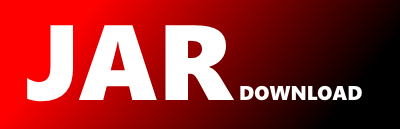
com.amazonaws.services.bedrock.model.CreateEvaluationJobRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-bedrock Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.bedrock.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateEvaluationJobRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the model evaluation job. Model evaluation job names must unique with your AWS account, and your
* account's AWS region.
*
*/
private String jobName;
/**
*
* A description of the model evaluation job.
*
*/
private String jobDescription;
/**
*
* A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If this token
* matches a previous request, Amazon Bedrock ignores the request, but does not return an error. For more
* information, see Ensuring
* idempotency.
*
*/
private String clientRequestToken;
/**
*
* The Amazon Resource Name (ARN) of an IAM service role that Amazon Bedrock can assume to perform tasks on your
* behalf. The service role must have Amazon Bedrock as the service principal, and provide access to any Amazon S3
* buckets specified in the EvaluationConfig
object. To pass this role to Amazon Bedrock, the caller of
* this API must have the iam:PassRole
permission. To learn more about the required permissions, see Required
* permissions.
*
*/
private String roleArn;
/**
*
* Specify your customer managed key ARN that will be used to encrypt your model evaluation job.
*
*/
private String customerEncryptionKeyId;
/**
*
* Tags to attach to the model evaluation job.
*
*/
private java.util.List jobTags;
/**
*
* Specifies whether the model evaluation job is automatic or uses human worker.
*
*/
private EvaluationConfig evaluationConfig;
/**
*
* Specify the models you want to use in your model evaluation job. Automatic model evaluation jobs support a single
* model, and model evaluation job that use human workers support two models.
*
*/
private EvaluationInferenceConfig inferenceConfig;
/**
*
* An object that defines where the results of model evaluation job will be saved in Amazon S3.
*
*/
private EvaluationOutputDataConfig outputDataConfig;
/**
*
* The name of the model evaluation job. Model evaluation job names must unique with your AWS account, and your
* account's AWS region.
*
*
* @param jobName
* The name of the model evaluation job. Model evaluation job names must unique with your AWS account, and
* your account's AWS region.
*/
public void setJobName(String jobName) {
this.jobName = jobName;
}
/**
*
* The name of the model evaluation job. Model evaluation job names must unique with your AWS account, and your
* account's AWS region.
*
*
* @return The name of the model evaluation job. Model evaluation job names must unique with your AWS account, and
* your account's AWS region.
*/
public String getJobName() {
return this.jobName;
}
/**
*
* The name of the model evaluation job. Model evaluation job names must unique with your AWS account, and your
* account's AWS region.
*
*
* @param jobName
* The name of the model evaluation job. Model evaluation job names must unique with your AWS account, and
* your account's AWS region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEvaluationJobRequest withJobName(String jobName) {
setJobName(jobName);
return this;
}
/**
*
* A description of the model evaluation job.
*
*
* @param jobDescription
* A description of the model evaluation job.
*/
public void setJobDescription(String jobDescription) {
this.jobDescription = jobDescription;
}
/**
*
* A description of the model evaluation job.
*
*
* @return A description of the model evaluation job.
*/
public String getJobDescription() {
return this.jobDescription;
}
/**
*
* A description of the model evaluation job.
*
*
* @param jobDescription
* A description of the model evaluation job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEvaluationJobRequest withJobDescription(String jobDescription) {
setJobDescription(jobDescription);
return this;
}
/**
*
* A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If this token
* matches a previous request, Amazon Bedrock ignores the request, but does not return an error. For more
* information, see Ensuring
* idempotency.
*
*
* @param clientRequestToken
* A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If
* this token matches a previous request, Amazon Bedrock ignores the request, but does not return an error.
* For more information, see Ensuring
* idempotency.
*/
public void setClientRequestToken(String clientRequestToken) {
this.clientRequestToken = clientRequestToken;
}
/**
*
* A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If this token
* matches a previous request, Amazon Bedrock ignores the request, but does not return an error. For more
* information, see Ensuring
* idempotency.
*
*
* @return A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If
* this token matches a previous request, Amazon Bedrock ignores the request, but does not return an error.
* For more information, see Ensuring
* idempotency.
*/
public String getClientRequestToken() {
return this.clientRequestToken;
}
/**
*
* A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If this token
* matches a previous request, Amazon Bedrock ignores the request, but does not return an error. For more
* information, see Ensuring
* idempotency.
*
*
* @param clientRequestToken
* A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If
* this token matches a previous request, Amazon Bedrock ignores the request, but does not return an error.
* For more information, see Ensuring
* idempotency.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEvaluationJobRequest withClientRequestToken(String clientRequestToken) {
setClientRequestToken(clientRequestToken);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM service role that Amazon Bedrock can assume to perform tasks on your
* behalf. The service role must have Amazon Bedrock as the service principal, and provide access to any Amazon S3
* buckets specified in the EvaluationConfig
object. To pass this role to Amazon Bedrock, the caller of
* this API must have the iam:PassRole
permission. To learn more about the required permissions, see Required
* permissions.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of an IAM service role that Amazon Bedrock can assume to perform tasks on
* your behalf. The service role must have Amazon Bedrock as the service principal, and provide access to any
* Amazon S3 buckets specified in the EvaluationConfig
object. To pass this role to Amazon
* Bedrock, the caller of this API must have the iam:PassRole
permission. To learn more about
* the required permissions, see Required
* permissions.
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM service role that Amazon Bedrock can assume to perform tasks on your
* behalf. The service role must have Amazon Bedrock as the service principal, and provide access to any Amazon S3
* buckets specified in the EvaluationConfig
object. To pass this role to Amazon Bedrock, the caller of
* this API must have the iam:PassRole
permission. To learn more about the required permissions, see Required
* permissions.
*
*
* @return The Amazon Resource Name (ARN) of an IAM service role that Amazon Bedrock can assume to perform tasks on
* your behalf. The service role must have Amazon Bedrock as the service principal, and provide access to
* any Amazon S3 buckets specified in the EvaluationConfig
object. To pass this role to Amazon
* Bedrock, the caller of this API must have the iam:PassRole
permission. To learn more about
* the required permissions, see Required
* permissions.
*/
public String getRoleArn() {
return this.roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM service role that Amazon Bedrock can assume to perform tasks on your
* behalf. The service role must have Amazon Bedrock as the service principal, and provide access to any Amazon S3
* buckets specified in the EvaluationConfig
object. To pass this role to Amazon Bedrock, the caller of
* this API must have the iam:PassRole
permission. To learn more about the required permissions, see Required
* permissions.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of an IAM service role that Amazon Bedrock can assume to perform tasks on
* your behalf. The service role must have Amazon Bedrock as the service principal, and provide access to any
* Amazon S3 buckets specified in the EvaluationConfig
object. To pass this role to Amazon
* Bedrock, the caller of this API must have the iam:PassRole
permission. To learn more about
* the required permissions, see Required
* permissions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEvaluationJobRequest withRoleArn(String roleArn) {
setRoleArn(roleArn);
return this;
}
/**
*
* Specify your customer managed key ARN that will be used to encrypt your model evaluation job.
*
*
* @param customerEncryptionKeyId
* Specify your customer managed key ARN that will be used to encrypt your model evaluation job.
*/
public void setCustomerEncryptionKeyId(String customerEncryptionKeyId) {
this.customerEncryptionKeyId = customerEncryptionKeyId;
}
/**
*
* Specify your customer managed key ARN that will be used to encrypt your model evaluation job.
*
*
* @return Specify your customer managed key ARN that will be used to encrypt your model evaluation job.
*/
public String getCustomerEncryptionKeyId() {
return this.customerEncryptionKeyId;
}
/**
*
* Specify your customer managed key ARN that will be used to encrypt your model evaluation job.
*
*
* @param customerEncryptionKeyId
* Specify your customer managed key ARN that will be used to encrypt your model evaluation job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEvaluationJobRequest withCustomerEncryptionKeyId(String customerEncryptionKeyId) {
setCustomerEncryptionKeyId(customerEncryptionKeyId);
return this;
}
/**
*
* Tags to attach to the model evaluation job.
*
*
* @return Tags to attach to the model evaluation job.
*/
public java.util.List getJobTags() {
return jobTags;
}
/**
*
* Tags to attach to the model evaluation job.
*
*
* @param jobTags
* Tags to attach to the model evaluation job.
*/
public void setJobTags(java.util.Collection jobTags) {
if (jobTags == null) {
this.jobTags = null;
return;
}
this.jobTags = new java.util.ArrayList(jobTags);
}
/**
*
* Tags to attach to the model evaluation job.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setJobTags(java.util.Collection)} or {@link #withJobTags(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param jobTags
* Tags to attach to the model evaluation job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEvaluationJobRequest withJobTags(Tag... jobTags) {
if (this.jobTags == null) {
setJobTags(new java.util.ArrayList(jobTags.length));
}
for (Tag ele : jobTags) {
this.jobTags.add(ele);
}
return this;
}
/**
*
* Tags to attach to the model evaluation job.
*
*
* @param jobTags
* Tags to attach to the model evaluation job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEvaluationJobRequest withJobTags(java.util.Collection jobTags) {
setJobTags(jobTags);
return this;
}
/**
*
* Specifies whether the model evaluation job is automatic or uses human worker.
*
*
* @param evaluationConfig
* Specifies whether the model evaluation job is automatic or uses human worker.
*/
public void setEvaluationConfig(EvaluationConfig evaluationConfig) {
this.evaluationConfig = evaluationConfig;
}
/**
*
* Specifies whether the model evaluation job is automatic or uses human worker.
*
*
* @return Specifies whether the model evaluation job is automatic or uses human worker.
*/
public EvaluationConfig getEvaluationConfig() {
return this.evaluationConfig;
}
/**
*
* Specifies whether the model evaluation job is automatic or uses human worker.
*
*
* @param evaluationConfig
* Specifies whether the model evaluation job is automatic or uses human worker.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEvaluationJobRequest withEvaluationConfig(EvaluationConfig evaluationConfig) {
setEvaluationConfig(evaluationConfig);
return this;
}
/**
*
* Specify the models you want to use in your model evaluation job. Automatic model evaluation jobs support a single
* model, and model evaluation job that use human workers support two models.
*
*
* @param inferenceConfig
* Specify the models you want to use in your model evaluation job. Automatic model evaluation jobs support a
* single model, and model evaluation job that use human workers support two models.
*/
public void setInferenceConfig(EvaluationInferenceConfig inferenceConfig) {
this.inferenceConfig = inferenceConfig;
}
/**
*
* Specify the models you want to use in your model evaluation job. Automatic model evaluation jobs support a single
* model, and model evaluation job that use human workers support two models.
*
*
* @return Specify the models you want to use in your model evaluation job. Automatic model evaluation jobs support
* a single model, and model evaluation job that use human workers support two models.
*/
public EvaluationInferenceConfig getInferenceConfig() {
return this.inferenceConfig;
}
/**
*
* Specify the models you want to use in your model evaluation job. Automatic model evaluation jobs support a single
* model, and model evaluation job that use human workers support two models.
*
*
* @param inferenceConfig
* Specify the models you want to use in your model evaluation job. Automatic model evaluation jobs support a
* single model, and model evaluation job that use human workers support two models.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEvaluationJobRequest withInferenceConfig(EvaluationInferenceConfig inferenceConfig) {
setInferenceConfig(inferenceConfig);
return this;
}
/**
*
* An object that defines where the results of model evaluation job will be saved in Amazon S3.
*
*
* @param outputDataConfig
* An object that defines where the results of model evaluation job will be saved in Amazon S3.
*/
public void setOutputDataConfig(EvaluationOutputDataConfig outputDataConfig) {
this.outputDataConfig = outputDataConfig;
}
/**
*
* An object that defines where the results of model evaluation job will be saved in Amazon S3.
*
*
* @return An object that defines where the results of model evaluation job will be saved in Amazon S3.
*/
public EvaluationOutputDataConfig getOutputDataConfig() {
return this.outputDataConfig;
}
/**
*
* An object that defines where the results of model evaluation job will be saved in Amazon S3.
*
*
* @param outputDataConfig
* An object that defines where the results of model evaluation job will be saved in Amazon S3.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEvaluationJobRequest withOutputDataConfig(EvaluationOutputDataConfig outputDataConfig) {
setOutputDataConfig(outputDataConfig);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getJobName() != null)
sb.append("JobName: ").append(getJobName()).append(",");
if (getJobDescription() != null)
sb.append("JobDescription: ").append("***Sensitive Data Redacted***").append(",");
if (getClientRequestToken() != null)
sb.append("ClientRequestToken: ").append(getClientRequestToken()).append(",");
if (getRoleArn() != null)
sb.append("RoleArn: ").append(getRoleArn()).append(",");
if (getCustomerEncryptionKeyId() != null)
sb.append("CustomerEncryptionKeyId: ").append(getCustomerEncryptionKeyId()).append(",");
if (getJobTags() != null)
sb.append("JobTags: ").append(getJobTags()).append(",");
if (getEvaluationConfig() != null)
sb.append("EvaluationConfig: ").append(getEvaluationConfig()).append(",");
if (getInferenceConfig() != null)
sb.append("InferenceConfig: ").append(getInferenceConfig()).append(",");
if (getOutputDataConfig() != null)
sb.append("OutputDataConfig: ").append(getOutputDataConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateEvaluationJobRequest == false)
return false;
CreateEvaluationJobRequest other = (CreateEvaluationJobRequest) obj;
if (other.getJobName() == null ^ this.getJobName() == null)
return false;
if (other.getJobName() != null && other.getJobName().equals(this.getJobName()) == false)
return false;
if (other.getJobDescription() == null ^ this.getJobDescription() == null)
return false;
if (other.getJobDescription() != null && other.getJobDescription().equals(this.getJobDescription()) == false)
return false;
if (other.getClientRequestToken() == null ^ this.getClientRequestToken() == null)
return false;
if (other.getClientRequestToken() != null && other.getClientRequestToken().equals(this.getClientRequestToken()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
if (other.getCustomerEncryptionKeyId() == null ^ this.getCustomerEncryptionKeyId() == null)
return false;
if (other.getCustomerEncryptionKeyId() != null && other.getCustomerEncryptionKeyId().equals(this.getCustomerEncryptionKeyId()) == false)
return false;
if (other.getJobTags() == null ^ this.getJobTags() == null)
return false;
if (other.getJobTags() != null && other.getJobTags().equals(this.getJobTags()) == false)
return false;
if (other.getEvaluationConfig() == null ^ this.getEvaluationConfig() == null)
return false;
if (other.getEvaluationConfig() != null && other.getEvaluationConfig().equals(this.getEvaluationConfig()) == false)
return false;
if (other.getInferenceConfig() == null ^ this.getInferenceConfig() == null)
return false;
if (other.getInferenceConfig() != null && other.getInferenceConfig().equals(this.getInferenceConfig()) == false)
return false;
if (other.getOutputDataConfig() == null ^ this.getOutputDataConfig() == null)
return false;
if (other.getOutputDataConfig() != null && other.getOutputDataConfig().equals(this.getOutputDataConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getJobName() == null) ? 0 : getJobName().hashCode());
hashCode = prime * hashCode + ((getJobDescription() == null) ? 0 : getJobDescription().hashCode());
hashCode = prime * hashCode + ((getClientRequestToken() == null) ? 0 : getClientRequestToken().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
hashCode = prime * hashCode + ((getCustomerEncryptionKeyId() == null) ? 0 : getCustomerEncryptionKeyId().hashCode());
hashCode = prime * hashCode + ((getJobTags() == null) ? 0 : getJobTags().hashCode());
hashCode = prime * hashCode + ((getEvaluationConfig() == null) ? 0 : getEvaluationConfig().hashCode());
hashCode = prime * hashCode + ((getInferenceConfig() == null) ? 0 : getInferenceConfig().hashCode());
hashCode = prime * hashCode + ((getOutputDataConfig() == null) ? 0 : getOutputDataConfig().hashCode());
return hashCode;
}
@Override
public CreateEvaluationJobRequest clone() {
return (CreateEvaluationJobRequest) super.clone();
}
}