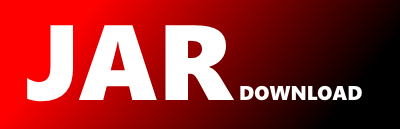
com.amazonaws.services.appsync.AWSAppSync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appsync Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appsync;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.appsync.model.*;
/**
* Interface for accessing AWSAppSync.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.appsync.AbstractAWSAppSync} instead.
*
*
*
* AppSync provides API actions for creating and interacting with data sources using GraphQL from your application.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSAppSync {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "appsync";
/**
*
* Maps an endpoint to your custom domain.
*
*
* @param associateApiRequest
* @return Result of the AssociateApi operation returned by the service.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @sample AWSAppSync.AssociateApi
* @see AWS API
* Documentation
*/
AssociateApiResult associateApi(AssociateApiRequest associateApiRequest);
/**
*
* Creates an association between a Merged API and source API using the source API's identifier.
*
*
* @param associateMergedGraphqlApiRequest
* @return Result of the AssociateMergedGraphqlApi operation returned by the service.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @sample AWSAppSync.AssociateMergedGraphqlApi
* @see AWS API Documentation
*/
AssociateMergedGraphqlApiResult associateMergedGraphqlApi(AssociateMergedGraphqlApiRequest associateMergedGraphqlApiRequest);
/**
*
* Creates an association between a Merged API and source API using the Merged API's identifier.
*
*
* @param associateSourceGraphqlApiRequest
* @return Result of the AssociateSourceGraphqlApi operation returned by the service.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @sample AWSAppSync.AssociateSourceGraphqlApi
* @see AWS API Documentation
*/
AssociateSourceGraphqlApiResult associateSourceGraphqlApi(AssociateSourceGraphqlApiRequest associateSourceGraphqlApiRequest);
/**
*
* Creates a cache for the GraphQL API.
*
*
* @param createApiCacheRequest
* Represents the input of a CreateApiCache
operation.
* @return Result of the CreateApiCache operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.CreateApiCache
* @see AWS API
* Documentation
*/
CreateApiCacheResult createApiCache(CreateApiCacheRequest createApiCacheRequest);
/**
*
* Creates a unique key that you can distribute to clients who invoke your API.
*
*
* @param createApiKeyRequest
* @return Result of the CreateApiKey operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws ApiKeyLimitExceededException
* The API key exceeded a limit. Try your request again.
* @throws ApiKeyValidityOutOfBoundsException
* The API key expiration must be set to a value between 1 and 365 days from creation (for
* CreateApiKey
) or from update (for UpdateApiKey
).
* @sample AWSAppSync.CreateApiKey
* @see AWS API
* Documentation
*/
CreateApiKeyResult createApiKey(CreateApiKeyRequest createApiKeyRequest);
/**
*
* Creates a DataSource
object.
*
*
* @param createDataSourceRequest
* @return Result of the CreateDataSource operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.CreateDataSource
* @see AWS API
* Documentation
*/
CreateDataSourceResult createDataSource(CreateDataSourceRequest createDataSourceRequest);
/**
*
* Creates a custom DomainName
object.
*
*
* @param createDomainNameRequest
* @return Result of the CreateDomainName operation returned by the service.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.CreateDomainName
* @see AWS API
* Documentation
*/
CreateDomainNameResult createDomainName(CreateDomainNameRequest createDomainNameRequest);
/**
*
* Creates a Function
object.
*
*
* A function is a reusable entity. You can use multiple functions to compose the resolver logic.
*
*
* @param createFunctionRequest
* @return Result of the CreateFunction operation returned by the service.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @sample AWSAppSync.CreateFunction
* @see AWS API
* Documentation
*/
CreateFunctionResult createFunction(CreateFunctionRequest createFunctionRequest);
/**
*
* Creates a GraphqlApi
object.
*
*
* @param createGraphqlApiRequest
* @return Result of the CreateGraphqlApi operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws ApiLimitExceededException
* The GraphQL API exceeded a limit. Try your request again.
* @sample AWSAppSync.CreateGraphqlApi
* @see AWS API
* Documentation
*/
CreateGraphqlApiResult createGraphqlApi(CreateGraphqlApiRequest createGraphqlApiRequest);
/**
*
* Creates a Resolver
object.
*
*
* A resolver converts incoming requests into a format that a data source can understand, and converts the data
* source's responses into GraphQL.
*
*
* @param createResolverRequest
* @return Result of the CreateResolver operation returned by the service.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @sample AWSAppSync.CreateResolver
* @see AWS API
* Documentation
*/
CreateResolverResult createResolver(CreateResolverRequest createResolverRequest);
/**
*
* Creates a Type
object.
*
*
* @param createTypeRequest
* @return Result of the CreateType operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.CreateType
* @see AWS API
* Documentation
*/
CreateTypeResult createType(CreateTypeRequest createTypeRequest);
/**
*
* Deletes an ApiCache
object.
*
*
* @param deleteApiCacheRequest
* Represents the input of a DeleteApiCache
operation.
* @return Result of the DeleteApiCache operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.DeleteApiCache
* @see AWS API
* Documentation
*/
DeleteApiCacheResult deleteApiCache(DeleteApiCacheRequest deleteApiCacheRequest);
/**
*
* Deletes an API key.
*
*
* @param deleteApiKeyRequest
* @return Result of the DeleteApiKey operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.DeleteApiKey
* @see AWS API
* Documentation
*/
DeleteApiKeyResult deleteApiKey(DeleteApiKeyRequest deleteApiKeyRequest);
/**
*
* Deletes a DataSource
object.
*
*
* @param deleteDataSourceRequest
* @return Result of the DeleteDataSource operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.DeleteDataSource
* @see AWS API
* Documentation
*/
DeleteDataSourceResult deleteDataSource(DeleteDataSourceRequest deleteDataSourceRequest);
/**
*
* Deletes a custom DomainName
object.
*
*
* @param deleteDomainNameRequest
* @return Result of the DeleteDomainName operation returned by the service.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @sample AWSAppSync.DeleteDomainName
* @see AWS API
* Documentation
*/
DeleteDomainNameResult deleteDomainName(DeleteDomainNameRequest deleteDomainNameRequest);
/**
*
* Deletes a Function
.
*
*
* @param deleteFunctionRequest
* @return Result of the DeleteFunction operation returned by the service.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @sample AWSAppSync.DeleteFunction
* @see AWS API
* Documentation
*/
DeleteFunctionResult deleteFunction(DeleteFunctionRequest deleteFunctionRequest);
/**
*
* Deletes a GraphqlApi
object.
*
*
* @param deleteGraphqlApiRequest
* @return Result of the DeleteGraphqlApi operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @sample AWSAppSync.DeleteGraphqlApi
* @see AWS API
* Documentation
*/
DeleteGraphqlApiResult deleteGraphqlApi(DeleteGraphqlApiRequest deleteGraphqlApiRequest);
/**
*
* Deletes a Resolver
object.
*
*
* @param deleteResolverRequest
* @return Result of the DeleteResolver operation returned by the service.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @sample AWSAppSync.DeleteResolver
* @see AWS API
* Documentation
*/
DeleteResolverResult deleteResolver(DeleteResolverRequest deleteResolverRequest);
/**
*
* Deletes a Type
object.
*
*
* @param deleteTypeRequest
* @return Result of the DeleteType operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.DeleteType
* @see AWS API
* Documentation
*/
DeleteTypeResult deleteType(DeleteTypeRequest deleteTypeRequest);
/**
*
* Removes an ApiAssociation
object from a custom domain.
*
*
* @param disassociateApiRequest
* @return Result of the DisassociateApi operation returned by the service.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @sample AWSAppSync.DisassociateApi
* @see AWS API
* Documentation
*/
DisassociateApiResult disassociateApi(DisassociateApiRequest disassociateApiRequest);
/**
*
* Deletes an association between a Merged API and source API using the source API's identifier and the association
* ID.
*
*
* @param disassociateMergedGraphqlApiRequest
* @return Result of the DisassociateMergedGraphqlApi operation returned by the service.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @sample AWSAppSync.DisassociateMergedGraphqlApi
* @see AWS API Documentation
*/
DisassociateMergedGraphqlApiResult disassociateMergedGraphqlApi(DisassociateMergedGraphqlApiRequest disassociateMergedGraphqlApiRequest);
/**
*
* Deletes an association between a Merged API and source API using the Merged API's identifier and the association
* ID.
*
*
* @param disassociateSourceGraphqlApiRequest
* @return Result of the DisassociateSourceGraphqlApi operation returned by the service.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @sample AWSAppSync.DisassociateSourceGraphqlApi
* @see AWS API Documentation
*/
DisassociateSourceGraphqlApiResult disassociateSourceGraphqlApi(DisassociateSourceGraphqlApiRequest disassociateSourceGraphqlApiRequest);
/**
*
* Evaluates the given code and returns the response. The code definition requirements depend on the specified
* runtime. For APPSYNC_JS
runtimes, the code defines the request and response functions. The request
* function takes the incoming request after a GraphQL operation is parsed and converts it into a request
* configuration for the selected data source operation. The response function interprets responses from the data
* source and maps it to the shape of the GraphQL field output type.
*
*
* @param evaluateCodeRequest
* @return Result of the EvaluateCode operation returned by the service.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @sample AWSAppSync.EvaluateCode
* @see AWS API
* Documentation
*/
EvaluateCodeResult evaluateCode(EvaluateCodeRequest evaluateCodeRequest);
/**
*
* Evaluates a given template and returns the response. The mapping template can be a request or response template.
*
*
* Request templates take the incoming request after a GraphQL operation is parsed and convert it into a request
* configuration for the selected data source operation. Response templates interpret responses from the data source
* and map it to the shape of the GraphQL field output type.
*
*
* Mapping templates are written in the Apache Velocity Template Language (VTL).
*
*
* @param evaluateMappingTemplateRequest
* @return Result of the EvaluateMappingTemplate operation returned by the service.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @sample AWSAppSync.EvaluateMappingTemplate
* @see AWS API Documentation
*/
EvaluateMappingTemplateResult evaluateMappingTemplate(EvaluateMappingTemplateRequest evaluateMappingTemplateRequest);
/**
*
* Flushes an ApiCache
object.
*
*
* @param flushApiCacheRequest
* Represents the input of a FlushApiCache
operation.
* @return Result of the FlushApiCache operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.FlushApiCache
* @see AWS API
* Documentation
*/
FlushApiCacheResult flushApiCache(FlushApiCacheRequest flushApiCacheRequest);
/**
*
* Retrieves an ApiAssociation
object.
*
*
* @param getApiAssociationRequest
* @return Result of the GetApiAssociation operation returned by the service.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @sample AWSAppSync.GetApiAssociation
* @see AWS API
* Documentation
*/
GetApiAssociationResult getApiAssociation(GetApiAssociationRequest getApiAssociationRequest);
/**
*
* Retrieves an ApiCache
object.
*
*
* @param getApiCacheRequest
* Represents the input of a GetApiCache
operation.
* @return Result of the GetApiCache operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.GetApiCache
* @see AWS API
* Documentation
*/
GetApiCacheResult getApiCache(GetApiCacheRequest getApiCacheRequest);
/**
*
* Retrieves a DataSource
object.
*
*
* @param getDataSourceRequest
* @return Result of the GetDataSource operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.GetDataSource
* @see AWS API
* Documentation
*/
GetDataSourceResult getDataSource(GetDataSourceRequest getDataSourceRequest);
/**
*
* Retrieves the record of an existing introspection. If the retrieval is successful, the result of the
* instrospection will also be returned. If the retrieval fails the operation, an error message will be returned
* instead.
*
*
* @param getDataSourceIntrospectionRequest
* @return Result of the GetDataSourceIntrospection operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.GetDataSourceIntrospection
* @see AWS API Documentation
*/
GetDataSourceIntrospectionResult getDataSourceIntrospection(GetDataSourceIntrospectionRequest getDataSourceIntrospectionRequest);
/**
*
* Retrieves a custom DomainName
object.
*
*
* @param getDomainNameRequest
* @return Result of the GetDomainName operation returned by the service.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @sample AWSAppSync.GetDomainName
* @see AWS API
* Documentation
*/
GetDomainNameResult getDomainName(GetDomainNameRequest getDomainNameRequest);
/**
*
* Get a Function
.
*
*
* @param getFunctionRequest
* @return Result of the GetFunction operation returned by the service.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @sample AWSAppSync.GetFunction
* @see AWS API
* Documentation
*/
GetFunctionResult getFunction(GetFunctionRequest getFunctionRequest);
/**
*
* Retrieves a GraphqlApi
object.
*
*
* @param getGraphqlApiRequest
* @return Result of the GetGraphqlApi operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @sample AWSAppSync.GetGraphqlApi
* @see AWS API
* Documentation
*/
GetGraphqlApiResult getGraphqlApi(GetGraphqlApiRequest getGraphqlApiRequest);
/**
*
* Retrieves the list of environmental variable key-value pairs associated with an API by its ID value.
*
*
* @param getGraphqlApiEnvironmentVariablesRequest
* @return Result of the GetGraphqlApiEnvironmentVariables operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @sample AWSAppSync.GetGraphqlApiEnvironmentVariables
* @see AWS API Documentation
*/
GetGraphqlApiEnvironmentVariablesResult getGraphqlApiEnvironmentVariables(GetGraphqlApiEnvironmentVariablesRequest getGraphqlApiEnvironmentVariablesRequest);
/**
*
* Retrieves the introspection schema for a GraphQL API.
*
*
* @param getIntrospectionSchemaRequest
* @return Result of the GetIntrospectionSchema operation returned by the service.
* @throws GraphQLSchemaException
* The GraphQL schema is not valid.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.GetIntrospectionSchema
* @see AWS
* API Documentation
*/
GetIntrospectionSchemaResult getIntrospectionSchema(GetIntrospectionSchemaRequest getIntrospectionSchemaRequest);
/**
*
* Retrieves a Resolver
object.
*
*
* @param getResolverRequest
* @return Result of the GetResolver operation returned by the service.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @sample AWSAppSync.GetResolver
* @see AWS API
* Documentation
*/
GetResolverResult getResolver(GetResolverRequest getResolverRequest);
/**
*
* Retrieves the current status of a schema creation operation.
*
*
* @param getSchemaCreationStatusRequest
* @return Result of the GetSchemaCreationStatus operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.GetSchemaCreationStatus
* @see AWS API Documentation
*/
GetSchemaCreationStatusResult getSchemaCreationStatus(GetSchemaCreationStatusRequest getSchemaCreationStatusRequest);
/**
*
* Retrieves a SourceApiAssociation
object.
*
*
* @param getSourceApiAssociationRequest
* @return Result of the GetSourceApiAssociation operation returned by the service.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @sample AWSAppSync.GetSourceApiAssociation
* @see AWS API Documentation
*/
GetSourceApiAssociationResult getSourceApiAssociation(GetSourceApiAssociationRequest getSourceApiAssociationRequest);
/**
*
* Retrieves a Type
object.
*
*
* @param getTypeRequest
* @return Result of the GetType operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.GetType
* @see AWS API
* Documentation
*/
GetTypeResult getType(GetTypeRequest getTypeRequest);
/**
*
* Lists the API keys for a given API.
*
*
*
* API keys are deleted automatically 60 days after they expire. However, they may still be included in the response
* until they have actually been deleted. You can safely call DeleteApiKey
to manually delete a key
* before it's automatically deleted.
*
*
*
* @param listApiKeysRequest
* @return Result of the ListApiKeys operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListApiKeys
* @see AWS API
* Documentation
*/
ListApiKeysResult listApiKeys(ListApiKeysRequest listApiKeysRequest);
/**
*
* Lists the data sources for a given API.
*
*
* @param listDataSourcesRequest
* @return Result of the ListDataSources operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListDataSources
* @see AWS API
* Documentation
*/
ListDataSourcesResult listDataSources(ListDataSourcesRequest listDataSourcesRequest);
/**
*
* Lists multiple custom domain names.
*
*
* @param listDomainNamesRequest
* @return Result of the ListDomainNames operation returned by the service.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListDomainNames
* @see AWS API
* Documentation
*/
ListDomainNamesResult listDomainNames(ListDomainNamesRequest listDomainNamesRequest);
/**
*
* List multiple functions.
*
*
* @param listFunctionsRequest
* @return Result of the ListFunctions operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListFunctions
* @see AWS API
* Documentation
*/
ListFunctionsResult listFunctions(ListFunctionsRequest listFunctionsRequest);
/**
*
* Lists your GraphQL APIs.
*
*
* @param listGraphqlApisRequest
* @return Result of the ListGraphqlApis operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListGraphqlApis
* @see AWS API
* Documentation
*/
ListGraphqlApisResult listGraphqlApis(ListGraphqlApisRequest listGraphqlApisRequest);
/**
*
* Lists the resolvers for a given API and type.
*
*
* @param listResolversRequest
* @return Result of the ListResolvers operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListResolvers
* @see AWS API
* Documentation
*/
ListResolversResult listResolvers(ListResolversRequest listResolversRequest);
/**
*
* List the resolvers that are associated with a specific function.
*
*
* @param listResolversByFunctionRequest
* @return Result of the ListResolversByFunction operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListResolversByFunction
* @see AWS API Documentation
*/
ListResolversByFunctionResult listResolversByFunction(ListResolversByFunctionRequest listResolversByFunctionRequest);
/**
*
* Lists the SourceApiAssociationSummary
data.
*
*
* @param listSourceApiAssociationsRequest
* @return Result of the ListSourceApiAssociations operation returned by the service.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @sample AWSAppSync.ListSourceApiAssociations
* @see AWS API Documentation
*/
ListSourceApiAssociationsResult listSourceApiAssociations(ListSourceApiAssociationsRequest listSourceApiAssociationsRequest);
/**
*
* Lists the tags for a resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @sample AWSAppSync.ListTagsForResource
* @see AWS
* API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists the types for a given API.
*
*
* @param listTypesRequest
* @return Result of the ListTypes operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListTypes
* @see AWS API
* Documentation
*/
ListTypesResult listTypes(ListTypesRequest listTypesRequest);
/**
*
* Lists Type
objects by the source API association ID.
*
*
* @param listTypesByAssociationRequest
* @return Result of the ListTypesByAssociation operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.ListTypesByAssociation
* @see AWS
* API Documentation
*/
ListTypesByAssociationResult listTypesByAssociation(ListTypesByAssociationRequest listTypesByAssociationRequest);
/**
*
* Creates a list of environmental variables in an API by its ID value.
*
*
* When creating an environmental variable, it must follow the constraints below:
*
*
* -
*
* Both JavaScript and VTL templates support environmental variables.
*
*
* -
*
* Environmental variables are not evaluated before function invocation.
*
*
* -
*
* Environmental variables only support string values.
*
*
* -
*
* Any defined value in an environmental variable is considered a string literal and not expanded.
*
*
* -
*
* Variable evaluations should ideally be performed in the function code.
*
*
*
*
* When creating an environmental variable key-value pair, it must follow the additional constraints below:
*
*
* -
*
* Keys must begin with a letter.
*
*
* -
*
* Keys must be at least two characters long.
*
*
* -
*
* Keys can only contain letters, numbers, and the underscore character (_).
*
*
* -
*
* Values can be up to 512 characters long.
*
*
* -
*
* You can configure up to 50 key-value pairs in a GraphQL API.
*
*
*
*
* You can create a list of environmental variables by adding it to the environmentVariables
payload as
* a list in the format {"key1":"value1","key2":"value2", …}
. Note that each call of the
* PutGraphqlApiEnvironmentVariables
action will result in the overwriting of the existing
* environmental variable list of that API. This means the existing environmental variables will be lost. To avoid
* this, you must include all existing and new environmental variables in the list each time you call this action.
*
*
* @param putGraphqlApiEnvironmentVariablesRequest
* @return Result of the PutGraphqlApiEnvironmentVariables operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @sample AWSAppSync.PutGraphqlApiEnvironmentVariables
* @see AWS API Documentation
*/
PutGraphqlApiEnvironmentVariablesResult putGraphqlApiEnvironmentVariables(PutGraphqlApiEnvironmentVariablesRequest putGraphqlApiEnvironmentVariablesRequest);
/**
*
* Creates a new introspection. Returns the introspectionId
of the new introspection after its
* creation.
*
*
* @param startDataSourceIntrospectionRequest
* @return Result of the StartDataSourceIntrospection operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @sample AWSAppSync.StartDataSourceIntrospection
* @see AWS API Documentation
*/
StartDataSourceIntrospectionResult startDataSourceIntrospection(StartDataSourceIntrospectionRequest startDataSourceIntrospectionRequest);
/**
*
* Adds a new schema to your GraphQL API.
*
*
* This operation is asynchronous. Use to determine when it has completed.
*
*
* @param startSchemaCreationRequest
* @return Result of the StartSchemaCreation operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.StartSchemaCreation
* @see AWS
* API Documentation
*/
StartSchemaCreationResult startSchemaCreation(StartSchemaCreationRequest startSchemaCreationRequest);
/**
*
* Initiates a merge operation. Returns a status that shows the result of the merge operation.
*
*
* @param startSchemaMergeRequest
* @return Result of the StartSchemaMerge operation returned by the service.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @sample AWSAppSync.StartSchemaMerge
* @see AWS API
* Documentation
*/
StartSchemaMergeResult startSchemaMerge(StartSchemaMergeRequest startSchemaMergeRequest);
/**
*
* Tags a resource with user-supplied tags.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @sample AWSAppSync.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Untags a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @sample AWSAppSync.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates the cache for the GraphQL API.
*
*
* @param updateApiCacheRequest
* Represents the input of a UpdateApiCache
operation.
* @return Result of the UpdateApiCache operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.UpdateApiCache
* @see AWS API
* Documentation
*/
UpdateApiCacheResult updateApiCache(UpdateApiCacheRequest updateApiCacheRequest);
/**
*
* Updates an API key. You can update the key as long as it's not deleted.
*
*
* @param updateApiKeyRequest
* @return Result of the UpdateApiKey operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws ApiKeyValidityOutOfBoundsException
* The API key expiration must be set to a value between 1 and 365 days from creation (for
* CreateApiKey
) or from update (for UpdateApiKey
).
* @sample AWSAppSync.UpdateApiKey
* @see AWS API
* Documentation
*/
UpdateApiKeyResult updateApiKey(UpdateApiKeyRequest updateApiKeyRequest);
/**
*
* Updates a DataSource
object.
*
*
* @param updateDataSourceRequest
* @return Result of the UpdateDataSource operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.UpdateDataSource
* @see AWS API
* Documentation
*/
UpdateDataSourceResult updateDataSource(UpdateDataSourceRequest updateDataSourceRequest);
/**
*
* Updates a custom DomainName
object.
*
*
* @param updateDomainNameRequest
* @return Result of the UpdateDomainName operation returned by the service.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @sample AWSAppSync.UpdateDomainName
* @see AWS API
* Documentation
*/
UpdateDomainNameResult updateDomainName(UpdateDomainNameRequest updateDomainNameRequest);
/**
*
* Updates a Function
object.
*
*
* @param updateFunctionRequest
* @return Result of the UpdateFunction operation returned by the service.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @sample AWSAppSync.UpdateFunction
* @see AWS API
* Documentation
*/
UpdateFunctionResult updateFunction(UpdateFunctionRequest updateFunctionRequest);
/**
*
* Updates a GraphqlApi
object.
*
*
* @param updateGraphqlApiRequest
* @return Result of the UpdateGraphqlApi operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws AccessDeniedException
* You don't have access to perform this operation on this resource.
* @sample AWSAppSync.UpdateGraphqlApi
* @see AWS API
* Documentation
*/
UpdateGraphqlApiResult updateGraphqlApi(UpdateGraphqlApiRequest updateGraphqlApiRequest);
/**
*
* Updates a Resolver
object.
*
*
* @param updateResolverRequest
* @return Result of the UpdateResolver operation returned by the service.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @sample AWSAppSync.UpdateResolver
* @see AWS API
* Documentation
*/
UpdateResolverResult updateResolver(UpdateResolverRequest updateResolverRequest);
/**
*
* Updates some of the configuration choices of a particular source API association.
*
*
* @param updateSourceApiAssociationRequest
* @return Result of the UpdateSourceApiAssociation operation returned by the service.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @sample AWSAppSync.UpdateSourceApiAssociation
* @see AWS API Documentation
*/
UpdateSourceApiAssociationResult updateSourceApiAssociation(UpdateSourceApiAssociationRequest updateSourceApiAssociationRequest);
/**
*
* Updates a Type
object.
*
*
* @param updateTypeRequest
* @return Result of the UpdateType operation returned by the service.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and then try again.
* @throws ConcurrentModificationException
* Another modification is in progress at this time and it must complete before you can make your change.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource, and then try again.
* @throws UnauthorizedException
* You aren't authorized to perform this operation.
* @throws InternalFailureException
* An internal AppSync error occurred. Try your request again.
* @sample AWSAppSync.UpdateType
* @see AWS API
* Documentation
*/
UpdateTypeResult updateType(UpdateTypeRequest updateTypeRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}