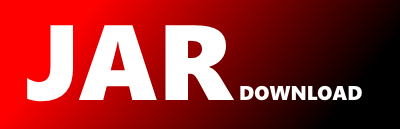
com.amazonaws.services.appsync.AWSAppSyncClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appsync Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appsync;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.appsync.AWSAppSyncClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.appsync.model.*;
import com.amazonaws.services.appsync.model.transform.*;
/**
* Client for accessing AWSAppSync. All service calls made using this client are blocking, and will not return until the
* service call completes.
*
*
* AppSync provides API actions for creating and interacting with data sources using GraphQL from your application.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSAppSyncClient extends AmazonWebServiceClient implements AWSAppSync {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSAppSync.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "appsync";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConcurrentModificationException").withExceptionUnmarshaller(
com.amazonaws.services.appsync.model.transform.ConcurrentModificationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalFailureException").withExceptionUnmarshaller(
com.amazonaws.services.appsync.model.transform.InternalFailureExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("GraphQLSchemaException").withExceptionUnmarshaller(
com.amazonaws.services.appsync.model.transform.GraphQLSchemaExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ApiKeyLimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.appsync.model.transform.ApiKeyLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("LimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.appsync.model.transform.LimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ApiLimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.appsync.model.transform.ApiLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("NotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.appsync.model.transform.NotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnauthorizedException").withExceptionUnmarshaller(
com.amazonaws.services.appsync.model.transform.UnauthorizedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.appsync.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ApiKeyValidityOutOfBoundsException").withExceptionUnmarshaller(
com.amazonaws.services.appsync.model.transform.ApiKeyValidityOutOfBoundsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("BadRequestException").withExceptionUnmarshaller(
com.amazonaws.services.appsync.model.transform.BadRequestExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.appsync.model.AWSAppSyncException.class));
public static AWSAppSyncClientBuilder builder() {
return AWSAppSyncClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on AWSAppSync using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSAppSyncClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on AWSAppSync using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSAppSyncClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("appsync.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/appsync/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/appsync/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Maps an endpoint to your custom domain.
*
*
* @param associateApiRequest
* @return Result of the AssociateApi operation returned by the service.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @sample AWSAppSync.AssociateApi
* @see AWS API
* Documentation
*/
@Override
public AssociateApiResult associateApi(AssociateApiRequest request) {
request = beforeClientExecution(request);
return executeAssociateApi(request);
}
@SdkInternalApi
final AssociateApiResult executeAssociateApi(AssociateApiRequest associateApiRequest) {
ExecutionContext executionContext = createExecutionContext(associateApiRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateApiRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(associateApiRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateApi");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new AssociateApiResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an association between a Merged API and source API using the source API's identifier.
*
*
* @param associateMergedGraphqlApiRequest
* @return Result of the AssociateMergedGraphqlApi operation returned by the service.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @sample AWSAppSync.AssociateMergedGraphqlApi
* @see AWS API Documentation
*/
@Override
public AssociateMergedGraphqlApiResult associateMergedGraphqlApi(AssociateMergedGraphqlApiRequest request) {
request = beforeClientExecution(request);
return executeAssociateMergedGraphqlApi(request);
}
@SdkInternalApi
final AssociateMergedGraphqlApiResult executeAssociateMergedGraphqlApi(AssociateMergedGraphqlApiRequest associateMergedGraphqlApiRequest) {
ExecutionContext executionContext = createExecutionContext(associateMergedGraphqlApiRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateMergedGraphqlApiRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associateMergedGraphqlApiRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateMergedGraphqlApi");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateMergedGraphqlApiResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an association between a Merged API and source API using the Merged API's identifier.
*
*
* @param associateSourceGraphqlApiRequest
* @return Result of the AssociateSourceGraphqlApi operation returned by the service.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @sample AWSAppSync.AssociateSourceGraphqlApi
* @see AWS API Documentation
*/
@Override
public AssociateSourceGraphqlApiResult associateSourceGraphqlApi(AssociateSourceGraphqlApiRequest request) {
request = beforeClientExecution(request);
return executeAssociateSourceGraphqlApi(request);
}
@SdkInternalApi
final AssociateSourceGraphqlApiResult executeAssociateSourceGraphqlApi(AssociateSourceGraphqlApiRequest associateSourceGraphqlApiRequest) {
ExecutionContext executionContext = createExecutionContext(associateSourceGraphqlApiRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateSourceGraphqlApiRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associateSourceGraphqlApiRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateSourceGraphqlApi");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateSourceGraphqlApiResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a cache for the GraphQL API.
*
*
* @param createApiCacheRequest
* Represents the input of a CreateApiCache
operation.
* @return Result of the CreateApiCache operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.CreateApiCache
* @see AWS API
* Documentation
*/
@Override
public CreateApiCacheResult createApiCache(CreateApiCacheRequest request) {
request = beforeClientExecution(request);
return executeCreateApiCache(request);
}
@SdkInternalApi
final CreateApiCacheResult executeCreateApiCache(CreateApiCacheRequest createApiCacheRequest) {
ExecutionContext executionContext = createExecutionContext(createApiCacheRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateApiCacheRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createApiCacheRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateApiCache");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateApiCacheResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a unique key that you can distribute to clients who invoke your API.
*
*
* @param createApiKeyRequest
* @return Result of the CreateApiKey operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws ApiKeyLimitExceededException
* The API key exceeded a limit. Try your request again.
* @throws ApiKeyValidityOutOfBoundsException
* The API key expiration must be set to a value between 1 and 365 days from creation (for
* CreateApiKey
) or from update (for UpdateApiKey
).
* @sample AWSAppSync.CreateApiKey
* @see AWS API
* Documentation
*/
@Override
public CreateApiKeyResult createApiKey(CreateApiKeyRequest request) {
request = beforeClientExecution(request);
return executeCreateApiKey(request);
}
@SdkInternalApi
final CreateApiKeyResult executeCreateApiKey(CreateApiKeyRequest createApiKeyRequest) {
ExecutionContext executionContext = createExecutionContext(createApiKeyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateApiKeyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createApiKeyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateApiKey");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateApiKeyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a DataSource
object.
*
*
* @param createDataSourceRequest
* @return Result of the CreateDataSource operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.CreateDataSource
* @see AWS API
* Documentation
*/
@Override
public CreateDataSourceResult createDataSource(CreateDataSourceRequest request) {
request = beforeClientExecution(request);
return executeCreateDataSource(request);
}
@SdkInternalApi
final CreateDataSourceResult executeCreateDataSource(CreateDataSourceRequest createDataSourceRequest) {
ExecutionContext executionContext = createExecutionContext(createDataSourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDataSourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createDataSourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDataSource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateDataSourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a custom DomainName
object.
*
*
* @param createDomainNameRequest
* @return Result of the CreateDomainName operation returned by the service.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.CreateDomainName
* @see AWS API
* Documentation
*/
@Override
public CreateDomainNameResult createDomainName(CreateDomainNameRequest request) {
request = beforeClientExecution(request);
return executeCreateDomainName(request);
}
@SdkInternalApi
final CreateDomainNameResult executeCreateDomainName(CreateDomainNameRequest createDomainNameRequest) {
ExecutionContext executionContext = createExecutionContext(createDomainNameRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDomainNameRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createDomainNameRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDomainName");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateDomainNameResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a Function
object.
*
*
* A function is a reusable entity. You can use multiple functions to compose the resolver logic.
*
*
* @param createFunctionRequest
* @return Result of the CreateFunction operation returned by the service.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @sample AWSAppSync.CreateFunction
* @see AWS API
* Documentation
*/
@Override
public CreateFunctionResult createFunction(CreateFunctionRequest request) {
request = beforeClientExecution(request);
return executeCreateFunction(request);
}
@SdkInternalApi
final CreateFunctionResult executeCreateFunction(CreateFunctionRequest createFunctionRequest) {
ExecutionContext executionContext = createExecutionContext(createFunctionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateFunctionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createFunctionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateFunction");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateFunctionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a GraphqlApi
object.
*
*
* @param createGraphqlApiRequest
* @return Result of the CreateGraphqlApi operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws ApiLimitExceededException
* The GraphQL API exceeded a limit. Try your request again.
* @sample AWSAppSync.CreateGraphqlApi
* @see AWS API
* Documentation
*/
@Override
public CreateGraphqlApiResult createGraphqlApi(CreateGraphqlApiRequest request) {
request = beforeClientExecution(request);
return executeCreateGraphqlApi(request);
}
@SdkInternalApi
final CreateGraphqlApiResult executeCreateGraphqlApi(CreateGraphqlApiRequest createGraphqlApiRequest) {
ExecutionContext executionContext = createExecutionContext(createGraphqlApiRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateGraphqlApiRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createGraphqlApiRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateGraphqlApi");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateGraphqlApiResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a Resolver
object.
*
*
* A resolver converts incoming requests into a format that a data source can understand, and converts the data
* source's responses into GraphQL.
*
*
* @param createResolverRequest
* @return Result of the CreateResolver operation returned by the service.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @sample AWSAppSync.CreateResolver
* @see AWS API
* Documentation
*/
@Override
public CreateResolverResult createResolver(CreateResolverRequest request) {
request = beforeClientExecution(request);
return executeCreateResolver(request);
}
@SdkInternalApi
final CreateResolverResult executeCreateResolver(CreateResolverRequest createResolverRequest) {
ExecutionContext executionContext = createExecutionContext(createResolverRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateResolverRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createResolverRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateResolver");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateResolverResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a Type
object.
*
*
* @param createTypeRequest
* @return Result of the CreateType operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.CreateType
* @see AWS API
* Documentation
*/
@Override
public CreateTypeResult createType(CreateTypeRequest request) {
request = beforeClientExecution(request);
return executeCreateType(request);
}
@SdkInternalApi
final CreateTypeResult executeCreateType(CreateTypeRequest createTypeRequest) {
ExecutionContext executionContext = createExecutionContext(createTypeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateTypeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createTypeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateType");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateTypeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an ApiCache
object.
*
*
* @param deleteApiCacheRequest
* Represents the input of a DeleteApiCache
operation.
* @return Result of the DeleteApiCache operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.DeleteApiCache
* @see AWS API
* Documentation
*/
@Override
public DeleteApiCacheResult deleteApiCache(DeleteApiCacheRequest request) {
request = beforeClientExecution(request);
return executeDeleteApiCache(request);
}
@SdkInternalApi
final DeleteApiCacheResult executeDeleteApiCache(DeleteApiCacheRequest deleteApiCacheRequest) {
ExecutionContext executionContext = createExecutionContext(deleteApiCacheRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteApiCacheRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteApiCacheRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteApiCache");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteApiCacheResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an API key.
*
*
* @param deleteApiKeyRequest
* @return Result of the DeleteApiKey operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.DeleteApiKey
* @see AWS API
* Documentation
*/
@Override
public DeleteApiKeyResult deleteApiKey(DeleteApiKeyRequest request) {
request = beforeClientExecution(request);
return executeDeleteApiKey(request);
}
@SdkInternalApi
final DeleteApiKeyResult executeDeleteApiKey(DeleteApiKeyRequest deleteApiKeyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteApiKeyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteApiKeyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteApiKeyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteApiKey");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteApiKeyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a DataSource
object.
*
*
* @param deleteDataSourceRequest
* @return Result of the DeleteDataSource operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.DeleteDataSource
* @see AWS API
* Documentation
*/
@Override
public DeleteDataSourceResult deleteDataSource(DeleteDataSourceRequest request) {
request = beforeClientExecution(request);
return executeDeleteDataSource(request);
}
@SdkInternalApi
final DeleteDataSourceResult executeDeleteDataSource(DeleteDataSourceRequest deleteDataSourceRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDataSourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDataSourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteDataSourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDataSource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteDataSourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a custom DomainName
object.
*
*
* @param deleteDomainNameRequest
* @return Result of the DeleteDomainName operation returned by the service.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @sample AWSAppSync.DeleteDomainName
* @see AWS API
* Documentation
*/
@Override
public DeleteDomainNameResult deleteDomainName(DeleteDomainNameRequest request) {
request = beforeClientExecution(request);
return executeDeleteDomainName(request);
}
@SdkInternalApi
final DeleteDomainNameResult executeDeleteDomainName(DeleteDomainNameRequest deleteDomainNameRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDomainNameRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDomainNameRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteDomainNameRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDomainName");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteDomainNameResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a Function
.
*
*
* @param deleteFunctionRequest
* @return Result of the DeleteFunction operation returned by the service.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @sample AWSAppSync.DeleteFunction
* @see AWS API
* Documentation
*/
@Override
public DeleteFunctionResult deleteFunction(DeleteFunctionRequest request) {
request = beforeClientExecution(request);
return executeDeleteFunction(request);
}
@SdkInternalApi
final DeleteFunctionResult executeDeleteFunction(DeleteFunctionRequest deleteFunctionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteFunctionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteFunctionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteFunctionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteFunction");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteFunctionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a GraphqlApi
object.
*
*
* @param deleteGraphqlApiRequest
* @return Result of the DeleteGraphqlApi operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @sample AWSAppSync.DeleteGraphqlApi
* @see AWS API
* Documentation
*/
@Override
public DeleteGraphqlApiResult deleteGraphqlApi(DeleteGraphqlApiRequest request) {
request = beforeClientExecution(request);
return executeDeleteGraphqlApi(request);
}
@SdkInternalApi
final DeleteGraphqlApiResult executeDeleteGraphqlApi(DeleteGraphqlApiRequest deleteGraphqlApiRequest) {
ExecutionContext executionContext = createExecutionContext(deleteGraphqlApiRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteGraphqlApiRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteGraphqlApiRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteGraphqlApi");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteGraphqlApiResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a Resolver
object.
*
*
* @param deleteResolverRequest
* @return Result of the DeleteResolver operation returned by the service.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @sample AWSAppSync.DeleteResolver
* @see AWS API
* Documentation
*/
@Override
public DeleteResolverResult deleteResolver(DeleteResolverRequest request) {
request = beforeClientExecution(request);
return executeDeleteResolver(request);
}
@SdkInternalApi
final DeleteResolverResult executeDeleteResolver(DeleteResolverRequest deleteResolverRequest) {
ExecutionContext executionContext = createExecutionContext(deleteResolverRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteResolverRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteResolverRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteResolver");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteResolverResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a Type
object.
*
*
* @param deleteTypeRequest
* @return Result of the DeleteType operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.DeleteType
* @see AWS API
* Documentation
*/
@Override
public DeleteTypeResult deleteType(DeleteTypeRequest request) {
request = beforeClientExecution(request);
return executeDeleteType(request);
}
@SdkInternalApi
final DeleteTypeResult executeDeleteType(DeleteTypeRequest deleteTypeRequest) {
ExecutionContext executionContext = createExecutionContext(deleteTypeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteTypeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteTypeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteType");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteTypeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes an ApiAssociation
object from a custom domain.
*
*
* @param disassociateApiRequest
* @return Result of the DisassociateApi operation returned by the service.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @sample AWSAppSync.DisassociateApi
* @see AWS API
* Documentation
*/
@Override
public DisassociateApiResult disassociateApi(DisassociateApiRequest request) {
request = beforeClientExecution(request);
return executeDisassociateApi(request);
}
@SdkInternalApi
final DisassociateApiResult executeDisassociateApi(DisassociateApiRequest disassociateApiRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateApiRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateApiRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(disassociateApiRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateApi");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DisassociateApiResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an association between a Merged API and source API using the source API's identifier and the association
* ID.
*
*
* @param disassociateMergedGraphqlApiRequest
* @return Result of the DisassociateMergedGraphqlApi operation returned by the service.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @sample AWSAppSync.DisassociateMergedGraphqlApi
* @see AWS API Documentation
*/
@Override
public DisassociateMergedGraphqlApiResult disassociateMergedGraphqlApi(DisassociateMergedGraphqlApiRequest request) {
request = beforeClientExecution(request);
return executeDisassociateMergedGraphqlApi(request);
}
@SdkInternalApi
final DisassociateMergedGraphqlApiResult executeDisassociateMergedGraphqlApi(DisassociateMergedGraphqlApiRequest disassociateMergedGraphqlApiRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateMergedGraphqlApiRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateMergedGraphqlApiRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateMergedGraphqlApiRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateMergedGraphqlApi");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateMergedGraphqlApiResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an association between a Merged API and source API using the Merged API's identifier and the association
* ID.
*
*
* @param disassociateSourceGraphqlApiRequest
* @return Result of the DisassociateSourceGraphqlApi operation returned by the service.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @sample AWSAppSync.DisassociateSourceGraphqlApi
* @see AWS API Documentation
*/
@Override
public DisassociateSourceGraphqlApiResult disassociateSourceGraphqlApi(DisassociateSourceGraphqlApiRequest request) {
request = beforeClientExecution(request);
return executeDisassociateSourceGraphqlApi(request);
}
@SdkInternalApi
final DisassociateSourceGraphqlApiResult executeDisassociateSourceGraphqlApi(DisassociateSourceGraphqlApiRequest disassociateSourceGraphqlApiRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateSourceGraphqlApiRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateSourceGraphqlApiRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateSourceGraphqlApiRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateSourceGraphqlApi");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateSourceGraphqlApiResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Evaluates the given code and returns the response. The code definition requirements depend on the specified
* runtime. For APPSYNC_JS
runtimes, the code defines the request and response functions. The request
* function takes the incoming request after a GraphQL operation is parsed and converts it into a request
* configuration for the selected data source operation. The response function interprets responses from the data
* source and maps it to the shape of the GraphQL field output type.
*
*
* @param evaluateCodeRequest
* @return Result of the EvaluateCode operation returned by the service.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @sample AWSAppSync.EvaluateCode
* @see AWS API
* Documentation
*/
@Override
public EvaluateCodeResult evaluateCode(EvaluateCodeRequest request) {
request = beforeClientExecution(request);
return executeEvaluateCode(request);
}
@SdkInternalApi
final EvaluateCodeResult executeEvaluateCode(EvaluateCodeRequest evaluateCodeRequest) {
ExecutionContext executionContext = createExecutionContext(evaluateCodeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new EvaluateCodeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(evaluateCodeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "EvaluateCode");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new EvaluateCodeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Evaluates a given template and returns the response. The mapping template can be a request or response template.
*
*
* Request templates take the incoming request after a GraphQL operation is parsed and convert it into a request
* configuration for the selected data source operation. Response templates interpret responses from the data source
* and map it to the shape of the GraphQL field output type.
*
*
* Mapping templates are written in the Apache Velocity Template Language (VTL).
*
*
* @param evaluateMappingTemplateRequest
* @return Result of the EvaluateMappingTemplate operation returned by the service.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @sample AWSAppSync.EvaluateMappingTemplate
* @see AWS API Documentation
*/
@Override
public EvaluateMappingTemplateResult evaluateMappingTemplate(EvaluateMappingTemplateRequest request) {
request = beforeClientExecution(request);
return executeEvaluateMappingTemplate(request);
}
@SdkInternalApi
final EvaluateMappingTemplateResult executeEvaluateMappingTemplate(EvaluateMappingTemplateRequest evaluateMappingTemplateRequest) {
ExecutionContext executionContext = createExecutionContext(evaluateMappingTemplateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new EvaluateMappingTemplateRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(evaluateMappingTemplateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "EvaluateMappingTemplate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new EvaluateMappingTemplateResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Flushes an ApiCache
object.
*
*
* @param flushApiCacheRequest
* Represents the input of a FlushApiCache
operation.
* @return Result of the FlushApiCache operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.FlushApiCache
* @see AWS API
* Documentation
*/
@Override
public FlushApiCacheResult flushApiCache(FlushApiCacheRequest request) {
request = beforeClientExecution(request);
return executeFlushApiCache(request);
}
@SdkInternalApi
final FlushApiCacheResult executeFlushApiCache(FlushApiCacheRequest flushApiCacheRequest) {
ExecutionContext executionContext = createExecutionContext(flushApiCacheRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new FlushApiCacheRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(flushApiCacheRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "FlushApiCache");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new FlushApiCacheResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves an ApiAssociation
object.
*
*
* @param getApiAssociationRequest
* @return Result of the GetApiAssociation operation returned by the service.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @sample AWSAppSync.GetApiAssociation
* @see AWS API
* Documentation
*/
@Override
public GetApiAssociationResult getApiAssociation(GetApiAssociationRequest request) {
request = beforeClientExecution(request);
return executeGetApiAssociation(request);
}
@SdkInternalApi
final GetApiAssociationResult executeGetApiAssociation(GetApiAssociationRequest getApiAssociationRequest) {
ExecutionContext executionContext = createExecutionContext(getApiAssociationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetApiAssociationRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getApiAssociationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetApiAssociation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetApiAssociationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves an ApiCache
object.
*
*
* @param getApiCacheRequest
* Represents the input of a GetApiCache
operation.
* @return Result of the GetApiCache operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.GetApiCache
* @see AWS API
* Documentation
*/
@Override
public GetApiCacheResult getApiCache(GetApiCacheRequest request) {
request = beforeClientExecution(request);
return executeGetApiCache(request);
}
@SdkInternalApi
final GetApiCacheResult executeGetApiCache(GetApiCacheRequest getApiCacheRequest) {
ExecutionContext executionContext = createExecutionContext(getApiCacheRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetApiCacheRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getApiCacheRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetApiCache");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetApiCacheResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a DataSource
object.
*
*
* @param getDataSourceRequest
* @return Result of the GetDataSource operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.GetDataSource
* @see AWS API
* Documentation
*/
@Override
public GetDataSourceResult getDataSource(GetDataSourceRequest request) {
request = beforeClientExecution(request);
return executeGetDataSource(request);
}
@SdkInternalApi
final GetDataSourceResult executeGetDataSource(GetDataSourceRequest getDataSourceRequest) {
ExecutionContext executionContext = createExecutionContext(getDataSourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDataSourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getDataSourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDataSource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetDataSourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the record of an existing introspection. If the retrieval is successful, the result of the
* instrospection will also be returned. If the retrieval fails the operation, an error message will be returned
* instead.
*
*
* @param getDataSourceIntrospectionRequest
* @return Result of the GetDataSourceIntrospection operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.GetDataSourceIntrospection
* @see AWS API Documentation
*/
@Override
public GetDataSourceIntrospectionResult getDataSourceIntrospection(GetDataSourceIntrospectionRequest request) {
request = beforeClientExecution(request);
return executeGetDataSourceIntrospection(request);
}
@SdkInternalApi
final GetDataSourceIntrospectionResult executeGetDataSourceIntrospection(GetDataSourceIntrospectionRequest getDataSourceIntrospectionRequest) {
ExecutionContext executionContext = createExecutionContext(getDataSourceIntrospectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDataSourceIntrospectionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getDataSourceIntrospectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDataSourceIntrospection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetDataSourceIntrospectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a custom DomainName
object.
*
*
* @param getDomainNameRequest
* @return Result of the GetDomainName operation returned by the service.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @sample AWSAppSync.GetDomainName
* @see AWS API
* Documentation
*/
@Override
public GetDomainNameResult getDomainName(GetDomainNameRequest request) {
request = beforeClientExecution(request);
return executeGetDomainName(request);
}
@SdkInternalApi
final GetDomainNameResult executeGetDomainName(GetDomainNameRequest getDomainNameRequest) {
ExecutionContext executionContext = createExecutionContext(getDomainNameRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDomainNameRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getDomainNameRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDomainName");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetDomainNameResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get a Function
.
*
*
* @param getFunctionRequest
* @return Result of the GetFunction operation returned by the service.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @sample AWSAppSync.GetFunction
* @see AWS API
* Documentation
*/
@Override
public GetFunctionResult getFunction(GetFunctionRequest request) {
request = beforeClientExecution(request);
return executeGetFunction(request);
}
@SdkInternalApi
final GetFunctionResult executeGetFunction(GetFunctionRequest getFunctionRequest) {
ExecutionContext executionContext = createExecutionContext(getFunctionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetFunctionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getFunctionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetFunction");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetFunctionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a GraphqlApi
object.
*
*
* @param getGraphqlApiRequest
* @return Result of the GetGraphqlApi operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @sample AWSAppSync.GetGraphqlApi
* @see AWS API
* Documentation
*/
@Override
public GetGraphqlApiResult getGraphqlApi(GetGraphqlApiRequest request) {
request = beforeClientExecution(request);
return executeGetGraphqlApi(request);
}
@SdkInternalApi
final GetGraphqlApiResult executeGetGraphqlApi(GetGraphqlApiRequest getGraphqlApiRequest) {
ExecutionContext executionContext = createExecutionContext(getGraphqlApiRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetGraphqlApiRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getGraphqlApiRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetGraphqlApi");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetGraphqlApiResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the list of environmental variable key-value pairs associated with an API by its ID value.
*
*
* @param getGraphqlApiEnvironmentVariablesRequest
* @return Result of the GetGraphqlApiEnvironmentVariables operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @sample AWSAppSync.GetGraphqlApiEnvironmentVariables
* @see AWS API Documentation
*/
@Override
public GetGraphqlApiEnvironmentVariablesResult getGraphqlApiEnvironmentVariables(GetGraphqlApiEnvironmentVariablesRequest request) {
request = beforeClientExecution(request);
return executeGetGraphqlApiEnvironmentVariables(request);
}
@SdkInternalApi
final GetGraphqlApiEnvironmentVariablesResult executeGetGraphqlApiEnvironmentVariables(
GetGraphqlApiEnvironmentVariablesRequest getGraphqlApiEnvironmentVariablesRequest) {
ExecutionContext executionContext = createExecutionContext(getGraphqlApiEnvironmentVariablesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetGraphqlApiEnvironmentVariablesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getGraphqlApiEnvironmentVariablesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetGraphqlApiEnvironmentVariables");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetGraphqlApiEnvironmentVariablesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the introspection schema for a GraphQL API.
*
*
* @param getIntrospectionSchemaRequest
* @return Result of the GetIntrospectionSchema operation returned by the service.
* @throws GraphQLSchemaException
* The GraphQL schema is not valid.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.GetIntrospectionSchema
* @see AWS
* API Documentation
*/
@Override
public GetIntrospectionSchemaResult getIntrospectionSchema(GetIntrospectionSchemaRequest request) {
request = beforeClientExecution(request);
return executeGetIntrospectionSchema(request);
}
@SdkInternalApi
final GetIntrospectionSchemaResult executeGetIntrospectionSchema(GetIntrospectionSchemaRequest getIntrospectionSchemaRequest) {
ExecutionContext executionContext = createExecutionContext(getIntrospectionSchemaRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetIntrospectionSchemaRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getIntrospectionSchemaRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetIntrospectionSchema");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(false).withHasStreamingSuccessResponse(false),
new GetIntrospectionSchemaResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a Resolver
object.
*
*
* @param getResolverRequest
* @return Result of the GetResolver operation returned by the service.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @sample AWSAppSync.GetResolver
* @see AWS API
* Documentation
*/
@Override
public GetResolverResult getResolver(GetResolverRequest request) {
request = beforeClientExecution(request);
return executeGetResolver(request);
}
@SdkInternalApi
final GetResolverResult executeGetResolver(GetResolverRequest getResolverRequest) {
ExecutionContext executionContext = createExecutionContext(getResolverRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetResolverRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getResolverRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetResolver");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetResolverResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the current status of a schema creation operation.
*
*
* @param getSchemaCreationStatusRequest
* @return Result of the GetSchemaCreationStatus operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.GetSchemaCreationStatus
* @see AWS API Documentation
*/
@Override
public GetSchemaCreationStatusResult getSchemaCreationStatus(GetSchemaCreationStatusRequest request) {
request = beforeClientExecution(request);
return executeGetSchemaCreationStatus(request);
}
@SdkInternalApi
final GetSchemaCreationStatusResult executeGetSchemaCreationStatus(GetSchemaCreationStatusRequest getSchemaCreationStatusRequest) {
ExecutionContext executionContext = createExecutionContext(getSchemaCreationStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSchemaCreationStatusRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getSchemaCreationStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSchemaCreationStatus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetSchemaCreationStatusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a SourceApiAssociation
object.
*
*
* @param getSourceApiAssociationRequest
* @return Result of the GetSourceApiAssociation operation returned by the service.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @sample AWSAppSync.GetSourceApiAssociation
* @see AWS API Documentation
*/
@Override
public GetSourceApiAssociationResult getSourceApiAssociation(GetSourceApiAssociationRequest request) {
request = beforeClientExecution(request);
return executeGetSourceApiAssociation(request);
}
@SdkInternalApi
final GetSourceApiAssociationResult executeGetSourceApiAssociation(GetSourceApiAssociationRequest getSourceApiAssociationRequest) {
ExecutionContext executionContext = createExecutionContext(getSourceApiAssociationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSourceApiAssociationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getSourceApiAssociationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSourceApiAssociation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetSourceApiAssociationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a Type
object.
*
*
* @param getTypeRequest
* @return Result of the GetType operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.GetType
* @see AWS API
* Documentation
*/
@Override
public GetTypeResult getType(GetTypeRequest request) {
request = beforeClientExecution(request);
return executeGetType(request);
}
@SdkInternalApi
final GetTypeResult executeGetType(GetTypeRequest getTypeRequest) {
ExecutionContext executionContext = createExecutionContext(getTypeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetTypeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getTypeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetType");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetTypeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the API keys for a given API.
*
*
*
* API keys are deleted automatically 60 days after they expire. However, they may still be included in the response
* until they have actually been deleted. You can safely call DeleteApiKey
to manually delete a key
* before it's automatically deleted.
*
*
*
* @param listApiKeysRequest
* @return Result of the ListApiKeys operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListApiKeys
* @see AWS API
* Documentation
*/
@Override
public ListApiKeysResult listApiKeys(ListApiKeysRequest request) {
request = beforeClientExecution(request);
return executeListApiKeys(request);
}
@SdkInternalApi
final ListApiKeysResult executeListApiKeys(ListApiKeysRequest listApiKeysRequest) {
ExecutionContext executionContext = createExecutionContext(listApiKeysRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListApiKeysRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listApiKeysRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListApiKeys");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListApiKeysResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the data sources for a given API.
*
*
* @param listDataSourcesRequest
* @return Result of the ListDataSources operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListDataSources
* @see AWS API
* Documentation
*/
@Override
public ListDataSourcesResult listDataSources(ListDataSourcesRequest request) {
request = beforeClientExecution(request);
return executeListDataSources(request);
}
@SdkInternalApi
final ListDataSourcesResult executeListDataSources(ListDataSourcesRequest listDataSourcesRequest) {
ExecutionContext executionContext = createExecutionContext(listDataSourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDataSourcesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDataSourcesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDataSources");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListDataSourcesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists multiple custom domain names.
*
*
* @param listDomainNamesRequest
* @return Result of the ListDomainNames operation returned by the service.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListDomainNames
* @see AWS API
* Documentation
*/
@Override
public ListDomainNamesResult listDomainNames(ListDomainNamesRequest request) {
request = beforeClientExecution(request);
return executeListDomainNames(request);
}
@SdkInternalApi
final ListDomainNamesResult executeListDomainNames(ListDomainNamesRequest listDomainNamesRequest) {
ExecutionContext executionContext = createExecutionContext(listDomainNamesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDomainNamesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDomainNamesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDomainNames");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListDomainNamesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List multiple functions.
*
*
* @param listFunctionsRequest
* @return Result of the ListFunctions operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListFunctions
* @see AWS API
* Documentation
*/
@Override
public ListFunctionsResult listFunctions(ListFunctionsRequest request) {
request = beforeClientExecution(request);
return executeListFunctions(request);
}
@SdkInternalApi
final ListFunctionsResult executeListFunctions(ListFunctionsRequest listFunctionsRequest) {
ExecutionContext executionContext = createExecutionContext(listFunctionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListFunctionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listFunctionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListFunctions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListFunctionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists your GraphQL APIs.
*
*
* @param listGraphqlApisRequest
* @return Result of the ListGraphqlApis operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListGraphqlApis
* @see AWS API
* Documentation
*/
@Override
public ListGraphqlApisResult listGraphqlApis(ListGraphqlApisRequest request) {
request = beforeClientExecution(request);
return executeListGraphqlApis(request);
}
@SdkInternalApi
final ListGraphqlApisResult executeListGraphqlApis(ListGraphqlApisRequest listGraphqlApisRequest) {
ExecutionContext executionContext = createExecutionContext(listGraphqlApisRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListGraphqlApisRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listGraphqlApisRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListGraphqlApis");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListGraphqlApisResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the resolvers for a given API and type.
*
*
* @param listResolversRequest
* @return Result of the ListResolvers operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListResolvers
* @see AWS API
* Documentation
*/
@Override
public ListResolversResult listResolvers(ListResolversRequest request) {
request = beforeClientExecution(request);
return executeListResolvers(request);
}
@SdkInternalApi
final ListResolversResult executeListResolvers(ListResolversRequest listResolversRequest) {
ExecutionContext executionContext = createExecutionContext(listResolversRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListResolversRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listResolversRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListResolvers");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListResolversResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List the resolvers that are associated with a specific function.
*
*
* @param listResolversByFunctionRequest
* @return Result of the ListResolversByFunction operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListResolversByFunction
* @see AWS API Documentation
*/
@Override
public ListResolversByFunctionResult listResolversByFunction(ListResolversByFunctionRequest request) {
request = beforeClientExecution(request);
return executeListResolversByFunction(request);
}
@SdkInternalApi
final ListResolversByFunctionResult executeListResolversByFunction(ListResolversByFunctionRequest listResolversByFunctionRequest) {
ExecutionContext executionContext = createExecutionContext(listResolversByFunctionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListResolversByFunctionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listResolversByFunctionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListResolversByFunction");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListResolversByFunctionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the SourceApiAssociationSummary
data.
*
*
* @param listSourceApiAssociationsRequest
* @return Result of the ListSourceApiAssociations operation returned by the service.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @sample AWSAppSync.ListSourceApiAssociations
* @see AWS API Documentation
*/
@Override
public ListSourceApiAssociationsResult listSourceApiAssociations(ListSourceApiAssociationsRequest request) {
request = beforeClientExecution(request);
return executeListSourceApiAssociations(request);
}
@SdkInternalApi
final ListSourceApiAssociationsResult executeListSourceApiAssociations(ListSourceApiAssociationsRequest listSourceApiAssociationsRequest) {
ExecutionContext executionContext = createExecutionContext(listSourceApiAssociationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListSourceApiAssociationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listSourceApiAssociationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListSourceApiAssociations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListSourceApiAssociationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the tags for a resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @sample AWSAppSync.ListTagsForResource
* @see AWS
* API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the types for a given API.
*
*
* @param listTypesRequest
* @return Result of the ListTypes operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListTypes
* @see AWS API
* Documentation
*/
@Override
public ListTypesResult listTypes(ListTypesRequest request) {
request = beforeClientExecution(request);
return executeListTypes(request);
}
@SdkInternalApi
final ListTypesResult executeListTypes(ListTypesRequest listTypesRequest) {
ExecutionContext executionContext = createExecutionContext(listTypesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTypesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTypesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTypes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTypesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists Type
objects by the source API association ID.
*
*
* @param listTypesByAssociationRequest
* @return Result of the ListTypesByAssociation operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListTypesByAssociation
* @see AWS
* API Documentation
*/
@Override
public ListTypesByAssociationResult listTypesByAssociation(ListTypesByAssociationRequest request) {
request = beforeClientExecution(request);
return executeListTypesByAssociation(request);
}
@SdkInternalApi
final ListTypesByAssociationResult executeListTypesByAssociation(ListTypesByAssociationRequest listTypesByAssociationRequest) {
ExecutionContext executionContext = createExecutionContext(listTypesByAssociationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTypesByAssociationRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTypesByAssociationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTypesByAssociation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListTypesByAssociationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a list of environmental variables in an API by its ID value.
*
*
* When creating an environmental variable, it must follow the constraints below:
*
*
* -
*
* Both JavaScript and VTL templates support environmental variables.
*
*
* -
*
* Environmental variables are not evaluated before function invocation.
*
*
* -
*
* Environmental variables only support string values.
*
*
* -
*
* Any defined value in an environmental variable is considered a string literal and not expanded.
*
*
* -
*
* Variable evaluations should ideally be performed in the function code.
*
*
*
*
* When creating an environmental variable key-value pair, it must follow the additional constraints below:
*
*
* -
*
* Keys must begin with a letter.
*
*
* -
*
* Keys must be at least two characters long.
*
*
* -
*
* Keys can only contain letters, numbers, and the underscore character (_).
*
*
* -
*
* Values can be up to 512 characters long.
*
*
* -
*
* You can configure up to 50 key-value pairs in a GraphQL API.
*
*
*
*
* You can create a list of environmental variables by adding it to the environmentVariables
payload as
* a list in the format {"key1":"value1","key2":"value2", …}
. Note that each call of the
* PutGraphqlApiEnvironmentVariables
action will result in the overwriting of the existing
* environmental variable list of that API. This means the existing environmental variables will be lost. To avoid
* this, you must include all existing and new environmental variables in the list each time you call this action.
*
*
* @param putGraphqlApiEnvironmentVariablesRequest
* @return Result of the PutGraphqlApiEnvironmentVariables operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @sample AWSAppSync.PutGraphqlApiEnvironmentVariables
* @see AWS API Documentation
*/
@Override
public PutGraphqlApiEnvironmentVariablesResult putGraphqlApiEnvironmentVariables(PutGraphqlApiEnvironmentVariablesRequest request) {
request = beforeClientExecution(request);
return executePutGraphqlApiEnvironmentVariables(request);
}
@SdkInternalApi
final PutGraphqlApiEnvironmentVariablesResult executePutGraphqlApiEnvironmentVariables(
PutGraphqlApiEnvironmentVariablesRequest putGraphqlApiEnvironmentVariablesRequest) {
ExecutionContext executionContext = createExecutionContext(putGraphqlApiEnvironmentVariablesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutGraphqlApiEnvironmentVariablesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putGraphqlApiEnvironmentVariablesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutGraphqlApiEnvironmentVariables");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutGraphqlApiEnvironmentVariablesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new introspection. Returns the introspectionId
of the new introspection after its
* creation.
*
*
* @param startDataSourceIntrospectionRequest
* @return Result of the StartDataSourceIntrospection operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @sample AWSAppSync.StartDataSourceIntrospection
* @see AWS API Documentation
*/
@Override
public StartDataSourceIntrospectionResult startDataSourceIntrospection(StartDataSourceIntrospectionRequest request) {
request = beforeClientExecution(request);
return executeStartDataSourceIntrospection(request);
}
@SdkInternalApi
final StartDataSourceIntrospectionResult executeStartDataSourceIntrospection(StartDataSourceIntrospectionRequest startDataSourceIntrospectionRequest) {
ExecutionContext executionContext = createExecutionContext(startDataSourceIntrospectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartDataSourceIntrospectionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(startDataSourceIntrospectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartDataSourceIntrospection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StartDataSourceIntrospectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds a new schema to your GraphQL API.
*
*
* This operation is asynchronous. Use to determine when it has completed.
*
*
* @param startSchemaCreationRequest
* @return Result of the StartSchemaCreation operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.StartSchemaCreation
* @see AWS
* API Documentation
*/
@Override
public StartSchemaCreationResult startSchemaCreation(StartSchemaCreationRequest request) {
request = beforeClientExecution(request);
return executeStartSchemaCreation(request);
}
@SdkInternalApi
final StartSchemaCreationResult executeStartSchemaCreation(StartSchemaCreationRequest startSchemaCreationRequest) {
ExecutionContext executionContext = createExecutionContext(startSchemaCreationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartSchemaCreationRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startSchemaCreationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartSchemaCreation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StartSchemaCreationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Initiates a merge operation. Returns a status that shows the result of the merge operation.
*
*
* @param startSchemaMergeRequest
* @return Result of the StartSchemaMerge operation returned by the service.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @sample AWSAppSync.StartSchemaMerge
* @see AWS API
* Documentation
*/
@Override
public StartSchemaMergeResult startSchemaMerge(StartSchemaMergeRequest request) {
request = beforeClientExecution(request);
return executeStartSchemaMerge(request);
}
@SdkInternalApi
final StartSchemaMergeResult executeStartSchemaMerge(StartSchemaMergeRequest startSchemaMergeRequest) {
ExecutionContext executionContext = createExecutionContext(startSchemaMergeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartSchemaMergeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startSchemaMergeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartSchemaMerge");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StartSchemaMergeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Tags a resource with user-supplied tags.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @sample AWSAppSync.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Untags a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @sample AWSAppSync.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the cache for the GraphQL API.
*
*
* @param updateApiCacheRequest
* Represents the input of a UpdateApiCache
operation.
* @return Result of the UpdateApiCache operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.UpdateApiCache
* @see AWS API
* Documentation
*/
@Override
public UpdateApiCacheResult updateApiCache(UpdateApiCacheRequest request) {
request = beforeClientExecution(request);
return executeUpdateApiCache(request);
}
@SdkInternalApi
final UpdateApiCacheResult executeUpdateApiCache(UpdateApiCacheRequest updateApiCacheRequest) {
ExecutionContext executionContext = createExecutionContext(updateApiCacheRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateApiCacheRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateApiCacheRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateApiCache");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateApiCacheResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an API key. You can update the key as long as it's not deleted.
*
*
* @param updateApiKeyRequest
* @return Result of the UpdateApiKey operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws ApiKeyValidityOutOfBoundsException
* The API key expiration must be set to a value between 1 and 365 days from creation (for
* CreateApiKey
) or from update (for UpdateApiKey
).
* @sample AWSAppSync.UpdateApiKey
* @see AWS API
* Documentation
*/
@Override
public UpdateApiKeyResult updateApiKey(UpdateApiKeyRequest request) {
request = beforeClientExecution(request);
return executeUpdateApiKey(request);
}
@SdkInternalApi
final UpdateApiKeyResult executeUpdateApiKey(UpdateApiKeyRequest updateApiKeyRequest) {
ExecutionContext executionContext = createExecutionContext(updateApiKeyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateApiKeyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateApiKeyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateApiKey");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateApiKeyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a DataSource
object.
*
*
* @param updateDataSourceRequest
* @return Result of the UpdateDataSource operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.UpdateDataSource
* @see AWS API
* Documentation
*/
@Override
public UpdateDataSourceResult updateDataSource(UpdateDataSourceRequest request) {
request = beforeClientExecution(request);
return executeUpdateDataSource(request);
}
@SdkInternalApi
final UpdateDataSourceResult executeUpdateDataSource(UpdateDataSourceRequest updateDataSourceRequest) {
ExecutionContext executionContext = createExecutionContext(updateDataSourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateDataSourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateDataSourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateDataSource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateDataSourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a custom DomainName
object.
*
*
* @param updateDomainNameRequest
* @return Result of the UpdateDomainName operation returned by the service.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @sample AWSAppSync.UpdateDomainName
* @see AWS API
* Documentation
*/
@Override
public UpdateDomainNameResult updateDomainName(UpdateDomainNameRequest request) {
request = beforeClientExecution(request);
return executeUpdateDomainName(request);
}
@SdkInternalApi
final UpdateDomainNameResult executeUpdateDomainName(UpdateDomainNameRequest updateDomainNameRequest) {
ExecutionContext executionContext = createExecutionContext(updateDomainNameRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateDomainNameRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateDomainNameRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateDomainName");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateDomainNameResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a Function
object.
*
*
* @param updateFunctionRequest
* @return Result of the UpdateFunction operation returned by the service.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @sample AWSAppSync.UpdateFunction
* @see AWS API
* Documentation
*/
@Override
public UpdateFunctionResult updateFunction(UpdateFunctionRequest request) {
request = beforeClientExecution(request);
return executeUpdateFunction(request);
}
@SdkInternalApi
final UpdateFunctionResult executeUpdateFunction(UpdateFunctionRequest updateFunctionRequest) {
ExecutionContext executionContext = createExecutionContext(updateFunctionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateFunctionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateFunctionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateFunction");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateFunctionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a GraphqlApi
object.
*
*
* @param updateGraphqlApiRequest
* @return Result of the UpdateGraphqlApi operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @sample AWSAppSync.UpdateGraphqlApi
* @see AWS API
* Documentation
*/
@Override
public UpdateGraphqlApiResult updateGraphqlApi(UpdateGraphqlApiRequest request) {
request = beforeClientExecution(request);
return executeUpdateGraphqlApi(request);
}
@SdkInternalApi
final UpdateGraphqlApiResult executeUpdateGraphqlApi(UpdateGraphqlApiRequest updateGraphqlApiRequest) {
ExecutionContext executionContext = createExecutionContext(updateGraphqlApiRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateGraphqlApiRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateGraphqlApiRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateGraphqlApi");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateGraphqlApiResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a Resolver
object.
*
*
* @param updateResolverRequest
* @return Result of the UpdateResolver operation returned by the service.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @sample AWSAppSync.UpdateResolver
* @see AWS API
* Documentation
*/
@Override
public UpdateResolverResult updateResolver(UpdateResolverRequest request) {
request = beforeClientExecution(request);
return executeUpdateResolver(request);
}
@SdkInternalApi
final UpdateResolverResult executeUpdateResolver(UpdateResolverRequest updateResolverRequest) {
ExecutionContext executionContext = createExecutionContext(updateResolverRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateResolverRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateResolverRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateResolver");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateResolverResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates some of the configuration choices of a particular source API association.
*
*
* @param updateSourceApiAssociationRequest
* @return Result of the UpdateSourceApiAssociation operation returned by the service.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @sample AWSAppSync.UpdateSourceApiAssociation
* @see AWS API Documentation
*/
@Override
public UpdateSourceApiAssociationResult updateSourceApiAssociation(UpdateSourceApiAssociationRequest request) {
request = beforeClientExecution(request);
return executeUpdateSourceApiAssociation(request);
}
@SdkInternalApi
final UpdateSourceApiAssociationResult executeUpdateSourceApiAssociation(UpdateSourceApiAssociationRequest updateSourceApiAssociationRequest) {
ExecutionContext executionContext = createExecutionContext(updateSourceApiAssociationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateSourceApiAssociationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateSourceApiAssociationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateSourceApiAssociation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateSourceApiAssociationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a Type
object.
*
*
* @param updateTypeRequest
* @return Result of the UpdateType operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.UpdateType
* @see AWS API
* Documentation
*/
@Override
public UpdateTypeResult updateType(UpdateTypeRequest request) {
request = beforeClientExecution(request);
return executeUpdateType(request);
}
@SdkInternalApi
final UpdateTypeResult executeUpdateType(UpdateTypeRequest updateTypeRequest) {
ExecutionContext executionContext = createExecutionContext(updateTypeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateTypeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateTypeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "AppSync");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateType");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateTypeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}