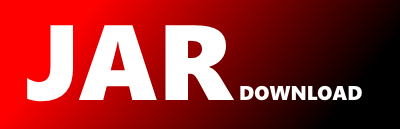
com.amazonaws.services.autoscaling.model.Ebs Maven / Gradle / Ivy
Show all versions of aws-java-sdk-autoscaling Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.autoscaling.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Describes an Amazon EBS volume. Used in combination with BlockDeviceMapping.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Ebs implements Serializable, Cloneable {
/**
*
* The snapshot ID of the volume to use.
*
*
* Conditional: This parameter is optional if you specify a volume size. If you specify both SnapshotId
* and VolumeSize
, VolumeSize
must be equal or greater than the size of the snapshot.
*
*/
private String snapshotId;
/**
*
* The volume size, in Gibibytes (GiB).
*
*
* This can be a number from 1-1,024 for standard
, 4-16,384 for io1
, 1-16,384 for
* gp2
, and 500-16,384 for st1
and sc1
. If you specify a snapshot, the volume
* size must be equal to or larger than the snapshot size.
*
*
* Default: If you create a volume from a snapshot and you don't specify a volume size, the default is the snapshot
* size.
*
*
*
* At least one of VolumeSize or SnapshotId is required.
*
*
*/
private Integer volumeSize;
/**
*
* The volume type, which can be standard
for Magnetic, io1
for Provisioned IOPS SSD,
* gp2
for General Purpose SSD, st1
for Throughput Optimized HDD, or sc1
for
* Cold HDD. For more information, see Amazon EBS Volume Types in the
* Amazon EC2 User Guide for Linux Instances.
*
*
* Valid Values: standard
| io1
| gp2
| st1
| sc1
*
*/
private String volumeType;
/**
*
* Indicates whether the volume is deleted on instance termination. For Amazon EC2 Auto Scaling, the default value
* is true
.
*
*/
private Boolean deleteOnTermination;
/**
*
* The number of I/O operations per second (IOPS) to provision for the volume. The maximum ratio of IOPS to volume
* size (in GiB) is 50:1. For more information, see Amazon EBS Volume Types in the
* Amazon EC2 User Guide for Linux Instances.
*
*
* Conditional: This parameter is required when the volume type is io1
. (Not used with
* standard
, gp2
, st1
, or sc1
volumes.)
*
*/
private Integer iops;
/**
*
* Specifies whether the volume should be encrypted. Encrypted EBS volumes can only be attached to instances that
* support Amazon EBS encryption. For more information, see Supported Instance Types. If your AMI uses encrypted volumes, you can also only launch it on supported
* instance types.
*
*
*
* If you are creating a volume from a snapshot, you cannot specify an encryption value. Volumes that are created
* from encrypted snapshots are automatically encrypted, and volumes that are created from unencrypted snapshots are
* automatically unencrypted. By default, encrypted snapshots use the AWS managed CMK that is used for EBS
* encryption, but you can specify a custom CMK when you create the snapshot. The ability to encrypt a snapshot
* during copying also allows you to apply a new CMK to an already-encrypted snapshot. Volumes restored from the
* resulting copy are only accessible using the new CMK.
*
*
* Enabling encryption by
* default results in all EBS volumes being encrypted with the AWS managed CMK or a customer managed CMK,
* whether or not the snapshot was encrypted.
*
*
*
* For more information, see Using
* Encryption with EBS-Backed AMIs in the Amazon EC2 User Guide for Linux Instances and Required
* CMK Key Policy for Use with Encrypted Volumes in the Amazon EC2 Auto Scaling User Guide.
*
*/
private Boolean encrypted;
/**
*
* The snapshot ID of the volume to use.
*
*
* Conditional: This parameter is optional if you specify a volume size. If you specify both SnapshotId
* and VolumeSize
, VolumeSize
must be equal or greater than the size of the snapshot.
*
*
* @param snapshotId
* The snapshot ID of the volume to use.
*
* Conditional: This parameter is optional if you specify a volume size. If you specify both
* SnapshotId
and VolumeSize
, VolumeSize
must be equal or greater than
* the size of the snapshot.
*/
public void setSnapshotId(String snapshotId) {
this.snapshotId = snapshotId;
}
/**
*
* The snapshot ID of the volume to use.
*
*
* Conditional: This parameter is optional if you specify a volume size. If you specify both SnapshotId
* and VolumeSize
, VolumeSize
must be equal or greater than the size of the snapshot.
*
*
* @return The snapshot ID of the volume to use.
*
* Conditional: This parameter is optional if you specify a volume size. If you specify both
* SnapshotId
and VolumeSize
, VolumeSize
must be equal or greater
* than the size of the snapshot.
*/
public String getSnapshotId() {
return this.snapshotId;
}
/**
*
* The snapshot ID of the volume to use.
*
*
* Conditional: This parameter is optional if you specify a volume size. If you specify both SnapshotId
* and VolumeSize
, VolumeSize
must be equal or greater than the size of the snapshot.
*
*
* @param snapshotId
* The snapshot ID of the volume to use.
*
* Conditional: This parameter is optional if you specify a volume size. If you specify both
* SnapshotId
and VolumeSize
, VolumeSize
must be equal or greater than
* the size of the snapshot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Ebs withSnapshotId(String snapshotId) {
setSnapshotId(snapshotId);
return this;
}
/**
*
* The volume size, in Gibibytes (GiB).
*
*
* This can be a number from 1-1,024 for standard
, 4-16,384 for io1
, 1-16,384 for
* gp2
, and 500-16,384 for st1
and sc1
. If you specify a snapshot, the volume
* size must be equal to or larger than the snapshot size.
*
*
* Default: If you create a volume from a snapshot and you don't specify a volume size, the default is the snapshot
* size.
*
*
*
* At least one of VolumeSize or SnapshotId is required.
*
*
*
* @param volumeSize
* The volume size, in Gibibytes (GiB).
*
* This can be a number from 1-1,024 for standard
, 4-16,384 for io1
, 1-16,384 for
* gp2
, and 500-16,384 for st1
and sc1
. If you specify a snapshot, the
* volume size must be equal to or larger than the snapshot size.
*
*
* Default: If you create a volume from a snapshot and you don't specify a volume size, the default is the
* snapshot size.
*
*
*
* At least one of VolumeSize or SnapshotId is required.
*
*/
public void setVolumeSize(Integer volumeSize) {
this.volumeSize = volumeSize;
}
/**
*
* The volume size, in Gibibytes (GiB).
*
*
* This can be a number from 1-1,024 for standard
, 4-16,384 for io1
, 1-16,384 for
* gp2
, and 500-16,384 for st1
and sc1
. If you specify a snapshot, the volume
* size must be equal to or larger than the snapshot size.
*
*
* Default: If you create a volume from a snapshot and you don't specify a volume size, the default is the snapshot
* size.
*
*
*
* At least one of VolumeSize or SnapshotId is required.
*
*
*
* @return The volume size, in Gibibytes (GiB).
*
* This can be a number from 1-1,024 for standard
, 4-16,384 for io1
, 1-16,384 for
* gp2
, and 500-16,384 for st1
and sc1
. If you specify a snapshot,
* the volume size must be equal to or larger than the snapshot size.
*
*
* Default: If you create a volume from a snapshot and you don't specify a volume size, the default is the
* snapshot size.
*
*
*
* At least one of VolumeSize or SnapshotId is required.
*
*/
public Integer getVolumeSize() {
return this.volumeSize;
}
/**
*
* The volume size, in Gibibytes (GiB).
*
*
* This can be a number from 1-1,024 for standard
, 4-16,384 for io1
, 1-16,384 for
* gp2
, and 500-16,384 for st1
and sc1
. If you specify a snapshot, the volume
* size must be equal to or larger than the snapshot size.
*
*
* Default: If you create a volume from a snapshot and you don't specify a volume size, the default is the snapshot
* size.
*
*
*
* At least one of VolumeSize or SnapshotId is required.
*
*
*
* @param volumeSize
* The volume size, in Gibibytes (GiB).
*
* This can be a number from 1-1,024 for standard
, 4-16,384 for io1
, 1-16,384 for
* gp2
, and 500-16,384 for st1
and sc1
. If you specify a snapshot, the
* volume size must be equal to or larger than the snapshot size.
*
*
* Default: If you create a volume from a snapshot and you don't specify a volume size, the default is the
* snapshot size.
*
*
*
* At least one of VolumeSize or SnapshotId is required.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Ebs withVolumeSize(Integer volumeSize) {
setVolumeSize(volumeSize);
return this;
}
/**
*
* The volume type, which can be standard
for Magnetic, io1
for Provisioned IOPS SSD,
* gp2
for General Purpose SSD, st1
for Throughput Optimized HDD, or sc1
for
* Cold HDD. For more information, see Amazon EBS Volume Types in the
* Amazon EC2 User Guide for Linux Instances.
*
*
* Valid Values: standard
| io1
| gp2
| st1
| sc1
*
*
* @param volumeType
* The volume type, which can be standard
for Magnetic, io1
for Provisioned IOPS
* SSD, gp2
for General Purpose SSD, st1
for Throughput Optimized HDD, or
* sc1
for Cold HDD. For more information, see Amazon EBS Volume Types
* in the Amazon EC2 User Guide for Linux Instances.
*
* Valid Values: standard
| io1
| gp2
| st1
|
* sc1
*/
public void setVolumeType(String volumeType) {
this.volumeType = volumeType;
}
/**
*
* The volume type, which can be standard
for Magnetic, io1
for Provisioned IOPS SSD,
* gp2
for General Purpose SSD, st1
for Throughput Optimized HDD, or sc1
for
* Cold HDD. For more information, see Amazon EBS Volume Types in the
* Amazon EC2 User Guide for Linux Instances.
*
*
* Valid Values: standard
| io1
| gp2
| st1
| sc1
*
*
* @return The volume type, which can be standard
for Magnetic, io1
for Provisioned IOPS
* SSD, gp2
for General Purpose SSD, st1
for Throughput Optimized HDD, or
* sc1
for Cold HDD. For more information, see Amazon EBS Volume
* Types in the Amazon EC2 User Guide for Linux Instances.
*
* Valid Values: standard
| io1
| gp2
| st1
|
* sc1
*/
public String getVolumeType() {
return this.volumeType;
}
/**
*
* The volume type, which can be standard
for Magnetic, io1
for Provisioned IOPS SSD,
* gp2
for General Purpose SSD, st1
for Throughput Optimized HDD, or sc1
for
* Cold HDD. For more information, see Amazon EBS Volume Types in the
* Amazon EC2 User Guide for Linux Instances.
*
*
* Valid Values: standard
| io1
| gp2
| st1
| sc1
*
*
* @param volumeType
* The volume type, which can be standard
for Magnetic, io1
for Provisioned IOPS
* SSD, gp2
for General Purpose SSD, st1
for Throughput Optimized HDD, or
* sc1
for Cold HDD. For more information, see Amazon EBS Volume Types
* in the Amazon EC2 User Guide for Linux Instances.
*
* Valid Values: standard
| io1
| gp2
| st1
|
* sc1
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Ebs withVolumeType(String volumeType) {
setVolumeType(volumeType);
return this;
}
/**
*
* Indicates whether the volume is deleted on instance termination. For Amazon EC2 Auto Scaling, the default value
* is true
.
*
*
* @param deleteOnTermination
* Indicates whether the volume is deleted on instance termination. For Amazon EC2 Auto Scaling, the default
* value is true
.
*/
public void setDeleteOnTermination(Boolean deleteOnTermination) {
this.deleteOnTermination = deleteOnTermination;
}
/**
*
* Indicates whether the volume is deleted on instance termination. For Amazon EC2 Auto Scaling, the default value
* is true
.
*
*
* @return Indicates whether the volume is deleted on instance termination. For Amazon EC2 Auto Scaling, the default
* value is true
.
*/
public Boolean getDeleteOnTermination() {
return this.deleteOnTermination;
}
/**
*
* Indicates whether the volume is deleted on instance termination. For Amazon EC2 Auto Scaling, the default value
* is true
.
*
*
* @param deleteOnTermination
* Indicates whether the volume is deleted on instance termination. For Amazon EC2 Auto Scaling, the default
* value is true
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Ebs withDeleteOnTermination(Boolean deleteOnTermination) {
setDeleteOnTermination(deleteOnTermination);
return this;
}
/**
*
* Indicates whether the volume is deleted on instance termination. For Amazon EC2 Auto Scaling, the default value
* is true
.
*
*
* @return Indicates whether the volume is deleted on instance termination. For Amazon EC2 Auto Scaling, the default
* value is true
.
*/
public Boolean isDeleteOnTermination() {
return this.deleteOnTermination;
}
/**
*
* The number of I/O operations per second (IOPS) to provision for the volume. The maximum ratio of IOPS to volume
* size (in GiB) is 50:1. For more information, see Amazon EBS Volume Types in the
* Amazon EC2 User Guide for Linux Instances.
*
*
* Conditional: This parameter is required when the volume type is io1
. (Not used with
* standard
, gp2
, st1
, or sc1
volumes.)
*
*
* @param iops
* The number of I/O operations per second (IOPS) to provision for the volume. The maximum ratio of IOPS to
* volume size (in GiB) is 50:1. For more information, see Amazon EBS Volume Types
* in the Amazon EC2 User Guide for Linux Instances.
*
* Conditional: This parameter is required when the volume type is io1
. (Not used with
* standard
, gp2
, st1
, or sc1
volumes.)
*/
public void setIops(Integer iops) {
this.iops = iops;
}
/**
*
* The number of I/O operations per second (IOPS) to provision for the volume. The maximum ratio of IOPS to volume
* size (in GiB) is 50:1. For more information, see Amazon EBS Volume Types in the
* Amazon EC2 User Guide for Linux Instances.
*
*
* Conditional: This parameter is required when the volume type is io1
. (Not used with
* standard
, gp2
, st1
, or sc1
volumes.)
*
*
* @return The number of I/O operations per second (IOPS) to provision for the volume. The maximum ratio of IOPS to
* volume size (in GiB) is 50:1. For more information, see Amazon EBS Volume
* Types in the Amazon EC2 User Guide for Linux Instances.
*
* Conditional: This parameter is required when the volume type is io1
. (Not used with
* standard
, gp2
, st1
, or sc1
volumes.)
*/
public Integer getIops() {
return this.iops;
}
/**
*
* The number of I/O operations per second (IOPS) to provision for the volume. The maximum ratio of IOPS to volume
* size (in GiB) is 50:1. For more information, see Amazon EBS Volume Types in the
* Amazon EC2 User Guide for Linux Instances.
*
*
* Conditional: This parameter is required when the volume type is io1
. (Not used with
* standard
, gp2
, st1
, or sc1
volumes.)
*
*
* @param iops
* The number of I/O operations per second (IOPS) to provision for the volume. The maximum ratio of IOPS to
* volume size (in GiB) is 50:1. For more information, see Amazon EBS Volume Types
* in the Amazon EC2 User Guide for Linux Instances.
*
* Conditional: This parameter is required when the volume type is io1
. (Not used with
* standard
, gp2
, st1
, or sc1
volumes.)
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Ebs withIops(Integer iops) {
setIops(iops);
return this;
}
/**
*
* Specifies whether the volume should be encrypted. Encrypted EBS volumes can only be attached to instances that
* support Amazon EBS encryption. For more information, see Supported Instance Types. If your AMI uses encrypted volumes, you can also only launch it on supported
* instance types.
*
*
*
* If you are creating a volume from a snapshot, you cannot specify an encryption value. Volumes that are created
* from encrypted snapshots are automatically encrypted, and volumes that are created from unencrypted snapshots are
* automatically unencrypted. By default, encrypted snapshots use the AWS managed CMK that is used for EBS
* encryption, but you can specify a custom CMK when you create the snapshot. The ability to encrypt a snapshot
* during copying also allows you to apply a new CMK to an already-encrypted snapshot. Volumes restored from the
* resulting copy are only accessible using the new CMK.
*
*
* Enabling encryption by
* default results in all EBS volumes being encrypted with the AWS managed CMK or a customer managed CMK,
* whether or not the snapshot was encrypted.
*
*
*
* For more information, see Using
* Encryption with EBS-Backed AMIs in the Amazon EC2 User Guide for Linux Instances and Required
* CMK Key Policy for Use with Encrypted Volumes in the Amazon EC2 Auto Scaling User Guide.
*
*
* @param encrypted
* Specifies whether the volume should be encrypted. Encrypted EBS volumes can only be attached to instances
* that support Amazon EBS encryption. For more information, see Supported Instance Types. If your AMI uses encrypted volumes, you can also only launch it on
* supported instance types.
*
* If you are creating a volume from a snapshot, you cannot specify an encryption value. Volumes that are
* created from encrypted snapshots are automatically encrypted, and volumes that are created from
* unencrypted snapshots are automatically unencrypted. By default, encrypted snapshots use the AWS managed
* CMK that is used for EBS encryption, but you can specify a custom CMK when you create the snapshot. The
* ability to encrypt a snapshot during copying also allows you to apply a new CMK to an already-encrypted
* snapshot. Volumes restored from the resulting copy are only accessible using the new CMK.
*
*
* Enabling encryption by default results in all EBS volumes being encrypted with the AWS managed CMK or a
* customer managed CMK, whether or not the snapshot was encrypted.
*
*
*
* For more information, see Using Encryption with
* EBS-Backed AMIs in the Amazon EC2 User Guide for Linux Instances and Required CMK Key Policy for Use with Encrypted Volumes in the Amazon EC2 Auto Scaling User
* Guide.
*/
public void setEncrypted(Boolean encrypted) {
this.encrypted = encrypted;
}
/**
*
* Specifies whether the volume should be encrypted. Encrypted EBS volumes can only be attached to instances that
* support Amazon EBS encryption. For more information, see Supported Instance Types. If your AMI uses encrypted volumes, you can also only launch it on supported
* instance types.
*
*
*
* If you are creating a volume from a snapshot, you cannot specify an encryption value. Volumes that are created
* from encrypted snapshots are automatically encrypted, and volumes that are created from unencrypted snapshots are
* automatically unencrypted. By default, encrypted snapshots use the AWS managed CMK that is used for EBS
* encryption, but you can specify a custom CMK when you create the snapshot. The ability to encrypt a snapshot
* during copying also allows you to apply a new CMK to an already-encrypted snapshot. Volumes restored from the
* resulting copy are only accessible using the new CMK.
*
*
* Enabling encryption by
* default results in all EBS volumes being encrypted with the AWS managed CMK or a customer managed CMK,
* whether or not the snapshot was encrypted.
*
*
*
* For more information, see Using
* Encryption with EBS-Backed AMIs in the Amazon EC2 User Guide for Linux Instances and Required
* CMK Key Policy for Use with Encrypted Volumes in the Amazon EC2 Auto Scaling User Guide.
*
*
* @return Specifies whether the volume should be encrypted. Encrypted EBS volumes can only be attached to instances
* that support Amazon EBS encryption. For more information, see Supported Instance Types. If your AMI uses encrypted volumes, you can also only launch it on
* supported instance types.
*
* If you are creating a volume from a snapshot, you cannot specify an encryption value. Volumes that are
* created from encrypted snapshots are automatically encrypted, and volumes that are created from
* unencrypted snapshots are automatically unencrypted. By default, encrypted snapshots use the AWS managed
* CMK that is used for EBS encryption, but you can specify a custom CMK when you create the snapshot. The
* ability to encrypt a snapshot during copying also allows you to apply a new CMK to an already-encrypted
* snapshot. Volumes restored from the resulting copy are only accessible using the new CMK.
*
*
* Enabling encryption by default results in all EBS volumes being encrypted with the AWS managed CMK or a
* customer managed CMK, whether or not the snapshot was encrypted.
*
*
*
* For more information, see Using Encryption with
* EBS-Backed AMIs in the Amazon EC2 User Guide for Linux Instances and Required CMK Key Policy for Use with Encrypted Volumes in the Amazon EC2 Auto Scaling User
* Guide.
*/
public Boolean getEncrypted() {
return this.encrypted;
}
/**
*
* Specifies whether the volume should be encrypted. Encrypted EBS volumes can only be attached to instances that
* support Amazon EBS encryption. For more information, see Supported Instance Types. If your AMI uses encrypted volumes, you can also only launch it on supported
* instance types.
*
*
*
* If you are creating a volume from a snapshot, you cannot specify an encryption value. Volumes that are created
* from encrypted snapshots are automatically encrypted, and volumes that are created from unencrypted snapshots are
* automatically unencrypted. By default, encrypted snapshots use the AWS managed CMK that is used for EBS
* encryption, but you can specify a custom CMK when you create the snapshot. The ability to encrypt a snapshot
* during copying also allows you to apply a new CMK to an already-encrypted snapshot. Volumes restored from the
* resulting copy are only accessible using the new CMK.
*
*
* Enabling encryption by
* default results in all EBS volumes being encrypted with the AWS managed CMK or a customer managed CMK,
* whether or not the snapshot was encrypted.
*
*
*
* For more information, see Using
* Encryption with EBS-Backed AMIs in the Amazon EC2 User Guide for Linux Instances and Required
* CMK Key Policy for Use with Encrypted Volumes in the Amazon EC2 Auto Scaling User Guide.
*
*
* @param encrypted
* Specifies whether the volume should be encrypted. Encrypted EBS volumes can only be attached to instances
* that support Amazon EBS encryption. For more information, see Supported Instance Types. If your AMI uses encrypted volumes, you can also only launch it on
* supported instance types.
*
* If you are creating a volume from a snapshot, you cannot specify an encryption value. Volumes that are
* created from encrypted snapshots are automatically encrypted, and volumes that are created from
* unencrypted snapshots are automatically unencrypted. By default, encrypted snapshots use the AWS managed
* CMK that is used for EBS encryption, but you can specify a custom CMK when you create the snapshot. The
* ability to encrypt a snapshot during copying also allows you to apply a new CMK to an already-encrypted
* snapshot. Volumes restored from the resulting copy are only accessible using the new CMK.
*
*
* Enabling encryption by default results in all EBS volumes being encrypted with the AWS managed CMK or a
* customer managed CMK, whether or not the snapshot was encrypted.
*
*
*
* For more information, see Using Encryption with
* EBS-Backed AMIs in the Amazon EC2 User Guide for Linux Instances and Required CMK Key Policy for Use with Encrypted Volumes in the Amazon EC2 Auto Scaling User
* Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Ebs withEncrypted(Boolean encrypted) {
setEncrypted(encrypted);
return this;
}
/**
*
* Specifies whether the volume should be encrypted. Encrypted EBS volumes can only be attached to instances that
* support Amazon EBS encryption. For more information, see Supported Instance Types. If your AMI uses encrypted volumes, you can also only launch it on supported
* instance types.
*
*
*
* If you are creating a volume from a snapshot, you cannot specify an encryption value. Volumes that are created
* from encrypted snapshots are automatically encrypted, and volumes that are created from unencrypted snapshots are
* automatically unencrypted. By default, encrypted snapshots use the AWS managed CMK that is used for EBS
* encryption, but you can specify a custom CMK when you create the snapshot. The ability to encrypt a snapshot
* during copying also allows you to apply a new CMK to an already-encrypted snapshot. Volumes restored from the
* resulting copy are only accessible using the new CMK.
*
*
* Enabling encryption by
* default results in all EBS volumes being encrypted with the AWS managed CMK or a customer managed CMK,
* whether or not the snapshot was encrypted.
*
*
*
* For more information, see Using
* Encryption with EBS-Backed AMIs in the Amazon EC2 User Guide for Linux Instances and Required
* CMK Key Policy for Use with Encrypted Volumes in the Amazon EC2 Auto Scaling User Guide.
*
*
* @return Specifies whether the volume should be encrypted. Encrypted EBS volumes can only be attached to instances
* that support Amazon EBS encryption. For more information, see Supported Instance Types. If your AMI uses encrypted volumes, you can also only launch it on
* supported instance types.
*
* If you are creating a volume from a snapshot, you cannot specify an encryption value. Volumes that are
* created from encrypted snapshots are automatically encrypted, and volumes that are created from
* unencrypted snapshots are automatically unencrypted. By default, encrypted snapshots use the AWS managed
* CMK that is used for EBS encryption, but you can specify a custom CMK when you create the snapshot. The
* ability to encrypt a snapshot during copying also allows you to apply a new CMK to an already-encrypted
* snapshot. Volumes restored from the resulting copy are only accessible using the new CMK.
*
*
* Enabling encryption by default results in all EBS volumes being encrypted with the AWS managed CMK or a
* customer managed CMK, whether or not the snapshot was encrypted.
*
*
*
* For more information, see Using Encryption with
* EBS-Backed AMIs in the Amazon EC2 User Guide for Linux Instances and Required CMK Key Policy for Use with Encrypted Volumes in the Amazon EC2 Auto Scaling User
* Guide.
*/
public Boolean isEncrypted() {
return this.encrypted;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getSnapshotId() != null)
sb.append("SnapshotId: ").append(getSnapshotId()).append(",");
if (getVolumeSize() != null)
sb.append("VolumeSize: ").append(getVolumeSize()).append(",");
if (getVolumeType() != null)
sb.append("VolumeType: ").append(getVolumeType()).append(",");
if (getDeleteOnTermination() != null)
sb.append("DeleteOnTermination: ").append(getDeleteOnTermination()).append(",");
if (getIops() != null)
sb.append("Iops: ").append(getIops()).append(",");
if (getEncrypted() != null)
sb.append("Encrypted: ").append(getEncrypted());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Ebs == false)
return false;
Ebs other = (Ebs) obj;
if (other.getSnapshotId() == null ^ this.getSnapshotId() == null)
return false;
if (other.getSnapshotId() != null && other.getSnapshotId().equals(this.getSnapshotId()) == false)
return false;
if (other.getVolumeSize() == null ^ this.getVolumeSize() == null)
return false;
if (other.getVolumeSize() != null && other.getVolumeSize().equals(this.getVolumeSize()) == false)
return false;
if (other.getVolumeType() == null ^ this.getVolumeType() == null)
return false;
if (other.getVolumeType() != null && other.getVolumeType().equals(this.getVolumeType()) == false)
return false;
if (other.getDeleteOnTermination() == null ^ this.getDeleteOnTermination() == null)
return false;
if (other.getDeleteOnTermination() != null && other.getDeleteOnTermination().equals(this.getDeleteOnTermination()) == false)
return false;
if (other.getIops() == null ^ this.getIops() == null)
return false;
if (other.getIops() != null && other.getIops().equals(this.getIops()) == false)
return false;
if (other.getEncrypted() == null ^ this.getEncrypted() == null)
return false;
if (other.getEncrypted() != null && other.getEncrypted().equals(this.getEncrypted()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getSnapshotId() == null) ? 0 : getSnapshotId().hashCode());
hashCode = prime * hashCode + ((getVolumeSize() == null) ? 0 : getVolumeSize().hashCode());
hashCode = prime * hashCode + ((getVolumeType() == null) ? 0 : getVolumeType().hashCode());
hashCode = prime * hashCode + ((getDeleteOnTermination() == null) ? 0 : getDeleteOnTermination().hashCode());
hashCode = prime * hashCode + ((getIops() == null) ? 0 : getIops().hashCode());
hashCode = prime * hashCode + ((getEncrypted() == null) ? 0 : getEncrypted().hashCode());
return hashCode;
}
@Override
public Ebs clone() {
try {
return (Ebs) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}