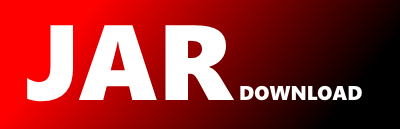
com.amazonaws.services.bedrock.model.FoundationModelDetails Maven / Gradle / Ivy
Show all versions of aws-java-sdk-bedrock Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.bedrock.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Information about a foundation model.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class FoundationModelDetails implements Serializable, Cloneable, StructuredPojo {
/**
*
* The model Amazon Resource Name (ARN).
*
*/
private String modelArn;
/**
*
* The model identifier.
*
*/
private String modelId;
/**
*
* The model name.
*
*/
private String modelName;
/**
*
* The model's provider name.
*
*/
private String providerName;
/**
*
* The input modalities that the model supports.
*
*/
private java.util.List inputModalities;
/**
*
* The output modalities that the model supports.
*
*/
private java.util.List outputModalities;
/**
*
* Indicates whether the model supports streaming.
*
*/
private Boolean responseStreamingSupported;
/**
*
* The customization that the model supports.
*
*/
private java.util.List customizationsSupported;
/**
*
* The inference types that the model supports.
*
*/
private java.util.List inferenceTypesSupported;
/**
*
* Contains details about whether a model version is available or deprecated
*
*/
private FoundationModelLifecycle modelLifecycle;
/**
*
* The model Amazon Resource Name (ARN).
*
*
* @param modelArn
* The model Amazon Resource Name (ARN).
*/
public void setModelArn(String modelArn) {
this.modelArn = modelArn;
}
/**
*
* The model Amazon Resource Name (ARN).
*
*
* @return The model Amazon Resource Name (ARN).
*/
public String getModelArn() {
return this.modelArn;
}
/**
*
* The model Amazon Resource Name (ARN).
*
*
* @param modelArn
* The model Amazon Resource Name (ARN).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FoundationModelDetails withModelArn(String modelArn) {
setModelArn(modelArn);
return this;
}
/**
*
* The model identifier.
*
*
* @param modelId
* The model identifier.
*/
public void setModelId(String modelId) {
this.modelId = modelId;
}
/**
*
* The model identifier.
*
*
* @return The model identifier.
*/
public String getModelId() {
return this.modelId;
}
/**
*
* The model identifier.
*
*
* @param modelId
* The model identifier.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FoundationModelDetails withModelId(String modelId) {
setModelId(modelId);
return this;
}
/**
*
* The model name.
*
*
* @param modelName
* The model name.
*/
public void setModelName(String modelName) {
this.modelName = modelName;
}
/**
*
* The model name.
*
*
* @return The model name.
*/
public String getModelName() {
return this.modelName;
}
/**
*
* The model name.
*
*
* @param modelName
* The model name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FoundationModelDetails withModelName(String modelName) {
setModelName(modelName);
return this;
}
/**
*
* The model's provider name.
*
*
* @param providerName
* The model's provider name.
*/
public void setProviderName(String providerName) {
this.providerName = providerName;
}
/**
*
* The model's provider name.
*
*
* @return The model's provider name.
*/
public String getProviderName() {
return this.providerName;
}
/**
*
* The model's provider name.
*
*
* @param providerName
* The model's provider name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FoundationModelDetails withProviderName(String providerName) {
setProviderName(providerName);
return this;
}
/**
*
* The input modalities that the model supports.
*
*
* @return The input modalities that the model supports.
* @see ModelModality
*/
public java.util.List getInputModalities() {
return inputModalities;
}
/**
*
* The input modalities that the model supports.
*
*
* @param inputModalities
* The input modalities that the model supports.
* @see ModelModality
*/
public void setInputModalities(java.util.Collection inputModalities) {
if (inputModalities == null) {
this.inputModalities = null;
return;
}
this.inputModalities = new java.util.ArrayList(inputModalities);
}
/**
*
* The input modalities that the model supports.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setInputModalities(java.util.Collection)} or {@link #withInputModalities(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param inputModalities
* The input modalities that the model supports.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelModality
*/
public FoundationModelDetails withInputModalities(String... inputModalities) {
if (this.inputModalities == null) {
setInputModalities(new java.util.ArrayList(inputModalities.length));
}
for (String ele : inputModalities) {
this.inputModalities.add(ele);
}
return this;
}
/**
*
* The input modalities that the model supports.
*
*
* @param inputModalities
* The input modalities that the model supports.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelModality
*/
public FoundationModelDetails withInputModalities(java.util.Collection inputModalities) {
setInputModalities(inputModalities);
return this;
}
/**
*
* The input modalities that the model supports.
*
*
* @param inputModalities
* The input modalities that the model supports.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelModality
*/
public FoundationModelDetails withInputModalities(ModelModality... inputModalities) {
java.util.ArrayList inputModalitiesCopy = new java.util.ArrayList(inputModalities.length);
for (ModelModality value : inputModalities) {
inputModalitiesCopy.add(value.toString());
}
if (getInputModalities() == null) {
setInputModalities(inputModalitiesCopy);
} else {
getInputModalities().addAll(inputModalitiesCopy);
}
return this;
}
/**
*
* The output modalities that the model supports.
*
*
* @return The output modalities that the model supports.
* @see ModelModality
*/
public java.util.List getOutputModalities() {
return outputModalities;
}
/**
*
* The output modalities that the model supports.
*
*
* @param outputModalities
* The output modalities that the model supports.
* @see ModelModality
*/
public void setOutputModalities(java.util.Collection outputModalities) {
if (outputModalities == null) {
this.outputModalities = null;
return;
}
this.outputModalities = new java.util.ArrayList(outputModalities);
}
/**
*
* The output modalities that the model supports.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setOutputModalities(java.util.Collection)} or {@link #withOutputModalities(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param outputModalities
* The output modalities that the model supports.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelModality
*/
public FoundationModelDetails withOutputModalities(String... outputModalities) {
if (this.outputModalities == null) {
setOutputModalities(new java.util.ArrayList(outputModalities.length));
}
for (String ele : outputModalities) {
this.outputModalities.add(ele);
}
return this;
}
/**
*
* The output modalities that the model supports.
*
*
* @param outputModalities
* The output modalities that the model supports.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelModality
*/
public FoundationModelDetails withOutputModalities(java.util.Collection outputModalities) {
setOutputModalities(outputModalities);
return this;
}
/**
*
* The output modalities that the model supports.
*
*
* @param outputModalities
* The output modalities that the model supports.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelModality
*/
public FoundationModelDetails withOutputModalities(ModelModality... outputModalities) {
java.util.ArrayList outputModalitiesCopy = new java.util.ArrayList(outputModalities.length);
for (ModelModality value : outputModalities) {
outputModalitiesCopy.add(value.toString());
}
if (getOutputModalities() == null) {
setOutputModalities(outputModalitiesCopy);
} else {
getOutputModalities().addAll(outputModalitiesCopy);
}
return this;
}
/**
*
* Indicates whether the model supports streaming.
*
*
* @param responseStreamingSupported
* Indicates whether the model supports streaming.
*/
public void setResponseStreamingSupported(Boolean responseStreamingSupported) {
this.responseStreamingSupported = responseStreamingSupported;
}
/**
*
* Indicates whether the model supports streaming.
*
*
* @return Indicates whether the model supports streaming.
*/
public Boolean getResponseStreamingSupported() {
return this.responseStreamingSupported;
}
/**
*
* Indicates whether the model supports streaming.
*
*
* @param responseStreamingSupported
* Indicates whether the model supports streaming.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FoundationModelDetails withResponseStreamingSupported(Boolean responseStreamingSupported) {
setResponseStreamingSupported(responseStreamingSupported);
return this;
}
/**
*
* Indicates whether the model supports streaming.
*
*
* @return Indicates whether the model supports streaming.
*/
public Boolean isResponseStreamingSupported() {
return this.responseStreamingSupported;
}
/**
*
* The customization that the model supports.
*
*
* @return The customization that the model supports.
* @see ModelCustomization
*/
public java.util.List getCustomizationsSupported() {
return customizationsSupported;
}
/**
*
* The customization that the model supports.
*
*
* @param customizationsSupported
* The customization that the model supports.
* @see ModelCustomization
*/
public void setCustomizationsSupported(java.util.Collection customizationsSupported) {
if (customizationsSupported == null) {
this.customizationsSupported = null;
return;
}
this.customizationsSupported = new java.util.ArrayList(customizationsSupported);
}
/**
*
* The customization that the model supports.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCustomizationsSupported(java.util.Collection)} or
* {@link #withCustomizationsSupported(java.util.Collection)} if you want to override the existing values.
*
*
* @param customizationsSupported
* The customization that the model supports.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelCustomization
*/
public FoundationModelDetails withCustomizationsSupported(String... customizationsSupported) {
if (this.customizationsSupported == null) {
setCustomizationsSupported(new java.util.ArrayList(customizationsSupported.length));
}
for (String ele : customizationsSupported) {
this.customizationsSupported.add(ele);
}
return this;
}
/**
*
* The customization that the model supports.
*
*
* @param customizationsSupported
* The customization that the model supports.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelCustomization
*/
public FoundationModelDetails withCustomizationsSupported(java.util.Collection customizationsSupported) {
setCustomizationsSupported(customizationsSupported);
return this;
}
/**
*
* The customization that the model supports.
*
*
* @param customizationsSupported
* The customization that the model supports.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelCustomization
*/
public FoundationModelDetails withCustomizationsSupported(ModelCustomization... customizationsSupported) {
java.util.ArrayList customizationsSupportedCopy = new java.util.ArrayList(customizationsSupported.length);
for (ModelCustomization value : customizationsSupported) {
customizationsSupportedCopy.add(value.toString());
}
if (getCustomizationsSupported() == null) {
setCustomizationsSupported(customizationsSupportedCopy);
} else {
getCustomizationsSupported().addAll(customizationsSupportedCopy);
}
return this;
}
/**
*
* The inference types that the model supports.
*
*
* @return The inference types that the model supports.
* @see InferenceType
*/
public java.util.List getInferenceTypesSupported() {
return inferenceTypesSupported;
}
/**
*
* The inference types that the model supports.
*
*
* @param inferenceTypesSupported
* The inference types that the model supports.
* @see InferenceType
*/
public void setInferenceTypesSupported(java.util.Collection inferenceTypesSupported) {
if (inferenceTypesSupported == null) {
this.inferenceTypesSupported = null;
return;
}
this.inferenceTypesSupported = new java.util.ArrayList(inferenceTypesSupported);
}
/**
*
* The inference types that the model supports.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setInferenceTypesSupported(java.util.Collection)} or
* {@link #withInferenceTypesSupported(java.util.Collection)} if you want to override the existing values.
*
*
* @param inferenceTypesSupported
* The inference types that the model supports.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InferenceType
*/
public FoundationModelDetails withInferenceTypesSupported(String... inferenceTypesSupported) {
if (this.inferenceTypesSupported == null) {
setInferenceTypesSupported(new java.util.ArrayList(inferenceTypesSupported.length));
}
for (String ele : inferenceTypesSupported) {
this.inferenceTypesSupported.add(ele);
}
return this;
}
/**
*
* The inference types that the model supports.
*
*
* @param inferenceTypesSupported
* The inference types that the model supports.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InferenceType
*/
public FoundationModelDetails withInferenceTypesSupported(java.util.Collection inferenceTypesSupported) {
setInferenceTypesSupported(inferenceTypesSupported);
return this;
}
/**
*
* The inference types that the model supports.
*
*
* @param inferenceTypesSupported
* The inference types that the model supports.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InferenceType
*/
public FoundationModelDetails withInferenceTypesSupported(InferenceType... inferenceTypesSupported) {
java.util.ArrayList inferenceTypesSupportedCopy = new java.util.ArrayList(inferenceTypesSupported.length);
for (InferenceType value : inferenceTypesSupported) {
inferenceTypesSupportedCopy.add(value.toString());
}
if (getInferenceTypesSupported() == null) {
setInferenceTypesSupported(inferenceTypesSupportedCopy);
} else {
getInferenceTypesSupported().addAll(inferenceTypesSupportedCopy);
}
return this;
}
/**
*
* Contains details about whether a model version is available or deprecated
*
*
* @param modelLifecycle
* Contains details about whether a model version is available or deprecated
*/
public void setModelLifecycle(FoundationModelLifecycle modelLifecycle) {
this.modelLifecycle = modelLifecycle;
}
/**
*
* Contains details about whether a model version is available or deprecated
*
*
* @return Contains details about whether a model version is available or deprecated
*/
public FoundationModelLifecycle getModelLifecycle() {
return this.modelLifecycle;
}
/**
*
* Contains details about whether a model version is available or deprecated
*
*
* @param modelLifecycle
* Contains details about whether a model version is available or deprecated
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FoundationModelDetails withModelLifecycle(FoundationModelLifecycle modelLifecycle) {
setModelLifecycle(modelLifecycle);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getModelArn() != null)
sb.append("ModelArn: ").append(getModelArn()).append(",");
if (getModelId() != null)
sb.append("ModelId: ").append(getModelId()).append(",");
if (getModelName() != null)
sb.append("ModelName: ").append(getModelName()).append(",");
if (getProviderName() != null)
sb.append("ProviderName: ").append(getProviderName()).append(",");
if (getInputModalities() != null)
sb.append("InputModalities: ").append(getInputModalities()).append(",");
if (getOutputModalities() != null)
sb.append("OutputModalities: ").append(getOutputModalities()).append(",");
if (getResponseStreamingSupported() != null)
sb.append("ResponseStreamingSupported: ").append(getResponseStreamingSupported()).append(",");
if (getCustomizationsSupported() != null)
sb.append("CustomizationsSupported: ").append(getCustomizationsSupported()).append(",");
if (getInferenceTypesSupported() != null)
sb.append("InferenceTypesSupported: ").append(getInferenceTypesSupported()).append(",");
if (getModelLifecycle() != null)
sb.append("ModelLifecycle: ").append(getModelLifecycle());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof FoundationModelDetails == false)
return false;
FoundationModelDetails other = (FoundationModelDetails) obj;
if (other.getModelArn() == null ^ this.getModelArn() == null)
return false;
if (other.getModelArn() != null && other.getModelArn().equals(this.getModelArn()) == false)
return false;
if (other.getModelId() == null ^ this.getModelId() == null)
return false;
if (other.getModelId() != null && other.getModelId().equals(this.getModelId()) == false)
return false;
if (other.getModelName() == null ^ this.getModelName() == null)
return false;
if (other.getModelName() != null && other.getModelName().equals(this.getModelName()) == false)
return false;
if (other.getProviderName() == null ^ this.getProviderName() == null)
return false;
if (other.getProviderName() != null && other.getProviderName().equals(this.getProviderName()) == false)
return false;
if (other.getInputModalities() == null ^ this.getInputModalities() == null)
return false;
if (other.getInputModalities() != null && other.getInputModalities().equals(this.getInputModalities()) == false)
return false;
if (other.getOutputModalities() == null ^ this.getOutputModalities() == null)
return false;
if (other.getOutputModalities() != null && other.getOutputModalities().equals(this.getOutputModalities()) == false)
return false;
if (other.getResponseStreamingSupported() == null ^ this.getResponseStreamingSupported() == null)
return false;
if (other.getResponseStreamingSupported() != null && other.getResponseStreamingSupported().equals(this.getResponseStreamingSupported()) == false)
return false;
if (other.getCustomizationsSupported() == null ^ this.getCustomizationsSupported() == null)
return false;
if (other.getCustomizationsSupported() != null && other.getCustomizationsSupported().equals(this.getCustomizationsSupported()) == false)
return false;
if (other.getInferenceTypesSupported() == null ^ this.getInferenceTypesSupported() == null)
return false;
if (other.getInferenceTypesSupported() != null && other.getInferenceTypesSupported().equals(this.getInferenceTypesSupported()) == false)
return false;
if (other.getModelLifecycle() == null ^ this.getModelLifecycle() == null)
return false;
if (other.getModelLifecycle() != null && other.getModelLifecycle().equals(this.getModelLifecycle()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getModelArn() == null) ? 0 : getModelArn().hashCode());
hashCode = prime * hashCode + ((getModelId() == null) ? 0 : getModelId().hashCode());
hashCode = prime * hashCode + ((getModelName() == null) ? 0 : getModelName().hashCode());
hashCode = prime * hashCode + ((getProviderName() == null) ? 0 : getProviderName().hashCode());
hashCode = prime * hashCode + ((getInputModalities() == null) ? 0 : getInputModalities().hashCode());
hashCode = prime * hashCode + ((getOutputModalities() == null) ? 0 : getOutputModalities().hashCode());
hashCode = prime * hashCode + ((getResponseStreamingSupported() == null) ? 0 : getResponseStreamingSupported().hashCode());
hashCode = prime * hashCode + ((getCustomizationsSupported() == null) ? 0 : getCustomizationsSupported().hashCode());
hashCode = prime * hashCode + ((getInferenceTypesSupported() == null) ? 0 : getInferenceTypesSupported().hashCode());
hashCode = prime * hashCode + ((getModelLifecycle() == null) ? 0 : getModelLifecycle().hashCode());
return hashCode;
}
@Override
public FoundationModelDetails clone() {
try {
return (FoundationModelDetails) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.bedrock.model.transform.FoundationModelDetailsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}