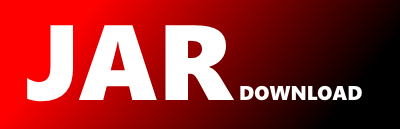
com.amazonaws.services.appsync.model.EnhancedMetricsConfig Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appsync Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appsync.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Enables and controls the enhanced metrics feature. Enhanced metrics emit granular data on API usage and performance
* such as AppSync request and error counts, latency, and cache hits/misses. All enhanced metric data is sent to your
* CloudWatch account, and you can configure the types of data that will be sent.
*
*
* Enhanced metrics can be configured at the resolver, data source, and operation levels.
* EnhancedMetricsConfig
contains three required parameters, each controlling one of these categories:
*
*
* -
*
* resolverLevelMetricsBehavior
: Controls how resolver metrics will be emitted to CloudWatch. Resolver
* metrics include:
*
*
* -
*
* GraphQL errors: The number of GraphQL errors that occurred.
*
*
* -
*
* Requests: The number of invocations that occurred during a request.
*
*
* -
*
* Latency: The time to complete a resolver invocation.
*
*
* -
*
* Cache hits: The number of cache hits during a request.
*
*
* -
*
* Cache misses: The number of cache misses during a request.
*
*
*
*
* These metrics can be emitted to CloudWatch per resolver or for all resolvers in the request. Metrics will be recorded
* by API ID and resolver name. resolverLevelMetricsBehavior
accepts one of these values at a time:
*
*
* -
*
* FULL_REQUEST_RESOLVER_METRICS
: Records and emits metric data for all resolvers in the request.
*
*
* -
*
* PER_RESOLVER_METRICS
: Records and emits metric data for resolvers that have the
* metricsConfig
value set to ENABLED
.
*
*
*
*
* -
*
* dataSourceLevelMetricsBehavior
: Controls how data source metrics will be emitted to CloudWatch. Data
* source metrics include:
*
*
* -
*
* Requests: The number of invocations that occured during a request.
*
*
* -
*
* Latency: The time to complete a data source invocation.
*
*
* -
*
* Errors: The number of errors that occurred during a data source invocation.
*
*
*
*
* These metrics can be emitted to CloudWatch per data source or for all data sources in the request. Metrics will be
* recorded by API ID and data source name. dataSourceLevelMetricsBehavior
accepts one of these values at a
* time:
*
*
* -
*
* FULL_REQUEST_DATA_SOURCE_METRICS
: Records and emits metric data for all data sources in the request.
*
*
* -
*
* PER_DATA_SOURCE_METRICS
: Records and emits metric data for data sources that have the
* metricsConfig
value set to ENABLED
.
*
*
*
*
* -
*
* operationLevelMetricsConfig
: Controls how operation metrics will be emitted to CloudWatch. Operation
* metrics include:
*
*
* -
*
* Requests: The number of times a specified GraphQL operation was called.
*
*
* -
*
* GraphQL errors: The number of GraphQL errors that occurred during a specified GraphQL operation.
*
*
*
*
* Metrics will be recorded by API ID and operation name. You can set the value to ENABLED
or
* DISABLED
.
*
*
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class EnhancedMetricsConfig implements Serializable, Cloneable, StructuredPojo {
/**
*
* Controls how resolver metrics will be emitted to CloudWatch. Resolver metrics include:
*
*
* -
*
* GraphQL errors: The number of GraphQL errors that occurred.
*
*
* -
*
* Requests: The number of invocations that occurred during a request.
*
*
* -
*
* Latency: The time to complete a resolver invocation.
*
*
* -
*
* Cache hits: The number of cache hits during a request.
*
*
* -
*
* Cache misses: The number of cache misses during a request.
*
*
*
*
* These metrics can be emitted to CloudWatch per resolver or for all resolvers in the request. Metrics will be
* recorded by API ID and resolver name. resolverLevelMetricsBehavior
accepts one of these values at a
* time:
*
*
* -
*
* FULL_REQUEST_RESOLVER_METRICS
: Records and emits metric data for all resolvers in the request.
*
*
* -
*
* PER_RESOLVER_METRICS
: Records and emits metric data for resolvers that have the
* metricsConfig
value set to ENABLED
.
*
*
*
*/
private String resolverLevelMetricsBehavior;
/**
*
* Controls how data source metrics will be emitted to CloudWatch. Data source metrics include:
*
*
* -
*
* Requests: The number of invocations that occured during a request.
*
*
* -
*
* Latency: The time to complete a data source invocation.
*
*
* -
*
* Errors: The number of errors that occurred during a data source invocation.
*
*
*
*
* These metrics can be emitted to CloudWatch per data source or for all data sources in the request. Metrics will
* be recorded by API ID and data source name. dataSourceLevelMetricsBehavior
accepts one of these
* values at a time:
*
*
* -
*
* FULL_REQUEST_DATA_SOURCE_METRICS
: Records and emits metric data for all data sources in the request.
*
*
* -
*
* PER_DATA_SOURCE_METRICS
: Records and emits metric data for data sources that have the
* metricsConfig
value set to ENABLED
.
*
*
*
*/
private String dataSourceLevelMetricsBehavior;
/**
*
* Controls how operation metrics will be emitted to CloudWatch. Operation metrics include:
*
*
* -
*
* Requests: The number of times a specified GraphQL operation was called.
*
*
* -
*
* GraphQL errors: The number of GraphQL errors that occurred during a specified GraphQL operation.
*
*
*
*
* Metrics will be recorded by API ID and operation name. You can set the value to ENABLED
or
* DISABLED
.
*
*/
private String operationLevelMetricsConfig;
/**
*
* Controls how resolver metrics will be emitted to CloudWatch. Resolver metrics include:
*
*
* -
*
* GraphQL errors: The number of GraphQL errors that occurred.
*
*
* -
*
* Requests: The number of invocations that occurred during a request.
*
*
* -
*
* Latency: The time to complete a resolver invocation.
*
*
* -
*
* Cache hits: The number of cache hits during a request.
*
*
* -
*
* Cache misses: The number of cache misses during a request.
*
*
*
*
* These metrics can be emitted to CloudWatch per resolver or for all resolvers in the request. Metrics will be
* recorded by API ID and resolver name. resolverLevelMetricsBehavior
accepts one of these values at a
* time:
*
*
* -
*
* FULL_REQUEST_RESOLVER_METRICS
: Records and emits metric data for all resolvers in the request.
*
*
* -
*
* PER_RESOLVER_METRICS
: Records and emits metric data for resolvers that have the
* metricsConfig
value set to ENABLED
.
*
*
*
*
* @param resolverLevelMetricsBehavior
* Controls how resolver metrics will be emitted to CloudWatch. Resolver metrics include:
*
* -
*
* GraphQL errors: The number of GraphQL errors that occurred.
*
*
* -
*
* Requests: The number of invocations that occurred during a request.
*
*
* -
*
* Latency: The time to complete a resolver invocation.
*
*
* -
*
* Cache hits: The number of cache hits during a request.
*
*
* -
*
* Cache misses: The number of cache misses during a request.
*
*
*
*
* These metrics can be emitted to CloudWatch per resolver or for all resolvers in the request. Metrics will
* be recorded by API ID and resolver name. resolverLevelMetricsBehavior
accepts one of these
* values at a time:
*
*
* -
*
* FULL_REQUEST_RESOLVER_METRICS
: Records and emits metric data for all resolvers in the
* request.
*
*
* -
*
* PER_RESOLVER_METRICS
: Records and emits metric data for resolvers that have the
* metricsConfig
value set to ENABLED
.
*
*
* @see ResolverLevelMetricsBehavior
*/
public void setResolverLevelMetricsBehavior(String resolverLevelMetricsBehavior) {
this.resolverLevelMetricsBehavior = resolverLevelMetricsBehavior;
}
/**
*
* Controls how resolver metrics will be emitted to CloudWatch. Resolver metrics include:
*
*
* -
*
* GraphQL errors: The number of GraphQL errors that occurred.
*
*
* -
*
* Requests: The number of invocations that occurred during a request.
*
*
* -
*
* Latency: The time to complete a resolver invocation.
*
*
* -
*
* Cache hits: The number of cache hits during a request.
*
*
* -
*
* Cache misses: The number of cache misses during a request.
*
*
*
*
* These metrics can be emitted to CloudWatch per resolver or for all resolvers in the request. Metrics will be
* recorded by API ID and resolver name. resolverLevelMetricsBehavior
accepts one of these values at a
* time:
*
*
* -
*
* FULL_REQUEST_RESOLVER_METRICS
: Records and emits metric data for all resolvers in the request.
*
*
* -
*
* PER_RESOLVER_METRICS
: Records and emits metric data for resolvers that have the
* metricsConfig
value set to ENABLED
.
*
*
*
*
* @return Controls how resolver metrics will be emitted to CloudWatch. Resolver metrics include:
*
* -
*
* GraphQL errors: The number of GraphQL errors that occurred.
*
*
* -
*
* Requests: The number of invocations that occurred during a request.
*
*
* -
*
* Latency: The time to complete a resolver invocation.
*
*
* -
*
* Cache hits: The number of cache hits during a request.
*
*
* -
*
* Cache misses: The number of cache misses during a request.
*
*
*
*
* These metrics can be emitted to CloudWatch per resolver or for all resolvers in the request. Metrics will
* be recorded by API ID and resolver name. resolverLevelMetricsBehavior
accepts one of these
* values at a time:
*
*
* -
*
* FULL_REQUEST_RESOLVER_METRICS
: Records and emits metric data for all resolvers in the
* request.
*
*
* -
*
* PER_RESOLVER_METRICS
: Records and emits metric data for resolvers that have the
* metricsConfig
value set to ENABLED
.
*
*
* @see ResolverLevelMetricsBehavior
*/
public String getResolverLevelMetricsBehavior() {
return this.resolverLevelMetricsBehavior;
}
/**
*
* Controls how resolver metrics will be emitted to CloudWatch. Resolver metrics include:
*
*
* -
*
* GraphQL errors: The number of GraphQL errors that occurred.
*
*
* -
*
* Requests: The number of invocations that occurred during a request.
*
*
* -
*
* Latency: The time to complete a resolver invocation.
*
*
* -
*
* Cache hits: The number of cache hits during a request.
*
*
* -
*
* Cache misses: The number of cache misses during a request.
*
*
*
*
* These metrics can be emitted to CloudWatch per resolver or for all resolvers in the request. Metrics will be
* recorded by API ID and resolver name. resolverLevelMetricsBehavior
accepts one of these values at a
* time:
*
*
* -
*
* FULL_REQUEST_RESOLVER_METRICS
: Records and emits metric data for all resolvers in the request.
*
*
* -
*
* PER_RESOLVER_METRICS
: Records and emits metric data for resolvers that have the
* metricsConfig
value set to ENABLED
.
*
*
*
*
* @param resolverLevelMetricsBehavior
* Controls how resolver metrics will be emitted to CloudWatch. Resolver metrics include:
*
* -
*
* GraphQL errors: The number of GraphQL errors that occurred.
*
*
* -
*
* Requests: The number of invocations that occurred during a request.
*
*
* -
*
* Latency: The time to complete a resolver invocation.
*
*
* -
*
* Cache hits: The number of cache hits during a request.
*
*
* -
*
* Cache misses: The number of cache misses during a request.
*
*
*
*
* These metrics can be emitted to CloudWatch per resolver or for all resolvers in the request. Metrics will
* be recorded by API ID and resolver name. resolverLevelMetricsBehavior
accepts one of these
* values at a time:
*
*
* -
*
* FULL_REQUEST_RESOLVER_METRICS
: Records and emits metric data for all resolvers in the
* request.
*
*
* -
*
* PER_RESOLVER_METRICS
: Records and emits metric data for resolvers that have the
* metricsConfig
value set to ENABLED
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResolverLevelMetricsBehavior
*/
public EnhancedMetricsConfig withResolverLevelMetricsBehavior(String resolverLevelMetricsBehavior) {
setResolverLevelMetricsBehavior(resolverLevelMetricsBehavior);
return this;
}
/**
*
* Controls how resolver metrics will be emitted to CloudWatch. Resolver metrics include:
*
*
* -
*
* GraphQL errors: The number of GraphQL errors that occurred.
*
*
* -
*
* Requests: The number of invocations that occurred during a request.
*
*
* -
*
* Latency: The time to complete a resolver invocation.
*
*
* -
*
* Cache hits: The number of cache hits during a request.
*
*
* -
*
* Cache misses: The number of cache misses during a request.
*
*
*
*
* These metrics can be emitted to CloudWatch per resolver or for all resolvers in the request. Metrics will be
* recorded by API ID and resolver name. resolverLevelMetricsBehavior
accepts one of these values at a
* time:
*
*
* -
*
* FULL_REQUEST_RESOLVER_METRICS
: Records and emits metric data for all resolvers in the request.
*
*
* -
*
* PER_RESOLVER_METRICS
: Records and emits metric data for resolvers that have the
* metricsConfig
value set to ENABLED
.
*
*
*
*
* @param resolverLevelMetricsBehavior
* Controls how resolver metrics will be emitted to CloudWatch. Resolver metrics include:
*
* -
*
* GraphQL errors: The number of GraphQL errors that occurred.
*
*
* -
*
* Requests: The number of invocations that occurred during a request.
*
*
* -
*
* Latency: The time to complete a resolver invocation.
*
*
* -
*
* Cache hits: The number of cache hits during a request.
*
*
* -
*
* Cache misses: The number of cache misses during a request.
*
*
*
*
* These metrics can be emitted to CloudWatch per resolver or for all resolvers in the request. Metrics will
* be recorded by API ID and resolver name. resolverLevelMetricsBehavior
accepts one of these
* values at a time:
*
*
* -
*
* FULL_REQUEST_RESOLVER_METRICS
: Records and emits metric data for all resolvers in the
* request.
*
*
* -
*
* PER_RESOLVER_METRICS
: Records and emits metric data for resolvers that have the
* metricsConfig
value set to ENABLED
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResolverLevelMetricsBehavior
*/
public EnhancedMetricsConfig withResolverLevelMetricsBehavior(ResolverLevelMetricsBehavior resolverLevelMetricsBehavior) {
this.resolverLevelMetricsBehavior = resolverLevelMetricsBehavior.toString();
return this;
}
/**
*
* Controls how data source metrics will be emitted to CloudWatch. Data source metrics include:
*
*
* -
*
* Requests: The number of invocations that occured during a request.
*
*
* -
*
* Latency: The time to complete a data source invocation.
*
*
* -
*
* Errors: The number of errors that occurred during a data source invocation.
*
*
*
*
* These metrics can be emitted to CloudWatch per data source or for all data sources in the request. Metrics will
* be recorded by API ID and data source name. dataSourceLevelMetricsBehavior
accepts one of these
* values at a time:
*
*
* -
*
* FULL_REQUEST_DATA_SOURCE_METRICS
: Records and emits metric data for all data sources in the request.
*
*
* -
*
* PER_DATA_SOURCE_METRICS
: Records and emits metric data for data sources that have the
* metricsConfig
value set to ENABLED
.
*
*
*
*
* @param dataSourceLevelMetricsBehavior
* Controls how data source metrics will be emitted to CloudWatch. Data source metrics include:
*
* -
*
* Requests: The number of invocations that occured during a request.
*
*
* -
*
* Latency: The time to complete a data source invocation.
*
*
* -
*
* Errors: The number of errors that occurred during a data source invocation.
*
*
*
*
* These metrics can be emitted to CloudWatch per data source or for all data sources in the request. Metrics
* will be recorded by API ID and data source name. dataSourceLevelMetricsBehavior
accepts one
* of these values at a time:
*
*
* -
*
* FULL_REQUEST_DATA_SOURCE_METRICS
: Records and emits metric data for all data sources in the
* request.
*
*
* -
*
* PER_DATA_SOURCE_METRICS
: Records and emits metric data for data sources that have the
* metricsConfig
value set to ENABLED
.
*
*
* @see DataSourceLevelMetricsBehavior
*/
public void setDataSourceLevelMetricsBehavior(String dataSourceLevelMetricsBehavior) {
this.dataSourceLevelMetricsBehavior = dataSourceLevelMetricsBehavior;
}
/**
*
* Controls how data source metrics will be emitted to CloudWatch. Data source metrics include:
*
*
* -
*
* Requests: The number of invocations that occured during a request.
*
*
* -
*
* Latency: The time to complete a data source invocation.
*
*
* -
*
* Errors: The number of errors that occurred during a data source invocation.
*
*
*
*
* These metrics can be emitted to CloudWatch per data source or for all data sources in the request. Metrics will
* be recorded by API ID and data source name. dataSourceLevelMetricsBehavior
accepts one of these
* values at a time:
*
*
* -
*
* FULL_REQUEST_DATA_SOURCE_METRICS
: Records and emits metric data for all data sources in the request.
*
*
* -
*
* PER_DATA_SOURCE_METRICS
: Records and emits metric data for data sources that have the
* metricsConfig
value set to ENABLED
.
*
*
*
*
* @return Controls how data source metrics will be emitted to CloudWatch. Data source metrics include:
*
* -
*
* Requests: The number of invocations that occured during a request.
*
*
* -
*
* Latency: The time to complete a data source invocation.
*
*
* -
*
* Errors: The number of errors that occurred during a data source invocation.
*
*
*
*
* These metrics can be emitted to CloudWatch per data source or for all data sources in the request.
* Metrics will be recorded by API ID and data source name. dataSourceLevelMetricsBehavior
* accepts one of these values at a time:
*
*
* -
*
* FULL_REQUEST_DATA_SOURCE_METRICS
: Records and emits metric data for all data sources in the
* request.
*
*
* -
*
* PER_DATA_SOURCE_METRICS
: Records and emits metric data for data sources that have the
* metricsConfig
value set to ENABLED
.
*
*
* @see DataSourceLevelMetricsBehavior
*/
public String getDataSourceLevelMetricsBehavior() {
return this.dataSourceLevelMetricsBehavior;
}
/**
*
* Controls how data source metrics will be emitted to CloudWatch. Data source metrics include:
*
*
* -
*
* Requests: The number of invocations that occured during a request.
*
*
* -
*
* Latency: The time to complete a data source invocation.
*
*
* -
*
* Errors: The number of errors that occurred during a data source invocation.
*
*
*
*
* These metrics can be emitted to CloudWatch per data source or for all data sources in the request. Metrics will
* be recorded by API ID and data source name. dataSourceLevelMetricsBehavior
accepts one of these
* values at a time:
*
*
* -
*
* FULL_REQUEST_DATA_SOURCE_METRICS
: Records and emits metric data for all data sources in the request.
*
*
* -
*
* PER_DATA_SOURCE_METRICS
: Records and emits metric data for data sources that have the
* metricsConfig
value set to ENABLED
.
*
*
*
*
* @param dataSourceLevelMetricsBehavior
* Controls how data source metrics will be emitted to CloudWatch. Data source metrics include:
*
* -
*
* Requests: The number of invocations that occured during a request.
*
*
* -
*
* Latency: The time to complete a data source invocation.
*
*
* -
*
* Errors: The number of errors that occurred during a data source invocation.
*
*
*
*
* These metrics can be emitted to CloudWatch per data source or for all data sources in the request. Metrics
* will be recorded by API ID and data source name. dataSourceLevelMetricsBehavior
accepts one
* of these values at a time:
*
*
* -
*
* FULL_REQUEST_DATA_SOURCE_METRICS
: Records and emits metric data for all data sources in the
* request.
*
*
* -
*
* PER_DATA_SOURCE_METRICS
: Records and emits metric data for data sources that have the
* metricsConfig
value set to ENABLED
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataSourceLevelMetricsBehavior
*/
public EnhancedMetricsConfig withDataSourceLevelMetricsBehavior(String dataSourceLevelMetricsBehavior) {
setDataSourceLevelMetricsBehavior(dataSourceLevelMetricsBehavior);
return this;
}
/**
*
* Controls how data source metrics will be emitted to CloudWatch. Data source metrics include:
*
*
* -
*
* Requests: The number of invocations that occured during a request.
*
*
* -
*
* Latency: The time to complete a data source invocation.
*
*
* -
*
* Errors: The number of errors that occurred during a data source invocation.
*
*
*
*
* These metrics can be emitted to CloudWatch per data source or for all data sources in the request. Metrics will
* be recorded by API ID and data source name. dataSourceLevelMetricsBehavior
accepts one of these
* values at a time:
*
*
* -
*
* FULL_REQUEST_DATA_SOURCE_METRICS
: Records and emits metric data for all data sources in the request.
*
*
* -
*
* PER_DATA_SOURCE_METRICS
: Records and emits metric data for data sources that have the
* metricsConfig
value set to ENABLED
.
*
*
*
*
* @param dataSourceLevelMetricsBehavior
* Controls how data source metrics will be emitted to CloudWatch. Data source metrics include:
*
* -
*
* Requests: The number of invocations that occured during a request.
*
*
* -
*
* Latency: The time to complete a data source invocation.
*
*
* -
*
* Errors: The number of errors that occurred during a data source invocation.
*
*
*
*
* These metrics can be emitted to CloudWatch per data source or for all data sources in the request. Metrics
* will be recorded by API ID and data source name. dataSourceLevelMetricsBehavior
accepts one
* of these values at a time:
*
*
* -
*
* FULL_REQUEST_DATA_SOURCE_METRICS
: Records and emits metric data for all data sources in the
* request.
*
*
* -
*
* PER_DATA_SOURCE_METRICS
: Records and emits metric data for data sources that have the
* metricsConfig
value set to ENABLED
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataSourceLevelMetricsBehavior
*/
public EnhancedMetricsConfig withDataSourceLevelMetricsBehavior(DataSourceLevelMetricsBehavior dataSourceLevelMetricsBehavior) {
this.dataSourceLevelMetricsBehavior = dataSourceLevelMetricsBehavior.toString();
return this;
}
/**
*
* Controls how operation metrics will be emitted to CloudWatch. Operation metrics include:
*
*
* -
*
* Requests: The number of times a specified GraphQL operation was called.
*
*
* -
*
* GraphQL errors: The number of GraphQL errors that occurred during a specified GraphQL operation.
*
*
*
*
* Metrics will be recorded by API ID and operation name. You can set the value to ENABLED
or
* DISABLED
.
*
*
* @param operationLevelMetricsConfig
* Controls how operation metrics will be emitted to CloudWatch. Operation metrics include:
*
* -
*
* Requests: The number of times a specified GraphQL operation was called.
*
*
* -
*
* GraphQL errors: The number of GraphQL errors that occurred during a specified GraphQL operation.
*
*
*
*
* Metrics will be recorded by API ID and operation name. You can set the value to ENABLED
or
* DISABLED
.
* @see OperationLevelMetricsConfig
*/
public void setOperationLevelMetricsConfig(String operationLevelMetricsConfig) {
this.operationLevelMetricsConfig = operationLevelMetricsConfig;
}
/**
*
* Controls how operation metrics will be emitted to CloudWatch. Operation metrics include:
*
*
* -
*
* Requests: The number of times a specified GraphQL operation was called.
*
*
* -
*
* GraphQL errors: The number of GraphQL errors that occurred during a specified GraphQL operation.
*
*
*
*
* Metrics will be recorded by API ID and operation name. You can set the value to ENABLED
or
* DISABLED
.
*
*
* @return Controls how operation metrics will be emitted to CloudWatch. Operation metrics include:
*
* -
*
* Requests: The number of times a specified GraphQL operation was called.
*
*
* -
*
* GraphQL errors: The number of GraphQL errors that occurred during a specified GraphQL operation.
*
*
*
*
* Metrics will be recorded by API ID and operation name. You can set the value to ENABLED
or
* DISABLED
.
* @see OperationLevelMetricsConfig
*/
public String getOperationLevelMetricsConfig() {
return this.operationLevelMetricsConfig;
}
/**
*
* Controls how operation metrics will be emitted to CloudWatch. Operation metrics include:
*
*
* -
*
* Requests: The number of times a specified GraphQL operation was called.
*
*
* -
*
* GraphQL errors: The number of GraphQL errors that occurred during a specified GraphQL operation.
*
*
*
*
* Metrics will be recorded by API ID and operation name. You can set the value to ENABLED
or
* DISABLED
.
*
*
* @param operationLevelMetricsConfig
* Controls how operation metrics will be emitted to CloudWatch. Operation metrics include:
*
* -
*
* Requests: The number of times a specified GraphQL operation was called.
*
*
* -
*
* GraphQL errors: The number of GraphQL errors that occurred during a specified GraphQL operation.
*
*
*
*
* Metrics will be recorded by API ID and operation name. You can set the value to ENABLED
or
* DISABLED
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see OperationLevelMetricsConfig
*/
public EnhancedMetricsConfig withOperationLevelMetricsConfig(String operationLevelMetricsConfig) {
setOperationLevelMetricsConfig(operationLevelMetricsConfig);
return this;
}
/**
*
* Controls how operation metrics will be emitted to CloudWatch. Operation metrics include:
*
*
* -
*
* Requests: The number of times a specified GraphQL operation was called.
*
*
* -
*
* GraphQL errors: The number of GraphQL errors that occurred during a specified GraphQL operation.
*
*
*
*
* Metrics will be recorded by API ID and operation name. You can set the value to ENABLED
or
* DISABLED
.
*
*
* @param operationLevelMetricsConfig
* Controls how operation metrics will be emitted to CloudWatch. Operation metrics include:
*
* -
*
* Requests: The number of times a specified GraphQL operation was called.
*
*
* -
*
* GraphQL errors: The number of GraphQL errors that occurred during a specified GraphQL operation.
*
*
*
*
* Metrics will be recorded by API ID and operation name. You can set the value to ENABLED
or
* DISABLED
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see OperationLevelMetricsConfig
*/
public EnhancedMetricsConfig withOperationLevelMetricsConfig(OperationLevelMetricsConfig operationLevelMetricsConfig) {
this.operationLevelMetricsConfig = operationLevelMetricsConfig.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getResolverLevelMetricsBehavior() != null)
sb.append("ResolverLevelMetricsBehavior: ").append(getResolverLevelMetricsBehavior()).append(",");
if (getDataSourceLevelMetricsBehavior() != null)
sb.append("DataSourceLevelMetricsBehavior: ").append(getDataSourceLevelMetricsBehavior()).append(",");
if (getOperationLevelMetricsConfig() != null)
sb.append("OperationLevelMetricsConfig: ").append(getOperationLevelMetricsConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof EnhancedMetricsConfig == false)
return false;
EnhancedMetricsConfig other = (EnhancedMetricsConfig) obj;
if (other.getResolverLevelMetricsBehavior() == null ^ this.getResolverLevelMetricsBehavior() == null)
return false;
if (other.getResolverLevelMetricsBehavior() != null && other.getResolverLevelMetricsBehavior().equals(this.getResolverLevelMetricsBehavior()) == false)
return false;
if (other.getDataSourceLevelMetricsBehavior() == null ^ this.getDataSourceLevelMetricsBehavior() == null)
return false;
if (other.getDataSourceLevelMetricsBehavior() != null
&& other.getDataSourceLevelMetricsBehavior().equals(this.getDataSourceLevelMetricsBehavior()) == false)
return false;
if (other.getOperationLevelMetricsConfig() == null ^ this.getOperationLevelMetricsConfig() == null)
return false;
if (other.getOperationLevelMetricsConfig() != null && other.getOperationLevelMetricsConfig().equals(this.getOperationLevelMetricsConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getResolverLevelMetricsBehavior() == null) ? 0 : getResolverLevelMetricsBehavior().hashCode());
hashCode = prime * hashCode + ((getDataSourceLevelMetricsBehavior() == null) ? 0 : getDataSourceLevelMetricsBehavior().hashCode());
hashCode = prime * hashCode + ((getOperationLevelMetricsConfig() == null) ? 0 : getOperationLevelMetricsConfig().hashCode());
return hashCode;
}
@Override
public EnhancedMetricsConfig clone() {
try {
return (EnhancedMetricsConfig) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.appsync.model.transform.EnhancedMetricsConfigMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}