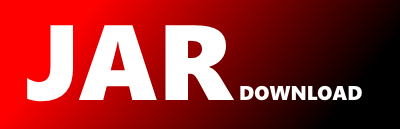
com.amazonaws.services.bedrock.AmazonBedrock Maven / Gradle / Ivy
Show all versions of aws-java-sdk-bedrock Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.bedrock;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.bedrock.model.*;
/**
* Interface for accessing Amazon Bedrock.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.bedrock.AbstractAmazonBedrock} instead.
*
*
*
* Describes the API operations for creating, managing, fine-turning, and evaluating Amazon Bedrock models.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonBedrock {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "bedrock";
/**
*
* API operation for creating and managing Amazon Bedrock automatic model evaluation jobs and model evaluation jobs
* that use human workers. To learn more about the requirements for creating a model evaluation job see, Model evaluations.
*
*
* @param createEvaluationJobRequest
* @return Result of the CreateEvaluationJob operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws ConflictException
* Error occurred because of a conflict while performing an operation.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ServiceQuotaExceededException
* The number of requests exceeds the service quota. Resubmit your request later.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.CreateEvaluationJob
* @see AWS
* API Documentation
*/
CreateEvaluationJobResult createEvaluationJob(CreateEvaluationJobRequest createEvaluationJobRequest);
/**
*
* Creates a guardrail to block topics and to implement safeguards for your generative AI applications.
*
*
* You can configure the following policies in a guardrail to avoid undesirable and harmful content, filter out
* denied topics and words, and remove sensitive information for privacy protection.
*
*
* -
*
* Content filters - Adjust filter strengths to block input prompts or model responses containing harmful
* content.
*
*
* -
*
* Denied topics - Define a set of topics that are undesirable in the context of your application. These
* topics will be blocked if detected in user queries or model responses.
*
*
* -
*
* Word filters - Configure filters to block undesirable words, phrases, and profanity. Such words can
* include offensive terms, competitor names etc.
*
*
* -
*
* Sensitive information filters - Block or mask sensitive information such as personally identifiable
* information (PII) or custom regex in user inputs and model responses.
*
*
*
*
* In addition to the above policies, you can also configure the messages to be returned to the user if a user input
* or model response is in violation of the policies defined in the guardrail.
*
*
* For more information, see Guardrails for Amazon Bedrock in
* the Amazon Bedrock User Guide.
*
*
* @param createGuardrailRequest
* @return Result of the CreateGuardrail operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws ConflictException
* Error occurred because of a conflict while performing an operation.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws TooManyTagsException
* The request contains more tags than can be associated with a resource (50 tags per resource). The maximum
* number of tags includes both existing tags and those included in your current request.
* @throws ServiceQuotaExceededException
* The number of requests exceeds the service quota. Resubmit your request later.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.CreateGuardrail
* @see AWS API
* Documentation
*/
CreateGuardrailResult createGuardrail(CreateGuardrailRequest createGuardrailRequest);
/**
*
* Creates a version of the guardrail. Use this API to create a snapshot of the guardrail when you are satisfied
* with a configuration, or to compare the configuration with another version.
*
*
* @param createGuardrailVersionRequest
* @return Result of the CreateGuardrailVersion operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws ConflictException
* Error occurred because of a conflict while performing an operation.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ServiceQuotaExceededException
* The number of requests exceeds the service quota. Resubmit your request later.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.CreateGuardrailVersion
* @see AWS
* API Documentation
*/
CreateGuardrailVersionResult createGuardrailVersion(CreateGuardrailVersionRequest createGuardrailVersionRequest);
/**
*
* Creates a fine-tuning job to customize a base model.
*
*
* You specify the base foundation model and the location of the training data. After the model-customization job
* completes successfully, your custom model resource will be ready to use. Amazon Bedrock returns validation loss
* metrics and output generations after the job completes.
*
*
* For information on the format of training and validation data, see Prepare the
* datasets.
*
*
* Model-customization jobs are asynchronous and the completion time depends on the base model and the
* training/validation data size. To monitor a job, use the GetModelCustomizationJob
operation to
* retrieve the job status.
*
*
* For more information, see Custom models in the Amazon
* Bedrock User Guide.
*
*
* @param createModelCustomizationJobRequest
* @return Result of the CreateModelCustomizationJob operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws ConflictException
* Error occurred because of a conflict while performing an operation.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws TooManyTagsException
* The request contains more tags than can be associated with a resource (50 tags per resource). The maximum
* number of tags includes both existing tags and those included in your current request.
* @throws ServiceQuotaExceededException
* The number of requests exceeds the service quota. Resubmit your request later.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.CreateModelCustomizationJob
* @see AWS API Documentation
*/
CreateModelCustomizationJobResult createModelCustomizationJob(CreateModelCustomizationJobRequest createModelCustomizationJobRequest);
/**
*
* Creates dedicated throughput for a base or custom model with the model units and for the duration that you
* specify. For pricing details, see Amazon Bedrock Pricing.
* For more information, see Provisioned Throughput in
* the Amazon Bedrock User Guide.
*
*
* @param createProvisionedModelThroughputRequest
* @return Result of the CreateProvisionedModelThroughput operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws TooManyTagsException
* The request contains more tags than can be associated with a resource (50 tags per resource). The maximum
* number of tags includes both existing tags and those included in your current request.
* @throws ServiceQuotaExceededException
* The number of requests exceeds the service quota. Resubmit your request later.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.CreateProvisionedModelThroughput
* @see AWS API Documentation
*/
CreateProvisionedModelThroughputResult createProvisionedModelThroughput(CreateProvisionedModelThroughputRequest createProvisionedModelThroughputRequest);
/**
*
* Deletes a custom model that you created earlier. For more information, see Custom models in the Amazon
* Bedrock User Guide.
*
*
* @param deleteCustomModelRequest
* @return Result of the DeleteCustomModel operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws ConflictException
* Error occurred because of a conflict while performing an operation.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.DeleteCustomModel
* @see AWS API
* Documentation
*/
DeleteCustomModelResult deleteCustomModel(DeleteCustomModelRequest deleteCustomModelRequest);
/**
*
* Deletes a guardrail.
*
*
* -
*
* To delete a guardrail, only specify the ARN of the guardrail in the guardrailIdentifier
field. If
* you delete a guardrail, all of its versions will be deleted.
*
*
* -
*
* To delete a version of a guardrail, specify the ARN of the guardrail in the guardrailIdentifier
* field and the version in the guardrailVersion
field.
*
*
*
*
* @param deleteGuardrailRequest
* @return Result of the DeleteGuardrail operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws ConflictException
* Error occurred because of a conflict while performing an operation.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.DeleteGuardrail
* @see AWS API
* Documentation
*/
DeleteGuardrailResult deleteGuardrail(DeleteGuardrailRequest deleteGuardrailRequest);
/**
*
* Delete the invocation logging.
*
*
* @param deleteModelInvocationLoggingConfigurationRequest
* @return Result of the DeleteModelInvocationLoggingConfiguration operation returned by the service.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.DeleteModelInvocationLoggingConfiguration
* @see AWS API Documentation
*/
DeleteModelInvocationLoggingConfigurationResult deleteModelInvocationLoggingConfiguration(
DeleteModelInvocationLoggingConfigurationRequest deleteModelInvocationLoggingConfigurationRequest);
/**
*
* Deletes a Provisioned Throughput. You can't delete a Provisioned Throughput before the commitment term is over.
* For more information, see Provisioned Throughput in
* the Amazon Bedrock User Guide.
*
*
* @param deleteProvisionedModelThroughputRequest
* @return Result of the DeleteProvisionedModelThroughput operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws ConflictException
* Error occurred because of a conflict while performing an operation.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.DeleteProvisionedModelThroughput
* @see AWS API Documentation
*/
DeleteProvisionedModelThroughputResult deleteProvisionedModelThroughput(DeleteProvisionedModelThroughputRequest deleteProvisionedModelThroughputRequest);
/**
*
* Get the properties associated with a Amazon Bedrock custom model that you have created.For more information, see
* Custom models in the Amazon
* Bedrock User Guide.
*
*
* @param getCustomModelRequest
* @return Result of the GetCustomModel operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.GetCustomModel
* @see AWS API
* Documentation
*/
GetCustomModelResult getCustomModel(GetCustomModelRequest getCustomModelRequest);
/**
*
* Retrieves the properties associated with a model evaluation job, including the status of the job. For more
* information, see Model
* evaluations.
*
*
* @param getEvaluationJobRequest
* @return Result of the GetEvaluationJob operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.GetEvaluationJob
* @see AWS API
* Documentation
*/
GetEvaluationJobResult getEvaluationJob(GetEvaluationJobRequest getEvaluationJobRequest);
/**
*
* Get details about a Amazon Bedrock foundation model.
*
*
* @param getFoundationModelRequest
* @return Result of the GetFoundationModel operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.GetFoundationModel
* @see AWS API
* Documentation
*/
GetFoundationModelResult getFoundationModel(GetFoundationModelRequest getFoundationModelRequest);
/**
*
* Gets details about a guardrail. If you don't specify a version, the response returns details for the
* DRAFT
version.
*
*
* @param getGuardrailRequest
* @return Result of the GetGuardrail operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.GetGuardrail
* @see AWS API
* Documentation
*/
GetGuardrailResult getGuardrail(GetGuardrailRequest getGuardrailRequest);
/**
*
* Retrieves the properties associated with a model-customization job, including the status of the job. For more
* information, see Custom
* models in the Amazon Bedrock User Guide.
*
*
* @param getModelCustomizationJobRequest
* @return Result of the GetModelCustomizationJob operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.GetModelCustomizationJob
* @see AWS API Documentation
*/
GetModelCustomizationJobResult getModelCustomizationJob(GetModelCustomizationJobRequest getModelCustomizationJobRequest);
/**
*
* Get the current configuration values for model invocation logging.
*
*
* @param getModelInvocationLoggingConfigurationRequest
* @return Result of the GetModelInvocationLoggingConfiguration operation returned by the service.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.GetModelInvocationLoggingConfiguration
* @see AWS API Documentation
*/
GetModelInvocationLoggingConfigurationResult getModelInvocationLoggingConfiguration(
GetModelInvocationLoggingConfigurationRequest getModelInvocationLoggingConfigurationRequest);
/**
*
* Returns details for a Provisioned Throughput. For more information, see Provisioned Throughput in
* the Amazon Bedrock User Guide.
*
*
* @param getProvisionedModelThroughputRequest
* @return Result of the GetProvisionedModelThroughput operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.GetProvisionedModelThroughput
* @see AWS API Documentation
*/
GetProvisionedModelThroughputResult getProvisionedModelThroughput(GetProvisionedModelThroughputRequest getProvisionedModelThroughputRequest);
/**
*
* Returns a list of the custom models that you have created with the CreateModelCustomizationJob
* operation.
*
*
* For more information, see Custom models in the Amazon
* Bedrock User Guide.
*
*
* @param listCustomModelsRequest
* @return Result of the ListCustomModels operation returned by the service.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.ListCustomModels
* @see AWS API
* Documentation
*/
ListCustomModelsResult listCustomModels(ListCustomModelsRequest listCustomModelsRequest);
/**
*
* Lists model evaluation jobs.
*
*
* @param listEvaluationJobsRequest
* @return Result of the ListEvaluationJobs operation returned by the service.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.ListEvaluationJobs
* @see AWS API
* Documentation
*/
ListEvaluationJobsResult listEvaluationJobs(ListEvaluationJobsRequest listEvaluationJobsRequest);
/**
*
* Lists Amazon Bedrock foundation models that you can use. You can filter the results with the request parameters.
* For more information, see Foundation models in the
* Amazon Bedrock User Guide.
*
*
* @param listFoundationModelsRequest
* @return Result of the ListFoundationModels operation returned by the service.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.ListFoundationModels
* @see AWS
* API Documentation
*/
ListFoundationModelsResult listFoundationModels(ListFoundationModelsRequest listFoundationModelsRequest);
/**
*
* Lists details about all the guardrails in an account. To list the DRAFT
version of all your
* guardrails, don't specify the guardrailIdentifier
field. To list all versions of a guardrail,
* specify the ARN of the guardrail in the guardrailIdentifier
field.
*
*
* You can set the maximum number of results to return in a response in the maxResults
field. If there
* are more results than the number you set, the response returns a nextToken
that you can send in
* another ListGuardrails
request to see the next batch of results.
*
*
* @param listGuardrailsRequest
* @return Result of the ListGuardrails operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.ListGuardrails
* @see AWS API
* Documentation
*/
ListGuardrailsResult listGuardrails(ListGuardrailsRequest listGuardrailsRequest);
/**
*
* Returns a list of model customization jobs that you have submitted. You can filter the jobs to return based on
* one or more criteria.
*
*
* For more information, see Custom models in the Amazon
* Bedrock User Guide.
*
*
* @param listModelCustomizationJobsRequest
* @return Result of the ListModelCustomizationJobs operation returned by the service.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.ListModelCustomizationJobs
* @see AWS API Documentation
*/
ListModelCustomizationJobsResult listModelCustomizationJobs(ListModelCustomizationJobsRequest listModelCustomizationJobsRequest);
/**
*
* Lists the Provisioned Throughputs in the account. For more information, see Provisioned Throughput in
* the Amazon Bedrock User Guide.
*
*
* @param listProvisionedModelThroughputsRequest
* @return Result of the ListProvisionedModelThroughputs operation returned by the service.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.ListProvisionedModelThroughputs
* @see AWS API Documentation
*/
ListProvisionedModelThroughputsResult listProvisionedModelThroughputs(ListProvisionedModelThroughputsRequest listProvisionedModelThroughputsRequest);
/**
*
* List the tags associated with the specified resource.
*
*
* For more information, see Tagging
* resources in the Amazon Bedrock User Guide.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.ListTagsForResource
* @see AWS
* API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Set the configuration values for model invocation logging.
*
*
* @param putModelInvocationLoggingConfigurationRequest
* @return Result of the PutModelInvocationLoggingConfiguration operation returned by the service.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.PutModelInvocationLoggingConfiguration
* @see AWS API Documentation
*/
PutModelInvocationLoggingConfigurationResult putModelInvocationLoggingConfiguration(
PutModelInvocationLoggingConfigurationRequest putModelInvocationLoggingConfigurationRequest);
/**
*
* Stops an in progress model evaluation job.
*
*
* @param stopEvaluationJobRequest
* @return Result of the StopEvaluationJob operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws ConflictException
* Error occurred because of a conflict while performing an operation.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.StopEvaluationJob
* @see AWS API
* Documentation
*/
StopEvaluationJobResult stopEvaluationJob(StopEvaluationJobRequest stopEvaluationJobRequest);
/**
*
* Stops an active model customization job. For more information, see Custom models in the Amazon
* Bedrock User Guide.
*
*
* @param stopModelCustomizationJobRequest
* @return Result of the StopModelCustomizationJob operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws ConflictException
* Error occurred because of a conflict while performing an operation.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.StopModelCustomizationJob
* @see AWS API Documentation
*/
StopModelCustomizationJobResult stopModelCustomizationJob(StopModelCustomizationJobRequest stopModelCustomizationJobRequest);
/**
*
* Associate tags with a resource. For more information, see Tagging resources in the Amazon
* Bedrock User Guide.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws TooManyTagsException
* The request contains more tags than can be associated with a resource (50 tags per resource). The maximum
* number of tags includes both existing tags and those included in your current request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Remove one or more tags from a resource. For more information, see Tagging resources in the Amazon
* Bedrock User Guide.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates a guardrail with the values you specify.
*
*
* -
*
* Specify a name
and optional description
.
*
*
* -
*
* Specify messages for when the guardrail successfully blocks a prompt or a model response in the
* blockedInputMessaging
and blockedOutputsMessaging
fields.
*
*
* -
*
* Specify topics for the guardrail to deny in the topicPolicyConfig
object. Each GuardrailTopicConfig object in the topicsConfig
list pertains to one topic.
*
*
* -
*
* Give a name
and description
so that the guardrail can properly identify the topic.
*
*
* -
*
* Specify DENY
in the type
field.
*
*
* -
*
* (Optional) Provide up to five prompts that you would categorize as belonging to the topic in the
* examples
list.
*
*
*
*
* -
*
* Specify filter strengths for the harmful categories defined in Amazon Bedrock in the
* contentPolicyConfig
object. Each GuardrailContentFilterConfig object in the filtersConfig
list pertains to a harmful category.
* For more information, see Content filters. For
* more information about the fields in a content filter, see GuardrailContentFilterConfig.
*
*
* -
*
* Specify the category in the type
field.
*
*
* -
*
* Specify the strength of the filter for prompts in the inputStrength
field and for model responses in
* the strength
field of the GuardrailContentFilterConfig.
*
*
*
*
* -
*
* (Optional) For security, include the ARN of a KMS key in the kmsKeyId
field.
*
*
*
*
* @param updateGuardrailRequest
* @return Result of the UpdateGuardrail operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws ConflictException
* Error occurred because of a conflict while performing an operation.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ServiceQuotaExceededException
* The number of requests exceeds the service quota. Resubmit your request later.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.UpdateGuardrail
* @see AWS API
* Documentation
*/
UpdateGuardrailResult updateGuardrail(UpdateGuardrailRequest updateGuardrailRequest);
/**
*
* Updates the name or associated model for a Provisioned Throughput. For more information, see Provisioned Throughput in
* the Amazon Bedrock User Guide.
*
*
* @param updateProvisionedModelThroughputRequest
* @return Result of the UpdateProvisionedModelThroughput operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource Amazon Resource Name (ARN) was not found. Check the Amazon Resource Name (ARN) and
* try your request again.
* @throws AccessDeniedException
* The request is denied because of missing access permissions.
* @throws ValidationException
* Input validation failed. Check your request parameters and retry the request.
* @throws InternalServerException
* An internal server error occurred. Retry your request.
* @throws ThrottlingException
* The number of requests exceeds the limit. Resubmit your request later.
* @sample AmazonBedrock.UpdateProvisionedModelThroughput
* @see AWS API Documentation
*/
UpdateProvisionedModelThroughputResult updateProvisionedModelThroughput(UpdateProvisionedModelThroughputRequest updateProvisionedModelThroughputRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}