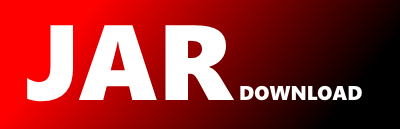
com.amazonaws.services.backup.AWSBackupAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-backup Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.backup;
import javax.annotation.Generated;
import com.amazonaws.services.backup.model.*;
/**
* Interface for accessing AWS Backup asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.backup.AbstractAWSBackupAsync} instead.
*
*
* Backup
*
* Backup is a unified backup service designed to protect Amazon Web Services services and their associated data. Backup
* simplifies the creation, migration, restoration, and deletion of backups, while also providing reporting and
* auditing.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSBackupAsync extends AWSBackup {
/**
*
* This action removes the specified legal hold on a recovery point. This action can only be performed by a user
* with sufficient permissions.
*
*
* @param cancelLegalHoldRequest
* @return A Java Future containing the result of the CancelLegalHold operation returned by the service.
* @sample AWSBackupAsync.CancelLegalHold
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelLegalHoldAsync(CancelLegalHoldRequest cancelLegalHoldRequest);
/**
*
* This action removes the specified legal hold on a recovery point. This action can only be performed by a user
* with sufficient permissions.
*
*
* @param cancelLegalHoldRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelLegalHold operation returned by the service.
* @sample AWSBackupAsyncHandler.CancelLegalHold
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelLegalHoldAsync(CancelLegalHoldRequest cancelLegalHoldRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a backup plan using a backup plan name and backup rules. A backup plan is a document that contains
* information that Backup uses to schedule tasks that create recovery points for resources.
*
*
* If you call CreateBackupPlan
with a plan that already exists, you receive an
* AlreadyExistsException
exception.
*
*
* @param createBackupPlanRequest
* @return A Java Future containing the result of the CreateBackupPlan operation returned by the service.
* @sample AWSBackupAsync.CreateBackupPlan
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createBackupPlanAsync(CreateBackupPlanRequest createBackupPlanRequest);
/**
*
* Creates a backup plan using a backup plan name and backup rules. A backup plan is a document that contains
* information that Backup uses to schedule tasks that create recovery points for resources.
*
*
* If you call CreateBackupPlan
with a plan that already exists, you receive an
* AlreadyExistsException
exception.
*
*
* @param createBackupPlanRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateBackupPlan operation returned by the service.
* @sample AWSBackupAsyncHandler.CreateBackupPlan
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createBackupPlanAsync(CreateBackupPlanRequest createBackupPlanRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a JSON document that specifies a set of resources to assign to a backup plan. For examples, see Assigning resources programmatically.
*
*
* @param createBackupSelectionRequest
* @return A Java Future containing the result of the CreateBackupSelection operation returned by the service.
* @sample AWSBackupAsync.CreateBackupSelection
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createBackupSelectionAsync(CreateBackupSelectionRequest createBackupSelectionRequest);
/**
*
* Creates a JSON document that specifies a set of resources to assign to a backup plan. For examples, see Assigning resources programmatically.
*
*
* @param createBackupSelectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateBackupSelection operation returned by the service.
* @sample AWSBackupAsyncHandler.CreateBackupSelection
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createBackupSelectionAsync(CreateBackupSelectionRequest createBackupSelectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a logical container where backups are stored. A CreateBackupVault
request includes a name,
* optionally one or more resource tags, an encryption key, and a request ID.
*
*
*
* Do not include sensitive data, such as passport numbers, in the name of a backup vault.
*
*
*
* @param createBackupVaultRequest
* @return A Java Future containing the result of the CreateBackupVault operation returned by the service.
* @sample AWSBackupAsync.CreateBackupVault
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createBackupVaultAsync(CreateBackupVaultRequest createBackupVaultRequest);
/**
*
* Creates a logical container where backups are stored. A CreateBackupVault
request includes a name,
* optionally one or more resource tags, an encryption key, and a request ID.
*
*
*
* Do not include sensitive data, such as passport numbers, in the name of a backup vault.
*
*
*
* @param createBackupVaultRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateBackupVault operation returned by the service.
* @sample AWSBackupAsyncHandler.CreateBackupVault
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createBackupVaultAsync(CreateBackupVaultRequest createBackupVaultRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a framework with one or more controls. A framework is a collection of controls that you can use to
* evaluate your backup practices. By using pre-built customizable controls to define your policies, you can
* evaluate whether your backup practices comply with your policies and which resources are not yet in compliance.
*
*
* @param createFrameworkRequest
* @return A Java Future containing the result of the CreateFramework operation returned by the service.
* @sample AWSBackupAsync.CreateFramework
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFrameworkAsync(CreateFrameworkRequest createFrameworkRequest);
/**
*
* Creates a framework with one or more controls. A framework is a collection of controls that you can use to
* evaluate your backup practices. By using pre-built customizable controls to define your policies, you can
* evaluate whether your backup practices comply with your policies and which resources are not yet in compliance.
*
*
* @param createFrameworkRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFramework operation returned by the service.
* @sample AWSBackupAsyncHandler.CreateFramework
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFrameworkAsync(CreateFrameworkRequest createFrameworkRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This action creates a legal hold on a recovery point (backup). A legal hold is a restraint on altering or
* deleting a backup until an authorized user cancels the legal hold. Any actions to delete or disassociate a
* recovery point will fail with an error if one or more active legal holds are on the recovery point.
*
*
* @param createLegalHoldRequest
* @return A Java Future containing the result of the CreateLegalHold operation returned by the service.
* @sample AWSBackupAsync.CreateLegalHold
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createLegalHoldAsync(CreateLegalHoldRequest createLegalHoldRequest);
/**
*
* This action creates a legal hold on a recovery point (backup). A legal hold is a restraint on altering or
* deleting a backup until an authorized user cancels the legal hold. Any actions to delete or disassociate a
* recovery point will fail with an error if one or more active legal holds are on the recovery point.
*
*
* @param createLegalHoldRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLegalHold operation returned by the service.
* @sample AWSBackupAsyncHandler.CreateLegalHold
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createLegalHoldAsync(CreateLegalHoldRequest createLegalHoldRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This request creates a logical container to where backups may be copied.
*
*
* This request includes a name, the Region, the maximum number of retention days, the minimum number of retention
* days, and optionally can include tags and a creator request ID.
*
*
*
* Do not include sensitive data, such as passport numbers, in the name of a backup vault.
*
*
*
* @param createLogicallyAirGappedBackupVaultRequest
* @return A Java Future containing the result of the CreateLogicallyAirGappedBackupVault operation returned by the
* service.
* @sample AWSBackupAsync.CreateLogicallyAirGappedBackupVault
* @see AWS API Documentation
*/
java.util.concurrent.Future createLogicallyAirGappedBackupVaultAsync(
CreateLogicallyAirGappedBackupVaultRequest createLogicallyAirGappedBackupVaultRequest);
/**
*
* This request creates a logical container to where backups may be copied.
*
*
* This request includes a name, the Region, the maximum number of retention days, the minimum number of retention
* days, and optionally can include tags and a creator request ID.
*
*
*
* Do not include sensitive data, such as passport numbers, in the name of a backup vault.
*
*
*
* @param createLogicallyAirGappedBackupVaultRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLogicallyAirGappedBackupVault operation returned by the
* service.
* @sample AWSBackupAsyncHandler.CreateLogicallyAirGappedBackupVault
* @see AWS API Documentation
*/
java.util.concurrent.Future createLogicallyAirGappedBackupVaultAsync(
CreateLogicallyAirGappedBackupVaultRequest createLogicallyAirGappedBackupVaultRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a report plan. A report plan is a document that contains information about the contents of the report and
* where Backup will deliver it.
*
*
* If you call CreateReportPlan
with a plan that already exists, you receive an
* AlreadyExistsException
exception.
*
*
* @param createReportPlanRequest
* @return A Java Future containing the result of the CreateReportPlan operation returned by the service.
* @sample AWSBackupAsync.CreateReportPlan
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createReportPlanAsync(CreateReportPlanRequest createReportPlanRequest);
/**
*
* Creates a report plan. A report plan is a document that contains information about the contents of the report and
* where Backup will deliver it.
*
*
* If you call CreateReportPlan
with a plan that already exists, you receive an
* AlreadyExistsException
exception.
*
*
* @param createReportPlanRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateReportPlan operation returned by the service.
* @sample AWSBackupAsyncHandler.CreateReportPlan
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createReportPlanAsync(CreateReportPlanRequest createReportPlanRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This is the first of two steps to create a restore testing plan; once this request is successful, finish the
* procedure with request CreateRestoreTestingSelection.
*
*
* You must include the parameter RestoreTestingPlan. You may optionally include CreatorRequestId and Tags.
*
*
* @param createRestoreTestingPlanRequest
* @return A Java Future containing the result of the CreateRestoreTestingPlan operation returned by the service.
* @sample AWSBackupAsync.CreateRestoreTestingPlan
* @see AWS API Documentation
*/
java.util.concurrent.Future createRestoreTestingPlanAsync(CreateRestoreTestingPlanRequest createRestoreTestingPlanRequest);
/**
*
* This is the first of two steps to create a restore testing plan; once this request is successful, finish the
* procedure with request CreateRestoreTestingSelection.
*
*
* You must include the parameter RestoreTestingPlan. You may optionally include CreatorRequestId and Tags.
*
*
* @param createRestoreTestingPlanRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRestoreTestingPlan operation returned by the service.
* @sample AWSBackupAsyncHandler.CreateRestoreTestingPlan
* @see AWS API Documentation
*/
java.util.concurrent.Future createRestoreTestingPlanAsync(CreateRestoreTestingPlanRequest createRestoreTestingPlanRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This request can be sent after CreateRestoreTestingPlan request returns successfully. This is the second part of
* creating a resource testing plan, and it must be completed sequentially.
*
*
* This consists of RestoreTestingSelectionName
, ProtectedResourceType
, and one of the
* following:
*
*
* -
*
* ProtectedResourceArns
*
*
* -
*
* ProtectedResourceConditions
*
*
*
*
* Each protected resource type can have one single value.
*
*
* A restore testing selection can include a wildcard value ("*") for ProtectedResourceArns
along with
* ProtectedResourceConditions
. Alternatively, you can include up to 30 specific protected resource
* ARNs in ProtectedResourceArns
.
*
*
* Cannot select by both protected resource types AND specific ARNs. Request will fail if both are included.
*
*
* @param createRestoreTestingSelectionRequest
* @return A Java Future containing the result of the CreateRestoreTestingSelection operation returned by the
* service.
* @sample AWSBackupAsync.CreateRestoreTestingSelection
* @see AWS API Documentation
*/
java.util.concurrent.Future createRestoreTestingSelectionAsync(
CreateRestoreTestingSelectionRequest createRestoreTestingSelectionRequest);
/**
*
* This request can be sent after CreateRestoreTestingPlan request returns successfully. This is the second part of
* creating a resource testing plan, and it must be completed sequentially.
*
*
* This consists of RestoreTestingSelectionName
, ProtectedResourceType
, and one of the
* following:
*
*
* -
*
* ProtectedResourceArns
*
*
* -
*
* ProtectedResourceConditions
*
*
*
*
* Each protected resource type can have one single value.
*
*
* A restore testing selection can include a wildcard value ("*") for ProtectedResourceArns
along with
* ProtectedResourceConditions
. Alternatively, you can include up to 30 specific protected resource
* ARNs in ProtectedResourceArns
.
*
*
* Cannot select by both protected resource types AND specific ARNs. Request will fail if both are included.
*
*
* @param createRestoreTestingSelectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRestoreTestingSelection operation returned by the
* service.
* @sample AWSBackupAsyncHandler.CreateRestoreTestingSelection
* @see AWS API Documentation
*/
java.util.concurrent.Future createRestoreTestingSelectionAsync(
CreateRestoreTestingSelectionRequest createRestoreTestingSelectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a backup plan. A backup plan can only be deleted after all associated selections of resources have been
* deleted. Deleting a backup plan deletes the current version of a backup plan. Previous versions, if any, will
* still exist.
*
*
* @param deleteBackupPlanRequest
* @return A Java Future containing the result of the DeleteBackupPlan operation returned by the service.
* @sample AWSBackupAsync.DeleteBackupPlan
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteBackupPlanAsync(DeleteBackupPlanRequest deleteBackupPlanRequest);
/**
*
* Deletes a backup plan. A backup plan can only be deleted after all associated selections of resources have been
* deleted. Deleting a backup plan deletes the current version of a backup plan. Previous versions, if any, will
* still exist.
*
*
* @param deleteBackupPlanRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteBackupPlan operation returned by the service.
* @sample AWSBackupAsyncHandler.DeleteBackupPlan
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteBackupPlanAsync(DeleteBackupPlanRequest deleteBackupPlanRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the resource selection associated with a backup plan that is specified by the SelectionId
.
*
*
* @param deleteBackupSelectionRequest
* @return A Java Future containing the result of the DeleteBackupSelection operation returned by the service.
* @sample AWSBackupAsync.DeleteBackupSelection
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteBackupSelectionAsync(DeleteBackupSelectionRequest deleteBackupSelectionRequest);
/**
*
* Deletes the resource selection associated with a backup plan that is specified by the SelectionId
.
*
*
* @param deleteBackupSelectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteBackupSelection operation returned by the service.
* @sample AWSBackupAsyncHandler.DeleteBackupSelection
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteBackupSelectionAsync(DeleteBackupSelectionRequest deleteBackupSelectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the backup vault identified by its name. A vault can be deleted only if it is empty.
*
*
* @param deleteBackupVaultRequest
* @return A Java Future containing the result of the DeleteBackupVault operation returned by the service.
* @sample AWSBackupAsync.DeleteBackupVault
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteBackupVaultAsync(DeleteBackupVaultRequest deleteBackupVaultRequest);
/**
*
* Deletes the backup vault identified by its name. A vault can be deleted only if it is empty.
*
*
* @param deleteBackupVaultRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteBackupVault operation returned by the service.
* @sample AWSBackupAsyncHandler.DeleteBackupVault
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteBackupVaultAsync(DeleteBackupVaultRequest deleteBackupVaultRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the policy document that manages permissions on a backup vault.
*
*
* @param deleteBackupVaultAccessPolicyRequest
* @return A Java Future containing the result of the DeleteBackupVaultAccessPolicy operation returned by the
* service.
* @sample AWSBackupAsync.DeleteBackupVaultAccessPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteBackupVaultAccessPolicyAsync(
DeleteBackupVaultAccessPolicyRequest deleteBackupVaultAccessPolicyRequest);
/**
*
* Deletes the policy document that manages permissions on a backup vault.
*
*
* @param deleteBackupVaultAccessPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteBackupVaultAccessPolicy operation returned by the
* service.
* @sample AWSBackupAsyncHandler.DeleteBackupVaultAccessPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteBackupVaultAccessPolicyAsync(
DeleteBackupVaultAccessPolicyRequest deleteBackupVaultAccessPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes Backup Vault Lock from a backup vault specified by a backup vault name.
*
*
* If the Vault Lock configuration is immutable, then you cannot delete Vault Lock using API operations, and you
* will receive an InvalidRequestException
if you attempt to do so. For more information, see Vault Lock in the Backup
* Developer Guide.
*
*
* @param deleteBackupVaultLockConfigurationRequest
* @return A Java Future containing the result of the DeleteBackupVaultLockConfiguration operation returned by the
* service.
* @sample AWSBackupAsync.DeleteBackupVaultLockConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteBackupVaultLockConfigurationAsync(
DeleteBackupVaultLockConfigurationRequest deleteBackupVaultLockConfigurationRequest);
/**
*
* Deletes Backup Vault Lock from a backup vault specified by a backup vault name.
*
*
* If the Vault Lock configuration is immutable, then you cannot delete Vault Lock using API operations, and you
* will receive an InvalidRequestException
if you attempt to do so. For more information, see Vault Lock in the Backup
* Developer Guide.
*
*
* @param deleteBackupVaultLockConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteBackupVaultLockConfiguration operation returned by the
* service.
* @sample AWSBackupAsyncHandler.DeleteBackupVaultLockConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteBackupVaultLockConfigurationAsync(
DeleteBackupVaultLockConfigurationRequest deleteBackupVaultLockConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes event notifications for the specified backup vault.
*
*
* @param deleteBackupVaultNotificationsRequest
* @return A Java Future containing the result of the DeleteBackupVaultNotifications operation returned by the
* service.
* @sample AWSBackupAsync.DeleteBackupVaultNotifications
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteBackupVaultNotificationsAsync(
DeleteBackupVaultNotificationsRequest deleteBackupVaultNotificationsRequest);
/**
*
* Deletes event notifications for the specified backup vault.
*
*
* @param deleteBackupVaultNotificationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteBackupVaultNotifications operation returned by the
* service.
* @sample AWSBackupAsyncHandler.DeleteBackupVaultNotifications
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteBackupVaultNotificationsAsync(
DeleteBackupVaultNotificationsRequest deleteBackupVaultNotificationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the framework specified by a framework name.
*
*
* @param deleteFrameworkRequest
* @return A Java Future containing the result of the DeleteFramework operation returned by the service.
* @sample AWSBackupAsync.DeleteFramework
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFrameworkAsync(DeleteFrameworkRequest deleteFrameworkRequest);
/**
*
* Deletes the framework specified by a framework name.
*
*
* @param deleteFrameworkRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFramework operation returned by the service.
* @sample AWSBackupAsyncHandler.DeleteFramework
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFrameworkAsync(DeleteFrameworkRequest deleteFrameworkRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the recovery point specified by a recovery point ID.
*
*
* If the recovery point ID belongs to a continuous backup, calling this endpoint deletes the existing continuous
* backup and stops future continuous backup.
*
*
* When an IAM role's permissions are insufficient to call this API, the service sends back an HTTP 200 response
* with an empty HTTP body, but the recovery point is not deleted. Instead, it enters an EXPIRED
state.
*
*
* EXPIRED
recovery points can be deleted with this API once the IAM role has the
* iam:CreateServiceLinkedRole
action. To learn more about adding this role, see
* Troubleshooting manual deletions.
*
*
* If the user or role is deleted or the permission within the role is removed, the deletion will not be successful
* and will enter an EXPIRED
state.
*
*
* @param deleteRecoveryPointRequest
* @return A Java Future containing the result of the DeleteRecoveryPoint operation returned by the service.
* @sample AWSBackupAsync.DeleteRecoveryPoint
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteRecoveryPointAsync(DeleteRecoveryPointRequest deleteRecoveryPointRequest);
/**
*
* Deletes the recovery point specified by a recovery point ID.
*
*
* If the recovery point ID belongs to a continuous backup, calling this endpoint deletes the existing continuous
* backup and stops future continuous backup.
*
*
* When an IAM role's permissions are insufficient to call this API, the service sends back an HTTP 200 response
* with an empty HTTP body, but the recovery point is not deleted. Instead, it enters an EXPIRED
state.
*
*
* EXPIRED
recovery points can be deleted with this API once the IAM role has the
* iam:CreateServiceLinkedRole
action. To learn more about adding this role, see
* Troubleshooting manual deletions.
*
*
* If the user or role is deleted or the permission within the role is removed, the deletion will not be successful
* and will enter an EXPIRED
state.
*
*
* @param deleteRecoveryPointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRecoveryPoint operation returned by the service.
* @sample AWSBackupAsyncHandler.DeleteRecoveryPoint
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteRecoveryPointAsync(DeleteRecoveryPointRequest deleteRecoveryPointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the report plan specified by a report plan name.
*
*
* @param deleteReportPlanRequest
* @return A Java Future containing the result of the DeleteReportPlan operation returned by the service.
* @sample AWSBackupAsync.DeleteReportPlan
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteReportPlanAsync(DeleteReportPlanRequest deleteReportPlanRequest);
/**
*
* Deletes the report plan specified by a report plan name.
*
*
* @param deleteReportPlanRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteReportPlan operation returned by the service.
* @sample AWSBackupAsyncHandler.DeleteReportPlan
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteReportPlanAsync(DeleteReportPlanRequest deleteReportPlanRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This request deletes the specified restore testing plan.
*
*
* Deletion can only successfully occur if all associated restore testing selections are deleted first.
*
*
* @param deleteRestoreTestingPlanRequest
* @return A Java Future containing the result of the DeleteRestoreTestingPlan operation returned by the service.
* @sample AWSBackupAsync.DeleteRestoreTestingPlan
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRestoreTestingPlanAsync(DeleteRestoreTestingPlanRequest deleteRestoreTestingPlanRequest);
/**
*
* This request deletes the specified restore testing plan.
*
*
* Deletion can only successfully occur if all associated restore testing selections are deleted first.
*
*
* @param deleteRestoreTestingPlanRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRestoreTestingPlan operation returned by the service.
* @sample AWSBackupAsyncHandler.DeleteRestoreTestingPlan
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRestoreTestingPlanAsync(DeleteRestoreTestingPlanRequest deleteRestoreTestingPlanRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Input the Restore Testing Plan name and Restore Testing Selection name.
*
*
* All testing selections associated with a restore testing plan must be deleted before the restore testing plan can
* be deleted.
*
*
* @param deleteRestoreTestingSelectionRequest
* @return A Java Future containing the result of the DeleteRestoreTestingSelection operation returned by the
* service.
* @sample AWSBackupAsync.DeleteRestoreTestingSelection
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRestoreTestingSelectionAsync(
DeleteRestoreTestingSelectionRequest deleteRestoreTestingSelectionRequest);
/**
*
* Input the Restore Testing Plan name and Restore Testing Selection name.
*
*
* All testing selections associated with a restore testing plan must be deleted before the restore testing plan can
* be deleted.
*
*
* @param deleteRestoreTestingSelectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRestoreTestingSelection operation returned by the
* service.
* @sample AWSBackupAsyncHandler.DeleteRestoreTestingSelection
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRestoreTestingSelectionAsync(
DeleteRestoreTestingSelectionRequest deleteRestoreTestingSelectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns backup job details for the specified BackupJobId
.
*
*
* @param describeBackupJobRequest
* @return A Java Future containing the result of the DescribeBackupJob operation returned by the service.
* @sample AWSBackupAsync.DescribeBackupJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeBackupJobAsync(DescribeBackupJobRequest describeBackupJobRequest);
/**
*
* Returns backup job details for the specified BackupJobId
.
*
*
* @param describeBackupJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeBackupJob operation returned by the service.
* @sample AWSBackupAsyncHandler.DescribeBackupJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeBackupJobAsync(DescribeBackupJobRequest describeBackupJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns metadata about a backup vault specified by its name.
*
*
* @param describeBackupVaultRequest
* @return A Java Future containing the result of the DescribeBackupVault operation returned by the service.
* @sample AWSBackupAsync.DescribeBackupVault
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeBackupVaultAsync(DescribeBackupVaultRequest describeBackupVaultRequest);
/**
*
* Returns metadata about a backup vault specified by its name.
*
*
* @param describeBackupVaultRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeBackupVault operation returned by the service.
* @sample AWSBackupAsyncHandler.DescribeBackupVault
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeBackupVaultAsync(DescribeBackupVaultRequest describeBackupVaultRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns metadata associated with creating a copy of a resource.
*
*
* @param describeCopyJobRequest
* @return A Java Future containing the result of the DescribeCopyJob operation returned by the service.
* @sample AWSBackupAsync.DescribeCopyJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeCopyJobAsync(DescribeCopyJobRequest describeCopyJobRequest);
/**
*
* Returns metadata associated with creating a copy of a resource.
*
*
* @param describeCopyJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeCopyJob operation returned by the service.
* @sample AWSBackupAsyncHandler.DescribeCopyJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeCopyJobAsync(DescribeCopyJobRequest describeCopyJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the framework details for the specified FrameworkName
.
*
*
* @param describeFrameworkRequest
* @return A Java Future containing the result of the DescribeFramework operation returned by the service.
* @sample AWSBackupAsync.DescribeFramework
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeFrameworkAsync(DescribeFrameworkRequest describeFrameworkRequest);
/**
*
* Returns the framework details for the specified FrameworkName
.
*
*
* @param describeFrameworkRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFramework operation returned by the service.
* @sample AWSBackupAsyncHandler.DescribeFramework
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeFrameworkAsync(DescribeFrameworkRequest describeFrameworkRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes whether the Amazon Web Services account is opted in to cross-account backup. Returns an error if the
* account is not a member of an Organizations organization. Example:
* describe-global-settings --region us-west-2
*
*
* @param describeGlobalSettingsRequest
* @return A Java Future containing the result of the DescribeGlobalSettings operation returned by the service.
* @sample AWSBackupAsync.DescribeGlobalSettings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeGlobalSettingsAsync(DescribeGlobalSettingsRequest describeGlobalSettingsRequest);
/**
*
* Describes whether the Amazon Web Services account is opted in to cross-account backup. Returns an error if the
* account is not a member of an Organizations organization. Example:
* describe-global-settings --region us-west-2
*
*
* @param describeGlobalSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeGlobalSettings operation returned by the service.
* @sample AWSBackupAsyncHandler.DescribeGlobalSettings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeGlobalSettingsAsync(DescribeGlobalSettingsRequest describeGlobalSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a saved resource, including the last time it was backed up, its Amazon Resource Name
* (ARN), and the Amazon Web Services service type of the saved resource.
*
*
* @param describeProtectedResourceRequest
* @return A Java Future containing the result of the DescribeProtectedResource operation returned by the service.
* @sample AWSBackupAsync.DescribeProtectedResource
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProtectedResourceAsync(
DescribeProtectedResourceRequest describeProtectedResourceRequest);
/**
*
* Returns information about a saved resource, including the last time it was backed up, its Amazon Resource Name
* (ARN), and the Amazon Web Services service type of the saved resource.
*
*
* @param describeProtectedResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeProtectedResource operation returned by the service.
* @sample AWSBackupAsyncHandler.DescribeProtectedResource
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProtectedResourceAsync(
DescribeProtectedResourceRequest describeProtectedResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns metadata associated with a recovery point, including ID, status, encryption, and lifecycle.
*
*
* @param describeRecoveryPointRequest
* @return A Java Future containing the result of the DescribeRecoveryPoint operation returned by the service.
* @sample AWSBackupAsync.DescribeRecoveryPoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeRecoveryPointAsync(DescribeRecoveryPointRequest describeRecoveryPointRequest);
/**
*
* Returns metadata associated with a recovery point, including ID, status, encryption, and lifecycle.
*
*
* @param describeRecoveryPointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRecoveryPoint operation returned by the service.
* @sample AWSBackupAsyncHandler.DescribeRecoveryPoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeRecoveryPointAsync(DescribeRecoveryPointRequest describeRecoveryPointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the current service opt-in settings for the Region. If service opt-in is enabled for a service, Backup
* tries to protect that service's resources in this Region, when the resource is included in an on-demand backup or
* scheduled backup plan. Otherwise, Backup does not try to protect that service's resources in this Region.
*
*
* @param describeRegionSettingsRequest
* @return A Java Future containing the result of the DescribeRegionSettings operation returned by the service.
* @sample AWSBackupAsync.DescribeRegionSettings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeRegionSettingsAsync(DescribeRegionSettingsRequest describeRegionSettingsRequest);
/**
*
* Returns the current service opt-in settings for the Region. If service opt-in is enabled for a service, Backup
* tries to protect that service's resources in this Region, when the resource is included in an on-demand backup or
* scheduled backup plan. Otherwise, Backup does not try to protect that service's resources in this Region.
*
*
* @param describeRegionSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRegionSettings operation returned by the service.
* @sample AWSBackupAsyncHandler.DescribeRegionSettings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeRegionSettingsAsync(DescribeRegionSettingsRequest describeRegionSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the details associated with creating a report as specified by its ReportJobId
.
*
*
* @param describeReportJobRequest
* @return A Java Future containing the result of the DescribeReportJob operation returned by the service.
* @sample AWSBackupAsync.DescribeReportJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeReportJobAsync(DescribeReportJobRequest describeReportJobRequest);
/**
*
* Returns the details associated with creating a report as specified by its ReportJobId
.
*
*
* @param describeReportJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReportJob operation returned by the service.
* @sample AWSBackupAsyncHandler.DescribeReportJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeReportJobAsync(DescribeReportJobRequest describeReportJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all report plans for an Amazon Web Services account and Amazon Web Services Region.
*
*
* @param describeReportPlanRequest
* @return A Java Future containing the result of the DescribeReportPlan operation returned by the service.
* @sample AWSBackupAsync.DescribeReportPlan
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeReportPlanAsync(DescribeReportPlanRequest describeReportPlanRequest);
/**
*
* Returns a list of all report plans for an Amazon Web Services account and Amazon Web Services Region.
*
*
* @param describeReportPlanRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReportPlan operation returned by the service.
* @sample AWSBackupAsyncHandler.DescribeReportPlan
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeReportPlanAsync(DescribeReportPlanRequest describeReportPlanRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns metadata associated with a restore job that is specified by a job ID.
*
*
* @param describeRestoreJobRequest
* @return A Java Future containing the result of the DescribeRestoreJob operation returned by the service.
* @sample AWSBackupAsync.DescribeRestoreJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeRestoreJobAsync(DescribeRestoreJobRequest describeRestoreJobRequest);
/**
*
* Returns metadata associated with a restore job that is specified by a job ID.
*
*
* @param describeRestoreJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRestoreJob operation returned by the service.
* @sample AWSBackupAsyncHandler.DescribeRestoreJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeRestoreJobAsync(DescribeRestoreJobRequest describeRestoreJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified continuous backup recovery point from Backup and releases control of that continuous backup
* to the source service, such as Amazon RDS. The source service will continue to create and retain continuous
* backups using the lifecycle that you specified in your original backup plan.
*
*
* Does not support snapshot backup recovery points.
*
*
* @param disassociateRecoveryPointRequest
* @return A Java Future containing the result of the DisassociateRecoveryPoint operation returned by the service.
* @sample AWSBackupAsync.DisassociateRecoveryPoint
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateRecoveryPointAsync(
DisassociateRecoveryPointRequest disassociateRecoveryPointRequest);
/**
*
* Deletes the specified continuous backup recovery point from Backup and releases control of that continuous backup
* to the source service, such as Amazon RDS. The source service will continue to create and retain continuous
* backups using the lifecycle that you specified in your original backup plan.
*
*
* Does not support snapshot backup recovery points.
*
*
* @param disassociateRecoveryPointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateRecoveryPoint operation returned by the service.
* @sample AWSBackupAsyncHandler.DisassociateRecoveryPoint
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateRecoveryPointAsync(
DisassociateRecoveryPointRequest disassociateRecoveryPointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This action to a specific child (nested) recovery point removes the relationship between the specified recovery
* point and its parent (composite) recovery point.
*
*
* @param disassociateRecoveryPointFromParentRequest
* @return A Java Future containing the result of the DisassociateRecoveryPointFromParent operation returned by the
* service.
* @sample AWSBackupAsync.DisassociateRecoveryPointFromParent
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateRecoveryPointFromParentAsync(
DisassociateRecoveryPointFromParentRequest disassociateRecoveryPointFromParentRequest);
/**
*
* This action to a specific child (nested) recovery point removes the relationship between the specified recovery
* point and its parent (composite) recovery point.
*
*
* @param disassociateRecoveryPointFromParentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateRecoveryPointFromParent operation returned by the
* service.
* @sample AWSBackupAsyncHandler.DisassociateRecoveryPointFromParent
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateRecoveryPointFromParentAsync(
DisassociateRecoveryPointFromParentRequest disassociateRecoveryPointFromParentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the backup plan that is specified by the plan ID as a backup template.
*
*
* @param exportBackupPlanTemplateRequest
* @return A Java Future containing the result of the ExportBackupPlanTemplate operation returned by the service.
* @sample AWSBackupAsync.ExportBackupPlanTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future exportBackupPlanTemplateAsync(ExportBackupPlanTemplateRequest exportBackupPlanTemplateRequest);
/**
*
* Returns the backup plan that is specified by the plan ID as a backup template.
*
*
* @param exportBackupPlanTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ExportBackupPlanTemplate operation returned by the service.
* @sample AWSBackupAsyncHandler.ExportBackupPlanTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future exportBackupPlanTemplateAsync(ExportBackupPlanTemplateRequest exportBackupPlanTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns BackupPlan
details for the specified BackupPlanId
. The details are the body of
* a backup plan in JSON format, in addition to plan metadata.
*
*
* @param getBackupPlanRequest
* @return A Java Future containing the result of the GetBackupPlan operation returned by the service.
* @sample AWSBackupAsync.GetBackupPlan
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBackupPlanAsync(GetBackupPlanRequest getBackupPlanRequest);
/**
*
* Returns BackupPlan
details for the specified BackupPlanId
. The details are the body of
* a backup plan in JSON format, in addition to plan metadata.
*
*
* @param getBackupPlanRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBackupPlan operation returned by the service.
* @sample AWSBackupAsyncHandler.GetBackupPlan
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBackupPlanAsync(GetBackupPlanRequest getBackupPlanRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a valid JSON document specifying a backup plan or an error.
*
*
* @param getBackupPlanFromJSONRequest
* @return A Java Future containing the result of the GetBackupPlanFromJSON operation returned by the service.
* @sample AWSBackupAsync.GetBackupPlanFromJSON
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getBackupPlanFromJSONAsync(GetBackupPlanFromJSONRequest getBackupPlanFromJSONRequest);
/**
*
* Returns a valid JSON document specifying a backup plan or an error.
*
*
* @param getBackupPlanFromJSONRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBackupPlanFromJSON operation returned by the service.
* @sample AWSBackupAsyncHandler.GetBackupPlanFromJSON
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getBackupPlanFromJSONAsync(GetBackupPlanFromJSONRequest getBackupPlanFromJSONRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the template specified by its templateId
as a backup plan.
*
*
* @param getBackupPlanFromTemplateRequest
* @return A Java Future containing the result of the GetBackupPlanFromTemplate operation returned by the service.
* @sample AWSBackupAsync.GetBackupPlanFromTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future getBackupPlanFromTemplateAsync(
GetBackupPlanFromTemplateRequest getBackupPlanFromTemplateRequest);
/**
*
* Returns the template specified by its templateId
as a backup plan.
*
*
* @param getBackupPlanFromTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBackupPlanFromTemplate operation returned by the service.
* @sample AWSBackupAsyncHandler.GetBackupPlanFromTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future getBackupPlanFromTemplateAsync(
GetBackupPlanFromTemplateRequest getBackupPlanFromTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns selection metadata and a document in JSON format that specifies a list of resources that are associated
* with a backup plan.
*
*
* @param getBackupSelectionRequest
* @return A Java Future containing the result of the GetBackupSelection operation returned by the service.
* @sample AWSBackupAsync.GetBackupSelection
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBackupSelectionAsync(GetBackupSelectionRequest getBackupSelectionRequest);
/**
*
* Returns selection metadata and a document in JSON format that specifies a list of resources that are associated
* with a backup plan.
*
*
* @param getBackupSelectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBackupSelection operation returned by the service.
* @sample AWSBackupAsyncHandler.GetBackupSelection
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBackupSelectionAsync(GetBackupSelectionRequest getBackupSelectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the access policy document that is associated with the named backup vault.
*
*
* @param getBackupVaultAccessPolicyRequest
* @return A Java Future containing the result of the GetBackupVaultAccessPolicy operation returned by the service.
* @sample AWSBackupAsync.GetBackupVaultAccessPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future getBackupVaultAccessPolicyAsync(
GetBackupVaultAccessPolicyRequest getBackupVaultAccessPolicyRequest);
/**
*
* Returns the access policy document that is associated with the named backup vault.
*
*
* @param getBackupVaultAccessPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBackupVaultAccessPolicy operation returned by the service.
* @sample AWSBackupAsyncHandler.GetBackupVaultAccessPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future getBackupVaultAccessPolicyAsync(
GetBackupVaultAccessPolicyRequest getBackupVaultAccessPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns event notifications for the specified backup vault.
*
*
* @param getBackupVaultNotificationsRequest
* @return A Java Future containing the result of the GetBackupVaultNotifications operation returned by the service.
* @sample AWSBackupAsync.GetBackupVaultNotifications
* @see AWS API Documentation
*/
java.util.concurrent.Future getBackupVaultNotificationsAsync(
GetBackupVaultNotificationsRequest getBackupVaultNotificationsRequest);
/**
*
* Returns event notifications for the specified backup vault.
*
*
* @param getBackupVaultNotificationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBackupVaultNotifications operation returned by the service.
* @sample AWSBackupAsyncHandler.GetBackupVaultNotifications
* @see AWS API Documentation
*/
java.util.concurrent.Future getBackupVaultNotificationsAsync(
GetBackupVaultNotificationsRequest getBackupVaultNotificationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This action returns details for a specified legal hold. The details are the body of a legal hold in JSON format,
* in addition to metadata.
*
*
* @param getLegalHoldRequest
* @return A Java Future containing the result of the GetLegalHold operation returned by the service.
* @sample AWSBackupAsync.GetLegalHold
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getLegalHoldAsync(GetLegalHoldRequest getLegalHoldRequest);
/**
*
* This action returns details for a specified legal hold. The details are the body of a legal hold in JSON format,
* in addition to metadata.
*
*
* @param getLegalHoldRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetLegalHold operation returned by the service.
* @sample AWSBackupAsyncHandler.GetLegalHold
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getLegalHoldAsync(GetLegalHoldRequest getLegalHoldRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a set of metadata key-value pairs that were used to create the backup.
*
*
* @param getRecoveryPointRestoreMetadataRequest
* @return A Java Future containing the result of the GetRecoveryPointRestoreMetadata operation returned by the
* service.
* @sample AWSBackupAsync.GetRecoveryPointRestoreMetadata
* @see AWS API Documentation
*/
java.util.concurrent.Future getRecoveryPointRestoreMetadataAsync(
GetRecoveryPointRestoreMetadataRequest getRecoveryPointRestoreMetadataRequest);
/**
*
* Returns a set of metadata key-value pairs that were used to create the backup.
*
*
* @param getRecoveryPointRestoreMetadataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetRecoveryPointRestoreMetadata operation returned by the
* service.
* @sample AWSBackupAsyncHandler.GetRecoveryPointRestoreMetadata
* @see AWS API Documentation
*/
java.util.concurrent.Future getRecoveryPointRestoreMetadataAsync(
GetRecoveryPointRestoreMetadataRequest getRecoveryPointRestoreMetadataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This request returns the metadata for the specified restore job.
*
*
* @param getRestoreJobMetadataRequest
* @return A Java Future containing the result of the GetRestoreJobMetadata operation returned by the service.
* @sample AWSBackupAsync.GetRestoreJobMetadata
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getRestoreJobMetadataAsync(GetRestoreJobMetadataRequest getRestoreJobMetadataRequest);
/**
*
* This request returns the metadata for the specified restore job.
*
*
* @param getRestoreJobMetadataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetRestoreJobMetadata operation returned by the service.
* @sample AWSBackupAsyncHandler.GetRestoreJobMetadata
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getRestoreJobMetadataAsync(GetRestoreJobMetadataRequest getRestoreJobMetadataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This request returns the minimal required set of metadata needed to start a restore job with secure default
* settings. BackupVaultName
and RecoveryPointArn
are required parameters.
* BackupVaultAccountId
is an optional parameter.
*
*
* @param getRestoreTestingInferredMetadataRequest
* @return A Java Future containing the result of the GetRestoreTestingInferredMetadata operation returned by the
* service.
* @sample AWSBackupAsync.GetRestoreTestingInferredMetadata
* @see AWS API Documentation
*/
java.util.concurrent.Future getRestoreTestingInferredMetadataAsync(
GetRestoreTestingInferredMetadataRequest getRestoreTestingInferredMetadataRequest);
/**
*
* This request returns the minimal required set of metadata needed to start a restore job with secure default
* settings. BackupVaultName
and RecoveryPointArn
are required parameters.
* BackupVaultAccountId
is an optional parameter.
*
*
* @param getRestoreTestingInferredMetadataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetRestoreTestingInferredMetadata operation returned by the
* service.
* @sample AWSBackupAsyncHandler.GetRestoreTestingInferredMetadata
* @see AWS API Documentation
*/
java.util.concurrent.Future getRestoreTestingInferredMetadataAsync(
GetRestoreTestingInferredMetadataRequest getRestoreTestingInferredMetadataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns RestoreTestingPlan
details for the specified RestoreTestingPlanName
. The
* details are the body of a restore testing plan in JSON format, in addition to plan metadata.
*
*
* @param getRestoreTestingPlanRequest
* @return A Java Future containing the result of the GetRestoreTestingPlan operation returned by the service.
* @sample AWSBackupAsync.GetRestoreTestingPlan
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getRestoreTestingPlanAsync(GetRestoreTestingPlanRequest getRestoreTestingPlanRequest);
/**
*
* Returns RestoreTestingPlan
details for the specified RestoreTestingPlanName
. The
* details are the body of a restore testing plan in JSON format, in addition to plan metadata.
*
*
* @param getRestoreTestingPlanRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetRestoreTestingPlan operation returned by the service.
* @sample AWSBackupAsyncHandler.GetRestoreTestingPlan
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getRestoreTestingPlanAsync(GetRestoreTestingPlanRequest getRestoreTestingPlanRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns RestoreTestingSelection, which displays resources and elements of the restore testing plan.
*
*
* @param getRestoreTestingSelectionRequest
* @return A Java Future containing the result of the GetRestoreTestingSelection operation returned by the service.
* @sample AWSBackupAsync.GetRestoreTestingSelection
* @see AWS API Documentation
*/
java.util.concurrent.Future getRestoreTestingSelectionAsync(
GetRestoreTestingSelectionRequest getRestoreTestingSelectionRequest);
/**
*
* Returns RestoreTestingSelection, which displays resources and elements of the restore testing plan.
*
*
* @param getRestoreTestingSelectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetRestoreTestingSelection operation returned by the service.
* @sample AWSBackupAsyncHandler.GetRestoreTestingSelection
* @see AWS API Documentation
*/
java.util.concurrent.Future getRestoreTestingSelectionAsync(
GetRestoreTestingSelectionRequest getRestoreTestingSelectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the Amazon Web Services resource types supported by Backup.
*
*
* @param getSupportedResourceTypesRequest
* @return A Java Future containing the result of the GetSupportedResourceTypes operation returned by the service.
* @sample AWSBackupAsync.GetSupportedResourceTypes
* @see AWS API Documentation
*/
java.util.concurrent.Future getSupportedResourceTypesAsync(
GetSupportedResourceTypesRequest getSupportedResourceTypesRequest);
/**
*
* Returns the Amazon Web Services resource types supported by Backup.
*
*
* @param getSupportedResourceTypesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSupportedResourceTypes operation returned by the service.
* @sample AWSBackupAsyncHandler.GetSupportedResourceTypes
* @see AWS API Documentation
*/
java.util.concurrent.Future getSupportedResourceTypesAsync(
GetSupportedResourceTypesRequest getSupportedResourceTypesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This is a request for a summary of backup jobs created or running within the most recent 30 days. You can include
* parameters AccountID, State, ResourceType, MessageCategory, AggregationPeriod, MaxResults, or NextToken to filter
* results.
*
*
* This request returns a summary that contains Region, Account, State, ResourceType, MessageCategory, StartTime,
* EndTime, and Count of included jobs.
*
*
* @param listBackupJobSummariesRequest
* @return A Java Future containing the result of the ListBackupJobSummaries operation returned by the service.
* @sample AWSBackupAsync.ListBackupJobSummaries
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listBackupJobSummariesAsync(ListBackupJobSummariesRequest listBackupJobSummariesRequest);
/**
*
* This is a request for a summary of backup jobs created or running within the most recent 30 days. You can include
* parameters AccountID, State, ResourceType, MessageCategory, AggregationPeriod, MaxResults, or NextToken to filter
* results.
*
*
* This request returns a summary that contains Region, Account, State, ResourceType, MessageCategory, StartTime,
* EndTime, and Count of included jobs.
*
*
* @param listBackupJobSummariesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListBackupJobSummaries operation returned by the service.
* @sample AWSBackupAsyncHandler.ListBackupJobSummaries
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listBackupJobSummariesAsync(ListBackupJobSummariesRequest listBackupJobSummariesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of existing backup jobs for an authenticated account for the last 30 days. For a longer period of
* time, consider using these monitoring tools.
*
*
* @param listBackupJobsRequest
* @return A Java Future containing the result of the ListBackupJobs operation returned by the service.
* @sample AWSBackupAsync.ListBackupJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listBackupJobsAsync(ListBackupJobsRequest listBackupJobsRequest);
/**
*
* Returns a list of existing backup jobs for an authenticated account for the last 30 days. For a longer period of
* time, consider using these monitoring tools.
*
*
* @param listBackupJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListBackupJobs operation returned by the service.
* @sample AWSBackupAsyncHandler.ListBackupJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listBackupJobsAsync(ListBackupJobsRequest listBackupJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns metadata of your saved backup plan templates, including the template ID, name, and the creation and
* deletion dates.
*
*
* @param listBackupPlanTemplatesRequest
* @return A Java Future containing the result of the ListBackupPlanTemplates operation returned by the service.
* @sample AWSBackupAsync.ListBackupPlanTemplates
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listBackupPlanTemplatesAsync(ListBackupPlanTemplatesRequest listBackupPlanTemplatesRequest);
/**
*
* Returns metadata of your saved backup plan templates, including the template ID, name, and the creation and
* deletion dates.
*
*
* @param listBackupPlanTemplatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListBackupPlanTemplates operation returned by the service.
* @sample AWSBackupAsyncHandler.ListBackupPlanTemplates
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listBackupPlanTemplatesAsync(ListBackupPlanTemplatesRequest listBackupPlanTemplatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns version metadata of your backup plans, including Amazon Resource Names (ARNs), backup plan IDs, creation
* and deletion dates, plan names, and version IDs.
*
*
* @param listBackupPlanVersionsRequest
* @return A Java Future containing the result of the ListBackupPlanVersions operation returned by the service.
* @sample AWSBackupAsync.ListBackupPlanVersions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listBackupPlanVersionsAsync(ListBackupPlanVersionsRequest listBackupPlanVersionsRequest);
/**
*
* Returns version metadata of your backup plans, including Amazon Resource Names (ARNs), backup plan IDs, creation
* and deletion dates, plan names, and version IDs.
*
*
* @param listBackupPlanVersionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListBackupPlanVersions operation returned by the service.
* @sample AWSBackupAsyncHandler.ListBackupPlanVersions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listBackupPlanVersionsAsync(ListBackupPlanVersionsRequest listBackupPlanVersionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all active backup plans for an authenticated account. The list contains information such as
* Amazon Resource Names (ARNs), plan IDs, creation and deletion dates, version IDs, plan names, and creator request
* IDs.
*
*
* @param listBackupPlansRequest
* @return A Java Future containing the result of the ListBackupPlans operation returned by the service.
* @sample AWSBackupAsync.ListBackupPlans
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listBackupPlansAsync(ListBackupPlansRequest listBackupPlansRequest);
/**
*
* Returns a list of all active backup plans for an authenticated account. The list contains information such as
* Amazon Resource Names (ARNs), plan IDs, creation and deletion dates, version IDs, plan names, and creator request
* IDs.
*
*
* @param listBackupPlansRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListBackupPlans operation returned by the service.
* @sample AWSBackupAsyncHandler.ListBackupPlans
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listBackupPlansAsync(ListBackupPlansRequest listBackupPlansRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an array containing metadata of the resources associated with the target backup plan.
*
*
* @param listBackupSelectionsRequest
* @return A Java Future containing the result of the ListBackupSelections operation returned by the service.
* @sample AWSBackupAsync.ListBackupSelections
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listBackupSelectionsAsync(ListBackupSelectionsRequest listBackupSelectionsRequest);
/**
*
* Returns an array containing metadata of the resources associated with the target backup plan.
*
*
* @param listBackupSelectionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListBackupSelections operation returned by the service.
* @sample AWSBackupAsyncHandler.ListBackupSelections
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listBackupSelectionsAsync(ListBackupSelectionsRequest listBackupSelectionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of recovery point storage containers along with information about them.
*
*
* @param listBackupVaultsRequest
* @return A Java Future containing the result of the ListBackupVaults operation returned by the service.
* @sample AWSBackupAsync.ListBackupVaults
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listBackupVaultsAsync(ListBackupVaultsRequest listBackupVaultsRequest);
/**
*
* Returns a list of recovery point storage containers along with information about them.
*
*
* @param listBackupVaultsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListBackupVaults operation returned by the service.
* @sample AWSBackupAsyncHandler.ListBackupVaults
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listBackupVaultsAsync(ListBackupVaultsRequest listBackupVaultsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This request obtains a list of copy jobs created or running within the the most recent 30 days. You can include
* parameters AccountID, State, ResourceType, MessageCategory, AggregationPeriod, MaxResults, or NextToken to filter
* results.
*
*
* This request returns a summary that contains Region, Account, State, RestourceType, MessageCategory, StartTime,
* EndTime, and Count of included jobs.
*
*
* @param listCopyJobSummariesRequest
* @return A Java Future containing the result of the ListCopyJobSummaries operation returned by the service.
* @sample AWSBackupAsync.ListCopyJobSummaries
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listCopyJobSummariesAsync(ListCopyJobSummariesRequest listCopyJobSummariesRequest);
/**
*
* This request obtains a list of copy jobs created or running within the the most recent 30 days. You can include
* parameters AccountID, State, ResourceType, MessageCategory, AggregationPeriod, MaxResults, or NextToken to filter
* results.
*
*
* This request returns a summary that contains Region, Account, State, RestourceType, MessageCategory, StartTime,
* EndTime, and Count of included jobs.
*
*
* @param listCopyJobSummariesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListCopyJobSummaries operation returned by the service.
* @sample AWSBackupAsyncHandler.ListCopyJobSummaries
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listCopyJobSummariesAsync(ListCopyJobSummariesRequest listCopyJobSummariesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns metadata about your copy jobs.
*
*
* @param listCopyJobsRequest
* @return A Java Future containing the result of the ListCopyJobs operation returned by the service.
* @sample AWSBackupAsync.ListCopyJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listCopyJobsAsync(ListCopyJobsRequest listCopyJobsRequest);
/**
*
* Returns metadata about your copy jobs.
*
*
* @param listCopyJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListCopyJobs operation returned by the service.
* @sample AWSBackupAsyncHandler.ListCopyJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listCopyJobsAsync(ListCopyJobsRequest listCopyJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all frameworks for an Amazon Web Services account and Amazon Web Services Region.
*
*
* @param listFrameworksRequest
* @return A Java Future containing the result of the ListFrameworks operation returned by the service.
* @sample AWSBackupAsync.ListFrameworks
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFrameworksAsync(ListFrameworksRequest listFrameworksRequest);
/**
*
* Returns a list of all frameworks for an Amazon Web Services account and Amazon Web Services Region.
*
*
* @param listFrameworksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFrameworks operation returned by the service.
* @sample AWSBackupAsyncHandler.ListFrameworks
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFrameworksAsync(ListFrameworksRequest listFrameworksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This action returns metadata about active and previous legal holds.
*
*
* @param listLegalHoldsRequest
* @return A Java Future containing the result of the ListLegalHolds operation returned by the service.
* @sample AWSBackupAsync.ListLegalHolds
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listLegalHoldsAsync(ListLegalHoldsRequest listLegalHoldsRequest);
/**
*
* This action returns metadata about active and previous legal holds.
*
*
* @param listLegalHoldsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListLegalHolds operation returned by the service.
* @sample AWSBackupAsyncHandler.ListLegalHolds
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listLegalHoldsAsync(ListLegalHoldsRequest listLegalHoldsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an array of resources successfully backed up by Backup, including the time the resource was saved, an
* Amazon Resource Name (ARN) of the resource, and a resource type.
*
*
* @param listProtectedResourcesRequest
* @return A Java Future containing the result of the ListProtectedResources operation returned by the service.
* @sample AWSBackupAsync.ListProtectedResources
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listProtectedResourcesAsync(ListProtectedResourcesRequest listProtectedResourcesRequest);
/**
*
* Returns an array of resources successfully backed up by Backup, including the time the resource was saved, an
* Amazon Resource Name (ARN) of the resource, and a resource type.
*
*
* @param listProtectedResourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListProtectedResources operation returned by the service.
* @sample AWSBackupAsyncHandler.ListProtectedResources
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listProtectedResourcesAsync(ListProtectedResourcesRequest listProtectedResourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This request lists the protected resources corresponding to each backup vault.
*
*
* @param listProtectedResourcesByBackupVaultRequest
* @return A Java Future containing the result of the ListProtectedResourcesByBackupVault operation returned by the
* service.
* @sample AWSBackupAsync.ListProtectedResourcesByBackupVault
* @see AWS API Documentation
*/
java.util.concurrent.Future listProtectedResourcesByBackupVaultAsync(
ListProtectedResourcesByBackupVaultRequest listProtectedResourcesByBackupVaultRequest);
/**
*
* This request lists the protected resources corresponding to each backup vault.
*
*
* @param listProtectedResourcesByBackupVaultRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListProtectedResourcesByBackupVault operation returned by the
* service.
* @sample AWSBackupAsyncHandler.ListProtectedResourcesByBackupVault
* @see AWS API Documentation
*/
java.util.concurrent.Future listProtectedResourcesByBackupVaultAsync(
ListProtectedResourcesByBackupVaultRequest listProtectedResourcesByBackupVaultRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns detailed information about the recovery points stored in a backup vault.
*
*
* @param listRecoveryPointsByBackupVaultRequest
* @return A Java Future containing the result of the ListRecoveryPointsByBackupVault operation returned by the
* service.
* @sample AWSBackupAsync.ListRecoveryPointsByBackupVault
* @see AWS API Documentation
*/
java.util.concurrent.Future listRecoveryPointsByBackupVaultAsync(
ListRecoveryPointsByBackupVaultRequest listRecoveryPointsByBackupVaultRequest);
/**
*
* Returns detailed information about the recovery points stored in a backup vault.
*
*
* @param listRecoveryPointsByBackupVaultRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRecoveryPointsByBackupVault operation returned by the
* service.
* @sample AWSBackupAsyncHandler.ListRecoveryPointsByBackupVault
* @see AWS API Documentation
*/
java.util.concurrent.Future listRecoveryPointsByBackupVaultAsync(
ListRecoveryPointsByBackupVaultRequest listRecoveryPointsByBackupVaultRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This action returns recovery point ARNs (Amazon Resource Names) of the specified legal hold.
*
*
* @param listRecoveryPointsByLegalHoldRequest
* @return A Java Future containing the result of the ListRecoveryPointsByLegalHold operation returned by the
* service.
* @sample AWSBackupAsync.ListRecoveryPointsByLegalHold
* @see AWS API Documentation
*/
java.util.concurrent.Future listRecoveryPointsByLegalHoldAsync(
ListRecoveryPointsByLegalHoldRequest listRecoveryPointsByLegalHoldRequest);
/**
*
* This action returns recovery point ARNs (Amazon Resource Names) of the specified legal hold.
*
*
* @param listRecoveryPointsByLegalHoldRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRecoveryPointsByLegalHold operation returned by the
* service.
* @sample AWSBackupAsyncHandler.ListRecoveryPointsByLegalHold
* @see AWS API Documentation
*/
java.util.concurrent.Future listRecoveryPointsByLegalHoldAsync(
ListRecoveryPointsByLegalHoldRequest listRecoveryPointsByLegalHoldRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns detailed information about all the recovery points of the type specified by a resource Amazon Resource
* Name (ARN).
*
*
*
* For Amazon EFS and Amazon EC2, this action only lists recovery points created by Backup.
*
*
*
* @param listRecoveryPointsByResourceRequest
* @return A Java Future containing the result of the ListRecoveryPointsByResource operation returned by the
* service.
* @sample AWSBackupAsync.ListRecoveryPointsByResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listRecoveryPointsByResourceAsync(
ListRecoveryPointsByResourceRequest listRecoveryPointsByResourceRequest);
/**
*
* Returns detailed information about all the recovery points of the type specified by a resource Amazon Resource
* Name (ARN).
*
*
*
* For Amazon EFS and Amazon EC2, this action only lists recovery points created by Backup.
*
*
*
* @param listRecoveryPointsByResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRecoveryPointsByResource operation returned by the
* service.
* @sample AWSBackupAsyncHandler.ListRecoveryPointsByResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listRecoveryPointsByResourceAsync(
ListRecoveryPointsByResourceRequest listRecoveryPointsByResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns details about your report jobs.
*
*
* @param listReportJobsRequest
* @return A Java Future containing the result of the ListReportJobs operation returned by the service.
* @sample AWSBackupAsync.ListReportJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listReportJobsAsync(ListReportJobsRequest listReportJobsRequest);
/**
*
* Returns details about your report jobs.
*
*
* @param listReportJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListReportJobs operation returned by the service.
* @sample AWSBackupAsyncHandler.ListReportJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listReportJobsAsync(ListReportJobsRequest listReportJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of your report plans. For detailed information about a single report plan, use
* DescribeReportPlan
.
*
*
* @param listReportPlansRequest
* @return A Java Future containing the result of the ListReportPlans operation returned by the service.
* @sample AWSBackupAsync.ListReportPlans
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listReportPlansAsync(ListReportPlansRequest listReportPlansRequest);
/**
*
* Returns a list of your report plans. For detailed information about a single report plan, use
* DescribeReportPlan
.
*
*
* @param listReportPlansRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListReportPlans operation returned by the service.
* @sample AWSBackupAsyncHandler.ListReportPlans
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listReportPlansAsync(ListReportPlansRequest listReportPlansRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This request obtains a summary of restore jobs created or running within the the most recent 30 days. You can
* include parameters AccountID, State, ResourceType, AggregationPeriod, MaxResults, or NextToken to filter results.
*
*
* This request returns a summary that contains Region, Account, State, RestourceType, MessageCategory, StartTime,
* EndTime, and Count of included jobs.
*
*
* @param listRestoreJobSummariesRequest
* @return A Java Future containing the result of the ListRestoreJobSummaries operation returned by the service.
* @sample AWSBackupAsync.ListRestoreJobSummaries
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listRestoreJobSummariesAsync(ListRestoreJobSummariesRequest listRestoreJobSummariesRequest);
/**
*
* This request obtains a summary of restore jobs created or running within the the most recent 30 days. You can
* include parameters AccountID, State, ResourceType, AggregationPeriod, MaxResults, or NextToken to filter results.
*
*
* This request returns a summary that contains Region, Account, State, RestourceType, MessageCategory, StartTime,
* EndTime, and Count of included jobs.
*
*
* @param listRestoreJobSummariesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRestoreJobSummaries operation returned by the service.
* @sample AWSBackupAsyncHandler.ListRestoreJobSummaries
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listRestoreJobSummariesAsync(ListRestoreJobSummariesRequest listRestoreJobSummariesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of jobs that Backup initiated to restore a saved resource, including details about the recovery
* process.
*
*
* @param listRestoreJobsRequest
* @return A Java Future containing the result of the ListRestoreJobs operation returned by the service.
* @sample AWSBackupAsync.ListRestoreJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listRestoreJobsAsync(ListRestoreJobsRequest listRestoreJobsRequest);
/**
*
* Returns a list of jobs that Backup initiated to restore a saved resource, including details about the recovery
* process.
*
*
* @param listRestoreJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRestoreJobs operation returned by the service.
* @sample AWSBackupAsyncHandler.ListRestoreJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listRestoreJobsAsync(ListRestoreJobsRequest listRestoreJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This returns restore jobs that contain the specified protected resource.
*
*
* You must include ResourceArn
. You can optionally include NextToken
,
* ByStatus
, MaxResults
, ByRecoveryPointCreationDateAfter
, and
* ByRecoveryPointCreationDateBefore
.
*
*
* @param listRestoreJobsByProtectedResourceRequest
* @return A Java Future containing the result of the ListRestoreJobsByProtectedResource operation returned by the
* service.
* @sample AWSBackupAsync.ListRestoreJobsByProtectedResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listRestoreJobsByProtectedResourceAsync(
ListRestoreJobsByProtectedResourceRequest listRestoreJobsByProtectedResourceRequest);
/**
*
* This returns restore jobs that contain the specified protected resource.
*
*
* You must include ResourceArn
. You can optionally include NextToken
,
* ByStatus
, MaxResults
, ByRecoveryPointCreationDateAfter
, and
* ByRecoveryPointCreationDateBefore
.
*
*
* @param listRestoreJobsByProtectedResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRestoreJobsByProtectedResource operation returned by the
* service.
* @sample AWSBackupAsyncHandler.ListRestoreJobsByProtectedResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listRestoreJobsByProtectedResourceAsync(
ListRestoreJobsByProtectedResourceRequest listRestoreJobsByProtectedResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of restore testing plans.
*
*
* @param listRestoreTestingPlansRequest
* @return A Java Future containing the result of the ListRestoreTestingPlans operation returned by the service.
* @sample AWSBackupAsync.ListRestoreTestingPlans
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listRestoreTestingPlansAsync(ListRestoreTestingPlansRequest listRestoreTestingPlansRequest);
/**
*
* Returns a list of restore testing plans.
*
*
* @param listRestoreTestingPlansRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRestoreTestingPlans operation returned by the service.
* @sample AWSBackupAsyncHandler.ListRestoreTestingPlans
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listRestoreTestingPlansAsync(ListRestoreTestingPlansRequest listRestoreTestingPlansRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of restore testing selections. Can be filtered by MaxResults
and
* RestoreTestingPlanName
.
*
*
* @param listRestoreTestingSelectionsRequest
* @return A Java Future containing the result of the ListRestoreTestingSelections operation returned by the
* service.
* @sample AWSBackupAsync.ListRestoreTestingSelections
* @see AWS API Documentation
*/
java.util.concurrent.Future listRestoreTestingSelectionsAsync(
ListRestoreTestingSelectionsRequest listRestoreTestingSelectionsRequest);
/**
*
* Returns a list of restore testing selections. Can be filtered by MaxResults
and
* RestoreTestingPlanName
.
*
*
* @param listRestoreTestingSelectionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRestoreTestingSelections operation returned by the
* service.
* @sample AWSBackupAsyncHandler.ListRestoreTestingSelections
* @see AWS API Documentation
*/
java.util.concurrent.Future listRestoreTestingSelectionsAsync(
ListRestoreTestingSelectionsRequest listRestoreTestingSelectionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of key-value pairs assigned to a target recovery point, backup plan, or backup vault.
*
*
* ListTags
only works for resource types that support full Backup management of their backups. Those
* resource types are listed in the "Full Backup management" section of the Feature
* availability by resource table.
*
*
* @param listTagsRequest
* @return A Java Future containing the result of the ListTags operation returned by the service.
* @sample AWSBackupAsync.ListTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsAsync(ListTagsRequest listTagsRequest);
/**
*
* Returns a list of key-value pairs assigned to a target recovery point, backup plan, or backup vault.
*
*
* ListTags
only works for resource types that support full Backup management of their backups. Those
* resource types are listed in the "Full Backup management" section of the Feature
* availability by resource table.
*
*
* @param listTagsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTags operation returned by the service.
* @sample AWSBackupAsyncHandler.ListTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsAsync(ListTagsRequest listTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets a resource-based policy that is used to manage access permissions on the target backup vault. Requires a
* backup vault name and an access policy document in JSON format.
*
*
* @param putBackupVaultAccessPolicyRequest
* @return A Java Future containing the result of the PutBackupVaultAccessPolicy operation returned by the service.
* @sample AWSBackupAsync.PutBackupVaultAccessPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future putBackupVaultAccessPolicyAsync(
PutBackupVaultAccessPolicyRequest putBackupVaultAccessPolicyRequest);
/**
*
* Sets a resource-based policy that is used to manage access permissions on the target backup vault. Requires a
* backup vault name and an access policy document in JSON format.
*
*
* @param putBackupVaultAccessPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutBackupVaultAccessPolicy operation returned by the service.
* @sample AWSBackupAsyncHandler.PutBackupVaultAccessPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future putBackupVaultAccessPolicyAsync(
PutBackupVaultAccessPolicyRequest putBackupVaultAccessPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Applies Backup Vault Lock to a backup vault, preventing attempts to delete any recovery point stored in or
* created in a backup vault. Vault Lock also prevents attempts to update the lifecycle policy that controls the
* retention period of any recovery point currently stored in a backup vault. If specified, Vault Lock enforces a
* minimum and maximum retention period for future backup and copy jobs that target a backup vault.
*
*
*
* Backup Vault Lock has been assessed by Cohasset Associates for use in environments that are subject to SEC 17a-4,
* CFTC, and FINRA regulations. For more information about how Backup Vault Lock relates to these regulations, see
* the Cohasset Associates Compliance Assessment.
*
*
*
* @param putBackupVaultLockConfigurationRequest
* @return A Java Future containing the result of the PutBackupVaultLockConfiguration operation returned by the
* service.
* @sample AWSBackupAsync.PutBackupVaultLockConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future putBackupVaultLockConfigurationAsync(
PutBackupVaultLockConfigurationRequest putBackupVaultLockConfigurationRequest);
/**
*
* Applies Backup Vault Lock to a backup vault, preventing attempts to delete any recovery point stored in or
* created in a backup vault. Vault Lock also prevents attempts to update the lifecycle policy that controls the
* retention period of any recovery point currently stored in a backup vault. If specified, Vault Lock enforces a
* minimum and maximum retention period for future backup and copy jobs that target a backup vault.
*
*
*
* Backup Vault Lock has been assessed by Cohasset Associates for use in environments that are subject to SEC 17a-4,
* CFTC, and FINRA regulations. For more information about how Backup Vault Lock relates to these regulations, see
* the Cohasset Associates Compliance Assessment.
*
*
*
* @param putBackupVaultLockConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutBackupVaultLockConfiguration operation returned by the
* service.
* @sample AWSBackupAsyncHandler.PutBackupVaultLockConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future putBackupVaultLockConfigurationAsync(
PutBackupVaultLockConfigurationRequest putBackupVaultLockConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Turns on notifications on a backup vault for the specified topic and events.
*
*
* @param putBackupVaultNotificationsRequest
* @return A Java Future containing the result of the PutBackupVaultNotifications operation returned by the service.
* @sample AWSBackupAsync.PutBackupVaultNotifications
* @see AWS API Documentation
*/
java.util.concurrent.Future putBackupVaultNotificationsAsync(
PutBackupVaultNotificationsRequest putBackupVaultNotificationsRequest);
/**
*
* Turns on notifications on a backup vault for the specified topic and events.
*
*
* @param putBackupVaultNotificationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutBackupVaultNotifications operation returned by the service.
* @sample AWSBackupAsyncHandler.PutBackupVaultNotifications
* @see AWS API Documentation
*/
java.util.concurrent.Future putBackupVaultNotificationsAsync(
PutBackupVaultNotificationsRequest putBackupVaultNotificationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This request allows you to send your independent self-run restore test validation results.
* RestoreJobId
and ValidationStatus
are required. Optionally, you can input a
* ValidationStatusMessage
.
*
*
* @param putRestoreValidationResultRequest
* @return A Java Future containing the result of the PutRestoreValidationResult operation returned by the service.
* @sample AWSBackupAsync.PutRestoreValidationResult
* @see AWS API Documentation
*/
java.util.concurrent.Future putRestoreValidationResultAsync(
PutRestoreValidationResultRequest putRestoreValidationResultRequest);
/**
*
* This request allows you to send your independent self-run restore test validation results.
* RestoreJobId
and ValidationStatus
are required. Optionally, you can input a
* ValidationStatusMessage
.
*
*
* @param putRestoreValidationResultRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutRestoreValidationResult operation returned by the service.
* @sample AWSBackupAsyncHandler.PutRestoreValidationResult
* @see AWS API Documentation
*/
java.util.concurrent.Future putRestoreValidationResultAsync(
PutRestoreValidationResultRequest putRestoreValidationResultRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts an on-demand backup job for the specified resource.
*
*
* @param startBackupJobRequest
* @return A Java Future containing the result of the StartBackupJob operation returned by the service.
* @sample AWSBackupAsync.StartBackupJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startBackupJobAsync(StartBackupJobRequest startBackupJobRequest);
/**
*
* Starts an on-demand backup job for the specified resource.
*
*
* @param startBackupJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartBackupJob operation returned by the service.
* @sample AWSBackupAsyncHandler.StartBackupJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startBackupJobAsync(StartBackupJobRequest startBackupJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts a job to create a one-time copy of the specified resource.
*
*
* Does not support continuous backups.
*
*
* @param startCopyJobRequest
* @return A Java Future containing the result of the StartCopyJob operation returned by the service.
* @sample AWSBackupAsync.StartCopyJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startCopyJobAsync(StartCopyJobRequest startCopyJobRequest);
/**
*
* Starts a job to create a one-time copy of the specified resource.
*
*
* Does not support continuous backups.
*
*
* @param startCopyJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartCopyJob operation returned by the service.
* @sample AWSBackupAsyncHandler.StartCopyJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startCopyJobAsync(StartCopyJobRequest startCopyJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts an on-demand report job for the specified report plan.
*
*
* @param startReportJobRequest
* @return A Java Future containing the result of the StartReportJob operation returned by the service.
* @sample AWSBackupAsync.StartReportJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startReportJobAsync(StartReportJobRequest startReportJobRequest);
/**
*
* Starts an on-demand report job for the specified report plan.
*
*
* @param startReportJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartReportJob operation returned by the service.
* @sample AWSBackupAsyncHandler.StartReportJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startReportJobAsync(StartReportJobRequest startReportJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Recovers the saved resource identified by an Amazon Resource Name (ARN).
*
*
* @param startRestoreJobRequest
* @return A Java Future containing the result of the StartRestoreJob operation returned by the service.
* @sample AWSBackupAsync.StartRestoreJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startRestoreJobAsync(StartRestoreJobRequest startRestoreJobRequest);
/**
*
* Recovers the saved resource identified by an Amazon Resource Name (ARN).
*
*
* @param startRestoreJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartRestoreJob operation returned by the service.
* @sample AWSBackupAsyncHandler.StartRestoreJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startRestoreJobAsync(StartRestoreJobRequest startRestoreJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Attempts to cancel a job to create a one-time backup of a resource.
*
*
* This action is not supported for the following services: Amazon FSx for Windows File Server, Amazon FSx for
* Lustre, Amazon FSx for NetApp ONTAP , Amazon FSx for OpenZFS, Amazon DocumentDB (with MongoDB compatibility),
* Amazon RDS, Amazon Aurora, and Amazon Neptune.
*
*
* @param stopBackupJobRequest
* @return A Java Future containing the result of the StopBackupJob operation returned by the service.
* @sample AWSBackupAsync.StopBackupJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopBackupJobAsync(StopBackupJobRequest stopBackupJobRequest);
/**
*
* Attempts to cancel a job to create a one-time backup of a resource.
*
*
* This action is not supported for the following services: Amazon FSx for Windows File Server, Amazon FSx for
* Lustre, Amazon FSx for NetApp ONTAP , Amazon FSx for OpenZFS, Amazon DocumentDB (with MongoDB compatibility),
* Amazon RDS, Amazon Aurora, and Amazon Neptune.
*
*
* @param stopBackupJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopBackupJob operation returned by the service.
* @sample AWSBackupAsyncHandler.StopBackupJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopBackupJobAsync(StopBackupJobRequest stopBackupJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns a set of key-value pairs to a recovery point, backup plan, or backup vault identified by an Amazon
* Resource Name (ARN).
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSBackupAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Assigns a set of key-value pairs to a recovery point, backup plan, or backup vault identified by an Amazon
* Resource Name (ARN).
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSBackupAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a set of key-value pairs from a recovery point, backup plan, or backup vault identified by an Amazon
* Resource Name (ARN)
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSBackupAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes a set of key-value pairs from a recovery point, backup plan, or backup vault identified by an Amazon
* Resource Name (ARN)
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSBackupAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing backup plan identified by its