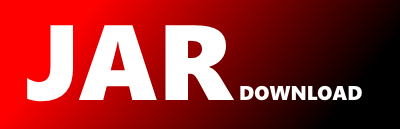
com.amazonaws.services.appsync.model.CreateGraphqlApiRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appsync Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appsync.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateGraphqlApiRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* A user-supplied name for the GraphqlApi
.
*
*/
private String name;
/**
*
* The Amazon CloudWatch Logs configuration.
*
*/
private LogConfig logConfig;
/**
*
* The authentication type: API key, Identity and Access Management (IAM), OpenID Connect (OIDC), Amazon Cognito
* user pools, or Lambda.
*
*/
private String authenticationType;
/**
*
* The Amazon Cognito user pool configuration.
*
*/
private UserPoolConfig userPoolConfig;
/**
*
* The OIDC configuration.
*
*/
private OpenIDConnectConfig openIDConnectConfig;
/**
*
* A TagMap
object.
*
*/
private java.util.Map tags;
/**
*
* A list of additional authentication providers for the GraphqlApi
API.
*
*/
private java.util.List additionalAuthenticationProviders;
/**
*
* A flag indicating whether to use X-Ray tracing for the GraphqlApi
.
*
*/
private Boolean xrayEnabled;
/**
*
* Configuration for Lambda function authorization.
*
*/
private LambdaAuthorizerConfig lambdaAuthorizerConfig;
/**
*
* Sets the value of the GraphQL API to public (GLOBAL
) or private (PRIVATE
). If no value
* is provided, the visibility will be set to GLOBAL
by default. This value cannot be changed once the
* API has been created.
*
*/
private String visibility;
/**
*
* The value that indicates whether the GraphQL API is a standard API (GRAPHQL
) or merged API (
* MERGED
).
*
*/
private String apiType;
/**
*
* The Identity and Access Management service role ARN for a merged API. The AppSync service assumes this role on
* behalf of the Merged API to validate access to source APIs at runtime and to prompt the AUTO_MERGE
* to update the merged API endpoint with the source API changes automatically.
*
*/
private String mergedApiExecutionRoleArn;
/**
*
* The owner contact information for an API resource.
*
*
* This field accepts any string input with a length of 0 - 256 characters.
*
*/
private String ownerContact;
/**
*
* Sets the value of the GraphQL API to enable (ENABLED
) or disable (DISABLED
)
* introspection. If no value is provided, the introspection configuration will be set to ENABLED
by
* default. This field will produce an error if the operation attempts to use the introspection feature while this
* field is disabled.
*
*
* For more information about introspection, see GraphQL
* introspection.
*
*/
private String introspectionConfig;
/**
*
* The maximum depth a query can have in a single request. Depth refers to the amount of nested levels allowed in
* the body of query. The default value is 0
(or unspecified), which indicates there's no depth limit.
* If you set a limit, it can be between 1
and 75
nested levels. This field will produce a
* limit error if the operation falls out of bounds.
*
*
* Note that fields can still be set to nullable or non-nullable. If a non-nullable field produces an error, the
* error will be thrown upwards to the first nullable field available.
*
*/
private Integer queryDepthLimit;
/**
*
* The maximum number of resolvers that can be invoked in a single request. The default value is 0
(or
* unspecified), which will set the limit to 10000
. When specified, the limit value can be between
* 1
and 10000
. This field will produce a limit error if the operation falls out of
* bounds.
*
*/
private Integer resolverCountLimit;
/**
*
* The enhancedMetricsConfig
object.
*
*/
private EnhancedMetricsConfig enhancedMetricsConfig;
/**
*
* A user-supplied name for the GraphqlApi
.
*
*
* @param name
* A user-supplied name for the GraphqlApi
.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* A user-supplied name for the GraphqlApi
.
*
*
* @return A user-supplied name for the GraphqlApi
.
*/
public String getName() {
return this.name;
}
/**
*
* A user-supplied name for the GraphqlApi
.
*
*
* @param name
* A user-supplied name for the GraphqlApi
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateGraphqlApiRequest withName(String name) {
setName(name);
return this;
}
/**
*
* The Amazon CloudWatch Logs configuration.
*
*
* @param logConfig
* The Amazon CloudWatch Logs configuration.
*/
public void setLogConfig(LogConfig logConfig) {
this.logConfig = logConfig;
}
/**
*
* The Amazon CloudWatch Logs configuration.
*
*
* @return The Amazon CloudWatch Logs configuration.
*/
public LogConfig getLogConfig() {
return this.logConfig;
}
/**
*
* The Amazon CloudWatch Logs configuration.
*
*
* @param logConfig
* The Amazon CloudWatch Logs configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateGraphqlApiRequest withLogConfig(LogConfig logConfig) {
setLogConfig(logConfig);
return this;
}
/**
*
* The authentication type: API key, Identity and Access Management (IAM), OpenID Connect (OIDC), Amazon Cognito
* user pools, or Lambda.
*
*
* @param authenticationType
* The authentication type: API key, Identity and Access Management (IAM), OpenID Connect (OIDC), Amazon
* Cognito user pools, or Lambda.
* @see AuthenticationType
*/
public void setAuthenticationType(String authenticationType) {
this.authenticationType = authenticationType;
}
/**
*
* The authentication type: API key, Identity and Access Management (IAM), OpenID Connect (OIDC), Amazon Cognito
* user pools, or Lambda.
*
*
* @return The authentication type: API key, Identity and Access Management (IAM), OpenID Connect (OIDC), Amazon
* Cognito user pools, or Lambda.
* @see AuthenticationType
*/
public String getAuthenticationType() {
return this.authenticationType;
}
/**
*
* The authentication type: API key, Identity and Access Management (IAM), OpenID Connect (OIDC), Amazon Cognito
* user pools, or Lambda.
*
*
* @param authenticationType
* The authentication type: API key, Identity and Access Management (IAM), OpenID Connect (OIDC), Amazon
* Cognito user pools, or Lambda.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthenticationType
*/
public CreateGraphqlApiRequest withAuthenticationType(String authenticationType) {
setAuthenticationType(authenticationType);
return this;
}
/**
*
* The authentication type: API key, Identity and Access Management (IAM), OpenID Connect (OIDC), Amazon Cognito
* user pools, or Lambda.
*
*
* @param authenticationType
* The authentication type: API key, Identity and Access Management (IAM), OpenID Connect (OIDC), Amazon
* Cognito user pools, or Lambda.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthenticationType
*/
public CreateGraphqlApiRequest withAuthenticationType(AuthenticationType authenticationType) {
this.authenticationType = authenticationType.toString();
return this;
}
/**
*
* The Amazon Cognito user pool configuration.
*
*
* @param userPoolConfig
* The Amazon Cognito user pool configuration.
*/
public void setUserPoolConfig(UserPoolConfig userPoolConfig) {
this.userPoolConfig = userPoolConfig;
}
/**
*
* The Amazon Cognito user pool configuration.
*
*
* @return The Amazon Cognito user pool configuration.
*/
public UserPoolConfig getUserPoolConfig() {
return this.userPoolConfig;
}
/**
*
* The Amazon Cognito user pool configuration.
*
*
* @param userPoolConfig
* The Amazon Cognito user pool configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateGraphqlApiRequest withUserPoolConfig(UserPoolConfig userPoolConfig) {
setUserPoolConfig(userPoolConfig);
return this;
}
/**
*
* The OIDC configuration.
*
*
* @param openIDConnectConfig
* The OIDC configuration.
*/
public void setOpenIDConnectConfig(OpenIDConnectConfig openIDConnectConfig) {
this.openIDConnectConfig = openIDConnectConfig;
}
/**
*
* The OIDC configuration.
*
*
* @return The OIDC configuration.
*/
public OpenIDConnectConfig getOpenIDConnectConfig() {
return this.openIDConnectConfig;
}
/**
*
* The OIDC configuration.
*
*
* @param openIDConnectConfig
* The OIDC configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateGraphqlApiRequest withOpenIDConnectConfig(OpenIDConnectConfig openIDConnectConfig) {
setOpenIDConnectConfig(openIDConnectConfig);
return this;
}
/**
*
* A TagMap
object.
*
*
* @return A TagMap
object.
*/
public java.util.Map getTags() {
return tags;
}
/**
*
* A TagMap
object.
*
*
* @param tags
* A TagMap
object.
*/
public void setTags(java.util.Map tags) {
this.tags = tags;
}
/**
*
* A TagMap
object.
*
*
* @param tags
* A TagMap
object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateGraphqlApiRequest withTags(java.util.Map tags) {
setTags(tags);
return this;
}
/**
* Add a single Tags entry
*
* @see CreateGraphqlApiRequest#withTags
* @returns a reference to this object so that method calls can be chained together.
*/
public CreateGraphqlApiRequest addTagsEntry(String key, String value) {
if (null == this.tags) {
this.tags = new java.util.HashMap();
}
if (this.tags.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.tags.put(key, value);
return this;
}
/**
* Removes all the entries added into Tags.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateGraphqlApiRequest clearTagsEntries() {
this.tags = null;
return this;
}
/**
*
* A list of additional authentication providers for the GraphqlApi
API.
*
*
* @return A list of additional authentication providers for the GraphqlApi
API.
*/
public java.util.List getAdditionalAuthenticationProviders() {
return additionalAuthenticationProviders;
}
/**
*
* A list of additional authentication providers for the GraphqlApi
API.
*
*
* @param additionalAuthenticationProviders
* A list of additional authentication providers for the GraphqlApi
API.
*/
public void setAdditionalAuthenticationProviders(java.util.Collection additionalAuthenticationProviders) {
if (additionalAuthenticationProviders == null) {
this.additionalAuthenticationProviders = null;
return;
}
this.additionalAuthenticationProviders = new java.util.ArrayList(additionalAuthenticationProviders);
}
/**
*
* A list of additional authentication providers for the GraphqlApi
API.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAdditionalAuthenticationProviders(java.util.Collection)} or
* {@link #withAdditionalAuthenticationProviders(java.util.Collection)} if you want to override the existing values.
*
*
* @param additionalAuthenticationProviders
* A list of additional authentication providers for the GraphqlApi
API.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateGraphqlApiRequest withAdditionalAuthenticationProviders(AdditionalAuthenticationProvider... additionalAuthenticationProviders) {
if (this.additionalAuthenticationProviders == null) {
setAdditionalAuthenticationProviders(new java.util.ArrayList(additionalAuthenticationProviders.length));
}
for (AdditionalAuthenticationProvider ele : additionalAuthenticationProviders) {
this.additionalAuthenticationProviders.add(ele);
}
return this;
}
/**
*
* A list of additional authentication providers for the GraphqlApi
API.
*
*
* @param additionalAuthenticationProviders
* A list of additional authentication providers for the GraphqlApi
API.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateGraphqlApiRequest withAdditionalAuthenticationProviders(
java.util.Collection additionalAuthenticationProviders) {
setAdditionalAuthenticationProviders(additionalAuthenticationProviders);
return this;
}
/**
*
* A flag indicating whether to use X-Ray tracing for the GraphqlApi
.
*
*
* @param xrayEnabled
* A flag indicating whether to use X-Ray tracing for the GraphqlApi
.
*/
public void setXrayEnabled(Boolean xrayEnabled) {
this.xrayEnabled = xrayEnabled;
}
/**
*
* A flag indicating whether to use X-Ray tracing for the GraphqlApi
.
*
*
* @return A flag indicating whether to use X-Ray tracing for the GraphqlApi
.
*/
public Boolean getXrayEnabled() {
return this.xrayEnabled;
}
/**
*
* A flag indicating whether to use X-Ray tracing for the GraphqlApi
.
*
*
* @param xrayEnabled
* A flag indicating whether to use X-Ray tracing for the GraphqlApi
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateGraphqlApiRequest withXrayEnabled(Boolean xrayEnabled) {
setXrayEnabled(xrayEnabled);
return this;
}
/**
*
* A flag indicating whether to use X-Ray tracing for the GraphqlApi
.
*
*
* @return A flag indicating whether to use X-Ray tracing for the GraphqlApi
.
*/
public Boolean isXrayEnabled() {
return this.xrayEnabled;
}
/**
*
* Configuration for Lambda function authorization.
*
*
* @param lambdaAuthorizerConfig
* Configuration for Lambda function authorization.
*/
public void setLambdaAuthorizerConfig(LambdaAuthorizerConfig lambdaAuthorizerConfig) {
this.lambdaAuthorizerConfig = lambdaAuthorizerConfig;
}
/**
*
* Configuration for Lambda function authorization.
*
*
* @return Configuration for Lambda function authorization.
*/
public LambdaAuthorizerConfig getLambdaAuthorizerConfig() {
return this.lambdaAuthorizerConfig;
}
/**
*
* Configuration for Lambda function authorization.
*
*
* @param lambdaAuthorizerConfig
* Configuration for Lambda function authorization.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateGraphqlApiRequest withLambdaAuthorizerConfig(LambdaAuthorizerConfig lambdaAuthorizerConfig) {
setLambdaAuthorizerConfig(lambdaAuthorizerConfig);
return this;
}
/**
*
* Sets the value of the GraphQL API to public (GLOBAL
) or private (PRIVATE
). If no value
* is provided, the visibility will be set to GLOBAL
by default. This value cannot be changed once the
* API has been created.
*
*
* @param visibility
* Sets the value of the GraphQL API to public (GLOBAL
) or private (PRIVATE
). If no
* value is provided, the visibility will be set to GLOBAL
by default. This value cannot be
* changed once the API has been created.
* @see GraphQLApiVisibility
*/
public void setVisibility(String visibility) {
this.visibility = visibility;
}
/**
*
* Sets the value of the GraphQL API to public (GLOBAL
) or private (PRIVATE
). If no value
* is provided, the visibility will be set to GLOBAL
by default. This value cannot be changed once the
* API has been created.
*
*
* @return Sets the value of the GraphQL API to public (GLOBAL
) or private (PRIVATE
). If
* no value is provided, the visibility will be set to GLOBAL
by default. This value cannot be
* changed once the API has been created.
* @see GraphQLApiVisibility
*/
public String getVisibility() {
return this.visibility;
}
/**
*
* Sets the value of the GraphQL API to public (GLOBAL
) or private (PRIVATE
). If no value
* is provided, the visibility will be set to GLOBAL
by default. This value cannot be changed once the
* API has been created.
*
*
* @param visibility
* Sets the value of the GraphQL API to public (GLOBAL
) or private (PRIVATE
). If no
* value is provided, the visibility will be set to GLOBAL
by default. This value cannot be
* changed once the API has been created.
* @return Returns a reference to this object so that method calls can be chained together.
* @see GraphQLApiVisibility
*/
public CreateGraphqlApiRequest withVisibility(String visibility) {
setVisibility(visibility);
return this;
}
/**
*
* Sets the value of the GraphQL API to public (GLOBAL
) or private (PRIVATE
). If no value
* is provided, the visibility will be set to GLOBAL
by default. This value cannot be changed once the
* API has been created.
*
*
* @param visibility
* Sets the value of the GraphQL API to public (GLOBAL
) or private (PRIVATE
). If no
* value is provided, the visibility will be set to GLOBAL
by default. This value cannot be
* changed once the API has been created.
* @return Returns a reference to this object so that method calls can be chained together.
* @see GraphQLApiVisibility
*/
public CreateGraphqlApiRequest withVisibility(GraphQLApiVisibility visibility) {
this.visibility = visibility.toString();
return this;
}
/**
*
* The value that indicates whether the GraphQL API is a standard API (GRAPHQL
) or merged API (
* MERGED
).
*
*
* @param apiType
* The value that indicates whether the GraphQL API is a standard API (GRAPHQL
) or merged API (
* MERGED
).
* @see GraphQLApiType
*/
public void setApiType(String apiType) {
this.apiType = apiType;
}
/**
*
* The value that indicates whether the GraphQL API is a standard API (GRAPHQL
) or merged API (
* MERGED
).
*
*
* @return The value that indicates whether the GraphQL API is a standard API (GRAPHQL
) or merged API (
* MERGED
).
* @see GraphQLApiType
*/
public String getApiType() {
return this.apiType;
}
/**
*
* The value that indicates whether the GraphQL API is a standard API (GRAPHQL
) or merged API (
* MERGED
).
*
*
* @param apiType
* The value that indicates whether the GraphQL API is a standard API (GRAPHQL
) or merged API (
* MERGED
).
* @return Returns a reference to this object so that method calls can be chained together.
* @see GraphQLApiType
*/
public CreateGraphqlApiRequest withApiType(String apiType) {
setApiType(apiType);
return this;
}
/**
*
* The value that indicates whether the GraphQL API is a standard API (GRAPHQL
) or merged API (
* MERGED
).
*
*
* @param apiType
* The value that indicates whether the GraphQL API is a standard API (GRAPHQL
) or merged API (
* MERGED
).
* @return Returns a reference to this object so that method calls can be chained together.
* @see GraphQLApiType
*/
public CreateGraphqlApiRequest withApiType(GraphQLApiType apiType) {
this.apiType = apiType.toString();
return this;
}
/**
*
* The Identity and Access Management service role ARN for a merged API. The AppSync service assumes this role on
* behalf of the Merged API to validate access to source APIs at runtime and to prompt the AUTO_MERGE
* to update the merged API endpoint with the source API changes automatically.
*
*
* @param mergedApiExecutionRoleArn
* The Identity and Access Management service role ARN for a merged API. The AppSync service assumes this
* role on behalf of the Merged API to validate access to source APIs at runtime and to prompt the
* AUTO_MERGE
to update the merged API endpoint with the source API changes automatically.
*/
public void setMergedApiExecutionRoleArn(String mergedApiExecutionRoleArn) {
this.mergedApiExecutionRoleArn = mergedApiExecutionRoleArn;
}
/**
*
* The Identity and Access Management service role ARN for a merged API. The AppSync service assumes this role on
* behalf of the Merged API to validate access to source APIs at runtime and to prompt the AUTO_MERGE
* to update the merged API endpoint with the source API changes automatically.
*
*
* @return The Identity and Access Management service role ARN for a merged API. The AppSync service assumes this
* role on behalf of the Merged API to validate access to source APIs at runtime and to prompt the
* AUTO_MERGE
to update the merged API endpoint with the source API changes automatically.
*/
public String getMergedApiExecutionRoleArn() {
return this.mergedApiExecutionRoleArn;
}
/**
*
* The Identity and Access Management service role ARN for a merged API. The AppSync service assumes this role on
* behalf of the Merged API to validate access to source APIs at runtime and to prompt the AUTO_MERGE
* to update the merged API endpoint with the source API changes automatically.
*
*
* @param mergedApiExecutionRoleArn
* The Identity and Access Management service role ARN for a merged API. The AppSync service assumes this
* role on behalf of the Merged API to validate access to source APIs at runtime and to prompt the
* AUTO_MERGE
to update the merged API endpoint with the source API changes automatically.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateGraphqlApiRequest withMergedApiExecutionRoleArn(String mergedApiExecutionRoleArn) {
setMergedApiExecutionRoleArn(mergedApiExecutionRoleArn);
return this;
}
/**
*
* The owner contact information for an API resource.
*
*
* This field accepts any string input with a length of 0 - 256 characters.
*
*
* @param ownerContact
* The owner contact information for an API resource.
*
* This field accepts any string input with a length of 0 - 256 characters.
*/
public void setOwnerContact(String ownerContact) {
this.ownerContact = ownerContact;
}
/**
*
* The owner contact information for an API resource.
*
*
* This field accepts any string input with a length of 0 - 256 characters.
*
*
* @return The owner contact information for an API resource.
*
* This field accepts any string input with a length of 0 - 256 characters.
*/
public String getOwnerContact() {
return this.ownerContact;
}
/**
*
* The owner contact information for an API resource.
*
*
* This field accepts any string input with a length of 0 - 256 characters.
*
*
* @param ownerContact
* The owner contact information for an API resource.
*
* This field accepts any string input with a length of 0 - 256 characters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateGraphqlApiRequest withOwnerContact(String ownerContact) {
setOwnerContact(ownerContact);
return this;
}
/**
*
* Sets the value of the GraphQL API to enable (ENABLED
) or disable (DISABLED
)
* introspection. If no value is provided, the introspection configuration will be set to ENABLED
by
* default. This field will produce an error if the operation attempts to use the introspection feature while this
* field is disabled.
*
*
* For more information about introspection, see GraphQL
* introspection.
*
*
* @param introspectionConfig
* Sets the value of the GraphQL API to enable (ENABLED
) or disable (DISABLED
)
* introspection. If no value is provided, the introspection configuration will be set to
* ENABLED
by default. This field will produce an error if the operation attempts to use the
* introspection feature while this field is disabled.
*
* For more information about introspection, see GraphQL
* introspection.
* @see GraphQLApiIntrospectionConfig
*/
public void setIntrospectionConfig(String introspectionConfig) {
this.introspectionConfig = introspectionConfig;
}
/**
*
* Sets the value of the GraphQL API to enable (ENABLED
) or disable (DISABLED
)
* introspection. If no value is provided, the introspection configuration will be set to ENABLED
by
* default. This field will produce an error if the operation attempts to use the introspection feature while this
* field is disabled.
*
*
* For more information about introspection, see GraphQL
* introspection.
*
*
* @return Sets the value of the GraphQL API to enable (ENABLED
) or disable (DISABLED
)
* introspection. If no value is provided, the introspection configuration will be set to
* ENABLED
by default. This field will produce an error if the operation attempts to use the
* introspection feature while this field is disabled.
*
* For more information about introspection, see GraphQL
* introspection.
* @see GraphQLApiIntrospectionConfig
*/
public String getIntrospectionConfig() {
return this.introspectionConfig;
}
/**
*
* Sets the value of the GraphQL API to enable (ENABLED
) or disable (DISABLED
)
* introspection. If no value is provided, the introspection configuration will be set to ENABLED
by
* default. This field will produce an error if the operation attempts to use the introspection feature while this
* field is disabled.
*
*
* For more information about introspection, see GraphQL
* introspection.
*
*
* @param introspectionConfig
* Sets the value of the GraphQL API to enable (ENABLED
) or disable (DISABLED
)
* introspection. If no value is provided, the introspection configuration will be set to
* ENABLED
by default. This field will produce an error if the operation attempts to use the
* introspection feature while this field is disabled.
*
* For more information about introspection, see GraphQL
* introspection.
* @return Returns a reference to this object so that method calls can be chained together.
* @see GraphQLApiIntrospectionConfig
*/
public CreateGraphqlApiRequest withIntrospectionConfig(String introspectionConfig) {
setIntrospectionConfig(introspectionConfig);
return this;
}
/**
*
* Sets the value of the GraphQL API to enable (ENABLED
) or disable (DISABLED
)
* introspection. If no value is provided, the introspection configuration will be set to ENABLED
by
* default. This field will produce an error if the operation attempts to use the introspection feature while this
* field is disabled.
*
*
* For more information about introspection, see GraphQL
* introspection.
*
*
* @param introspectionConfig
* Sets the value of the GraphQL API to enable (ENABLED
) or disable (DISABLED
)
* introspection. If no value is provided, the introspection configuration will be set to
* ENABLED
by default. This field will produce an error if the operation attempts to use the
* introspection feature while this field is disabled.
*
* For more information about introspection, see GraphQL
* introspection.
* @return Returns a reference to this object so that method calls can be chained together.
* @see GraphQLApiIntrospectionConfig
*/
public CreateGraphqlApiRequest withIntrospectionConfig(GraphQLApiIntrospectionConfig introspectionConfig) {
this.introspectionConfig = introspectionConfig.toString();
return this;
}
/**
*
* The maximum depth a query can have in a single request. Depth refers to the amount of nested levels allowed in
* the body of query. The default value is 0
(or unspecified), which indicates there's no depth limit.
* If you set a limit, it can be between 1
and 75
nested levels. This field will produce a
* limit error if the operation falls out of bounds.
*
*
* Note that fields can still be set to nullable or non-nullable. If a non-nullable field produces an error, the
* error will be thrown upwards to the first nullable field available.
*
*
* @param queryDepthLimit
* The maximum depth a query can have in a single request. Depth refers to the amount of nested levels
* allowed in the body of query. The default value is 0
(or unspecified), which indicates
* there's no depth limit. If you set a limit, it can be between 1
and 75
nested
* levels. This field will produce a limit error if the operation falls out of bounds.
*
* Note that fields can still be set to nullable or non-nullable. If a non-nullable field produces an error,
* the error will be thrown upwards to the first nullable field available.
*/
public void setQueryDepthLimit(Integer queryDepthLimit) {
this.queryDepthLimit = queryDepthLimit;
}
/**
*
* The maximum depth a query can have in a single request. Depth refers to the amount of nested levels allowed in
* the body of query. The default value is 0
(or unspecified), which indicates there's no depth limit.
* If you set a limit, it can be between 1
and 75
nested levels. This field will produce a
* limit error if the operation falls out of bounds.
*
*
* Note that fields can still be set to nullable or non-nullable. If a non-nullable field produces an error, the
* error will be thrown upwards to the first nullable field available.
*
*
* @return The maximum depth a query can have in a single request. Depth refers to the amount of nested levels
* allowed in the body of query. The default value is 0
(or unspecified), which indicates
* there's no depth limit. If you set a limit, it can be between 1
and 75
nested
* levels. This field will produce a limit error if the operation falls out of bounds.
*
* Note that fields can still be set to nullable or non-nullable. If a non-nullable field produces an error,
* the error will be thrown upwards to the first nullable field available.
*/
public Integer getQueryDepthLimit() {
return this.queryDepthLimit;
}
/**
*
* The maximum depth a query can have in a single request. Depth refers to the amount of nested levels allowed in
* the body of query. The default value is 0
(or unspecified), which indicates there's no depth limit.
* If you set a limit, it can be between 1
and 75
nested levels. This field will produce a
* limit error if the operation falls out of bounds.
*
*
* Note that fields can still be set to nullable or non-nullable. If a non-nullable field produces an error, the
* error will be thrown upwards to the first nullable field available.
*
*
* @param queryDepthLimit
* The maximum depth a query can have in a single request. Depth refers to the amount of nested levels
* allowed in the body of query. The default value is 0
(or unspecified), which indicates
* there's no depth limit. If you set a limit, it can be between 1
and 75
nested
* levels. This field will produce a limit error if the operation falls out of bounds.
*
* Note that fields can still be set to nullable or non-nullable. If a non-nullable field produces an error,
* the error will be thrown upwards to the first nullable field available.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateGraphqlApiRequest withQueryDepthLimit(Integer queryDepthLimit) {
setQueryDepthLimit(queryDepthLimit);
return this;
}
/**
*
* The maximum number of resolvers that can be invoked in a single request. The default value is 0
(or
* unspecified), which will set the limit to 10000
. When specified, the limit value can be between
* 1
and 10000
. This field will produce a limit error if the operation falls out of
* bounds.
*
*
* @param resolverCountLimit
* The maximum number of resolvers that can be invoked in a single request. The default value is
* 0
(or unspecified), which will set the limit to 10000
. When specified, the limit
* value can be between 1
and 10000
. This field will produce a limit error if the
* operation falls out of bounds.
*/
public void setResolverCountLimit(Integer resolverCountLimit) {
this.resolverCountLimit = resolverCountLimit;
}
/**
*
* The maximum number of resolvers that can be invoked in a single request. The default value is 0
(or
* unspecified), which will set the limit to 10000
. When specified, the limit value can be between
* 1
and 10000
. This field will produce a limit error if the operation falls out of
* bounds.
*
*
* @return The maximum number of resolvers that can be invoked in a single request. The default value is
* 0
(or unspecified), which will set the limit to 10000
. When specified, the
* limit value can be between 1
and 10000
. This field will produce a limit error
* if the operation falls out of bounds.
*/
public Integer getResolverCountLimit() {
return this.resolverCountLimit;
}
/**
*
* The maximum number of resolvers that can be invoked in a single request. The default value is 0
(or
* unspecified), which will set the limit to 10000
. When specified, the limit value can be between
* 1
and 10000
. This field will produce a limit error if the operation falls out of
* bounds.
*
*
* @param resolverCountLimit
* The maximum number of resolvers that can be invoked in a single request. The default value is
* 0
(or unspecified), which will set the limit to 10000
. When specified, the limit
* value can be between 1
and 10000
. This field will produce a limit error if the
* operation falls out of bounds.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateGraphqlApiRequest withResolverCountLimit(Integer resolverCountLimit) {
setResolverCountLimit(resolverCountLimit);
return this;
}
/**
*
* The enhancedMetricsConfig
object.
*
*
* @param enhancedMetricsConfig
* The enhancedMetricsConfig
object.
*/
public void setEnhancedMetricsConfig(EnhancedMetricsConfig enhancedMetricsConfig) {
this.enhancedMetricsConfig = enhancedMetricsConfig;
}
/**
*
* The enhancedMetricsConfig
object.
*
*
* @return The enhancedMetricsConfig
object.
*/
public EnhancedMetricsConfig getEnhancedMetricsConfig() {
return this.enhancedMetricsConfig;
}
/**
*
* The enhancedMetricsConfig
object.
*
*
* @param enhancedMetricsConfig
* The enhancedMetricsConfig
object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateGraphqlApiRequest withEnhancedMetricsConfig(EnhancedMetricsConfig enhancedMetricsConfig) {
setEnhancedMetricsConfig(enhancedMetricsConfig);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getLogConfig() != null)
sb.append("LogConfig: ").append(getLogConfig()).append(",");
if (getAuthenticationType() != null)
sb.append("AuthenticationType: ").append(getAuthenticationType()).append(",");
if (getUserPoolConfig() != null)
sb.append("UserPoolConfig: ").append(getUserPoolConfig()).append(",");
if (getOpenIDConnectConfig() != null)
sb.append("OpenIDConnectConfig: ").append(getOpenIDConnectConfig()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getAdditionalAuthenticationProviders() != null)
sb.append("AdditionalAuthenticationProviders: ").append(getAdditionalAuthenticationProviders()).append(",");
if (getXrayEnabled() != null)
sb.append("XrayEnabled: ").append(getXrayEnabled()).append(",");
if (getLambdaAuthorizerConfig() != null)
sb.append("LambdaAuthorizerConfig: ").append(getLambdaAuthorizerConfig()).append(",");
if (getVisibility() != null)
sb.append("Visibility: ").append(getVisibility()).append(",");
if (getApiType() != null)
sb.append("ApiType: ").append(getApiType()).append(",");
if (getMergedApiExecutionRoleArn() != null)
sb.append("MergedApiExecutionRoleArn: ").append(getMergedApiExecutionRoleArn()).append(",");
if (getOwnerContact() != null)
sb.append("OwnerContact: ").append(getOwnerContact()).append(",");
if (getIntrospectionConfig() != null)
sb.append("IntrospectionConfig: ").append(getIntrospectionConfig()).append(",");
if (getQueryDepthLimit() != null)
sb.append("QueryDepthLimit: ").append(getQueryDepthLimit()).append(",");
if (getResolverCountLimit() != null)
sb.append("ResolverCountLimit: ").append(getResolverCountLimit()).append(",");
if (getEnhancedMetricsConfig() != null)
sb.append("EnhancedMetricsConfig: ").append(getEnhancedMetricsConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateGraphqlApiRequest == false)
return false;
CreateGraphqlApiRequest other = (CreateGraphqlApiRequest) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getLogConfig() == null ^ this.getLogConfig() == null)
return false;
if (other.getLogConfig() != null && other.getLogConfig().equals(this.getLogConfig()) == false)
return false;
if (other.getAuthenticationType() == null ^ this.getAuthenticationType() == null)
return false;
if (other.getAuthenticationType() != null && other.getAuthenticationType().equals(this.getAuthenticationType()) == false)
return false;
if (other.getUserPoolConfig() == null ^ this.getUserPoolConfig() == null)
return false;
if (other.getUserPoolConfig() != null && other.getUserPoolConfig().equals(this.getUserPoolConfig()) == false)
return false;
if (other.getOpenIDConnectConfig() == null ^ this.getOpenIDConnectConfig() == null)
return false;
if (other.getOpenIDConnectConfig() != null && other.getOpenIDConnectConfig().equals(this.getOpenIDConnectConfig()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getAdditionalAuthenticationProviders() == null ^ this.getAdditionalAuthenticationProviders() == null)
return false;
if (other.getAdditionalAuthenticationProviders() != null
&& other.getAdditionalAuthenticationProviders().equals(this.getAdditionalAuthenticationProviders()) == false)
return false;
if (other.getXrayEnabled() == null ^ this.getXrayEnabled() == null)
return false;
if (other.getXrayEnabled() != null && other.getXrayEnabled().equals(this.getXrayEnabled()) == false)
return false;
if (other.getLambdaAuthorizerConfig() == null ^ this.getLambdaAuthorizerConfig() == null)
return false;
if (other.getLambdaAuthorizerConfig() != null && other.getLambdaAuthorizerConfig().equals(this.getLambdaAuthorizerConfig()) == false)
return false;
if (other.getVisibility() == null ^ this.getVisibility() == null)
return false;
if (other.getVisibility() != null && other.getVisibility().equals(this.getVisibility()) == false)
return false;
if (other.getApiType() == null ^ this.getApiType() == null)
return false;
if (other.getApiType() != null && other.getApiType().equals(this.getApiType()) == false)
return false;
if (other.getMergedApiExecutionRoleArn() == null ^ this.getMergedApiExecutionRoleArn() == null)
return false;
if (other.getMergedApiExecutionRoleArn() != null && other.getMergedApiExecutionRoleArn().equals(this.getMergedApiExecutionRoleArn()) == false)
return false;
if (other.getOwnerContact() == null ^ this.getOwnerContact() == null)
return false;
if (other.getOwnerContact() != null && other.getOwnerContact().equals(this.getOwnerContact()) == false)
return false;
if (other.getIntrospectionConfig() == null ^ this.getIntrospectionConfig() == null)
return false;
if (other.getIntrospectionConfig() != null && other.getIntrospectionConfig().equals(this.getIntrospectionConfig()) == false)
return false;
if (other.getQueryDepthLimit() == null ^ this.getQueryDepthLimit() == null)
return false;
if (other.getQueryDepthLimit() != null && other.getQueryDepthLimit().equals(this.getQueryDepthLimit()) == false)
return false;
if (other.getResolverCountLimit() == null ^ this.getResolverCountLimit() == null)
return false;
if (other.getResolverCountLimit() != null && other.getResolverCountLimit().equals(this.getResolverCountLimit()) == false)
return false;
if (other.getEnhancedMetricsConfig() == null ^ this.getEnhancedMetricsConfig() == null)
return false;
if (other.getEnhancedMetricsConfig() != null && other.getEnhancedMetricsConfig().equals(this.getEnhancedMetricsConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getLogConfig() == null) ? 0 : getLogConfig().hashCode());
hashCode = prime * hashCode + ((getAuthenticationType() == null) ? 0 : getAuthenticationType().hashCode());
hashCode = prime * hashCode + ((getUserPoolConfig() == null) ? 0 : getUserPoolConfig().hashCode());
hashCode = prime * hashCode + ((getOpenIDConnectConfig() == null) ? 0 : getOpenIDConnectConfig().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getAdditionalAuthenticationProviders() == null) ? 0 : getAdditionalAuthenticationProviders().hashCode());
hashCode = prime * hashCode + ((getXrayEnabled() == null) ? 0 : getXrayEnabled().hashCode());
hashCode = prime * hashCode + ((getLambdaAuthorizerConfig() == null) ? 0 : getLambdaAuthorizerConfig().hashCode());
hashCode = prime * hashCode + ((getVisibility() == null) ? 0 : getVisibility().hashCode());
hashCode = prime * hashCode + ((getApiType() == null) ? 0 : getApiType().hashCode());
hashCode = prime * hashCode + ((getMergedApiExecutionRoleArn() == null) ? 0 : getMergedApiExecutionRoleArn().hashCode());
hashCode = prime * hashCode + ((getOwnerContact() == null) ? 0 : getOwnerContact().hashCode());
hashCode = prime * hashCode + ((getIntrospectionConfig() == null) ? 0 : getIntrospectionConfig().hashCode());
hashCode = prime * hashCode + ((getQueryDepthLimit() == null) ? 0 : getQueryDepthLimit().hashCode());
hashCode = prime * hashCode + ((getResolverCountLimit() == null) ? 0 : getResolverCountLimit().hashCode());
hashCode = prime * hashCode + ((getEnhancedMetricsConfig() == null) ? 0 : getEnhancedMetricsConfig().hashCode());
return hashCode;
}
@Override
public CreateGraphqlApiRequest clone() {
return (CreateGraphqlApiRequest) super.clone();
}
}