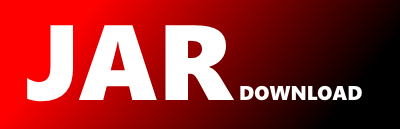
com.amazonaws.services.appsync.model.UpdateGraphqlApiRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appsync Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appsync.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateGraphqlApiRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The API ID.
*
*/
private String apiId;
/**
*
* The new name for the GraphqlApi
object.
*
*/
private String name;
/**
*
* The Amazon CloudWatch Logs configuration for the GraphqlApi
object.
*
*/
private LogConfig logConfig;
/**
*
* The new authentication type for the GraphqlApi
object.
*
*/
private String authenticationType;
/**
*
* The new Amazon Cognito user pool configuration for the ~GraphqlApi
object.
*
*/
private UserPoolConfig userPoolConfig;
/**
*
* The OpenID Connect configuration for the GraphqlApi
object.
*
*/
private OpenIDConnectConfig openIDConnectConfig;
/**
*
* A list of additional authentication providers for the GraphqlApi
API.
*
*/
private java.util.List additionalAuthenticationProviders;
/**
*
* A flag indicating whether to use X-Ray tracing for the GraphqlApi
.
*
*/
private Boolean xrayEnabled;
/**
*
* Configuration for Lambda function authorization.
*
*/
private LambdaAuthorizerConfig lambdaAuthorizerConfig;
/**
*
* The Identity and Access Management service role ARN for a merged API. The AppSync service assumes this role on
* behalf of the Merged API to validate access to source APIs at runtime and to prompt the AUTO_MERGE
* to update the merged API endpoint with the source API changes automatically.
*
*/
private String mergedApiExecutionRoleArn;
/**
*
* The owner contact information for an API resource.
*
*
* This field accepts any string input with a length of 0 - 256 characters.
*
*/
private String ownerContact;
/**
*
* Sets the value of the GraphQL API to enable (ENABLED
) or disable (DISABLED
)
* introspection. If no value is provided, the introspection configuration will be set to ENABLED
by
* default. This field will produce an error if the operation attempts to use the introspection feature while this
* field is disabled.
*
*
* For more information about introspection, see GraphQL
* introspection.
*
*/
private String introspectionConfig;
/**
*
* The maximum depth a query can have in a single request. Depth refers to the amount of nested levels allowed in
* the body of query. The default value is 0
(or unspecified), which indicates there's no depth limit.
* If you set a limit, it can be between 1
and 75
nested levels. This field will produce a
* limit error if the operation falls out of bounds.
*
*
* Note that fields can still be set to nullable or non-nullable. If a non-nullable field produces an error, the
* error will be thrown upwards to the first nullable field available.
*
*/
private Integer queryDepthLimit;
/**
*
* The maximum number of resolvers that can be invoked in a single request. The default value is 0
(or
* unspecified), which will set the limit to 10000
. When specified, the limit value can be between
* 1
and 10000
. This field will produce a limit error if the operation falls out of
* bounds.
*
*/
private Integer resolverCountLimit;
/**
*
* The enhancedMetricsConfig
object.
*
*/
private EnhancedMetricsConfig enhancedMetricsConfig;
/**
*
* The API ID.
*
*
* @param apiId
* The API ID.
*/
public void setApiId(String apiId) {
this.apiId = apiId;
}
/**
*
* The API ID.
*
*
* @return The API ID.
*/
public String getApiId() {
return this.apiId;
}
/**
*
* The API ID.
*
*
* @param apiId
* The API ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateGraphqlApiRequest withApiId(String apiId) {
setApiId(apiId);
return this;
}
/**
*
* The new name for the GraphqlApi
object.
*
*
* @param name
* The new name for the GraphqlApi
object.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The new name for the GraphqlApi
object.
*
*
* @return The new name for the GraphqlApi
object.
*/
public String getName() {
return this.name;
}
/**
*
* The new name for the GraphqlApi
object.
*
*
* @param name
* The new name for the GraphqlApi
object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateGraphqlApiRequest withName(String name) {
setName(name);
return this;
}
/**
*
* The Amazon CloudWatch Logs configuration for the GraphqlApi
object.
*
*
* @param logConfig
* The Amazon CloudWatch Logs configuration for the GraphqlApi
object.
*/
public void setLogConfig(LogConfig logConfig) {
this.logConfig = logConfig;
}
/**
*
* The Amazon CloudWatch Logs configuration for the GraphqlApi
object.
*
*
* @return The Amazon CloudWatch Logs configuration for the GraphqlApi
object.
*/
public LogConfig getLogConfig() {
return this.logConfig;
}
/**
*
* The Amazon CloudWatch Logs configuration for the GraphqlApi
object.
*
*
* @param logConfig
* The Amazon CloudWatch Logs configuration for the GraphqlApi
object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateGraphqlApiRequest withLogConfig(LogConfig logConfig) {
setLogConfig(logConfig);
return this;
}
/**
*
* The new authentication type for the GraphqlApi
object.
*
*
* @param authenticationType
* The new authentication type for the GraphqlApi
object.
* @see AuthenticationType
*/
public void setAuthenticationType(String authenticationType) {
this.authenticationType = authenticationType;
}
/**
*
* The new authentication type for the GraphqlApi
object.
*
*
* @return The new authentication type for the GraphqlApi
object.
* @see AuthenticationType
*/
public String getAuthenticationType() {
return this.authenticationType;
}
/**
*
* The new authentication type for the GraphqlApi
object.
*
*
* @param authenticationType
* The new authentication type for the GraphqlApi
object.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthenticationType
*/
public UpdateGraphqlApiRequest withAuthenticationType(String authenticationType) {
setAuthenticationType(authenticationType);
return this;
}
/**
*
* The new authentication type for the GraphqlApi
object.
*
*
* @param authenticationType
* The new authentication type for the GraphqlApi
object.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthenticationType
*/
public UpdateGraphqlApiRequest withAuthenticationType(AuthenticationType authenticationType) {
this.authenticationType = authenticationType.toString();
return this;
}
/**
*
* The new Amazon Cognito user pool configuration for the ~GraphqlApi
object.
*
*
* @param userPoolConfig
* The new Amazon Cognito user pool configuration for the ~GraphqlApi
object.
*/
public void setUserPoolConfig(UserPoolConfig userPoolConfig) {
this.userPoolConfig = userPoolConfig;
}
/**
*
* The new Amazon Cognito user pool configuration for the ~GraphqlApi
object.
*
*
* @return The new Amazon Cognito user pool configuration for the ~GraphqlApi
object.
*/
public UserPoolConfig getUserPoolConfig() {
return this.userPoolConfig;
}
/**
*
* The new Amazon Cognito user pool configuration for the ~GraphqlApi
object.
*
*
* @param userPoolConfig
* The new Amazon Cognito user pool configuration for the ~GraphqlApi
object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateGraphqlApiRequest withUserPoolConfig(UserPoolConfig userPoolConfig) {
setUserPoolConfig(userPoolConfig);
return this;
}
/**
*
* The OpenID Connect configuration for the GraphqlApi
object.
*
*
* @param openIDConnectConfig
* The OpenID Connect configuration for the GraphqlApi
object.
*/
public void setOpenIDConnectConfig(OpenIDConnectConfig openIDConnectConfig) {
this.openIDConnectConfig = openIDConnectConfig;
}
/**
*
* The OpenID Connect configuration for the GraphqlApi
object.
*
*
* @return The OpenID Connect configuration for the GraphqlApi
object.
*/
public OpenIDConnectConfig getOpenIDConnectConfig() {
return this.openIDConnectConfig;
}
/**
*
* The OpenID Connect configuration for the GraphqlApi
object.
*
*
* @param openIDConnectConfig
* The OpenID Connect configuration for the GraphqlApi
object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateGraphqlApiRequest withOpenIDConnectConfig(OpenIDConnectConfig openIDConnectConfig) {
setOpenIDConnectConfig(openIDConnectConfig);
return this;
}
/**
*
* A list of additional authentication providers for the GraphqlApi
API.
*
*
* @return A list of additional authentication providers for the GraphqlApi
API.
*/
public java.util.List getAdditionalAuthenticationProviders() {
return additionalAuthenticationProviders;
}
/**
*
* A list of additional authentication providers for the GraphqlApi
API.
*
*
* @param additionalAuthenticationProviders
* A list of additional authentication providers for the GraphqlApi
API.
*/
public void setAdditionalAuthenticationProviders(java.util.Collection additionalAuthenticationProviders) {
if (additionalAuthenticationProviders == null) {
this.additionalAuthenticationProviders = null;
return;
}
this.additionalAuthenticationProviders = new java.util.ArrayList(additionalAuthenticationProviders);
}
/**
*
* A list of additional authentication providers for the GraphqlApi
API.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAdditionalAuthenticationProviders(java.util.Collection)} or
* {@link #withAdditionalAuthenticationProviders(java.util.Collection)} if you want to override the existing values.
*
*
* @param additionalAuthenticationProviders
* A list of additional authentication providers for the GraphqlApi
API.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateGraphqlApiRequest withAdditionalAuthenticationProviders(AdditionalAuthenticationProvider... additionalAuthenticationProviders) {
if (this.additionalAuthenticationProviders == null) {
setAdditionalAuthenticationProviders(new java.util.ArrayList(additionalAuthenticationProviders.length));
}
for (AdditionalAuthenticationProvider ele : additionalAuthenticationProviders) {
this.additionalAuthenticationProviders.add(ele);
}
return this;
}
/**
*
* A list of additional authentication providers for the GraphqlApi
API.
*
*
* @param additionalAuthenticationProviders
* A list of additional authentication providers for the GraphqlApi
API.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateGraphqlApiRequest withAdditionalAuthenticationProviders(
java.util.Collection additionalAuthenticationProviders) {
setAdditionalAuthenticationProviders(additionalAuthenticationProviders);
return this;
}
/**
*
* A flag indicating whether to use X-Ray tracing for the GraphqlApi
.
*
*
* @param xrayEnabled
* A flag indicating whether to use X-Ray tracing for the GraphqlApi
.
*/
public void setXrayEnabled(Boolean xrayEnabled) {
this.xrayEnabled = xrayEnabled;
}
/**
*
* A flag indicating whether to use X-Ray tracing for the GraphqlApi
.
*
*
* @return A flag indicating whether to use X-Ray tracing for the GraphqlApi
.
*/
public Boolean getXrayEnabled() {
return this.xrayEnabled;
}
/**
*
* A flag indicating whether to use X-Ray tracing for the GraphqlApi
.
*
*
* @param xrayEnabled
* A flag indicating whether to use X-Ray tracing for the GraphqlApi
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateGraphqlApiRequest withXrayEnabled(Boolean xrayEnabled) {
setXrayEnabled(xrayEnabled);
return this;
}
/**
*
* A flag indicating whether to use X-Ray tracing for the GraphqlApi
.
*
*
* @return A flag indicating whether to use X-Ray tracing for the GraphqlApi
.
*/
public Boolean isXrayEnabled() {
return this.xrayEnabled;
}
/**
*
* Configuration for Lambda function authorization.
*
*
* @param lambdaAuthorizerConfig
* Configuration for Lambda function authorization.
*/
public void setLambdaAuthorizerConfig(LambdaAuthorizerConfig lambdaAuthorizerConfig) {
this.lambdaAuthorizerConfig = lambdaAuthorizerConfig;
}
/**
*
* Configuration for Lambda function authorization.
*
*
* @return Configuration for Lambda function authorization.
*/
public LambdaAuthorizerConfig getLambdaAuthorizerConfig() {
return this.lambdaAuthorizerConfig;
}
/**
*
* Configuration for Lambda function authorization.
*
*
* @param lambdaAuthorizerConfig
* Configuration for Lambda function authorization.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateGraphqlApiRequest withLambdaAuthorizerConfig(LambdaAuthorizerConfig lambdaAuthorizerConfig) {
setLambdaAuthorizerConfig(lambdaAuthorizerConfig);
return this;
}
/**
*
* The Identity and Access Management service role ARN for a merged API. The AppSync service assumes this role on
* behalf of the Merged API to validate access to source APIs at runtime and to prompt the AUTO_MERGE
* to update the merged API endpoint with the source API changes automatically.
*
*
* @param mergedApiExecutionRoleArn
* The Identity and Access Management service role ARN for a merged API. The AppSync service assumes this
* role on behalf of the Merged API to validate access to source APIs at runtime and to prompt the
* AUTO_MERGE
to update the merged API endpoint with the source API changes automatically.
*/
public void setMergedApiExecutionRoleArn(String mergedApiExecutionRoleArn) {
this.mergedApiExecutionRoleArn = mergedApiExecutionRoleArn;
}
/**
*
* The Identity and Access Management service role ARN for a merged API. The AppSync service assumes this role on
* behalf of the Merged API to validate access to source APIs at runtime and to prompt the AUTO_MERGE
* to update the merged API endpoint with the source API changes automatically.
*
*
* @return The Identity and Access Management service role ARN for a merged API. The AppSync service assumes this
* role on behalf of the Merged API to validate access to source APIs at runtime and to prompt the
* AUTO_MERGE
to update the merged API endpoint with the source API changes automatically.
*/
public String getMergedApiExecutionRoleArn() {
return this.mergedApiExecutionRoleArn;
}
/**
*
* The Identity and Access Management service role ARN for a merged API. The AppSync service assumes this role on
* behalf of the Merged API to validate access to source APIs at runtime and to prompt the AUTO_MERGE
* to update the merged API endpoint with the source API changes automatically.
*
*
* @param mergedApiExecutionRoleArn
* The Identity and Access Management service role ARN for a merged API. The AppSync service assumes this
* role on behalf of the Merged API to validate access to source APIs at runtime and to prompt the
* AUTO_MERGE
to update the merged API endpoint with the source API changes automatically.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateGraphqlApiRequest withMergedApiExecutionRoleArn(String mergedApiExecutionRoleArn) {
setMergedApiExecutionRoleArn(mergedApiExecutionRoleArn);
return this;
}
/**
*
* The owner contact information for an API resource.
*
*
* This field accepts any string input with a length of 0 - 256 characters.
*
*
* @param ownerContact
* The owner contact information for an API resource.
*
* This field accepts any string input with a length of 0 - 256 characters.
*/
public void setOwnerContact(String ownerContact) {
this.ownerContact = ownerContact;
}
/**
*
* The owner contact information for an API resource.
*
*
* This field accepts any string input with a length of 0 - 256 characters.
*
*
* @return The owner contact information for an API resource.
*
* This field accepts any string input with a length of 0 - 256 characters.
*/
public String getOwnerContact() {
return this.ownerContact;
}
/**
*
* The owner contact information for an API resource.
*
*
* This field accepts any string input with a length of 0 - 256 characters.
*
*
* @param ownerContact
* The owner contact information for an API resource.
*
* This field accepts any string input with a length of 0 - 256 characters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateGraphqlApiRequest withOwnerContact(String ownerContact) {
setOwnerContact(ownerContact);
return this;
}
/**
*
* Sets the value of the GraphQL API to enable (ENABLED
) or disable (DISABLED
)
* introspection. If no value is provided, the introspection configuration will be set to ENABLED
by
* default. This field will produce an error if the operation attempts to use the introspection feature while this
* field is disabled.
*
*
* For more information about introspection, see GraphQL
* introspection.
*
*
* @param introspectionConfig
* Sets the value of the GraphQL API to enable (ENABLED
) or disable (DISABLED
)
* introspection. If no value is provided, the introspection configuration will be set to
* ENABLED
by default. This field will produce an error if the operation attempts to use the
* introspection feature while this field is disabled.
*
* For more information about introspection, see GraphQL
* introspection.
* @see GraphQLApiIntrospectionConfig
*/
public void setIntrospectionConfig(String introspectionConfig) {
this.introspectionConfig = introspectionConfig;
}
/**
*
* Sets the value of the GraphQL API to enable (ENABLED
) or disable (DISABLED
)
* introspection. If no value is provided, the introspection configuration will be set to ENABLED
by
* default. This field will produce an error if the operation attempts to use the introspection feature while this
* field is disabled.
*
*
* For more information about introspection, see GraphQL
* introspection.
*
*
* @return Sets the value of the GraphQL API to enable (ENABLED
) or disable (DISABLED
)
* introspection. If no value is provided, the introspection configuration will be set to
* ENABLED
by default. This field will produce an error if the operation attempts to use the
* introspection feature while this field is disabled.
*
* For more information about introspection, see GraphQL
* introspection.
* @see GraphQLApiIntrospectionConfig
*/
public String getIntrospectionConfig() {
return this.introspectionConfig;
}
/**
*
* Sets the value of the GraphQL API to enable (ENABLED
) or disable (DISABLED
)
* introspection. If no value is provided, the introspection configuration will be set to ENABLED
by
* default. This field will produce an error if the operation attempts to use the introspection feature while this
* field is disabled.
*
*
* For more information about introspection, see GraphQL
* introspection.
*
*
* @param introspectionConfig
* Sets the value of the GraphQL API to enable (ENABLED
) or disable (DISABLED
)
* introspection. If no value is provided, the introspection configuration will be set to
* ENABLED
by default. This field will produce an error if the operation attempts to use the
* introspection feature while this field is disabled.
*
* For more information about introspection, see GraphQL
* introspection.
* @return Returns a reference to this object so that method calls can be chained together.
* @see GraphQLApiIntrospectionConfig
*/
public UpdateGraphqlApiRequest withIntrospectionConfig(String introspectionConfig) {
setIntrospectionConfig(introspectionConfig);
return this;
}
/**
*
* Sets the value of the GraphQL API to enable (ENABLED
) or disable (DISABLED
)
* introspection. If no value is provided, the introspection configuration will be set to ENABLED
by
* default. This field will produce an error if the operation attempts to use the introspection feature while this
* field is disabled.
*
*
* For more information about introspection, see GraphQL
* introspection.
*
*
* @param introspectionConfig
* Sets the value of the GraphQL API to enable (ENABLED
) or disable (DISABLED
)
* introspection. If no value is provided, the introspection configuration will be set to
* ENABLED
by default. This field will produce an error if the operation attempts to use the
* introspection feature while this field is disabled.
*
* For more information about introspection, see GraphQL
* introspection.
* @return Returns a reference to this object so that method calls can be chained together.
* @see GraphQLApiIntrospectionConfig
*/
public UpdateGraphqlApiRequest withIntrospectionConfig(GraphQLApiIntrospectionConfig introspectionConfig) {
this.introspectionConfig = introspectionConfig.toString();
return this;
}
/**
*
* The maximum depth a query can have in a single request. Depth refers to the amount of nested levels allowed in
* the body of query. The default value is 0
(or unspecified), which indicates there's no depth limit.
* If you set a limit, it can be between 1
and 75
nested levels. This field will produce a
* limit error if the operation falls out of bounds.
*
*
* Note that fields can still be set to nullable or non-nullable. If a non-nullable field produces an error, the
* error will be thrown upwards to the first nullable field available.
*
*
* @param queryDepthLimit
* The maximum depth a query can have in a single request. Depth refers to the amount of nested levels
* allowed in the body of query. The default value is 0
(or unspecified), which indicates
* there's no depth limit. If you set a limit, it can be between 1
and 75
nested
* levels. This field will produce a limit error if the operation falls out of bounds.
*
* Note that fields can still be set to nullable or non-nullable. If a non-nullable field produces an error,
* the error will be thrown upwards to the first nullable field available.
*/
public void setQueryDepthLimit(Integer queryDepthLimit) {
this.queryDepthLimit = queryDepthLimit;
}
/**
*
* The maximum depth a query can have in a single request. Depth refers to the amount of nested levels allowed in
* the body of query. The default value is 0
(or unspecified), which indicates there's no depth limit.
* If you set a limit, it can be between 1
and 75
nested levels. This field will produce a
* limit error if the operation falls out of bounds.
*
*
* Note that fields can still be set to nullable or non-nullable. If a non-nullable field produces an error, the
* error will be thrown upwards to the first nullable field available.
*
*
* @return The maximum depth a query can have in a single request. Depth refers to the amount of nested levels
* allowed in the body of query. The default value is 0
(or unspecified), which indicates
* there's no depth limit. If you set a limit, it can be between 1
and 75
nested
* levels. This field will produce a limit error if the operation falls out of bounds.
*
* Note that fields can still be set to nullable or non-nullable. If a non-nullable field produces an error,
* the error will be thrown upwards to the first nullable field available.
*/
public Integer getQueryDepthLimit() {
return this.queryDepthLimit;
}
/**
*
* The maximum depth a query can have in a single request. Depth refers to the amount of nested levels allowed in
* the body of query. The default value is 0
(or unspecified), which indicates there's no depth limit.
* If you set a limit, it can be between 1
and 75
nested levels. This field will produce a
* limit error if the operation falls out of bounds.
*
*
* Note that fields can still be set to nullable or non-nullable. If a non-nullable field produces an error, the
* error will be thrown upwards to the first nullable field available.
*
*
* @param queryDepthLimit
* The maximum depth a query can have in a single request. Depth refers to the amount of nested levels
* allowed in the body of query. The default value is 0
(or unspecified), which indicates
* there's no depth limit. If you set a limit, it can be between 1
and 75
nested
* levels. This field will produce a limit error if the operation falls out of bounds.
*
* Note that fields can still be set to nullable or non-nullable. If a non-nullable field produces an error,
* the error will be thrown upwards to the first nullable field available.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateGraphqlApiRequest withQueryDepthLimit(Integer queryDepthLimit) {
setQueryDepthLimit(queryDepthLimit);
return this;
}
/**
*
* The maximum number of resolvers that can be invoked in a single request. The default value is 0
(or
* unspecified), which will set the limit to 10000
. When specified, the limit value can be between
* 1
and 10000
. This field will produce a limit error if the operation falls out of
* bounds.
*
*
* @param resolverCountLimit
* The maximum number of resolvers that can be invoked in a single request. The default value is
* 0
(or unspecified), which will set the limit to 10000
. When specified, the limit
* value can be between 1
and 10000
. This field will produce a limit error if the
* operation falls out of bounds.
*/
public void setResolverCountLimit(Integer resolverCountLimit) {
this.resolverCountLimit = resolverCountLimit;
}
/**
*
* The maximum number of resolvers that can be invoked in a single request. The default value is 0
(or
* unspecified), which will set the limit to 10000
. When specified, the limit value can be between
* 1
and 10000
. This field will produce a limit error if the operation falls out of
* bounds.
*
*
* @return The maximum number of resolvers that can be invoked in a single request. The default value is
* 0
(or unspecified), which will set the limit to 10000
. When specified, the
* limit value can be between 1
and 10000
. This field will produce a limit error
* if the operation falls out of bounds.
*/
public Integer getResolverCountLimit() {
return this.resolverCountLimit;
}
/**
*
* The maximum number of resolvers that can be invoked in a single request. The default value is 0
(or
* unspecified), which will set the limit to 10000
. When specified, the limit value can be between
* 1
and 10000
. This field will produce a limit error if the operation falls out of
* bounds.
*
*
* @param resolverCountLimit
* The maximum number of resolvers that can be invoked in a single request. The default value is
* 0
(or unspecified), which will set the limit to 10000
. When specified, the limit
* value can be between 1
and 10000
. This field will produce a limit error if the
* operation falls out of bounds.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateGraphqlApiRequest withResolverCountLimit(Integer resolverCountLimit) {
setResolverCountLimit(resolverCountLimit);
return this;
}
/**
*
* The enhancedMetricsConfig
object.
*
*
* @param enhancedMetricsConfig
* The enhancedMetricsConfig
object.
*/
public void setEnhancedMetricsConfig(EnhancedMetricsConfig enhancedMetricsConfig) {
this.enhancedMetricsConfig = enhancedMetricsConfig;
}
/**
*
* The enhancedMetricsConfig
object.
*
*
* @return The enhancedMetricsConfig
object.
*/
public EnhancedMetricsConfig getEnhancedMetricsConfig() {
return this.enhancedMetricsConfig;
}
/**
*
* The enhancedMetricsConfig
object.
*
*
* @param enhancedMetricsConfig
* The enhancedMetricsConfig
object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateGraphqlApiRequest withEnhancedMetricsConfig(EnhancedMetricsConfig enhancedMetricsConfig) {
setEnhancedMetricsConfig(enhancedMetricsConfig);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getApiId() != null)
sb.append("ApiId: ").append(getApiId()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getLogConfig() != null)
sb.append("LogConfig: ").append(getLogConfig()).append(",");
if (getAuthenticationType() != null)
sb.append("AuthenticationType: ").append(getAuthenticationType()).append(",");
if (getUserPoolConfig() != null)
sb.append("UserPoolConfig: ").append(getUserPoolConfig()).append(",");
if (getOpenIDConnectConfig() != null)
sb.append("OpenIDConnectConfig: ").append(getOpenIDConnectConfig()).append(",");
if (getAdditionalAuthenticationProviders() != null)
sb.append("AdditionalAuthenticationProviders: ").append(getAdditionalAuthenticationProviders()).append(",");
if (getXrayEnabled() != null)
sb.append("XrayEnabled: ").append(getXrayEnabled()).append(",");
if (getLambdaAuthorizerConfig() != null)
sb.append("LambdaAuthorizerConfig: ").append(getLambdaAuthorizerConfig()).append(",");
if (getMergedApiExecutionRoleArn() != null)
sb.append("MergedApiExecutionRoleArn: ").append(getMergedApiExecutionRoleArn()).append(",");
if (getOwnerContact() != null)
sb.append("OwnerContact: ").append(getOwnerContact()).append(",");
if (getIntrospectionConfig() != null)
sb.append("IntrospectionConfig: ").append(getIntrospectionConfig()).append(",");
if (getQueryDepthLimit() != null)
sb.append("QueryDepthLimit: ").append(getQueryDepthLimit()).append(",");
if (getResolverCountLimit() != null)
sb.append("ResolverCountLimit: ").append(getResolverCountLimit()).append(",");
if (getEnhancedMetricsConfig() != null)
sb.append("EnhancedMetricsConfig: ").append(getEnhancedMetricsConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateGraphqlApiRequest == false)
return false;
UpdateGraphqlApiRequest other = (UpdateGraphqlApiRequest) obj;
if (other.getApiId() == null ^ this.getApiId() == null)
return false;
if (other.getApiId() != null && other.getApiId().equals(this.getApiId()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getLogConfig() == null ^ this.getLogConfig() == null)
return false;
if (other.getLogConfig() != null && other.getLogConfig().equals(this.getLogConfig()) == false)
return false;
if (other.getAuthenticationType() == null ^ this.getAuthenticationType() == null)
return false;
if (other.getAuthenticationType() != null && other.getAuthenticationType().equals(this.getAuthenticationType()) == false)
return false;
if (other.getUserPoolConfig() == null ^ this.getUserPoolConfig() == null)
return false;
if (other.getUserPoolConfig() != null && other.getUserPoolConfig().equals(this.getUserPoolConfig()) == false)
return false;
if (other.getOpenIDConnectConfig() == null ^ this.getOpenIDConnectConfig() == null)
return false;
if (other.getOpenIDConnectConfig() != null && other.getOpenIDConnectConfig().equals(this.getOpenIDConnectConfig()) == false)
return false;
if (other.getAdditionalAuthenticationProviders() == null ^ this.getAdditionalAuthenticationProviders() == null)
return false;
if (other.getAdditionalAuthenticationProviders() != null
&& other.getAdditionalAuthenticationProviders().equals(this.getAdditionalAuthenticationProviders()) == false)
return false;
if (other.getXrayEnabled() == null ^ this.getXrayEnabled() == null)
return false;
if (other.getXrayEnabled() != null && other.getXrayEnabled().equals(this.getXrayEnabled()) == false)
return false;
if (other.getLambdaAuthorizerConfig() == null ^ this.getLambdaAuthorizerConfig() == null)
return false;
if (other.getLambdaAuthorizerConfig() != null && other.getLambdaAuthorizerConfig().equals(this.getLambdaAuthorizerConfig()) == false)
return false;
if (other.getMergedApiExecutionRoleArn() == null ^ this.getMergedApiExecutionRoleArn() == null)
return false;
if (other.getMergedApiExecutionRoleArn() != null && other.getMergedApiExecutionRoleArn().equals(this.getMergedApiExecutionRoleArn()) == false)
return false;
if (other.getOwnerContact() == null ^ this.getOwnerContact() == null)
return false;
if (other.getOwnerContact() != null && other.getOwnerContact().equals(this.getOwnerContact()) == false)
return false;
if (other.getIntrospectionConfig() == null ^ this.getIntrospectionConfig() == null)
return false;
if (other.getIntrospectionConfig() != null && other.getIntrospectionConfig().equals(this.getIntrospectionConfig()) == false)
return false;
if (other.getQueryDepthLimit() == null ^ this.getQueryDepthLimit() == null)
return false;
if (other.getQueryDepthLimit() != null && other.getQueryDepthLimit().equals(this.getQueryDepthLimit()) == false)
return false;
if (other.getResolverCountLimit() == null ^ this.getResolverCountLimit() == null)
return false;
if (other.getResolverCountLimit() != null && other.getResolverCountLimit().equals(this.getResolverCountLimit()) == false)
return false;
if (other.getEnhancedMetricsConfig() == null ^ this.getEnhancedMetricsConfig() == null)
return false;
if (other.getEnhancedMetricsConfig() != null && other.getEnhancedMetricsConfig().equals(this.getEnhancedMetricsConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getApiId() == null) ? 0 : getApiId().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getLogConfig() == null) ? 0 : getLogConfig().hashCode());
hashCode = prime * hashCode + ((getAuthenticationType() == null) ? 0 : getAuthenticationType().hashCode());
hashCode = prime * hashCode + ((getUserPoolConfig() == null) ? 0 : getUserPoolConfig().hashCode());
hashCode = prime * hashCode + ((getOpenIDConnectConfig() == null) ? 0 : getOpenIDConnectConfig().hashCode());
hashCode = prime * hashCode + ((getAdditionalAuthenticationProviders() == null) ? 0 : getAdditionalAuthenticationProviders().hashCode());
hashCode = prime * hashCode + ((getXrayEnabled() == null) ? 0 : getXrayEnabled().hashCode());
hashCode = prime * hashCode + ((getLambdaAuthorizerConfig() == null) ? 0 : getLambdaAuthorizerConfig().hashCode());
hashCode = prime * hashCode + ((getMergedApiExecutionRoleArn() == null) ? 0 : getMergedApiExecutionRoleArn().hashCode());
hashCode = prime * hashCode + ((getOwnerContact() == null) ? 0 : getOwnerContact().hashCode());
hashCode = prime * hashCode + ((getIntrospectionConfig() == null) ? 0 : getIntrospectionConfig().hashCode());
hashCode = prime * hashCode + ((getQueryDepthLimit() == null) ? 0 : getQueryDepthLimit().hashCode());
hashCode = prime * hashCode + ((getResolverCountLimit() == null) ? 0 : getResolverCountLimit().hashCode());
hashCode = prime * hashCode + ((getEnhancedMetricsConfig() == null) ? 0 : getEnhancedMetricsConfig().hashCode());
return hashCode;
}
@Override
public UpdateGraphqlApiRequest clone() {
return (UpdateGraphqlApiRequest) super.clone();
}
}