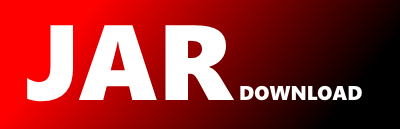
com.amazonaws.services.backup.model.CopyJob Maven / Gradle / Ivy
Show all versions of aws-java-sdk-backup Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.backup.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Contains detailed information about a copy job.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CopyJob implements Serializable, Cloneable, StructuredPojo {
/**
*
* The account ID that owns the copy job.
*
*/
private String accountId;
/**
*
* Uniquely identifies a copy job.
*
*/
private String copyJobId;
/**
*
* An Amazon Resource Name (ARN) that uniquely identifies a source copy vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*
*/
private String sourceBackupVaultArn;
/**
*
* An ARN that uniquely identifies a source recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*
*/
private String sourceRecoveryPointArn;
/**
*
* An Amazon Resource Name (ARN) that uniquely identifies a destination copy vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*
*/
private String destinationBackupVaultArn;
/**
*
* An ARN that uniquely identifies a destination recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*
*/
private String destinationRecoveryPointArn;
/**
*
* The Amazon Web Services resource to be copied; for example, an Amazon Elastic Block Store (Amazon EBS) volume or
* an Amazon Relational Database Service (Amazon RDS) database.
*
*/
private String resourceArn;
/**
*
* The date and time a copy job is created, in Unix format and Coordinated Universal Time (UTC). The value of
* CreationDate
is accurate to milliseconds. For example, the value 1516925490.087 represents Friday,
* January 26, 2018 12:11:30.087 AM.
*
*/
private java.util.Date creationDate;
/**
*
* The date and time a copy job is completed, in Unix format and Coordinated Universal Time (UTC). The value of
* CompletionDate
is accurate to milliseconds. For example, the value 1516925490.087 represents Friday,
* January 26, 2018 12:11:30.087 AM.
*
*/
private java.util.Date completionDate;
/**
*
* The current state of a copy job.
*
*/
private String state;
/**
*
* A detailed message explaining the status of the job to copy a resource.
*
*/
private String statusMessage;
/**
*
* The size, in bytes, of a copy job.
*
*/
private Long backupSizeInBytes;
/**
*
* Specifies the IAM role ARN used to copy the target recovery point; for example,
* arn:aws:iam::123456789012:role/S3Access
.
*
*/
private String iamRoleArn;
private RecoveryPointCreator createdBy;
/**
*
* The type of Amazon Web Services resource to be copied; for example, an Amazon Elastic Block Store (Amazon EBS)
* volume or an Amazon Relational Database Service (Amazon RDS) database.
*
*/
private String resourceType;
/**
*
* This uniquely identifies a request to Backup to copy a resource. The return will be the parent (composite) job
* ID.
*
*/
private String parentJobId;
/**
*
* This is a boolean value indicating this is a parent (composite) copy job.
*
*/
private Boolean isParent;
/**
*
* This is the identifier of a resource within a composite group, such as nested (child) recovery point belonging to
* a composite (parent) stack. The ID is transferred from the logical ID within a stack.
*
*/
private String compositeMemberIdentifier;
/**
*
* This is the number of child (nested) copy jobs.
*
*/
private Long numberOfChildJobs;
/**
*
* This returns the statistics of the included child (nested) copy jobs.
*
*/
private java.util.Map childJobsInState;
/**
*
* This is the non-unique name of the resource that belongs to the specified backup.
*
*/
private String resourceName;
/**
*
* This parameter is the job count for the specified message category.
*
*
* Example strings may include AccessDenied
, SUCCESS
, AGGREGATE_ALL
, and
* InvalidParameters
. See Monitoring for a list of
* MessageCategory strings.
*
*
* The the value ANY returns count of all message categories.
*
*
* AGGREGATE_ALL
aggregates job counts for all message categories and returns the sum
*
*/
private String messageCategory;
/**
*
* The account ID that owns the copy job.
*
*
* @param accountId
* The account ID that owns the copy job.
*/
public void setAccountId(String accountId) {
this.accountId = accountId;
}
/**
*
* The account ID that owns the copy job.
*
*
* @return The account ID that owns the copy job.
*/
public String getAccountId() {
return this.accountId;
}
/**
*
* The account ID that owns the copy job.
*
*
* @param accountId
* The account ID that owns the copy job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob withAccountId(String accountId) {
setAccountId(accountId);
return this;
}
/**
*
* Uniquely identifies a copy job.
*
*
* @param copyJobId
* Uniquely identifies a copy job.
*/
public void setCopyJobId(String copyJobId) {
this.copyJobId = copyJobId;
}
/**
*
* Uniquely identifies a copy job.
*
*
* @return Uniquely identifies a copy job.
*/
public String getCopyJobId() {
return this.copyJobId;
}
/**
*
* Uniquely identifies a copy job.
*
*
* @param copyJobId
* Uniquely identifies a copy job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob withCopyJobId(String copyJobId) {
setCopyJobId(copyJobId);
return this;
}
/**
*
* An Amazon Resource Name (ARN) that uniquely identifies a source copy vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*
*
* @param sourceBackupVaultArn
* An Amazon Resource Name (ARN) that uniquely identifies a source copy vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*/
public void setSourceBackupVaultArn(String sourceBackupVaultArn) {
this.sourceBackupVaultArn = sourceBackupVaultArn;
}
/**
*
* An Amazon Resource Name (ARN) that uniquely identifies a source copy vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*
*
* @return An Amazon Resource Name (ARN) that uniquely identifies a source copy vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*/
public String getSourceBackupVaultArn() {
return this.sourceBackupVaultArn;
}
/**
*
* An Amazon Resource Name (ARN) that uniquely identifies a source copy vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*
*
* @param sourceBackupVaultArn
* An Amazon Resource Name (ARN) that uniquely identifies a source copy vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob withSourceBackupVaultArn(String sourceBackupVaultArn) {
setSourceBackupVaultArn(sourceBackupVaultArn);
return this;
}
/**
*
* An ARN that uniquely identifies a source recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*
*
* @param sourceRecoveryPointArn
* An ARN that uniquely identifies a source recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*/
public void setSourceRecoveryPointArn(String sourceRecoveryPointArn) {
this.sourceRecoveryPointArn = sourceRecoveryPointArn;
}
/**
*
* An ARN that uniquely identifies a source recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*
*
* @return An ARN that uniquely identifies a source recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*/
public String getSourceRecoveryPointArn() {
return this.sourceRecoveryPointArn;
}
/**
*
* An ARN that uniquely identifies a source recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*
*
* @param sourceRecoveryPointArn
* An ARN that uniquely identifies a source recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob withSourceRecoveryPointArn(String sourceRecoveryPointArn) {
setSourceRecoveryPointArn(sourceRecoveryPointArn);
return this;
}
/**
*
* An Amazon Resource Name (ARN) that uniquely identifies a destination copy vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*
*
* @param destinationBackupVaultArn
* An Amazon Resource Name (ARN) that uniquely identifies a destination copy vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*/
public void setDestinationBackupVaultArn(String destinationBackupVaultArn) {
this.destinationBackupVaultArn = destinationBackupVaultArn;
}
/**
*
* An Amazon Resource Name (ARN) that uniquely identifies a destination copy vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*
*
* @return An Amazon Resource Name (ARN) that uniquely identifies a destination copy vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*/
public String getDestinationBackupVaultArn() {
return this.destinationBackupVaultArn;
}
/**
*
* An Amazon Resource Name (ARN) that uniquely identifies a destination copy vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*
*
* @param destinationBackupVaultArn
* An Amazon Resource Name (ARN) that uniquely identifies a destination copy vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob withDestinationBackupVaultArn(String destinationBackupVaultArn) {
setDestinationBackupVaultArn(destinationBackupVaultArn);
return this;
}
/**
*
* An ARN that uniquely identifies a destination recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*
*
* @param destinationRecoveryPointArn
* An ARN that uniquely identifies a destination recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*/
public void setDestinationRecoveryPointArn(String destinationRecoveryPointArn) {
this.destinationRecoveryPointArn = destinationRecoveryPointArn;
}
/**
*
* An ARN that uniquely identifies a destination recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*
*
* @return An ARN that uniquely identifies a destination recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*/
public String getDestinationRecoveryPointArn() {
return this.destinationRecoveryPointArn;
}
/**
*
* An ARN that uniquely identifies a destination recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*
*
* @param destinationRecoveryPointArn
* An ARN that uniquely identifies a destination recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob withDestinationRecoveryPointArn(String destinationRecoveryPointArn) {
setDestinationRecoveryPointArn(destinationRecoveryPointArn);
return this;
}
/**
*
* The Amazon Web Services resource to be copied; for example, an Amazon Elastic Block Store (Amazon EBS) volume or
* an Amazon Relational Database Service (Amazon RDS) database.
*
*
* @param resourceArn
* The Amazon Web Services resource to be copied; for example, an Amazon Elastic Block Store (Amazon EBS)
* volume or an Amazon Relational Database Service (Amazon RDS) database.
*/
public void setResourceArn(String resourceArn) {
this.resourceArn = resourceArn;
}
/**
*
* The Amazon Web Services resource to be copied; for example, an Amazon Elastic Block Store (Amazon EBS) volume or
* an Amazon Relational Database Service (Amazon RDS) database.
*
*
* @return The Amazon Web Services resource to be copied; for example, an Amazon Elastic Block Store (Amazon EBS)
* volume or an Amazon Relational Database Service (Amazon RDS) database.
*/
public String getResourceArn() {
return this.resourceArn;
}
/**
*
* The Amazon Web Services resource to be copied; for example, an Amazon Elastic Block Store (Amazon EBS) volume or
* an Amazon Relational Database Service (Amazon RDS) database.
*
*
* @param resourceArn
* The Amazon Web Services resource to be copied; for example, an Amazon Elastic Block Store (Amazon EBS)
* volume or an Amazon Relational Database Service (Amazon RDS) database.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob withResourceArn(String resourceArn) {
setResourceArn(resourceArn);
return this;
}
/**
*
* The date and time a copy job is created, in Unix format and Coordinated Universal Time (UTC). The value of
* CreationDate
is accurate to milliseconds. For example, the value 1516925490.087 represents Friday,
* January 26, 2018 12:11:30.087 AM.
*
*
* @param creationDate
* The date and time a copy job is created, in Unix format and Coordinated Universal Time (UTC). The value of
* CreationDate
is accurate to milliseconds. For example, the value 1516925490.087 represents
* Friday, January 26, 2018 12:11:30.087 AM.
*/
public void setCreationDate(java.util.Date creationDate) {
this.creationDate = creationDate;
}
/**
*
* The date and time a copy job is created, in Unix format and Coordinated Universal Time (UTC). The value of
* CreationDate
is accurate to milliseconds. For example, the value 1516925490.087 represents Friday,
* January 26, 2018 12:11:30.087 AM.
*
*
* @return The date and time a copy job is created, in Unix format and Coordinated Universal Time (UTC). The value
* of CreationDate
is accurate to milliseconds. For example, the value 1516925490.087
* represents Friday, January 26, 2018 12:11:30.087 AM.
*/
public java.util.Date getCreationDate() {
return this.creationDate;
}
/**
*
* The date and time a copy job is created, in Unix format and Coordinated Universal Time (UTC). The value of
* CreationDate
is accurate to milliseconds. For example, the value 1516925490.087 represents Friday,
* January 26, 2018 12:11:30.087 AM.
*
*
* @param creationDate
* The date and time a copy job is created, in Unix format and Coordinated Universal Time (UTC). The value of
* CreationDate
is accurate to milliseconds. For example, the value 1516925490.087 represents
* Friday, January 26, 2018 12:11:30.087 AM.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob withCreationDate(java.util.Date creationDate) {
setCreationDate(creationDate);
return this;
}
/**
*
* The date and time a copy job is completed, in Unix format and Coordinated Universal Time (UTC). The value of
* CompletionDate
is accurate to milliseconds. For example, the value 1516925490.087 represents Friday,
* January 26, 2018 12:11:30.087 AM.
*
*
* @param completionDate
* The date and time a copy job is completed, in Unix format and Coordinated Universal Time (UTC). The value
* of CompletionDate
is accurate to milliseconds. For example, the value 1516925490.087
* represents Friday, January 26, 2018 12:11:30.087 AM.
*/
public void setCompletionDate(java.util.Date completionDate) {
this.completionDate = completionDate;
}
/**
*
* The date and time a copy job is completed, in Unix format and Coordinated Universal Time (UTC). The value of
* CompletionDate
is accurate to milliseconds. For example, the value 1516925490.087 represents Friday,
* January 26, 2018 12:11:30.087 AM.
*
*
* @return The date and time a copy job is completed, in Unix format and Coordinated Universal Time (UTC). The value
* of CompletionDate
is accurate to milliseconds. For example, the value 1516925490.087
* represents Friday, January 26, 2018 12:11:30.087 AM.
*/
public java.util.Date getCompletionDate() {
return this.completionDate;
}
/**
*
* The date and time a copy job is completed, in Unix format and Coordinated Universal Time (UTC). The value of
* CompletionDate
is accurate to milliseconds. For example, the value 1516925490.087 represents Friday,
* January 26, 2018 12:11:30.087 AM.
*
*
* @param completionDate
* The date and time a copy job is completed, in Unix format and Coordinated Universal Time (UTC). The value
* of CompletionDate
is accurate to milliseconds. For example, the value 1516925490.087
* represents Friday, January 26, 2018 12:11:30.087 AM.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob withCompletionDate(java.util.Date completionDate) {
setCompletionDate(completionDate);
return this;
}
/**
*
* The current state of a copy job.
*
*
* @param state
* The current state of a copy job.
* @see CopyJobState
*/
public void setState(String state) {
this.state = state;
}
/**
*
* The current state of a copy job.
*
*
* @return The current state of a copy job.
* @see CopyJobState
*/
public String getState() {
return this.state;
}
/**
*
* The current state of a copy job.
*
*
* @param state
* The current state of a copy job.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CopyJobState
*/
public CopyJob withState(String state) {
setState(state);
return this;
}
/**
*
* The current state of a copy job.
*
*
* @param state
* The current state of a copy job.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CopyJobState
*/
public CopyJob withState(CopyJobState state) {
this.state = state.toString();
return this;
}
/**
*
* A detailed message explaining the status of the job to copy a resource.
*
*
* @param statusMessage
* A detailed message explaining the status of the job to copy a resource.
*/
public void setStatusMessage(String statusMessage) {
this.statusMessage = statusMessage;
}
/**
*
* A detailed message explaining the status of the job to copy a resource.
*
*
* @return A detailed message explaining the status of the job to copy a resource.
*/
public String getStatusMessage() {
return this.statusMessage;
}
/**
*
* A detailed message explaining the status of the job to copy a resource.
*
*
* @param statusMessage
* A detailed message explaining the status of the job to copy a resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob withStatusMessage(String statusMessage) {
setStatusMessage(statusMessage);
return this;
}
/**
*
* The size, in bytes, of a copy job.
*
*
* @param backupSizeInBytes
* The size, in bytes, of a copy job.
*/
public void setBackupSizeInBytes(Long backupSizeInBytes) {
this.backupSizeInBytes = backupSizeInBytes;
}
/**
*
* The size, in bytes, of a copy job.
*
*
* @return The size, in bytes, of a copy job.
*/
public Long getBackupSizeInBytes() {
return this.backupSizeInBytes;
}
/**
*
* The size, in bytes, of a copy job.
*
*
* @param backupSizeInBytes
* The size, in bytes, of a copy job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob withBackupSizeInBytes(Long backupSizeInBytes) {
setBackupSizeInBytes(backupSizeInBytes);
return this;
}
/**
*
* Specifies the IAM role ARN used to copy the target recovery point; for example,
* arn:aws:iam::123456789012:role/S3Access
.
*
*
* @param iamRoleArn
* Specifies the IAM role ARN used to copy the target recovery point; for example,
* arn:aws:iam::123456789012:role/S3Access
.
*/
public void setIamRoleArn(String iamRoleArn) {
this.iamRoleArn = iamRoleArn;
}
/**
*
* Specifies the IAM role ARN used to copy the target recovery point; for example,
* arn:aws:iam::123456789012:role/S3Access
.
*
*
* @return Specifies the IAM role ARN used to copy the target recovery point; for example,
* arn:aws:iam::123456789012:role/S3Access
.
*/
public String getIamRoleArn() {
return this.iamRoleArn;
}
/**
*
* Specifies the IAM role ARN used to copy the target recovery point; for example,
* arn:aws:iam::123456789012:role/S3Access
.
*
*
* @param iamRoleArn
* Specifies the IAM role ARN used to copy the target recovery point; for example,
* arn:aws:iam::123456789012:role/S3Access
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob withIamRoleArn(String iamRoleArn) {
setIamRoleArn(iamRoleArn);
return this;
}
/**
* @param createdBy
*/
public void setCreatedBy(RecoveryPointCreator createdBy) {
this.createdBy = createdBy;
}
/**
* @return
*/
public RecoveryPointCreator getCreatedBy() {
return this.createdBy;
}
/**
* @param createdBy
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob withCreatedBy(RecoveryPointCreator createdBy) {
setCreatedBy(createdBy);
return this;
}
/**
*
* The type of Amazon Web Services resource to be copied; for example, an Amazon Elastic Block Store (Amazon EBS)
* volume or an Amazon Relational Database Service (Amazon RDS) database.
*
*
* @param resourceType
* The type of Amazon Web Services resource to be copied; for example, an Amazon Elastic Block Store (Amazon
* EBS) volume or an Amazon Relational Database Service (Amazon RDS) database.
*/
public void setResourceType(String resourceType) {
this.resourceType = resourceType;
}
/**
*
* The type of Amazon Web Services resource to be copied; for example, an Amazon Elastic Block Store (Amazon EBS)
* volume or an Amazon Relational Database Service (Amazon RDS) database.
*
*
* @return The type of Amazon Web Services resource to be copied; for example, an Amazon Elastic Block Store (Amazon
* EBS) volume or an Amazon Relational Database Service (Amazon RDS) database.
*/
public String getResourceType() {
return this.resourceType;
}
/**
*
* The type of Amazon Web Services resource to be copied; for example, an Amazon Elastic Block Store (Amazon EBS)
* volume or an Amazon Relational Database Service (Amazon RDS) database.
*
*
* @param resourceType
* The type of Amazon Web Services resource to be copied; for example, an Amazon Elastic Block Store (Amazon
* EBS) volume or an Amazon Relational Database Service (Amazon RDS) database.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob withResourceType(String resourceType) {
setResourceType(resourceType);
return this;
}
/**
*
* This uniquely identifies a request to Backup to copy a resource. The return will be the parent (composite) job
* ID.
*
*
* @param parentJobId
* This uniquely identifies a request to Backup to copy a resource. The return will be the parent (composite)
* job ID.
*/
public void setParentJobId(String parentJobId) {
this.parentJobId = parentJobId;
}
/**
*
* This uniquely identifies a request to Backup to copy a resource. The return will be the parent (composite) job
* ID.
*
*
* @return This uniquely identifies a request to Backup to copy a resource. The return will be the parent
* (composite) job ID.
*/
public String getParentJobId() {
return this.parentJobId;
}
/**
*
* This uniquely identifies a request to Backup to copy a resource. The return will be the parent (composite) job
* ID.
*
*
* @param parentJobId
* This uniquely identifies a request to Backup to copy a resource. The return will be the parent (composite)
* job ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob withParentJobId(String parentJobId) {
setParentJobId(parentJobId);
return this;
}
/**
*
* This is a boolean value indicating this is a parent (composite) copy job.
*
*
* @param isParent
* This is a boolean value indicating this is a parent (composite) copy job.
*/
public void setIsParent(Boolean isParent) {
this.isParent = isParent;
}
/**
*
* This is a boolean value indicating this is a parent (composite) copy job.
*
*
* @return This is a boolean value indicating this is a parent (composite) copy job.
*/
public Boolean getIsParent() {
return this.isParent;
}
/**
*
* This is a boolean value indicating this is a parent (composite) copy job.
*
*
* @param isParent
* This is a boolean value indicating this is a parent (composite) copy job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob withIsParent(Boolean isParent) {
setIsParent(isParent);
return this;
}
/**
*
* This is a boolean value indicating this is a parent (composite) copy job.
*
*
* @return This is a boolean value indicating this is a parent (composite) copy job.
*/
public Boolean isParent() {
return this.isParent;
}
/**
*
* This is the identifier of a resource within a composite group, such as nested (child) recovery point belonging to
* a composite (parent) stack. The ID is transferred from the logical ID within a stack.
*
*
* @param compositeMemberIdentifier
* This is the identifier of a resource within a composite group, such as nested (child) recovery point
* belonging to a composite (parent) stack. The ID is transferred from the logical ID within a stack.
*/
public void setCompositeMemberIdentifier(String compositeMemberIdentifier) {
this.compositeMemberIdentifier = compositeMemberIdentifier;
}
/**
*
* This is the identifier of a resource within a composite group, such as nested (child) recovery point belonging to
* a composite (parent) stack. The ID is transferred from the logical ID within a stack.
*
*
* @return This is the identifier of a resource within a composite group, such as nested (child) recovery point
* belonging to a composite (parent) stack. The ID is transferred from the logical ID within a stack.
*/
public String getCompositeMemberIdentifier() {
return this.compositeMemberIdentifier;
}
/**
*
* This is the identifier of a resource within a composite group, such as nested (child) recovery point belonging to
* a composite (parent) stack. The ID is transferred from the logical ID within a stack.
*
*
* @param compositeMemberIdentifier
* This is the identifier of a resource within a composite group, such as nested (child) recovery point
* belonging to a composite (parent) stack. The ID is transferred from the logical ID within a stack.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob withCompositeMemberIdentifier(String compositeMemberIdentifier) {
setCompositeMemberIdentifier(compositeMemberIdentifier);
return this;
}
/**
*
* This is the number of child (nested) copy jobs.
*
*
* @param numberOfChildJobs
* This is the number of child (nested) copy jobs.
*/
public void setNumberOfChildJobs(Long numberOfChildJobs) {
this.numberOfChildJobs = numberOfChildJobs;
}
/**
*
* This is the number of child (nested) copy jobs.
*
*
* @return This is the number of child (nested) copy jobs.
*/
public Long getNumberOfChildJobs() {
return this.numberOfChildJobs;
}
/**
*
* This is the number of child (nested) copy jobs.
*
*
* @param numberOfChildJobs
* This is the number of child (nested) copy jobs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob withNumberOfChildJobs(Long numberOfChildJobs) {
setNumberOfChildJobs(numberOfChildJobs);
return this;
}
/**
*
* This returns the statistics of the included child (nested) copy jobs.
*
*
* @return This returns the statistics of the included child (nested) copy jobs.
*/
public java.util.Map getChildJobsInState() {
return childJobsInState;
}
/**
*
* This returns the statistics of the included child (nested) copy jobs.
*
*
* @param childJobsInState
* This returns the statistics of the included child (nested) copy jobs.
*/
public void setChildJobsInState(java.util.Map childJobsInState) {
this.childJobsInState = childJobsInState;
}
/**
*
* This returns the statistics of the included child (nested) copy jobs.
*
*
* @param childJobsInState
* This returns the statistics of the included child (nested) copy jobs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob withChildJobsInState(java.util.Map childJobsInState) {
setChildJobsInState(childJobsInState);
return this;
}
/**
* Add a single ChildJobsInState entry
*
* @see CopyJob#withChildJobsInState
* @returns a reference to this object so that method calls can be chained together.
*/
public CopyJob addChildJobsInStateEntry(String key, Long value) {
if (null == this.childJobsInState) {
this.childJobsInState = new java.util.HashMap();
}
if (this.childJobsInState.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.childJobsInState.put(key, value);
return this;
}
/**
* Removes all the entries added into ChildJobsInState.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob clearChildJobsInStateEntries() {
this.childJobsInState = null;
return this;
}
/**
*
* This is the non-unique name of the resource that belongs to the specified backup.
*
*
* @param resourceName
* This is the non-unique name of the resource that belongs to the specified backup.
*/
public void setResourceName(String resourceName) {
this.resourceName = resourceName;
}
/**
*
* This is the non-unique name of the resource that belongs to the specified backup.
*
*
* @return This is the non-unique name of the resource that belongs to the specified backup.
*/
public String getResourceName() {
return this.resourceName;
}
/**
*
* This is the non-unique name of the resource that belongs to the specified backup.
*
*
* @param resourceName
* This is the non-unique name of the resource that belongs to the specified backup.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob withResourceName(String resourceName) {
setResourceName(resourceName);
return this;
}
/**
*
* This parameter is the job count for the specified message category.
*
*
* Example strings may include AccessDenied
, SUCCESS
, AGGREGATE_ALL
, and
* InvalidParameters
. See Monitoring for a list of
* MessageCategory strings.
*
*
* The the value ANY returns count of all message categories.
*
*
* AGGREGATE_ALL
aggregates job counts for all message categories and returns the sum
*
*
* @param messageCategory
* This parameter is the job count for the specified message category.
*
* Example strings may include AccessDenied
, SUCCESS
, AGGREGATE_ALL
,
* and InvalidParameters
. See Monitoring for a list of
* MessageCategory strings.
*
*
* The the value ANY returns count of all message categories.
*
*
* AGGREGATE_ALL
aggregates job counts for all message categories and returns the sum
*/
public void setMessageCategory(String messageCategory) {
this.messageCategory = messageCategory;
}
/**
*
* This parameter is the job count for the specified message category.
*
*
* Example strings may include AccessDenied
, SUCCESS
, AGGREGATE_ALL
, and
* InvalidParameters
. See Monitoring for a list of
* MessageCategory strings.
*
*
* The the value ANY returns count of all message categories.
*
*
* AGGREGATE_ALL
aggregates job counts for all message categories and returns the sum
*
*
* @return This parameter is the job count for the specified message category.
*
* Example strings may include AccessDenied
, SUCCESS
, AGGREGATE_ALL
,
* and InvalidParameters
. See Monitoring for a list
* of MessageCategory strings.
*
*
* The the value ANY returns count of all message categories.
*
*
* AGGREGATE_ALL
aggregates job counts for all message categories and returns the sum
*/
public String getMessageCategory() {
return this.messageCategory;
}
/**
*
* This parameter is the job count for the specified message category.
*
*
* Example strings may include AccessDenied
, SUCCESS
, AGGREGATE_ALL
, and
* InvalidParameters
. See Monitoring for a list of
* MessageCategory strings.
*
*
* The the value ANY returns count of all message categories.
*
*
* AGGREGATE_ALL
aggregates job counts for all message categories and returns the sum
*
*
* @param messageCategory
* This parameter is the job count for the specified message category.
*
* Example strings may include AccessDenied
, SUCCESS
, AGGREGATE_ALL
,
* and InvalidParameters
. See Monitoring for a list of
* MessageCategory strings.
*
*
* The the value ANY returns count of all message categories.
*
*
* AGGREGATE_ALL
aggregates job counts for all message categories and returns the sum
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyJob withMessageCategory(String messageCategory) {
setMessageCategory(messageCategory);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAccountId() != null)
sb.append("AccountId: ").append(getAccountId()).append(",");
if (getCopyJobId() != null)
sb.append("CopyJobId: ").append(getCopyJobId()).append(",");
if (getSourceBackupVaultArn() != null)
sb.append("SourceBackupVaultArn: ").append(getSourceBackupVaultArn()).append(",");
if (getSourceRecoveryPointArn() != null)
sb.append("SourceRecoveryPointArn: ").append(getSourceRecoveryPointArn()).append(",");
if (getDestinationBackupVaultArn() != null)
sb.append("DestinationBackupVaultArn: ").append(getDestinationBackupVaultArn()).append(",");
if (getDestinationRecoveryPointArn() != null)
sb.append("DestinationRecoveryPointArn: ").append(getDestinationRecoveryPointArn()).append(",");
if (getResourceArn() != null)
sb.append("ResourceArn: ").append(getResourceArn()).append(",");
if (getCreationDate() != null)
sb.append("CreationDate: ").append(getCreationDate()).append(",");
if (getCompletionDate() != null)
sb.append("CompletionDate: ").append(getCompletionDate()).append(",");
if (getState() != null)
sb.append("State: ").append(getState()).append(",");
if (getStatusMessage() != null)
sb.append("StatusMessage: ").append(getStatusMessage()).append(",");
if (getBackupSizeInBytes() != null)
sb.append("BackupSizeInBytes: ").append(getBackupSizeInBytes()).append(",");
if (getIamRoleArn() != null)
sb.append("IamRoleArn: ").append(getIamRoleArn()).append(",");
if (getCreatedBy() != null)
sb.append("CreatedBy: ").append(getCreatedBy()).append(",");
if (getResourceType() != null)
sb.append("ResourceType: ").append(getResourceType()).append(",");
if (getParentJobId() != null)
sb.append("ParentJobId: ").append(getParentJobId()).append(",");
if (getIsParent() != null)
sb.append("IsParent: ").append(getIsParent()).append(",");
if (getCompositeMemberIdentifier() != null)
sb.append("CompositeMemberIdentifier: ").append(getCompositeMemberIdentifier()).append(",");
if (getNumberOfChildJobs() != null)
sb.append("NumberOfChildJobs: ").append(getNumberOfChildJobs()).append(",");
if (getChildJobsInState() != null)
sb.append("ChildJobsInState: ").append(getChildJobsInState()).append(",");
if (getResourceName() != null)
sb.append("ResourceName: ").append(getResourceName()).append(",");
if (getMessageCategory() != null)
sb.append("MessageCategory: ").append(getMessageCategory());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CopyJob == false)
return false;
CopyJob other = (CopyJob) obj;
if (other.getAccountId() == null ^ this.getAccountId() == null)
return false;
if (other.getAccountId() != null && other.getAccountId().equals(this.getAccountId()) == false)
return false;
if (other.getCopyJobId() == null ^ this.getCopyJobId() == null)
return false;
if (other.getCopyJobId() != null && other.getCopyJobId().equals(this.getCopyJobId()) == false)
return false;
if (other.getSourceBackupVaultArn() == null ^ this.getSourceBackupVaultArn() == null)
return false;
if (other.getSourceBackupVaultArn() != null && other.getSourceBackupVaultArn().equals(this.getSourceBackupVaultArn()) == false)
return false;
if (other.getSourceRecoveryPointArn() == null ^ this.getSourceRecoveryPointArn() == null)
return false;
if (other.getSourceRecoveryPointArn() != null && other.getSourceRecoveryPointArn().equals(this.getSourceRecoveryPointArn()) == false)
return false;
if (other.getDestinationBackupVaultArn() == null ^ this.getDestinationBackupVaultArn() == null)
return false;
if (other.getDestinationBackupVaultArn() != null && other.getDestinationBackupVaultArn().equals(this.getDestinationBackupVaultArn()) == false)
return false;
if (other.getDestinationRecoveryPointArn() == null ^ this.getDestinationRecoveryPointArn() == null)
return false;
if (other.getDestinationRecoveryPointArn() != null && other.getDestinationRecoveryPointArn().equals(this.getDestinationRecoveryPointArn()) == false)
return false;
if (other.getResourceArn() == null ^ this.getResourceArn() == null)
return false;
if (other.getResourceArn() != null && other.getResourceArn().equals(this.getResourceArn()) == false)
return false;
if (other.getCreationDate() == null ^ this.getCreationDate() == null)
return false;
if (other.getCreationDate() != null && other.getCreationDate().equals(this.getCreationDate()) == false)
return false;
if (other.getCompletionDate() == null ^ this.getCompletionDate() == null)
return false;
if (other.getCompletionDate() != null && other.getCompletionDate().equals(this.getCompletionDate()) == false)
return false;
if (other.getState() == null ^ this.getState() == null)
return false;
if (other.getState() != null && other.getState().equals(this.getState()) == false)
return false;
if (other.getStatusMessage() == null ^ this.getStatusMessage() == null)
return false;
if (other.getStatusMessage() != null && other.getStatusMessage().equals(this.getStatusMessage()) == false)
return false;
if (other.getBackupSizeInBytes() == null ^ this.getBackupSizeInBytes() == null)
return false;
if (other.getBackupSizeInBytes() != null && other.getBackupSizeInBytes().equals(this.getBackupSizeInBytes()) == false)
return false;
if (other.getIamRoleArn() == null ^ this.getIamRoleArn() == null)
return false;
if (other.getIamRoleArn() != null && other.getIamRoleArn().equals(this.getIamRoleArn()) == false)
return false;
if (other.getCreatedBy() == null ^ this.getCreatedBy() == null)
return false;
if (other.getCreatedBy() != null && other.getCreatedBy().equals(this.getCreatedBy()) == false)
return false;
if (other.getResourceType() == null ^ this.getResourceType() == null)
return false;
if (other.getResourceType() != null && other.getResourceType().equals(this.getResourceType()) == false)
return false;
if (other.getParentJobId() == null ^ this.getParentJobId() == null)
return false;
if (other.getParentJobId() != null && other.getParentJobId().equals(this.getParentJobId()) == false)
return false;
if (other.getIsParent() == null ^ this.getIsParent() == null)
return false;
if (other.getIsParent() != null && other.getIsParent().equals(this.getIsParent()) == false)
return false;
if (other.getCompositeMemberIdentifier() == null ^ this.getCompositeMemberIdentifier() == null)
return false;
if (other.getCompositeMemberIdentifier() != null && other.getCompositeMemberIdentifier().equals(this.getCompositeMemberIdentifier()) == false)
return false;
if (other.getNumberOfChildJobs() == null ^ this.getNumberOfChildJobs() == null)
return false;
if (other.getNumberOfChildJobs() != null && other.getNumberOfChildJobs().equals(this.getNumberOfChildJobs()) == false)
return false;
if (other.getChildJobsInState() == null ^ this.getChildJobsInState() == null)
return false;
if (other.getChildJobsInState() != null && other.getChildJobsInState().equals(this.getChildJobsInState()) == false)
return false;
if (other.getResourceName() == null ^ this.getResourceName() == null)
return false;
if (other.getResourceName() != null && other.getResourceName().equals(this.getResourceName()) == false)
return false;
if (other.getMessageCategory() == null ^ this.getMessageCategory() == null)
return false;
if (other.getMessageCategory() != null && other.getMessageCategory().equals(this.getMessageCategory()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAccountId() == null) ? 0 : getAccountId().hashCode());
hashCode = prime * hashCode + ((getCopyJobId() == null) ? 0 : getCopyJobId().hashCode());
hashCode = prime * hashCode + ((getSourceBackupVaultArn() == null) ? 0 : getSourceBackupVaultArn().hashCode());
hashCode = prime * hashCode + ((getSourceRecoveryPointArn() == null) ? 0 : getSourceRecoveryPointArn().hashCode());
hashCode = prime * hashCode + ((getDestinationBackupVaultArn() == null) ? 0 : getDestinationBackupVaultArn().hashCode());
hashCode = prime * hashCode + ((getDestinationRecoveryPointArn() == null) ? 0 : getDestinationRecoveryPointArn().hashCode());
hashCode = prime * hashCode + ((getResourceArn() == null) ? 0 : getResourceArn().hashCode());
hashCode = prime * hashCode + ((getCreationDate() == null) ? 0 : getCreationDate().hashCode());
hashCode = prime * hashCode + ((getCompletionDate() == null) ? 0 : getCompletionDate().hashCode());
hashCode = prime * hashCode + ((getState() == null) ? 0 : getState().hashCode());
hashCode = prime * hashCode + ((getStatusMessage() == null) ? 0 : getStatusMessage().hashCode());
hashCode = prime * hashCode + ((getBackupSizeInBytes() == null) ? 0 : getBackupSizeInBytes().hashCode());
hashCode = prime * hashCode + ((getIamRoleArn() == null) ? 0 : getIamRoleArn().hashCode());
hashCode = prime * hashCode + ((getCreatedBy() == null) ? 0 : getCreatedBy().hashCode());
hashCode = prime * hashCode + ((getResourceType() == null) ? 0 : getResourceType().hashCode());
hashCode = prime * hashCode + ((getParentJobId() == null) ? 0 : getParentJobId().hashCode());
hashCode = prime * hashCode + ((getIsParent() == null) ? 0 : getIsParent().hashCode());
hashCode = prime * hashCode + ((getCompositeMemberIdentifier() == null) ? 0 : getCompositeMemberIdentifier().hashCode());
hashCode = prime * hashCode + ((getNumberOfChildJobs() == null) ? 0 : getNumberOfChildJobs().hashCode());
hashCode = prime * hashCode + ((getChildJobsInState() == null) ? 0 : getChildJobsInState().hashCode());
hashCode = prime * hashCode + ((getResourceName() == null) ? 0 : getResourceName().hashCode());
hashCode = prime * hashCode + ((getMessageCategory() == null) ? 0 : getMessageCategory().hashCode());
return hashCode;
}
@Override
public CopyJob clone() {
try {
return (CopyJob) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.backup.model.transform.CopyJobMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}