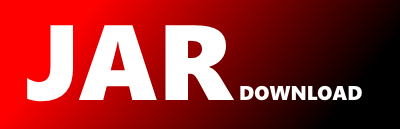
com.amazonaws.services.backup.model.StartRestoreJobRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-backup Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.backup.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StartRestoreJobRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* An ARN that uniquely identifies a recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*
*/
private String recoveryPointArn;
/**
*
* A set of metadata key-value pairs. Contains information, such as a resource name, required to restore a recovery
* point.
*
*
* You can get configuration metadata about a resource at the time it was backed up by calling
* GetRecoveryPointRestoreMetadata
. However, values in addition to those provided by
* GetRecoveryPointRestoreMetadata
might be required to restore a resource. For example, you might need
* to provide a new resource name if the original already exists.
*
*
* You need to specify specific metadata to restore an Amazon Elastic File System (Amazon EFS) instance:
*
*
* -
*
* file-system-id
: The ID of the Amazon EFS file system that is backed up by Backup. Returned in
* GetRecoveryPointRestoreMetadata
.
*
*
* -
*
* Encrypted
: A Boolean value that, if true, specifies that the file system is encrypted. If
* KmsKeyId
is specified, Encrypted
must be set to true
.
*
*
* -
*
* KmsKeyId
: Specifies the Amazon Web Services KMS key that is used to encrypt the restored file
* system. You can specify a key from another Amazon Web Services account provided that key it is properly shared
* with your account via Amazon Web Services KMS.
*
*
* -
*
* PerformanceMode
: Specifies the throughput mode of the file system.
*
*
* -
*
* CreationToken
: A user-supplied value that ensures the uniqueness (idempotency) of the request.
*
*
* -
*
* newFileSystem
: A Boolean value that, if true, specifies that the recovery point is restored to a new
* Amazon EFS file system.
*
*
* -
*
* ItemsToRestore
: An array of one to five strings where each string is a file path. Use
* ItemsToRestore
to restore specific files or directories rather than the entire file system. This
* parameter is optional. For example, "itemsToRestore":"[\"/my.test\"]"
.
*
*
*
*/
private java.util.Map metadata;
/**
*
* The Amazon Resource Name (ARN) of the IAM role that Backup uses to create the target resource; for example:
* arn:aws:iam::123456789012:role/S3Access
.
*
*/
private String iamRoleArn;
/**
*
* A customer-chosen string that you can use to distinguish between otherwise identical calls to
* StartRestoreJob
. Retrying a successful request with the same idempotency token results in a success
* message with no action taken.
*
*/
private String idempotencyToken;
/**
*
* Starts a job to restore a recovery point for one of the following resources:
*
*
* -
*
* Aurora
for Amazon Aurora
*
*
* -
*
* DocumentDB
for Amazon DocumentDB (with MongoDB compatibility)
*
*
* -
*
* DynamoDB
for Amazon DynamoDB
*
*
* -
*
* EBS
for Amazon Elastic Block Store
*
*
* -
*
* EC2
for Amazon Elastic Compute Cloud
*
*
* -
*
* EFS
for Amazon Elastic File System
*
*
* -
*
* FSx
for Amazon FSx
*
*
* -
*
* Neptune
for Amazon Neptune
*
*
* -
*
* RDS
for Amazon Relational Database Service
*
*
* -
*
* Storage Gateway
for Storage Gateway
*
*
* -
*
* S3
for Amazon S3
*
*
* -
*
* VirtualMachine
for virtual machines
*
*
*
*/
private String resourceType;
/**
*
* An ARN that uniquely identifies a recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*
*
* @param recoveryPointArn
* An ARN that uniquely identifies a recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*/
public void setRecoveryPointArn(String recoveryPointArn) {
this.recoveryPointArn = recoveryPointArn;
}
/**
*
* An ARN that uniquely identifies a recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*
*
* @return An ARN that uniquely identifies a recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*/
public String getRecoveryPointArn() {
return this.recoveryPointArn;
}
/**
*
* An ARN that uniquely identifies a recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*
*
* @param recoveryPointArn
* An ARN that uniquely identifies a recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartRestoreJobRequest withRecoveryPointArn(String recoveryPointArn) {
setRecoveryPointArn(recoveryPointArn);
return this;
}
/**
*
* A set of metadata key-value pairs. Contains information, such as a resource name, required to restore a recovery
* point.
*
*
* You can get configuration metadata about a resource at the time it was backed up by calling
* GetRecoveryPointRestoreMetadata
. However, values in addition to those provided by
* GetRecoveryPointRestoreMetadata
might be required to restore a resource. For example, you might need
* to provide a new resource name if the original already exists.
*
*
* You need to specify specific metadata to restore an Amazon Elastic File System (Amazon EFS) instance:
*
*
* -
*
* file-system-id
: The ID of the Amazon EFS file system that is backed up by Backup. Returned in
* GetRecoveryPointRestoreMetadata
.
*
*
* -
*
* Encrypted
: A Boolean value that, if true, specifies that the file system is encrypted. If
* KmsKeyId
is specified, Encrypted
must be set to true
.
*
*
* -
*
* KmsKeyId
: Specifies the Amazon Web Services KMS key that is used to encrypt the restored file
* system. You can specify a key from another Amazon Web Services account provided that key it is properly shared
* with your account via Amazon Web Services KMS.
*
*
* -
*
* PerformanceMode
: Specifies the throughput mode of the file system.
*
*
* -
*
* CreationToken
: A user-supplied value that ensures the uniqueness (idempotency) of the request.
*
*
* -
*
* newFileSystem
: A Boolean value that, if true, specifies that the recovery point is restored to a new
* Amazon EFS file system.
*
*
* -
*
* ItemsToRestore
: An array of one to five strings where each string is a file path. Use
* ItemsToRestore
to restore specific files or directories rather than the entire file system. This
* parameter is optional. For example, "itemsToRestore":"[\"/my.test\"]"
.
*
*
*
*
* @return A set of metadata key-value pairs. Contains information, such as a resource name, required to restore a
* recovery point.
*
* You can get configuration metadata about a resource at the time it was backed up by calling
* GetRecoveryPointRestoreMetadata
. However, values in addition to those provided by
* GetRecoveryPointRestoreMetadata
might be required to restore a resource. For example, you
* might need to provide a new resource name if the original already exists.
*
*
* You need to specify specific metadata to restore an Amazon Elastic File System (Amazon EFS) instance:
*
*
* -
*
* file-system-id
: The ID of the Amazon EFS file system that is backed up by Backup. Returned
* in GetRecoveryPointRestoreMetadata
.
*
*
* -
*
* Encrypted
: A Boolean value that, if true, specifies that the file system is encrypted. If
* KmsKeyId
is specified, Encrypted
must be set to true
.
*
*
* -
*
* KmsKeyId
: Specifies the Amazon Web Services KMS key that is used to encrypt the restored
* file system. You can specify a key from another Amazon Web Services account provided that key it is
* properly shared with your account via Amazon Web Services KMS.
*
*
* -
*
* PerformanceMode
: Specifies the throughput mode of the file system.
*
*
* -
*
* CreationToken
: A user-supplied value that ensures the uniqueness (idempotency) of the
* request.
*
*
* -
*
* newFileSystem
: A Boolean value that, if true, specifies that the recovery point is restored
* to a new Amazon EFS file system.
*
*
* -
*
* ItemsToRestore
: An array of one to five strings where each string is a file path. Use
* ItemsToRestore
to restore specific files or directories rather than the entire file system.
* This parameter is optional. For example, "itemsToRestore":"[\"/my.test\"]"
.
*
*
*/
public java.util.Map getMetadata() {
return metadata;
}
/**
*
* A set of metadata key-value pairs. Contains information, such as a resource name, required to restore a recovery
* point.
*
*
* You can get configuration metadata about a resource at the time it was backed up by calling
* GetRecoveryPointRestoreMetadata
. However, values in addition to those provided by
* GetRecoveryPointRestoreMetadata
might be required to restore a resource. For example, you might need
* to provide a new resource name if the original already exists.
*
*
* You need to specify specific metadata to restore an Amazon Elastic File System (Amazon EFS) instance:
*
*
* -
*
* file-system-id
: The ID of the Amazon EFS file system that is backed up by Backup. Returned in
* GetRecoveryPointRestoreMetadata
.
*
*
* -
*
* Encrypted
: A Boolean value that, if true, specifies that the file system is encrypted. If
* KmsKeyId
is specified, Encrypted
must be set to true
.
*
*
* -
*
* KmsKeyId
: Specifies the Amazon Web Services KMS key that is used to encrypt the restored file
* system. You can specify a key from another Amazon Web Services account provided that key it is properly shared
* with your account via Amazon Web Services KMS.
*
*
* -
*
* PerformanceMode
: Specifies the throughput mode of the file system.
*
*
* -
*
* CreationToken
: A user-supplied value that ensures the uniqueness (idempotency) of the request.
*
*
* -
*
* newFileSystem
: A Boolean value that, if true, specifies that the recovery point is restored to a new
* Amazon EFS file system.
*
*
* -
*
* ItemsToRestore
: An array of one to five strings where each string is a file path. Use
* ItemsToRestore
to restore specific files or directories rather than the entire file system. This
* parameter is optional. For example, "itemsToRestore":"[\"/my.test\"]"
.
*
*
*
*
* @param metadata
* A set of metadata key-value pairs. Contains information, such as a resource name, required to restore a
* recovery point.
*
* You can get configuration metadata about a resource at the time it was backed up by calling
* GetRecoveryPointRestoreMetadata
. However, values in addition to those provided by
* GetRecoveryPointRestoreMetadata
might be required to restore a resource. For example, you
* might need to provide a new resource name if the original already exists.
*
*
* You need to specify specific metadata to restore an Amazon Elastic File System (Amazon EFS) instance:
*
*
* -
*
* file-system-id
: The ID of the Amazon EFS file system that is backed up by Backup. Returned in
* GetRecoveryPointRestoreMetadata
.
*
*
* -
*
* Encrypted
: A Boolean value that, if true, specifies that the file system is encrypted. If
* KmsKeyId
is specified, Encrypted
must be set to true
.
*
*
* -
*
* KmsKeyId
: Specifies the Amazon Web Services KMS key that is used to encrypt the restored file
* system. You can specify a key from another Amazon Web Services account provided that key it is properly
* shared with your account via Amazon Web Services KMS.
*
*
* -
*
* PerformanceMode
: Specifies the throughput mode of the file system.
*
*
* -
*
* CreationToken
: A user-supplied value that ensures the uniqueness (idempotency) of the
* request.
*
*
* -
*
* newFileSystem
: A Boolean value that, if true, specifies that the recovery point is restored
* to a new Amazon EFS file system.
*
*
* -
*
* ItemsToRestore
: An array of one to five strings where each string is a file path. Use
* ItemsToRestore
to restore specific files or directories rather than the entire file system.
* This parameter is optional. For example, "itemsToRestore":"[\"/my.test\"]"
.
*
*
*/
public void setMetadata(java.util.Map metadata) {
this.metadata = metadata;
}
/**
*
* A set of metadata key-value pairs. Contains information, such as a resource name, required to restore a recovery
* point.
*
*
* You can get configuration metadata about a resource at the time it was backed up by calling
* GetRecoveryPointRestoreMetadata
. However, values in addition to those provided by
* GetRecoveryPointRestoreMetadata
might be required to restore a resource. For example, you might need
* to provide a new resource name if the original already exists.
*
*
* You need to specify specific metadata to restore an Amazon Elastic File System (Amazon EFS) instance:
*
*
* -
*
* file-system-id
: The ID of the Amazon EFS file system that is backed up by Backup. Returned in
* GetRecoveryPointRestoreMetadata
.
*
*
* -
*
* Encrypted
: A Boolean value that, if true, specifies that the file system is encrypted. If
* KmsKeyId
is specified, Encrypted
must be set to true
.
*
*
* -
*
* KmsKeyId
: Specifies the Amazon Web Services KMS key that is used to encrypt the restored file
* system. You can specify a key from another Amazon Web Services account provided that key it is properly shared
* with your account via Amazon Web Services KMS.
*
*
* -
*
* PerformanceMode
: Specifies the throughput mode of the file system.
*
*
* -
*
* CreationToken
: A user-supplied value that ensures the uniqueness (idempotency) of the request.
*
*
* -
*
* newFileSystem
: A Boolean value that, if true, specifies that the recovery point is restored to a new
* Amazon EFS file system.
*
*
* -
*
* ItemsToRestore
: An array of one to five strings where each string is a file path. Use
* ItemsToRestore
to restore specific files or directories rather than the entire file system. This
* parameter is optional. For example, "itemsToRestore":"[\"/my.test\"]"
.
*
*
*
*
* @param metadata
* A set of metadata key-value pairs. Contains information, such as a resource name, required to restore a
* recovery point.
*
* You can get configuration metadata about a resource at the time it was backed up by calling
* GetRecoveryPointRestoreMetadata
. However, values in addition to those provided by
* GetRecoveryPointRestoreMetadata
might be required to restore a resource. For example, you
* might need to provide a new resource name if the original already exists.
*
*
* You need to specify specific metadata to restore an Amazon Elastic File System (Amazon EFS) instance:
*
*
* -
*
* file-system-id
: The ID of the Amazon EFS file system that is backed up by Backup. Returned in
* GetRecoveryPointRestoreMetadata
.
*
*
* -
*
* Encrypted
: A Boolean value that, if true, specifies that the file system is encrypted. If
* KmsKeyId
is specified, Encrypted
must be set to true
.
*
*
* -
*
* KmsKeyId
: Specifies the Amazon Web Services KMS key that is used to encrypt the restored file
* system. You can specify a key from another Amazon Web Services account provided that key it is properly
* shared with your account via Amazon Web Services KMS.
*
*
* -
*
* PerformanceMode
: Specifies the throughput mode of the file system.
*
*
* -
*
* CreationToken
: A user-supplied value that ensures the uniqueness (idempotency) of the
* request.
*
*
* -
*
* newFileSystem
: A Boolean value that, if true, specifies that the recovery point is restored
* to a new Amazon EFS file system.
*
*
* -
*
* ItemsToRestore
: An array of one to five strings where each string is a file path. Use
* ItemsToRestore
to restore specific files or directories rather than the entire file system.
* This parameter is optional. For example, "itemsToRestore":"[\"/my.test\"]"
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartRestoreJobRequest withMetadata(java.util.Map metadata) {
setMetadata(metadata);
return this;
}
/**
* Add a single Metadata entry
*
* @see StartRestoreJobRequest#withMetadata
* @returns a reference to this object so that method calls can be chained together.
*/
public StartRestoreJobRequest addMetadataEntry(String key, String value) {
if (null == this.metadata) {
this.metadata = new java.util.HashMap();
}
if (this.metadata.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.metadata.put(key, value);
return this;
}
/**
* Removes all the entries added into Metadata.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartRestoreJobRequest clearMetadataEntries() {
this.metadata = null;
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role that Backup uses to create the target resource; for example:
* arn:aws:iam::123456789012:role/S3Access
.
*
*
* @param iamRoleArn
* The Amazon Resource Name (ARN) of the IAM role that Backup uses to create the target resource; for
* example: arn:aws:iam::123456789012:role/S3Access
.
*/
public void setIamRoleArn(String iamRoleArn) {
this.iamRoleArn = iamRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role that Backup uses to create the target resource; for example:
* arn:aws:iam::123456789012:role/S3Access
.
*
*
* @return The Amazon Resource Name (ARN) of the IAM role that Backup uses to create the target resource; for
* example: arn:aws:iam::123456789012:role/S3Access
.
*/
public String getIamRoleArn() {
return this.iamRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role that Backup uses to create the target resource; for example:
* arn:aws:iam::123456789012:role/S3Access
.
*
*
* @param iamRoleArn
* The Amazon Resource Name (ARN) of the IAM role that Backup uses to create the target resource; for
* example: arn:aws:iam::123456789012:role/S3Access
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartRestoreJobRequest withIamRoleArn(String iamRoleArn) {
setIamRoleArn(iamRoleArn);
return this;
}
/**
*
* A customer-chosen string that you can use to distinguish between otherwise identical calls to
* StartRestoreJob
. Retrying a successful request with the same idempotency token results in a success
* message with no action taken.
*
*
* @param idempotencyToken
* A customer-chosen string that you can use to distinguish between otherwise identical calls to
* StartRestoreJob
. Retrying a successful request with the same idempotency token results in a
* success message with no action taken.
*/
public void setIdempotencyToken(String idempotencyToken) {
this.idempotencyToken = idempotencyToken;
}
/**
*
* A customer-chosen string that you can use to distinguish between otherwise identical calls to
* StartRestoreJob
. Retrying a successful request with the same idempotency token results in a success
* message with no action taken.
*
*
* @return A customer-chosen string that you can use to distinguish between otherwise identical calls to
* StartRestoreJob
. Retrying a successful request with the same idempotency token results in a
* success message with no action taken.
*/
public String getIdempotencyToken() {
return this.idempotencyToken;
}
/**
*
* A customer-chosen string that you can use to distinguish between otherwise identical calls to
* StartRestoreJob
. Retrying a successful request with the same idempotency token results in a success
* message with no action taken.
*
*
* @param idempotencyToken
* A customer-chosen string that you can use to distinguish between otherwise identical calls to
* StartRestoreJob
. Retrying a successful request with the same idempotency token results in a
* success message with no action taken.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartRestoreJobRequest withIdempotencyToken(String idempotencyToken) {
setIdempotencyToken(idempotencyToken);
return this;
}
/**
*
* Starts a job to restore a recovery point for one of the following resources:
*
*
* -
*
* Aurora
for Amazon Aurora
*
*
* -
*
* DocumentDB
for Amazon DocumentDB (with MongoDB compatibility)
*
*
* -
*
* DynamoDB
for Amazon DynamoDB
*
*
* -
*
* EBS
for Amazon Elastic Block Store
*
*
* -
*
* EC2
for Amazon Elastic Compute Cloud
*
*
* -
*
* EFS
for Amazon Elastic File System
*
*
* -
*
* FSx
for Amazon FSx
*
*
* -
*
* Neptune
for Amazon Neptune
*
*
* -
*
* RDS
for Amazon Relational Database Service
*
*
* -
*
* Storage Gateway
for Storage Gateway
*
*
* -
*
* S3
for Amazon S3
*
*
* -
*
* VirtualMachine
for virtual machines
*
*
*
*
* @param resourceType
* Starts a job to restore a recovery point for one of the following resources:
*
* -
*
* Aurora
for Amazon Aurora
*
*
* -
*
* DocumentDB
for Amazon DocumentDB (with MongoDB compatibility)
*
*
* -
*
* DynamoDB
for Amazon DynamoDB
*
*
* -
*
* EBS
for Amazon Elastic Block Store
*
*
* -
*
* EC2
for Amazon Elastic Compute Cloud
*
*
* -
*
* EFS
for Amazon Elastic File System
*
*
* -
*
* FSx
for Amazon FSx
*
*
* -
*
* Neptune
for Amazon Neptune
*
*
* -
*
* RDS
for Amazon Relational Database Service
*
*
* -
*
* Storage Gateway
for Storage Gateway
*
*
* -
*
* S3
for Amazon S3
*
*
* -
*
* VirtualMachine
for virtual machines
*
*
*/
public void setResourceType(String resourceType) {
this.resourceType = resourceType;
}
/**
*
* Starts a job to restore a recovery point for one of the following resources:
*
*
* -
*
* Aurora
for Amazon Aurora
*
*
* -
*
* DocumentDB
for Amazon DocumentDB (with MongoDB compatibility)
*
*
* -
*
* DynamoDB
for Amazon DynamoDB
*
*
* -
*
* EBS
for Amazon Elastic Block Store
*
*
* -
*
* EC2
for Amazon Elastic Compute Cloud
*
*
* -
*
* EFS
for Amazon Elastic File System
*
*
* -
*
* FSx
for Amazon FSx
*
*
* -
*
* Neptune
for Amazon Neptune
*
*
* -
*
* RDS
for Amazon Relational Database Service
*
*
* -
*
* Storage Gateway
for Storage Gateway
*
*
* -
*
* S3
for Amazon S3
*
*
* -
*
* VirtualMachine
for virtual machines
*
*
*
*
* @return Starts a job to restore a recovery point for one of the following resources:
*
* -
*
* Aurora
for Amazon Aurora
*
*
* -
*
* DocumentDB
for Amazon DocumentDB (with MongoDB compatibility)
*
*
* -
*
* DynamoDB
for Amazon DynamoDB
*
*
* -
*
* EBS
for Amazon Elastic Block Store
*
*
* -
*
* EC2
for Amazon Elastic Compute Cloud
*
*
* -
*
* EFS
for Amazon Elastic File System
*
*
* -
*
* FSx
for Amazon FSx
*
*
* -
*
* Neptune
for Amazon Neptune
*
*
* -
*
* RDS
for Amazon Relational Database Service
*
*
* -
*
* Storage Gateway
for Storage Gateway
*
*
* -
*
* S3
for Amazon S3
*
*
* -
*
* VirtualMachine
for virtual machines
*
*
*/
public String getResourceType() {
return this.resourceType;
}
/**
*
* Starts a job to restore a recovery point for one of the following resources:
*
*
* -
*
* Aurora
for Amazon Aurora
*
*
* -
*
* DocumentDB
for Amazon DocumentDB (with MongoDB compatibility)
*
*
* -
*
* DynamoDB
for Amazon DynamoDB
*
*
* -
*
* EBS
for Amazon Elastic Block Store
*
*
* -
*
* EC2
for Amazon Elastic Compute Cloud
*
*
* -
*
* EFS
for Amazon Elastic File System
*
*
* -
*
* FSx
for Amazon FSx
*
*
* -
*
* Neptune
for Amazon Neptune
*
*
* -
*
* RDS
for Amazon Relational Database Service
*
*
* -
*
* Storage Gateway
for Storage Gateway
*
*
* -
*
* S3
for Amazon S3
*
*
* -
*
* VirtualMachine
for virtual machines
*
*
*
*
* @param resourceType
* Starts a job to restore a recovery point for one of the following resources:
*
* -
*
* Aurora
for Amazon Aurora
*
*
* -
*
* DocumentDB
for Amazon DocumentDB (with MongoDB compatibility)
*
*
* -
*
* DynamoDB
for Amazon DynamoDB
*
*
* -
*
* EBS
for Amazon Elastic Block Store
*
*
* -
*
* EC2
for Amazon Elastic Compute Cloud
*
*
* -
*
* EFS
for Amazon Elastic File System
*
*
* -
*
* FSx
for Amazon FSx
*
*
* -
*
* Neptune
for Amazon Neptune
*
*
* -
*
* RDS
for Amazon Relational Database Service
*
*
* -
*
* Storage Gateway
for Storage Gateway
*
*
* -
*
* S3
for Amazon S3
*
*
* -
*
* VirtualMachine
for virtual machines
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartRestoreJobRequest withResourceType(String resourceType) {
setResourceType(resourceType);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getRecoveryPointArn() != null)
sb.append("RecoveryPointArn: ").append(getRecoveryPointArn()).append(",");
if (getMetadata() != null)
sb.append("Metadata: ").append("***Sensitive Data Redacted***").append(",");
if (getIamRoleArn() != null)
sb.append("IamRoleArn: ").append(getIamRoleArn()).append(",");
if (getIdempotencyToken() != null)
sb.append("IdempotencyToken: ").append(getIdempotencyToken()).append(",");
if (getResourceType() != null)
sb.append("ResourceType: ").append(getResourceType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StartRestoreJobRequest == false)
return false;
StartRestoreJobRequest other = (StartRestoreJobRequest) obj;
if (other.getRecoveryPointArn() == null ^ this.getRecoveryPointArn() == null)
return false;
if (other.getRecoveryPointArn() != null && other.getRecoveryPointArn().equals(this.getRecoveryPointArn()) == false)
return false;
if (other.getMetadata() == null ^ this.getMetadata() == null)
return false;
if (other.getMetadata() != null && other.getMetadata().equals(this.getMetadata()) == false)
return false;
if (other.getIamRoleArn() == null ^ this.getIamRoleArn() == null)
return false;
if (other.getIamRoleArn() != null && other.getIamRoleArn().equals(this.getIamRoleArn()) == false)
return false;
if (other.getIdempotencyToken() == null ^ this.getIdempotencyToken() == null)
return false;
if (other.getIdempotencyToken() != null && other.getIdempotencyToken().equals(this.getIdempotencyToken()) == false)
return false;
if (other.getResourceType() == null ^ this.getResourceType() == null)
return false;
if (other.getResourceType() != null && other.getResourceType().equals(this.getResourceType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getRecoveryPointArn() == null) ? 0 : getRecoveryPointArn().hashCode());
hashCode = prime * hashCode + ((getMetadata() == null) ? 0 : getMetadata().hashCode());
hashCode = prime * hashCode + ((getIamRoleArn() == null) ? 0 : getIamRoleArn().hashCode());
hashCode = prime * hashCode + ((getIdempotencyToken() == null) ? 0 : getIdempotencyToken().hashCode());
hashCode = prime * hashCode + ((getResourceType() == null) ? 0 : getResourceType().hashCode());
return hashCode;
}
@Override
public StartRestoreJobRequest clone() {
return (StartRestoreJobRequest) super.clone();
}
}