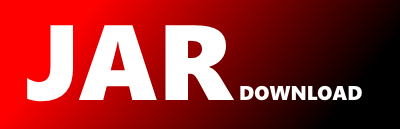
com.amazonaws.services.backup.model.GetRecoveryPointRestoreMetadataResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-backup Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.backup.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetRecoveryPointRestoreMetadataResult extends com.amazonaws.AmazonWebServiceResult implements Serializable,
Cloneable {
/**
*
* An ARN that uniquely identifies a backup vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*
*/
private String backupVaultArn;
/**
*
* An ARN that uniquely identifies a recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*
*/
private String recoveryPointArn;
/**
*
* The set of metadata key-value pairs that describe the original configuration of the backed-up resource. These
* values vary depending on the service that is being restored.
*
*/
private java.util.Map restoreMetadata;
/**
*
* This is the resource type associated with the recovery point.
*
*/
private String resourceType;
/**
*
* An ARN that uniquely identifies a backup vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*
*
* @param backupVaultArn
* An ARN that uniquely identifies a backup vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*/
public void setBackupVaultArn(String backupVaultArn) {
this.backupVaultArn = backupVaultArn;
}
/**
*
* An ARN that uniquely identifies a backup vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*
*
* @return An ARN that uniquely identifies a backup vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*/
public String getBackupVaultArn() {
return this.backupVaultArn;
}
/**
*
* An ARN that uniquely identifies a backup vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*
*
* @param backupVaultArn
* An ARN that uniquely identifies a backup vault; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetRecoveryPointRestoreMetadataResult withBackupVaultArn(String backupVaultArn) {
setBackupVaultArn(backupVaultArn);
return this;
}
/**
*
* An ARN that uniquely identifies a recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*
*
* @param recoveryPointArn
* An ARN that uniquely identifies a recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*/
public void setRecoveryPointArn(String recoveryPointArn) {
this.recoveryPointArn = recoveryPointArn;
}
/**
*
* An ARN that uniquely identifies a recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*
*
* @return An ARN that uniquely identifies a recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*/
public String getRecoveryPointArn() {
return this.recoveryPointArn;
}
/**
*
* An ARN that uniquely identifies a recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
*
*
* @param recoveryPointArn
* An ARN that uniquely identifies a recovery point; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetRecoveryPointRestoreMetadataResult withRecoveryPointArn(String recoveryPointArn) {
setRecoveryPointArn(recoveryPointArn);
return this;
}
/**
*
* The set of metadata key-value pairs that describe the original configuration of the backed-up resource. These
* values vary depending on the service that is being restored.
*
*
* @return The set of metadata key-value pairs that describe the original configuration of the backed-up resource.
* These values vary depending on the service that is being restored.
*/
public java.util.Map getRestoreMetadata() {
return restoreMetadata;
}
/**
*
* The set of metadata key-value pairs that describe the original configuration of the backed-up resource. These
* values vary depending on the service that is being restored.
*
*
* @param restoreMetadata
* The set of metadata key-value pairs that describe the original configuration of the backed-up resource.
* These values vary depending on the service that is being restored.
*/
public void setRestoreMetadata(java.util.Map restoreMetadata) {
this.restoreMetadata = restoreMetadata;
}
/**
*
* The set of metadata key-value pairs that describe the original configuration of the backed-up resource. These
* values vary depending on the service that is being restored.
*
*
* @param restoreMetadata
* The set of metadata key-value pairs that describe the original configuration of the backed-up resource.
* These values vary depending on the service that is being restored.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetRecoveryPointRestoreMetadataResult withRestoreMetadata(java.util.Map restoreMetadata) {
setRestoreMetadata(restoreMetadata);
return this;
}
/**
* Add a single RestoreMetadata entry
*
* @see GetRecoveryPointRestoreMetadataResult#withRestoreMetadata
* @returns a reference to this object so that method calls can be chained together.
*/
public GetRecoveryPointRestoreMetadataResult addRestoreMetadataEntry(String key, String value) {
if (null == this.restoreMetadata) {
this.restoreMetadata = new java.util.HashMap();
}
if (this.restoreMetadata.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.restoreMetadata.put(key, value);
return this;
}
/**
* Removes all the entries added into RestoreMetadata.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetRecoveryPointRestoreMetadataResult clearRestoreMetadataEntries() {
this.restoreMetadata = null;
return this;
}
/**
*
* This is the resource type associated with the recovery point.
*
*
* @param resourceType
* This is the resource type associated with the recovery point.
*/
public void setResourceType(String resourceType) {
this.resourceType = resourceType;
}
/**
*
* This is the resource type associated with the recovery point.
*
*
* @return This is the resource type associated with the recovery point.
*/
public String getResourceType() {
return this.resourceType;
}
/**
*
* This is the resource type associated with the recovery point.
*
*
* @param resourceType
* This is the resource type associated with the recovery point.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetRecoveryPointRestoreMetadataResult withResourceType(String resourceType) {
setResourceType(resourceType);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getBackupVaultArn() != null)
sb.append("BackupVaultArn: ").append(getBackupVaultArn()).append(",");
if (getRecoveryPointArn() != null)
sb.append("RecoveryPointArn: ").append(getRecoveryPointArn()).append(",");
if (getRestoreMetadata() != null)
sb.append("RestoreMetadata: ").append("***Sensitive Data Redacted***").append(",");
if (getResourceType() != null)
sb.append("ResourceType: ").append(getResourceType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetRecoveryPointRestoreMetadataResult == false)
return false;
GetRecoveryPointRestoreMetadataResult other = (GetRecoveryPointRestoreMetadataResult) obj;
if (other.getBackupVaultArn() == null ^ this.getBackupVaultArn() == null)
return false;
if (other.getBackupVaultArn() != null && other.getBackupVaultArn().equals(this.getBackupVaultArn()) == false)
return false;
if (other.getRecoveryPointArn() == null ^ this.getRecoveryPointArn() == null)
return false;
if (other.getRecoveryPointArn() != null && other.getRecoveryPointArn().equals(this.getRecoveryPointArn()) == false)
return false;
if (other.getRestoreMetadata() == null ^ this.getRestoreMetadata() == null)
return false;
if (other.getRestoreMetadata() != null && other.getRestoreMetadata().equals(this.getRestoreMetadata()) == false)
return false;
if (other.getResourceType() == null ^ this.getResourceType() == null)
return false;
if (other.getResourceType() != null && other.getResourceType().equals(this.getResourceType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getBackupVaultArn() == null) ? 0 : getBackupVaultArn().hashCode());
hashCode = prime * hashCode + ((getRecoveryPointArn() == null) ? 0 : getRecoveryPointArn().hashCode());
hashCode = prime * hashCode + ((getRestoreMetadata() == null) ? 0 : getRestoreMetadata().hashCode());
hashCode = prime * hashCode + ((getResourceType() == null) ? 0 : getResourceType().hashCode());
return hashCode;
}
@Override
public GetRecoveryPointRestoreMetadataResult clone() {
try {
return (GetRecoveryPointRestoreMetadataResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}