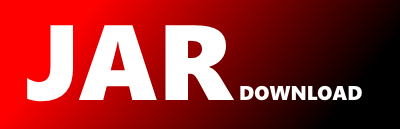
com.amazonaws.services.appsync.model.Resolver Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appsync Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appsync.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes a resolver.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Resolver implements Serializable, Cloneable, StructuredPojo {
/**
*
* The resolver type name.
*
*/
private String typeName;
/**
*
* The resolver field name.
*
*/
private String fieldName;
/**
*
* The resolver data source name.
*
*/
private String dataSourceName;
/**
*
* The resolver Amazon Resource Name (ARN).
*
*/
private String resolverArn;
/**
*
* The request mapping template.
*
*/
private String requestMappingTemplate;
/**
*
* The response mapping template.
*
*/
private String responseMappingTemplate;
/**
*
* The resolver type.
*
*
* -
*
* UNIT: A UNIT resolver type. A UNIT resolver is the default resolver type. You can use a UNIT resolver to
* run a GraphQL query against a single data source.
*
*
* -
*
* PIPELINE: A PIPELINE resolver type. You can use a PIPELINE resolver to invoke a series of
* Function
objects in a serial manner. You can use a pipeline resolver to run a GraphQL query against
* multiple data sources.
*
*
*
*/
private String kind;
/**
*
* The PipelineConfig
.
*
*/
private PipelineConfig pipelineConfig;
/**
*
* The SyncConfig
for a resolver attached to a versioned data source.
*
*/
private SyncConfig syncConfig;
/**
*
* The caching configuration for the resolver.
*
*/
private CachingConfig cachingConfig;
/**
*
* The maximum batching size for a resolver.
*
*/
private Integer maxBatchSize;
private AppSyncRuntime runtime;
/**
*
* The resolver
code that contains the request and response functions. When code is used, the
* runtime
is required. The runtime
value must be APPSYNC_JS
.
*
*/
private String code;
/**
*
* Enables or disables enhanced resolver metrics for specified resolvers. Note that metricsConfig
won't
* be used unless the resolverLevelMetricsBehavior
value is set to PER_RESOLVER_METRICS
.
* If the resolverLevelMetricsBehavior
is set to FULL_REQUEST_RESOLVER_METRICS
instead,
* metricsConfig
will be ignored. However, you can still set its value.
*
*
* metricsConfig
can be ENABLED
or DISABLED
.
*
*/
private String metricsConfig;
/**
*
* The resolver type name.
*
*
* @param typeName
* The resolver type name.
*/
public void setTypeName(String typeName) {
this.typeName = typeName;
}
/**
*
* The resolver type name.
*
*
* @return The resolver type name.
*/
public String getTypeName() {
return this.typeName;
}
/**
*
* The resolver type name.
*
*
* @param typeName
* The resolver type name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Resolver withTypeName(String typeName) {
setTypeName(typeName);
return this;
}
/**
*
* The resolver field name.
*
*
* @param fieldName
* The resolver field name.
*/
public void setFieldName(String fieldName) {
this.fieldName = fieldName;
}
/**
*
* The resolver field name.
*
*
* @return The resolver field name.
*/
public String getFieldName() {
return this.fieldName;
}
/**
*
* The resolver field name.
*
*
* @param fieldName
* The resolver field name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Resolver withFieldName(String fieldName) {
setFieldName(fieldName);
return this;
}
/**
*
* The resolver data source name.
*
*
* @param dataSourceName
* The resolver data source name.
*/
public void setDataSourceName(String dataSourceName) {
this.dataSourceName = dataSourceName;
}
/**
*
* The resolver data source name.
*
*
* @return The resolver data source name.
*/
public String getDataSourceName() {
return this.dataSourceName;
}
/**
*
* The resolver data source name.
*
*
* @param dataSourceName
* The resolver data source name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Resolver withDataSourceName(String dataSourceName) {
setDataSourceName(dataSourceName);
return this;
}
/**
*
* The resolver Amazon Resource Name (ARN).
*
*
* @param resolverArn
* The resolver Amazon Resource Name (ARN).
*/
public void setResolverArn(String resolverArn) {
this.resolverArn = resolverArn;
}
/**
*
* The resolver Amazon Resource Name (ARN).
*
*
* @return The resolver Amazon Resource Name (ARN).
*/
public String getResolverArn() {
return this.resolverArn;
}
/**
*
* The resolver Amazon Resource Name (ARN).
*
*
* @param resolverArn
* The resolver Amazon Resource Name (ARN).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Resolver withResolverArn(String resolverArn) {
setResolverArn(resolverArn);
return this;
}
/**
*
* The request mapping template.
*
*
* @param requestMappingTemplate
* The request mapping template.
*/
public void setRequestMappingTemplate(String requestMappingTemplate) {
this.requestMappingTemplate = requestMappingTemplate;
}
/**
*
* The request mapping template.
*
*
* @return The request mapping template.
*/
public String getRequestMappingTemplate() {
return this.requestMappingTemplate;
}
/**
*
* The request mapping template.
*
*
* @param requestMappingTemplate
* The request mapping template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Resolver withRequestMappingTemplate(String requestMappingTemplate) {
setRequestMappingTemplate(requestMappingTemplate);
return this;
}
/**
*
* The response mapping template.
*
*
* @param responseMappingTemplate
* The response mapping template.
*/
public void setResponseMappingTemplate(String responseMappingTemplate) {
this.responseMappingTemplate = responseMappingTemplate;
}
/**
*
* The response mapping template.
*
*
* @return The response mapping template.
*/
public String getResponseMappingTemplate() {
return this.responseMappingTemplate;
}
/**
*
* The response mapping template.
*
*
* @param responseMappingTemplate
* The response mapping template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Resolver withResponseMappingTemplate(String responseMappingTemplate) {
setResponseMappingTemplate(responseMappingTemplate);
return this;
}
/**
*
* The resolver type.
*
*
* -
*
* UNIT: A UNIT resolver type. A UNIT resolver is the default resolver type. You can use a UNIT resolver to
* run a GraphQL query against a single data source.
*
*
* -
*
* PIPELINE: A PIPELINE resolver type. You can use a PIPELINE resolver to invoke a series of
* Function
objects in a serial manner. You can use a pipeline resolver to run a GraphQL query against
* multiple data sources.
*
*
*
*
* @param kind
* The resolver type.
*
* -
*
* UNIT: A UNIT resolver type. A UNIT resolver is the default resolver type. You can use a UNIT
* resolver to run a GraphQL query against a single data source.
*
*
* -
*
* PIPELINE: A PIPELINE resolver type. You can use a PIPELINE resolver to invoke a series of
* Function
objects in a serial manner. You can use a pipeline resolver to run a GraphQL query
* against multiple data sources.
*
*
* @see ResolverKind
*/
public void setKind(String kind) {
this.kind = kind;
}
/**
*
* The resolver type.
*
*
* -
*
* UNIT: A UNIT resolver type. A UNIT resolver is the default resolver type. You can use a UNIT resolver to
* run a GraphQL query against a single data source.
*
*
* -
*
* PIPELINE: A PIPELINE resolver type. You can use a PIPELINE resolver to invoke a series of
* Function
objects in a serial manner. You can use a pipeline resolver to run a GraphQL query against
* multiple data sources.
*
*
*
*
* @return The resolver type.
*
* -
*
* UNIT: A UNIT resolver type. A UNIT resolver is the default resolver type. You can use a UNIT
* resolver to run a GraphQL query against a single data source.
*
*
* -
*
* PIPELINE: A PIPELINE resolver type. You can use a PIPELINE resolver to invoke a series of
* Function
objects in a serial manner. You can use a pipeline resolver to run a GraphQL query
* against multiple data sources.
*
*
* @see ResolverKind
*/
public String getKind() {
return this.kind;
}
/**
*
* The resolver type.
*
*
* -
*
* UNIT: A UNIT resolver type. A UNIT resolver is the default resolver type. You can use a UNIT resolver to
* run a GraphQL query against a single data source.
*
*
* -
*
* PIPELINE: A PIPELINE resolver type. You can use a PIPELINE resolver to invoke a series of
* Function
objects in a serial manner. You can use a pipeline resolver to run a GraphQL query against
* multiple data sources.
*
*
*
*
* @param kind
* The resolver type.
*
* -
*
* UNIT: A UNIT resolver type. A UNIT resolver is the default resolver type. You can use a UNIT
* resolver to run a GraphQL query against a single data source.
*
*
* -
*
* PIPELINE: A PIPELINE resolver type. You can use a PIPELINE resolver to invoke a series of
* Function
objects in a serial manner. You can use a pipeline resolver to run a GraphQL query
* against multiple data sources.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResolverKind
*/
public Resolver withKind(String kind) {
setKind(kind);
return this;
}
/**
*
* The resolver type.
*
*
* -
*
* UNIT: A UNIT resolver type. A UNIT resolver is the default resolver type. You can use a UNIT resolver to
* run a GraphQL query against a single data source.
*
*
* -
*
* PIPELINE: A PIPELINE resolver type. You can use a PIPELINE resolver to invoke a series of
* Function
objects in a serial manner. You can use a pipeline resolver to run a GraphQL query against
* multiple data sources.
*
*
*
*
* @param kind
* The resolver type.
*
* -
*
* UNIT: A UNIT resolver type. A UNIT resolver is the default resolver type. You can use a UNIT
* resolver to run a GraphQL query against a single data source.
*
*
* -
*
* PIPELINE: A PIPELINE resolver type. You can use a PIPELINE resolver to invoke a series of
* Function
objects in a serial manner. You can use a pipeline resolver to run a GraphQL query
* against multiple data sources.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResolverKind
*/
public Resolver withKind(ResolverKind kind) {
this.kind = kind.toString();
return this;
}
/**
*
* The PipelineConfig
.
*
*
* @param pipelineConfig
* The PipelineConfig
.
*/
public void setPipelineConfig(PipelineConfig pipelineConfig) {
this.pipelineConfig = pipelineConfig;
}
/**
*
* The PipelineConfig
.
*
*
* @return The PipelineConfig
.
*/
public PipelineConfig getPipelineConfig() {
return this.pipelineConfig;
}
/**
*
* The PipelineConfig
.
*
*
* @param pipelineConfig
* The PipelineConfig
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Resolver withPipelineConfig(PipelineConfig pipelineConfig) {
setPipelineConfig(pipelineConfig);
return this;
}
/**
*
* The SyncConfig
for a resolver attached to a versioned data source.
*
*
* @param syncConfig
* The SyncConfig
for a resolver attached to a versioned data source.
*/
public void setSyncConfig(SyncConfig syncConfig) {
this.syncConfig = syncConfig;
}
/**
*
* The SyncConfig
for a resolver attached to a versioned data source.
*
*
* @return The SyncConfig
for a resolver attached to a versioned data source.
*/
public SyncConfig getSyncConfig() {
return this.syncConfig;
}
/**
*
* The SyncConfig
for a resolver attached to a versioned data source.
*
*
* @param syncConfig
* The SyncConfig
for a resolver attached to a versioned data source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Resolver withSyncConfig(SyncConfig syncConfig) {
setSyncConfig(syncConfig);
return this;
}
/**
*
* The caching configuration for the resolver.
*
*
* @param cachingConfig
* The caching configuration for the resolver.
*/
public void setCachingConfig(CachingConfig cachingConfig) {
this.cachingConfig = cachingConfig;
}
/**
*
* The caching configuration for the resolver.
*
*
* @return The caching configuration for the resolver.
*/
public CachingConfig getCachingConfig() {
return this.cachingConfig;
}
/**
*
* The caching configuration for the resolver.
*
*
* @param cachingConfig
* The caching configuration for the resolver.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Resolver withCachingConfig(CachingConfig cachingConfig) {
setCachingConfig(cachingConfig);
return this;
}
/**
*
* The maximum batching size for a resolver.
*
*
* @param maxBatchSize
* The maximum batching size for a resolver.
*/
public void setMaxBatchSize(Integer maxBatchSize) {
this.maxBatchSize = maxBatchSize;
}
/**
*
* The maximum batching size for a resolver.
*
*
* @return The maximum batching size for a resolver.
*/
public Integer getMaxBatchSize() {
return this.maxBatchSize;
}
/**
*
* The maximum batching size for a resolver.
*
*
* @param maxBatchSize
* The maximum batching size for a resolver.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Resolver withMaxBatchSize(Integer maxBatchSize) {
setMaxBatchSize(maxBatchSize);
return this;
}
/**
* @param runtime
*/
public void setRuntime(AppSyncRuntime runtime) {
this.runtime = runtime;
}
/**
* @return
*/
public AppSyncRuntime getRuntime() {
return this.runtime;
}
/**
* @param runtime
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Resolver withRuntime(AppSyncRuntime runtime) {
setRuntime(runtime);
return this;
}
/**
*
* The resolver
code that contains the request and response functions. When code is used, the
* runtime
is required. The runtime
value must be APPSYNC_JS
.
*
*
* @param code
* The resolver
code that contains the request and response functions. When code is used, the
* runtime
is required. The runtime
value must be APPSYNC_JS
.
*/
public void setCode(String code) {
this.code = code;
}
/**
*
* The resolver
code that contains the request and response functions. When code is used, the
* runtime
is required. The runtime
value must be APPSYNC_JS
.
*
*
* @return The resolver
code that contains the request and response functions. When code is used, the
* runtime
is required. The runtime
value must be APPSYNC_JS
.
*/
public String getCode() {
return this.code;
}
/**
*
* The resolver
code that contains the request and response functions. When code is used, the
* runtime
is required. The runtime
value must be APPSYNC_JS
.
*
*
* @param code
* The resolver
code that contains the request and response functions. When code is used, the
* runtime
is required. The runtime
value must be APPSYNC_JS
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Resolver withCode(String code) {
setCode(code);
return this;
}
/**
*
* Enables or disables enhanced resolver metrics for specified resolvers. Note that metricsConfig
won't
* be used unless the resolverLevelMetricsBehavior
value is set to PER_RESOLVER_METRICS
.
* If the resolverLevelMetricsBehavior
is set to FULL_REQUEST_RESOLVER_METRICS
instead,
* metricsConfig
will be ignored. However, you can still set its value.
*
*
* metricsConfig
can be ENABLED
or DISABLED
.
*
*
* @param metricsConfig
* Enables or disables enhanced resolver metrics for specified resolvers. Note that
* metricsConfig
won't be used unless the resolverLevelMetricsBehavior
value is set
* to PER_RESOLVER_METRICS
. If the resolverLevelMetricsBehavior
is set to
* FULL_REQUEST_RESOLVER_METRICS
instead, metricsConfig
will be ignored. However,
* you can still set its value.
*
* metricsConfig
can be ENABLED
or DISABLED
.
* @see ResolverLevelMetricsConfig
*/
public void setMetricsConfig(String metricsConfig) {
this.metricsConfig = metricsConfig;
}
/**
*
* Enables or disables enhanced resolver metrics for specified resolvers. Note that metricsConfig
won't
* be used unless the resolverLevelMetricsBehavior
value is set to PER_RESOLVER_METRICS
.
* If the resolverLevelMetricsBehavior
is set to FULL_REQUEST_RESOLVER_METRICS
instead,
* metricsConfig
will be ignored. However, you can still set its value.
*
*
* metricsConfig
can be ENABLED
or DISABLED
.
*
*
* @return Enables or disables enhanced resolver metrics for specified resolvers. Note that
* metricsConfig
won't be used unless the resolverLevelMetricsBehavior
value is
* set to PER_RESOLVER_METRICS
. If the resolverLevelMetricsBehavior
is set to
* FULL_REQUEST_RESOLVER_METRICS
instead, metricsConfig
will be ignored. However,
* you can still set its value.
*
* metricsConfig
can be ENABLED
or DISABLED
.
* @see ResolverLevelMetricsConfig
*/
public String getMetricsConfig() {
return this.metricsConfig;
}
/**
*
* Enables or disables enhanced resolver metrics for specified resolvers. Note that metricsConfig
won't
* be used unless the resolverLevelMetricsBehavior
value is set to PER_RESOLVER_METRICS
.
* If the resolverLevelMetricsBehavior
is set to FULL_REQUEST_RESOLVER_METRICS
instead,
* metricsConfig
will be ignored. However, you can still set its value.
*
*
* metricsConfig
can be ENABLED
or DISABLED
.
*
*
* @param metricsConfig
* Enables or disables enhanced resolver metrics for specified resolvers. Note that
* metricsConfig
won't be used unless the resolverLevelMetricsBehavior
value is set
* to PER_RESOLVER_METRICS
. If the resolverLevelMetricsBehavior
is set to
* FULL_REQUEST_RESOLVER_METRICS
instead, metricsConfig
will be ignored. However,
* you can still set its value.
*
* metricsConfig
can be ENABLED
or DISABLED
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResolverLevelMetricsConfig
*/
public Resolver withMetricsConfig(String metricsConfig) {
setMetricsConfig(metricsConfig);
return this;
}
/**
*
* Enables or disables enhanced resolver metrics for specified resolvers. Note that metricsConfig
won't
* be used unless the resolverLevelMetricsBehavior
value is set to PER_RESOLVER_METRICS
.
* If the resolverLevelMetricsBehavior
is set to FULL_REQUEST_RESOLVER_METRICS
instead,
* metricsConfig
will be ignored. However, you can still set its value.
*
*
* metricsConfig
can be ENABLED
or DISABLED
.
*
*
* @param metricsConfig
* Enables or disables enhanced resolver metrics for specified resolvers. Note that
* metricsConfig
won't be used unless the resolverLevelMetricsBehavior
value is set
* to PER_RESOLVER_METRICS
. If the resolverLevelMetricsBehavior
is set to
* FULL_REQUEST_RESOLVER_METRICS
instead, metricsConfig
will be ignored. However,
* you can still set its value.
*
* metricsConfig
can be ENABLED
or DISABLED
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResolverLevelMetricsConfig
*/
public Resolver withMetricsConfig(ResolverLevelMetricsConfig metricsConfig) {
this.metricsConfig = metricsConfig.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTypeName() != null)
sb.append("TypeName: ").append(getTypeName()).append(",");
if (getFieldName() != null)
sb.append("FieldName: ").append(getFieldName()).append(",");
if (getDataSourceName() != null)
sb.append("DataSourceName: ").append(getDataSourceName()).append(",");
if (getResolverArn() != null)
sb.append("ResolverArn: ").append(getResolverArn()).append(",");
if (getRequestMappingTemplate() != null)
sb.append("RequestMappingTemplate: ").append(getRequestMappingTemplate()).append(",");
if (getResponseMappingTemplate() != null)
sb.append("ResponseMappingTemplate: ").append(getResponseMappingTemplate()).append(",");
if (getKind() != null)
sb.append("Kind: ").append(getKind()).append(",");
if (getPipelineConfig() != null)
sb.append("PipelineConfig: ").append(getPipelineConfig()).append(",");
if (getSyncConfig() != null)
sb.append("SyncConfig: ").append(getSyncConfig()).append(",");
if (getCachingConfig() != null)
sb.append("CachingConfig: ").append(getCachingConfig()).append(",");
if (getMaxBatchSize() != null)
sb.append("MaxBatchSize: ").append(getMaxBatchSize()).append(",");
if (getRuntime() != null)
sb.append("Runtime: ").append(getRuntime()).append(",");
if (getCode() != null)
sb.append("Code: ").append(getCode()).append(",");
if (getMetricsConfig() != null)
sb.append("MetricsConfig: ").append(getMetricsConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Resolver == false)
return false;
Resolver other = (Resolver) obj;
if (other.getTypeName() == null ^ this.getTypeName() == null)
return false;
if (other.getTypeName() != null && other.getTypeName().equals(this.getTypeName()) == false)
return false;
if (other.getFieldName() == null ^ this.getFieldName() == null)
return false;
if (other.getFieldName() != null && other.getFieldName().equals(this.getFieldName()) == false)
return false;
if (other.getDataSourceName() == null ^ this.getDataSourceName() == null)
return false;
if (other.getDataSourceName() != null && other.getDataSourceName().equals(this.getDataSourceName()) == false)
return false;
if (other.getResolverArn() == null ^ this.getResolverArn() == null)
return false;
if (other.getResolverArn() != null && other.getResolverArn().equals(this.getResolverArn()) == false)
return false;
if (other.getRequestMappingTemplate() == null ^ this.getRequestMappingTemplate() == null)
return false;
if (other.getRequestMappingTemplate() != null && other.getRequestMappingTemplate().equals(this.getRequestMappingTemplate()) == false)
return false;
if (other.getResponseMappingTemplate() == null ^ this.getResponseMappingTemplate() == null)
return false;
if (other.getResponseMappingTemplate() != null && other.getResponseMappingTemplate().equals(this.getResponseMappingTemplate()) == false)
return false;
if (other.getKind() == null ^ this.getKind() == null)
return false;
if (other.getKind() != null && other.getKind().equals(this.getKind()) == false)
return false;
if (other.getPipelineConfig() == null ^ this.getPipelineConfig() == null)
return false;
if (other.getPipelineConfig() != null && other.getPipelineConfig().equals(this.getPipelineConfig()) == false)
return false;
if (other.getSyncConfig() == null ^ this.getSyncConfig() == null)
return false;
if (other.getSyncConfig() != null && other.getSyncConfig().equals(this.getSyncConfig()) == false)
return false;
if (other.getCachingConfig() == null ^ this.getCachingConfig() == null)
return false;
if (other.getCachingConfig() != null && other.getCachingConfig().equals(this.getCachingConfig()) == false)
return false;
if (other.getMaxBatchSize() == null ^ this.getMaxBatchSize() == null)
return false;
if (other.getMaxBatchSize() != null && other.getMaxBatchSize().equals(this.getMaxBatchSize()) == false)
return false;
if (other.getRuntime() == null ^ this.getRuntime() == null)
return false;
if (other.getRuntime() != null && other.getRuntime().equals(this.getRuntime()) == false)
return false;
if (other.getCode() == null ^ this.getCode() == null)
return false;
if (other.getCode() != null && other.getCode().equals(this.getCode()) == false)
return false;
if (other.getMetricsConfig() == null ^ this.getMetricsConfig() == null)
return false;
if (other.getMetricsConfig() != null && other.getMetricsConfig().equals(this.getMetricsConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTypeName() == null) ? 0 : getTypeName().hashCode());
hashCode = prime * hashCode + ((getFieldName() == null) ? 0 : getFieldName().hashCode());
hashCode = prime * hashCode + ((getDataSourceName() == null) ? 0 : getDataSourceName().hashCode());
hashCode = prime * hashCode + ((getResolverArn() == null) ? 0 : getResolverArn().hashCode());
hashCode = prime * hashCode + ((getRequestMappingTemplate() == null) ? 0 : getRequestMappingTemplate().hashCode());
hashCode = prime * hashCode + ((getResponseMappingTemplate() == null) ? 0 : getResponseMappingTemplate().hashCode());
hashCode = prime * hashCode + ((getKind() == null) ? 0 : getKind().hashCode());
hashCode = prime * hashCode + ((getPipelineConfig() == null) ? 0 : getPipelineConfig().hashCode());
hashCode = prime * hashCode + ((getSyncConfig() == null) ? 0 : getSyncConfig().hashCode());
hashCode = prime * hashCode + ((getCachingConfig() == null) ? 0 : getCachingConfig().hashCode());
hashCode = prime * hashCode + ((getMaxBatchSize() == null) ? 0 : getMaxBatchSize().hashCode());
hashCode = prime * hashCode + ((getRuntime() == null) ? 0 : getRuntime().hashCode());
hashCode = prime * hashCode + ((getCode() == null) ? 0 : getCode().hashCode());
hashCode = prime * hashCode + ((getMetricsConfig() == null) ? 0 : getMetricsConfig().hashCode());
return hashCode;
}
@Override
public Resolver clone() {
try {
return (Resolver) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.appsync.model.transform.ResolverMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}