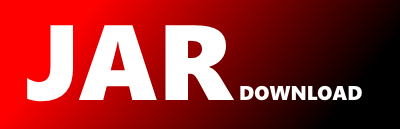
com.amazonaws.services.backup.model.ListRestoreJobsRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-backup Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.backup.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ListRestoreJobsRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The next item following a partial list of returned items. For example, if a request is made to return
* MaxResults
number of items, NextToken
allows you to return more items in your list
* starting at the location pointed to by the next token.
*
*/
private String nextToken;
/**
*
* The maximum number of items to be returned.
*
*/
private Integer maxResults;
/**
*
* The account ID to list the jobs from. Returns only restore jobs associated with the specified account ID.
*
*/
private String byAccountId;
/**
*
* Include this parameter to return only restore jobs for the specified resources:
*
*
* -
*
* Aurora
for Amazon Aurora
*
*
* -
*
* CloudFormation
for CloudFormation
*
*
* -
*
* DocumentDB
for Amazon DocumentDB (with MongoDB compatibility)
*
*
* -
*
* DynamoDB
for Amazon DynamoDB
*
*
* -
*
* EBS
for Amazon Elastic Block Store
*
*
* -
*
* EC2
for Amazon Elastic Compute Cloud
*
*
* -
*
* EFS
for Amazon Elastic File System
*
*
* -
*
* FSx
for Amazon FSx
*
*
* -
*
* Neptune
for Amazon Neptune
*
*
* -
*
* Redshift
for Amazon Redshift
*
*
* -
*
* RDS
for Amazon Relational Database Service
*
*
* -
*
* SAP HANA on Amazon EC2
for SAP HANA databases
*
*
* -
*
* Storage Gateway
for Storage Gateway
*
*
* -
*
* S3
for Amazon S3
*
*
* -
*
* Timestream
for Amazon Timestream
*
*
* -
*
* VirtualMachine
for virtual machines
*
*
*
*/
private String byResourceType;
/**
*
* Returns only restore jobs that were created before the specified date.
*
*/
private java.util.Date byCreatedBefore;
/**
*
* Returns only restore jobs that were created after the specified date.
*
*/
private java.util.Date byCreatedAfter;
/**
*
* Returns only restore jobs associated with the specified job status.
*
*/
private String byStatus;
/**
*
* Returns only copy jobs completed before a date expressed in Unix format and Coordinated Universal Time (UTC).
*
*/
private java.util.Date byCompleteBefore;
/**
*
* Returns only copy jobs completed after a date expressed in Unix format and Coordinated Universal Time (UTC).
*
*/
private java.util.Date byCompleteAfter;
/**
*
* This returns only restore testing jobs that match the specified resource Amazon Resource Name (ARN).
*
*/
private String byRestoreTestingPlanArn;
/**
*
* The next item following a partial list of returned items. For example, if a request is made to return
* MaxResults
number of items, NextToken
allows you to return more items in your list
* starting at the location pointed to by the next token.
*
*
* @param nextToken
* The next item following a partial list of returned items. For example, if a request is made to return
* MaxResults
number of items, NextToken
allows you to return more items in your
* list starting at the location pointed to by the next token.
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
*
* The next item following a partial list of returned items. For example, if a request is made to return
* MaxResults
number of items, NextToken
allows you to return more items in your list
* starting at the location pointed to by the next token.
*
*
* @return The next item following a partial list of returned items. For example, if a request is made to return
* MaxResults
number of items, NextToken
allows you to return more items in your
* list starting at the location pointed to by the next token.
*/
public String getNextToken() {
return this.nextToken;
}
/**
*
* The next item following a partial list of returned items. For example, if a request is made to return
* MaxResults
number of items, NextToken
allows you to return more items in your list
* starting at the location pointed to by the next token.
*
*
* @param nextToken
* The next item following a partial list of returned items. For example, if a request is made to return
* MaxResults
number of items, NextToken
allows you to return more items in your
* list starting at the location pointed to by the next token.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListRestoreJobsRequest withNextToken(String nextToken) {
setNextToken(nextToken);
return this;
}
/**
*
* The maximum number of items to be returned.
*
*
* @param maxResults
* The maximum number of items to be returned.
*/
public void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
/**
*
* The maximum number of items to be returned.
*
*
* @return The maximum number of items to be returned.
*/
public Integer getMaxResults() {
return this.maxResults;
}
/**
*
* The maximum number of items to be returned.
*
*
* @param maxResults
* The maximum number of items to be returned.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListRestoreJobsRequest withMaxResults(Integer maxResults) {
setMaxResults(maxResults);
return this;
}
/**
*
* The account ID to list the jobs from. Returns only restore jobs associated with the specified account ID.
*
*
* @param byAccountId
* The account ID to list the jobs from. Returns only restore jobs associated with the specified account ID.
*/
public void setByAccountId(String byAccountId) {
this.byAccountId = byAccountId;
}
/**
*
* The account ID to list the jobs from. Returns only restore jobs associated with the specified account ID.
*
*
* @return The account ID to list the jobs from. Returns only restore jobs associated with the specified account ID.
*/
public String getByAccountId() {
return this.byAccountId;
}
/**
*
* The account ID to list the jobs from. Returns only restore jobs associated with the specified account ID.
*
*
* @param byAccountId
* The account ID to list the jobs from. Returns only restore jobs associated with the specified account ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListRestoreJobsRequest withByAccountId(String byAccountId) {
setByAccountId(byAccountId);
return this;
}
/**
*
* Include this parameter to return only restore jobs for the specified resources:
*
*
* -
*
* Aurora
for Amazon Aurora
*
*
* -
*
* CloudFormation
for CloudFormation
*
*
* -
*
* DocumentDB
for Amazon DocumentDB (with MongoDB compatibility)
*
*
* -
*
* DynamoDB
for Amazon DynamoDB
*
*
* -
*
* EBS
for Amazon Elastic Block Store
*
*
* -
*
* EC2
for Amazon Elastic Compute Cloud
*
*
* -
*
* EFS
for Amazon Elastic File System
*
*
* -
*
* FSx
for Amazon FSx
*
*
* -
*
* Neptune
for Amazon Neptune
*
*
* -
*
* Redshift
for Amazon Redshift
*
*
* -
*
* RDS
for Amazon Relational Database Service
*
*
* -
*
* SAP HANA on Amazon EC2
for SAP HANA databases
*
*
* -
*
* Storage Gateway
for Storage Gateway
*
*
* -
*
* S3
for Amazon S3
*
*
* -
*
* Timestream
for Amazon Timestream
*
*
* -
*
* VirtualMachine
for virtual machines
*
*
*
*
* @param byResourceType
* Include this parameter to return only restore jobs for the specified resources:
*
* -
*
* Aurora
for Amazon Aurora
*
*
* -
*
* CloudFormation
for CloudFormation
*
*
* -
*
* DocumentDB
for Amazon DocumentDB (with MongoDB compatibility)
*
*
* -
*
* DynamoDB
for Amazon DynamoDB
*
*
* -
*
* EBS
for Amazon Elastic Block Store
*
*
* -
*
* EC2
for Amazon Elastic Compute Cloud
*
*
* -
*
* EFS
for Amazon Elastic File System
*
*
* -
*
* FSx
for Amazon FSx
*
*
* -
*
* Neptune
for Amazon Neptune
*
*
* -
*
* Redshift
for Amazon Redshift
*
*
* -
*
* RDS
for Amazon Relational Database Service
*
*
* -
*
* SAP HANA on Amazon EC2
for SAP HANA databases
*
*
* -
*
* Storage Gateway
for Storage Gateway
*
*
* -
*
* S3
for Amazon S3
*
*
* -
*
* Timestream
for Amazon Timestream
*
*
* -
*
* VirtualMachine
for virtual machines
*
*
*/
public void setByResourceType(String byResourceType) {
this.byResourceType = byResourceType;
}
/**
*
* Include this parameter to return only restore jobs for the specified resources:
*
*
* -
*
* Aurora
for Amazon Aurora
*
*
* -
*
* CloudFormation
for CloudFormation
*
*
* -
*
* DocumentDB
for Amazon DocumentDB (with MongoDB compatibility)
*
*
* -
*
* DynamoDB
for Amazon DynamoDB
*
*
* -
*
* EBS
for Amazon Elastic Block Store
*
*
* -
*
* EC2
for Amazon Elastic Compute Cloud
*
*
* -
*
* EFS
for Amazon Elastic File System
*
*
* -
*
* FSx
for Amazon FSx
*
*
* -
*
* Neptune
for Amazon Neptune
*
*
* -
*
* Redshift
for Amazon Redshift
*
*
* -
*
* RDS
for Amazon Relational Database Service
*
*
* -
*
* SAP HANA on Amazon EC2
for SAP HANA databases
*
*
* -
*
* Storage Gateway
for Storage Gateway
*
*
* -
*
* S3
for Amazon S3
*
*
* -
*
* Timestream
for Amazon Timestream
*
*
* -
*
* VirtualMachine
for virtual machines
*
*
*
*
* @return Include this parameter to return only restore jobs for the specified resources:
*
* -
*
* Aurora
for Amazon Aurora
*
*
* -
*
* CloudFormation
for CloudFormation
*
*
* -
*
* DocumentDB
for Amazon DocumentDB (with MongoDB compatibility)
*
*
* -
*
* DynamoDB
for Amazon DynamoDB
*
*
* -
*
* EBS
for Amazon Elastic Block Store
*
*
* -
*
* EC2
for Amazon Elastic Compute Cloud
*
*
* -
*
* EFS
for Amazon Elastic File System
*
*
* -
*
* FSx
for Amazon FSx
*
*
* -
*
* Neptune
for Amazon Neptune
*
*
* -
*
* Redshift
for Amazon Redshift
*
*
* -
*
* RDS
for Amazon Relational Database Service
*
*
* -
*
* SAP HANA on Amazon EC2
for SAP HANA databases
*
*
* -
*
* Storage Gateway
for Storage Gateway
*
*
* -
*
* S3
for Amazon S3
*
*
* -
*
* Timestream
for Amazon Timestream
*
*
* -
*
* VirtualMachine
for virtual machines
*
*
*/
public String getByResourceType() {
return this.byResourceType;
}
/**
*
* Include this parameter to return only restore jobs for the specified resources:
*
*
* -
*
* Aurora
for Amazon Aurora
*
*
* -
*
* CloudFormation
for CloudFormation
*
*
* -
*
* DocumentDB
for Amazon DocumentDB (with MongoDB compatibility)
*
*
* -
*
* DynamoDB
for Amazon DynamoDB
*
*
* -
*
* EBS
for Amazon Elastic Block Store
*
*
* -
*
* EC2
for Amazon Elastic Compute Cloud
*
*
* -
*
* EFS
for Amazon Elastic File System
*
*
* -
*
* FSx
for Amazon FSx
*
*
* -
*
* Neptune
for Amazon Neptune
*
*
* -
*
* Redshift
for Amazon Redshift
*
*
* -
*
* RDS
for Amazon Relational Database Service
*
*
* -
*
* SAP HANA on Amazon EC2
for SAP HANA databases
*
*
* -
*
* Storage Gateway
for Storage Gateway
*
*
* -
*
* S3
for Amazon S3
*
*
* -
*
* Timestream
for Amazon Timestream
*
*
* -
*
* VirtualMachine
for virtual machines
*
*
*
*
* @param byResourceType
* Include this parameter to return only restore jobs for the specified resources:
*
* -
*
* Aurora
for Amazon Aurora
*
*
* -
*
* CloudFormation
for CloudFormation
*
*
* -
*
* DocumentDB
for Amazon DocumentDB (with MongoDB compatibility)
*
*
* -
*
* DynamoDB
for Amazon DynamoDB
*
*
* -
*
* EBS
for Amazon Elastic Block Store
*
*
* -
*
* EC2
for Amazon Elastic Compute Cloud
*
*
* -
*
* EFS
for Amazon Elastic File System
*
*
* -
*
* FSx
for Amazon FSx
*
*
* -
*
* Neptune
for Amazon Neptune
*
*
* -
*
* Redshift
for Amazon Redshift
*
*
* -
*
* RDS
for Amazon Relational Database Service
*
*
* -
*
* SAP HANA on Amazon EC2
for SAP HANA databases
*
*
* -
*
* Storage Gateway
for Storage Gateway
*
*
* -
*
* S3
for Amazon S3
*
*
* -
*
* Timestream
for Amazon Timestream
*
*
* -
*
* VirtualMachine
for virtual machines
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListRestoreJobsRequest withByResourceType(String byResourceType) {
setByResourceType(byResourceType);
return this;
}
/**
*
* Returns only restore jobs that were created before the specified date.
*
*
* @param byCreatedBefore
* Returns only restore jobs that were created before the specified date.
*/
public void setByCreatedBefore(java.util.Date byCreatedBefore) {
this.byCreatedBefore = byCreatedBefore;
}
/**
*
* Returns only restore jobs that were created before the specified date.
*
*
* @return Returns only restore jobs that were created before the specified date.
*/
public java.util.Date getByCreatedBefore() {
return this.byCreatedBefore;
}
/**
*
* Returns only restore jobs that were created before the specified date.
*
*
* @param byCreatedBefore
* Returns only restore jobs that were created before the specified date.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListRestoreJobsRequest withByCreatedBefore(java.util.Date byCreatedBefore) {
setByCreatedBefore(byCreatedBefore);
return this;
}
/**
*
* Returns only restore jobs that were created after the specified date.
*
*
* @param byCreatedAfter
* Returns only restore jobs that were created after the specified date.
*/
public void setByCreatedAfter(java.util.Date byCreatedAfter) {
this.byCreatedAfter = byCreatedAfter;
}
/**
*
* Returns only restore jobs that were created after the specified date.
*
*
* @return Returns only restore jobs that were created after the specified date.
*/
public java.util.Date getByCreatedAfter() {
return this.byCreatedAfter;
}
/**
*
* Returns only restore jobs that were created after the specified date.
*
*
* @param byCreatedAfter
* Returns only restore jobs that were created after the specified date.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListRestoreJobsRequest withByCreatedAfter(java.util.Date byCreatedAfter) {
setByCreatedAfter(byCreatedAfter);
return this;
}
/**
*
* Returns only restore jobs associated with the specified job status.
*
*
* @param byStatus
* Returns only restore jobs associated with the specified job status.
* @see RestoreJobStatus
*/
public void setByStatus(String byStatus) {
this.byStatus = byStatus;
}
/**
*
* Returns only restore jobs associated with the specified job status.
*
*
* @return Returns only restore jobs associated with the specified job status.
* @see RestoreJobStatus
*/
public String getByStatus() {
return this.byStatus;
}
/**
*
* Returns only restore jobs associated with the specified job status.
*
*
* @param byStatus
* Returns only restore jobs associated with the specified job status.
* @return Returns a reference to this object so that method calls can be chained together.
* @see RestoreJobStatus
*/
public ListRestoreJobsRequest withByStatus(String byStatus) {
setByStatus(byStatus);
return this;
}
/**
*
* Returns only restore jobs associated with the specified job status.
*
*
* @param byStatus
* Returns only restore jobs associated with the specified job status.
* @return Returns a reference to this object so that method calls can be chained together.
* @see RestoreJobStatus
*/
public ListRestoreJobsRequest withByStatus(RestoreJobStatus byStatus) {
this.byStatus = byStatus.toString();
return this;
}
/**
*
* Returns only copy jobs completed before a date expressed in Unix format and Coordinated Universal Time (UTC).
*
*
* @param byCompleteBefore
* Returns only copy jobs completed before a date expressed in Unix format and Coordinated Universal Time
* (UTC).
*/
public void setByCompleteBefore(java.util.Date byCompleteBefore) {
this.byCompleteBefore = byCompleteBefore;
}
/**
*
* Returns only copy jobs completed before a date expressed in Unix format and Coordinated Universal Time (UTC).
*
*
* @return Returns only copy jobs completed before a date expressed in Unix format and Coordinated Universal Time
* (UTC).
*/
public java.util.Date getByCompleteBefore() {
return this.byCompleteBefore;
}
/**
*
* Returns only copy jobs completed before a date expressed in Unix format and Coordinated Universal Time (UTC).
*
*
* @param byCompleteBefore
* Returns only copy jobs completed before a date expressed in Unix format and Coordinated Universal Time
* (UTC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListRestoreJobsRequest withByCompleteBefore(java.util.Date byCompleteBefore) {
setByCompleteBefore(byCompleteBefore);
return this;
}
/**
*
* Returns only copy jobs completed after a date expressed in Unix format and Coordinated Universal Time (UTC).
*
*
* @param byCompleteAfter
* Returns only copy jobs completed after a date expressed in Unix format and Coordinated Universal Time
* (UTC).
*/
public void setByCompleteAfter(java.util.Date byCompleteAfter) {
this.byCompleteAfter = byCompleteAfter;
}
/**
*
* Returns only copy jobs completed after a date expressed in Unix format and Coordinated Universal Time (UTC).
*
*
* @return Returns only copy jobs completed after a date expressed in Unix format and Coordinated Universal Time
* (UTC).
*/
public java.util.Date getByCompleteAfter() {
return this.byCompleteAfter;
}
/**
*
* Returns only copy jobs completed after a date expressed in Unix format and Coordinated Universal Time (UTC).
*
*
* @param byCompleteAfter
* Returns only copy jobs completed after a date expressed in Unix format and Coordinated Universal Time
* (UTC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListRestoreJobsRequest withByCompleteAfter(java.util.Date byCompleteAfter) {
setByCompleteAfter(byCompleteAfter);
return this;
}
/**
*
* This returns only restore testing jobs that match the specified resource Amazon Resource Name (ARN).
*
*
* @param byRestoreTestingPlanArn
* This returns only restore testing jobs that match the specified resource Amazon Resource Name (ARN).
*/
public void setByRestoreTestingPlanArn(String byRestoreTestingPlanArn) {
this.byRestoreTestingPlanArn = byRestoreTestingPlanArn;
}
/**
*
* This returns only restore testing jobs that match the specified resource Amazon Resource Name (ARN).
*
*
* @return This returns only restore testing jobs that match the specified resource Amazon Resource Name (ARN).
*/
public String getByRestoreTestingPlanArn() {
return this.byRestoreTestingPlanArn;
}
/**
*
* This returns only restore testing jobs that match the specified resource Amazon Resource Name (ARN).
*
*
* @param byRestoreTestingPlanArn
* This returns only restore testing jobs that match the specified resource Amazon Resource Name (ARN).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListRestoreJobsRequest withByRestoreTestingPlanArn(String byRestoreTestingPlanArn) {
setByRestoreTestingPlanArn(byRestoreTestingPlanArn);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getNextToken() != null)
sb.append("NextToken: ").append(getNextToken()).append(",");
if (getMaxResults() != null)
sb.append("MaxResults: ").append(getMaxResults()).append(",");
if (getByAccountId() != null)
sb.append("ByAccountId: ").append(getByAccountId()).append(",");
if (getByResourceType() != null)
sb.append("ByResourceType: ").append(getByResourceType()).append(",");
if (getByCreatedBefore() != null)
sb.append("ByCreatedBefore: ").append(getByCreatedBefore()).append(",");
if (getByCreatedAfter() != null)
sb.append("ByCreatedAfter: ").append(getByCreatedAfter()).append(",");
if (getByStatus() != null)
sb.append("ByStatus: ").append(getByStatus()).append(",");
if (getByCompleteBefore() != null)
sb.append("ByCompleteBefore: ").append(getByCompleteBefore()).append(",");
if (getByCompleteAfter() != null)
sb.append("ByCompleteAfter: ").append(getByCompleteAfter()).append(",");
if (getByRestoreTestingPlanArn() != null)
sb.append("ByRestoreTestingPlanArn: ").append(getByRestoreTestingPlanArn());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ListRestoreJobsRequest == false)
return false;
ListRestoreJobsRequest other = (ListRestoreJobsRequest) obj;
if (other.getNextToken() == null ^ this.getNextToken() == null)
return false;
if (other.getNextToken() != null && other.getNextToken().equals(this.getNextToken()) == false)
return false;
if (other.getMaxResults() == null ^ this.getMaxResults() == null)
return false;
if (other.getMaxResults() != null && other.getMaxResults().equals(this.getMaxResults()) == false)
return false;
if (other.getByAccountId() == null ^ this.getByAccountId() == null)
return false;
if (other.getByAccountId() != null && other.getByAccountId().equals(this.getByAccountId()) == false)
return false;
if (other.getByResourceType() == null ^ this.getByResourceType() == null)
return false;
if (other.getByResourceType() != null && other.getByResourceType().equals(this.getByResourceType()) == false)
return false;
if (other.getByCreatedBefore() == null ^ this.getByCreatedBefore() == null)
return false;
if (other.getByCreatedBefore() != null && other.getByCreatedBefore().equals(this.getByCreatedBefore()) == false)
return false;
if (other.getByCreatedAfter() == null ^ this.getByCreatedAfter() == null)
return false;
if (other.getByCreatedAfter() != null && other.getByCreatedAfter().equals(this.getByCreatedAfter()) == false)
return false;
if (other.getByStatus() == null ^ this.getByStatus() == null)
return false;
if (other.getByStatus() != null && other.getByStatus().equals(this.getByStatus()) == false)
return false;
if (other.getByCompleteBefore() == null ^ this.getByCompleteBefore() == null)
return false;
if (other.getByCompleteBefore() != null && other.getByCompleteBefore().equals(this.getByCompleteBefore()) == false)
return false;
if (other.getByCompleteAfter() == null ^ this.getByCompleteAfter() == null)
return false;
if (other.getByCompleteAfter() != null && other.getByCompleteAfter().equals(this.getByCompleteAfter()) == false)
return false;
if (other.getByRestoreTestingPlanArn() == null ^ this.getByRestoreTestingPlanArn() == null)
return false;
if (other.getByRestoreTestingPlanArn() != null && other.getByRestoreTestingPlanArn().equals(this.getByRestoreTestingPlanArn()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getNextToken() == null) ? 0 : getNextToken().hashCode());
hashCode = prime * hashCode + ((getMaxResults() == null) ? 0 : getMaxResults().hashCode());
hashCode = prime * hashCode + ((getByAccountId() == null) ? 0 : getByAccountId().hashCode());
hashCode = prime * hashCode + ((getByResourceType() == null) ? 0 : getByResourceType().hashCode());
hashCode = prime * hashCode + ((getByCreatedBefore() == null) ? 0 : getByCreatedBefore().hashCode());
hashCode = prime * hashCode + ((getByCreatedAfter() == null) ? 0 : getByCreatedAfter().hashCode());
hashCode = prime * hashCode + ((getByStatus() == null) ? 0 : getByStatus().hashCode());
hashCode = prime * hashCode + ((getByCompleteBefore() == null) ? 0 : getByCompleteBefore().hashCode());
hashCode = prime * hashCode + ((getByCompleteAfter() == null) ? 0 : getByCompleteAfter().hashCode());
hashCode = prime * hashCode + ((getByRestoreTestingPlanArn() == null) ? 0 : getByRestoreTestingPlanArn().hashCode());
return hashCode;
}
@Override
public ListRestoreJobsRequest clone() {
return (ListRestoreJobsRequest) super.clone();
}
}