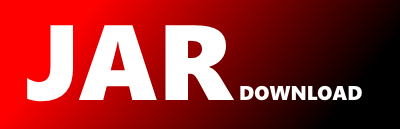
com.amazonaws.services.backup.model.StartCopyJobRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-backup Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.backup.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StartCopyJobRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* An ARN that uniquely identifies a recovery point to use for the copy job; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45.
*
*/
private String recoveryPointArn;
/**
*
* The name of a logical source container where backups are stored. Backup vaults are identified by names that are
* unique to the account used to create them and the Amazon Web Services Region where they are created. They consist
* of lowercase letters, numbers, and hyphens.
*
*/
private String sourceBackupVaultName;
/**
*
* An Amazon Resource Name (ARN) that uniquely identifies a destination backup vault to copy to; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*
*/
private String destinationBackupVaultArn;
/**
*
* Specifies the IAM role ARN used to copy the target recovery point; for example,
* arn:aws:iam::123456789012:role/S3Access
.
*
*/
private String iamRoleArn;
/**
*
* A customer-chosen string that you can use to distinguish between otherwise identical calls to
* StartCopyJob
. Retrying a successful request with the same idempotency token results in a success
* message with no action taken.
*
*/
private String idempotencyToken;
private Lifecycle lifecycle;
/**
*
* An ARN that uniquely identifies a recovery point to use for the copy job; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45.
*
*
* @param recoveryPointArn
* An ARN that uniquely identifies a recovery point to use for the copy job; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45.
*/
public void setRecoveryPointArn(String recoveryPointArn) {
this.recoveryPointArn = recoveryPointArn;
}
/**
*
* An ARN that uniquely identifies a recovery point to use for the copy job; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45.
*
*
* @return An ARN that uniquely identifies a recovery point to use for the copy job; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45.
*/
public String getRecoveryPointArn() {
return this.recoveryPointArn;
}
/**
*
* An ARN that uniquely identifies a recovery point to use for the copy job; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45.
*
*
* @param recoveryPointArn
* An ARN that uniquely identifies a recovery point to use for the copy job; for example,
* arn:aws:backup:us-east-1:123456789012:recovery-point:1EB3B5E7-9EB0-435A-A80B-108B488B0D45.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartCopyJobRequest withRecoveryPointArn(String recoveryPointArn) {
setRecoveryPointArn(recoveryPointArn);
return this;
}
/**
*
* The name of a logical source container where backups are stored. Backup vaults are identified by names that are
* unique to the account used to create them and the Amazon Web Services Region where they are created. They consist
* of lowercase letters, numbers, and hyphens.
*
*
* @param sourceBackupVaultName
* The name of a logical source container where backups are stored. Backup vaults are identified by names
* that are unique to the account used to create them and the Amazon Web Services Region where they are
* created. They consist of lowercase letters, numbers, and hyphens.
*/
public void setSourceBackupVaultName(String sourceBackupVaultName) {
this.sourceBackupVaultName = sourceBackupVaultName;
}
/**
*
* The name of a logical source container where backups are stored. Backup vaults are identified by names that are
* unique to the account used to create them and the Amazon Web Services Region where they are created. They consist
* of lowercase letters, numbers, and hyphens.
*
*
* @return The name of a logical source container where backups are stored. Backup vaults are identified by names
* that are unique to the account used to create them and the Amazon Web Services Region where they are
* created. They consist of lowercase letters, numbers, and hyphens.
*/
public String getSourceBackupVaultName() {
return this.sourceBackupVaultName;
}
/**
*
* The name of a logical source container where backups are stored. Backup vaults are identified by names that are
* unique to the account used to create them and the Amazon Web Services Region where they are created. They consist
* of lowercase letters, numbers, and hyphens.
*
*
* @param sourceBackupVaultName
* The name of a logical source container where backups are stored. Backup vaults are identified by names
* that are unique to the account used to create them and the Amazon Web Services Region where they are
* created. They consist of lowercase letters, numbers, and hyphens.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartCopyJobRequest withSourceBackupVaultName(String sourceBackupVaultName) {
setSourceBackupVaultName(sourceBackupVaultName);
return this;
}
/**
*
* An Amazon Resource Name (ARN) that uniquely identifies a destination backup vault to copy to; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*
*
* @param destinationBackupVaultArn
* An Amazon Resource Name (ARN) that uniquely identifies a destination backup vault to copy to; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*/
public void setDestinationBackupVaultArn(String destinationBackupVaultArn) {
this.destinationBackupVaultArn = destinationBackupVaultArn;
}
/**
*
* An Amazon Resource Name (ARN) that uniquely identifies a destination backup vault to copy to; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*
*
* @return An Amazon Resource Name (ARN) that uniquely identifies a destination backup vault to copy to; for
* example, arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*/
public String getDestinationBackupVaultArn() {
return this.destinationBackupVaultArn;
}
/**
*
* An Amazon Resource Name (ARN) that uniquely identifies a destination backup vault to copy to; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
*
*
* @param destinationBackupVaultArn
* An Amazon Resource Name (ARN) that uniquely identifies a destination backup vault to copy to; for example,
* arn:aws:backup:us-east-1:123456789012:vault:aBackupVault
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartCopyJobRequest withDestinationBackupVaultArn(String destinationBackupVaultArn) {
setDestinationBackupVaultArn(destinationBackupVaultArn);
return this;
}
/**
*
* Specifies the IAM role ARN used to copy the target recovery point; for example,
* arn:aws:iam::123456789012:role/S3Access
.
*
*
* @param iamRoleArn
* Specifies the IAM role ARN used to copy the target recovery point; for example,
* arn:aws:iam::123456789012:role/S3Access
.
*/
public void setIamRoleArn(String iamRoleArn) {
this.iamRoleArn = iamRoleArn;
}
/**
*
* Specifies the IAM role ARN used to copy the target recovery point; for example,
* arn:aws:iam::123456789012:role/S3Access
.
*
*
* @return Specifies the IAM role ARN used to copy the target recovery point; for example,
* arn:aws:iam::123456789012:role/S3Access
.
*/
public String getIamRoleArn() {
return this.iamRoleArn;
}
/**
*
* Specifies the IAM role ARN used to copy the target recovery point; for example,
* arn:aws:iam::123456789012:role/S3Access
.
*
*
* @param iamRoleArn
* Specifies the IAM role ARN used to copy the target recovery point; for example,
* arn:aws:iam::123456789012:role/S3Access
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartCopyJobRequest withIamRoleArn(String iamRoleArn) {
setIamRoleArn(iamRoleArn);
return this;
}
/**
*
* A customer-chosen string that you can use to distinguish between otherwise identical calls to
* StartCopyJob
. Retrying a successful request with the same idempotency token results in a success
* message with no action taken.
*
*
* @param idempotencyToken
* A customer-chosen string that you can use to distinguish between otherwise identical calls to
* StartCopyJob
. Retrying a successful request with the same idempotency token results in a
* success message with no action taken.
*/
public void setIdempotencyToken(String idempotencyToken) {
this.idempotencyToken = idempotencyToken;
}
/**
*
* A customer-chosen string that you can use to distinguish between otherwise identical calls to
* StartCopyJob
. Retrying a successful request with the same idempotency token results in a success
* message with no action taken.
*
*
* @return A customer-chosen string that you can use to distinguish between otherwise identical calls to
* StartCopyJob
. Retrying a successful request with the same idempotency token results in a
* success message with no action taken.
*/
public String getIdempotencyToken() {
return this.idempotencyToken;
}
/**
*
* A customer-chosen string that you can use to distinguish between otherwise identical calls to
* StartCopyJob
. Retrying a successful request with the same idempotency token results in a success
* message with no action taken.
*
*
* @param idempotencyToken
* A customer-chosen string that you can use to distinguish between otherwise identical calls to
* StartCopyJob
. Retrying a successful request with the same idempotency token results in a
* success message with no action taken.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartCopyJobRequest withIdempotencyToken(String idempotencyToken) {
setIdempotencyToken(idempotencyToken);
return this;
}
/**
* @param lifecycle
*/
public void setLifecycle(Lifecycle lifecycle) {
this.lifecycle = lifecycle;
}
/**
* @return
*/
public Lifecycle getLifecycle() {
return this.lifecycle;
}
/**
* @param lifecycle
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartCopyJobRequest withLifecycle(Lifecycle lifecycle) {
setLifecycle(lifecycle);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getRecoveryPointArn() != null)
sb.append("RecoveryPointArn: ").append(getRecoveryPointArn()).append(",");
if (getSourceBackupVaultName() != null)
sb.append("SourceBackupVaultName: ").append(getSourceBackupVaultName()).append(",");
if (getDestinationBackupVaultArn() != null)
sb.append("DestinationBackupVaultArn: ").append(getDestinationBackupVaultArn()).append(",");
if (getIamRoleArn() != null)
sb.append("IamRoleArn: ").append(getIamRoleArn()).append(",");
if (getIdempotencyToken() != null)
sb.append("IdempotencyToken: ").append(getIdempotencyToken()).append(",");
if (getLifecycle() != null)
sb.append("Lifecycle: ").append(getLifecycle());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StartCopyJobRequest == false)
return false;
StartCopyJobRequest other = (StartCopyJobRequest) obj;
if (other.getRecoveryPointArn() == null ^ this.getRecoveryPointArn() == null)
return false;
if (other.getRecoveryPointArn() != null && other.getRecoveryPointArn().equals(this.getRecoveryPointArn()) == false)
return false;
if (other.getSourceBackupVaultName() == null ^ this.getSourceBackupVaultName() == null)
return false;
if (other.getSourceBackupVaultName() != null && other.getSourceBackupVaultName().equals(this.getSourceBackupVaultName()) == false)
return false;
if (other.getDestinationBackupVaultArn() == null ^ this.getDestinationBackupVaultArn() == null)
return false;
if (other.getDestinationBackupVaultArn() != null && other.getDestinationBackupVaultArn().equals(this.getDestinationBackupVaultArn()) == false)
return false;
if (other.getIamRoleArn() == null ^ this.getIamRoleArn() == null)
return false;
if (other.getIamRoleArn() != null && other.getIamRoleArn().equals(this.getIamRoleArn()) == false)
return false;
if (other.getIdempotencyToken() == null ^ this.getIdempotencyToken() == null)
return false;
if (other.getIdempotencyToken() != null && other.getIdempotencyToken().equals(this.getIdempotencyToken()) == false)
return false;
if (other.getLifecycle() == null ^ this.getLifecycle() == null)
return false;
if (other.getLifecycle() != null && other.getLifecycle().equals(this.getLifecycle()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getRecoveryPointArn() == null) ? 0 : getRecoveryPointArn().hashCode());
hashCode = prime * hashCode + ((getSourceBackupVaultName() == null) ? 0 : getSourceBackupVaultName().hashCode());
hashCode = prime * hashCode + ((getDestinationBackupVaultArn() == null) ? 0 : getDestinationBackupVaultArn().hashCode());
hashCode = prime * hashCode + ((getIamRoleArn() == null) ? 0 : getIamRoleArn().hashCode());
hashCode = prime * hashCode + ((getIdempotencyToken() == null) ? 0 : getIdempotencyToken().hashCode());
hashCode = prime * hashCode + ((getLifecycle() == null) ? 0 : getLifecycle().hashCode());
return hashCode;
}
@Override
public StartCopyJobRequest clone() {
return (StartCopyJobRequest) super.clone();
}
}