
public String getAwsRegion() {
return this.awsRegion;
}
/**
*
* The AWS region in which the user pool was created.
*
*
* @param awsRegion
* The AWS region in which the user pool was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserPoolConfig withAwsRegion(String awsRegion) {
setAwsRegion(awsRegion);
return this;
}
/**
*
* The action that you want your GraphQL API to take when a request that uses Amazon Cognito User Pool
* authentication doesn't match the Amazon Cognito User Pool configuration.
*
*
* @param defaultAction
* The action that you want your GraphQL API to take when a request that uses Amazon Cognito User Pool
* authentication doesn't match the Amazon Cognito User Pool configuration.
* @see DefaultAction
*/
public void setDefaultAction(String defaultAction) {
this.defaultAction = defaultAction;
}
/**
*
* The action that you want your GraphQL API to take when a request that uses Amazon Cognito User Pool
* authentication doesn't match the Amazon Cognito User Pool configuration.
*
*
* @return The action that you want your GraphQL API to take when a request that uses Amazon Cognito User Pool
* authentication doesn't match the Amazon Cognito User Pool configuration.
* @see DefaultAction
*/
public String getDefaultAction() {
return this.defaultAction;
}
/**
*
* The action that you want your GraphQL API to take when a request that uses Amazon Cognito User Pool
* authentication doesn't match the Amazon Cognito User Pool configuration.
*
*
* @param defaultAction
* The action that you want your GraphQL API to take when a request that uses Amazon Cognito User Pool
* authentication doesn't match the Amazon Cognito User Pool configuration.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DefaultAction
*/
public UserPoolConfig withDefaultAction(String defaultAction) {
setDefaultAction(defaultAction);
return this;
}
/**
*
* The action that you want your GraphQL API to take when a request that uses Amazon Cognito User Pool
* authentication doesn't match the Amazon Cognito User Pool configuration.
*
*
* @param defaultAction
* The action that you want your GraphQL API to take when a request that uses Amazon Cognito User Pool
* authentication doesn't match the Amazon Cognito User Pool configuration.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DefaultAction
*/
public UserPoolConfig withDefaultAction(DefaultAction defaultAction) {
this.defaultAction = defaultAction.toString();
return this;
}
/**
*
* A regular expression for validating the incoming Amazon Cognito User Pool app client ID.
*
*
* @param appIdClientRegex
* A regular expression for validating the incoming Amazon Cognito User Pool app client ID.
*/
public void setAppIdClientRegex(String appIdClientRegex) {
this.appIdClientRegex = appIdClientRegex;
}
/**
*
* A regular expression for validating the incoming Amazon Cognito User Pool app client ID.
*
*
* @return A regular expression for validating the incoming Amazon Cognito User Pool app client ID.
*/
public String getAppIdClientRegex() {
return this.appIdClientRegex;
}
/**
*
* A regular expression for validating the incoming Amazon Cognito User Pool app client ID.
*
*
* @param appIdClientRegex
* A regular expression for validating the incoming Amazon Cognito User Pool app client ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserPoolConfig withAppIdClientRegex(String appIdClientRegex) {
setAppIdClientRegex(appIdClientRegex);
return this;
}
/**
* Returns a string representation of this object; useful for testing and debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getUserPoolId() != null)
sb.append("UserPoolId: ").append(getUserPoolId()).append(",");
if (getAwsRegion() != null)
sb.append("AwsRegion: ").append(getAwsRegion()).append(",");
if (getDefaultAction() != null)
sb.append("DefaultAction: ").append(getDefaultAction()).append(",");
if (getAppIdClientRegex() != null)
sb.append("AppIdClientRegex: ").append(getAppIdClientRegex());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UserPoolConfig == false)
return false;
UserPoolConfig other = (UserPoolConfig) obj;
if (other.getUserPoolId() == null ^ this.getUserPoolId() == null)
return false;
if (other.getUserPoolId() != null && other.getUserPoolId().equals(this.getUserPoolId()) == false)
return false;
if (other.getAwsRegion() == null ^ this.getAwsRegion() == null)
return false;
if (other.getAwsRegion() != null && other.getAwsRegion().equals(this.getAwsRegion()) == false)
return false;
if (other.getDefaultAction() == null ^ this.getDefaultAction() == null)
return false;
if (other.getDefaultAction() != null && other.getDefaultAction().equals(this.getDefaultAction()) == false)
return false;
if (other.getAppIdClientRegex() == null ^ this.getAppIdClientRegex() == null)
return false;
if (other.getAppIdClientRegex() != null && other.getAppIdClientRegex().equals(this.getAppIdClientRegex()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getUserPoolId() == null) ? 0 : getUserPoolId().hashCode());
hashCode = prime * hashCode + ((getAwsRegion() == null) ? 0 : getAwsRegion().hashCode());
hashCode = prime * hashCode + ((getDefaultAction() == null) ? 0 : getDefaultAction().hashCode());
hashCode = prime * hashCode + ((getAppIdClientRegex() == null) ? 0 : getAppIdClientRegex().hashCode());
return hashCode;
}
@Override
public UserPoolConfig clone() {
try {
return (UserPoolConfig) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.appsync.model.transform.UserPoolConfigMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}