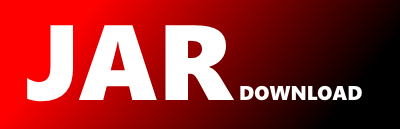
com.amazonaws.services.auditmanager.AWSAuditManagerAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-auditmanager Show documentation
/*
* Copyright 2016-2021 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.auditmanager;
import javax.annotation.Generated;
import com.amazonaws.services.auditmanager.model.*;
/**
* Interface for accessing AWS Audit Manager asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.auditmanager.AbstractAWSAuditManagerAsync} instead.
*
*
*
* Welcome to the AWS Audit Manager API reference. This guide is for developers who need detailed information about the
* AWS Audit Manager API operations, data types, and errors.
*
*
* AWS Audit Manager is a service that provides automated evidence collection so that you can continuously audit your
* AWS usage, and assess the effectiveness of your controls to better manage risk and simplify compliance.
*
*
* AWS Audit Manager provides pre-built frameworks that structure and automate assessments for a given compliance
* standard. Frameworks include a pre-built collection of controls with descriptions and testing procedures, which are
* grouped according to the requirements of the specified compliance standard or regulation. You can also customize
* frameworks and controls to support internal audits with unique requirements.
*
*
* Use the following links to get started with the AWS Audit Manager API:
*
*
* -
*
* Actions: An
* alphabetical list of all AWS Audit Manager API operations.
*
*
* -
*
* Data types: An
* alphabetical list of all AWS Audit Manager data types.
*
*
* -
*
* Common parameters:
* Parameters that all Query operations can use.
*
*
* -
*
* Common errors: Client
* and server errors that all operations can return.
*
*
*
*
* If you're new to AWS Audit Manager, we recommend that you review the AWS Audit Manager User Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSAuditManagerAsync extends AWSAuditManager {
/**
*
* Associates an evidence folder to the specified assessment report in AWS Audit Manager.
*
*
* @param associateAssessmentReportEvidenceFolderRequest
* @return A Java Future containing the result of the AssociateAssessmentReportEvidenceFolder operation returned by
* the service.
* @sample AWSAuditManagerAsync.AssociateAssessmentReportEvidenceFolder
* @see AWS API Documentation
*/
java.util.concurrent.Future associateAssessmentReportEvidenceFolderAsync(
AssociateAssessmentReportEvidenceFolderRequest associateAssessmentReportEvidenceFolderRequest);
/**
*
* Associates an evidence folder to the specified assessment report in AWS Audit Manager.
*
*
* @param associateAssessmentReportEvidenceFolderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateAssessmentReportEvidenceFolder operation returned by
* the service.
* @sample AWSAuditManagerAsyncHandler.AssociateAssessmentReportEvidenceFolder
* @see AWS API Documentation
*/
java.util.concurrent.Future associateAssessmentReportEvidenceFolderAsync(
AssociateAssessmentReportEvidenceFolderRequest associateAssessmentReportEvidenceFolderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a list of evidence to an assessment report in an AWS Audit Manager assessment.
*
*
* @param batchAssociateAssessmentReportEvidenceRequest
* @return A Java Future containing the result of the BatchAssociateAssessmentReportEvidence operation returned by
* the service.
* @sample AWSAuditManagerAsync.BatchAssociateAssessmentReportEvidence
* @see AWS API Documentation
*/
java.util.concurrent.Future batchAssociateAssessmentReportEvidenceAsync(
BatchAssociateAssessmentReportEvidenceRequest batchAssociateAssessmentReportEvidenceRequest);
/**
*
* Associates a list of evidence to an assessment report in an AWS Audit Manager assessment.
*
*
* @param batchAssociateAssessmentReportEvidenceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchAssociateAssessmentReportEvidence operation returned by
* the service.
* @sample AWSAuditManagerAsyncHandler.BatchAssociateAssessmentReportEvidence
* @see AWS API Documentation
*/
java.util.concurrent.Future batchAssociateAssessmentReportEvidenceAsync(
BatchAssociateAssessmentReportEvidenceRequest batchAssociateAssessmentReportEvidenceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create a batch of delegations for a specified assessment in AWS Audit Manager.
*
*
* @param batchCreateDelegationByAssessmentRequest
* @return A Java Future containing the result of the BatchCreateDelegationByAssessment operation returned by the
* service.
* @sample AWSAuditManagerAsync.BatchCreateDelegationByAssessment
* @see AWS API Documentation
*/
java.util.concurrent.Future batchCreateDelegationByAssessmentAsync(
BatchCreateDelegationByAssessmentRequest batchCreateDelegationByAssessmentRequest);
/**
*
* Create a batch of delegations for a specified assessment in AWS Audit Manager.
*
*
* @param batchCreateDelegationByAssessmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchCreateDelegationByAssessment operation returned by the
* service.
* @sample AWSAuditManagerAsyncHandler.BatchCreateDelegationByAssessment
* @see AWS API Documentation
*/
java.util.concurrent.Future batchCreateDelegationByAssessmentAsync(
BatchCreateDelegationByAssessmentRequest batchCreateDelegationByAssessmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the delegations in the specified AWS Audit Manager assessment.
*
*
* @param batchDeleteDelegationByAssessmentRequest
* @return A Java Future containing the result of the BatchDeleteDelegationByAssessment operation returned by the
* service.
* @sample AWSAuditManagerAsync.BatchDeleteDelegationByAssessment
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDeleteDelegationByAssessmentAsync(
BatchDeleteDelegationByAssessmentRequest batchDeleteDelegationByAssessmentRequest);
/**
*
* Deletes the delegations in the specified AWS Audit Manager assessment.
*
*
* @param batchDeleteDelegationByAssessmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchDeleteDelegationByAssessment operation returned by the
* service.
* @sample AWSAuditManagerAsyncHandler.BatchDeleteDelegationByAssessment
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDeleteDelegationByAssessmentAsync(
BatchDeleteDelegationByAssessmentRequest batchDeleteDelegationByAssessmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates a list of evidence from the specified assessment report in AWS Audit Manager.
*
*
* @param batchDisassociateAssessmentReportEvidenceRequest
* @return A Java Future containing the result of the BatchDisassociateAssessmentReportEvidence operation returned
* by the service.
* @sample AWSAuditManagerAsync.BatchDisassociateAssessmentReportEvidence
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDisassociateAssessmentReportEvidenceAsync(
BatchDisassociateAssessmentReportEvidenceRequest batchDisassociateAssessmentReportEvidenceRequest);
/**
*
* Disassociates a list of evidence from the specified assessment report in AWS Audit Manager.
*
*
* @param batchDisassociateAssessmentReportEvidenceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchDisassociateAssessmentReportEvidence operation returned
* by the service.
* @sample AWSAuditManagerAsyncHandler.BatchDisassociateAssessmentReportEvidence
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDisassociateAssessmentReportEvidenceAsync(
BatchDisassociateAssessmentReportEvidenceRequest batchDisassociateAssessmentReportEvidenceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Uploads one or more pieces of evidence to the specified control in the assessment in AWS Audit Manager.
*
*
* @param batchImportEvidenceToAssessmentControlRequest
* @return A Java Future containing the result of the BatchImportEvidenceToAssessmentControl operation returned by
* the service.
* @sample AWSAuditManagerAsync.BatchImportEvidenceToAssessmentControl
* @see AWS API Documentation
*/
java.util.concurrent.Future batchImportEvidenceToAssessmentControlAsync(
BatchImportEvidenceToAssessmentControlRequest batchImportEvidenceToAssessmentControlRequest);
/**
*
* Uploads one or more pieces of evidence to the specified control in the assessment in AWS Audit Manager.
*
*
* @param batchImportEvidenceToAssessmentControlRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchImportEvidenceToAssessmentControl operation returned by
* the service.
* @sample AWSAuditManagerAsyncHandler.BatchImportEvidenceToAssessmentControl
* @see AWS API Documentation
*/
java.util.concurrent.Future batchImportEvidenceToAssessmentControlAsync(
BatchImportEvidenceToAssessmentControlRequest batchImportEvidenceToAssessmentControlRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an assessment in AWS Audit Manager.
*
*
* @param createAssessmentRequest
* @return A Java Future containing the result of the CreateAssessment operation returned by the service.
* @sample AWSAuditManagerAsync.CreateAssessment
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createAssessmentAsync(CreateAssessmentRequest createAssessmentRequest);
/**
*
* Creates an assessment in AWS Audit Manager.
*
*
* @param createAssessmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAssessment operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.CreateAssessment
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createAssessmentAsync(CreateAssessmentRequest createAssessmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a custom framework in AWS Audit Manager.
*
*
* @param createAssessmentFrameworkRequest
* @return A Java Future containing the result of the CreateAssessmentFramework operation returned by the service.
* @sample AWSAuditManagerAsync.CreateAssessmentFramework
* @see AWS API Documentation
*/
java.util.concurrent.Future createAssessmentFrameworkAsync(
CreateAssessmentFrameworkRequest createAssessmentFrameworkRequest);
/**
*
* Creates a custom framework in AWS Audit Manager.
*
*
* @param createAssessmentFrameworkRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAssessmentFramework operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.CreateAssessmentFramework
* @see AWS API Documentation
*/
java.util.concurrent.Future createAssessmentFrameworkAsync(
CreateAssessmentFrameworkRequest createAssessmentFrameworkRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an assessment report for the specified assessment.
*
*
* @param createAssessmentReportRequest
* @return A Java Future containing the result of the CreateAssessmentReport operation returned by the service.
* @sample AWSAuditManagerAsync.CreateAssessmentReport
* @see AWS API Documentation
*/
java.util.concurrent.Future createAssessmentReportAsync(CreateAssessmentReportRequest createAssessmentReportRequest);
/**
*
* Creates an assessment report for the specified assessment.
*
*
* @param createAssessmentReportRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAssessmentReport operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.CreateAssessmentReport
* @see AWS API Documentation
*/
java.util.concurrent.Future createAssessmentReportAsync(CreateAssessmentReportRequest createAssessmentReportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new custom control in AWS Audit Manager.
*
*
* @param createControlRequest
* @return A Java Future containing the result of the CreateControl operation returned by the service.
* @sample AWSAuditManagerAsync.CreateControl
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createControlAsync(CreateControlRequest createControlRequest);
/**
*
* Creates a new custom control in AWS Audit Manager.
*
*
* @param createControlRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateControl operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.CreateControl
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createControlAsync(CreateControlRequest createControlRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an assessment in AWS Audit Manager.
*
*
* @param deleteAssessmentRequest
* @return A Java Future containing the result of the DeleteAssessment operation returned by the service.
* @sample AWSAuditManagerAsync.DeleteAssessment
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteAssessmentAsync(DeleteAssessmentRequest deleteAssessmentRequest);
/**
*
* Deletes an assessment in AWS Audit Manager.
*
*
* @param deleteAssessmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAssessment operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.DeleteAssessment
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteAssessmentAsync(DeleteAssessmentRequest deleteAssessmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a custom framework in AWS Audit Manager.
*
*
* @param deleteAssessmentFrameworkRequest
* @return A Java Future containing the result of the DeleteAssessmentFramework operation returned by the service.
* @sample AWSAuditManagerAsync.DeleteAssessmentFramework
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAssessmentFrameworkAsync(
DeleteAssessmentFrameworkRequest deleteAssessmentFrameworkRequest);
/**
*
* Deletes a custom framework in AWS Audit Manager.
*
*
* @param deleteAssessmentFrameworkRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAssessmentFramework operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.DeleteAssessmentFramework
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAssessmentFrameworkAsync(
DeleteAssessmentFrameworkRequest deleteAssessmentFrameworkRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an assessment report from an assessment in AWS Audit Manager.
*
*
* @param deleteAssessmentReportRequest
* @return A Java Future containing the result of the DeleteAssessmentReport operation returned by the service.
* @sample AWSAuditManagerAsync.DeleteAssessmentReport
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAssessmentReportAsync(DeleteAssessmentReportRequest deleteAssessmentReportRequest);
/**
*
* Deletes an assessment report from an assessment in AWS Audit Manager.
*
*
* @param deleteAssessmentReportRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAssessmentReport operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.DeleteAssessmentReport
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAssessmentReportAsync(DeleteAssessmentReportRequest deleteAssessmentReportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a custom control in AWS Audit Manager.
*
*
* @param deleteControlRequest
* @return A Java Future containing the result of the DeleteControl operation returned by the service.
* @sample AWSAuditManagerAsync.DeleteControl
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteControlAsync(DeleteControlRequest deleteControlRequest);
/**
*
* Deletes a custom control in AWS Audit Manager.
*
*
* @param deleteControlRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteControl operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.DeleteControl
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteControlAsync(DeleteControlRequest deleteControlRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deregisters an account in AWS Audit Manager.
*
*
* @param deregisterAccountRequest
* @return A Java Future containing the result of the DeregisterAccount operation returned by the service.
* @sample AWSAuditManagerAsync.DeregisterAccount
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deregisterAccountAsync(DeregisterAccountRequest deregisterAccountRequest);
/**
*
* Deregisters an account in AWS Audit Manager.
*
*
* @param deregisterAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeregisterAccount operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.DeregisterAccount
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deregisterAccountAsync(DeregisterAccountRequest deregisterAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deregisters the delegated AWS administrator account from the AWS organization.
*
*
* @param deregisterOrganizationAdminAccountRequest
* @return A Java Future containing the result of the DeregisterOrganizationAdminAccount operation returned by the
* service.
* @sample AWSAuditManagerAsync.DeregisterOrganizationAdminAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future deregisterOrganizationAdminAccountAsync(
DeregisterOrganizationAdminAccountRequest deregisterOrganizationAdminAccountRequest);
/**
*
* Deregisters the delegated AWS administrator account from the AWS organization.
*
*
* @param deregisterOrganizationAdminAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeregisterOrganizationAdminAccount operation returned by the
* service.
* @sample AWSAuditManagerAsyncHandler.DeregisterOrganizationAdminAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future deregisterOrganizationAdminAccountAsync(
DeregisterOrganizationAdminAccountRequest deregisterOrganizationAdminAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates an evidence folder from the specified assessment report in AWS Audit Manager.
*
*
* @param disassociateAssessmentReportEvidenceFolderRequest
* @return A Java Future containing the result of the DisassociateAssessmentReportEvidenceFolder operation returned
* by the service.
* @sample AWSAuditManagerAsync.DisassociateAssessmentReportEvidenceFolder
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateAssessmentReportEvidenceFolderAsync(
DisassociateAssessmentReportEvidenceFolderRequest disassociateAssessmentReportEvidenceFolderRequest);
/**
*
* Disassociates an evidence folder from the specified assessment report in AWS Audit Manager.
*
*
* @param disassociateAssessmentReportEvidenceFolderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateAssessmentReportEvidenceFolder operation returned
* by the service.
* @sample AWSAuditManagerAsyncHandler.DisassociateAssessmentReportEvidenceFolder
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateAssessmentReportEvidenceFolderAsync(
DisassociateAssessmentReportEvidenceFolderRequest disassociateAssessmentReportEvidenceFolderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the registration status of an account in AWS Audit Manager.
*
*
* @param getAccountStatusRequest
* @return A Java Future containing the result of the GetAccountStatus operation returned by the service.
* @sample AWSAuditManagerAsync.GetAccountStatus
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getAccountStatusAsync(GetAccountStatusRequest getAccountStatusRequest);
/**
*
* Returns the registration status of an account in AWS Audit Manager.
*
*
* @param getAccountStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAccountStatus operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.GetAccountStatus
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getAccountStatusAsync(GetAccountStatusRequest getAccountStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an assessment from AWS Audit Manager.
*
*
* @param getAssessmentRequest
* @return A Java Future containing the result of the GetAssessment operation returned by the service.
* @sample AWSAuditManagerAsync.GetAssessment
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAssessmentAsync(GetAssessmentRequest getAssessmentRequest);
/**
*
* Returns an assessment from AWS Audit Manager.
*
*
* @param getAssessmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAssessment operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.GetAssessment
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAssessmentAsync(GetAssessmentRequest getAssessmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a framework from AWS Audit Manager.
*
*
* @param getAssessmentFrameworkRequest
* @return A Java Future containing the result of the GetAssessmentFramework operation returned by the service.
* @sample AWSAuditManagerAsync.GetAssessmentFramework
* @see AWS API Documentation
*/
java.util.concurrent.Future getAssessmentFrameworkAsync(GetAssessmentFrameworkRequest getAssessmentFrameworkRequest);
/**
*
* Returns a framework from AWS Audit Manager.
*
*
* @param getAssessmentFrameworkRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAssessmentFramework operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.GetAssessmentFramework
* @see AWS API Documentation
*/
java.util.concurrent.Future getAssessmentFrameworkAsync(GetAssessmentFrameworkRequest getAssessmentFrameworkRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the URL of a specified assessment report in AWS Audit Manager.
*
*
* @param getAssessmentReportUrlRequest
* @return A Java Future containing the result of the GetAssessmentReportUrl operation returned by the service.
* @sample AWSAuditManagerAsync.GetAssessmentReportUrl
* @see AWS API Documentation
*/
java.util.concurrent.Future getAssessmentReportUrlAsync(GetAssessmentReportUrlRequest getAssessmentReportUrlRequest);
/**
*
* Returns the URL of a specified assessment report in AWS Audit Manager.
*
*
* @param getAssessmentReportUrlRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAssessmentReportUrl operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.GetAssessmentReportUrl
* @see AWS API Documentation
*/
java.util.concurrent.Future getAssessmentReportUrlAsync(GetAssessmentReportUrlRequest getAssessmentReportUrlRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of changelogs from AWS Audit Manager.
*
*
* @param getChangeLogsRequest
* @return A Java Future containing the result of the GetChangeLogs operation returned by the service.
* @sample AWSAuditManagerAsync.GetChangeLogs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getChangeLogsAsync(GetChangeLogsRequest getChangeLogsRequest);
/**
*
* Returns a list of changelogs from AWS Audit Manager.
*
*
* @param getChangeLogsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetChangeLogs operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.GetChangeLogs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getChangeLogsAsync(GetChangeLogsRequest getChangeLogsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a control from AWS Audit Manager.
*
*
* @param getControlRequest
* @return A Java Future containing the result of the GetControl operation returned by the service.
* @sample AWSAuditManagerAsync.GetControl
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getControlAsync(GetControlRequest getControlRequest);
/**
*
* Returns a control from AWS Audit Manager.
*
*
* @param getControlRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetControl operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.GetControl
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getControlAsync(GetControlRequest getControlRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of delegations from an audit owner to a delegate.
*
*
* @param getDelegationsRequest
* @return A Java Future containing the result of the GetDelegations operation returned by the service.
* @sample AWSAuditManagerAsync.GetDelegations
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDelegationsAsync(GetDelegationsRequest getDelegationsRequest);
/**
*
* Returns a list of delegations from an audit owner to a delegate.
*
*
* @param getDelegationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDelegations operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.GetDelegations
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getDelegationsAsync(GetDelegationsRequest getDelegationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns evidence from AWS Audit Manager.
*
*
* @param getEvidenceRequest
* @return A Java Future containing the result of the GetEvidence operation returned by the service.
* @sample AWSAuditManagerAsync.GetEvidence
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getEvidenceAsync(GetEvidenceRequest getEvidenceRequest);
/**
*
* Returns evidence from AWS Audit Manager.
*
*
* @param getEvidenceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEvidence operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.GetEvidence
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getEvidenceAsync(GetEvidenceRequest getEvidenceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns all evidence from a specified evidence folder in AWS Audit Manager.
*
*
* @param getEvidenceByEvidenceFolderRequest
* @return A Java Future containing the result of the GetEvidenceByEvidenceFolder operation returned by the service.
* @sample AWSAuditManagerAsync.GetEvidenceByEvidenceFolder
* @see AWS API Documentation
*/
java.util.concurrent.Future getEvidenceByEvidenceFolderAsync(
GetEvidenceByEvidenceFolderRequest getEvidenceByEvidenceFolderRequest);
/**
*
* Returns all evidence from a specified evidence folder in AWS Audit Manager.
*
*
* @param getEvidenceByEvidenceFolderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEvidenceByEvidenceFolder operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.GetEvidenceByEvidenceFolder
* @see AWS API Documentation
*/
java.util.concurrent.Future getEvidenceByEvidenceFolderAsync(
GetEvidenceByEvidenceFolderRequest getEvidenceByEvidenceFolderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an evidence folder from the specified assessment in AWS Audit Manager.
*
*
* @param getEvidenceFolderRequest
* @return A Java Future containing the result of the GetEvidenceFolder operation returned by the service.
* @sample AWSAuditManagerAsync.GetEvidenceFolder
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getEvidenceFolderAsync(GetEvidenceFolderRequest getEvidenceFolderRequest);
/**
*
* Returns an evidence folder from the specified assessment in AWS Audit Manager.
*
*
* @param getEvidenceFolderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEvidenceFolder operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.GetEvidenceFolder
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getEvidenceFolderAsync(GetEvidenceFolderRequest getEvidenceFolderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the evidence folders from a specified assessment in AWS Audit Manager.
*
*
* @param getEvidenceFoldersByAssessmentRequest
* @return A Java Future containing the result of the GetEvidenceFoldersByAssessment operation returned by the
* service.
* @sample AWSAuditManagerAsync.GetEvidenceFoldersByAssessment
* @see AWS API Documentation
*/
java.util.concurrent.Future getEvidenceFoldersByAssessmentAsync(
GetEvidenceFoldersByAssessmentRequest getEvidenceFoldersByAssessmentRequest);
/**
*
* Returns the evidence folders from a specified assessment in AWS Audit Manager.
*
*
* @param getEvidenceFoldersByAssessmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEvidenceFoldersByAssessment operation returned by the
* service.
* @sample AWSAuditManagerAsyncHandler.GetEvidenceFoldersByAssessment
* @see AWS API Documentation
*/
java.util.concurrent.Future getEvidenceFoldersByAssessmentAsync(
GetEvidenceFoldersByAssessmentRequest getEvidenceFoldersByAssessmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of evidence folders associated with a specified control of an assessment in AWS Audit Manager.
*
*
* @param getEvidenceFoldersByAssessmentControlRequest
* @return A Java Future containing the result of the GetEvidenceFoldersByAssessmentControl operation returned by
* the service.
* @sample AWSAuditManagerAsync.GetEvidenceFoldersByAssessmentControl
* @see AWS API Documentation
*/
java.util.concurrent.Future getEvidenceFoldersByAssessmentControlAsync(
GetEvidenceFoldersByAssessmentControlRequest getEvidenceFoldersByAssessmentControlRequest);
/**
*
* Returns a list of evidence folders associated with a specified control of an assessment in AWS Audit Manager.
*
*
* @param getEvidenceFoldersByAssessmentControlRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEvidenceFoldersByAssessmentControl operation returned by
* the service.
* @sample AWSAuditManagerAsyncHandler.GetEvidenceFoldersByAssessmentControl
* @see AWS API Documentation
*/
java.util.concurrent.Future getEvidenceFoldersByAssessmentControlAsync(
GetEvidenceFoldersByAssessmentControlRequest getEvidenceFoldersByAssessmentControlRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the name of the delegated AWS administrator account for the AWS organization.
*
*
* @param getOrganizationAdminAccountRequest
* @return A Java Future containing the result of the GetOrganizationAdminAccount operation returned by the service.
* @sample AWSAuditManagerAsync.GetOrganizationAdminAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future getOrganizationAdminAccountAsync(
GetOrganizationAdminAccountRequest getOrganizationAdminAccountRequest);
/**
*
* Returns the name of the delegated AWS administrator account for the AWS organization.
*
*
* @param getOrganizationAdminAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetOrganizationAdminAccount operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.GetOrganizationAdminAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future getOrganizationAdminAccountAsync(
GetOrganizationAdminAccountRequest getOrganizationAdminAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of the in-scope AWS services for the specified assessment.
*
*
* @param getServicesInScopeRequest
* @return A Java Future containing the result of the GetServicesInScope operation returned by the service.
* @sample AWSAuditManagerAsync.GetServicesInScope
* @see AWS API Documentation
*/
java.util.concurrent.Future getServicesInScopeAsync(GetServicesInScopeRequest getServicesInScopeRequest);
/**
*
* Returns a list of the in-scope AWS services for the specified assessment.
*
*
* @param getServicesInScopeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetServicesInScope operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.GetServicesInScope
* @see AWS API Documentation
*/
java.util.concurrent.Future getServicesInScopeAsync(GetServicesInScopeRequest getServicesInScopeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the settings for the specified AWS account.
*
*
* @param getSettingsRequest
* @return A Java Future containing the result of the GetSettings operation returned by the service.
* @sample AWSAuditManagerAsync.GetSettings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSettingsAsync(GetSettingsRequest getSettingsRequest);
/**
*
* Returns the settings for the specified AWS account.
*
*
* @param getSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSettings operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.GetSettings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSettingsAsync(GetSettingsRequest getSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of the frameworks available in the AWS Audit Manager framework library.
*
*
* @param listAssessmentFrameworksRequest
* @return A Java Future containing the result of the ListAssessmentFrameworks operation returned by the service.
* @sample AWSAuditManagerAsync.ListAssessmentFrameworks
* @see AWS API Documentation
*/
java.util.concurrent.Future listAssessmentFrameworksAsync(ListAssessmentFrameworksRequest listAssessmentFrameworksRequest);
/**
*
* Returns a list of the frameworks available in the AWS Audit Manager framework library.
*
*
* @param listAssessmentFrameworksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAssessmentFrameworks operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.ListAssessmentFrameworks
* @see AWS API Documentation
*/
java.util.concurrent.Future listAssessmentFrameworksAsync(ListAssessmentFrameworksRequest listAssessmentFrameworksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of assessment reports created in AWS Audit Manager.
*
*
* @param listAssessmentReportsRequest
* @return A Java Future containing the result of the ListAssessmentReports operation returned by the service.
* @sample AWSAuditManagerAsync.ListAssessmentReports
* @see AWS API Documentation
*/
java.util.concurrent.Future listAssessmentReportsAsync(ListAssessmentReportsRequest listAssessmentReportsRequest);
/**
*
* Returns a list of assessment reports created in AWS Audit Manager.
*
*
* @param listAssessmentReportsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAssessmentReports operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.ListAssessmentReports
* @see AWS API Documentation
*/
java.util.concurrent.Future listAssessmentReportsAsync(ListAssessmentReportsRequest listAssessmentReportsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of current and past assessments from AWS Audit Manager.
*
*
* @param listAssessmentsRequest
* @return A Java Future containing the result of the ListAssessments operation returned by the service.
* @sample AWSAuditManagerAsync.ListAssessments
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAssessmentsAsync(ListAssessmentsRequest listAssessmentsRequest);
/**
*
* Returns a list of current and past assessments from AWS Audit Manager.
*
*
* @param listAssessmentsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAssessments operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.ListAssessments
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAssessmentsAsync(ListAssessmentsRequest listAssessmentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of controls from AWS Audit Manager.
*
*
* @param listControlsRequest
* @return A Java Future containing the result of the ListControls operation returned by the service.
* @sample AWSAuditManagerAsync.ListControls
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listControlsAsync(ListControlsRequest listControlsRequest);
/**
*
* Returns a list of controls from AWS Audit Manager.
*
*
* @param listControlsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListControls operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.ListControls
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listControlsAsync(ListControlsRequest listControlsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of keywords that pre-mapped to the specified control data source.
*
*
* @param listKeywordsForDataSourceRequest
* @return A Java Future containing the result of the ListKeywordsForDataSource operation returned by the service.
* @sample AWSAuditManagerAsync.ListKeywordsForDataSource
* @see AWS API Documentation
*/
java.util.concurrent.Future listKeywordsForDataSourceAsync(
ListKeywordsForDataSourceRequest listKeywordsForDataSourceRequest);
/**
*
* Returns a list of keywords that pre-mapped to the specified control data source.
*
*
* @param listKeywordsForDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListKeywordsForDataSource operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.ListKeywordsForDataSource
* @see AWS API Documentation
*/
java.util.concurrent.Future listKeywordsForDataSourceAsync(
ListKeywordsForDataSourceRequest listKeywordsForDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all AWS Audit Manager notifications.
*
*
* @param listNotificationsRequest
* @return A Java Future containing the result of the ListNotifications operation returned by the service.
* @sample AWSAuditManagerAsync.ListNotifications
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listNotificationsAsync(ListNotificationsRequest listNotificationsRequest);
/**
*
* Returns a list of all AWS Audit Manager notifications.
*
*
* @param listNotificationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListNotifications operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.ListNotifications
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listNotificationsAsync(ListNotificationsRequest listNotificationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of tags for the specified resource in AWS Audit Manager.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSAuditManagerAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Returns a list of tags for the specified resource in AWS Audit Manager.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables AWS Audit Manager for the specified AWS account.
*
*
* @param registerAccountRequest
* @return A Java Future containing the result of the RegisterAccount operation returned by the service.
* @sample AWSAuditManagerAsync.RegisterAccount
* @see AWS
* API Documentation
*/
java.util.concurrent.Future registerAccountAsync(RegisterAccountRequest registerAccountRequest);
/**
*
* Enables AWS Audit Manager for the specified AWS account.
*
*
* @param registerAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RegisterAccount operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.RegisterAccount
* @see AWS
* API Documentation
*/
java.util.concurrent.Future registerAccountAsync(RegisterAccountRequest registerAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables an AWS account within the organization as the delegated administrator for AWS Audit Manager.
*
*
* @param registerOrganizationAdminAccountRequest
* @return A Java Future containing the result of the RegisterOrganizationAdminAccount operation returned by the
* service.
* @sample AWSAuditManagerAsync.RegisterOrganizationAdminAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future registerOrganizationAdminAccountAsync(
RegisterOrganizationAdminAccountRequest registerOrganizationAdminAccountRequest);
/**
*
* Enables an AWS account within the organization as the delegated administrator for AWS Audit Manager.
*
*
* @param registerOrganizationAdminAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RegisterOrganizationAdminAccount operation returned by the
* service.
* @sample AWSAuditManagerAsyncHandler.RegisterOrganizationAdminAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future registerOrganizationAdminAccountAsync(
RegisterOrganizationAdminAccountRequest registerOrganizationAdminAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Tags the specified resource in AWS Audit Manager.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSAuditManagerAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Tags the specified resource in AWS Audit Manager.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a tag from a resource in AWS Audit Manager.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSAuditManagerAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes a tag from a resource in AWS Audit Manager.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Edits an AWS Audit Manager assessment.
*
*
* @param updateAssessmentRequest
* @return A Java Future containing the result of the UpdateAssessment operation returned by the service.
* @sample AWSAuditManagerAsync.UpdateAssessment
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateAssessmentAsync(UpdateAssessmentRequest updateAssessmentRequest);
/**
*
* Edits an AWS Audit Manager assessment.
*
*
* @param updateAssessmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAssessment operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.UpdateAssessment
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateAssessmentAsync(UpdateAssessmentRequest updateAssessmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a control within an assessment in AWS Audit Manager.
*
*
* @param updateAssessmentControlRequest
* @return A Java Future containing the result of the UpdateAssessmentControl operation returned by the service.
* @sample AWSAuditManagerAsync.UpdateAssessmentControl
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAssessmentControlAsync(UpdateAssessmentControlRequest updateAssessmentControlRequest);
/**
*
* Updates a control within an assessment in AWS Audit Manager.
*
*
* @param updateAssessmentControlRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAssessmentControl operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.UpdateAssessmentControl
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAssessmentControlAsync(UpdateAssessmentControlRequest updateAssessmentControlRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the status of a control set in an AWS Audit Manager assessment.
*
*
* @param updateAssessmentControlSetStatusRequest
* @return A Java Future containing the result of the UpdateAssessmentControlSetStatus operation returned by the
* service.
* @sample AWSAuditManagerAsync.UpdateAssessmentControlSetStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAssessmentControlSetStatusAsync(
UpdateAssessmentControlSetStatusRequest updateAssessmentControlSetStatusRequest);
/**
*
* Updates the status of a control set in an AWS Audit Manager assessment.
*
*
* @param updateAssessmentControlSetStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAssessmentControlSetStatus operation returned by the
* service.
* @sample AWSAuditManagerAsyncHandler.UpdateAssessmentControlSetStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAssessmentControlSetStatusAsync(
UpdateAssessmentControlSetStatusRequest updateAssessmentControlSetStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a custom framework in AWS Audit Manager.
*
*
* @param updateAssessmentFrameworkRequest
* @return A Java Future containing the result of the UpdateAssessmentFramework operation returned by the service.
* @sample AWSAuditManagerAsync.UpdateAssessmentFramework
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAssessmentFrameworkAsync(
UpdateAssessmentFrameworkRequest updateAssessmentFrameworkRequest);
/**
*
* Updates a custom framework in AWS Audit Manager.
*
*
* @param updateAssessmentFrameworkRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAssessmentFramework operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.UpdateAssessmentFramework
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAssessmentFrameworkAsync(
UpdateAssessmentFrameworkRequest updateAssessmentFrameworkRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the status of an assessment in AWS Audit Manager.
*
*
* @param updateAssessmentStatusRequest
* @return A Java Future containing the result of the UpdateAssessmentStatus operation returned by the service.
* @sample AWSAuditManagerAsync.UpdateAssessmentStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAssessmentStatusAsync(UpdateAssessmentStatusRequest updateAssessmentStatusRequest);
/**
*
* Updates the status of an assessment in AWS Audit Manager.
*
*
* @param updateAssessmentStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAssessmentStatus operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.UpdateAssessmentStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAssessmentStatusAsync(UpdateAssessmentStatusRequest updateAssessmentStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a custom control in AWS Audit Manager.
*
*
* @param updateControlRequest
* @return A Java Future containing the result of the UpdateControl operation returned by the service.
* @sample AWSAuditManagerAsync.UpdateControl
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateControlAsync(UpdateControlRequest updateControlRequest);
/**
*
* Updates a custom control in AWS Audit Manager.
*
*
* @param updateControlRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateControl operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.UpdateControl
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateControlAsync(UpdateControlRequest updateControlRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates AWS Audit Manager settings for the current user account.
*
*
* @param updateSettingsRequest
* @return A Java Future containing the result of the UpdateSettings operation returned by the service.
* @sample AWSAuditManagerAsync.UpdateSettings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateSettingsAsync(UpdateSettingsRequest updateSettingsRequest);
/**
*
* Updates AWS Audit Manager settings for the current user account.
*
*
* @param updateSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSettings operation returned by the service.
* @sample AWSAuditManagerAsyncHandler.UpdateSettings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateSettingsAsync(UpdateSettingsRequest updateSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Validates the integrity of an assessment report in AWS Audit Manager.
*
*
* @param validateAssessmentReportIntegrityRequest
* @return A Java Future containing the result of the ValidateAssessmentReportIntegrity operation returned by the
* service.
* @sample AWSAuditManagerAsync.ValidateAssessmentReportIntegrity
* @see AWS API Documentation
*/
java.util.concurrent.Future validateAssessmentReportIntegrityAsync(
ValidateAssessmentReportIntegrityRequest validateAssessmentReportIntegrityRequest);
/**
*
* Validates the integrity of an assessment report in AWS Audit Manager.
*
*
* @param validateAssessmentReportIntegrityRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ValidateAssessmentReportIntegrity operation returned by the
* service.
* @sample AWSAuditManagerAsyncHandler.ValidateAssessmentReportIntegrity
* @see AWS API Documentation
*/
java.util.concurrent.Future validateAssessmentReportIntegrityAsync(
ValidateAssessmentReportIntegrityRequest validateAssessmentReportIntegrityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}