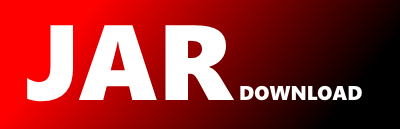
com.amazonaws.services.backup.AWSBackupClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-backup Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.backup;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.backup.AWSBackupClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.backup.model.*;
import com.amazonaws.services.backup.model.transform.*;
/**
* Client for accessing AWS Backup. All service calls made using this client are blocking, and will not return until the
* service call completes.
*
* Backup
*
* Backup is a unified backup service designed to protect Amazon Web Services services and their associated data. Backup
* simplifies the creation, migration, restoration, and deletion of backups, while also providing reporting and
* auditing.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSBackupClient extends AmazonWebServiceClient implements AWSBackup {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSBackup.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "backup";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("MissingParameterValueException").withExceptionUnmarshaller(
com.amazonaws.services.backup.model.transform.MissingParameterValueExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidRequestException").withExceptionUnmarshaller(
com.amazonaws.services.backup.model.transform.InvalidRequestExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("LimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.backup.model.transform.LimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("DependencyFailureException").withExceptionUnmarshaller(
com.amazonaws.services.backup.model.transform.DependencyFailureExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.backup.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AlreadyExistsException").withExceptionUnmarshaller(
com.amazonaws.services.backup.model.transform.AlreadyExistsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidParameterValueException").withExceptionUnmarshaller(
com.amazonaws.services.backup.model.transform.InvalidParameterValueExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.backup.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidResourceStateException").withExceptionUnmarshaller(
com.amazonaws.services.backup.model.transform.InvalidResourceStateExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceUnavailableException").withExceptionUnmarshaller(
com.amazonaws.services.backup.model.transform.ServiceUnavailableExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.backup.model.AWSBackupException.class));
public static AWSBackupClientBuilder builder() {
return AWSBackupClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on AWS Backup using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSBackupClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on AWS Backup using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSBackupClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("backup.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/backup/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/backup/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* This action removes the specified legal hold on a recovery point. This action can only be performed by a user
* with sufficient permissions.
*
*
* @param cancelLegalHoldRequest
* @return Result of the CancelLegalHold operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws InvalidResourceStateException
* Backup is already performing an action on this recovery point. It can't perform the action you requested
* until the first action finishes. Try again later.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @sample AWSBackup.CancelLegalHold
* @see AWS API
* Documentation
*/
@Override
public CancelLegalHoldResult cancelLegalHold(CancelLegalHoldRequest request) {
request = beforeClientExecution(request);
return executeCancelLegalHold(request);
}
@SdkInternalApi
final CancelLegalHoldResult executeCancelLegalHold(CancelLegalHoldRequest cancelLegalHoldRequest) {
ExecutionContext executionContext = createExecutionContext(cancelLegalHoldRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CancelLegalHoldRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(cancelLegalHoldRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CancelLegalHold");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CancelLegalHoldResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a backup plan using a backup plan name and backup rules. A backup plan is a document that contains
* information that Backup uses to schedule tasks that create recovery points for resources.
*
*
* If you call CreateBackupPlan
with a plan that already exists, you receive an
* AlreadyExistsException
exception.
*
*
* @param createBackupPlanRequest
* @return Result of the CreateBackupPlan operation returned by the service.
* @throws LimitExceededException
* A limit in the request has been exceeded; for example, a maximum number of items allowed in a request.
* @throws AlreadyExistsException
* The required resource already exists.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.CreateBackupPlan
* @see AWS API
* Documentation
*/
@Override
public CreateBackupPlanResult createBackupPlan(CreateBackupPlanRequest request) {
request = beforeClientExecution(request);
return executeCreateBackupPlan(request);
}
@SdkInternalApi
final CreateBackupPlanResult executeCreateBackupPlan(CreateBackupPlanRequest createBackupPlanRequest) {
ExecutionContext executionContext = createExecutionContext(createBackupPlanRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateBackupPlanRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createBackupPlanRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateBackupPlan");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateBackupPlanResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a JSON document that specifies a set of resources to assign to a backup plan. For examples, see Assigning resources programmatically.
*
*
* @param createBackupSelectionRequest
* @return Result of the CreateBackupSelection operation returned by the service.
* @throws LimitExceededException
* A limit in the request has been exceeded; for example, a maximum number of items allowed in a request.
* @throws AlreadyExistsException
* The required resource already exists.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.CreateBackupSelection
* @see AWS
* API Documentation
*/
@Override
public CreateBackupSelectionResult createBackupSelection(CreateBackupSelectionRequest request) {
request = beforeClientExecution(request);
return executeCreateBackupSelection(request);
}
@SdkInternalApi
final CreateBackupSelectionResult executeCreateBackupSelection(CreateBackupSelectionRequest createBackupSelectionRequest) {
ExecutionContext executionContext = createExecutionContext(createBackupSelectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateBackupSelectionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createBackupSelectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateBackupSelection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateBackupSelectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a logical container where backups are stored. A CreateBackupVault
request includes a name,
* optionally one or more resource tags, an encryption key, and a request ID.
*
*
*
* Do not include sensitive data, such as passport numbers, in the name of a backup vault.
*
*
*
* @param createBackupVaultRequest
* @return Result of the CreateBackupVault operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws LimitExceededException
* A limit in the request has been exceeded; for example, a maximum number of items allowed in a request.
* @throws AlreadyExistsException
* The required resource already exists.
* @sample AWSBackup.CreateBackupVault
* @see AWS API
* Documentation
*/
@Override
public CreateBackupVaultResult createBackupVault(CreateBackupVaultRequest request) {
request = beforeClientExecution(request);
return executeCreateBackupVault(request);
}
@SdkInternalApi
final CreateBackupVaultResult executeCreateBackupVault(CreateBackupVaultRequest createBackupVaultRequest) {
ExecutionContext executionContext = createExecutionContext(createBackupVaultRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateBackupVaultRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createBackupVaultRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateBackupVault");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateBackupVaultResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a framework with one or more controls. A framework is a collection of controls that you can use to
* evaluate your backup practices. By using pre-built customizable controls to define your policies, you can
* evaluate whether your backup practices comply with your policies and which resources are not yet in compliance.
*
*
* @param createFrameworkRequest
* @return Result of the CreateFramework operation returned by the service.
* @throws AlreadyExistsException
* The required resource already exists.
* @throws LimitExceededException
* A limit in the request has been exceeded; for example, a maximum number of items allowed in a request.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.CreateFramework
* @see AWS API
* Documentation
*/
@Override
public CreateFrameworkResult createFramework(CreateFrameworkRequest request) {
request = beforeClientExecution(request);
return executeCreateFramework(request);
}
@SdkInternalApi
final CreateFrameworkResult executeCreateFramework(CreateFrameworkRequest createFrameworkRequest) {
ExecutionContext executionContext = createExecutionContext(createFrameworkRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateFrameworkRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createFrameworkRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateFramework");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateFrameworkResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This action creates a legal hold on a recovery point (backup). A legal hold is a restraint on altering or
* deleting a backup until an authorized user cancels the legal hold. Any actions to delete or disassociate a
* recovery point will fail with an error if one or more active legal holds are on the recovery point.
*
*
* @param createLegalHoldRequest
* @return Result of the CreateLegalHold operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws LimitExceededException
* A limit in the request has been exceeded; for example, a maximum number of items allowed in a request.
* @sample AWSBackup.CreateLegalHold
* @see AWS API
* Documentation
*/
@Override
public CreateLegalHoldResult createLegalHold(CreateLegalHoldRequest request) {
request = beforeClientExecution(request);
return executeCreateLegalHold(request);
}
@SdkInternalApi
final CreateLegalHoldResult executeCreateLegalHold(CreateLegalHoldRequest createLegalHoldRequest) {
ExecutionContext executionContext = createExecutionContext(createLegalHoldRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateLegalHoldRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createLegalHoldRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateLegalHold");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateLegalHoldResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This request creates a logical container to where backups may be copied.
*
*
* This request includes a name, the Region, the maximum number of retention days, the minimum number of retention
* days, and optionally can include tags and a creator request ID.
*
*
*
* Do not include sensitive data, such as passport numbers, in the name of a backup vault.
*
*
*
* @param createLogicallyAirGappedBackupVaultRequest
* @return Result of the CreateLogicallyAirGappedBackupVault operation returned by the service.
* @throws AlreadyExistsException
* The required resource already exists.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws LimitExceededException
* A limit in the request has been exceeded; for example, a maximum number of items allowed in a request.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws InvalidRequestException
* Indicates that something is wrong with the input to the request. For example, a parameter is of the wrong
* type.
* @sample AWSBackup.CreateLogicallyAirGappedBackupVault
* @see AWS API Documentation
*/
@Override
public CreateLogicallyAirGappedBackupVaultResult createLogicallyAirGappedBackupVault(CreateLogicallyAirGappedBackupVaultRequest request) {
request = beforeClientExecution(request);
return executeCreateLogicallyAirGappedBackupVault(request);
}
@SdkInternalApi
final CreateLogicallyAirGappedBackupVaultResult executeCreateLogicallyAirGappedBackupVault(
CreateLogicallyAirGappedBackupVaultRequest createLogicallyAirGappedBackupVaultRequest) {
ExecutionContext executionContext = createExecutionContext(createLogicallyAirGappedBackupVaultRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateLogicallyAirGappedBackupVaultRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createLogicallyAirGappedBackupVaultRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateLogicallyAirGappedBackupVault");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateLogicallyAirGappedBackupVaultResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a report plan. A report plan is a document that contains information about the contents of the report and
* where Backup will deliver it.
*
*
* If you call CreateReportPlan
with a plan that already exists, you receive an
* AlreadyExistsException
exception.
*
*
* @param createReportPlanRequest
* @return Result of the CreateReportPlan operation returned by the service.
* @throws AlreadyExistsException
* The required resource already exists.
* @throws LimitExceededException
* A limit in the request has been exceeded; for example, a maximum number of items allowed in a request.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @sample AWSBackup.CreateReportPlan
* @see AWS API
* Documentation
*/
@Override
public CreateReportPlanResult createReportPlan(CreateReportPlanRequest request) {
request = beforeClientExecution(request);
return executeCreateReportPlan(request);
}
@SdkInternalApi
final CreateReportPlanResult executeCreateReportPlan(CreateReportPlanRequest createReportPlanRequest) {
ExecutionContext executionContext = createExecutionContext(createReportPlanRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateReportPlanRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createReportPlanRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateReportPlan");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateReportPlanResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This is the first of two steps to create a restore testing plan; once this request is successful, finish the
* procedure with request CreateRestoreTestingSelection.
*
*
* You must include the parameter RestoreTestingPlan. You may optionally include CreatorRequestId and Tags.
*
*
* @param createRestoreTestingPlanRequest
* @return Result of the CreateRestoreTestingPlan operation returned by the service.
* @throws AlreadyExistsException
* The required resource already exists.
* @throws ConflictException
* Backup can't perform the action that you requested until it finishes performing a previous action. Try
* again later.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws LimitExceededException
* A limit in the request has been exceeded; for example, a maximum number of items allowed in a request.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.CreateRestoreTestingPlan
* @see AWS API Documentation
*/
@Override
public CreateRestoreTestingPlanResult createRestoreTestingPlan(CreateRestoreTestingPlanRequest request) {
request = beforeClientExecution(request);
return executeCreateRestoreTestingPlan(request);
}
@SdkInternalApi
final CreateRestoreTestingPlanResult executeCreateRestoreTestingPlan(CreateRestoreTestingPlanRequest createRestoreTestingPlanRequest) {
ExecutionContext executionContext = createExecutionContext(createRestoreTestingPlanRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateRestoreTestingPlanRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createRestoreTestingPlanRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateRestoreTestingPlan");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateRestoreTestingPlanResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This request can be sent after CreateRestoreTestingPlan request returns successfully. This is the second part of
* creating a resource testing plan, and it must be completed sequentially.
*
*
* This consists of RestoreTestingSelectionName
, ProtectedResourceType
, and one of the
* following:
*
*
* -
*
* ProtectedResourceArns
*
*
* -
*
* ProtectedResourceConditions
*
*
*
*
* Each protected resource type can have one single value.
*
*
* A restore testing selection can include a wildcard value ("*") for ProtectedResourceArns
along with
* ProtectedResourceConditions
. Alternatively, you can include up to 30 specific protected resource
* ARNs in ProtectedResourceArns
.
*
*
* Cannot select by both protected resource types AND specific ARNs. Request will fail if both are included.
*
*
* @param createRestoreTestingSelectionRequest
* @return Result of the CreateRestoreTestingSelection operation returned by the service.
* @throws AlreadyExistsException
* The required resource already exists.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws LimitExceededException
* A limit in the request has been exceeded; for example, a maximum number of items allowed in a request.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.CreateRestoreTestingSelection
* @see AWS API Documentation
*/
@Override
public CreateRestoreTestingSelectionResult createRestoreTestingSelection(CreateRestoreTestingSelectionRequest request) {
request = beforeClientExecution(request);
return executeCreateRestoreTestingSelection(request);
}
@SdkInternalApi
final CreateRestoreTestingSelectionResult executeCreateRestoreTestingSelection(CreateRestoreTestingSelectionRequest createRestoreTestingSelectionRequest) {
ExecutionContext executionContext = createExecutionContext(createRestoreTestingSelectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateRestoreTestingSelectionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createRestoreTestingSelectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateRestoreTestingSelection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateRestoreTestingSelectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a backup plan. A backup plan can only be deleted after all associated selections of resources have been
* deleted. Deleting a backup plan deletes the current version of a backup plan. Previous versions, if any, will
* still exist.
*
*
* @param deleteBackupPlanRequest
* @return Result of the DeleteBackupPlan operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws InvalidRequestException
* Indicates that something is wrong with the input to the request. For example, a parameter is of the wrong
* type.
* @sample AWSBackup.DeleteBackupPlan
* @see AWS API
* Documentation
*/
@Override
public DeleteBackupPlanResult deleteBackupPlan(DeleteBackupPlanRequest request) {
request = beforeClientExecution(request);
return executeDeleteBackupPlan(request);
}
@SdkInternalApi
final DeleteBackupPlanResult executeDeleteBackupPlan(DeleteBackupPlanRequest deleteBackupPlanRequest) {
ExecutionContext executionContext = createExecutionContext(deleteBackupPlanRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteBackupPlanRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteBackupPlanRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteBackupPlan");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteBackupPlanResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the resource selection associated with a backup plan that is specified by the SelectionId
.
*
*
* @param deleteBackupSelectionRequest
* @return Result of the DeleteBackupSelection operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.DeleteBackupSelection
* @see AWS
* API Documentation
*/
@Override
public DeleteBackupSelectionResult deleteBackupSelection(DeleteBackupSelectionRequest request) {
request = beforeClientExecution(request);
return executeDeleteBackupSelection(request);
}
@SdkInternalApi
final DeleteBackupSelectionResult executeDeleteBackupSelection(DeleteBackupSelectionRequest deleteBackupSelectionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteBackupSelectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteBackupSelectionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteBackupSelectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteBackupSelection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteBackupSelectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the backup vault identified by its name. A vault can be deleted only if it is empty.
*
*
* @param deleteBackupVaultRequest
* @return Result of the DeleteBackupVault operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws InvalidRequestException
* Indicates that something is wrong with the input to the request. For example, a parameter is of the wrong
* type.
* @sample AWSBackup.DeleteBackupVault
* @see AWS API
* Documentation
*/
@Override
public DeleteBackupVaultResult deleteBackupVault(DeleteBackupVaultRequest request) {
request = beforeClientExecution(request);
return executeDeleteBackupVault(request);
}
@SdkInternalApi
final DeleteBackupVaultResult executeDeleteBackupVault(DeleteBackupVaultRequest deleteBackupVaultRequest) {
ExecutionContext executionContext = createExecutionContext(deleteBackupVaultRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteBackupVaultRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteBackupVaultRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteBackupVault");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteBackupVaultResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the policy document that manages permissions on a backup vault.
*
*
* @param deleteBackupVaultAccessPolicyRequest
* @return Result of the DeleteBackupVaultAccessPolicy operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.DeleteBackupVaultAccessPolicy
* @see AWS API Documentation
*/
@Override
public DeleteBackupVaultAccessPolicyResult deleteBackupVaultAccessPolicy(DeleteBackupVaultAccessPolicyRequest request) {
request = beforeClientExecution(request);
return executeDeleteBackupVaultAccessPolicy(request);
}
@SdkInternalApi
final DeleteBackupVaultAccessPolicyResult executeDeleteBackupVaultAccessPolicy(DeleteBackupVaultAccessPolicyRequest deleteBackupVaultAccessPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteBackupVaultAccessPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteBackupVaultAccessPolicyRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteBackupVaultAccessPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteBackupVaultAccessPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteBackupVaultAccessPolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes Backup Vault Lock from a backup vault specified by a backup vault name.
*
*
* If the Vault Lock configuration is immutable, then you cannot delete Vault Lock using API operations, and you
* will receive an InvalidRequestException
if you attempt to do so. For more information, see Vault Lock in the Backup
* Developer Guide.
*
*
* @param deleteBackupVaultLockConfigurationRequest
* @return Result of the DeleteBackupVaultLockConfiguration operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws InvalidRequestException
* Indicates that something is wrong with the input to the request. For example, a parameter is of the wrong
* type.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.DeleteBackupVaultLockConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteBackupVaultLockConfigurationResult deleteBackupVaultLockConfiguration(DeleteBackupVaultLockConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDeleteBackupVaultLockConfiguration(request);
}
@SdkInternalApi
final DeleteBackupVaultLockConfigurationResult executeDeleteBackupVaultLockConfiguration(
DeleteBackupVaultLockConfigurationRequest deleteBackupVaultLockConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteBackupVaultLockConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteBackupVaultLockConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteBackupVaultLockConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteBackupVaultLockConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteBackupVaultLockConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes event notifications for the specified backup vault.
*
*
* @param deleteBackupVaultNotificationsRequest
* @return Result of the DeleteBackupVaultNotifications operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.DeleteBackupVaultNotifications
* @see AWS API Documentation
*/
@Override
public DeleteBackupVaultNotificationsResult deleteBackupVaultNotifications(DeleteBackupVaultNotificationsRequest request) {
request = beforeClientExecution(request);
return executeDeleteBackupVaultNotifications(request);
}
@SdkInternalApi
final DeleteBackupVaultNotificationsResult executeDeleteBackupVaultNotifications(DeleteBackupVaultNotificationsRequest deleteBackupVaultNotificationsRequest) {
ExecutionContext executionContext = createExecutionContext(deleteBackupVaultNotificationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteBackupVaultNotificationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteBackupVaultNotificationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteBackupVaultNotifications");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteBackupVaultNotificationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the framework specified by a framework name.
*
*
* @param deleteFrameworkRequest
* @return Result of the DeleteFramework operation returned by the service.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws ConflictException
* Backup can't perform the action that you requested until it finishes performing a previous action. Try
* again later.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @sample AWSBackup.DeleteFramework
* @see AWS API
* Documentation
*/
@Override
public DeleteFrameworkResult deleteFramework(DeleteFrameworkRequest request) {
request = beforeClientExecution(request);
return executeDeleteFramework(request);
}
@SdkInternalApi
final DeleteFrameworkResult executeDeleteFramework(DeleteFrameworkRequest deleteFrameworkRequest) {
ExecutionContext executionContext = createExecutionContext(deleteFrameworkRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteFrameworkRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteFrameworkRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteFramework");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteFrameworkResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the recovery point specified by a recovery point ID.
*
*
* If the recovery point ID belongs to a continuous backup, calling this endpoint deletes the existing continuous
* backup and stops future continuous backup.
*
*
* When an IAM role's permissions are insufficient to call this API, the service sends back an HTTP 200 response
* with an empty HTTP body, but the recovery point is not deleted. Instead, it enters an EXPIRED
state.
*
*
* EXPIRED
recovery points can be deleted with this API once the IAM role has the
* iam:CreateServiceLinkedRole
action. To learn more about adding this role, see
* Troubleshooting manual deletions.
*
*
* If the user or role is deleted or the permission within the role is removed, the deletion will not be successful
* and will enter an EXPIRED
state.
*
*
* @param deleteRecoveryPointRequest
* @return Result of the DeleteRecoveryPoint operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws InvalidResourceStateException
* Backup is already performing an action on this recovery point. It can't perform the action you requested
* until the first action finishes. Try again later.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws InvalidRequestException
* Indicates that something is wrong with the input to the request. For example, a parameter is of the wrong
* type.
* @sample AWSBackup.DeleteRecoveryPoint
* @see AWS API
* Documentation
*/
@Override
public DeleteRecoveryPointResult deleteRecoveryPoint(DeleteRecoveryPointRequest request) {
request = beforeClientExecution(request);
return executeDeleteRecoveryPoint(request);
}
@SdkInternalApi
final DeleteRecoveryPointResult executeDeleteRecoveryPoint(DeleteRecoveryPointRequest deleteRecoveryPointRequest) {
ExecutionContext executionContext = createExecutionContext(deleteRecoveryPointRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteRecoveryPointRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteRecoveryPointRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteRecoveryPoint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteRecoveryPointResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the report plan specified by a report plan name.
*
*
* @param deleteReportPlanRequest
* @return Result of the DeleteReportPlan operation returned by the service.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws ConflictException
* Backup can't perform the action that you requested until it finishes performing a previous action. Try
* again later.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @sample AWSBackup.DeleteReportPlan
* @see AWS API
* Documentation
*/
@Override
public DeleteReportPlanResult deleteReportPlan(DeleteReportPlanRequest request) {
request = beforeClientExecution(request);
return executeDeleteReportPlan(request);
}
@SdkInternalApi
final DeleteReportPlanResult executeDeleteReportPlan(DeleteReportPlanRequest deleteReportPlanRequest) {
ExecutionContext executionContext = createExecutionContext(deleteReportPlanRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteReportPlanRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteReportPlanRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteReportPlan");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteReportPlanResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This request deletes the specified restore testing plan.
*
*
* Deletion can only successfully occur if all associated restore testing selections are deleted first.
*
*
* @param deleteRestoreTestingPlanRequest
* @return Result of the DeleteRestoreTestingPlan operation returned by the service.
* @throws InvalidRequestException
* Indicates that something is wrong with the input to the request. For example, a parameter is of the wrong
* type.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.DeleteRestoreTestingPlan
* @see AWS API Documentation
*/
@Override
public DeleteRestoreTestingPlanResult deleteRestoreTestingPlan(DeleteRestoreTestingPlanRequest request) {
request = beforeClientExecution(request);
return executeDeleteRestoreTestingPlan(request);
}
@SdkInternalApi
final DeleteRestoreTestingPlanResult executeDeleteRestoreTestingPlan(DeleteRestoreTestingPlanRequest deleteRestoreTestingPlanRequest) {
ExecutionContext executionContext = createExecutionContext(deleteRestoreTestingPlanRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteRestoreTestingPlanRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteRestoreTestingPlanRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteRestoreTestingPlan");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteRestoreTestingPlanResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Input the Restore Testing Plan name and Restore Testing Selection name.
*
*
* All testing selections associated with a restore testing plan must be deleted before the restore testing plan can
* be deleted.
*
*
* @param deleteRestoreTestingSelectionRequest
* @return Result of the DeleteRestoreTestingSelection operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.DeleteRestoreTestingSelection
* @see AWS API Documentation
*/
@Override
public DeleteRestoreTestingSelectionResult deleteRestoreTestingSelection(DeleteRestoreTestingSelectionRequest request) {
request = beforeClientExecution(request);
return executeDeleteRestoreTestingSelection(request);
}
@SdkInternalApi
final DeleteRestoreTestingSelectionResult executeDeleteRestoreTestingSelection(DeleteRestoreTestingSelectionRequest deleteRestoreTestingSelectionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteRestoreTestingSelectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteRestoreTestingSelectionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteRestoreTestingSelectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteRestoreTestingSelection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteRestoreTestingSelectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns backup job details for the specified BackupJobId
.
*
*
* @param describeBackupJobRequest
* @return Result of the DescribeBackupJob operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws DependencyFailureException
* A dependent Amazon Web Services service or resource returned an error to the Backup service, and the
* action cannot be completed.
* @sample AWSBackup.DescribeBackupJob
* @see AWS API
* Documentation
*/
@Override
public DescribeBackupJobResult describeBackupJob(DescribeBackupJobRequest request) {
request = beforeClientExecution(request);
return executeDescribeBackupJob(request);
}
@SdkInternalApi
final DescribeBackupJobResult executeDescribeBackupJob(DescribeBackupJobRequest describeBackupJobRequest) {
ExecutionContext executionContext = createExecutionContext(describeBackupJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeBackupJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeBackupJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeBackupJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeBackupJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns metadata about a backup vault specified by its name.
*
*
* @param describeBackupVaultRequest
* @return Result of the DescribeBackupVault operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.DescribeBackupVault
* @see AWS API
* Documentation
*/
@Override
public DescribeBackupVaultResult describeBackupVault(DescribeBackupVaultRequest request) {
request = beforeClientExecution(request);
return executeDescribeBackupVault(request);
}
@SdkInternalApi
final DescribeBackupVaultResult executeDescribeBackupVault(DescribeBackupVaultRequest describeBackupVaultRequest) {
ExecutionContext executionContext = createExecutionContext(describeBackupVaultRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeBackupVaultRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeBackupVaultRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeBackupVault");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeBackupVaultResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns metadata associated with creating a copy of a resource.
*
*
* @param describeCopyJobRequest
* @return Result of the DescribeCopyJob operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.DescribeCopyJob
* @see AWS API
* Documentation
*/
@Override
public DescribeCopyJobResult describeCopyJob(DescribeCopyJobRequest request) {
request = beforeClientExecution(request);
return executeDescribeCopyJob(request);
}
@SdkInternalApi
final DescribeCopyJobResult executeDescribeCopyJob(DescribeCopyJobRequest describeCopyJobRequest) {
ExecutionContext executionContext = createExecutionContext(describeCopyJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeCopyJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeCopyJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeCopyJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeCopyJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the framework details for the specified FrameworkName
.
*
*
* @param describeFrameworkRequest
* @return Result of the DescribeFramework operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.DescribeFramework
* @see AWS API
* Documentation
*/
@Override
public DescribeFrameworkResult describeFramework(DescribeFrameworkRequest request) {
request = beforeClientExecution(request);
return executeDescribeFramework(request);
}
@SdkInternalApi
final DescribeFrameworkResult executeDescribeFramework(DescribeFrameworkRequest describeFrameworkRequest) {
ExecutionContext executionContext = createExecutionContext(describeFrameworkRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeFrameworkRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeFrameworkRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeFramework");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeFrameworkResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes whether the Amazon Web Services account is opted in to cross-account backup. Returns an error if the
* account is not a member of an Organizations organization. Example:
* describe-global-settings --region us-west-2
*
*
* @param describeGlobalSettingsRequest
* @return Result of the DescribeGlobalSettings operation returned by the service.
* @throws InvalidRequestException
* Indicates that something is wrong with the input to the request. For example, a parameter is of the wrong
* type.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.DescribeGlobalSettings
* @see AWS
* API Documentation
*/
@Override
public DescribeGlobalSettingsResult describeGlobalSettings(DescribeGlobalSettingsRequest request) {
request = beforeClientExecution(request);
return executeDescribeGlobalSettings(request);
}
@SdkInternalApi
final DescribeGlobalSettingsResult executeDescribeGlobalSettings(DescribeGlobalSettingsRequest describeGlobalSettingsRequest) {
ExecutionContext executionContext = createExecutionContext(describeGlobalSettingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeGlobalSettingsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeGlobalSettingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeGlobalSettings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeGlobalSettingsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about a saved resource, including the last time it was backed up, its Amazon Resource Name
* (ARN), and the Amazon Web Services service type of the saved resource.
*
*
* @param describeProtectedResourceRequest
* @return Result of the DescribeProtectedResource operation returned by the service.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @sample AWSBackup.DescribeProtectedResource
* @see AWS API Documentation
*/
@Override
public DescribeProtectedResourceResult describeProtectedResource(DescribeProtectedResourceRequest request) {
request = beforeClientExecution(request);
return executeDescribeProtectedResource(request);
}
@SdkInternalApi
final DescribeProtectedResourceResult executeDescribeProtectedResource(DescribeProtectedResourceRequest describeProtectedResourceRequest) {
ExecutionContext executionContext = createExecutionContext(describeProtectedResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeProtectedResourceRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeProtectedResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeProtectedResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeProtectedResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns metadata associated with a recovery point, including ID, status, encryption, and lifecycle.
*
*
* @param describeRecoveryPointRequest
* @return Result of the DescribeRecoveryPoint operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.DescribeRecoveryPoint
* @see AWS
* API Documentation
*/
@Override
public DescribeRecoveryPointResult describeRecoveryPoint(DescribeRecoveryPointRequest request) {
request = beforeClientExecution(request);
return executeDescribeRecoveryPoint(request);
}
@SdkInternalApi
final DescribeRecoveryPointResult executeDescribeRecoveryPoint(DescribeRecoveryPointRequest describeRecoveryPointRequest) {
ExecutionContext executionContext = createExecutionContext(describeRecoveryPointRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeRecoveryPointRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeRecoveryPointRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeRecoveryPoint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeRecoveryPointResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the current service opt-in settings for the Region. If service opt-in is enabled for a service, Backup
* tries to protect that service's resources in this Region, when the resource is included in an on-demand backup or
* scheduled backup plan. Otherwise, Backup does not try to protect that service's resources in this Region.
*
*
* @param describeRegionSettingsRequest
* @return Result of the DescribeRegionSettings operation returned by the service.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.DescribeRegionSettings
* @see AWS
* API Documentation
*/
@Override
public DescribeRegionSettingsResult describeRegionSettings(DescribeRegionSettingsRequest request) {
request = beforeClientExecution(request);
return executeDescribeRegionSettings(request);
}
@SdkInternalApi
final DescribeRegionSettingsResult executeDescribeRegionSettings(DescribeRegionSettingsRequest describeRegionSettingsRequest) {
ExecutionContext executionContext = createExecutionContext(describeRegionSettingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeRegionSettingsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeRegionSettingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeRegionSettings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeRegionSettingsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the details associated with creating a report as specified by its ReportJobId
.
*
*
* @param describeReportJobRequest
* @return Result of the DescribeReportJob operation returned by the service.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @sample AWSBackup.DescribeReportJob
* @see AWS API
* Documentation
*/
@Override
public DescribeReportJobResult describeReportJob(DescribeReportJobRequest request) {
request = beforeClientExecution(request);
return executeDescribeReportJob(request);
}
@SdkInternalApi
final DescribeReportJobResult executeDescribeReportJob(DescribeReportJobRequest describeReportJobRequest) {
ExecutionContext executionContext = createExecutionContext(describeReportJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeReportJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeReportJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeReportJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeReportJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of all report plans for an Amazon Web Services account and Amazon Web Services Region.
*
*
* @param describeReportPlanRequest
* @return Result of the DescribeReportPlan operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.DescribeReportPlan
* @see AWS API
* Documentation
*/
@Override
public DescribeReportPlanResult describeReportPlan(DescribeReportPlanRequest request) {
request = beforeClientExecution(request);
return executeDescribeReportPlan(request);
}
@SdkInternalApi
final DescribeReportPlanResult executeDescribeReportPlan(DescribeReportPlanRequest describeReportPlanRequest) {
ExecutionContext executionContext = createExecutionContext(describeReportPlanRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeReportPlanRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeReportPlanRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeReportPlan");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeReportPlanResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns metadata associated with a restore job that is specified by a job ID.
*
*
* @param describeRestoreJobRequest
* @return Result of the DescribeRestoreJob operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws DependencyFailureException
* A dependent Amazon Web Services service or resource returned an error to the Backup service, and the
* action cannot be completed.
* @sample AWSBackup.DescribeRestoreJob
* @see AWS API
* Documentation
*/
@Override
public DescribeRestoreJobResult describeRestoreJob(DescribeRestoreJobRequest request) {
request = beforeClientExecution(request);
return executeDescribeRestoreJob(request);
}
@SdkInternalApi
final DescribeRestoreJobResult executeDescribeRestoreJob(DescribeRestoreJobRequest describeRestoreJobRequest) {
ExecutionContext executionContext = createExecutionContext(describeRestoreJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeRestoreJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeRestoreJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeRestoreJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeRestoreJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified continuous backup recovery point from Backup and releases control of that continuous backup
* to the source service, such as Amazon RDS. The source service will continue to create and retain continuous
* backups using the lifecycle that you specified in your original backup plan.
*
*
* Does not support snapshot backup recovery points.
*
*
* @param disassociateRecoveryPointRequest
* @return Result of the DisassociateRecoveryPoint operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws InvalidResourceStateException
* Backup is already performing an action on this recovery point. It can't perform the action you requested
* until the first action finishes. Try again later.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws InvalidRequestException
* Indicates that something is wrong with the input to the request. For example, a parameter is of the wrong
* type.
* @sample AWSBackup.DisassociateRecoveryPoint
* @see AWS API Documentation
*/
@Override
public DisassociateRecoveryPointResult disassociateRecoveryPoint(DisassociateRecoveryPointRequest request) {
request = beforeClientExecution(request);
return executeDisassociateRecoveryPoint(request);
}
@SdkInternalApi
final DisassociateRecoveryPointResult executeDisassociateRecoveryPoint(DisassociateRecoveryPointRequest disassociateRecoveryPointRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateRecoveryPointRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateRecoveryPointRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateRecoveryPointRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateRecoveryPoint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateRecoveryPointResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This action to a specific child (nested) recovery point removes the relationship between the specified recovery
* point and its parent (composite) recovery point.
*
*
* @param disassociateRecoveryPointFromParentRequest
* @return Result of the DisassociateRecoveryPointFromParent operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws InvalidRequestException
* Indicates that something is wrong with the input to the request. For example, a parameter is of the wrong
* type.
* @sample AWSBackup.DisassociateRecoveryPointFromParent
* @see AWS API Documentation
*/
@Override
public DisassociateRecoveryPointFromParentResult disassociateRecoveryPointFromParent(DisassociateRecoveryPointFromParentRequest request) {
request = beforeClientExecution(request);
return executeDisassociateRecoveryPointFromParent(request);
}
@SdkInternalApi
final DisassociateRecoveryPointFromParentResult executeDisassociateRecoveryPointFromParent(
DisassociateRecoveryPointFromParentRequest disassociateRecoveryPointFromParentRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateRecoveryPointFromParentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateRecoveryPointFromParentRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateRecoveryPointFromParentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateRecoveryPointFromParent");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateRecoveryPointFromParentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the backup plan that is specified by the plan ID as a backup template.
*
*
* @param exportBackupPlanTemplateRequest
* @return Result of the ExportBackupPlanTemplate operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @sample AWSBackup.ExportBackupPlanTemplate
* @see AWS API Documentation
*/
@Override
public ExportBackupPlanTemplateResult exportBackupPlanTemplate(ExportBackupPlanTemplateRequest request) {
request = beforeClientExecution(request);
return executeExportBackupPlanTemplate(request);
}
@SdkInternalApi
final ExportBackupPlanTemplateResult executeExportBackupPlanTemplate(ExportBackupPlanTemplateRequest exportBackupPlanTemplateRequest) {
ExecutionContext executionContext = createExecutionContext(exportBackupPlanTemplateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ExportBackupPlanTemplateRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(exportBackupPlanTemplateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ExportBackupPlanTemplate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ExportBackupPlanTemplateResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns BackupPlan
details for the specified BackupPlanId
. The details are the body of
* a backup plan in JSON format, in addition to plan metadata.
*
*
* @param getBackupPlanRequest
* @return Result of the GetBackupPlan operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.GetBackupPlan
* @see AWS API
* Documentation
*/
@Override
public GetBackupPlanResult getBackupPlan(GetBackupPlanRequest request) {
request = beforeClientExecution(request);
return executeGetBackupPlan(request);
}
@SdkInternalApi
final GetBackupPlanResult executeGetBackupPlan(GetBackupPlanRequest getBackupPlanRequest) {
ExecutionContext executionContext = createExecutionContext(getBackupPlanRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetBackupPlanRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getBackupPlanRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetBackupPlan");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetBackupPlanResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a valid JSON document specifying a backup plan or an error.
*
*
* @param getBackupPlanFromJSONRequest
* @return Result of the GetBackupPlanFromJSON operation returned by the service.
* @throws LimitExceededException
* A limit in the request has been exceeded; for example, a maximum number of items allowed in a request.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws InvalidRequestException
* Indicates that something is wrong with the input to the request. For example, a parameter is of the wrong
* type.
* @sample AWSBackup.GetBackupPlanFromJSON
* @see AWS
* API Documentation
*/
@Override
public GetBackupPlanFromJSONResult getBackupPlanFromJSON(GetBackupPlanFromJSONRequest request) {
request = beforeClientExecution(request);
return executeGetBackupPlanFromJSON(request);
}
@SdkInternalApi
final GetBackupPlanFromJSONResult executeGetBackupPlanFromJSON(GetBackupPlanFromJSONRequest getBackupPlanFromJSONRequest) {
ExecutionContext executionContext = createExecutionContext(getBackupPlanFromJSONRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetBackupPlanFromJSONRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getBackupPlanFromJSONRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetBackupPlanFromJSON");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetBackupPlanFromJSONResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the template specified by its templateId
as a backup plan.
*
*
* @param getBackupPlanFromTemplateRequest
* @return Result of the GetBackupPlanFromTemplate operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @sample AWSBackup.GetBackupPlanFromTemplate
* @see AWS API Documentation
*/
@Override
public GetBackupPlanFromTemplateResult getBackupPlanFromTemplate(GetBackupPlanFromTemplateRequest request) {
request = beforeClientExecution(request);
return executeGetBackupPlanFromTemplate(request);
}
@SdkInternalApi
final GetBackupPlanFromTemplateResult executeGetBackupPlanFromTemplate(GetBackupPlanFromTemplateRequest getBackupPlanFromTemplateRequest) {
ExecutionContext executionContext = createExecutionContext(getBackupPlanFromTemplateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetBackupPlanFromTemplateRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getBackupPlanFromTemplateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetBackupPlanFromTemplate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetBackupPlanFromTemplateResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns selection metadata and a document in JSON format that specifies a list of resources that are associated
* with a backup plan.
*
*
* @param getBackupSelectionRequest
* @return Result of the GetBackupSelection operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.GetBackupSelection
* @see AWS API
* Documentation
*/
@Override
public GetBackupSelectionResult getBackupSelection(GetBackupSelectionRequest request) {
request = beforeClientExecution(request);
return executeGetBackupSelection(request);
}
@SdkInternalApi
final GetBackupSelectionResult executeGetBackupSelection(GetBackupSelectionRequest getBackupSelectionRequest) {
ExecutionContext executionContext = createExecutionContext(getBackupSelectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetBackupSelectionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getBackupSelectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetBackupSelection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetBackupSelectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the access policy document that is associated with the named backup vault.
*
*
* @param getBackupVaultAccessPolicyRequest
* @return Result of the GetBackupVaultAccessPolicy operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.GetBackupVaultAccessPolicy
* @see AWS API Documentation
*/
@Override
public GetBackupVaultAccessPolicyResult getBackupVaultAccessPolicy(GetBackupVaultAccessPolicyRequest request) {
request = beforeClientExecution(request);
return executeGetBackupVaultAccessPolicy(request);
}
@SdkInternalApi
final GetBackupVaultAccessPolicyResult executeGetBackupVaultAccessPolicy(GetBackupVaultAccessPolicyRequest getBackupVaultAccessPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getBackupVaultAccessPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetBackupVaultAccessPolicyRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getBackupVaultAccessPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetBackupVaultAccessPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetBackupVaultAccessPolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns event notifications for the specified backup vault.
*
*
* @param getBackupVaultNotificationsRequest
* @return Result of the GetBackupVaultNotifications operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.GetBackupVaultNotifications
* @see AWS API Documentation
*/
@Override
public GetBackupVaultNotificationsResult getBackupVaultNotifications(GetBackupVaultNotificationsRequest request) {
request = beforeClientExecution(request);
return executeGetBackupVaultNotifications(request);
}
@SdkInternalApi
final GetBackupVaultNotificationsResult executeGetBackupVaultNotifications(GetBackupVaultNotificationsRequest getBackupVaultNotificationsRequest) {
ExecutionContext executionContext = createExecutionContext(getBackupVaultNotificationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetBackupVaultNotificationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getBackupVaultNotificationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetBackupVaultNotifications");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetBackupVaultNotificationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This action returns details for a specified legal hold. The details are the body of a legal hold in JSON format,
* in addition to metadata.
*
*
* @param getLegalHoldRequest
* @return Result of the GetLegalHold operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @sample AWSBackup.GetLegalHold
* @see AWS API
* Documentation
*/
@Override
public GetLegalHoldResult getLegalHold(GetLegalHoldRequest request) {
request = beforeClientExecution(request);
return executeGetLegalHold(request);
}
@SdkInternalApi
final GetLegalHoldResult executeGetLegalHold(GetLegalHoldRequest getLegalHoldRequest) {
ExecutionContext executionContext = createExecutionContext(getLegalHoldRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetLegalHoldRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getLegalHoldRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetLegalHold");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetLegalHoldResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a set of metadata key-value pairs that were used to create the backup.
*
*
* @param getRecoveryPointRestoreMetadataRequest
* @return Result of the GetRecoveryPointRestoreMetadata operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.GetRecoveryPointRestoreMetadata
* @see AWS API Documentation
*/
@Override
public GetRecoveryPointRestoreMetadataResult getRecoveryPointRestoreMetadata(GetRecoveryPointRestoreMetadataRequest request) {
request = beforeClientExecution(request);
return executeGetRecoveryPointRestoreMetadata(request);
}
@SdkInternalApi
final GetRecoveryPointRestoreMetadataResult executeGetRecoveryPointRestoreMetadata(
GetRecoveryPointRestoreMetadataRequest getRecoveryPointRestoreMetadataRequest) {
ExecutionContext executionContext = createExecutionContext(getRecoveryPointRestoreMetadataRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetRecoveryPointRestoreMetadataRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getRecoveryPointRestoreMetadataRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetRecoveryPointRestoreMetadata");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetRecoveryPointRestoreMetadataResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This request returns the metadata for the specified restore job.
*
*
* @param getRestoreJobMetadataRequest
* @return Result of the GetRestoreJobMetadata operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.GetRestoreJobMetadata
* @see AWS
* API Documentation
*/
@Override
public GetRestoreJobMetadataResult getRestoreJobMetadata(GetRestoreJobMetadataRequest request) {
request = beforeClientExecution(request);
return executeGetRestoreJobMetadata(request);
}
@SdkInternalApi
final GetRestoreJobMetadataResult executeGetRestoreJobMetadata(GetRestoreJobMetadataRequest getRestoreJobMetadataRequest) {
ExecutionContext executionContext = createExecutionContext(getRestoreJobMetadataRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetRestoreJobMetadataRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getRestoreJobMetadataRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetRestoreJobMetadata");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetRestoreJobMetadataResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This request returns the minimal required set of metadata needed to start a restore job with secure default
* settings. BackupVaultName
and RecoveryPointArn
are required parameters.
* BackupVaultAccountId
is an optional parameter.
*
*
* @param getRestoreTestingInferredMetadataRequest
* @return Result of the GetRestoreTestingInferredMetadata operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.GetRestoreTestingInferredMetadata
* @see AWS API Documentation
*/
@Override
public GetRestoreTestingInferredMetadataResult getRestoreTestingInferredMetadata(GetRestoreTestingInferredMetadataRequest request) {
request = beforeClientExecution(request);
return executeGetRestoreTestingInferredMetadata(request);
}
@SdkInternalApi
final GetRestoreTestingInferredMetadataResult executeGetRestoreTestingInferredMetadata(
GetRestoreTestingInferredMetadataRequest getRestoreTestingInferredMetadataRequest) {
ExecutionContext executionContext = createExecutionContext(getRestoreTestingInferredMetadataRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetRestoreTestingInferredMetadataRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getRestoreTestingInferredMetadataRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetRestoreTestingInferredMetadata");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetRestoreTestingInferredMetadataResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns RestoreTestingPlan
details for the specified RestoreTestingPlanName
. The
* details are the body of a restore testing plan in JSON format, in addition to plan metadata.
*
*
* @param getRestoreTestingPlanRequest
* @return Result of the GetRestoreTestingPlan operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.GetRestoreTestingPlan
* @see AWS
* API Documentation
*/
@Override
public GetRestoreTestingPlanResult getRestoreTestingPlan(GetRestoreTestingPlanRequest request) {
request = beforeClientExecution(request);
return executeGetRestoreTestingPlan(request);
}
@SdkInternalApi
final GetRestoreTestingPlanResult executeGetRestoreTestingPlan(GetRestoreTestingPlanRequest getRestoreTestingPlanRequest) {
ExecutionContext executionContext = createExecutionContext(getRestoreTestingPlanRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetRestoreTestingPlanRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getRestoreTestingPlanRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetRestoreTestingPlan");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetRestoreTestingPlanResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns RestoreTestingSelection, which displays resources and elements of the restore testing plan.
*
*
* @param getRestoreTestingSelectionRequest
* @return Result of the GetRestoreTestingSelection operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.GetRestoreTestingSelection
* @see AWS API Documentation
*/
@Override
public GetRestoreTestingSelectionResult getRestoreTestingSelection(GetRestoreTestingSelectionRequest request) {
request = beforeClientExecution(request);
return executeGetRestoreTestingSelection(request);
}
@SdkInternalApi
final GetRestoreTestingSelectionResult executeGetRestoreTestingSelection(GetRestoreTestingSelectionRequest getRestoreTestingSelectionRequest) {
ExecutionContext executionContext = createExecutionContext(getRestoreTestingSelectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetRestoreTestingSelectionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getRestoreTestingSelectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetRestoreTestingSelection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetRestoreTestingSelectionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the Amazon Web Services resource types supported by Backup.
*
*
* @param getSupportedResourceTypesRequest
* @return Result of the GetSupportedResourceTypes operation returned by the service.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.GetSupportedResourceTypes
* @see AWS API Documentation
*/
@Override
public GetSupportedResourceTypesResult getSupportedResourceTypes(GetSupportedResourceTypesRequest request) {
request = beforeClientExecution(request);
return executeGetSupportedResourceTypes(request);
}
@SdkInternalApi
final GetSupportedResourceTypesResult executeGetSupportedResourceTypes(GetSupportedResourceTypesRequest getSupportedResourceTypesRequest) {
ExecutionContext executionContext = createExecutionContext(getSupportedResourceTypesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSupportedResourceTypesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getSupportedResourceTypesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSupportedResourceTypes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetSupportedResourceTypesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This is a request for a summary of backup jobs created or running within the most recent 30 days. You can include
* parameters AccountID, State, ResourceType, MessageCategory, AggregationPeriod, MaxResults, or NextToken to filter
* results.
*
*
* This request returns a summary that contains Region, Account, State, ResourceType, MessageCategory, StartTime,
* EndTime, and Count of included jobs.
*
*
* @param listBackupJobSummariesRequest
* @return Result of the ListBackupJobSummaries operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListBackupJobSummaries
* @see AWS
* API Documentation
*/
@Override
public ListBackupJobSummariesResult listBackupJobSummaries(ListBackupJobSummariesRequest request) {
request = beforeClientExecution(request);
return executeListBackupJobSummaries(request);
}
@SdkInternalApi
final ListBackupJobSummariesResult executeListBackupJobSummaries(ListBackupJobSummariesRequest listBackupJobSummariesRequest) {
ExecutionContext executionContext = createExecutionContext(listBackupJobSummariesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListBackupJobSummariesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listBackupJobSummariesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListBackupJobSummaries");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListBackupJobSummariesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of existing backup jobs for an authenticated account for the last 30 days. For a longer period of
* time, consider using these monitoring tools.
*
*
* @param listBackupJobsRequest
* @return Result of the ListBackupJobs operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListBackupJobs
* @see AWS API
* Documentation
*/
@Override
public ListBackupJobsResult listBackupJobs(ListBackupJobsRequest request) {
request = beforeClientExecution(request);
return executeListBackupJobs(request);
}
@SdkInternalApi
final ListBackupJobsResult executeListBackupJobs(ListBackupJobsRequest listBackupJobsRequest) {
ExecutionContext executionContext = createExecutionContext(listBackupJobsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListBackupJobsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listBackupJobsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListBackupJobs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListBackupJobsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns metadata of your saved backup plan templates, including the template ID, name, and the creation and
* deletion dates.
*
*
* @param listBackupPlanTemplatesRequest
* @return Result of the ListBackupPlanTemplates operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @sample AWSBackup.ListBackupPlanTemplates
* @see AWS
* API Documentation
*/
@Override
public ListBackupPlanTemplatesResult listBackupPlanTemplates(ListBackupPlanTemplatesRequest request) {
request = beforeClientExecution(request);
return executeListBackupPlanTemplates(request);
}
@SdkInternalApi
final ListBackupPlanTemplatesResult executeListBackupPlanTemplates(ListBackupPlanTemplatesRequest listBackupPlanTemplatesRequest) {
ExecutionContext executionContext = createExecutionContext(listBackupPlanTemplatesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListBackupPlanTemplatesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listBackupPlanTemplatesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListBackupPlanTemplates");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListBackupPlanTemplatesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns version metadata of your backup plans, including Amazon Resource Names (ARNs), backup plan IDs, creation
* and deletion dates, plan names, and version IDs.
*
*
* @param listBackupPlanVersionsRequest
* @return Result of the ListBackupPlanVersions operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListBackupPlanVersions
* @see AWS
* API Documentation
*/
@Override
public ListBackupPlanVersionsResult listBackupPlanVersions(ListBackupPlanVersionsRequest request) {
request = beforeClientExecution(request);
return executeListBackupPlanVersions(request);
}
@SdkInternalApi
final ListBackupPlanVersionsResult executeListBackupPlanVersions(ListBackupPlanVersionsRequest listBackupPlanVersionsRequest) {
ExecutionContext executionContext = createExecutionContext(listBackupPlanVersionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListBackupPlanVersionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listBackupPlanVersionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListBackupPlanVersions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListBackupPlanVersionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of all active backup plans for an authenticated account. The list contains information such as
* Amazon Resource Names (ARNs), plan IDs, creation and deletion dates, version IDs, plan names, and creator request
* IDs.
*
*
* @param listBackupPlansRequest
* @return Result of the ListBackupPlans operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListBackupPlans
* @see AWS API
* Documentation
*/
@Override
public ListBackupPlansResult listBackupPlans(ListBackupPlansRequest request) {
request = beforeClientExecution(request);
return executeListBackupPlans(request);
}
@SdkInternalApi
final ListBackupPlansResult executeListBackupPlans(ListBackupPlansRequest listBackupPlansRequest) {
ExecutionContext executionContext = createExecutionContext(listBackupPlansRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListBackupPlansRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listBackupPlansRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListBackupPlans");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListBackupPlansResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns an array containing metadata of the resources associated with the target backup plan.
*
*
* @param listBackupSelectionsRequest
* @return Result of the ListBackupSelections operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListBackupSelections
* @see AWS
* API Documentation
*/
@Override
public ListBackupSelectionsResult listBackupSelections(ListBackupSelectionsRequest request) {
request = beforeClientExecution(request);
return executeListBackupSelections(request);
}
@SdkInternalApi
final ListBackupSelectionsResult executeListBackupSelections(ListBackupSelectionsRequest listBackupSelectionsRequest) {
ExecutionContext executionContext = createExecutionContext(listBackupSelectionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListBackupSelectionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listBackupSelectionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListBackupSelections");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListBackupSelectionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of recovery point storage containers along with information about them.
*
*
* @param listBackupVaultsRequest
* @return Result of the ListBackupVaults operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListBackupVaults
* @see AWS API
* Documentation
*/
@Override
public ListBackupVaultsResult listBackupVaults(ListBackupVaultsRequest request) {
request = beforeClientExecution(request);
return executeListBackupVaults(request);
}
@SdkInternalApi
final ListBackupVaultsResult executeListBackupVaults(ListBackupVaultsRequest listBackupVaultsRequest) {
ExecutionContext executionContext = createExecutionContext(listBackupVaultsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListBackupVaultsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listBackupVaultsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListBackupVaults");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListBackupVaultsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This request obtains a list of copy jobs created or running within the the most recent 30 days. You can include
* parameters AccountID, State, ResourceType, MessageCategory, AggregationPeriod, MaxResults, or NextToken to filter
* results.
*
*
* This request returns a summary that contains Region, Account, State, RestourceType, MessageCategory, StartTime,
* EndTime, and Count of included jobs.
*
*
* @param listCopyJobSummariesRequest
* @return Result of the ListCopyJobSummaries operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListCopyJobSummaries
* @see AWS
* API Documentation
*/
@Override
public ListCopyJobSummariesResult listCopyJobSummaries(ListCopyJobSummariesRequest request) {
request = beforeClientExecution(request);
return executeListCopyJobSummaries(request);
}
@SdkInternalApi
final ListCopyJobSummariesResult executeListCopyJobSummaries(ListCopyJobSummariesRequest listCopyJobSummariesRequest) {
ExecutionContext executionContext = createExecutionContext(listCopyJobSummariesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListCopyJobSummariesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listCopyJobSummariesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListCopyJobSummaries");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListCopyJobSummariesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns metadata about your copy jobs.
*
*
* @param listCopyJobsRequest
* @return Result of the ListCopyJobs operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListCopyJobs
* @see AWS API
* Documentation
*/
@Override
public ListCopyJobsResult listCopyJobs(ListCopyJobsRequest request) {
request = beforeClientExecution(request);
return executeListCopyJobs(request);
}
@SdkInternalApi
final ListCopyJobsResult executeListCopyJobs(ListCopyJobsRequest listCopyJobsRequest) {
ExecutionContext executionContext = createExecutionContext(listCopyJobsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListCopyJobsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listCopyJobsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListCopyJobs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListCopyJobsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of all frameworks for an Amazon Web Services account and Amazon Web Services Region.
*
*
* @param listFrameworksRequest
* @return Result of the ListFrameworks operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListFrameworks
* @see AWS API
* Documentation
*/
@Override
public ListFrameworksResult listFrameworks(ListFrameworksRequest request) {
request = beforeClientExecution(request);
return executeListFrameworks(request);
}
@SdkInternalApi
final ListFrameworksResult executeListFrameworks(ListFrameworksRequest listFrameworksRequest) {
ExecutionContext executionContext = createExecutionContext(listFrameworksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListFrameworksRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listFrameworksRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListFrameworks");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListFrameworksResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This action returns metadata about active and previous legal holds.
*
*
* @param listLegalHoldsRequest
* @return Result of the ListLegalHolds operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListLegalHolds
* @see AWS API
* Documentation
*/
@Override
public ListLegalHoldsResult listLegalHolds(ListLegalHoldsRequest request) {
request = beforeClientExecution(request);
return executeListLegalHolds(request);
}
@SdkInternalApi
final ListLegalHoldsResult executeListLegalHolds(ListLegalHoldsRequest listLegalHoldsRequest) {
ExecutionContext executionContext = createExecutionContext(listLegalHoldsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListLegalHoldsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listLegalHoldsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListLegalHolds");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListLegalHoldsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns an array of resources successfully backed up by Backup, including the time the resource was saved, an
* Amazon Resource Name (ARN) of the resource, and a resource type.
*
*
* @param listProtectedResourcesRequest
* @return Result of the ListProtectedResources operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListProtectedResources
* @see AWS
* API Documentation
*/
@Override
public ListProtectedResourcesResult listProtectedResources(ListProtectedResourcesRequest request) {
request = beforeClientExecution(request);
return executeListProtectedResources(request);
}
@SdkInternalApi
final ListProtectedResourcesResult executeListProtectedResources(ListProtectedResourcesRequest listProtectedResourcesRequest) {
ExecutionContext executionContext = createExecutionContext(listProtectedResourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListProtectedResourcesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listProtectedResourcesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListProtectedResources");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListProtectedResourcesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This request lists the protected resources corresponding to each backup vault.
*
*
* @param listProtectedResourcesByBackupVaultRequest
* @return Result of the ListProtectedResourcesByBackupVault operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListProtectedResourcesByBackupVault
* @see AWS API Documentation
*/
@Override
public ListProtectedResourcesByBackupVaultResult listProtectedResourcesByBackupVault(ListProtectedResourcesByBackupVaultRequest request) {
request = beforeClientExecution(request);
return executeListProtectedResourcesByBackupVault(request);
}
@SdkInternalApi
final ListProtectedResourcesByBackupVaultResult executeListProtectedResourcesByBackupVault(
ListProtectedResourcesByBackupVaultRequest listProtectedResourcesByBackupVaultRequest) {
ExecutionContext executionContext = createExecutionContext(listProtectedResourcesByBackupVaultRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListProtectedResourcesByBackupVaultRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listProtectedResourcesByBackupVaultRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListProtectedResourcesByBackupVault");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListProtectedResourcesByBackupVaultResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns detailed information about the recovery points stored in a backup vault.
*
*
* @param listRecoveryPointsByBackupVaultRequest
* @return Result of the ListRecoveryPointsByBackupVault operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListRecoveryPointsByBackupVault
* @see AWS API Documentation
*/
@Override
public ListRecoveryPointsByBackupVaultResult listRecoveryPointsByBackupVault(ListRecoveryPointsByBackupVaultRequest request) {
request = beforeClientExecution(request);
return executeListRecoveryPointsByBackupVault(request);
}
@SdkInternalApi
final ListRecoveryPointsByBackupVaultResult executeListRecoveryPointsByBackupVault(
ListRecoveryPointsByBackupVaultRequest listRecoveryPointsByBackupVaultRequest) {
ExecutionContext executionContext = createExecutionContext(listRecoveryPointsByBackupVaultRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListRecoveryPointsByBackupVaultRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listRecoveryPointsByBackupVaultRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListRecoveryPointsByBackupVault");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListRecoveryPointsByBackupVaultResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This action returns recovery point ARNs (Amazon Resource Names) of the specified legal hold.
*
*
* @param listRecoveryPointsByLegalHoldRequest
* @return Result of the ListRecoveryPointsByLegalHold operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListRecoveryPointsByLegalHold
* @see AWS API Documentation
*/
@Override
public ListRecoveryPointsByLegalHoldResult listRecoveryPointsByLegalHold(ListRecoveryPointsByLegalHoldRequest request) {
request = beforeClientExecution(request);
return executeListRecoveryPointsByLegalHold(request);
}
@SdkInternalApi
final ListRecoveryPointsByLegalHoldResult executeListRecoveryPointsByLegalHold(ListRecoveryPointsByLegalHoldRequest listRecoveryPointsByLegalHoldRequest) {
ExecutionContext executionContext = createExecutionContext(listRecoveryPointsByLegalHoldRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListRecoveryPointsByLegalHoldRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listRecoveryPointsByLegalHoldRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListRecoveryPointsByLegalHold");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListRecoveryPointsByLegalHoldResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns detailed information about all the recovery points of the type specified by a resource Amazon Resource
* Name (ARN).
*
*
*
* For Amazon EFS and Amazon EC2, this action only lists recovery points created by Backup.
*
*
*
* @param listRecoveryPointsByResourceRequest
* @return Result of the ListRecoveryPointsByResource operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListRecoveryPointsByResource
* @see AWS API Documentation
*/
@Override
public ListRecoveryPointsByResourceResult listRecoveryPointsByResource(ListRecoveryPointsByResourceRequest request) {
request = beforeClientExecution(request);
return executeListRecoveryPointsByResource(request);
}
@SdkInternalApi
final ListRecoveryPointsByResourceResult executeListRecoveryPointsByResource(ListRecoveryPointsByResourceRequest listRecoveryPointsByResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listRecoveryPointsByResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListRecoveryPointsByResourceRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listRecoveryPointsByResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListRecoveryPointsByResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListRecoveryPointsByResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns details about your report jobs.
*
*
* @param listReportJobsRequest
* @return Result of the ListReportJobs operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @sample AWSBackup.ListReportJobs
* @see AWS API
* Documentation
*/
@Override
public ListReportJobsResult listReportJobs(ListReportJobsRequest request) {
request = beforeClientExecution(request);
return executeListReportJobs(request);
}
@SdkInternalApi
final ListReportJobsResult executeListReportJobs(ListReportJobsRequest listReportJobsRequest) {
ExecutionContext executionContext = createExecutionContext(listReportJobsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListReportJobsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listReportJobsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListReportJobs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListReportJobsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of your report plans. For detailed information about a single report plan, use
* DescribeReportPlan
.
*
*
* @param listReportPlansRequest
* @return Result of the ListReportPlans operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListReportPlans
* @see AWS API
* Documentation
*/
@Override
public ListReportPlansResult listReportPlans(ListReportPlansRequest request) {
request = beforeClientExecution(request);
return executeListReportPlans(request);
}
@SdkInternalApi
final ListReportPlansResult executeListReportPlans(ListReportPlansRequest listReportPlansRequest) {
ExecutionContext executionContext = createExecutionContext(listReportPlansRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListReportPlansRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listReportPlansRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListReportPlans");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListReportPlansResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This request obtains a summary of restore jobs created or running within the the most recent 30 days. You can
* include parameters AccountID, State, ResourceType, AggregationPeriod, MaxResults, or NextToken to filter results.
*
*
* This request returns a summary that contains Region, Account, State, RestourceType, MessageCategory, StartTime,
* EndTime, and Count of included jobs.
*
*
* @param listRestoreJobSummariesRequest
* @return Result of the ListRestoreJobSummaries operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListRestoreJobSummaries
* @see AWS
* API Documentation
*/
@Override
public ListRestoreJobSummariesResult listRestoreJobSummaries(ListRestoreJobSummariesRequest request) {
request = beforeClientExecution(request);
return executeListRestoreJobSummaries(request);
}
@SdkInternalApi
final ListRestoreJobSummariesResult executeListRestoreJobSummaries(ListRestoreJobSummariesRequest listRestoreJobSummariesRequest) {
ExecutionContext executionContext = createExecutionContext(listRestoreJobSummariesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListRestoreJobSummariesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listRestoreJobSummariesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListRestoreJobSummaries");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListRestoreJobSummariesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of jobs that Backup initiated to restore a saved resource, including details about the recovery
* process.
*
*
* @param listRestoreJobsRequest
* @return Result of the ListRestoreJobs operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListRestoreJobs
* @see AWS API
* Documentation
*/
@Override
public ListRestoreJobsResult listRestoreJobs(ListRestoreJobsRequest request) {
request = beforeClientExecution(request);
return executeListRestoreJobs(request);
}
@SdkInternalApi
final ListRestoreJobsResult executeListRestoreJobs(ListRestoreJobsRequest listRestoreJobsRequest) {
ExecutionContext executionContext = createExecutionContext(listRestoreJobsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListRestoreJobsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listRestoreJobsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListRestoreJobs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListRestoreJobsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This returns restore jobs that contain the specified protected resource.
*
*
* You must include ResourceArn
. You can optionally include NextToken
,
* ByStatus
, MaxResults
, ByRecoveryPointCreationDateAfter
, and
* ByRecoveryPointCreationDateBefore
.
*
*
* @param listRestoreJobsByProtectedResourceRequest
* @return Result of the ListRestoreJobsByProtectedResource operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListRestoreJobsByProtectedResource
* @see AWS API Documentation
*/
@Override
public ListRestoreJobsByProtectedResourceResult listRestoreJobsByProtectedResource(ListRestoreJobsByProtectedResourceRequest request) {
request = beforeClientExecution(request);
return executeListRestoreJobsByProtectedResource(request);
}
@SdkInternalApi
final ListRestoreJobsByProtectedResourceResult executeListRestoreJobsByProtectedResource(
ListRestoreJobsByProtectedResourceRequest listRestoreJobsByProtectedResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listRestoreJobsByProtectedResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListRestoreJobsByProtectedResourceRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listRestoreJobsByProtectedResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListRestoreJobsByProtectedResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListRestoreJobsByProtectedResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of restore testing plans.
*
*
* @param listRestoreTestingPlansRequest
* @return Result of the ListRestoreTestingPlans operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListRestoreTestingPlans
* @see AWS
* API Documentation
*/
@Override
public ListRestoreTestingPlansResult listRestoreTestingPlans(ListRestoreTestingPlansRequest request) {
request = beforeClientExecution(request);
return executeListRestoreTestingPlans(request);
}
@SdkInternalApi
final ListRestoreTestingPlansResult executeListRestoreTestingPlans(ListRestoreTestingPlansRequest listRestoreTestingPlansRequest) {
ExecutionContext executionContext = createExecutionContext(listRestoreTestingPlansRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListRestoreTestingPlansRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listRestoreTestingPlansRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListRestoreTestingPlans");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListRestoreTestingPlansResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of restore testing selections. Can be filtered by MaxResults
and
* RestoreTestingPlanName
.
*
*
* @param listRestoreTestingSelectionsRequest
* @return Result of the ListRestoreTestingSelections operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListRestoreTestingSelections
* @see AWS API Documentation
*/
@Override
public ListRestoreTestingSelectionsResult listRestoreTestingSelections(ListRestoreTestingSelectionsRequest request) {
request = beforeClientExecution(request);
return executeListRestoreTestingSelections(request);
}
@SdkInternalApi
final ListRestoreTestingSelectionsResult executeListRestoreTestingSelections(ListRestoreTestingSelectionsRequest listRestoreTestingSelectionsRequest) {
ExecutionContext executionContext = createExecutionContext(listRestoreTestingSelectionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListRestoreTestingSelectionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listRestoreTestingSelectionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListRestoreTestingSelections");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListRestoreTestingSelectionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of key-value pairs assigned to a target recovery point, backup plan, or backup vault.
*
*
* ListTags
only works for resource types that support full Backup management of their backups. Those
* resource types are listed in the "Full Backup management" section of the Feature
* availability by resource table.
*
*
* @param listTagsRequest
* @return Result of the ListTags operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.ListTags
* @see AWS API
* Documentation
*/
@Override
public ListTagsResult listTags(ListTagsRequest request) {
request = beforeClientExecution(request);
return executeListTags(request);
}
@SdkInternalApi
final ListTagsResult executeListTags(ListTagsRequest listTagsRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTags");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Sets a resource-based policy that is used to manage access permissions on the target backup vault. Requires a
* backup vault name and an access policy document in JSON format.
*
*
* @param putBackupVaultAccessPolicyRequest
* @return Result of the PutBackupVaultAccessPolicy operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.PutBackupVaultAccessPolicy
* @see AWS API Documentation
*/
@Override
public PutBackupVaultAccessPolicyResult putBackupVaultAccessPolicy(PutBackupVaultAccessPolicyRequest request) {
request = beforeClientExecution(request);
return executePutBackupVaultAccessPolicy(request);
}
@SdkInternalApi
final PutBackupVaultAccessPolicyResult executePutBackupVaultAccessPolicy(PutBackupVaultAccessPolicyRequest putBackupVaultAccessPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(putBackupVaultAccessPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutBackupVaultAccessPolicyRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putBackupVaultAccessPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutBackupVaultAccessPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutBackupVaultAccessPolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Applies Backup Vault Lock to a backup vault, preventing attempts to delete any recovery point stored in or
* created in a backup vault. Vault Lock also prevents attempts to update the lifecycle policy that controls the
* retention period of any recovery point currently stored in a backup vault. If specified, Vault Lock enforces a
* minimum and maximum retention period for future backup and copy jobs that target a backup vault.
*
*
*
* Backup Vault Lock has been assessed by Cohasset Associates for use in environments that are subject to SEC 17a-4,
* CFTC, and FINRA regulations. For more information about how Backup Vault Lock relates to these regulations, see
* the Cohasset Associates Compliance Assessment.
*
*
*
* @param putBackupVaultLockConfigurationRequest
* @return Result of the PutBackupVaultLockConfiguration operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws InvalidRequestException
* Indicates that something is wrong with the input to the request. For example, a parameter is of the wrong
* type.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.PutBackupVaultLockConfiguration
* @see AWS API Documentation
*/
@Override
public PutBackupVaultLockConfigurationResult putBackupVaultLockConfiguration(PutBackupVaultLockConfigurationRequest request) {
request = beforeClientExecution(request);
return executePutBackupVaultLockConfiguration(request);
}
@SdkInternalApi
final PutBackupVaultLockConfigurationResult executePutBackupVaultLockConfiguration(
PutBackupVaultLockConfigurationRequest putBackupVaultLockConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(putBackupVaultLockConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutBackupVaultLockConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putBackupVaultLockConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutBackupVaultLockConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutBackupVaultLockConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Turns on notifications on a backup vault for the specified topic and events.
*
*
* @param putBackupVaultNotificationsRequest
* @return Result of the PutBackupVaultNotifications operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.PutBackupVaultNotifications
* @see AWS API Documentation
*/
@Override
public PutBackupVaultNotificationsResult putBackupVaultNotifications(PutBackupVaultNotificationsRequest request) {
request = beforeClientExecution(request);
return executePutBackupVaultNotifications(request);
}
@SdkInternalApi
final PutBackupVaultNotificationsResult executePutBackupVaultNotifications(PutBackupVaultNotificationsRequest putBackupVaultNotificationsRequest) {
ExecutionContext executionContext = createExecutionContext(putBackupVaultNotificationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutBackupVaultNotificationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putBackupVaultNotificationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutBackupVaultNotifications");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutBackupVaultNotificationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This request allows you to send your independent self-run restore test validation results.
* RestoreJobId
and ValidationStatus
are required. Optionally, you can input a
* ValidationStatusMessage
.
*
*
* @param putRestoreValidationResultRequest
* @return Result of the PutRestoreValidationResult operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws InvalidRequestException
* Indicates that something is wrong with the input to the request. For example, a parameter is of the wrong
* type.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.PutRestoreValidationResult
* @see AWS API Documentation
*/
@Override
public PutRestoreValidationResultResult putRestoreValidationResult(PutRestoreValidationResultRequest request) {
request = beforeClientExecution(request);
return executePutRestoreValidationResult(request);
}
@SdkInternalApi
final PutRestoreValidationResultResult executePutRestoreValidationResult(PutRestoreValidationResultRequest putRestoreValidationResultRequest) {
ExecutionContext executionContext = createExecutionContext(putRestoreValidationResultRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutRestoreValidationResultRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putRestoreValidationResultRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutRestoreValidationResult");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutRestoreValidationResultResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts an on-demand backup job for the specified resource.
*
*
* @param startBackupJobRequest
* @return Result of the StartBackupJob operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws InvalidRequestException
* Indicates that something is wrong with the input to the request. For example, a parameter is of the wrong
* type.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws LimitExceededException
* A limit in the request has been exceeded; for example, a maximum number of items allowed in a request.
* @sample AWSBackup.StartBackupJob
* @see AWS API
* Documentation
*/
@Override
public StartBackupJobResult startBackupJob(StartBackupJobRequest request) {
request = beforeClientExecution(request);
return executeStartBackupJob(request);
}
@SdkInternalApi
final StartBackupJobResult executeStartBackupJob(StartBackupJobRequest startBackupJobRequest) {
ExecutionContext executionContext = createExecutionContext(startBackupJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartBackupJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startBackupJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartBackupJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StartBackupJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts a job to create a one-time copy of the specified resource.
*
*
* Does not support continuous backups.
*
*
* @param startCopyJobRequest
* @return Result of the StartCopyJob operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws LimitExceededException
* A limit in the request has been exceeded; for example, a maximum number of items allowed in a request.
* @throws InvalidRequestException
* Indicates that something is wrong with the input to the request. For example, a parameter is of the wrong
* type.
* @sample AWSBackup.StartCopyJob
* @see AWS API
* Documentation
*/
@Override
public StartCopyJobResult startCopyJob(StartCopyJobRequest request) {
request = beforeClientExecution(request);
return executeStartCopyJob(request);
}
@SdkInternalApi
final StartCopyJobResult executeStartCopyJob(StartCopyJobRequest startCopyJobRequest) {
ExecutionContext executionContext = createExecutionContext(startCopyJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartCopyJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startCopyJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartCopyJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StartCopyJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts an on-demand report job for the specified report plan.
*
*
* @param startReportJobRequest
* @return Result of the StartReportJob operation returned by the service.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @sample AWSBackup.StartReportJob
* @see AWS API
* Documentation
*/
@Override
public StartReportJobResult startReportJob(StartReportJobRequest request) {
request = beforeClientExecution(request);
return executeStartReportJob(request);
}
@SdkInternalApi
final StartReportJobResult executeStartReportJob(StartReportJobRequest startReportJobRequest) {
ExecutionContext executionContext = createExecutionContext(startReportJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartReportJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startReportJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartReportJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StartReportJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Recovers the saved resource identified by an Amazon Resource Name (ARN).
*
*
* @param startRestoreJobRequest
* @return Result of the StartRestoreJob operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws InvalidRequestException
* Indicates that something is wrong with the input to the request. For example, a parameter is of the wrong
* type.
* @sample AWSBackup.StartRestoreJob
* @see AWS API
* Documentation
*/
@Override
public StartRestoreJobResult startRestoreJob(StartRestoreJobRequest request) {
request = beforeClientExecution(request);
return executeStartRestoreJob(request);
}
@SdkInternalApi
final StartRestoreJobResult executeStartRestoreJob(StartRestoreJobRequest startRestoreJobRequest) {
ExecutionContext executionContext = createExecutionContext(startRestoreJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartRestoreJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startRestoreJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartRestoreJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StartRestoreJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Attempts to cancel a job to create a one-time backup of a resource.
*
*
* This action is not supported for the following services: Amazon FSx for Windows File Server, Amazon FSx for
* Lustre, Amazon FSx for NetApp ONTAP , Amazon FSx for OpenZFS, Amazon DocumentDB (with MongoDB compatibility),
* Amazon RDS, Amazon Aurora, and Amazon Neptune.
*
*
* @param stopBackupJobRequest
* @return Result of the StopBackupJob operation returned by the service.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws InvalidRequestException
* Indicates that something is wrong with the input to the request. For example, a parameter is of the wrong
* type.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.StopBackupJob
* @see AWS API
* Documentation
*/
@Override
public StopBackupJobResult stopBackupJob(StopBackupJobRequest request) {
request = beforeClientExecution(request);
return executeStopBackupJob(request);
}
@SdkInternalApi
final StopBackupJobResult executeStopBackupJob(StopBackupJobRequest stopBackupJobRequest) {
ExecutionContext executionContext = createExecutionContext(stopBackupJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StopBackupJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(stopBackupJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StopBackupJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StopBackupJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Assigns a set of key-value pairs to a recovery point, backup plan, or backup vault identified by an Amazon
* Resource Name (ARN).
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @throws LimitExceededException
* A limit in the request has been exceeded; for example, a maximum number of items allowed in a request.
* @sample AWSBackup.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes a set of key-value pairs from a recovery point, backup plan, or backup vault identified by an Amazon
* Resource Name (ARN)
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* A resource that is required for the action doesn't exist.
* @throws InvalidParameterValueException
* Indicates that something is wrong with a parameter's value. For example, the value is out of range.
* @throws MissingParameterValueException
* Indicates that a required parameter is missing.
* @throws ServiceUnavailableException
* The request failed due to a temporary failure of the server.
* @sample AWSBackup.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Backup");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an existing backup plan identified by its