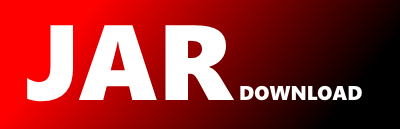
com.amazonaws.services.autoscaling.model.PredictiveScalingMetricSpecification Maven / Gradle / Ivy
Show all versions of aws-java-sdk-autoscaling Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.autoscaling.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* This structure specifies the metrics and target utilization settings for a predictive scaling policy.
*
*
* You must specify either a metric pair, or a load metric and a scaling metric individually. Specifying a metric pair
* instead of individual metrics provides a simpler way to configure metrics for a scaling policy. You choose the metric
* pair, and the policy automatically knows the correct sum and average statistics to use for the load metric and the
* scaling metric.
*
*
* Example
*
*
* -
*
* You create a predictive scaling policy and specify ALBRequestCount
as the value for the metric pair and
* 1000.0
as the target value. For this type of metric, you must provide the metric dimension for the
* corresponding target group, so you also provide a resource label for the Application Load Balancer target group that
* is attached to your Auto Scaling group.
*
*
* -
*
* The number of requests the target group receives per minute provides the load metric, and the request count averaged
* between the members of the target group provides the scaling metric. In CloudWatch, this refers to the
* RequestCount
and RequestCountPerTarget
metrics, respectively.
*
*
* -
*
* For optimal use of predictive scaling, you adhere to the best practice of using a dynamic scaling policy to
* automatically scale between the minimum capacity and maximum capacity in response to real-time changes in resource
* utilization.
*
*
* -
*
* Amazon EC2 Auto Scaling consumes data points for the load metric over the last 14 days and creates an hourly load
* forecast for predictive scaling. (A minimum of 24 hours of data is required.)
*
*
* -
*
* After creating the load forecast, Amazon EC2 Auto Scaling determines when to reduce or increase the capacity of your
* Auto Scaling group in each hour of the forecast period so that the average number of requests received by each
* instance is as close to 1000 requests per minute as possible at all times.
*
*
*
*
* For information about using custom metrics with predictive scaling, see Advanced predictive scaling policy configurations using custom metrics in the Amazon EC2 Auto Scaling User
* Guide.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PredictiveScalingMetricSpecification implements Serializable, Cloneable {
/**
*
* Specifies the target utilization.
*
*
*
* Some metrics are based on a count instead of a percentage, such as the request count for an Application Load
* Balancer or the number of messages in an SQS queue. If the scaling policy specifies one of these metrics, specify
* the target utilization as the optimal average request or message count per instance during any one-minute
* interval.
*
*
*/
private Double targetValue;
/**
*
* The predefined metric pair specification from which Amazon EC2 Auto Scaling determines the appropriate scaling
* metric and load metric to use.
*
*/
private PredictiveScalingPredefinedMetricPair predefinedMetricPairSpecification;
/**
*
* The predefined scaling metric specification.
*
*/
private PredictiveScalingPredefinedScalingMetric predefinedScalingMetricSpecification;
/**
*
* The predefined load metric specification.
*
*/
private PredictiveScalingPredefinedLoadMetric predefinedLoadMetricSpecification;
/**
*
* The customized scaling metric specification.
*
*/
private PredictiveScalingCustomizedScalingMetric customizedScalingMetricSpecification;
/**
*
* The customized load metric specification.
*
*/
private PredictiveScalingCustomizedLoadMetric customizedLoadMetricSpecification;
/**
*
* The customized capacity metric specification.
*
*/
private PredictiveScalingCustomizedCapacityMetric customizedCapacityMetricSpecification;
/**
*
* Specifies the target utilization.
*
*
*
* Some metrics are based on a count instead of a percentage, such as the request count for an Application Load
* Balancer or the number of messages in an SQS queue. If the scaling policy specifies one of these metrics, specify
* the target utilization as the optimal average request or message count per instance during any one-minute
* interval.
*
*
*
* @param targetValue
* Specifies the target utilization.
*
* Some metrics are based on a count instead of a percentage, such as the request count for an Application
* Load Balancer or the number of messages in an SQS queue. If the scaling policy specifies one of these
* metrics, specify the target utilization as the optimal average request or message count per instance
* during any one-minute interval.
*
*/
public void setTargetValue(Double targetValue) {
this.targetValue = targetValue;
}
/**
*
* Specifies the target utilization.
*
*
*
* Some metrics are based on a count instead of a percentage, such as the request count for an Application Load
* Balancer or the number of messages in an SQS queue. If the scaling policy specifies one of these metrics, specify
* the target utilization as the optimal average request or message count per instance during any one-minute
* interval.
*
*
*
* @return Specifies the target utilization.
*
* Some metrics are based on a count instead of a percentage, such as the request count for an Application
* Load Balancer or the number of messages in an SQS queue. If the scaling policy specifies one of these
* metrics, specify the target utilization as the optimal average request or message count per instance
* during any one-minute interval.
*
*/
public Double getTargetValue() {
return this.targetValue;
}
/**
*
* Specifies the target utilization.
*
*
*
* Some metrics are based on a count instead of a percentage, such as the request count for an Application Load
* Balancer or the number of messages in an SQS queue. If the scaling policy specifies one of these metrics, specify
* the target utilization as the optimal average request or message count per instance during any one-minute
* interval.
*
*
*
* @param targetValue
* Specifies the target utilization.
*
* Some metrics are based on a count instead of a percentage, such as the request count for an Application
* Load Balancer or the number of messages in an SQS queue. If the scaling policy specifies one of these
* metrics, specify the target utilization as the optimal average request or message count per instance
* during any one-minute interval.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PredictiveScalingMetricSpecification withTargetValue(Double targetValue) {
setTargetValue(targetValue);
return this;
}
/**
*
* The predefined metric pair specification from which Amazon EC2 Auto Scaling determines the appropriate scaling
* metric and load metric to use.
*
*
* @param predefinedMetricPairSpecification
* The predefined metric pair specification from which Amazon EC2 Auto Scaling determines the appropriate
* scaling metric and load metric to use.
*/
public void setPredefinedMetricPairSpecification(PredictiveScalingPredefinedMetricPair predefinedMetricPairSpecification) {
this.predefinedMetricPairSpecification = predefinedMetricPairSpecification;
}
/**
*
* The predefined metric pair specification from which Amazon EC2 Auto Scaling determines the appropriate scaling
* metric and load metric to use.
*
*
* @return The predefined metric pair specification from which Amazon EC2 Auto Scaling determines the appropriate
* scaling metric and load metric to use.
*/
public PredictiveScalingPredefinedMetricPair getPredefinedMetricPairSpecification() {
return this.predefinedMetricPairSpecification;
}
/**
*
* The predefined metric pair specification from which Amazon EC2 Auto Scaling determines the appropriate scaling
* metric and load metric to use.
*
*
* @param predefinedMetricPairSpecification
* The predefined metric pair specification from which Amazon EC2 Auto Scaling determines the appropriate
* scaling metric and load metric to use.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PredictiveScalingMetricSpecification withPredefinedMetricPairSpecification(PredictiveScalingPredefinedMetricPair predefinedMetricPairSpecification) {
setPredefinedMetricPairSpecification(predefinedMetricPairSpecification);
return this;
}
/**
*
* The predefined scaling metric specification.
*
*
* @param predefinedScalingMetricSpecification
* The predefined scaling metric specification.
*/
public void setPredefinedScalingMetricSpecification(PredictiveScalingPredefinedScalingMetric predefinedScalingMetricSpecification) {
this.predefinedScalingMetricSpecification = predefinedScalingMetricSpecification;
}
/**
*
* The predefined scaling metric specification.
*
*
* @return The predefined scaling metric specification.
*/
public PredictiveScalingPredefinedScalingMetric getPredefinedScalingMetricSpecification() {
return this.predefinedScalingMetricSpecification;
}
/**
*
* The predefined scaling metric specification.
*
*
* @param predefinedScalingMetricSpecification
* The predefined scaling metric specification.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PredictiveScalingMetricSpecification withPredefinedScalingMetricSpecification(
PredictiveScalingPredefinedScalingMetric predefinedScalingMetricSpecification) {
setPredefinedScalingMetricSpecification(predefinedScalingMetricSpecification);
return this;
}
/**
*
* The predefined load metric specification.
*
*
* @param predefinedLoadMetricSpecification
* The predefined load metric specification.
*/
public void setPredefinedLoadMetricSpecification(PredictiveScalingPredefinedLoadMetric predefinedLoadMetricSpecification) {
this.predefinedLoadMetricSpecification = predefinedLoadMetricSpecification;
}
/**
*
* The predefined load metric specification.
*
*
* @return The predefined load metric specification.
*/
public PredictiveScalingPredefinedLoadMetric getPredefinedLoadMetricSpecification() {
return this.predefinedLoadMetricSpecification;
}
/**
*
* The predefined load metric specification.
*
*
* @param predefinedLoadMetricSpecification
* The predefined load metric specification.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PredictiveScalingMetricSpecification withPredefinedLoadMetricSpecification(PredictiveScalingPredefinedLoadMetric predefinedLoadMetricSpecification) {
setPredefinedLoadMetricSpecification(predefinedLoadMetricSpecification);
return this;
}
/**
*
* The customized scaling metric specification.
*
*
* @param customizedScalingMetricSpecification
* The customized scaling metric specification.
*/
public void setCustomizedScalingMetricSpecification(PredictiveScalingCustomizedScalingMetric customizedScalingMetricSpecification) {
this.customizedScalingMetricSpecification = customizedScalingMetricSpecification;
}
/**
*
* The customized scaling metric specification.
*
*
* @return The customized scaling metric specification.
*/
public PredictiveScalingCustomizedScalingMetric getCustomizedScalingMetricSpecification() {
return this.customizedScalingMetricSpecification;
}
/**
*
* The customized scaling metric specification.
*
*
* @param customizedScalingMetricSpecification
* The customized scaling metric specification.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PredictiveScalingMetricSpecification withCustomizedScalingMetricSpecification(
PredictiveScalingCustomizedScalingMetric customizedScalingMetricSpecification) {
setCustomizedScalingMetricSpecification(customizedScalingMetricSpecification);
return this;
}
/**
*
* The customized load metric specification.
*
*
* @param customizedLoadMetricSpecification
* The customized load metric specification.
*/
public void setCustomizedLoadMetricSpecification(PredictiveScalingCustomizedLoadMetric customizedLoadMetricSpecification) {
this.customizedLoadMetricSpecification = customizedLoadMetricSpecification;
}
/**
*
* The customized load metric specification.
*
*
* @return The customized load metric specification.
*/
public PredictiveScalingCustomizedLoadMetric getCustomizedLoadMetricSpecification() {
return this.customizedLoadMetricSpecification;
}
/**
*
* The customized load metric specification.
*
*
* @param customizedLoadMetricSpecification
* The customized load metric specification.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PredictiveScalingMetricSpecification withCustomizedLoadMetricSpecification(PredictiveScalingCustomizedLoadMetric customizedLoadMetricSpecification) {
setCustomizedLoadMetricSpecification(customizedLoadMetricSpecification);
return this;
}
/**
*
* The customized capacity metric specification.
*
*
* @param customizedCapacityMetricSpecification
* The customized capacity metric specification.
*/
public void setCustomizedCapacityMetricSpecification(PredictiveScalingCustomizedCapacityMetric customizedCapacityMetricSpecification) {
this.customizedCapacityMetricSpecification = customizedCapacityMetricSpecification;
}
/**
*
* The customized capacity metric specification.
*
*
* @return The customized capacity metric specification.
*/
public PredictiveScalingCustomizedCapacityMetric getCustomizedCapacityMetricSpecification() {
return this.customizedCapacityMetricSpecification;
}
/**
*
* The customized capacity metric specification.
*
*
* @param customizedCapacityMetricSpecification
* The customized capacity metric specification.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PredictiveScalingMetricSpecification withCustomizedCapacityMetricSpecification(
PredictiveScalingCustomizedCapacityMetric customizedCapacityMetricSpecification) {
setCustomizedCapacityMetricSpecification(customizedCapacityMetricSpecification);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTargetValue() != null)
sb.append("TargetValue: ").append(getTargetValue()).append(",");
if (getPredefinedMetricPairSpecification() != null)
sb.append("PredefinedMetricPairSpecification: ").append(getPredefinedMetricPairSpecification()).append(",");
if (getPredefinedScalingMetricSpecification() != null)
sb.append("PredefinedScalingMetricSpecification: ").append(getPredefinedScalingMetricSpecification()).append(",");
if (getPredefinedLoadMetricSpecification() != null)
sb.append("PredefinedLoadMetricSpecification: ").append(getPredefinedLoadMetricSpecification()).append(",");
if (getCustomizedScalingMetricSpecification() != null)
sb.append("CustomizedScalingMetricSpecification: ").append(getCustomizedScalingMetricSpecification()).append(",");
if (getCustomizedLoadMetricSpecification() != null)
sb.append("CustomizedLoadMetricSpecification: ").append(getCustomizedLoadMetricSpecification()).append(",");
if (getCustomizedCapacityMetricSpecification() != null)
sb.append("CustomizedCapacityMetricSpecification: ").append(getCustomizedCapacityMetricSpecification());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PredictiveScalingMetricSpecification == false)
return false;
PredictiveScalingMetricSpecification other = (PredictiveScalingMetricSpecification) obj;
if (other.getTargetValue() == null ^ this.getTargetValue() == null)
return false;
if (other.getTargetValue() != null && other.getTargetValue().equals(this.getTargetValue()) == false)
return false;
if (other.getPredefinedMetricPairSpecification() == null ^ this.getPredefinedMetricPairSpecification() == null)
return false;
if (other.getPredefinedMetricPairSpecification() != null
&& other.getPredefinedMetricPairSpecification().equals(this.getPredefinedMetricPairSpecification()) == false)
return false;
if (other.getPredefinedScalingMetricSpecification() == null ^ this.getPredefinedScalingMetricSpecification() == null)
return false;
if (other.getPredefinedScalingMetricSpecification() != null
&& other.getPredefinedScalingMetricSpecification().equals(this.getPredefinedScalingMetricSpecification()) == false)
return false;
if (other.getPredefinedLoadMetricSpecification() == null ^ this.getPredefinedLoadMetricSpecification() == null)
return false;
if (other.getPredefinedLoadMetricSpecification() != null
&& other.getPredefinedLoadMetricSpecification().equals(this.getPredefinedLoadMetricSpecification()) == false)
return false;
if (other.getCustomizedScalingMetricSpecification() == null ^ this.getCustomizedScalingMetricSpecification() == null)
return false;
if (other.getCustomizedScalingMetricSpecification() != null
&& other.getCustomizedScalingMetricSpecification().equals(this.getCustomizedScalingMetricSpecification()) == false)
return false;
if (other.getCustomizedLoadMetricSpecification() == null ^ this.getCustomizedLoadMetricSpecification() == null)
return false;
if (other.getCustomizedLoadMetricSpecification() != null
&& other.getCustomizedLoadMetricSpecification().equals(this.getCustomizedLoadMetricSpecification()) == false)
return false;
if (other.getCustomizedCapacityMetricSpecification() == null ^ this.getCustomizedCapacityMetricSpecification() == null)
return false;
if (other.getCustomizedCapacityMetricSpecification() != null
&& other.getCustomizedCapacityMetricSpecification().equals(this.getCustomizedCapacityMetricSpecification()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTargetValue() == null) ? 0 : getTargetValue().hashCode());
hashCode = prime * hashCode + ((getPredefinedMetricPairSpecification() == null) ? 0 : getPredefinedMetricPairSpecification().hashCode());
hashCode = prime * hashCode + ((getPredefinedScalingMetricSpecification() == null) ? 0 : getPredefinedScalingMetricSpecification().hashCode());
hashCode = prime * hashCode + ((getPredefinedLoadMetricSpecification() == null) ? 0 : getPredefinedLoadMetricSpecification().hashCode());
hashCode = prime * hashCode + ((getCustomizedScalingMetricSpecification() == null) ? 0 : getCustomizedScalingMetricSpecification().hashCode());
hashCode = prime * hashCode + ((getCustomizedLoadMetricSpecification() == null) ? 0 : getCustomizedLoadMetricSpecification().hashCode());
hashCode = prime * hashCode + ((getCustomizedCapacityMetricSpecification() == null) ? 0 : getCustomizedCapacityMetricSpecification().hashCode());
return hashCode;
}
@Override
public PredictiveScalingMetricSpecification clone() {
try {
return (PredictiveScalingMetricSpecification) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}