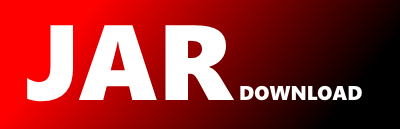
com.amazonaws.services.arczonalshift.model.ZonalShiftSummary Maven / Gradle / Ivy
Show all versions of aws-java-sdk-arczonalshift Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.arczonalshift.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Lists information about zonal shifts in Amazon Route 53 Application Recovery Controller, including zonal shifts that
* you start yourself and zonal shifts that Route 53 ARC starts on your behalf for practice runs with zonal autoshift.
*
*
* Zonal shifts are temporary, including customer-initiated zonal shifts and the zonal autoshift practice run zonal
* shifts that Route 53 ARC starts weekly, on your behalf. A zonal shift that a customer starts can be active for up to
* three days (72 hours). A practice run zonal shift has a 30 minute duration.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ZonalShiftSummary implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Availability Zone (for example, use1-az1
) that traffic is moved away from for a resource when
* you start a zonal shift. Until the zonal shift expires or you cancel it, traffic for the resource is instead
* moved to other Availability Zones in the Amazon Web Services Region.
*
*/
private String awayFrom;
/**
*
* A comment that you enter about the zonal shift. Only the latest comment is retained; no comment history is
* maintained. That is, a new comment overwrites any existing comment string.
*
*/
private String comment;
/**
*
* The expiry time (expiration time) for a customer-initiated zonal shift. A zonal shift is temporary and must be
* set to expire when you start the zonal shift. You can initially set a zonal shift to expire in a maximum of three
* days (72 hours). However, you can update a zonal shift to set a new expiration at any time.
*
*
* When you start a zonal shift, you specify how long you want it to be active, which Route 53 ARC converts to an
* expiry time (expiration time). You can cancel a zonal shift when you're ready to restore traffic to the
* Availability Zone, or just wait for it to expire. Or you can update the zonal shift to specify another length of
* time to expire in.
*
*/
private java.util.Date expiryTime;
/**
*
* The outcome, or end state, of a practice run. The following values can be returned:
*
*
* -
*
* PENDING: Outcome value when the practice run is in progress.
*
*
* -
*
* SUCCEEDED: Outcome value when the outcome alarm specified for the practice run configuration does not go
* into an ALARM
state during the practice run, and the practice run was not interrupted before it
* completed.
*
*
* -
*
* INTERRUPTED: Outcome value when the practice run did not run for the expected 30 minutes or there was
* another problem with the practice run that created an inconclusive outcome.
*
*
* -
*
* FAILED: Outcome value when the outcome alarm specified for the practice run configuration goes into an
* ALARM
state during the practice run, and the practice run was not interrupted before it completed.
*
*
*
*
* For more information about practice run outcomes, see Considerations when
* you configure zonal autoshift in the Amazon Route 53 Application Recovery Controller Developer Guide.
*
*/
private String practiceRunOutcome;
/**
*
* The identifier for the resource to include in a zonal shift. The identifier is the Amazon Resource Name (ARN) for
* the resource.
*
*
* At this time, you can only start a zonal shift for Network Load Balancers and Application Load Balancers with
* cross-zone load balancing turned off.
*
*/
private String resourceIdentifier;
/**
*
* The time (UTC) when the zonal shift starts.
*
*/
private java.util.Date startTime;
/**
*
* A status for a zonal shift.
*
*
* The Status
for a zonal shift can have one of the following values:
*
*
* -
*
* ACTIVE: The zonal shift has been started and active.
*
*
* -
*
* EXPIRED: The zonal shift has expired (the expiry time was exceeded).
*
*
* -
*
* CANCELED: The zonal shift was canceled.
*
*
*
*/
private String status;
/**
*
* The identifier of a zonal shift.
*
*/
private String zonalShiftId;
/**
*
* The Availability Zone (for example, use1-az1
) that traffic is moved away from for a resource when
* you start a zonal shift. Until the zonal shift expires or you cancel it, traffic for the resource is instead
* moved to other Availability Zones in the Amazon Web Services Region.
*
*
* @param awayFrom
* The Availability Zone (for example, use1-az1
) that traffic is moved away from for a resource
* when you start a zonal shift. Until the zonal shift expires or you cancel it, traffic for the resource is
* instead moved to other Availability Zones in the Amazon Web Services Region.
*/
public void setAwayFrom(String awayFrom) {
this.awayFrom = awayFrom;
}
/**
*
* The Availability Zone (for example, use1-az1
) that traffic is moved away from for a resource when
* you start a zonal shift. Until the zonal shift expires or you cancel it, traffic for the resource is instead
* moved to other Availability Zones in the Amazon Web Services Region.
*
*
* @return The Availability Zone (for example, use1-az1
) that traffic is moved away from for a resource
* when you start a zonal shift. Until the zonal shift expires or you cancel it, traffic for the resource is
* instead moved to other Availability Zones in the Amazon Web Services Region.
*/
public String getAwayFrom() {
return this.awayFrom;
}
/**
*
* The Availability Zone (for example, use1-az1
) that traffic is moved away from for a resource when
* you start a zonal shift. Until the zonal shift expires or you cancel it, traffic for the resource is instead
* moved to other Availability Zones in the Amazon Web Services Region.
*
*
* @param awayFrom
* The Availability Zone (for example, use1-az1
) that traffic is moved away from for a resource
* when you start a zonal shift. Until the zonal shift expires or you cancel it, traffic for the resource is
* instead moved to other Availability Zones in the Amazon Web Services Region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ZonalShiftSummary withAwayFrom(String awayFrom) {
setAwayFrom(awayFrom);
return this;
}
/**
*
* A comment that you enter about the zonal shift. Only the latest comment is retained; no comment history is
* maintained. That is, a new comment overwrites any existing comment string.
*
*
* @param comment
* A comment that you enter about the zonal shift. Only the latest comment is retained; no comment history is
* maintained. That is, a new comment overwrites any existing comment string.
*/
public void setComment(String comment) {
this.comment = comment;
}
/**
*
* A comment that you enter about the zonal shift. Only the latest comment is retained; no comment history is
* maintained. That is, a new comment overwrites any existing comment string.
*
*
* @return A comment that you enter about the zonal shift. Only the latest comment is retained; no comment history
* is maintained. That is, a new comment overwrites any existing comment string.
*/
public String getComment() {
return this.comment;
}
/**
*
* A comment that you enter about the zonal shift. Only the latest comment is retained; no comment history is
* maintained. That is, a new comment overwrites any existing comment string.
*
*
* @param comment
* A comment that you enter about the zonal shift. Only the latest comment is retained; no comment history is
* maintained. That is, a new comment overwrites any existing comment string.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ZonalShiftSummary withComment(String comment) {
setComment(comment);
return this;
}
/**
*
* The expiry time (expiration time) for a customer-initiated zonal shift. A zonal shift is temporary and must be
* set to expire when you start the zonal shift. You can initially set a zonal shift to expire in a maximum of three
* days (72 hours). However, you can update a zonal shift to set a new expiration at any time.
*
*
* When you start a zonal shift, you specify how long you want it to be active, which Route 53 ARC converts to an
* expiry time (expiration time). You can cancel a zonal shift when you're ready to restore traffic to the
* Availability Zone, or just wait for it to expire. Or you can update the zonal shift to specify another length of
* time to expire in.
*
*
* @param expiryTime
* The expiry time (expiration time) for a customer-initiated zonal shift. A zonal shift is temporary and
* must be set to expire when you start the zonal shift. You can initially set a zonal shift to expire in a
* maximum of three days (72 hours). However, you can update a zonal shift to set a new expiration at any
* time.
*
* When you start a zonal shift, you specify how long you want it to be active, which Route 53 ARC converts
* to an expiry time (expiration time). You can cancel a zonal shift when you're ready to restore traffic to
* the Availability Zone, or just wait for it to expire. Or you can update the zonal shift to specify another
* length of time to expire in.
*/
public void setExpiryTime(java.util.Date expiryTime) {
this.expiryTime = expiryTime;
}
/**
*
* The expiry time (expiration time) for a customer-initiated zonal shift. A zonal shift is temporary and must be
* set to expire when you start the zonal shift. You can initially set a zonal shift to expire in a maximum of three
* days (72 hours). However, you can update a zonal shift to set a new expiration at any time.
*
*
* When you start a zonal shift, you specify how long you want it to be active, which Route 53 ARC converts to an
* expiry time (expiration time). You can cancel a zonal shift when you're ready to restore traffic to the
* Availability Zone, or just wait for it to expire. Or you can update the zonal shift to specify another length of
* time to expire in.
*
*
* @return The expiry time (expiration time) for a customer-initiated zonal shift. A zonal shift is temporary and
* must be set to expire when you start the zonal shift. You can initially set a zonal shift to expire in a
* maximum of three days (72 hours). However, you can update a zonal shift to set a new expiration at any
* time.
*
* When you start a zonal shift, you specify how long you want it to be active, which Route 53 ARC converts
* to an expiry time (expiration time). You can cancel a zonal shift when you're ready to restore traffic to
* the Availability Zone, or just wait for it to expire. Or you can update the zonal shift to specify
* another length of time to expire in.
*/
public java.util.Date getExpiryTime() {
return this.expiryTime;
}
/**
*
* The expiry time (expiration time) for a customer-initiated zonal shift. A zonal shift is temporary and must be
* set to expire when you start the zonal shift. You can initially set a zonal shift to expire in a maximum of three
* days (72 hours). However, you can update a zonal shift to set a new expiration at any time.
*
*
* When you start a zonal shift, you specify how long you want it to be active, which Route 53 ARC converts to an
* expiry time (expiration time). You can cancel a zonal shift when you're ready to restore traffic to the
* Availability Zone, or just wait for it to expire. Or you can update the zonal shift to specify another length of
* time to expire in.
*
*
* @param expiryTime
* The expiry time (expiration time) for a customer-initiated zonal shift. A zonal shift is temporary and
* must be set to expire when you start the zonal shift. You can initially set a zonal shift to expire in a
* maximum of three days (72 hours). However, you can update a zonal shift to set a new expiration at any
* time.
*
* When you start a zonal shift, you specify how long you want it to be active, which Route 53 ARC converts
* to an expiry time (expiration time). You can cancel a zonal shift when you're ready to restore traffic to
* the Availability Zone, or just wait for it to expire. Or you can update the zonal shift to specify another
* length of time to expire in.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ZonalShiftSummary withExpiryTime(java.util.Date expiryTime) {
setExpiryTime(expiryTime);
return this;
}
/**
*
* The outcome, or end state, of a practice run. The following values can be returned:
*
*
* -
*
* PENDING: Outcome value when the practice run is in progress.
*
*
* -
*
* SUCCEEDED: Outcome value when the outcome alarm specified for the practice run configuration does not go
* into an ALARM
state during the practice run, and the practice run was not interrupted before it
* completed.
*
*
* -
*
* INTERRUPTED: Outcome value when the practice run did not run for the expected 30 minutes or there was
* another problem with the practice run that created an inconclusive outcome.
*
*
* -
*
* FAILED: Outcome value when the outcome alarm specified for the practice run configuration goes into an
* ALARM
state during the practice run, and the practice run was not interrupted before it completed.
*
*
*
*
* For more information about practice run outcomes, see Considerations when
* you configure zonal autoshift in the Amazon Route 53 Application Recovery Controller Developer Guide.
*
*
* @param practiceRunOutcome
* The outcome, or end state, of a practice run. The following values can be returned:
*
* -
*
* PENDING: Outcome value when the practice run is in progress.
*
*
* -
*
* SUCCEEDED: Outcome value when the outcome alarm specified for the practice run configuration does
* not go into an ALARM
state during the practice run, and the practice run was not interrupted
* before it completed.
*
*
* -
*
* INTERRUPTED: Outcome value when the practice run did not run for the expected 30 minutes or there
* was another problem with the practice run that created an inconclusive outcome.
*
*
* -
*
* FAILED: Outcome value when the outcome alarm specified for the practice run configuration goes into
* an ALARM
state during the practice run, and the practice run was not interrupted before it
* completed.
*
*
*
*
* For more information about practice run outcomes, see
* Considerations when you configure zonal autoshift in the Amazon Route 53 Application Recovery
* Controller Developer Guide.
* @see PracticeRunOutcome
*/
public void setPracticeRunOutcome(String practiceRunOutcome) {
this.practiceRunOutcome = practiceRunOutcome;
}
/**
*
* The outcome, or end state, of a practice run. The following values can be returned:
*
*
* -
*
* PENDING: Outcome value when the practice run is in progress.
*
*
* -
*
* SUCCEEDED: Outcome value when the outcome alarm specified for the practice run configuration does not go
* into an ALARM
state during the practice run, and the practice run was not interrupted before it
* completed.
*
*
* -
*
* INTERRUPTED: Outcome value when the practice run did not run for the expected 30 minutes or there was
* another problem with the practice run that created an inconclusive outcome.
*
*
* -
*
* FAILED: Outcome value when the outcome alarm specified for the practice run configuration goes into an
* ALARM
state during the practice run, and the practice run was not interrupted before it completed.
*
*
*
*
* For more information about practice run outcomes, see Considerations when
* you configure zonal autoshift in the Amazon Route 53 Application Recovery Controller Developer Guide.
*
*
* @return The outcome, or end state, of a practice run. The following values can be returned:
*
* -
*
* PENDING: Outcome value when the practice run is in progress.
*
*
* -
*
* SUCCEEDED: Outcome value when the outcome alarm specified for the practice run configuration does
* not go into an ALARM
state during the practice run, and the practice run was not interrupted
* before it completed.
*
*
* -
*
* INTERRUPTED: Outcome value when the practice run did not run for the expected 30 minutes or there
* was another problem with the practice run that created an inconclusive outcome.
*
*
* -
*
* FAILED: Outcome value when the outcome alarm specified for the practice run configuration goes
* into an ALARM
state during the practice run, and the practice run was not interrupted before
* it completed.
*
*
*
*
* For more information about practice run outcomes, see
* Considerations when you configure zonal autoshift in the Amazon Route 53 Application Recovery
* Controller Developer Guide.
* @see PracticeRunOutcome
*/
public String getPracticeRunOutcome() {
return this.practiceRunOutcome;
}
/**
*
* The outcome, or end state, of a practice run. The following values can be returned:
*
*
* -
*
* PENDING: Outcome value when the practice run is in progress.
*
*
* -
*
* SUCCEEDED: Outcome value when the outcome alarm specified for the practice run configuration does not go
* into an ALARM
state during the practice run, and the practice run was not interrupted before it
* completed.
*
*
* -
*
* INTERRUPTED: Outcome value when the practice run did not run for the expected 30 minutes or there was
* another problem with the practice run that created an inconclusive outcome.
*
*
* -
*
* FAILED: Outcome value when the outcome alarm specified for the practice run configuration goes into an
* ALARM
state during the practice run, and the practice run was not interrupted before it completed.
*
*
*
*
* For more information about practice run outcomes, see Considerations when
* you configure zonal autoshift in the Amazon Route 53 Application Recovery Controller Developer Guide.
*
*
* @param practiceRunOutcome
* The outcome, or end state, of a practice run. The following values can be returned:
*
* -
*
* PENDING: Outcome value when the practice run is in progress.
*
*
* -
*
* SUCCEEDED: Outcome value when the outcome alarm specified for the practice run configuration does
* not go into an ALARM
state during the practice run, and the practice run was not interrupted
* before it completed.
*
*
* -
*
* INTERRUPTED: Outcome value when the practice run did not run for the expected 30 minutes or there
* was another problem with the practice run that created an inconclusive outcome.
*
*
* -
*
* FAILED: Outcome value when the outcome alarm specified for the practice run configuration goes into
* an ALARM
state during the practice run, and the practice run was not interrupted before it
* completed.
*
*
*
*
* For more information about practice run outcomes, see
* Considerations when you configure zonal autoshift in the Amazon Route 53 Application Recovery
* Controller Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PracticeRunOutcome
*/
public ZonalShiftSummary withPracticeRunOutcome(String practiceRunOutcome) {
setPracticeRunOutcome(practiceRunOutcome);
return this;
}
/**
*
* The outcome, or end state, of a practice run. The following values can be returned:
*
*
* -
*
* PENDING: Outcome value when the practice run is in progress.
*
*
* -
*
* SUCCEEDED: Outcome value when the outcome alarm specified for the practice run configuration does not go
* into an ALARM
state during the practice run, and the practice run was not interrupted before it
* completed.
*
*
* -
*
* INTERRUPTED: Outcome value when the practice run did not run for the expected 30 minutes or there was
* another problem with the practice run that created an inconclusive outcome.
*
*
* -
*
* FAILED: Outcome value when the outcome alarm specified for the practice run configuration goes into an
* ALARM
state during the practice run, and the practice run was not interrupted before it completed.
*
*
*
*
* For more information about practice run outcomes, see Considerations when
* you configure zonal autoshift in the Amazon Route 53 Application Recovery Controller Developer Guide.
*
*
* @param practiceRunOutcome
* The outcome, or end state, of a practice run. The following values can be returned:
*
* -
*
* PENDING: Outcome value when the practice run is in progress.
*
*
* -
*
* SUCCEEDED: Outcome value when the outcome alarm specified for the practice run configuration does
* not go into an ALARM
state during the practice run, and the practice run was not interrupted
* before it completed.
*
*
* -
*
* INTERRUPTED: Outcome value when the practice run did not run for the expected 30 minutes or there
* was another problem with the practice run that created an inconclusive outcome.
*
*
* -
*
* FAILED: Outcome value when the outcome alarm specified for the practice run configuration goes into
* an ALARM
state during the practice run, and the practice run was not interrupted before it
* completed.
*
*
*
*
* For more information about practice run outcomes, see
* Considerations when you configure zonal autoshift in the Amazon Route 53 Application Recovery
* Controller Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PracticeRunOutcome
*/
public ZonalShiftSummary withPracticeRunOutcome(PracticeRunOutcome practiceRunOutcome) {
this.practiceRunOutcome = practiceRunOutcome.toString();
return this;
}
/**
*
* The identifier for the resource to include in a zonal shift. The identifier is the Amazon Resource Name (ARN) for
* the resource.
*
*
* At this time, you can only start a zonal shift for Network Load Balancers and Application Load Balancers with
* cross-zone load balancing turned off.
*
*
* @param resourceIdentifier
* The identifier for the resource to include in a zonal shift. The identifier is the Amazon Resource Name
* (ARN) for the resource.
*
* At this time, you can only start a zonal shift for Network Load Balancers and Application Load Balancers
* with cross-zone load balancing turned off.
*/
public void setResourceIdentifier(String resourceIdentifier) {
this.resourceIdentifier = resourceIdentifier;
}
/**
*
* The identifier for the resource to include in a zonal shift. The identifier is the Amazon Resource Name (ARN) for
* the resource.
*
*
* At this time, you can only start a zonal shift for Network Load Balancers and Application Load Balancers with
* cross-zone load balancing turned off.
*
*
* @return The identifier for the resource to include in a zonal shift. The identifier is the Amazon Resource Name
* (ARN) for the resource.
*
* At this time, you can only start a zonal shift for Network Load Balancers and Application Load Balancers
* with cross-zone load balancing turned off.
*/
public String getResourceIdentifier() {
return this.resourceIdentifier;
}
/**
*
* The identifier for the resource to include in a zonal shift. The identifier is the Amazon Resource Name (ARN) for
* the resource.
*
*
* At this time, you can only start a zonal shift for Network Load Balancers and Application Load Balancers with
* cross-zone load balancing turned off.
*
*
* @param resourceIdentifier
* The identifier for the resource to include in a zonal shift. The identifier is the Amazon Resource Name
* (ARN) for the resource.
*
* At this time, you can only start a zonal shift for Network Load Balancers and Application Load Balancers
* with cross-zone load balancing turned off.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ZonalShiftSummary withResourceIdentifier(String resourceIdentifier) {
setResourceIdentifier(resourceIdentifier);
return this;
}
/**
*
* The time (UTC) when the zonal shift starts.
*
*
* @param startTime
* The time (UTC) when the zonal shift starts.
*/
public void setStartTime(java.util.Date startTime) {
this.startTime = startTime;
}
/**
*
* The time (UTC) when the zonal shift starts.
*
*
* @return The time (UTC) when the zonal shift starts.
*/
public java.util.Date getStartTime() {
return this.startTime;
}
/**
*
* The time (UTC) when the zonal shift starts.
*
*
* @param startTime
* The time (UTC) when the zonal shift starts.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ZonalShiftSummary withStartTime(java.util.Date startTime) {
setStartTime(startTime);
return this;
}
/**
*
* A status for a zonal shift.
*
*
* The Status
for a zonal shift can have one of the following values:
*
*
* -
*
* ACTIVE: The zonal shift has been started and active.
*
*
* -
*
* EXPIRED: The zonal shift has expired (the expiry time was exceeded).
*
*
* -
*
* CANCELED: The zonal shift was canceled.
*
*
*
*
* @param status
* A status for a zonal shift.
*
* The Status
for a zonal shift can have one of the following values:
*
*
* -
*
* ACTIVE: The zonal shift has been started and active.
*
*
* -
*
* EXPIRED: The zonal shift has expired (the expiry time was exceeded).
*
*
* -
*
* CANCELED: The zonal shift was canceled.
*
*
* @see ZonalShiftStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* A status for a zonal shift.
*
*
* The Status
for a zonal shift can have one of the following values:
*
*
* -
*
* ACTIVE: The zonal shift has been started and active.
*
*
* -
*
* EXPIRED: The zonal shift has expired (the expiry time was exceeded).
*
*
* -
*
* CANCELED: The zonal shift was canceled.
*
*
*
*
* @return A status for a zonal shift.
*
* The Status
for a zonal shift can have one of the following values:
*
*
* -
*
* ACTIVE: The zonal shift has been started and active.
*
*
* -
*
* EXPIRED: The zonal shift has expired (the expiry time was exceeded).
*
*
* -
*
* CANCELED: The zonal shift was canceled.
*
*
* @see ZonalShiftStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* A status for a zonal shift.
*
*
* The Status
for a zonal shift can have one of the following values:
*
*
* -
*
* ACTIVE: The zonal shift has been started and active.
*
*
* -
*
* EXPIRED: The zonal shift has expired (the expiry time was exceeded).
*
*
* -
*
* CANCELED: The zonal shift was canceled.
*
*
*
*
* @param status
* A status for a zonal shift.
*
* The Status
for a zonal shift can have one of the following values:
*
*
* -
*
* ACTIVE: The zonal shift has been started and active.
*
*
* -
*
* EXPIRED: The zonal shift has expired (the expiry time was exceeded).
*
*
* -
*
* CANCELED: The zonal shift was canceled.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ZonalShiftStatus
*/
public ZonalShiftSummary withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* A status for a zonal shift.
*
*
* The Status
for a zonal shift can have one of the following values:
*
*
* -
*
* ACTIVE: The zonal shift has been started and active.
*
*
* -
*
* EXPIRED: The zonal shift has expired (the expiry time was exceeded).
*
*
* -
*
* CANCELED: The zonal shift was canceled.
*
*
*
*
* @param status
* A status for a zonal shift.
*
* The Status
for a zonal shift can have one of the following values:
*
*
* -
*
* ACTIVE: The zonal shift has been started and active.
*
*
* -
*
* EXPIRED: The zonal shift has expired (the expiry time was exceeded).
*
*
* -
*
* CANCELED: The zonal shift was canceled.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ZonalShiftStatus
*/
public ZonalShiftSummary withStatus(ZonalShiftStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The identifier of a zonal shift.
*
*
* @param zonalShiftId
* The identifier of a zonal shift.
*/
public void setZonalShiftId(String zonalShiftId) {
this.zonalShiftId = zonalShiftId;
}
/**
*
* The identifier of a zonal shift.
*
*
* @return The identifier of a zonal shift.
*/
public String getZonalShiftId() {
return this.zonalShiftId;
}
/**
*
* The identifier of a zonal shift.
*
*
* @param zonalShiftId
* The identifier of a zonal shift.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ZonalShiftSummary withZonalShiftId(String zonalShiftId) {
setZonalShiftId(zonalShiftId);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAwayFrom() != null)
sb.append("AwayFrom: ").append(getAwayFrom()).append(",");
if (getComment() != null)
sb.append("Comment: ").append(getComment()).append(",");
if (getExpiryTime() != null)
sb.append("ExpiryTime: ").append(getExpiryTime()).append(",");
if (getPracticeRunOutcome() != null)
sb.append("PracticeRunOutcome: ").append(getPracticeRunOutcome()).append(",");
if (getResourceIdentifier() != null)
sb.append("ResourceIdentifier: ").append(getResourceIdentifier()).append(",");
if (getStartTime() != null)
sb.append("StartTime: ").append(getStartTime()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getZonalShiftId() != null)
sb.append("ZonalShiftId: ").append(getZonalShiftId());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ZonalShiftSummary == false)
return false;
ZonalShiftSummary other = (ZonalShiftSummary) obj;
if (other.getAwayFrom() == null ^ this.getAwayFrom() == null)
return false;
if (other.getAwayFrom() != null && other.getAwayFrom().equals(this.getAwayFrom()) == false)
return false;
if (other.getComment() == null ^ this.getComment() == null)
return false;
if (other.getComment() != null && other.getComment().equals(this.getComment()) == false)
return false;
if (other.getExpiryTime() == null ^ this.getExpiryTime() == null)
return false;
if (other.getExpiryTime() != null && other.getExpiryTime().equals(this.getExpiryTime()) == false)
return false;
if (other.getPracticeRunOutcome() == null ^ this.getPracticeRunOutcome() == null)
return false;
if (other.getPracticeRunOutcome() != null && other.getPracticeRunOutcome().equals(this.getPracticeRunOutcome()) == false)
return false;
if (other.getResourceIdentifier() == null ^ this.getResourceIdentifier() == null)
return false;
if (other.getResourceIdentifier() != null && other.getResourceIdentifier().equals(this.getResourceIdentifier()) == false)
return false;
if (other.getStartTime() == null ^ this.getStartTime() == null)
return false;
if (other.getStartTime() != null && other.getStartTime().equals(this.getStartTime()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getZonalShiftId() == null ^ this.getZonalShiftId() == null)
return false;
if (other.getZonalShiftId() != null && other.getZonalShiftId().equals(this.getZonalShiftId()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAwayFrom() == null) ? 0 : getAwayFrom().hashCode());
hashCode = prime * hashCode + ((getComment() == null) ? 0 : getComment().hashCode());
hashCode = prime * hashCode + ((getExpiryTime() == null) ? 0 : getExpiryTime().hashCode());
hashCode = prime * hashCode + ((getPracticeRunOutcome() == null) ? 0 : getPracticeRunOutcome().hashCode());
hashCode = prime * hashCode + ((getResourceIdentifier() == null) ? 0 : getResourceIdentifier().hashCode());
hashCode = prime * hashCode + ((getStartTime() == null) ? 0 : getStartTime().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getZonalShiftId() == null) ? 0 : getZonalShiftId().hashCode());
return hashCode;
}
@Override
public ZonalShiftSummary clone() {
try {
return (ZonalShiftSummary) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.arczonalshift.model.transform.ZonalShiftSummaryMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}