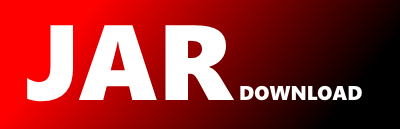
com.amazonaws.services.bcmdataexports.AWSBCMDataExportsAsync Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.bcmdataexports;
import javax.annotation.Generated;
import com.amazonaws.services.bcmdataexports.model.*;
/**
* Interface for accessing AWS Billing and Cost Management Data Exports asynchronously. Each asynchronous method will
* return a Java Future object representing the asynchronous operation; overloads which accept an {@code AsyncHandler}
* can be used to receive notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.bcmdataexports.AbstractAWSBCMDataExportsAsync} instead.
*
*
*
* You can use the Data Exports API to create customized exports from multiple Amazon Web Services cost management and
* billing datasets, such as cost and usage data and cost optimization recommendations.
*
*
* The Data Exports API provides the following endpoint:
*
*
* -
*
* https://bcm-data-exports.us-east-1.api.aws
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSBCMDataExportsAsync extends AWSBCMDataExports {
/**
*
* Creates a data export and specifies the data query, the delivery preference, and any optional resource tags.
*
*
* A DataQuery
consists of both a QueryStatement
and TableConfigurations
.
*
*
* The QueryStatement
is an SQL statement. Data Exports only supports a limited subset of the SQL
* syntax. For more information on the SQL syntax that is supported, see Data query. To view the available
* tables and columns, see the Data Exports table
* dictionary.
*
*
* The TableConfigurations
is a collection of specified TableProperties
for the table
* being queried in the QueryStatement
. TableProperties are additional configurations you can provide
* to change the data and schema of a table. Each table can have different TableProperties. However, tables are not
* required to have any TableProperties. Each table property has a default value that it assumes if not specified.
* For more information on table configurations, see Data query. To view the table
* properties available for each table, see the Data Exports table
* dictionary or use the ListTables
API to get a response of all tables and their available
* properties.
*
*
* @param createExportRequest
* @return A Java Future containing the result of the CreateExport operation returned by the service.
* @sample AWSBCMDataExportsAsync.CreateExport
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createExportAsync(CreateExportRequest createExportRequest);
/**
*
* Creates a data export and specifies the data query, the delivery preference, and any optional resource tags.
*
*
* A DataQuery
consists of both a QueryStatement
and TableConfigurations
.
*
*
* The QueryStatement
is an SQL statement. Data Exports only supports a limited subset of the SQL
* syntax. For more information on the SQL syntax that is supported, see Data query. To view the available
* tables and columns, see the Data Exports table
* dictionary.
*
*
* The TableConfigurations
is a collection of specified TableProperties
for the table
* being queried in the QueryStatement
. TableProperties are additional configurations you can provide
* to change the data and schema of a table. Each table can have different TableProperties. However, tables are not
* required to have any TableProperties. Each table property has a default value that it assumes if not specified.
* For more information on table configurations, see Data query. To view the table
* properties available for each table, see the Data Exports table
* dictionary or use the ListTables
API to get a response of all tables and their available
* properties.
*
*
* @param createExportRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateExport operation returned by the service.
* @sample AWSBCMDataExportsAsyncHandler.CreateExport
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createExportAsync(CreateExportRequest createExportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing data export.
*
*
* @param deleteExportRequest
* @return A Java Future containing the result of the DeleteExport operation returned by the service.
* @sample AWSBCMDataExportsAsync.DeleteExport
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteExportAsync(DeleteExportRequest deleteExportRequest);
/**
*
* Deletes an existing data export.
*
*
* @param deleteExportRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteExport operation returned by the service.
* @sample AWSBCMDataExportsAsyncHandler.DeleteExport
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteExportAsync(DeleteExportRequest deleteExportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Exports data based on the source data update.
*
*
* @param getExecutionRequest
* @return A Java Future containing the result of the GetExecution operation returned by the service.
* @sample AWSBCMDataExportsAsync.GetExecution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getExecutionAsync(GetExecutionRequest getExecutionRequest);
/**
*
* Exports data based on the source data update.
*
*
* @param getExecutionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetExecution operation returned by the service.
* @sample AWSBCMDataExportsAsyncHandler.GetExecution
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getExecutionAsync(GetExecutionRequest getExecutionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Views the definition of an existing data export.
*
*
* @param getExportRequest
* @return A Java Future containing the result of the GetExport operation returned by the service.
* @sample AWSBCMDataExportsAsync.GetExport
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getExportAsync(GetExportRequest getExportRequest);
/**
*
* Views the definition of an existing data export.
*
*
* @param getExportRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetExport operation returned by the service.
* @sample AWSBCMDataExportsAsyncHandler.GetExport
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getExportAsync(GetExportRequest getExportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the metadata for the specified table and table properties. This includes the list of columns in the table
* schema, their data types, and column descriptions.
*
*
* @param getTableRequest
* @return A Java Future containing the result of the GetTable operation returned by the service.
* @sample AWSBCMDataExportsAsync.GetTable
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTableAsync(GetTableRequest getTableRequest);
/**
*
* Returns the metadata for the specified table and table properties. This includes the list of columns in the table
* schema, their data types, and column descriptions.
*
*
* @param getTableRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTable operation returned by the service.
* @sample AWSBCMDataExportsAsyncHandler.GetTable
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTableAsync(GetTableRequest getTableRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the historical executions for the export.
*
*
* @param listExecutionsRequest
* @return A Java Future containing the result of the ListExecutions operation returned by the service.
* @sample AWSBCMDataExportsAsync.ListExecutions
* @see AWS API Documentation
*/
java.util.concurrent.Future listExecutionsAsync(ListExecutionsRequest listExecutionsRequest);
/**
*
* Lists the historical executions for the export.
*
*
* @param listExecutionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListExecutions operation returned by the service.
* @sample AWSBCMDataExportsAsyncHandler.ListExecutions
* @see AWS API Documentation
*/
java.util.concurrent.Future listExecutionsAsync(ListExecutionsRequest listExecutionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all data export definitions.
*
*
* @param listExportsRequest
* @return A Java Future containing the result of the ListExports operation returned by the service.
* @sample AWSBCMDataExportsAsync.ListExports
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listExportsAsync(ListExportsRequest listExportsRequest);
/**
*
* Lists all data export definitions.
*
*
* @param listExportsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListExports operation returned by the service.
* @sample AWSBCMDataExportsAsyncHandler.ListExports
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listExportsAsync(ListExportsRequest listExportsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all available tables in data exports.
*
*
* @param listTablesRequest
* @return A Java Future containing the result of the ListTables operation returned by the service.
* @sample AWSBCMDataExportsAsync.ListTables
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTablesAsync(ListTablesRequest listTablesRequest);
/**
*
* Lists all available tables in data exports.
*
*
* @param listTablesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTables operation returned by the service.
* @sample AWSBCMDataExportsAsyncHandler.ListTables
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTablesAsync(ListTablesRequest listTablesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List tags associated with an existing data export.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSBCMDataExportsAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* List tags associated with an existing data export.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSBCMDataExportsAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds tags for an existing data export definition.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSBCMDataExportsAsync.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds tags for an existing data export definition.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSBCMDataExportsAsyncHandler.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes tags associated with an existing data export definition.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSBCMDataExportsAsync.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Deletes tags associated with an existing data export definition.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSBCMDataExportsAsyncHandler.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing data export by overwriting all export parameters. All export parameters must be provided in
* the UpdateExport request.
*
*
* @param updateExportRequest
* @return A Java Future containing the result of the UpdateExport operation returned by the service.
* @sample AWSBCMDataExportsAsync.UpdateExport
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateExportAsync(UpdateExportRequest updateExportRequest);
/**
*
* Updates an existing data export by overwriting all export parameters. All export parameters must be provided in
* the UpdateExport request.
*
*
* @param updateExportRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateExport operation returned by the service.
* @sample AWSBCMDataExportsAsyncHandler.UpdateExport
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateExportAsync(UpdateExportRequest updateExportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}