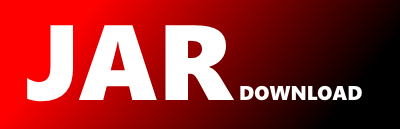
com.amazonaws.services.backup.model.BackupRuleInput Maven / Gradle / Ivy
Show all versions of aws-java-sdk-backup Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.backup.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Specifies a scheduled task used to back up a selection of resources.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class BackupRuleInput implements Serializable, Cloneable, StructuredPojo {
/**
*
* A display name for a backup rule. Must contain 1 to 50 alphanumeric or '-_.' characters.
*
*/
private String ruleName;
/**
*
* The name of a logical container where backups are stored. Backup vaults are identified by names that are unique
* to the account used to create them and the Amazon Web Services Region where they are created. They consist of
* lowercase letters, numbers, and hyphens.
*
*/
private String targetBackupVaultName;
/**
*
* A CRON expression in UTC specifying when Backup initiates a backup job.
*
*/
private String scheduleExpression;
/**
*
* A value in minutes after a backup is scheduled before a job will be canceled if it doesn't start successfully.
* This value is optional. If this value is included, it must be at least 60 minutes to avoid errors.
*
*
* This parameter has a maximum value of 100 years (52,560,000 minutes).
*
*
* During the start window, the backup job status remains in CREATED
status until it has successfully
* begun or until the start window time has run out. If within the start window time Backup receives an error that
* allows the job to be retried, Backup will automatically retry to begin the job at least every 10 minutes until
* the backup successfully begins (the job status changes to RUNNING
) or until the job status changes
* to EXPIRED
(which is expected to occur when the start window time is over).
*
*/
private Long startWindowMinutes;
/**
*
* A value in minutes after a backup job is successfully started before it must be completed or it will be canceled
* by Backup. This value is optional.
*
*/
private Long completionWindowMinutes;
/**
*
* The lifecycle defines when a protected resource is transitioned to cold storage and when it expires. Backup will
* transition and expire backups automatically according to the lifecycle that you define.
*
*
* Backups transitioned to cold storage must be stored in cold storage for a minimum of 90 days. Therefore, the
* “retention” setting must be 90 days greater than the “transition to cold after days” setting. The “transition to
* cold after days” setting cannot be changed after a backup has been transitioned to cold.
*
*
* Resource types that are able to be transitioned to cold storage are listed in the "Lifecycle to cold storage"
* section of the Feature
* availability by resource table. Backup ignores this expression for other resource types.
*
*
* This parameter has a maximum value of 100 years (36,500 days).
*
*/
private Lifecycle lifecycle;
/**
*
* To help organize your resources, you can assign your own metadata to the resources that you create. Each tag is a
* key-value pair.
*
*/
private java.util.Map recoveryPointTags;
/**
*
* An array of CopyAction
objects, which contains the details of the copy operation.
*
*/
private java.util.List copyActions;
/**
*
* Specifies whether Backup creates continuous backups. True causes Backup to create continuous backups capable of
* point-in-time restore (PITR). False (or not specified) causes Backup to create snapshot backups.
*
*/
private Boolean enableContinuousBackup;
/**
*
* This is the timezone in which the schedule expression is set. By default, ScheduleExpressions are in UTC. You can
* modify this to a specified timezone.
*
*/
private String scheduleExpressionTimezone;
/**
*
* A display name for a backup rule. Must contain 1 to 50 alphanumeric or '-_.' characters.
*
*
* @param ruleName
* A display name for a backup rule. Must contain 1 to 50 alphanumeric or '-_.' characters.
*/
public void setRuleName(String ruleName) {
this.ruleName = ruleName;
}
/**
*
* A display name for a backup rule. Must contain 1 to 50 alphanumeric or '-_.' characters.
*
*
* @return A display name for a backup rule. Must contain 1 to 50 alphanumeric or '-_.' characters.
*/
public String getRuleName() {
return this.ruleName;
}
/**
*
* A display name for a backup rule. Must contain 1 to 50 alphanumeric or '-_.' characters.
*
*
* @param ruleName
* A display name for a backup rule. Must contain 1 to 50 alphanumeric or '-_.' characters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BackupRuleInput withRuleName(String ruleName) {
setRuleName(ruleName);
return this;
}
/**
*
* The name of a logical container where backups are stored. Backup vaults are identified by names that are unique
* to the account used to create them and the Amazon Web Services Region where they are created. They consist of
* lowercase letters, numbers, and hyphens.
*
*
* @param targetBackupVaultName
* The name of a logical container where backups are stored. Backup vaults are identified by names that are
* unique to the account used to create them and the Amazon Web Services Region where they are created. They
* consist of lowercase letters, numbers, and hyphens.
*/
public void setTargetBackupVaultName(String targetBackupVaultName) {
this.targetBackupVaultName = targetBackupVaultName;
}
/**
*
* The name of a logical container where backups are stored. Backup vaults are identified by names that are unique
* to the account used to create them and the Amazon Web Services Region where they are created. They consist of
* lowercase letters, numbers, and hyphens.
*
*
* @return The name of a logical container where backups are stored. Backup vaults are identified by names that are
* unique to the account used to create them and the Amazon Web Services Region where they are created. They
* consist of lowercase letters, numbers, and hyphens.
*/
public String getTargetBackupVaultName() {
return this.targetBackupVaultName;
}
/**
*
* The name of a logical container where backups are stored. Backup vaults are identified by names that are unique
* to the account used to create them and the Amazon Web Services Region where they are created. They consist of
* lowercase letters, numbers, and hyphens.
*
*
* @param targetBackupVaultName
* The name of a logical container where backups are stored. Backup vaults are identified by names that are
* unique to the account used to create them and the Amazon Web Services Region where they are created. They
* consist of lowercase letters, numbers, and hyphens.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BackupRuleInput withTargetBackupVaultName(String targetBackupVaultName) {
setTargetBackupVaultName(targetBackupVaultName);
return this;
}
/**
*
* A CRON expression in UTC specifying when Backup initiates a backup job.
*
*
* @param scheduleExpression
* A CRON expression in UTC specifying when Backup initiates a backup job.
*/
public void setScheduleExpression(String scheduleExpression) {
this.scheduleExpression = scheduleExpression;
}
/**
*
* A CRON expression in UTC specifying when Backup initiates a backup job.
*
*
* @return A CRON expression in UTC specifying when Backup initiates a backup job.
*/
public String getScheduleExpression() {
return this.scheduleExpression;
}
/**
*
* A CRON expression in UTC specifying when Backup initiates a backup job.
*
*
* @param scheduleExpression
* A CRON expression in UTC specifying when Backup initiates a backup job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BackupRuleInput withScheduleExpression(String scheduleExpression) {
setScheduleExpression(scheduleExpression);
return this;
}
/**
*
* A value in minutes after a backup is scheduled before a job will be canceled if it doesn't start successfully.
* This value is optional. If this value is included, it must be at least 60 minutes to avoid errors.
*
*
* This parameter has a maximum value of 100 years (52,560,000 minutes).
*
*
* During the start window, the backup job status remains in CREATED
status until it has successfully
* begun or until the start window time has run out. If within the start window time Backup receives an error that
* allows the job to be retried, Backup will automatically retry to begin the job at least every 10 minutes until
* the backup successfully begins (the job status changes to RUNNING
) or until the job status changes
* to EXPIRED
(which is expected to occur when the start window time is over).
*
*
* @param startWindowMinutes
* A value in minutes after a backup is scheduled before a job will be canceled if it doesn't start
* successfully. This value is optional. If this value is included, it must be at least 60 minutes to avoid
* errors.
*
* This parameter has a maximum value of 100 years (52,560,000 minutes).
*
*
* During the start window, the backup job status remains in CREATED
status until it has
* successfully begun or until the start window time has run out. If within the start window time Backup
* receives an error that allows the job to be retried, Backup will automatically retry to begin the job at
* least every 10 minutes until the backup successfully begins (the job status changes to
* RUNNING
) or until the job status changes to EXPIRED
(which is expected to occur
* when the start window time is over).
*/
public void setStartWindowMinutes(Long startWindowMinutes) {
this.startWindowMinutes = startWindowMinutes;
}
/**
*
* A value in minutes after a backup is scheduled before a job will be canceled if it doesn't start successfully.
* This value is optional. If this value is included, it must be at least 60 minutes to avoid errors.
*
*
* This parameter has a maximum value of 100 years (52,560,000 minutes).
*
*
* During the start window, the backup job status remains in CREATED
status until it has successfully
* begun or until the start window time has run out. If within the start window time Backup receives an error that
* allows the job to be retried, Backup will automatically retry to begin the job at least every 10 minutes until
* the backup successfully begins (the job status changes to RUNNING
) or until the job status changes
* to EXPIRED
(which is expected to occur when the start window time is over).
*
*
* @return A value in minutes after a backup is scheduled before a job will be canceled if it doesn't start
* successfully. This value is optional. If this value is included, it must be at least 60 minutes to avoid
* errors.
*
* This parameter has a maximum value of 100 years (52,560,000 minutes).
*
*
* During the start window, the backup job status remains in CREATED
status until it has
* successfully begun or until the start window time has run out. If within the start window time Backup
* receives an error that allows the job to be retried, Backup will automatically retry to begin the job at
* least every 10 minutes until the backup successfully begins (the job status changes to
* RUNNING
) or until the job status changes to EXPIRED
(which is expected to occur
* when the start window time is over).
*/
public Long getStartWindowMinutes() {
return this.startWindowMinutes;
}
/**
*
* A value in minutes after a backup is scheduled before a job will be canceled if it doesn't start successfully.
* This value is optional. If this value is included, it must be at least 60 minutes to avoid errors.
*
*
* This parameter has a maximum value of 100 years (52,560,000 minutes).
*
*
* During the start window, the backup job status remains in CREATED
status until it has successfully
* begun or until the start window time has run out. If within the start window time Backup receives an error that
* allows the job to be retried, Backup will automatically retry to begin the job at least every 10 minutes until
* the backup successfully begins (the job status changes to RUNNING
) or until the job status changes
* to EXPIRED
(which is expected to occur when the start window time is over).
*
*
* @param startWindowMinutes
* A value in minutes after a backup is scheduled before a job will be canceled if it doesn't start
* successfully. This value is optional. If this value is included, it must be at least 60 minutes to avoid
* errors.
*
* This parameter has a maximum value of 100 years (52,560,000 minutes).
*
*
* During the start window, the backup job status remains in CREATED
status until it has
* successfully begun or until the start window time has run out. If within the start window time Backup
* receives an error that allows the job to be retried, Backup will automatically retry to begin the job at
* least every 10 minutes until the backup successfully begins (the job status changes to
* RUNNING
) or until the job status changes to EXPIRED
(which is expected to occur
* when the start window time is over).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BackupRuleInput withStartWindowMinutes(Long startWindowMinutes) {
setStartWindowMinutes(startWindowMinutes);
return this;
}
/**
*
* A value in minutes after a backup job is successfully started before it must be completed or it will be canceled
* by Backup. This value is optional.
*
*
* @param completionWindowMinutes
* A value in minutes after a backup job is successfully started before it must be completed or it will be
* canceled by Backup. This value is optional.
*/
public void setCompletionWindowMinutes(Long completionWindowMinutes) {
this.completionWindowMinutes = completionWindowMinutes;
}
/**
*
* A value in minutes after a backup job is successfully started before it must be completed or it will be canceled
* by Backup. This value is optional.
*
*
* @return A value in minutes after a backup job is successfully started before it must be completed or it will be
* canceled by Backup. This value is optional.
*/
public Long getCompletionWindowMinutes() {
return this.completionWindowMinutes;
}
/**
*
* A value in minutes after a backup job is successfully started before it must be completed or it will be canceled
* by Backup. This value is optional.
*
*
* @param completionWindowMinutes
* A value in minutes after a backup job is successfully started before it must be completed or it will be
* canceled by Backup. This value is optional.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BackupRuleInput withCompletionWindowMinutes(Long completionWindowMinutes) {
setCompletionWindowMinutes(completionWindowMinutes);
return this;
}
/**
*
* The lifecycle defines when a protected resource is transitioned to cold storage and when it expires. Backup will
* transition and expire backups automatically according to the lifecycle that you define.
*
*
* Backups transitioned to cold storage must be stored in cold storage for a minimum of 90 days. Therefore, the
* “retention” setting must be 90 days greater than the “transition to cold after days” setting. The “transition to
* cold after days” setting cannot be changed after a backup has been transitioned to cold.
*
*
* Resource types that are able to be transitioned to cold storage are listed in the "Lifecycle to cold storage"
* section of the Feature
* availability by resource table. Backup ignores this expression for other resource types.
*
*
* This parameter has a maximum value of 100 years (36,500 days).
*
*
* @param lifecycle
* The lifecycle defines when a protected resource is transitioned to cold storage and when it expires.
* Backup will transition and expire backups automatically according to the lifecycle that you define.
*
* Backups transitioned to cold storage must be stored in cold storage for a minimum of 90 days. Therefore,
* the “retention” setting must be 90 days greater than the “transition to cold after days” setting. The
* “transition to cold after days” setting cannot be changed after a backup has been transitioned to cold.
*
*
* Resource types that are able to be transitioned to cold storage are listed in the
* "Lifecycle to cold storage" section of the
* Feature availability by resource table. Backup ignores this expression for other resource types.
*
*
* This parameter has a maximum value of 100 years (36,500 days).
*/
public void setLifecycle(Lifecycle lifecycle) {
this.lifecycle = lifecycle;
}
/**
*
* The lifecycle defines when a protected resource is transitioned to cold storage and when it expires. Backup will
* transition and expire backups automatically according to the lifecycle that you define.
*
*
* Backups transitioned to cold storage must be stored in cold storage for a minimum of 90 days. Therefore, the
* “retention” setting must be 90 days greater than the “transition to cold after days” setting. The “transition to
* cold after days” setting cannot be changed after a backup has been transitioned to cold.
*
*
* Resource types that are able to be transitioned to cold storage are listed in the "Lifecycle to cold storage"
* section of the Feature
* availability by resource table. Backup ignores this expression for other resource types.
*
*
* This parameter has a maximum value of 100 years (36,500 days).
*
*
* @return The lifecycle defines when a protected resource is transitioned to cold storage and when it expires.
* Backup will transition and expire backups automatically according to the lifecycle that you define.
*
* Backups transitioned to cold storage must be stored in cold storage for a minimum of 90 days. Therefore,
* the “retention” setting must be 90 days greater than the “transition to cold after days” setting. The
* “transition to cold after days” setting cannot be changed after a backup has been transitioned to cold.
*
*
* Resource types that are able to be transitioned to cold storage are listed in the
* "Lifecycle to cold storage" section of the
* Feature availability by resource table. Backup ignores this expression for other resource types.
*
*
* This parameter has a maximum value of 100 years (36,500 days).
*/
public Lifecycle getLifecycle() {
return this.lifecycle;
}
/**
*
* The lifecycle defines when a protected resource is transitioned to cold storage and when it expires. Backup will
* transition and expire backups automatically according to the lifecycle that you define.
*
*
* Backups transitioned to cold storage must be stored in cold storage for a minimum of 90 days. Therefore, the
* “retention” setting must be 90 days greater than the “transition to cold after days” setting. The “transition to
* cold after days” setting cannot be changed after a backup has been transitioned to cold.
*
*
* Resource types that are able to be transitioned to cold storage are listed in the "Lifecycle to cold storage"
* section of the Feature
* availability by resource table. Backup ignores this expression for other resource types.
*
*
* This parameter has a maximum value of 100 years (36,500 days).
*
*
* @param lifecycle
* The lifecycle defines when a protected resource is transitioned to cold storage and when it expires.
* Backup will transition and expire backups automatically according to the lifecycle that you define.
*
* Backups transitioned to cold storage must be stored in cold storage for a minimum of 90 days. Therefore,
* the “retention” setting must be 90 days greater than the “transition to cold after days” setting. The
* “transition to cold after days” setting cannot be changed after a backup has been transitioned to cold.
*
*
* Resource types that are able to be transitioned to cold storage are listed in the
* "Lifecycle to cold storage" section of the
* Feature availability by resource table. Backup ignores this expression for other resource types.
*
*
* This parameter has a maximum value of 100 years (36,500 days).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BackupRuleInput withLifecycle(Lifecycle lifecycle) {
setLifecycle(lifecycle);
return this;
}
/**
*
* To help organize your resources, you can assign your own metadata to the resources that you create. Each tag is a
* key-value pair.
*
*
* @return To help organize your resources, you can assign your own metadata to the resources that you create. Each
* tag is a key-value pair.
*/
public java.util.Map getRecoveryPointTags() {
return recoveryPointTags;
}
/**
*
* To help organize your resources, you can assign your own metadata to the resources that you create. Each tag is a
* key-value pair.
*
*
* @param recoveryPointTags
* To help organize your resources, you can assign your own metadata to the resources that you create. Each
* tag is a key-value pair.
*/
public void setRecoveryPointTags(java.util.Map recoveryPointTags) {
this.recoveryPointTags = recoveryPointTags;
}
/**
*
* To help organize your resources, you can assign your own metadata to the resources that you create. Each tag is a
* key-value pair.
*
*
* @param recoveryPointTags
* To help organize your resources, you can assign your own metadata to the resources that you create. Each
* tag is a key-value pair.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BackupRuleInput withRecoveryPointTags(java.util.Map recoveryPointTags) {
setRecoveryPointTags(recoveryPointTags);
return this;
}
/**
* Add a single RecoveryPointTags entry
*
* @see BackupRuleInput#withRecoveryPointTags
* @returns a reference to this object so that method calls can be chained together.
*/
public BackupRuleInput addRecoveryPointTagsEntry(String key, String value) {
if (null == this.recoveryPointTags) {
this.recoveryPointTags = new java.util.HashMap();
}
if (this.recoveryPointTags.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.recoveryPointTags.put(key, value);
return this;
}
/**
* Removes all the entries added into RecoveryPointTags.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BackupRuleInput clearRecoveryPointTagsEntries() {
this.recoveryPointTags = null;
return this;
}
/**
*
* An array of CopyAction
objects, which contains the details of the copy operation.
*
*
* @return An array of CopyAction
objects, which contains the details of the copy operation.
*/
public java.util.List getCopyActions() {
return copyActions;
}
/**
*
* An array of CopyAction
objects, which contains the details of the copy operation.
*
*
* @param copyActions
* An array of CopyAction
objects, which contains the details of the copy operation.
*/
public void setCopyActions(java.util.Collection copyActions) {
if (copyActions == null) {
this.copyActions = null;
return;
}
this.copyActions = new java.util.ArrayList(copyActions);
}
/**
*
* An array of CopyAction
objects, which contains the details of the copy operation.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCopyActions(java.util.Collection)} or {@link #withCopyActions(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param copyActions
* An array of CopyAction
objects, which contains the details of the copy operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BackupRuleInput withCopyActions(CopyAction... copyActions) {
if (this.copyActions == null) {
setCopyActions(new java.util.ArrayList(copyActions.length));
}
for (CopyAction ele : copyActions) {
this.copyActions.add(ele);
}
return this;
}
/**
*
* An array of CopyAction
objects, which contains the details of the copy operation.
*
*
* @param copyActions
* An array of CopyAction
objects, which contains the details of the copy operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BackupRuleInput withCopyActions(java.util.Collection copyActions) {
setCopyActions(copyActions);
return this;
}
/**
*
* Specifies whether Backup creates continuous backups. True causes Backup to create continuous backups capable of
* point-in-time restore (PITR). False (or not specified) causes Backup to create snapshot backups.
*
*
* @param enableContinuousBackup
* Specifies whether Backup creates continuous backups. True causes Backup to create continuous backups
* capable of point-in-time restore (PITR). False (or not specified) causes Backup to create snapshot
* backups.
*/
public void setEnableContinuousBackup(Boolean enableContinuousBackup) {
this.enableContinuousBackup = enableContinuousBackup;
}
/**
*
* Specifies whether Backup creates continuous backups. True causes Backup to create continuous backups capable of
* point-in-time restore (PITR). False (or not specified) causes Backup to create snapshot backups.
*
*
* @return Specifies whether Backup creates continuous backups. True causes Backup to create continuous backups
* capable of point-in-time restore (PITR). False (or not specified) causes Backup to create snapshot
* backups.
*/
public Boolean getEnableContinuousBackup() {
return this.enableContinuousBackup;
}
/**
*
* Specifies whether Backup creates continuous backups. True causes Backup to create continuous backups capable of
* point-in-time restore (PITR). False (or not specified) causes Backup to create snapshot backups.
*
*
* @param enableContinuousBackup
* Specifies whether Backup creates continuous backups. True causes Backup to create continuous backups
* capable of point-in-time restore (PITR). False (or not specified) causes Backup to create snapshot
* backups.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BackupRuleInput withEnableContinuousBackup(Boolean enableContinuousBackup) {
setEnableContinuousBackup(enableContinuousBackup);
return this;
}
/**
*
* Specifies whether Backup creates continuous backups. True causes Backup to create continuous backups capable of
* point-in-time restore (PITR). False (or not specified) causes Backup to create snapshot backups.
*
*
* @return Specifies whether Backup creates continuous backups. True causes Backup to create continuous backups
* capable of point-in-time restore (PITR). False (or not specified) causes Backup to create snapshot
* backups.
*/
public Boolean isEnableContinuousBackup() {
return this.enableContinuousBackup;
}
/**
*
* This is the timezone in which the schedule expression is set. By default, ScheduleExpressions are in UTC. You can
* modify this to a specified timezone.
*
*
* @param scheduleExpressionTimezone
* This is the timezone in which the schedule expression is set. By default, ScheduleExpressions are in UTC.
* You can modify this to a specified timezone.
*/
public void setScheduleExpressionTimezone(String scheduleExpressionTimezone) {
this.scheduleExpressionTimezone = scheduleExpressionTimezone;
}
/**
*
* This is the timezone in which the schedule expression is set. By default, ScheduleExpressions are in UTC. You can
* modify this to a specified timezone.
*
*
* @return This is the timezone in which the schedule expression is set. By default, ScheduleExpressions are in UTC.
* You can modify this to a specified timezone.
*/
public String getScheduleExpressionTimezone() {
return this.scheduleExpressionTimezone;
}
/**
*
* This is the timezone in which the schedule expression is set. By default, ScheduleExpressions are in UTC. You can
* modify this to a specified timezone.
*
*
* @param scheduleExpressionTimezone
* This is the timezone in which the schedule expression is set. By default, ScheduleExpressions are in UTC.
* You can modify this to a specified timezone.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public BackupRuleInput withScheduleExpressionTimezone(String scheduleExpressionTimezone) {
setScheduleExpressionTimezone(scheduleExpressionTimezone);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getRuleName() != null)
sb.append("RuleName: ").append(getRuleName()).append(",");
if (getTargetBackupVaultName() != null)
sb.append("TargetBackupVaultName: ").append(getTargetBackupVaultName()).append(",");
if (getScheduleExpression() != null)
sb.append("ScheduleExpression: ").append(getScheduleExpression()).append(",");
if (getStartWindowMinutes() != null)
sb.append("StartWindowMinutes: ").append(getStartWindowMinutes()).append(",");
if (getCompletionWindowMinutes() != null)
sb.append("CompletionWindowMinutes: ").append(getCompletionWindowMinutes()).append(",");
if (getLifecycle() != null)
sb.append("Lifecycle: ").append(getLifecycle()).append(",");
if (getRecoveryPointTags() != null)
sb.append("RecoveryPointTags: ").append("***Sensitive Data Redacted***").append(",");
if (getCopyActions() != null)
sb.append("CopyActions: ").append(getCopyActions()).append(",");
if (getEnableContinuousBackup() != null)
sb.append("EnableContinuousBackup: ").append(getEnableContinuousBackup()).append(",");
if (getScheduleExpressionTimezone() != null)
sb.append("ScheduleExpressionTimezone: ").append(getScheduleExpressionTimezone());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof BackupRuleInput == false)
return false;
BackupRuleInput other = (BackupRuleInput) obj;
if (other.getRuleName() == null ^ this.getRuleName() == null)
return false;
if (other.getRuleName() != null && other.getRuleName().equals(this.getRuleName()) == false)
return false;
if (other.getTargetBackupVaultName() == null ^ this.getTargetBackupVaultName() == null)
return false;
if (other.getTargetBackupVaultName() != null && other.getTargetBackupVaultName().equals(this.getTargetBackupVaultName()) == false)
return false;
if (other.getScheduleExpression() == null ^ this.getScheduleExpression() == null)
return false;
if (other.getScheduleExpression() != null && other.getScheduleExpression().equals(this.getScheduleExpression()) == false)
return false;
if (other.getStartWindowMinutes() == null ^ this.getStartWindowMinutes() == null)
return false;
if (other.getStartWindowMinutes() != null && other.getStartWindowMinutes().equals(this.getStartWindowMinutes()) == false)
return false;
if (other.getCompletionWindowMinutes() == null ^ this.getCompletionWindowMinutes() == null)
return false;
if (other.getCompletionWindowMinutes() != null && other.getCompletionWindowMinutes().equals(this.getCompletionWindowMinutes()) == false)
return false;
if (other.getLifecycle() == null ^ this.getLifecycle() == null)
return false;
if (other.getLifecycle() != null && other.getLifecycle().equals(this.getLifecycle()) == false)
return false;
if (other.getRecoveryPointTags() == null ^ this.getRecoveryPointTags() == null)
return false;
if (other.getRecoveryPointTags() != null && other.getRecoveryPointTags().equals(this.getRecoveryPointTags()) == false)
return false;
if (other.getCopyActions() == null ^ this.getCopyActions() == null)
return false;
if (other.getCopyActions() != null && other.getCopyActions().equals(this.getCopyActions()) == false)
return false;
if (other.getEnableContinuousBackup() == null ^ this.getEnableContinuousBackup() == null)
return false;
if (other.getEnableContinuousBackup() != null && other.getEnableContinuousBackup().equals(this.getEnableContinuousBackup()) == false)
return false;
if (other.getScheduleExpressionTimezone() == null ^ this.getScheduleExpressionTimezone() == null)
return false;
if (other.getScheduleExpressionTimezone() != null && other.getScheduleExpressionTimezone().equals(this.getScheduleExpressionTimezone()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getRuleName() == null) ? 0 : getRuleName().hashCode());
hashCode = prime * hashCode + ((getTargetBackupVaultName() == null) ? 0 : getTargetBackupVaultName().hashCode());
hashCode = prime * hashCode + ((getScheduleExpression() == null) ? 0 : getScheduleExpression().hashCode());
hashCode = prime * hashCode + ((getStartWindowMinutes() == null) ? 0 : getStartWindowMinutes().hashCode());
hashCode = prime * hashCode + ((getCompletionWindowMinutes() == null) ? 0 : getCompletionWindowMinutes().hashCode());
hashCode = prime * hashCode + ((getLifecycle() == null) ? 0 : getLifecycle().hashCode());
hashCode = prime * hashCode + ((getRecoveryPointTags() == null) ? 0 : getRecoveryPointTags().hashCode());
hashCode = prime * hashCode + ((getCopyActions() == null) ? 0 : getCopyActions().hashCode());
hashCode = prime * hashCode + ((getEnableContinuousBackup() == null) ? 0 : getEnableContinuousBackup().hashCode());
hashCode = prime * hashCode + ((getScheduleExpressionTimezone() == null) ? 0 : getScheduleExpressionTimezone().hashCode());
return hashCode;
}
@Override
public BackupRuleInput clone() {
try {
return (BackupRuleInput) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.backup.model.transform.BackupRuleInputMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}