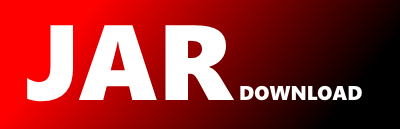
com.amazonaws.services.appsync.AWSAppSyncAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appsync Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appsync;
import javax.annotation.Generated;
import com.amazonaws.services.appsync.model.*;
/**
* Interface for accessing AWSAppSync asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.appsync.AbstractAWSAppSyncAsync} instead.
*
*
*
* AppSync provides API actions for creating and interacting with data sources using GraphQL from your application.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSAppSyncAsync extends AWSAppSync {
/**
*
* Maps an endpoint to your custom domain.
*
*
* @param associateApiRequest
* @return A Java Future containing the result of the AssociateApi operation returned by the service.
* @sample AWSAppSyncAsync.AssociateApi
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateApiAsync(AssociateApiRequest associateApiRequest);
/**
*
* Maps an endpoint to your custom domain.
*
*
* @param associateApiRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateApi operation returned by the service.
* @sample AWSAppSyncAsyncHandler.AssociateApi
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateApiAsync(AssociateApiRequest associateApiRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an association between a Merged API and source API using the source API's identifier.
*
*
* @param associateMergedGraphqlApiRequest
* @return A Java Future containing the result of the AssociateMergedGraphqlApi operation returned by the service.
* @sample AWSAppSyncAsync.AssociateMergedGraphqlApi
* @see AWS API Documentation
*/
java.util.concurrent.Future associateMergedGraphqlApiAsync(
AssociateMergedGraphqlApiRequest associateMergedGraphqlApiRequest);
/**
*
* Creates an association between a Merged API and source API using the source API's identifier.
*
*
* @param associateMergedGraphqlApiRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateMergedGraphqlApi operation returned by the service.
* @sample AWSAppSyncAsyncHandler.AssociateMergedGraphqlApi
* @see AWS API Documentation
*/
java.util.concurrent.Future associateMergedGraphqlApiAsync(
AssociateMergedGraphqlApiRequest associateMergedGraphqlApiRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an association between a Merged API and source API using the Merged API's identifier.
*
*
* @param associateSourceGraphqlApiRequest
* @return A Java Future containing the result of the AssociateSourceGraphqlApi operation returned by the service.
* @sample AWSAppSyncAsync.AssociateSourceGraphqlApi
* @see AWS API Documentation
*/
java.util.concurrent.Future associateSourceGraphqlApiAsync(
AssociateSourceGraphqlApiRequest associateSourceGraphqlApiRequest);
/**
*
* Creates an association between a Merged API and source API using the Merged API's identifier.
*
*
* @param associateSourceGraphqlApiRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateSourceGraphqlApi operation returned by the service.
* @sample AWSAppSyncAsyncHandler.AssociateSourceGraphqlApi
* @see AWS API Documentation
*/
java.util.concurrent.Future associateSourceGraphqlApiAsync(
AssociateSourceGraphqlApiRequest associateSourceGraphqlApiRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a cache for the GraphQL API.
*
*
* @param createApiCacheRequest
* Represents the input of a CreateApiCache
operation.
* @return A Java Future containing the result of the CreateApiCache operation returned by the service.
* @sample AWSAppSyncAsync.CreateApiCache
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createApiCacheAsync(CreateApiCacheRequest createApiCacheRequest);
/**
*
* Creates a cache for the GraphQL API.
*
*
* @param createApiCacheRequest
* Represents the input of a CreateApiCache
operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateApiCache operation returned by the service.
* @sample AWSAppSyncAsyncHandler.CreateApiCache
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createApiCacheAsync(CreateApiCacheRequest createApiCacheRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a unique key that you can distribute to clients who invoke your API.
*
*
* @param createApiKeyRequest
* @return A Java Future containing the result of the CreateApiKey operation returned by the service.
* @sample AWSAppSyncAsync.CreateApiKey
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createApiKeyAsync(CreateApiKeyRequest createApiKeyRequest);
/**
*
* Creates a unique key that you can distribute to clients who invoke your API.
*
*
* @param createApiKeyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateApiKey operation returned by the service.
* @sample AWSAppSyncAsyncHandler.CreateApiKey
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createApiKeyAsync(CreateApiKeyRequest createApiKeyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a DataSource
object.
*
*
* @param createDataSourceRequest
* @return A Java Future containing the result of the CreateDataSource operation returned by the service.
* @sample AWSAppSyncAsync.CreateDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDataSourceAsync(CreateDataSourceRequest createDataSourceRequest);
/**
*
* Creates a DataSource
object.
*
*
* @param createDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDataSource operation returned by the service.
* @sample AWSAppSyncAsyncHandler.CreateDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDataSourceAsync(CreateDataSourceRequest createDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a custom DomainName
object.
*
*
* @param createDomainNameRequest
* @return A Java Future containing the result of the CreateDomainName operation returned by the service.
* @sample AWSAppSyncAsync.CreateDomainName
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDomainNameAsync(CreateDomainNameRequest createDomainNameRequest);
/**
*
* Creates a custom DomainName
object.
*
*
* @param createDomainNameRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDomainName operation returned by the service.
* @sample AWSAppSyncAsyncHandler.CreateDomainName
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDomainNameAsync(CreateDomainNameRequest createDomainNameRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Function
object.
*
*
* A function is a reusable entity. You can use multiple functions to compose the resolver logic.
*
*
* @param createFunctionRequest
* @return A Java Future containing the result of the CreateFunction operation returned by the service.
* @sample AWSAppSyncAsync.CreateFunction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFunctionAsync(CreateFunctionRequest createFunctionRequest);
/**
*
* Creates a Function
object.
*
*
* A function is a reusable entity. You can use multiple functions to compose the resolver logic.
*
*
* @param createFunctionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFunction operation returned by the service.
* @sample AWSAppSyncAsyncHandler.CreateFunction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFunctionAsync(CreateFunctionRequest createFunctionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a GraphqlApi
object.
*
*
* @param createGraphqlApiRequest
* @return A Java Future containing the result of the CreateGraphqlApi operation returned by the service.
* @sample AWSAppSyncAsync.CreateGraphqlApi
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createGraphqlApiAsync(CreateGraphqlApiRequest createGraphqlApiRequest);
/**
*
* Creates a GraphqlApi
object.
*
*
* @param createGraphqlApiRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateGraphqlApi operation returned by the service.
* @sample AWSAppSyncAsyncHandler.CreateGraphqlApi
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createGraphqlApiAsync(CreateGraphqlApiRequest createGraphqlApiRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Resolver
object.
*
*
* A resolver converts incoming requests into a format that a data source can understand, and converts the data
* source's responses into GraphQL.
*
*
* @param createResolverRequest
* @return A Java Future containing the result of the CreateResolver operation returned by the service.
* @sample AWSAppSyncAsync.CreateResolver
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createResolverAsync(CreateResolverRequest createResolverRequest);
/**
*
* Creates a Resolver
object.
*
*
* A resolver converts incoming requests into a format that a data source can understand, and converts the data
* source's responses into GraphQL.
*
*
* @param createResolverRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateResolver operation returned by the service.
* @sample AWSAppSyncAsyncHandler.CreateResolver
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createResolverAsync(CreateResolverRequest createResolverRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Type
object.
*
*
* @param createTypeRequest
* @return A Java Future containing the result of the CreateType operation returned by the service.
* @sample AWSAppSyncAsync.CreateType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTypeAsync(CreateTypeRequest createTypeRequest);
/**
*
* Creates a Type
object.
*
*
* @param createTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateType operation returned by the service.
* @sample AWSAppSyncAsyncHandler.CreateType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTypeAsync(CreateTypeRequest createTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an ApiCache
object.
*
*
* @param deleteApiCacheRequest
* Represents the input of a DeleteApiCache
operation.
* @return A Java Future containing the result of the DeleteApiCache operation returned by the service.
* @sample AWSAppSyncAsync.DeleteApiCache
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteApiCacheAsync(DeleteApiCacheRequest deleteApiCacheRequest);
/**
*
* Deletes an ApiCache
object.
*
*
* @param deleteApiCacheRequest
* Represents the input of a DeleteApiCache
operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteApiCache operation returned by the service.
* @sample AWSAppSyncAsyncHandler.DeleteApiCache
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteApiCacheAsync(DeleteApiCacheRequest deleteApiCacheRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an API key.
*
*
* @param deleteApiKeyRequest
* @return A Java Future containing the result of the DeleteApiKey operation returned by the service.
* @sample AWSAppSyncAsync.DeleteApiKey
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteApiKeyAsync(DeleteApiKeyRequest deleteApiKeyRequest);
/**
*
* Deletes an API key.
*
*
* @param deleteApiKeyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteApiKey operation returned by the service.
* @sample AWSAppSyncAsyncHandler.DeleteApiKey
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteApiKeyAsync(DeleteApiKeyRequest deleteApiKeyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a DataSource
object.
*
*
* @param deleteDataSourceRequest
* @return A Java Future containing the result of the DeleteDataSource operation returned by the service.
* @sample AWSAppSyncAsync.DeleteDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDataSourceAsync(DeleteDataSourceRequest deleteDataSourceRequest);
/**
*
* Deletes a DataSource
object.
*
*
* @param deleteDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDataSource operation returned by the service.
* @sample AWSAppSyncAsyncHandler.DeleteDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDataSourceAsync(DeleteDataSourceRequest deleteDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a custom DomainName
object.
*
*
* @param deleteDomainNameRequest
* @return A Java Future containing the result of the DeleteDomainName operation returned by the service.
* @sample AWSAppSyncAsync.DeleteDomainName
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDomainNameAsync(DeleteDomainNameRequest deleteDomainNameRequest);
/**
*
* Deletes a custom DomainName
object.
*
*
* @param deleteDomainNameRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDomainName operation returned by the service.
* @sample AWSAppSyncAsyncHandler.DeleteDomainName
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDomainNameAsync(DeleteDomainNameRequest deleteDomainNameRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a Function
.
*
*
* @param deleteFunctionRequest
* @return A Java Future containing the result of the DeleteFunction operation returned by the service.
* @sample AWSAppSyncAsync.DeleteFunction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFunctionAsync(DeleteFunctionRequest deleteFunctionRequest);
/**
*
* Deletes a Function
.
*
*
* @param deleteFunctionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFunction operation returned by the service.
* @sample AWSAppSyncAsyncHandler.DeleteFunction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFunctionAsync(DeleteFunctionRequest deleteFunctionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a GraphqlApi
object.
*
*
* @param deleteGraphqlApiRequest
* @return A Java Future containing the result of the DeleteGraphqlApi operation returned by the service.
* @sample AWSAppSyncAsync.DeleteGraphqlApi
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteGraphqlApiAsync(DeleteGraphqlApiRequest deleteGraphqlApiRequest);
/**
*
* Deletes a GraphqlApi
object.
*
*
* @param deleteGraphqlApiRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteGraphqlApi operation returned by the service.
* @sample AWSAppSyncAsyncHandler.DeleteGraphqlApi
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteGraphqlApiAsync(DeleteGraphqlApiRequest deleteGraphqlApiRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a Resolver
object.
*
*
* @param deleteResolverRequest
* @return A Java Future containing the result of the DeleteResolver operation returned by the service.
* @sample AWSAppSyncAsync.DeleteResolver
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteResolverAsync(DeleteResolverRequest deleteResolverRequest);
/**
*
* Deletes a Resolver
object.
*
*
* @param deleteResolverRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteResolver operation returned by the service.
* @sample AWSAppSyncAsyncHandler.DeleteResolver
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteResolverAsync(DeleteResolverRequest deleteResolverRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a Type
object.
*
*
* @param deleteTypeRequest
* @return A Java Future containing the result of the DeleteType operation returned by the service.
* @sample AWSAppSyncAsync.DeleteType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTypeAsync(DeleteTypeRequest deleteTypeRequest);
/**
*
* Deletes a Type
object.
*
*
* @param deleteTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteType operation returned by the service.
* @sample AWSAppSyncAsyncHandler.DeleteType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTypeAsync(DeleteTypeRequest deleteTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes an ApiAssociation
object from a custom domain.
*
*
* @param disassociateApiRequest
* @return A Java Future containing the result of the DisassociateApi operation returned by the service.
* @sample AWSAppSyncAsync.DisassociateApi
* @see AWS API
* Documentation
*/
java.util.concurrent.Future disassociateApiAsync(DisassociateApiRequest disassociateApiRequest);
/**
*
* Removes an ApiAssociation
object from a custom domain.
*
*
* @param disassociateApiRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateApi operation returned by the service.
* @sample AWSAppSyncAsyncHandler.DisassociateApi
* @see AWS API
* Documentation
*/
java.util.concurrent.Future disassociateApiAsync(DisassociateApiRequest disassociateApiRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an association between a Merged API and source API using the source API's identifier and the association
* ID.
*
*
* @param disassociateMergedGraphqlApiRequest
* @return A Java Future containing the result of the DisassociateMergedGraphqlApi operation returned by the
* service.
* @sample AWSAppSyncAsync.DisassociateMergedGraphqlApi
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateMergedGraphqlApiAsync(
DisassociateMergedGraphqlApiRequest disassociateMergedGraphqlApiRequest);
/**
*
* Deletes an association between a Merged API and source API using the source API's identifier and the association
* ID.
*
*
* @param disassociateMergedGraphqlApiRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateMergedGraphqlApi operation returned by the
* service.
* @sample AWSAppSyncAsyncHandler.DisassociateMergedGraphqlApi
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateMergedGraphqlApiAsync(
DisassociateMergedGraphqlApiRequest disassociateMergedGraphqlApiRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an association between a Merged API and source API using the Merged API's identifier and the association
* ID.
*
*
* @param disassociateSourceGraphqlApiRequest
* @return A Java Future containing the result of the DisassociateSourceGraphqlApi operation returned by the
* service.
* @sample AWSAppSyncAsync.DisassociateSourceGraphqlApi
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateSourceGraphqlApiAsync(
DisassociateSourceGraphqlApiRequest disassociateSourceGraphqlApiRequest);
/**
*
* Deletes an association between a Merged API and source API using the Merged API's identifier and the association
* ID.
*
*
* @param disassociateSourceGraphqlApiRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateSourceGraphqlApi operation returned by the
* service.
* @sample AWSAppSyncAsyncHandler.DisassociateSourceGraphqlApi
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateSourceGraphqlApiAsync(
DisassociateSourceGraphqlApiRequest disassociateSourceGraphqlApiRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Evaluates the given code and returns the response. The code definition requirements depend on the specified
* runtime. For APPSYNC_JS
runtimes, the code defines the request and response functions. The request
* function takes the incoming request after a GraphQL operation is parsed and converts it into a request
* configuration for the selected data source operation. The response function interprets responses from the data
* source and maps it to the shape of the GraphQL field output type.
*
*
* @param evaluateCodeRequest
* @return A Java Future containing the result of the EvaluateCode operation returned by the service.
* @sample AWSAppSyncAsync.EvaluateCode
* @see AWS API
* Documentation
*/
java.util.concurrent.Future evaluateCodeAsync(EvaluateCodeRequest evaluateCodeRequest);
/**
*
* Evaluates the given code and returns the response. The code definition requirements depend on the specified
* runtime. For APPSYNC_JS
runtimes, the code defines the request and response functions. The request
* function takes the incoming request after a GraphQL operation is parsed and converts it into a request
* configuration for the selected data source operation. The response function interprets responses from the data
* source and maps it to the shape of the GraphQL field output type.
*
*
* @param evaluateCodeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the EvaluateCode operation returned by the service.
* @sample AWSAppSyncAsyncHandler.EvaluateCode
* @see AWS API
* Documentation
*/
java.util.concurrent.Future evaluateCodeAsync(EvaluateCodeRequest evaluateCodeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Evaluates a given template and returns the response. The mapping template can be a request or response template.
*
*
* Request templates take the incoming request after a GraphQL operation is parsed and convert it into a request
* configuration for the selected data source operation. Response templates interpret responses from the data source
* and map it to the shape of the GraphQL field output type.
*
*
* Mapping templates are written in the Apache Velocity Template Language (VTL).
*
*
* @param evaluateMappingTemplateRequest
* @return A Java Future containing the result of the EvaluateMappingTemplate operation returned by the service.
* @sample AWSAppSyncAsync.EvaluateMappingTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future evaluateMappingTemplateAsync(EvaluateMappingTemplateRequest evaluateMappingTemplateRequest);
/**
*
* Evaluates a given template and returns the response. The mapping template can be a request or response template.
*
*
* Request templates take the incoming request after a GraphQL operation is parsed and convert it into a request
* configuration for the selected data source operation. Response templates interpret responses from the data source
* and map it to the shape of the GraphQL field output type.
*
*
* Mapping templates are written in the Apache Velocity Template Language (VTL).
*
*
* @param evaluateMappingTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the EvaluateMappingTemplate operation returned by the service.
* @sample AWSAppSyncAsyncHandler.EvaluateMappingTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future evaluateMappingTemplateAsync(EvaluateMappingTemplateRequest evaluateMappingTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Flushes an ApiCache
object.
*
*
* @param flushApiCacheRequest
* Represents the input of a FlushApiCache
operation.
* @return A Java Future containing the result of the FlushApiCache operation returned by the service.
* @sample AWSAppSyncAsync.FlushApiCache
* @see AWS API
* Documentation
*/
java.util.concurrent.Future flushApiCacheAsync(FlushApiCacheRequest flushApiCacheRequest);
/**
*
* Flushes an ApiCache
object.
*
*
* @param flushApiCacheRequest
* Represents the input of a FlushApiCache
operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the FlushApiCache operation returned by the service.
* @sample AWSAppSyncAsyncHandler.FlushApiCache
* @see AWS API
* Documentation
*/
java.util.concurrent.Future flushApiCacheAsync(FlushApiCacheRequest flushApiCacheRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves an ApiAssociation
object.
*
*
* @param getApiAssociationRequest
* @return A Java Future containing the result of the GetApiAssociation operation returned by the service.
* @sample AWSAppSyncAsync.GetApiAssociation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getApiAssociationAsync(GetApiAssociationRequest getApiAssociationRequest);
/**
*
* Retrieves an ApiAssociation
object.
*
*
* @param getApiAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetApiAssociation operation returned by the service.
* @sample AWSAppSyncAsyncHandler.GetApiAssociation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getApiAssociationAsync(GetApiAssociationRequest getApiAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves an ApiCache
object.
*
*
* @param getApiCacheRequest
* Represents the input of a GetApiCache
operation.
* @return A Java Future containing the result of the GetApiCache operation returned by the service.
* @sample AWSAppSyncAsync.GetApiCache
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getApiCacheAsync(GetApiCacheRequest getApiCacheRequest);
/**
*
* Retrieves an ApiCache
object.
*
*
* @param getApiCacheRequest
* Represents the input of a GetApiCache
operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetApiCache operation returned by the service.
* @sample AWSAppSyncAsyncHandler.GetApiCache
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getApiCacheAsync(GetApiCacheRequest getApiCacheRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a DataSource
object.
*
*
* @param getDataSourceRequest
* @return A Java Future containing the result of the GetDataSource operation returned by the service.
* @sample AWSAppSyncAsync.GetDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDataSourceAsync(GetDataSourceRequest getDataSourceRequest);
/**
*
* Retrieves a DataSource
object.
*
*
* @param getDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDataSource operation returned by the service.
* @sample AWSAppSyncAsyncHandler.GetDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDataSourceAsync(GetDataSourceRequest getDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the record of an existing introspection. If the retrieval is successful, the result of the
* instrospection will also be returned. If the retrieval fails the operation, an error message will be returned
* instead.
*
*
* @param getDataSourceIntrospectionRequest
* @return A Java Future containing the result of the GetDataSourceIntrospection operation returned by the service.
* @sample AWSAppSyncAsync.GetDataSourceIntrospection
* @see AWS API Documentation
*/
java.util.concurrent.Future getDataSourceIntrospectionAsync(
GetDataSourceIntrospectionRequest getDataSourceIntrospectionRequest);
/**
*
* Retrieves the record of an existing introspection. If the retrieval is successful, the result of the
* instrospection will also be returned. If the retrieval fails the operation, an error message will be returned
* instead.
*
*
* @param getDataSourceIntrospectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDataSourceIntrospection operation returned by the service.
* @sample AWSAppSyncAsyncHandler.GetDataSourceIntrospection
* @see AWS API Documentation
*/
java.util.concurrent.Future getDataSourceIntrospectionAsync(
GetDataSourceIntrospectionRequest getDataSourceIntrospectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a custom DomainName
object.
*
*
* @param getDomainNameRequest
* @return A Java Future containing the result of the GetDomainName operation returned by the service.
* @sample AWSAppSyncAsync.GetDomainName
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDomainNameAsync(GetDomainNameRequest getDomainNameRequest);
/**
*
* Retrieves a custom DomainName
object.
*
*
* @param getDomainNameRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDomainName operation returned by the service.
* @sample AWSAppSyncAsyncHandler.GetDomainName
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDomainNameAsync(GetDomainNameRequest getDomainNameRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get a Function
.
*
*
* @param getFunctionRequest
* @return A Java Future containing the result of the GetFunction operation returned by the service.
* @sample AWSAppSyncAsync.GetFunction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFunctionAsync(GetFunctionRequest getFunctionRequest);
/**
*
* Get a Function
.
*
*
* @param getFunctionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetFunction operation returned by the service.
* @sample AWSAppSyncAsyncHandler.GetFunction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFunctionAsync(GetFunctionRequest getFunctionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a GraphqlApi
object.
*
*
* @param getGraphqlApiRequest
* @return A Java Future containing the result of the GetGraphqlApi operation returned by the service.
* @sample AWSAppSyncAsync.GetGraphqlApi
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getGraphqlApiAsync(GetGraphqlApiRequest getGraphqlApiRequest);
/**
*
* Retrieves a GraphqlApi
object.
*
*
* @param getGraphqlApiRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetGraphqlApi operation returned by the service.
* @sample AWSAppSyncAsyncHandler.GetGraphqlApi
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getGraphqlApiAsync(GetGraphqlApiRequest getGraphqlApiRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the list of environmental variable key-value pairs associated with an API by its ID value.
*
*
* @param getGraphqlApiEnvironmentVariablesRequest
* @return A Java Future containing the result of the GetGraphqlApiEnvironmentVariables operation returned by the
* service.
* @sample AWSAppSyncAsync.GetGraphqlApiEnvironmentVariables
* @see AWS API Documentation
*/
java.util.concurrent.Future getGraphqlApiEnvironmentVariablesAsync(
GetGraphqlApiEnvironmentVariablesRequest getGraphqlApiEnvironmentVariablesRequest);
/**
*
* Retrieves the list of environmental variable key-value pairs associated with an API by its ID value.
*
*
* @param getGraphqlApiEnvironmentVariablesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetGraphqlApiEnvironmentVariables operation returned by the
* service.
* @sample AWSAppSyncAsyncHandler.GetGraphqlApiEnvironmentVariables
* @see AWS API Documentation
*/
java.util.concurrent.Future getGraphqlApiEnvironmentVariablesAsync(
GetGraphqlApiEnvironmentVariablesRequest getGraphqlApiEnvironmentVariablesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the introspection schema for a GraphQL API.
*
*
* @param getIntrospectionSchemaRequest
* @return A Java Future containing the result of the GetIntrospectionSchema operation returned by the service.
* @sample AWSAppSyncAsync.GetIntrospectionSchema
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getIntrospectionSchemaAsync(GetIntrospectionSchemaRequest getIntrospectionSchemaRequest);
/**
*
* Retrieves the introspection schema for a GraphQL API.
*
*
* @param getIntrospectionSchemaRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetIntrospectionSchema operation returned by the service.
* @sample AWSAppSyncAsyncHandler.GetIntrospectionSchema
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getIntrospectionSchemaAsync(GetIntrospectionSchemaRequest getIntrospectionSchemaRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a Resolver
object.
*
*
* @param getResolverRequest
* @return A Java Future containing the result of the GetResolver operation returned by the service.
* @sample AWSAppSyncAsync.GetResolver
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getResolverAsync(GetResolverRequest getResolverRequest);
/**
*
* Retrieves a Resolver
object.
*
*
* @param getResolverRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetResolver operation returned by the service.
* @sample AWSAppSyncAsyncHandler.GetResolver
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getResolverAsync(GetResolverRequest getResolverRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the current status of a schema creation operation.
*
*
* @param getSchemaCreationStatusRequest
* @return A Java Future containing the result of the GetSchemaCreationStatus operation returned by the service.
* @sample AWSAppSyncAsync.GetSchemaCreationStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future getSchemaCreationStatusAsync(GetSchemaCreationStatusRequest getSchemaCreationStatusRequest);
/**
*
* Retrieves the current status of a schema creation operation.
*
*
* @param getSchemaCreationStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSchemaCreationStatus operation returned by the service.
* @sample AWSAppSyncAsyncHandler.GetSchemaCreationStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future getSchemaCreationStatusAsync(GetSchemaCreationStatusRequest getSchemaCreationStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a SourceApiAssociation
object.
*
*
* @param getSourceApiAssociationRequest
* @return A Java Future containing the result of the GetSourceApiAssociation operation returned by the service.
* @sample AWSAppSyncAsync.GetSourceApiAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future getSourceApiAssociationAsync(GetSourceApiAssociationRequest getSourceApiAssociationRequest);
/**
*
* Retrieves a SourceApiAssociation
object.
*
*
* @param getSourceApiAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSourceApiAssociation operation returned by the service.
* @sample AWSAppSyncAsyncHandler.GetSourceApiAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future getSourceApiAssociationAsync(GetSourceApiAssociationRequest getSourceApiAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a Type
object.
*
*
* @param getTypeRequest
* @return A Java Future containing the result of the GetType operation returned by the service.
* @sample AWSAppSyncAsync.GetType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTypeAsync(GetTypeRequest getTypeRequest);
/**
*
* Retrieves a Type
object.
*
*
* @param getTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetType operation returned by the service.
* @sample AWSAppSyncAsyncHandler.GetType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTypeAsync(GetTypeRequest getTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the API keys for a given API.
*
*
*
* API keys are deleted automatically 60 days after they expire. However, they may still be included in the response
* until they have actually been deleted. You can safely call DeleteApiKey
to manually delete a key
* before it's automatically deleted.
*
*
*
* @param listApiKeysRequest
* @return A Java Future containing the result of the ListApiKeys operation returned by the service.
* @sample AWSAppSyncAsync.ListApiKeys
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listApiKeysAsync(ListApiKeysRequest listApiKeysRequest);
/**
*
* Lists the API keys for a given API.
*
*
*
* API keys are deleted automatically 60 days after they expire. However, they may still be included in the response
* until they have actually been deleted. You can safely call DeleteApiKey
to manually delete a key
* before it's automatically deleted.
*
*
*
* @param listApiKeysRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListApiKeys operation returned by the service.
* @sample AWSAppSyncAsyncHandler.ListApiKeys
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listApiKeysAsync(ListApiKeysRequest listApiKeysRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the data sources for a given API.
*
*
* @param listDataSourcesRequest
* @return A Java Future containing the result of the ListDataSources operation returned by the service.
* @sample AWSAppSyncAsync.ListDataSources
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDataSourcesAsync(ListDataSourcesRequest listDataSourcesRequest);
/**
*
* Lists the data sources for a given API.
*
*
* @param listDataSourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDataSources operation returned by the service.
* @sample AWSAppSyncAsyncHandler.ListDataSources
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDataSourcesAsync(ListDataSourcesRequest listDataSourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists multiple custom domain names.
*
*
* @param listDomainNamesRequest
* @return A Java Future containing the result of the ListDomainNames operation returned by the service.
* @sample AWSAppSyncAsync.ListDomainNames
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDomainNamesAsync(ListDomainNamesRequest listDomainNamesRequest);
/**
*
* Lists multiple custom domain names.
*
*
* @param listDomainNamesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDomainNames operation returned by the service.
* @sample AWSAppSyncAsyncHandler.ListDomainNames
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDomainNamesAsync(ListDomainNamesRequest listDomainNamesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List multiple functions.
*
*
* @param listFunctionsRequest
* @return A Java Future containing the result of the ListFunctions operation returned by the service.
* @sample AWSAppSyncAsync.ListFunctions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFunctionsAsync(ListFunctionsRequest listFunctionsRequest);
/**
*
* List multiple functions.
*
*
* @param listFunctionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFunctions operation returned by the service.
* @sample AWSAppSyncAsyncHandler.ListFunctions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFunctionsAsync(ListFunctionsRequest listFunctionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists your GraphQL APIs.
*
*
* @param listGraphqlApisRequest
* @return A Java Future containing the result of the ListGraphqlApis operation returned by the service.
* @sample AWSAppSyncAsync.ListGraphqlApis
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listGraphqlApisAsync(ListGraphqlApisRequest listGraphqlApisRequest);
/**
*
* Lists your GraphQL APIs.
*
*
* @param listGraphqlApisRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListGraphqlApis operation returned by the service.
* @sample AWSAppSyncAsyncHandler.ListGraphqlApis
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listGraphqlApisAsync(ListGraphqlApisRequest listGraphqlApisRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the resolvers for a given API and type.
*
*
* @param listResolversRequest
* @return A Java Future containing the result of the ListResolvers operation returned by the service.
* @sample AWSAppSyncAsync.ListResolvers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listResolversAsync(ListResolversRequest listResolversRequest);
/**
*
* Lists the resolvers for a given API and type.
*
*
* @param listResolversRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListResolvers operation returned by the service.
* @sample AWSAppSyncAsyncHandler.ListResolvers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listResolversAsync(ListResolversRequest listResolversRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List the resolvers that are associated with a specific function.
*
*
* @param listResolversByFunctionRequest
* @return A Java Future containing the result of the ListResolversByFunction operation returned by the service.
* @sample AWSAppSyncAsync.ListResolversByFunction
* @see AWS API Documentation
*/
java.util.concurrent.Future listResolversByFunctionAsync(ListResolversByFunctionRequest listResolversByFunctionRequest);
/**
*
* List the resolvers that are associated with a specific function.
*
*
* @param listResolversByFunctionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListResolversByFunction operation returned by the service.
* @sample AWSAppSyncAsyncHandler.ListResolversByFunction
* @see AWS API Documentation
*/
java.util.concurrent.Future listResolversByFunctionAsync(ListResolversByFunctionRequest listResolversByFunctionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the SourceApiAssociationSummary
data.
*
*
* @param listSourceApiAssociationsRequest
* @return A Java Future containing the result of the ListSourceApiAssociations operation returned by the service.
* @sample AWSAppSyncAsync.ListSourceApiAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future listSourceApiAssociationsAsync(
ListSourceApiAssociationsRequest listSourceApiAssociationsRequest);
/**
*
* Lists the SourceApiAssociationSummary
data.
*
*
* @param listSourceApiAssociationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSourceApiAssociations operation returned by the service.
* @sample AWSAppSyncAsyncHandler.ListSourceApiAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future listSourceApiAssociationsAsync(
ListSourceApiAssociationsRequest listSourceApiAssociationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the tags for a resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSAppSyncAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists the tags for a resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSAppSyncAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the types for a given API.
*
*
* @param listTypesRequest
* @return A Java Future containing the result of the ListTypes operation returned by the service.
* @sample AWSAppSyncAsync.ListTypes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTypesAsync(ListTypesRequest listTypesRequest);
/**
*
* Lists the types for a given API.
*
*
* @param listTypesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTypes operation returned by the service.
* @sample AWSAppSyncAsyncHandler.ListTypes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTypesAsync(ListTypesRequest listTypesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists Type
objects by the source API association ID.
*
*
* @param listTypesByAssociationRequest
* @return A Java Future containing the result of the ListTypesByAssociation operation returned by the service.
* @sample AWSAppSyncAsync.ListTypesByAssociation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTypesByAssociationAsync(ListTypesByAssociationRequest listTypesByAssociationRequest);
/**
*
* Lists Type
objects by the source API association ID.
*
*
* @param listTypesByAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTypesByAssociation operation returned by the service.
* @sample AWSAppSyncAsyncHandler.ListTypesByAssociation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTypesByAssociationAsync(ListTypesByAssociationRequest listTypesByAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a list of environmental variables in an API by its ID value.
*
*
* When creating an environmental variable, it must follow the constraints below:
*
*
* -
*
* Both JavaScript and VTL templates support environmental variables.
*
*
* -
*
* Environmental variables are not evaluated before function invocation.
*
*
* -
*
* Environmental variables only support string values.
*
*
* -
*
* Any defined value in an environmental variable is considered a string literal and not expanded.
*
*
* -
*
* Variable evaluations should ideally be performed in the function code.
*
*
*
*
* When creating an environmental variable key-value pair, it must follow the additional constraints below:
*
*
* -
*
* Keys must begin with a letter.
*
*
* -
*
* Keys must be at least two characters long.
*
*
* -
*
* Keys can only contain letters, numbers, and the underscore character (_).
*
*
* -
*
* Values can be up to 512 characters long.
*
*
* -
*
* You can configure up to 50 key-value pairs in a GraphQL API.
*
*
*
*
* You can create a list of environmental variables by adding it to the environmentVariables
payload as
* a list in the format {"key1":"value1","key2":"value2", …}
. Note that each call of the
* PutGraphqlApiEnvironmentVariables
action will result in the overwriting of the existing
* environmental variable list of that API. This means the existing environmental variables will be lost. To avoid
* this, you must include all existing and new environmental variables in the list each time you call this action.
*
*
* @param putGraphqlApiEnvironmentVariablesRequest
* @return A Java Future containing the result of the PutGraphqlApiEnvironmentVariables operation returned by the
* service.
* @sample AWSAppSyncAsync.PutGraphqlApiEnvironmentVariables
* @see AWS API Documentation
*/
java.util.concurrent.Future putGraphqlApiEnvironmentVariablesAsync(
PutGraphqlApiEnvironmentVariablesRequest putGraphqlApiEnvironmentVariablesRequest);
/**
*
* Creates a list of environmental variables in an API by its ID value.
*
*
* When creating an environmental variable, it must follow the constraints below:
*
*
* -
*
* Both JavaScript and VTL templates support environmental variables.
*
*
* -
*
* Environmental variables are not evaluated before function invocation.
*
*
* -
*
* Environmental variables only support string values.
*
*
* -
*
* Any defined value in an environmental variable is considered a string literal and not expanded.
*
*
* -
*
* Variable evaluations should ideally be performed in the function code.
*
*
*
*
* When creating an environmental variable key-value pair, it must follow the additional constraints below:
*
*
* -
*
* Keys must begin with a letter.
*
*
* -
*
* Keys must be at least two characters long.
*
*
* -
*
* Keys can only contain letters, numbers, and the underscore character (_).
*
*
* -
*
* Values can be up to 512 characters long.
*
*
* -
*
* You can configure up to 50 key-value pairs in a GraphQL API.
*
*
*
*
* You can create a list of environmental variables by adding it to the environmentVariables
payload as
* a list in the format {"key1":"value1","key2":"value2", …}
. Note that each call of the
* PutGraphqlApiEnvironmentVariables
action will result in the overwriting of the existing
* environmental variable list of that API. This means the existing environmental variables will be lost. To avoid
* this, you must include all existing and new environmental variables in the list each time you call this action.
*
*
* @param putGraphqlApiEnvironmentVariablesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutGraphqlApiEnvironmentVariables operation returned by the
* service.
* @sample AWSAppSyncAsyncHandler.PutGraphqlApiEnvironmentVariables
* @see AWS API Documentation
*/
java.util.concurrent.Future putGraphqlApiEnvironmentVariablesAsync(
PutGraphqlApiEnvironmentVariablesRequest putGraphqlApiEnvironmentVariablesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new introspection. Returns the introspectionId
of the new introspection after its
* creation.
*
*
* @param startDataSourceIntrospectionRequest
* @return A Java Future containing the result of the StartDataSourceIntrospection operation returned by the
* service.
* @sample AWSAppSyncAsync.StartDataSourceIntrospection
* @see AWS API Documentation
*/
java.util.concurrent.Future startDataSourceIntrospectionAsync(
StartDataSourceIntrospectionRequest startDataSourceIntrospectionRequest);
/**
*
* Creates a new introspection. Returns the introspectionId
of the new introspection after its
* creation.
*
*
* @param startDataSourceIntrospectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartDataSourceIntrospection operation returned by the
* service.
* @sample AWSAppSyncAsyncHandler.StartDataSourceIntrospection
* @see AWS API Documentation
*/
java.util.concurrent.Future startDataSourceIntrospectionAsync(
StartDataSourceIntrospectionRequest startDataSourceIntrospectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds a new schema to your GraphQL API.
*
*
* This operation is asynchronous. Use to determine when it has completed.
*
*
* @param startSchemaCreationRequest
* @return A Java Future containing the result of the StartSchemaCreation operation returned by the service.
* @sample AWSAppSyncAsync.StartSchemaCreation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future startSchemaCreationAsync(StartSchemaCreationRequest startSchemaCreationRequest);
/**
*
* Adds a new schema to your GraphQL API.
*
*
* This operation is asynchronous. Use to determine when it has completed.
*
*
* @param startSchemaCreationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartSchemaCreation operation returned by the service.
* @sample AWSAppSyncAsyncHandler.StartSchemaCreation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future startSchemaCreationAsync(StartSchemaCreationRequest startSchemaCreationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Initiates a merge operation. Returns a status that shows the result of the merge operation.
*
*
* @param startSchemaMergeRequest
* @return A Java Future containing the result of the StartSchemaMerge operation returned by the service.
* @sample AWSAppSyncAsync.StartSchemaMerge
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startSchemaMergeAsync(StartSchemaMergeRequest startSchemaMergeRequest);
/**
*
* Initiates a merge operation. Returns a status that shows the result of the merge operation.
*
*
* @param startSchemaMergeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartSchemaMerge operation returned by the service.
* @sample AWSAppSyncAsyncHandler.StartSchemaMerge
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startSchemaMergeAsync(StartSchemaMergeRequest startSchemaMergeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Tags a resource with user-supplied tags.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSAppSyncAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Tags a resource with user-supplied tags.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSAppSyncAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Untags a resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSAppSyncAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Untags a resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSAppSyncAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the cache for the GraphQL API.
*
*
* @param updateApiCacheRequest
* Represents the input of a UpdateApiCache
operation.
* @return A Java Future containing the result of the UpdateApiCache operation returned by the service.
* @sample AWSAppSyncAsync.UpdateApiCache
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateApiCacheAsync(UpdateApiCacheRequest updateApiCacheRequest);
/**
*
* Updates the cache for the GraphQL API.
*
*
* @param updateApiCacheRequest
* Represents the input of a UpdateApiCache
operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateApiCache operation returned by the service.
* @sample AWSAppSyncAsyncHandler.UpdateApiCache
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateApiCacheAsync(UpdateApiCacheRequest updateApiCacheRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an API key. You can update the key as long as it's not deleted.
*
*
* @param updateApiKeyRequest
* @return A Java Future containing the result of the UpdateApiKey operation returned by the service.
* @sample AWSAppSyncAsync.UpdateApiKey
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateApiKeyAsync(UpdateApiKeyRequest updateApiKeyRequest);
/**
*
* Updates an API key. You can update the key as long as it's not deleted.
*
*
* @param updateApiKeyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateApiKey operation returned by the service.
* @sample AWSAppSyncAsyncHandler.UpdateApiKey
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateApiKeyAsync(UpdateApiKeyRequest updateApiKeyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a DataSource
object.
*
*
* @param updateDataSourceRequest
* @return A Java Future containing the result of the UpdateDataSource operation returned by the service.
* @sample AWSAppSyncAsync.UpdateDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateDataSourceAsync(UpdateDataSourceRequest updateDataSourceRequest);
/**
*
* Updates a DataSource
object.
*
*
* @param updateDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDataSource operation returned by the service.
* @sample AWSAppSyncAsyncHandler.UpdateDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateDataSourceAsync(UpdateDataSourceRequest updateDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a custom DomainName
object.
*
*
* @param updateDomainNameRequest
* @return A Java Future containing the result of the UpdateDomainName operation returned by the service.
* @sample AWSAppSyncAsync.UpdateDomainName
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateDomainNameAsync(UpdateDomainNameRequest updateDomainNameRequest);
/**
*
* Updates a custom DomainName
object.
*
*
* @param updateDomainNameRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDomainName operation returned by the service.
* @sample AWSAppSyncAsyncHandler.UpdateDomainName
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateDomainNameAsync(UpdateDomainNameRequest updateDomainNameRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a Function
object.
*
*
* @param updateFunctionRequest
* @return A Java Future containing the result of the UpdateFunction operation returned by the service.
* @sample AWSAppSyncAsync.UpdateFunction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateFunctionAsync(UpdateFunctionRequest updateFunctionRequest);
/**
*
* Updates a Function
object.
*
*
* @param updateFunctionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateFunction operation returned by the service.
* @sample AWSAppSyncAsyncHandler.UpdateFunction
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateFunctionAsync(UpdateFunctionRequest updateFunctionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a GraphqlApi
object.
*
*
* @param updateGraphqlApiRequest
* @return A Java Future containing the result of the UpdateGraphqlApi operation returned by the service.
* @sample AWSAppSyncAsync.UpdateGraphqlApi
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateGraphqlApiAsync(UpdateGraphqlApiRequest updateGraphqlApiRequest);
/**
*
* Updates a GraphqlApi
object.
*
*
* @param updateGraphqlApiRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateGraphqlApi operation returned by the service.
* @sample AWSAppSyncAsyncHandler.UpdateGraphqlApi
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateGraphqlApiAsync(UpdateGraphqlApiRequest updateGraphqlApiRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a Resolver
object.
*
*
* @param updateResolverRequest
* @return A Java Future containing the result of the UpdateResolver operation returned by the service.
* @sample AWSAppSyncAsync.UpdateResolver
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateResolverAsync(UpdateResolverRequest updateResolverRequest);
/**
*
* Updates a Resolver
object.
*
*
* @param updateResolverRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateResolver operation returned by the service.
* @sample AWSAppSyncAsyncHandler.UpdateResolver
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateResolverAsync(UpdateResolverRequest updateResolverRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates some of the configuration choices of a particular source API association.
*
*
* @param updateSourceApiAssociationRequest
* @return A Java Future containing the result of the UpdateSourceApiAssociation operation returned by the service.
* @sample AWSAppSyncAsync.UpdateSourceApiAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSourceApiAssociationAsync(
UpdateSourceApiAssociationRequest updateSourceApiAssociationRequest);
/**
*
* Updates some of the configuration choices of a particular source API association.
*
*
* @param updateSourceApiAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSourceApiAssociation operation returned by the service.
* @sample AWSAppSyncAsyncHandler.UpdateSourceApiAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSourceApiAssociationAsync(
UpdateSourceApiAssociationRequest updateSourceApiAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a Type
object.
*
*
* @param updateTypeRequest
* @return A Java Future containing the result of the UpdateType operation returned by the service.
* @sample AWSAppSyncAsync.UpdateType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateTypeAsync(UpdateTypeRequest updateTypeRequest);
/**
*
* Updates a Type
object.
*
*
* @param updateTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateType operation returned by the service.
* @sample AWSAppSyncAsyncHandler.UpdateType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateTypeAsync(UpdateTypeRequest updateTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}