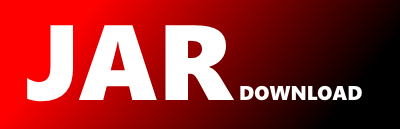
com.amazonaws.services.appsync.model.SourceApiAssociation Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appsync Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appsync.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes the configuration of a source API. A source API is a GraphQL API that is linked to a merged API. There can
* be multiple source APIs attached to each merged API. When linked to a merged API, the source API's schema, data
* sources, and resolvers will be combined with other linked source API data to form a new, singular API.
*
*
* Source APIs can originate from your account or from other accounts via Amazon Web Services Resource Access Manager.
* For more information about sharing resources from other accounts, see What is Amazon Web Services Resource Access
* Manager? in the Amazon Web Services Resource Access Manager guide.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class SourceApiAssociation implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ID generated by the AppSync service for the source API association.
*
*/
private String associationId;
/**
*
* The Amazon Resource Name (ARN) of the source API association.
*
*/
private String associationArn;
/**
*
* The ID of the AppSync source API.
*
*/
private String sourceApiId;
/**
*
* The Amazon Resource Name (ARN) of the AppSync source API.
*
*/
private String sourceApiArn;
/**
*
* The Amazon Resource Name (ARN) of the AppSync Merged API.
*
*/
private String mergedApiArn;
/**
*
* The ID of the AppSync Merged API.
*
*/
private String mergedApiId;
/**
*
* The description field.
*
*/
private String description;
/**
*
* The SourceApiAssociationConfig
object data.
*
*/
private SourceApiAssociationConfig sourceApiAssociationConfig;
/**
*
* The state of the source API association.
*
*/
private String sourceApiAssociationStatus;
/**
*
* The detailed message related to the current state of the source API association.
*
*/
private String sourceApiAssociationStatusDetail;
/**
*
* The datetime value of the last successful merge of the source API association. The result will be in UTC format
* and your local time zone.
*
*/
private java.util.Date lastSuccessfulMergeDate;
/**
*
* The ID generated by the AppSync service for the source API association.
*
*
* @param associationId
* The ID generated by the AppSync service for the source API association.
*/
public void setAssociationId(String associationId) {
this.associationId = associationId;
}
/**
*
* The ID generated by the AppSync service for the source API association.
*
*
* @return The ID generated by the AppSync service for the source API association.
*/
public String getAssociationId() {
return this.associationId;
}
/**
*
* The ID generated by the AppSync service for the source API association.
*
*
* @param associationId
* The ID generated by the AppSync service for the source API association.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SourceApiAssociation withAssociationId(String associationId) {
setAssociationId(associationId);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the source API association.
*
*
* @param associationArn
* The Amazon Resource Name (ARN) of the source API association.
*/
public void setAssociationArn(String associationArn) {
this.associationArn = associationArn;
}
/**
*
* The Amazon Resource Name (ARN) of the source API association.
*
*
* @return The Amazon Resource Name (ARN) of the source API association.
*/
public String getAssociationArn() {
return this.associationArn;
}
/**
*
* The Amazon Resource Name (ARN) of the source API association.
*
*
* @param associationArn
* The Amazon Resource Name (ARN) of the source API association.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SourceApiAssociation withAssociationArn(String associationArn) {
setAssociationArn(associationArn);
return this;
}
/**
*
* The ID of the AppSync source API.
*
*
* @param sourceApiId
* The ID of the AppSync source API.
*/
public void setSourceApiId(String sourceApiId) {
this.sourceApiId = sourceApiId;
}
/**
*
* The ID of the AppSync source API.
*
*
* @return The ID of the AppSync source API.
*/
public String getSourceApiId() {
return this.sourceApiId;
}
/**
*
* The ID of the AppSync source API.
*
*
* @param sourceApiId
* The ID of the AppSync source API.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SourceApiAssociation withSourceApiId(String sourceApiId) {
setSourceApiId(sourceApiId);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the AppSync source API.
*
*
* @param sourceApiArn
* The Amazon Resource Name (ARN) of the AppSync source API.
*/
public void setSourceApiArn(String sourceApiArn) {
this.sourceApiArn = sourceApiArn;
}
/**
*
* The Amazon Resource Name (ARN) of the AppSync source API.
*
*
* @return The Amazon Resource Name (ARN) of the AppSync source API.
*/
public String getSourceApiArn() {
return this.sourceApiArn;
}
/**
*
* The Amazon Resource Name (ARN) of the AppSync source API.
*
*
* @param sourceApiArn
* The Amazon Resource Name (ARN) of the AppSync source API.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SourceApiAssociation withSourceApiArn(String sourceApiArn) {
setSourceApiArn(sourceApiArn);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the AppSync Merged API.
*
*
* @param mergedApiArn
* The Amazon Resource Name (ARN) of the AppSync Merged API.
*/
public void setMergedApiArn(String mergedApiArn) {
this.mergedApiArn = mergedApiArn;
}
/**
*
* The Amazon Resource Name (ARN) of the AppSync Merged API.
*
*
* @return The Amazon Resource Name (ARN) of the AppSync Merged API.
*/
public String getMergedApiArn() {
return this.mergedApiArn;
}
/**
*
* The Amazon Resource Name (ARN) of the AppSync Merged API.
*
*
* @param mergedApiArn
* The Amazon Resource Name (ARN) of the AppSync Merged API.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SourceApiAssociation withMergedApiArn(String mergedApiArn) {
setMergedApiArn(mergedApiArn);
return this;
}
/**
*
* The ID of the AppSync Merged API.
*
*
* @param mergedApiId
* The ID of the AppSync Merged API.
*/
public void setMergedApiId(String mergedApiId) {
this.mergedApiId = mergedApiId;
}
/**
*
* The ID of the AppSync Merged API.
*
*
* @return The ID of the AppSync Merged API.
*/
public String getMergedApiId() {
return this.mergedApiId;
}
/**
*
* The ID of the AppSync Merged API.
*
*
* @param mergedApiId
* The ID of the AppSync Merged API.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SourceApiAssociation withMergedApiId(String mergedApiId) {
setMergedApiId(mergedApiId);
return this;
}
/**
*
* The description field.
*
*
* @param description
* The description field.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The description field.
*
*
* @return The description field.
*/
public String getDescription() {
return this.description;
}
/**
*
* The description field.
*
*
* @param description
* The description field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SourceApiAssociation withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The SourceApiAssociationConfig
object data.
*
*
* @param sourceApiAssociationConfig
* The SourceApiAssociationConfig
object data.
*/
public void setSourceApiAssociationConfig(SourceApiAssociationConfig sourceApiAssociationConfig) {
this.sourceApiAssociationConfig = sourceApiAssociationConfig;
}
/**
*
* The SourceApiAssociationConfig
object data.
*
*
* @return The SourceApiAssociationConfig
object data.
*/
public SourceApiAssociationConfig getSourceApiAssociationConfig() {
return this.sourceApiAssociationConfig;
}
/**
*
* The SourceApiAssociationConfig
object data.
*
*
* @param sourceApiAssociationConfig
* The SourceApiAssociationConfig
object data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SourceApiAssociation withSourceApiAssociationConfig(SourceApiAssociationConfig sourceApiAssociationConfig) {
setSourceApiAssociationConfig(sourceApiAssociationConfig);
return this;
}
/**
*
* The state of the source API association.
*
*
* @param sourceApiAssociationStatus
* The state of the source API association.
* @see SourceApiAssociationStatus
*/
public void setSourceApiAssociationStatus(String sourceApiAssociationStatus) {
this.sourceApiAssociationStatus = sourceApiAssociationStatus;
}
/**
*
* The state of the source API association.
*
*
* @return The state of the source API association.
* @see SourceApiAssociationStatus
*/
public String getSourceApiAssociationStatus() {
return this.sourceApiAssociationStatus;
}
/**
*
* The state of the source API association.
*
*
* @param sourceApiAssociationStatus
* The state of the source API association.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SourceApiAssociationStatus
*/
public SourceApiAssociation withSourceApiAssociationStatus(String sourceApiAssociationStatus) {
setSourceApiAssociationStatus(sourceApiAssociationStatus);
return this;
}
/**
*
* The state of the source API association.
*
*
* @param sourceApiAssociationStatus
* The state of the source API association.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SourceApiAssociationStatus
*/
public SourceApiAssociation withSourceApiAssociationStatus(SourceApiAssociationStatus sourceApiAssociationStatus) {
this.sourceApiAssociationStatus = sourceApiAssociationStatus.toString();
return this;
}
/**
*
* The detailed message related to the current state of the source API association.
*
*
* @param sourceApiAssociationStatusDetail
* The detailed message related to the current state of the source API association.
*/
public void setSourceApiAssociationStatusDetail(String sourceApiAssociationStatusDetail) {
this.sourceApiAssociationStatusDetail = sourceApiAssociationStatusDetail;
}
/**
*
* The detailed message related to the current state of the source API association.
*
*
* @return The detailed message related to the current state of the source API association.
*/
public String getSourceApiAssociationStatusDetail() {
return this.sourceApiAssociationStatusDetail;
}
/**
*
* The detailed message related to the current state of the source API association.
*
*
* @param sourceApiAssociationStatusDetail
* The detailed message related to the current state of the source API association.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SourceApiAssociation withSourceApiAssociationStatusDetail(String sourceApiAssociationStatusDetail) {
setSourceApiAssociationStatusDetail(sourceApiAssociationStatusDetail);
return this;
}
/**
*
* The datetime value of the last successful merge of the source API association. The result will be in UTC format
* and your local time zone.
*
*
* @param lastSuccessfulMergeDate
* The datetime value of the last successful merge of the source API association. The result will be in UTC
* format and your local time zone.
*/
public void setLastSuccessfulMergeDate(java.util.Date lastSuccessfulMergeDate) {
this.lastSuccessfulMergeDate = lastSuccessfulMergeDate;
}
/**
*
* The datetime value of the last successful merge of the source API association. The result will be in UTC format
* and your local time zone.
*
*
* @return The datetime value of the last successful merge of the source API association. The result will be in UTC
* format and your local time zone.
*/
public java.util.Date getLastSuccessfulMergeDate() {
return this.lastSuccessfulMergeDate;
}
/**
*
* The datetime value of the last successful merge of the source API association. The result will be in UTC format
* and your local time zone.
*
*
* @param lastSuccessfulMergeDate
* The datetime value of the last successful merge of the source API association. The result will be in UTC
* format and your local time zone.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SourceApiAssociation withLastSuccessfulMergeDate(java.util.Date lastSuccessfulMergeDate) {
setLastSuccessfulMergeDate(lastSuccessfulMergeDate);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAssociationId() != null)
sb.append("AssociationId: ").append(getAssociationId()).append(",");
if (getAssociationArn() != null)
sb.append("AssociationArn: ").append(getAssociationArn()).append(",");
if (getSourceApiId() != null)
sb.append("SourceApiId: ").append(getSourceApiId()).append(",");
if (getSourceApiArn() != null)
sb.append("SourceApiArn: ").append(getSourceApiArn()).append(",");
if (getMergedApiArn() != null)
sb.append("MergedApiArn: ").append(getMergedApiArn()).append(",");
if (getMergedApiId() != null)
sb.append("MergedApiId: ").append(getMergedApiId()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getSourceApiAssociationConfig() != null)
sb.append("SourceApiAssociationConfig: ").append(getSourceApiAssociationConfig()).append(",");
if (getSourceApiAssociationStatus() != null)
sb.append("SourceApiAssociationStatus: ").append(getSourceApiAssociationStatus()).append(",");
if (getSourceApiAssociationStatusDetail() != null)
sb.append("SourceApiAssociationStatusDetail: ").append(getSourceApiAssociationStatusDetail()).append(",");
if (getLastSuccessfulMergeDate() != null)
sb.append("LastSuccessfulMergeDate: ").append(getLastSuccessfulMergeDate());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof SourceApiAssociation == false)
return false;
SourceApiAssociation other = (SourceApiAssociation) obj;
if (other.getAssociationId() == null ^ this.getAssociationId() == null)
return false;
if (other.getAssociationId() != null && other.getAssociationId().equals(this.getAssociationId()) == false)
return false;
if (other.getAssociationArn() == null ^ this.getAssociationArn() == null)
return false;
if (other.getAssociationArn() != null && other.getAssociationArn().equals(this.getAssociationArn()) == false)
return false;
if (other.getSourceApiId() == null ^ this.getSourceApiId() == null)
return false;
if (other.getSourceApiId() != null && other.getSourceApiId().equals(this.getSourceApiId()) == false)
return false;
if (other.getSourceApiArn() == null ^ this.getSourceApiArn() == null)
return false;
if (other.getSourceApiArn() != null && other.getSourceApiArn().equals(this.getSourceApiArn()) == false)
return false;
if (other.getMergedApiArn() == null ^ this.getMergedApiArn() == null)
return false;
if (other.getMergedApiArn() != null && other.getMergedApiArn().equals(this.getMergedApiArn()) == false)
return false;
if (other.getMergedApiId() == null ^ this.getMergedApiId() == null)
return false;
if (other.getMergedApiId() != null && other.getMergedApiId().equals(this.getMergedApiId()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getSourceApiAssociationConfig() == null ^ this.getSourceApiAssociationConfig() == null)
return false;
if (other.getSourceApiAssociationConfig() != null && other.getSourceApiAssociationConfig().equals(this.getSourceApiAssociationConfig()) == false)
return false;
if (other.getSourceApiAssociationStatus() == null ^ this.getSourceApiAssociationStatus() == null)
return false;
if (other.getSourceApiAssociationStatus() != null && other.getSourceApiAssociationStatus().equals(this.getSourceApiAssociationStatus()) == false)
return false;
if (other.getSourceApiAssociationStatusDetail() == null ^ this.getSourceApiAssociationStatusDetail() == null)
return false;
if (other.getSourceApiAssociationStatusDetail() != null
&& other.getSourceApiAssociationStatusDetail().equals(this.getSourceApiAssociationStatusDetail()) == false)
return false;
if (other.getLastSuccessfulMergeDate() == null ^ this.getLastSuccessfulMergeDate() == null)
return false;
if (other.getLastSuccessfulMergeDate() != null && other.getLastSuccessfulMergeDate().equals(this.getLastSuccessfulMergeDate()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAssociationId() == null) ? 0 : getAssociationId().hashCode());
hashCode = prime * hashCode + ((getAssociationArn() == null) ? 0 : getAssociationArn().hashCode());
hashCode = prime * hashCode + ((getSourceApiId() == null) ? 0 : getSourceApiId().hashCode());
hashCode = prime * hashCode + ((getSourceApiArn() == null) ? 0 : getSourceApiArn().hashCode());
hashCode = prime * hashCode + ((getMergedApiArn() == null) ? 0 : getMergedApiArn().hashCode());
hashCode = prime * hashCode + ((getMergedApiId() == null) ? 0 : getMergedApiId().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getSourceApiAssociationConfig() == null) ? 0 : getSourceApiAssociationConfig().hashCode());
hashCode = prime * hashCode + ((getSourceApiAssociationStatus() == null) ? 0 : getSourceApiAssociationStatus().hashCode());
hashCode = prime * hashCode + ((getSourceApiAssociationStatusDetail() == null) ? 0 : getSourceApiAssociationStatusDetail().hashCode());
hashCode = prime * hashCode + ((getLastSuccessfulMergeDate() == null) ? 0 : getLastSuccessfulMergeDate().hashCode());
return hashCode;
}
@Override
public SourceApiAssociation clone() {
try {
return (SourceApiAssociation) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.appsync.model.transform.SourceApiAssociationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}