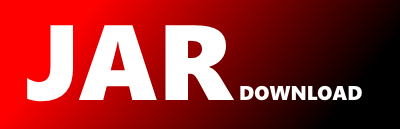
com.amazonaws.services.auditmanager.model.Evidence Maven / Gradle / Ivy
Show all versions of aws-java-sdk-auditmanager Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.auditmanager.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A record that contains the information needed to demonstrate compliance with the requirements specified by a control.
* Examples of evidence include change activity invoked by a user, or a system configuration snapshot.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Evidence implements Serializable, Cloneable, StructuredPojo {
/**
*
* The data source where the evidence was collected from.
*
*/
private String dataSource;
/**
*
* The identifier for the Amazon Web Services account.
*
*/
private String evidenceAwsAccountId;
/**
*
* The timestamp that represents when the evidence was collected.
*
*/
private java.util.Date time;
/**
*
* The Amazon Web Service that the evidence is collected from.
*
*/
private String eventSource;
/**
*
* The name of the evidence event.
*
*/
private String eventName;
/**
*
* The type of automated evidence.
*
*/
private String evidenceByType;
/**
*
* The list of resources that are assessed to generate the evidence.
*
*/
private java.util.List resourcesIncluded;
/**
*
* The names and values that are used by the evidence event. This includes an attribute name (such as
* allowUsersToChangePassword
) and value (such as true
or false
).
*
*/
private java.util.Map attributes;
/**
*
* The unique identifier for the user or role that's associated with the evidence.
*
*/
private String iamId;
/**
*
* The evaluation status for automated evidence that falls under the compliance check category.
*
*
* -
*
* Audit Manager classes evidence as non-compliant if Security Hub reports a Fail result, or if Config
* reports a Non-compliant result.
*
*
* -
*
* Audit Manager classes evidence as compliant if Security Hub reports a Pass result, or if Config reports a
* Compliant result.
*
*
* -
*
* If a compliance check isn't available or applicable, then no compliance evaluation can be made for that evidence.
* This is the case if the evidence uses Config or Security Hub as the underlying data source type, but those
* services aren't enabled. This is also the case if the evidence uses an underlying data source type that doesn't
* support compliance checks (such as manual evidence, Amazon Web Services API calls, or CloudTrail).
*
*
*
*/
private String complianceCheck;
/**
*
* The Amazon Web Services account that the evidence is collected from, and its organization path.
*
*/
private String awsOrganization;
/**
*
* The identifier for the Amazon Web Services account.
*
*/
private String awsAccountId;
/**
*
* The identifier for the folder that the evidence is stored in.
*
*/
private String evidenceFolderId;
/**
*
* The identifier for the evidence.
*
*/
private String id;
/**
*
* Specifies whether the evidence is included in the assessment report.
*
*/
private String assessmentReportSelection;
/**
*
* The data source where the evidence was collected from.
*
*
* @param dataSource
* The data source where the evidence was collected from.
*/
public void setDataSource(String dataSource) {
this.dataSource = dataSource;
}
/**
*
* The data source where the evidence was collected from.
*
*
* @return The data source where the evidence was collected from.
*/
public String getDataSource() {
return this.dataSource;
}
/**
*
* The data source where the evidence was collected from.
*
*
* @param dataSource
* The data source where the evidence was collected from.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Evidence withDataSource(String dataSource) {
setDataSource(dataSource);
return this;
}
/**
*
* The identifier for the Amazon Web Services account.
*
*
* @param evidenceAwsAccountId
* The identifier for the Amazon Web Services account.
*/
public void setEvidenceAwsAccountId(String evidenceAwsAccountId) {
this.evidenceAwsAccountId = evidenceAwsAccountId;
}
/**
*
* The identifier for the Amazon Web Services account.
*
*
* @return The identifier for the Amazon Web Services account.
*/
public String getEvidenceAwsAccountId() {
return this.evidenceAwsAccountId;
}
/**
*
* The identifier for the Amazon Web Services account.
*
*
* @param evidenceAwsAccountId
* The identifier for the Amazon Web Services account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Evidence withEvidenceAwsAccountId(String evidenceAwsAccountId) {
setEvidenceAwsAccountId(evidenceAwsAccountId);
return this;
}
/**
*
* The timestamp that represents when the evidence was collected.
*
*
* @param time
* The timestamp that represents when the evidence was collected.
*/
public void setTime(java.util.Date time) {
this.time = time;
}
/**
*
* The timestamp that represents when the evidence was collected.
*
*
* @return The timestamp that represents when the evidence was collected.
*/
public java.util.Date getTime() {
return this.time;
}
/**
*
* The timestamp that represents when the evidence was collected.
*
*
* @param time
* The timestamp that represents when the evidence was collected.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Evidence withTime(java.util.Date time) {
setTime(time);
return this;
}
/**
*
* The Amazon Web Service that the evidence is collected from.
*
*
* @param eventSource
* The Amazon Web Service that the evidence is collected from.
*/
public void setEventSource(String eventSource) {
this.eventSource = eventSource;
}
/**
*
* The Amazon Web Service that the evidence is collected from.
*
*
* @return The Amazon Web Service that the evidence is collected from.
*/
public String getEventSource() {
return this.eventSource;
}
/**
*
* The Amazon Web Service that the evidence is collected from.
*
*
* @param eventSource
* The Amazon Web Service that the evidence is collected from.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Evidence withEventSource(String eventSource) {
setEventSource(eventSource);
return this;
}
/**
*
* The name of the evidence event.
*
*
* @param eventName
* The name of the evidence event.
*/
public void setEventName(String eventName) {
this.eventName = eventName;
}
/**
*
* The name of the evidence event.
*
*
* @return The name of the evidence event.
*/
public String getEventName() {
return this.eventName;
}
/**
*
* The name of the evidence event.
*
*
* @param eventName
* The name of the evidence event.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Evidence withEventName(String eventName) {
setEventName(eventName);
return this;
}
/**
*
* The type of automated evidence.
*
*
* @param evidenceByType
* The type of automated evidence.
*/
public void setEvidenceByType(String evidenceByType) {
this.evidenceByType = evidenceByType;
}
/**
*
* The type of automated evidence.
*
*
* @return The type of automated evidence.
*/
public String getEvidenceByType() {
return this.evidenceByType;
}
/**
*
* The type of automated evidence.
*
*
* @param evidenceByType
* The type of automated evidence.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Evidence withEvidenceByType(String evidenceByType) {
setEvidenceByType(evidenceByType);
return this;
}
/**
*
* The list of resources that are assessed to generate the evidence.
*
*
* @return The list of resources that are assessed to generate the evidence.
*/
public java.util.List getResourcesIncluded() {
return resourcesIncluded;
}
/**
*
* The list of resources that are assessed to generate the evidence.
*
*
* @param resourcesIncluded
* The list of resources that are assessed to generate the evidence.
*/
public void setResourcesIncluded(java.util.Collection resourcesIncluded) {
if (resourcesIncluded == null) {
this.resourcesIncluded = null;
return;
}
this.resourcesIncluded = new java.util.ArrayList(resourcesIncluded);
}
/**
*
* The list of resources that are assessed to generate the evidence.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setResourcesIncluded(java.util.Collection)} or {@link #withResourcesIncluded(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param resourcesIncluded
* The list of resources that are assessed to generate the evidence.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Evidence withResourcesIncluded(Resource... resourcesIncluded) {
if (this.resourcesIncluded == null) {
setResourcesIncluded(new java.util.ArrayList(resourcesIncluded.length));
}
for (Resource ele : resourcesIncluded) {
this.resourcesIncluded.add(ele);
}
return this;
}
/**
*
* The list of resources that are assessed to generate the evidence.
*
*
* @param resourcesIncluded
* The list of resources that are assessed to generate the evidence.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Evidence withResourcesIncluded(java.util.Collection resourcesIncluded) {
setResourcesIncluded(resourcesIncluded);
return this;
}
/**
*
* The names and values that are used by the evidence event. This includes an attribute name (such as
* allowUsersToChangePassword
) and value (such as true
or false
).
*
*
* @return The names and values that are used by the evidence event. This includes an attribute name (such as
* allowUsersToChangePassword
) and value (such as true
or false
).
*/
public java.util.Map getAttributes() {
return attributes;
}
/**
*
* The names and values that are used by the evidence event. This includes an attribute name (such as
* allowUsersToChangePassword
) and value (such as true
or false
).
*
*
* @param attributes
* The names and values that are used by the evidence event. This includes an attribute name (such as
* allowUsersToChangePassword
) and value (such as true
or false
).
*/
public void setAttributes(java.util.Map attributes) {
this.attributes = attributes;
}
/**
*
* The names and values that are used by the evidence event. This includes an attribute name (such as
* allowUsersToChangePassword
) and value (such as true
or false
).
*
*
* @param attributes
* The names and values that are used by the evidence event. This includes an attribute name (such as
* allowUsersToChangePassword
) and value (such as true
or false
).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Evidence withAttributes(java.util.Map attributes) {
setAttributes(attributes);
return this;
}
/**
* Add a single Attributes entry
*
* @see Evidence#withAttributes
* @returns a reference to this object so that method calls can be chained together.
*/
public Evidence addAttributesEntry(String key, String value) {
if (null == this.attributes) {
this.attributes = new java.util.HashMap();
}
if (this.attributes.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.attributes.put(key, value);
return this;
}
/**
* Removes all the entries added into Attributes.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Evidence clearAttributesEntries() {
this.attributes = null;
return this;
}
/**
*
* The unique identifier for the user or role that's associated with the evidence.
*
*
* @param iamId
* The unique identifier for the user or role that's associated with the evidence.
*/
public void setIamId(String iamId) {
this.iamId = iamId;
}
/**
*
* The unique identifier for the user or role that's associated with the evidence.
*
*
* @return The unique identifier for the user or role that's associated with the evidence.
*/
public String getIamId() {
return this.iamId;
}
/**
*
* The unique identifier for the user or role that's associated with the evidence.
*
*
* @param iamId
* The unique identifier for the user or role that's associated with the evidence.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Evidence withIamId(String iamId) {
setIamId(iamId);
return this;
}
/**
*
* The evaluation status for automated evidence that falls under the compliance check category.
*
*
* -
*
* Audit Manager classes evidence as non-compliant if Security Hub reports a Fail result, or if Config
* reports a Non-compliant result.
*
*
* -
*
* Audit Manager classes evidence as compliant if Security Hub reports a Pass result, or if Config reports a
* Compliant result.
*
*
* -
*
* If a compliance check isn't available or applicable, then no compliance evaluation can be made for that evidence.
* This is the case if the evidence uses Config or Security Hub as the underlying data source type, but those
* services aren't enabled. This is also the case if the evidence uses an underlying data source type that doesn't
* support compliance checks (such as manual evidence, Amazon Web Services API calls, or CloudTrail).
*
*
*
*
* @param complianceCheck
* The evaluation status for automated evidence that falls under the compliance check category.
*
* -
*
* Audit Manager classes evidence as non-compliant if Security Hub reports a Fail result, or if Config
* reports a Non-compliant result.
*
*
* -
*
* Audit Manager classes evidence as compliant if Security Hub reports a Pass result, or if Config
* reports a Compliant result.
*
*
* -
*
* If a compliance check isn't available or applicable, then no compliance evaluation can be made for that
* evidence. This is the case if the evidence uses Config or Security Hub as the underlying data source type,
* but those services aren't enabled. This is also the case if the evidence uses an underlying data source
* type that doesn't support compliance checks (such as manual evidence, Amazon Web Services API calls, or
* CloudTrail).
*
*
*/
public void setComplianceCheck(String complianceCheck) {
this.complianceCheck = complianceCheck;
}
/**
*
* The evaluation status for automated evidence that falls under the compliance check category.
*
*
* -
*
* Audit Manager classes evidence as non-compliant if Security Hub reports a Fail result, or if Config
* reports a Non-compliant result.
*
*
* -
*
* Audit Manager classes evidence as compliant if Security Hub reports a Pass result, or if Config reports a
* Compliant result.
*
*
* -
*
* If a compliance check isn't available or applicable, then no compliance evaluation can be made for that evidence.
* This is the case if the evidence uses Config or Security Hub as the underlying data source type, but those
* services aren't enabled. This is also the case if the evidence uses an underlying data source type that doesn't
* support compliance checks (such as manual evidence, Amazon Web Services API calls, or CloudTrail).
*
*
*
*
* @return The evaluation status for automated evidence that falls under the compliance check category.
*
* -
*
* Audit Manager classes evidence as non-compliant if Security Hub reports a Fail result, or if
* Config reports a Non-compliant result.
*
*
* -
*
* Audit Manager classes evidence as compliant if Security Hub reports a Pass result, or if Config
* reports a Compliant result.
*
*
* -
*
* If a compliance check isn't available or applicable, then no compliance evaluation can be made for that
* evidence. This is the case if the evidence uses Config or Security Hub as the underlying data source
* type, but those services aren't enabled. This is also the case if the evidence uses an underlying data
* source type that doesn't support compliance checks (such as manual evidence, Amazon Web Services API
* calls, or CloudTrail).
*
*
*/
public String getComplianceCheck() {
return this.complianceCheck;
}
/**
*
* The evaluation status for automated evidence that falls under the compliance check category.
*
*
* -
*
* Audit Manager classes evidence as non-compliant if Security Hub reports a Fail result, or if Config
* reports a Non-compliant result.
*
*
* -
*
* Audit Manager classes evidence as compliant if Security Hub reports a Pass result, or if Config reports a
* Compliant result.
*
*
* -
*
* If a compliance check isn't available or applicable, then no compliance evaluation can be made for that evidence.
* This is the case if the evidence uses Config or Security Hub as the underlying data source type, but those
* services aren't enabled. This is also the case if the evidence uses an underlying data source type that doesn't
* support compliance checks (such as manual evidence, Amazon Web Services API calls, or CloudTrail).
*
*
*
*
* @param complianceCheck
* The evaluation status for automated evidence that falls under the compliance check category.
*
* -
*
* Audit Manager classes evidence as non-compliant if Security Hub reports a Fail result, or if Config
* reports a Non-compliant result.
*
*
* -
*
* Audit Manager classes evidence as compliant if Security Hub reports a Pass result, or if Config
* reports a Compliant result.
*
*
* -
*
* If a compliance check isn't available or applicable, then no compliance evaluation can be made for that
* evidence. This is the case if the evidence uses Config or Security Hub as the underlying data source type,
* but those services aren't enabled. This is also the case if the evidence uses an underlying data source
* type that doesn't support compliance checks (such as manual evidence, Amazon Web Services API calls, or
* CloudTrail).
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Evidence withComplianceCheck(String complianceCheck) {
setComplianceCheck(complianceCheck);
return this;
}
/**
*
* The Amazon Web Services account that the evidence is collected from, and its organization path.
*
*
* @param awsOrganization
* The Amazon Web Services account that the evidence is collected from, and its organization path.
*/
public void setAwsOrganization(String awsOrganization) {
this.awsOrganization = awsOrganization;
}
/**
*
* The Amazon Web Services account that the evidence is collected from, and its organization path.
*
*
* @return The Amazon Web Services account that the evidence is collected from, and its organization path.
*/
public String getAwsOrganization() {
return this.awsOrganization;
}
/**
*
* The Amazon Web Services account that the evidence is collected from, and its organization path.
*
*
* @param awsOrganization
* The Amazon Web Services account that the evidence is collected from, and its organization path.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Evidence withAwsOrganization(String awsOrganization) {
setAwsOrganization(awsOrganization);
return this;
}
/**
*
* The identifier for the Amazon Web Services account.
*
*
* @param awsAccountId
* The identifier for the Amazon Web Services account.
*/
public void setAwsAccountId(String awsAccountId) {
this.awsAccountId = awsAccountId;
}
/**
*
* The identifier for the Amazon Web Services account.
*
*
* @return The identifier for the Amazon Web Services account.
*/
public String getAwsAccountId() {
return this.awsAccountId;
}
/**
*
* The identifier for the Amazon Web Services account.
*
*
* @param awsAccountId
* The identifier for the Amazon Web Services account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Evidence withAwsAccountId(String awsAccountId) {
setAwsAccountId(awsAccountId);
return this;
}
/**
*
* The identifier for the folder that the evidence is stored in.
*
*
* @param evidenceFolderId
* The identifier for the folder that the evidence is stored in.
*/
public void setEvidenceFolderId(String evidenceFolderId) {
this.evidenceFolderId = evidenceFolderId;
}
/**
*
* The identifier for the folder that the evidence is stored in.
*
*
* @return The identifier for the folder that the evidence is stored in.
*/
public String getEvidenceFolderId() {
return this.evidenceFolderId;
}
/**
*
* The identifier for the folder that the evidence is stored in.
*
*
* @param evidenceFolderId
* The identifier for the folder that the evidence is stored in.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Evidence withEvidenceFolderId(String evidenceFolderId) {
setEvidenceFolderId(evidenceFolderId);
return this;
}
/**
*
* The identifier for the evidence.
*
*
* @param id
* The identifier for the evidence.
*/
public void setId(String id) {
this.id = id;
}
/**
*
* The identifier for the evidence.
*
*
* @return The identifier for the evidence.
*/
public String getId() {
return this.id;
}
/**
*
* The identifier for the evidence.
*
*
* @param id
* The identifier for the evidence.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Evidence withId(String id) {
setId(id);
return this;
}
/**
*
* Specifies whether the evidence is included in the assessment report.
*
*
* @param assessmentReportSelection
* Specifies whether the evidence is included in the assessment report.
*/
public void setAssessmentReportSelection(String assessmentReportSelection) {
this.assessmentReportSelection = assessmentReportSelection;
}
/**
*
* Specifies whether the evidence is included in the assessment report.
*
*
* @return Specifies whether the evidence is included in the assessment report.
*/
public String getAssessmentReportSelection() {
return this.assessmentReportSelection;
}
/**
*
* Specifies whether the evidence is included in the assessment report.
*
*
* @param assessmentReportSelection
* Specifies whether the evidence is included in the assessment report.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Evidence withAssessmentReportSelection(String assessmentReportSelection) {
setAssessmentReportSelection(assessmentReportSelection);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDataSource() != null)
sb.append("DataSource: ").append(getDataSource()).append(",");
if (getEvidenceAwsAccountId() != null)
sb.append("EvidenceAwsAccountId: ").append(getEvidenceAwsAccountId()).append(",");
if (getTime() != null)
sb.append("Time: ").append(getTime()).append(",");
if (getEventSource() != null)
sb.append("EventSource: ").append(getEventSource()).append(",");
if (getEventName() != null)
sb.append("EventName: ").append(getEventName()).append(",");
if (getEvidenceByType() != null)
sb.append("EvidenceByType: ").append(getEvidenceByType()).append(",");
if (getResourcesIncluded() != null)
sb.append("ResourcesIncluded: ").append(getResourcesIncluded()).append(",");
if (getAttributes() != null)
sb.append("Attributes: ").append(getAttributes()).append(",");
if (getIamId() != null)
sb.append("IamId: ").append(getIamId()).append(",");
if (getComplianceCheck() != null)
sb.append("ComplianceCheck: ").append(getComplianceCheck()).append(",");
if (getAwsOrganization() != null)
sb.append("AwsOrganization: ").append(getAwsOrganization()).append(",");
if (getAwsAccountId() != null)
sb.append("AwsAccountId: ").append(getAwsAccountId()).append(",");
if (getEvidenceFolderId() != null)
sb.append("EvidenceFolderId: ").append(getEvidenceFolderId()).append(",");
if (getId() != null)
sb.append("Id: ").append(getId()).append(",");
if (getAssessmentReportSelection() != null)
sb.append("AssessmentReportSelection: ").append(getAssessmentReportSelection());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Evidence == false)
return false;
Evidence other = (Evidence) obj;
if (other.getDataSource() == null ^ this.getDataSource() == null)
return false;
if (other.getDataSource() != null && other.getDataSource().equals(this.getDataSource()) == false)
return false;
if (other.getEvidenceAwsAccountId() == null ^ this.getEvidenceAwsAccountId() == null)
return false;
if (other.getEvidenceAwsAccountId() != null && other.getEvidenceAwsAccountId().equals(this.getEvidenceAwsAccountId()) == false)
return false;
if (other.getTime() == null ^ this.getTime() == null)
return false;
if (other.getTime() != null && other.getTime().equals(this.getTime()) == false)
return false;
if (other.getEventSource() == null ^ this.getEventSource() == null)
return false;
if (other.getEventSource() != null && other.getEventSource().equals(this.getEventSource()) == false)
return false;
if (other.getEventName() == null ^ this.getEventName() == null)
return false;
if (other.getEventName() != null && other.getEventName().equals(this.getEventName()) == false)
return false;
if (other.getEvidenceByType() == null ^ this.getEvidenceByType() == null)
return false;
if (other.getEvidenceByType() != null && other.getEvidenceByType().equals(this.getEvidenceByType()) == false)
return false;
if (other.getResourcesIncluded() == null ^ this.getResourcesIncluded() == null)
return false;
if (other.getResourcesIncluded() != null && other.getResourcesIncluded().equals(this.getResourcesIncluded()) == false)
return false;
if (other.getAttributes() == null ^ this.getAttributes() == null)
return false;
if (other.getAttributes() != null && other.getAttributes().equals(this.getAttributes()) == false)
return false;
if (other.getIamId() == null ^ this.getIamId() == null)
return false;
if (other.getIamId() != null && other.getIamId().equals(this.getIamId()) == false)
return false;
if (other.getComplianceCheck() == null ^ this.getComplianceCheck() == null)
return false;
if (other.getComplianceCheck() != null && other.getComplianceCheck().equals(this.getComplianceCheck()) == false)
return false;
if (other.getAwsOrganization() == null ^ this.getAwsOrganization() == null)
return false;
if (other.getAwsOrganization() != null && other.getAwsOrganization().equals(this.getAwsOrganization()) == false)
return false;
if (other.getAwsAccountId() == null ^ this.getAwsAccountId() == null)
return false;
if (other.getAwsAccountId() != null && other.getAwsAccountId().equals(this.getAwsAccountId()) == false)
return false;
if (other.getEvidenceFolderId() == null ^ this.getEvidenceFolderId() == null)
return false;
if (other.getEvidenceFolderId() != null && other.getEvidenceFolderId().equals(this.getEvidenceFolderId()) == false)
return false;
if (other.getId() == null ^ this.getId() == null)
return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false)
return false;
if (other.getAssessmentReportSelection() == null ^ this.getAssessmentReportSelection() == null)
return false;
if (other.getAssessmentReportSelection() != null && other.getAssessmentReportSelection().equals(this.getAssessmentReportSelection()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDataSource() == null) ? 0 : getDataSource().hashCode());
hashCode = prime * hashCode + ((getEvidenceAwsAccountId() == null) ? 0 : getEvidenceAwsAccountId().hashCode());
hashCode = prime * hashCode + ((getTime() == null) ? 0 : getTime().hashCode());
hashCode = prime * hashCode + ((getEventSource() == null) ? 0 : getEventSource().hashCode());
hashCode = prime * hashCode + ((getEventName() == null) ? 0 : getEventName().hashCode());
hashCode = prime * hashCode + ((getEvidenceByType() == null) ? 0 : getEvidenceByType().hashCode());
hashCode = prime * hashCode + ((getResourcesIncluded() == null) ? 0 : getResourcesIncluded().hashCode());
hashCode = prime * hashCode + ((getAttributes() == null) ? 0 : getAttributes().hashCode());
hashCode = prime * hashCode + ((getIamId() == null) ? 0 : getIamId().hashCode());
hashCode = prime * hashCode + ((getComplianceCheck() == null) ? 0 : getComplianceCheck().hashCode());
hashCode = prime * hashCode + ((getAwsOrganization() == null) ? 0 : getAwsOrganization().hashCode());
hashCode = prime * hashCode + ((getAwsAccountId() == null) ? 0 : getAwsAccountId().hashCode());
hashCode = prime * hashCode + ((getEvidenceFolderId() == null) ? 0 : getEvidenceFolderId().hashCode());
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getAssessmentReportSelection() == null) ? 0 : getAssessmentReportSelection().hashCode());
return hashCode;
}
@Override
public Evidence clone() {
try {
return (Evidence) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.auditmanager.model.transform.EvidenceMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}