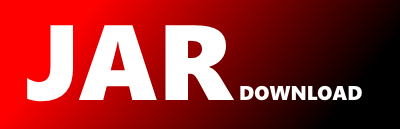
com.amazonaws.services.bedrock.AmazonBedrockAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-bedrock Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.bedrock;
import javax.annotation.Generated;
import com.amazonaws.services.bedrock.model.*;
/**
* Interface for accessing Amazon Bedrock asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.bedrock.AbstractAmazonBedrockAsync} instead.
*
*
*
* Describes the API operations for creating, managing, fine-turning, and evaluating Amazon Bedrock models.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonBedrockAsync extends AmazonBedrock {
/**
*
* API operation for creating and managing Amazon Bedrock automatic model evaluation jobs and model evaluation jobs
* that use human workers. To learn more about the requirements for creating a model evaluation job see, Model evaluations.
*
*
* @param createEvaluationJobRequest
* @return A Java Future containing the result of the CreateEvaluationJob operation returned by the service.
* @sample AmazonBedrockAsync.CreateEvaluationJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createEvaluationJobAsync(CreateEvaluationJobRequest createEvaluationJobRequest);
/**
*
* API operation for creating and managing Amazon Bedrock automatic model evaluation jobs and model evaluation jobs
* that use human workers. To learn more about the requirements for creating a model evaluation job see, Model evaluations.
*
*
* @param createEvaluationJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEvaluationJob operation returned by the service.
* @sample AmazonBedrockAsyncHandler.CreateEvaluationJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createEvaluationJobAsync(CreateEvaluationJobRequest createEvaluationJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a guardrail to block topics and to implement safeguards for your generative AI applications.
*
*
* You can configure the following policies in a guardrail to avoid undesirable and harmful content, filter out
* denied topics and words, and remove sensitive information for privacy protection.
*
*
* -
*
* Content filters - Adjust filter strengths to block input prompts or model responses containing harmful
* content.
*
*
* -
*
* Denied topics - Define a set of topics that are undesirable in the context of your application. These
* topics will be blocked if detected in user queries or model responses.
*
*
* -
*
* Word filters - Configure filters to block undesirable words, phrases, and profanity. Such words can
* include offensive terms, competitor names etc.
*
*
* -
*
* Sensitive information filters - Block or mask sensitive information such as personally identifiable
* information (PII) or custom regex in user inputs and model responses.
*
*
*
*
* In addition to the above policies, you can also configure the messages to be returned to the user if a user input
* or model response is in violation of the policies defined in the guardrail.
*
*
* For more information, see Guardrails for Amazon Bedrock in
* the Amazon Bedrock User Guide.
*
*
* @param createGuardrailRequest
* @return A Java Future containing the result of the CreateGuardrail operation returned by the service.
* @sample AmazonBedrockAsync.CreateGuardrail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createGuardrailAsync(CreateGuardrailRequest createGuardrailRequest);
/**
*
* Creates a guardrail to block topics and to implement safeguards for your generative AI applications.
*
*
* You can configure the following policies in a guardrail to avoid undesirable and harmful content, filter out
* denied topics and words, and remove sensitive information for privacy protection.
*
*
* -
*
* Content filters - Adjust filter strengths to block input prompts or model responses containing harmful
* content.
*
*
* -
*
* Denied topics - Define a set of topics that are undesirable in the context of your application. These
* topics will be blocked if detected in user queries or model responses.
*
*
* -
*
* Word filters - Configure filters to block undesirable words, phrases, and profanity. Such words can
* include offensive terms, competitor names etc.
*
*
* -
*
* Sensitive information filters - Block or mask sensitive information such as personally identifiable
* information (PII) or custom regex in user inputs and model responses.
*
*
*
*
* In addition to the above policies, you can also configure the messages to be returned to the user if a user input
* or model response is in violation of the policies defined in the guardrail.
*
*
* For more information, see Guardrails for Amazon Bedrock in
* the Amazon Bedrock User Guide.
*
*
* @param createGuardrailRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateGuardrail operation returned by the service.
* @sample AmazonBedrockAsyncHandler.CreateGuardrail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createGuardrailAsync(CreateGuardrailRequest createGuardrailRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a version of the guardrail. Use this API to create a snapshot of the guardrail when you are satisfied
* with a configuration, or to compare the configuration with another version.
*
*
* @param createGuardrailVersionRequest
* @return A Java Future containing the result of the CreateGuardrailVersion operation returned by the service.
* @sample AmazonBedrockAsync.CreateGuardrailVersion
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createGuardrailVersionAsync(CreateGuardrailVersionRequest createGuardrailVersionRequest);
/**
*
* Creates a version of the guardrail. Use this API to create a snapshot of the guardrail when you are satisfied
* with a configuration, or to compare the configuration with another version.
*
*
* @param createGuardrailVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateGuardrailVersion operation returned by the service.
* @sample AmazonBedrockAsyncHandler.CreateGuardrailVersion
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createGuardrailVersionAsync(CreateGuardrailVersionRequest createGuardrailVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a fine-tuning job to customize a base model.
*
*
* You specify the base foundation model and the location of the training data. After the model-customization job
* completes successfully, your custom model resource will be ready to use. Amazon Bedrock returns validation loss
* metrics and output generations after the job completes.
*
*
* For information on the format of training and validation data, see Prepare the
* datasets.
*
*
* Model-customization jobs are asynchronous and the completion time depends on the base model and the
* training/validation data size. To monitor a job, use the GetModelCustomizationJob
operation to
* retrieve the job status.
*
*
* For more information, see Custom models in the Amazon
* Bedrock User Guide.
*
*
* @param createModelCustomizationJobRequest
* @return A Java Future containing the result of the CreateModelCustomizationJob operation returned by the service.
* @sample AmazonBedrockAsync.CreateModelCustomizationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future createModelCustomizationJobAsync(
CreateModelCustomizationJobRequest createModelCustomizationJobRequest);
/**
*
* Creates a fine-tuning job to customize a base model.
*
*
* You specify the base foundation model and the location of the training data. After the model-customization job
* completes successfully, your custom model resource will be ready to use. Amazon Bedrock returns validation loss
* metrics and output generations after the job completes.
*
*
* For information on the format of training and validation data, see Prepare the
* datasets.
*
*
* Model-customization jobs are asynchronous and the completion time depends on the base model and the
* training/validation data size. To monitor a job, use the GetModelCustomizationJob
operation to
* retrieve the job status.
*
*
* For more information, see Custom models in the Amazon
* Bedrock User Guide.
*
*
* @param createModelCustomizationJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateModelCustomizationJob operation returned by the service.
* @sample AmazonBedrockAsyncHandler.CreateModelCustomizationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future createModelCustomizationJobAsync(
CreateModelCustomizationJobRequest createModelCustomizationJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates dedicated throughput for a base or custom model with the model units and for the duration that you
* specify. For pricing details, see Amazon Bedrock Pricing.
* For more information, see Provisioned Throughput in
* the Amazon Bedrock User Guide.
*
*
* @param createProvisionedModelThroughputRequest
* @return A Java Future containing the result of the CreateProvisionedModelThroughput operation returned by the
* service.
* @sample AmazonBedrockAsync.CreateProvisionedModelThroughput
* @see AWS API Documentation
*/
java.util.concurrent.Future createProvisionedModelThroughputAsync(
CreateProvisionedModelThroughputRequest createProvisionedModelThroughputRequest);
/**
*
* Creates dedicated throughput for a base or custom model with the model units and for the duration that you
* specify. For pricing details, see Amazon Bedrock Pricing.
* For more information, see Provisioned Throughput in
* the Amazon Bedrock User Guide.
*
*
* @param createProvisionedModelThroughputRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateProvisionedModelThroughput operation returned by the
* service.
* @sample AmazonBedrockAsyncHandler.CreateProvisionedModelThroughput
* @see AWS API Documentation
*/
java.util.concurrent.Future createProvisionedModelThroughputAsync(
CreateProvisionedModelThroughputRequest createProvisionedModelThroughputRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a custom model that you created earlier. For more information, see Custom models in the Amazon
* Bedrock User Guide.
*
*
* @param deleteCustomModelRequest
* @return A Java Future containing the result of the DeleteCustomModel operation returned by the service.
* @sample AmazonBedrockAsync.DeleteCustomModel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteCustomModelAsync(DeleteCustomModelRequest deleteCustomModelRequest);
/**
*
* Deletes a custom model that you created earlier. For more information, see Custom models in the Amazon
* Bedrock User Guide.
*
*
* @param deleteCustomModelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCustomModel operation returned by the service.
* @sample AmazonBedrockAsyncHandler.DeleteCustomModel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteCustomModelAsync(DeleteCustomModelRequest deleteCustomModelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a guardrail.
*
*
* -
*
* To delete a guardrail, only specify the ARN of the guardrail in the guardrailIdentifier
field. If
* you delete a guardrail, all of its versions will be deleted.
*
*
* -
*
* To delete a version of a guardrail, specify the ARN of the guardrail in the guardrailIdentifier
* field and the version in the guardrailVersion
field.
*
*
*
*
* @param deleteGuardrailRequest
* @return A Java Future containing the result of the DeleteGuardrail operation returned by the service.
* @sample AmazonBedrockAsync.DeleteGuardrail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteGuardrailAsync(DeleteGuardrailRequest deleteGuardrailRequest);
/**
*
* Deletes a guardrail.
*
*
* -
*
* To delete a guardrail, only specify the ARN of the guardrail in the guardrailIdentifier
field. If
* you delete a guardrail, all of its versions will be deleted.
*
*
* -
*
* To delete a version of a guardrail, specify the ARN of the guardrail in the guardrailIdentifier
* field and the version in the guardrailVersion
field.
*
*
*
*
* @param deleteGuardrailRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteGuardrail operation returned by the service.
* @sample AmazonBedrockAsyncHandler.DeleteGuardrail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteGuardrailAsync(DeleteGuardrailRequest deleteGuardrailRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete the invocation logging.
*
*
* @param deleteModelInvocationLoggingConfigurationRequest
* @return A Java Future containing the result of the DeleteModelInvocationLoggingConfiguration operation returned
* by the service.
* @sample AmazonBedrockAsync.DeleteModelInvocationLoggingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteModelInvocationLoggingConfigurationAsync(
DeleteModelInvocationLoggingConfigurationRequest deleteModelInvocationLoggingConfigurationRequest);
/**
*
* Delete the invocation logging.
*
*
* @param deleteModelInvocationLoggingConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteModelInvocationLoggingConfiguration operation returned
* by the service.
* @sample AmazonBedrockAsyncHandler.DeleteModelInvocationLoggingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteModelInvocationLoggingConfigurationAsync(
DeleteModelInvocationLoggingConfigurationRequest deleteModelInvocationLoggingConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a Provisioned Throughput. You can't delete a Provisioned Throughput before the commitment term is over.
* For more information, see Provisioned Throughput in
* the Amazon Bedrock User Guide.
*
*
* @param deleteProvisionedModelThroughputRequest
* @return A Java Future containing the result of the DeleteProvisionedModelThroughput operation returned by the
* service.
* @sample AmazonBedrockAsync.DeleteProvisionedModelThroughput
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteProvisionedModelThroughputAsync(
DeleteProvisionedModelThroughputRequest deleteProvisionedModelThroughputRequest);
/**
*
* Deletes a Provisioned Throughput. You can't delete a Provisioned Throughput before the commitment term is over.
* For more information, see Provisioned Throughput in
* the Amazon Bedrock User Guide.
*
*
* @param deleteProvisionedModelThroughputRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteProvisionedModelThroughput operation returned by the
* service.
* @sample AmazonBedrockAsyncHandler.DeleteProvisionedModelThroughput
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteProvisionedModelThroughputAsync(
DeleteProvisionedModelThroughputRequest deleteProvisionedModelThroughputRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the properties associated with a Amazon Bedrock custom model that you have created.For more information, see
* Custom models in the Amazon
* Bedrock User Guide.
*
*
* @param getCustomModelRequest
* @return A Java Future containing the result of the GetCustomModel operation returned by the service.
* @sample AmazonBedrockAsync.GetCustomModel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getCustomModelAsync(GetCustomModelRequest getCustomModelRequest);
/**
*
* Get the properties associated with a Amazon Bedrock custom model that you have created.For more information, see
* Custom models in the Amazon
* Bedrock User Guide.
*
*
* @param getCustomModelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetCustomModel operation returned by the service.
* @sample AmazonBedrockAsyncHandler.GetCustomModel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getCustomModelAsync(GetCustomModelRequest getCustomModelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the properties associated with a model evaluation job, including the status of the job. For more
* information, see Model
* evaluations.
*
*
* @param getEvaluationJobRequest
* @return A Java Future containing the result of the GetEvaluationJob operation returned by the service.
* @sample AmazonBedrockAsync.GetEvaluationJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getEvaluationJobAsync(GetEvaluationJobRequest getEvaluationJobRequest);
/**
*
* Retrieves the properties associated with a model evaluation job, including the status of the job. For more
* information, see Model
* evaluations.
*
*
* @param getEvaluationJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEvaluationJob operation returned by the service.
* @sample AmazonBedrockAsyncHandler.GetEvaluationJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getEvaluationJobAsync(GetEvaluationJobRequest getEvaluationJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get details about a Amazon Bedrock foundation model.
*
*
* @param getFoundationModelRequest
* @return A Java Future containing the result of the GetFoundationModel operation returned by the service.
* @sample AmazonBedrockAsync.GetFoundationModel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFoundationModelAsync(GetFoundationModelRequest getFoundationModelRequest);
/**
*
* Get details about a Amazon Bedrock foundation model.
*
*
* @param getFoundationModelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetFoundationModel operation returned by the service.
* @sample AmazonBedrockAsyncHandler.GetFoundationModel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFoundationModelAsync(GetFoundationModelRequest getFoundationModelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets details about a guardrail. If you don't specify a version, the response returns details for the
* DRAFT
version.
*
*
* @param getGuardrailRequest
* @return A Java Future containing the result of the GetGuardrail operation returned by the service.
* @sample AmazonBedrockAsync.GetGuardrail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getGuardrailAsync(GetGuardrailRequest getGuardrailRequest);
/**
*
* Gets details about a guardrail. If you don't specify a version, the response returns details for the
* DRAFT
version.
*
*
* @param getGuardrailRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetGuardrail operation returned by the service.
* @sample AmazonBedrockAsyncHandler.GetGuardrail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getGuardrailAsync(GetGuardrailRequest getGuardrailRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the properties associated with a model-customization job, including the status of the job. For more
* information, see Custom
* models in the Amazon Bedrock User Guide.
*
*
* @param getModelCustomizationJobRequest
* @return A Java Future containing the result of the GetModelCustomizationJob operation returned by the service.
* @sample AmazonBedrockAsync.GetModelCustomizationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future getModelCustomizationJobAsync(GetModelCustomizationJobRequest getModelCustomizationJobRequest);
/**
*
* Retrieves the properties associated with a model-customization job, including the status of the job. For more
* information, see Custom
* models in the Amazon Bedrock User Guide.
*
*
* @param getModelCustomizationJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetModelCustomizationJob operation returned by the service.
* @sample AmazonBedrockAsyncHandler.GetModelCustomizationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future getModelCustomizationJobAsync(GetModelCustomizationJobRequest getModelCustomizationJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get the current configuration values for model invocation logging.
*
*
* @param getModelInvocationLoggingConfigurationRequest
* @return A Java Future containing the result of the GetModelInvocationLoggingConfiguration operation returned by
* the service.
* @sample AmazonBedrockAsync.GetModelInvocationLoggingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getModelInvocationLoggingConfigurationAsync(
GetModelInvocationLoggingConfigurationRequest getModelInvocationLoggingConfigurationRequest);
/**
*
* Get the current configuration values for model invocation logging.
*
*
* @param getModelInvocationLoggingConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetModelInvocationLoggingConfiguration operation returned by
* the service.
* @sample AmazonBedrockAsyncHandler.GetModelInvocationLoggingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getModelInvocationLoggingConfigurationAsync(
GetModelInvocationLoggingConfigurationRequest getModelInvocationLoggingConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns details for a Provisioned Throughput. For more information, see Provisioned Throughput in
* the Amazon Bedrock User Guide.
*
*
* @param getProvisionedModelThroughputRequest
* @return A Java Future containing the result of the GetProvisionedModelThroughput operation returned by the
* service.
* @sample AmazonBedrockAsync.GetProvisionedModelThroughput
* @see AWS API Documentation
*/
java.util.concurrent.Future getProvisionedModelThroughputAsync(
GetProvisionedModelThroughputRequest getProvisionedModelThroughputRequest);
/**
*
* Returns details for a Provisioned Throughput. For more information, see Provisioned Throughput in
* the Amazon Bedrock User Guide.
*
*
* @param getProvisionedModelThroughputRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetProvisionedModelThroughput operation returned by the
* service.
* @sample AmazonBedrockAsyncHandler.GetProvisionedModelThroughput
* @see AWS API Documentation
*/
java.util.concurrent.Future getProvisionedModelThroughputAsync(
GetProvisionedModelThroughputRequest getProvisionedModelThroughputRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of the custom models that you have created with the CreateModelCustomizationJob
* operation.
*
*
* For more information, see Custom models in the Amazon
* Bedrock User Guide.
*
*
* @param listCustomModelsRequest
* @return A Java Future containing the result of the ListCustomModels operation returned by the service.
* @sample AmazonBedrockAsync.ListCustomModels
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listCustomModelsAsync(ListCustomModelsRequest listCustomModelsRequest);
/**
*
* Returns a list of the custom models that you have created with the CreateModelCustomizationJob
* operation.
*
*
* For more information, see Custom models in the Amazon
* Bedrock User Guide.
*
*
* @param listCustomModelsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListCustomModels operation returned by the service.
* @sample AmazonBedrockAsyncHandler.ListCustomModels
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listCustomModelsAsync(ListCustomModelsRequest listCustomModelsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists model evaluation jobs.
*
*
* @param listEvaluationJobsRequest
* @return A Java Future containing the result of the ListEvaluationJobs operation returned by the service.
* @sample AmazonBedrockAsync.ListEvaluationJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listEvaluationJobsAsync(ListEvaluationJobsRequest listEvaluationJobsRequest);
/**
*
* Lists model evaluation jobs.
*
*
* @param listEvaluationJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListEvaluationJobs operation returned by the service.
* @sample AmazonBedrockAsyncHandler.ListEvaluationJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listEvaluationJobsAsync(ListEvaluationJobsRequest listEvaluationJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists Amazon Bedrock foundation models that you can use. You can filter the results with the request parameters.
* For more information, see Foundation models in the
* Amazon Bedrock User Guide.
*
*
* @param listFoundationModelsRequest
* @return A Java Future containing the result of the ListFoundationModels operation returned by the service.
* @sample AmazonBedrockAsync.ListFoundationModels
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listFoundationModelsAsync(ListFoundationModelsRequest listFoundationModelsRequest);
/**
*
* Lists Amazon Bedrock foundation models that you can use. You can filter the results with the request parameters.
* For more information, see Foundation models in the
* Amazon Bedrock User Guide.
*
*
* @param listFoundationModelsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFoundationModels operation returned by the service.
* @sample AmazonBedrockAsyncHandler.ListFoundationModels
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listFoundationModelsAsync(ListFoundationModelsRequest listFoundationModelsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists details about all the guardrails in an account. To list the DRAFT
version of all your
* guardrails, don't specify the guardrailIdentifier
field. To list all versions of a guardrail,
* specify the ARN of the guardrail in the guardrailIdentifier
field.
*
*
* You can set the maximum number of results to return in a response in the maxResults
field. If there
* are more results than the number you set, the response returns a nextToken
that you can send in
* another ListGuardrails
request to see the next batch of results.
*
*
* @param listGuardrailsRequest
* @return A Java Future containing the result of the ListGuardrails operation returned by the service.
* @sample AmazonBedrockAsync.ListGuardrails
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listGuardrailsAsync(ListGuardrailsRequest listGuardrailsRequest);
/**
*
* Lists details about all the guardrails in an account. To list the DRAFT
version of all your
* guardrails, don't specify the guardrailIdentifier
field. To list all versions of a guardrail,
* specify the ARN of the guardrail in the guardrailIdentifier
field.
*
*
* You can set the maximum number of results to return in a response in the maxResults
field. If there
* are more results than the number you set, the response returns a nextToken
that you can send in
* another ListGuardrails
request to see the next batch of results.
*
*
* @param listGuardrailsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListGuardrails operation returned by the service.
* @sample AmazonBedrockAsyncHandler.ListGuardrails
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listGuardrailsAsync(ListGuardrailsRequest listGuardrailsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of model customization jobs that you have submitted. You can filter the jobs to return based on
* one or more criteria.
*
*
* For more information, see Custom models in the Amazon
* Bedrock User Guide.
*
*
* @param listModelCustomizationJobsRequest
* @return A Java Future containing the result of the ListModelCustomizationJobs operation returned by the service.
* @sample AmazonBedrockAsync.ListModelCustomizationJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listModelCustomizationJobsAsync(
ListModelCustomizationJobsRequest listModelCustomizationJobsRequest);
/**
*
* Returns a list of model customization jobs that you have submitted. You can filter the jobs to return based on
* one or more criteria.
*
*
* For more information, see Custom models in the Amazon
* Bedrock User Guide.
*
*
* @param listModelCustomizationJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListModelCustomizationJobs operation returned by the service.
* @sample AmazonBedrockAsyncHandler.ListModelCustomizationJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listModelCustomizationJobsAsync(
ListModelCustomizationJobsRequest listModelCustomizationJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the Provisioned Throughputs in the account. For more information, see Provisioned Throughput in
* the Amazon Bedrock User Guide.
*
*
* @param listProvisionedModelThroughputsRequest
* @return A Java Future containing the result of the ListProvisionedModelThroughputs operation returned by the
* service.
* @sample AmazonBedrockAsync.ListProvisionedModelThroughputs
* @see AWS API Documentation
*/
java.util.concurrent.Future listProvisionedModelThroughputsAsync(
ListProvisionedModelThroughputsRequest listProvisionedModelThroughputsRequest);
/**
*
* Lists the Provisioned Throughputs in the account. For more information, see Provisioned Throughput in
* the Amazon Bedrock User Guide.
*
*
* @param listProvisionedModelThroughputsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListProvisionedModelThroughputs operation returned by the
* service.
* @sample AmazonBedrockAsyncHandler.ListProvisionedModelThroughputs
* @see AWS API Documentation
*/
java.util.concurrent.Future listProvisionedModelThroughputsAsync(
ListProvisionedModelThroughputsRequest listProvisionedModelThroughputsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List the tags associated with the specified resource.
*
*
* For more information, see Tagging
* resources in the Amazon Bedrock User Guide.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonBedrockAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* List the tags associated with the specified resource.
*
*
* For more information, see Tagging
* resources in the Amazon Bedrock User Guide.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonBedrockAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Set the configuration values for model invocation logging.
*
*
* @param putModelInvocationLoggingConfigurationRequest
* @return A Java Future containing the result of the PutModelInvocationLoggingConfiguration operation returned by
* the service.
* @sample AmazonBedrockAsync.PutModelInvocationLoggingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future putModelInvocationLoggingConfigurationAsync(
PutModelInvocationLoggingConfigurationRequest putModelInvocationLoggingConfigurationRequest);
/**
*
* Set the configuration values for model invocation logging.
*
*
* @param putModelInvocationLoggingConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutModelInvocationLoggingConfiguration operation returned by
* the service.
* @sample AmazonBedrockAsyncHandler.PutModelInvocationLoggingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future putModelInvocationLoggingConfigurationAsync(
PutModelInvocationLoggingConfigurationRequest putModelInvocationLoggingConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops an in progress model evaluation job.
*
*
* @param stopEvaluationJobRequest
* @return A Java Future containing the result of the StopEvaluationJob operation returned by the service.
* @sample AmazonBedrockAsync.StopEvaluationJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopEvaluationJobAsync(StopEvaluationJobRequest stopEvaluationJobRequest);
/**
*
* Stops an in progress model evaluation job.
*
*
* @param stopEvaluationJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopEvaluationJob operation returned by the service.
* @sample AmazonBedrockAsyncHandler.StopEvaluationJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopEvaluationJobAsync(StopEvaluationJobRequest stopEvaluationJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops an active model customization job. For more information, see Custom models in the Amazon
* Bedrock User Guide.
*
*
* @param stopModelCustomizationJobRequest
* @return A Java Future containing the result of the StopModelCustomizationJob operation returned by the service.
* @sample AmazonBedrockAsync.StopModelCustomizationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future stopModelCustomizationJobAsync(
StopModelCustomizationJobRequest stopModelCustomizationJobRequest);
/**
*
* Stops an active model customization job. For more information, see Custom models in the Amazon
* Bedrock User Guide.
*
*
* @param stopModelCustomizationJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopModelCustomizationJob operation returned by the service.
* @sample AmazonBedrockAsyncHandler.StopModelCustomizationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future stopModelCustomizationJobAsync(
StopModelCustomizationJobRequest stopModelCustomizationJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associate tags with a resource. For more information, see Tagging resources in the Amazon
* Bedrock User Guide.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonBedrockAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Associate tags with a resource. For more information, see Tagging resources in the Amazon
* Bedrock User Guide.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonBedrockAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Remove one or more tags from a resource. For more information, see Tagging resources in the Amazon
* Bedrock User Guide.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonBedrockAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Remove one or more tags from a resource. For more information, see Tagging resources in the Amazon
* Bedrock User Guide.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonBedrockAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a guardrail with the values you specify.
*
*
* -
*
* Specify a name
and optional description
.
*
*
* -
*
* Specify messages for when the guardrail successfully blocks a prompt or a model response in the
* blockedInputMessaging
and blockedOutputsMessaging
fields.
*
*
* -
*
* Specify topics for the guardrail to deny in the topicPolicyConfig
object. Each GuardrailTopicConfig object in the topicsConfig
list pertains to one topic.
*
*
* -
*
* Give a name
and description
so that the guardrail can properly identify the topic.
*
*
* -
*
* Specify DENY
in the type
field.
*
*
* -
*
* (Optional) Provide up to five prompts that you would categorize as belonging to the topic in the
* examples
list.
*
*
*
*
* -
*
* Specify filter strengths for the harmful categories defined in Amazon Bedrock in the
* contentPolicyConfig
object. Each GuardrailContentFilterConfig object in the filtersConfig
list pertains to a harmful category.
* For more information, see Content filters. For
* more information about the fields in a content filter, see GuardrailContentFilterConfig.
*
*
* -
*
* Specify the category in the type
field.
*
*
* -
*
* Specify the strength of the filter for prompts in the inputStrength
field and for model responses in
* the strength
field of the GuardrailContentFilterConfig.
*
*
*
*
* -
*
* (Optional) For security, include the ARN of a KMS key in the kmsKeyId
field.
*
*
*
*
* @param updateGuardrailRequest
* @return A Java Future containing the result of the UpdateGuardrail operation returned by the service.
* @sample AmazonBedrockAsync.UpdateGuardrail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateGuardrailAsync(UpdateGuardrailRequest updateGuardrailRequest);
/**
*
* Updates a guardrail with the values you specify.
*
*
* -
*
* Specify a name
and optional description
.
*
*
* -
*
* Specify messages for when the guardrail successfully blocks a prompt or a model response in the
* blockedInputMessaging
and blockedOutputsMessaging
fields.
*
*
* -
*
* Specify topics for the guardrail to deny in the topicPolicyConfig
object. Each GuardrailTopicConfig object in the topicsConfig
list pertains to one topic.
*
*
* -
*
* Give a name
and description
so that the guardrail can properly identify the topic.
*
*
* -
*
* Specify DENY
in the type
field.
*
*
* -
*
* (Optional) Provide up to five prompts that you would categorize as belonging to the topic in the
* examples
list.
*
*
*
*
* -
*
* Specify filter strengths for the harmful categories defined in Amazon Bedrock in the
* contentPolicyConfig
object. Each GuardrailContentFilterConfig object in the filtersConfig
list pertains to a harmful category.
* For more information, see Content filters. For
* more information about the fields in a content filter, see GuardrailContentFilterConfig.
*
*
* -
*
* Specify the category in the type
field.
*
*
* -
*
* Specify the strength of the filter for prompts in the inputStrength
field and for model responses in
* the strength
field of the GuardrailContentFilterConfig.
*
*
*
*
* -
*
* (Optional) For security, include the ARN of a KMS key in the kmsKeyId
field.
*
*
*
*
* @param updateGuardrailRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateGuardrail operation returned by the service.
* @sample AmazonBedrockAsyncHandler.UpdateGuardrail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateGuardrailAsync(UpdateGuardrailRequest updateGuardrailRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the name or associated model for a Provisioned Throughput. For more information, see Provisioned Throughput in
* the Amazon Bedrock User Guide.
*
*
* @param updateProvisionedModelThroughputRequest
* @return A Java Future containing the result of the UpdateProvisionedModelThroughput operation returned by the
* service.
* @sample AmazonBedrockAsync.UpdateProvisionedModelThroughput
* @see AWS API Documentation
*/
java.util.concurrent.Future updateProvisionedModelThroughputAsync(
UpdateProvisionedModelThroughputRequest updateProvisionedModelThroughputRequest);
/**
*
* Updates the name or associated model for a Provisioned Throughput. For more information, see Provisioned Throughput in
* the Amazon Bedrock User Guide.
*
*
* @param updateProvisionedModelThroughputRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateProvisionedModelThroughput operation returned by the
* service.
* @sample AmazonBedrockAsyncHandler.UpdateProvisionedModelThroughput
* @see AWS API Documentation
*/
java.util.concurrent.Future updateProvisionedModelThroughputAsync(
UpdateProvisionedModelThroughputRequest updateProvisionedModelThroughputRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}